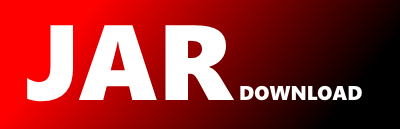
com.zuora.model.CreateCreditMemoFromChargeRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.CreditMemoItemFromChargeDetail;
import com.zuora.model.CustomRates;
import java.io.IOException;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* CreateCreditMemoFromChargeRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreateCreditMemoFromChargeRequest {
public static final String SERIALIZED_NAME_ACCOUNT_ID = "accountId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "accountNumber";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_AUTO_POST = "autoPost";
@SerializedName(SERIALIZED_NAME_AUTO_POST)
private Boolean autoPost = false;
public static final String SERIALIZED_NAME_CHARGES = "charges";
@SerializedName(SERIALIZED_NAME_CHARGES)
private List charges;
public static final String SERIALIZED_NAME_COMMENT = "comment";
@SerializedName(SERIALIZED_NAME_COMMENT)
private String comment;
public static final String SERIALIZED_NAME_CUSTOM_RATES = "customRates";
@SerializedName(SERIALIZED_NAME_CUSTOM_RATES)
private List customRates;
public static final String SERIALIZED_NAME_EFFECTIVE_DATE = "effectiveDate";
@SerializedName(SERIALIZED_NAME_EFFECTIVE_DATE)
private LocalDate effectiveDate;
public static final String SERIALIZED_NAME_EXCLUDE_FROM_AUTO_APPLY_RULES = "excludeFromAutoApplyRules";
@SerializedName(SERIALIZED_NAME_EXCLUDE_FROM_AUTO_APPLY_RULES)
private Boolean excludeFromAutoApplyRules = false;
public static final String SERIALIZED_NAME_REASON_CODE = "reasonCode";
@SerializedName(SERIALIZED_NAME_REASON_CODE)
private String reasonCode;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_NUMBER = "number";
@SerializedName(SERIALIZED_NAME_NUMBER)
private String number;
public static final String SERIALIZED_NAME_INTEGRATION_ID_N_S = "IntegrationId__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_ID_N_S)
private String integrationIdNS;
public static final String SERIALIZED_NAME_INTEGRATION_STATUS_N_S = "IntegrationStatus__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_STATUS_N_S)
private String integrationStatusNS;
public static final String SERIALIZED_NAME_ORIGIN_N_S = "Origin__NS";
@SerializedName(SERIALIZED_NAME_ORIGIN_N_S)
private String originNS;
public static final String SERIALIZED_NAME_SYNC_DATE_N_S = "SyncDate__NS";
@SerializedName(SERIALIZED_NAME_SYNC_DATE_N_S)
private String syncDateNS;
public static final String SERIALIZED_NAME_TRANSACTION_N_S = "Transaction__NS";
@SerializedName(SERIALIZED_NAME_TRANSACTION_N_S)
private String transactionNS;
public CreateCreditMemoFromChargeRequest() {
}
public CreateCreditMemoFromChargeRequest accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* The ID of the account associated with the credit memo. **Note**: When creating credit memos from product rate plan charges, you must specify `accountNumber`, `accountId`, or both in the request body. If both fields are specified, they must correspond to the same account.
* @return accountId
*/
@javax.annotation.Nullable
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public CreateCreditMemoFromChargeRequest accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* The number of the customer account associated with the credit memo. **Note**: When creating credit memos from product rate plan charges, you must specify `accountNumber`, `accountId`, or both in the request body. If both fields are specified, they must correspond to the same account.
* @return accountNumber
*/
@javax.annotation.Nullable
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public CreateCreditMemoFromChargeRequest autoPost(Boolean autoPost) {
this.autoPost = autoPost;
return this;
}
/**
* Whether to automatically post the credit memo after it is created. Setting this field to `true`, you do not need to separately call the [Post a credit memo](https://www.zuora.com/developer/api-references/api/operation/Put_PostCreditMemo) operation to post the credit memo.
* @return autoPost
*/
@javax.annotation.Nullable
public Boolean getAutoPost() {
return autoPost;
}
public void setAutoPost(Boolean autoPost) {
this.autoPost = autoPost;
}
public CreateCreditMemoFromChargeRequest charges(List charges) {
this.charges = charges;
return this;
}
public CreateCreditMemoFromChargeRequest addChargesItem(CreditMemoItemFromChargeDetail chargesItem) {
if (this.charges == null) {
this.charges = new ArrayList<>();
}
this.charges.add(chargesItem);
return this;
}
/**
* Container for product rate plan charges. The maximum number of items is 1,000.
* @return charges
*/
@javax.annotation.Nullable
public List getCharges() {
return charges;
}
public void setCharges(List charges) {
this.charges = charges;
}
public CreateCreditMemoFromChargeRequest comment(String comment) {
this.comment = comment;
return this;
}
/**
* Comments about the credit memo.
* @return comment
*/
@javax.annotation.Nullable
public String getComment() {
return comment;
}
public void setComment(String comment) {
this.comment = comment;
}
public CreateCreditMemoFromChargeRequest customRates(List customRates) {
this.customRates = customRates;
return this;
}
public CreateCreditMemoFromChargeRequest addCustomRatesItem(CustomRates customRatesItem) {
if (this.customRates == null) {
this.customRates = new ArrayList<>();
}
this.customRates.add(customRatesItem);
return this;
}
/**
* It contains Home currency and Reporting currency custom rates currencies. The maximum number of items is 2 (you can pass the Home currency item or Reporting currency item or both). **Note**: The API custom rate feature is permission controlled.
* @return customRates
*/
@javax.annotation.Nullable
public List getCustomRates() {
return customRates;
}
public void setCustomRates(List customRates) {
this.customRates = customRates;
}
public CreateCreditMemoFromChargeRequest effectiveDate(LocalDate effectiveDate) {
this.effectiveDate = effectiveDate;
return this;
}
/**
* The date when the credit memo takes effect.
* @return effectiveDate
*/
@javax.annotation.Nullable
public LocalDate getEffectiveDate() {
return effectiveDate;
}
public void setEffectiveDate(LocalDate effectiveDate) {
this.effectiveDate = effectiveDate;
}
public CreateCreditMemoFromChargeRequest excludeFromAutoApplyRules(Boolean excludeFromAutoApplyRules) {
this.excludeFromAutoApplyRules = excludeFromAutoApplyRules;
return this;
}
/**
* Whether the credit memo is excluded from the rule of automatically applying unapplied credit memos to invoices and debit memos during payment runs. If you set this field to `true`, a payment run does not pick up this credit memo or apply it to other invoices or debit memos.
* @return excludeFromAutoApplyRules
*/
@javax.annotation.Nullable
public Boolean getExcludeFromAutoApplyRules() {
return excludeFromAutoApplyRules;
}
public void setExcludeFromAutoApplyRules(Boolean excludeFromAutoApplyRules) {
this.excludeFromAutoApplyRules = excludeFromAutoApplyRules;
}
public CreateCreditMemoFromChargeRequest reasonCode(String reasonCode) {
this.reasonCode = reasonCode;
return this;
}
/**
* A code identifying the reason for the transaction. The value must be an existing reason code or empty. If you do not specify a value, Zuora uses the default reason code.
* @return reasonCode
*/
@javax.annotation.Nullable
public String getReasonCode() {
return reasonCode;
}
public void setReasonCode(String reasonCode) {
this.reasonCode = reasonCode;
}
public CreateCreditMemoFromChargeRequest currency(String currency) {
this.currency = currency;
return this;
}
/**
* The code of a currency as defined in Billing Settings through the Zuora UI. If you do not specify a currency during credit memo creation, the default account currency is applied. The currency that you specify in the request must be configured and activated in Billing Settings. **Note**: This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_customers_at_subscription_level/Multiple_Currencies\" target=\"_blank\">Multiple Currencies</a> feature in the **Early Adopter** phase enabled.
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public CreateCreditMemoFromChargeRequest number(String number) {
this.number = number;
return this;
}
/**
* A customized memo number with the following format requirements: - Max length: 32 - Acceptable characters: a-z,A-Z,0-9,-,_, The value must be unique in the system, otherwise it may cause issues with bill runs and subscribe/amend. If it is not provided, memo number will be auto-generated.
* @return number
*/
@javax.annotation.Nullable
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public CreateCreditMemoFromChargeRequest integrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
return this;
}
/**
* ID of the corresponding object in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationIdNS
*/
@javax.annotation.Nullable
public String getIntegrationIdNS() {
return integrationIdNS;
}
public void setIntegrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
}
public CreateCreditMemoFromChargeRequest integrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
return this;
}
/**
* Status of the credit memo's synchronization with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationStatusNS
*/
@javax.annotation.Nullable
public String getIntegrationStatusNS() {
return integrationStatusNS;
}
public void setIntegrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
}
public CreateCreditMemoFromChargeRequest originNS(String originNS) {
this.originNS = originNS;
return this;
}
/**
* Origin of the corresponding object in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return originNS
*/
@javax.annotation.Nullable
public String getOriginNS() {
return originNS;
}
public void setOriginNS(String originNS) {
this.originNS = originNS;
}
public CreateCreditMemoFromChargeRequest syncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
return this;
}
/**
* Date when the credit memo was synchronized with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return syncDateNS
*/
@javax.annotation.Nullable
public String getSyncDateNS() {
return syncDateNS;
}
public void setSyncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
}
public CreateCreditMemoFromChargeRequest transactionNS(String transactionNS) {
this.transactionNS = transactionNS;
return this;
}
/**
* Related transaction in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return transactionNS
*/
@javax.annotation.Nullable
public String getTransactionNS() {
return transactionNS;
}
public void setTransactionNS(String transactionNS) {
this.transactionNS = transactionNS;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreateCreditMemoFromChargeRequest instance itself
*/
public CreateCreditMemoFromChargeRequest putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateCreditMemoFromChargeRequest createCreditMemoFromChargeRequest = (CreateCreditMemoFromChargeRequest) o;
return Objects.equals(this.accountId, createCreditMemoFromChargeRequest.accountId) &&
Objects.equals(this.accountNumber, createCreditMemoFromChargeRequest.accountNumber) &&
Objects.equals(this.autoPost, createCreditMemoFromChargeRequest.autoPost) &&
Objects.equals(this.charges, createCreditMemoFromChargeRequest.charges) &&
Objects.equals(this.comment, createCreditMemoFromChargeRequest.comment) &&
Objects.equals(this.customRates, createCreditMemoFromChargeRequest.customRates) &&
Objects.equals(this.effectiveDate, createCreditMemoFromChargeRequest.effectiveDate) &&
Objects.equals(this.excludeFromAutoApplyRules, createCreditMemoFromChargeRequest.excludeFromAutoApplyRules) &&
Objects.equals(this.reasonCode, createCreditMemoFromChargeRequest.reasonCode) &&
Objects.equals(this.currency, createCreditMemoFromChargeRequest.currency) &&
Objects.equals(this.number, createCreditMemoFromChargeRequest.number) &&
Objects.equals(this.integrationIdNS, createCreditMemoFromChargeRequest.integrationIdNS) &&
Objects.equals(this.integrationStatusNS, createCreditMemoFromChargeRequest.integrationStatusNS) &&
Objects.equals(this.originNS, createCreditMemoFromChargeRequest.originNS) &&
Objects.equals(this.syncDateNS, createCreditMemoFromChargeRequest.syncDateNS) &&
Objects.equals(this.transactionNS, createCreditMemoFromChargeRequest.transactionNS)&&
Objects.equals(this.additionalProperties, createCreditMemoFromChargeRequest.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(accountId, accountNumber, autoPost, charges, comment, customRates, effectiveDate, excludeFromAutoApplyRules, reasonCode, currency, number, integrationIdNS, integrationStatusNS, originNS, syncDateNS, transactionNS, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateCreditMemoFromChargeRequest {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" autoPost: ").append(toIndentedString(autoPost)).append("\n");
sb.append(" charges: ").append(toIndentedString(charges)).append("\n");
sb.append(" comment: ").append(toIndentedString(comment)).append("\n");
sb.append(" customRates: ").append(toIndentedString(customRates)).append("\n");
sb.append(" effectiveDate: ").append(toIndentedString(effectiveDate)).append("\n");
sb.append(" excludeFromAutoApplyRules: ").append(toIndentedString(excludeFromAutoApplyRules)).append("\n");
sb.append(" reasonCode: ").append(toIndentedString(reasonCode)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" number: ").append(toIndentedString(number)).append("\n");
sb.append(" integrationIdNS: ").append(toIndentedString(integrationIdNS)).append("\n");
sb.append(" integrationStatusNS: ").append(toIndentedString(integrationStatusNS)).append("\n");
sb.append(" originNS: ").append(toIndentedString(originNS)).append("\n");
sb.append(" syncDateNS: ").append(toIndentedString(syncDateNS)).append("\n");
sb.append(" transactionNS: ").append(toIndentedString(transactionNS)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("accountId");
openapiFields.add("accountNumber");
openapiFields.add("autoPost");
openapiFields.add("charges");
openapiFields.add("comment");
openapiFields.add("customRates");
openapiFields.add("effectiveDate");
openapiFields.add("excludeFromAutoApplyRules");
openapiFields.add("reasonCode");
openapiFields.add("currency");
openapiFields.add("number");
openapiFields.add("IntegrationId__NS");
openapiFields.add("IntegrationStatus__NS");
openapiFields.add("Origin__NS");
openapiFields.add("SyncDate__NS");
openapiFields.add("Transaction__NS");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreateCreditMemoFromChargeRequest
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreateCreditMemoFromChargeRequest.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreateCreditMemoFromChargeRequest is not found in the empty JSON string", CreateCreditMemoFromChargeRequest.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("accountId") != null && !jsonObj.get("accountId").isJsonNull()) && !jsonObj.get("accountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountId").toString()));
}
if ((jsonObj.get("accountNumber") != null && !jsonObj.get("accountNumber").isJsonNull()) && !jsonObj.get("accountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountNumber").toString()));
}
if (jsonObj.get("charges") != null && !jsonObj.get("charges").isJsonNull()) {
JsonArray jsonArraycharges = jsonObj.getAsJsonArray("charges");
if (jsonArraycharges != null) {
// ensure the json data is an array
if (!jsonObj.get("charges").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `charges` to be an array in the JSON string but got `%s`", jsonObj.get("charges").toString()));
}
// validate the optional field `charges` (array)
for (int i = 0; i < jsonArraycharges.size(); i++) {
CreditMemoItemFromChargeDetail.validateJsonElement(jsonArraycharges.get(i));
};
}
}
if ((jsonObj.get("comment") != null && !jsonObj.get("comment").isJsonNull()) && !jsonObj.get("comment").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `comment` to be a primitive type in the JSON string but got `%s`", jsonObj.get("comment").toString()));
}
if (jsonObj.get("customRates") != null && !jsonObj.get("customRates").isJsonNull()) {
JsonArray jsonArraycustomRates = jsonObj.getAsJsonArray("customRates");
if (jsonArraycustomRates != null) {
// ensure the json data is an array
if (!jsonObj.get("customRates").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `customRates` to be an array in the JSON string but got `%s`", jsonObj.get("customRates").toString()));
}
// validate the optional field `customRates` (array)
for (int i = 0; i < jsonArraycustomRates.size(); i++) {
CustomRates.validateJsonElement(jsonArraycustomRates.get(i));
};
}
}
if ((jsonObj.get("reasonCode") != null && !jsonObj.get("reasonCode").isJsonNull()) && !jsonObj.get("reasonCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `reasonCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("reasonCode").toString()));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("number") != null && !jsonObj.get("number").isJsonNull()) && !jsonObj.get("number").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `number` to be a primitive type in the JSON string but got `%s`", jsonObj.get("number").toString()));
}
if ((jsonObj.get("IntegrationId__NS") != null && !jsonObj.get("IntegrationId__NS").isJsonNull()) && !jsonObj.get("IntegrationId__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationId__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationId__NS").toString()));
}
if ((jsonObj.get("IntegrationStatus__NS") != null && !jsonObj.get("IntegrationStatus__NS").isJsonNull()) && !jsonObj.get("IntegrationStatus__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationStatus__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationStatus__NS").toString()));
}
if ((jsonObj.get("Origin__NS") != null && !jsonObj.get("Origin__NS").isJsonNull()) && !jsonObj.get("Origin__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Origin__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Origin__NS").toString()));
}
if ((jsonObj.get("SyncDate__NS") != null && !jsonObj.get("SyncDate__NS").isJsonNull()) && !jsonObj.get("SyncDate__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SyncDate__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SyncDate__NS").toString()));
}
if ((jsonObj.get("Transaction__NS") != null && !jsonObj.get("Transaction__NS").isJsonNull()) && !jsonObj.get("Transaction__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Transaction__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Transaction__NS").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreateCreditMemoFromChargeRequest.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreateCreditMemoFromChargeRequest' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreateCreditMemoFromChargeRequest.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreateCreditMemoFromChargeRequest value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreateCreditMemoFromChargeRequest read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreateCreditMemoFromChargeRequest instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreateCreditMemoFromChargeRequest given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreateCreditMemoFromChargeRequest
* @throws IOException if the JSON string is invalid with respect to CreateCreditMemoFromChargeRequest
*/
public static CreateCreditMemoFromChargeRequest fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreateCreditMemoFromChargeRequest.class);
}
/**
* Convert an instance of CreateCreditMemoFromChargeRequest to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy