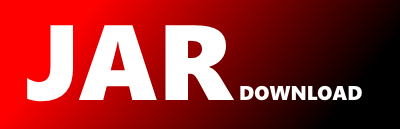
com.zuora.model.CreateInvoiceCollectRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.time.LocalDate;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* CreateInvoiceCollectRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreateInvoiceCollectRequest {
public static final String SERIALIZED_NAME_ACCOUNT_KEY = "accountKey";
@SerializedName(SERIALIZED_NAME_ACCOUNT_KEY)
private String accountKey;
public static final String SERIALIZED_NAME_DOCUMENT_DATE = "documentDate";
@SerializedName(SERIALIZED_NAME_DOCUMENT_DATE)
private LocalDate documentDate;
public static final String SERIALIZED_NAME_INVOICE_DATE = "invoiceDate";
@SerializedName(SERIALIZED_NAME_INVOICE_DATE)
private LocalDate invoiceDate;
public static final String SERIALIZED_NAME_INVOICE_ID = "invoiceId";
@SerializedName(SERIALIZED_NAME_INVOICE_ID)
private String invoiceId;
public static final String SERIALIZED_NAME_INVOICE_NUMBER = "invoiceNumber";
@SerializedName(SERIALIZED_NAME_INVOICE_NUMBER)
private String invoiceNumber;
public static final String SERIALIZED_NAME_INVOICE_TARGET_DATE = "invoiceTargetDate";
@SerializedName(SERIALIZED_NAME_INVOICE_TARGET_DATE)
private LocalDate invoiceTargetDate;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY = "paymentGateway";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY)
private String paymentGateway;
public static final String SERIALIZED_NAME_TARGET_DATE = "targetDate";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "paymentMethodId";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public CreateInvoiceCollectRequest() {
}
public CreateInvoiceCollectRequest accountKey(String accountKey) {
this.accountKey = accountKey;
return this;
}
/**
* Customer account ID or account number.
* @return accountKey
*/
@javax.annotation.Nonnull
public String getAccountKey() {
return accountKey;
}
public void setAccountKey(String accountKey) {
this.accountKey = accountKey;
}
public CreateInvoiceCollectRequest documentDate(LocalDate documentDate) {
this.documentDate = documentDate;
return this;
}
/**
* The date that should appear on the invoice and credit memo being generated, in `yyyy-mm-dd` format. If this field is omitted and `invoiceId` is not specified, the current date is used by default. **Note:** The credit memo is only available if you have the Invoice Settlement feature enabled. This field is in Zuora REST API version control. Supported minor versions are `215.0` and later. To use this field in the method, you must set the `zuora-version` parameter to the minor version number in the request header.
* @return documentDate
*/
@javax.annotation.Nullable
public LocalDate getDocumentDate() {
return documentDate;
}
public void setDocumentDate(LocalDate documentDate) {
this.documentDate = documentDate;
}
public CreateInvoiceCollectRequest invoiceDate(LocalDate invoiceDate) {
this.invoiceDate = invoiceDate;
return this;
}
/**
* **Note:** This field has been replaced by the `documentDate` field in Zuora REST API version `215.0` and later. The `invoiceDate` field is only available for backward compatibility. The date that should appear on the invoice being generated, in `yyyy-mm-dd` format. If this field is omitted and `invoiceId` is not specified, the current date is used by default. This field is in Zuora REST API version control. Supported minor versions are `214.0` and earlier.
* @return invoiceDate
*/
@javax.annotation.Nullable
public LocalDate getInvoiceDate() {
return invoiceDate;
}
public void setInvoiceDate(LocalDate invoiceDate) {
this.invoiceDate = invoiceDate;
}
public CreateInvoiceCollectRequest invoiceId(String invoiceId) {
this.invoiceId = invoiceId;
return this;
}
/**
* The ID of an existing invoice for which to collect payment using the account's default payment method. If this value is specified, no new invoice is generated, and the following fields are ignored: - `invoiceDate` and `invoiceTargetDate` (if the Zuora REST API version is 214.0 or earlier) - `documentDate` and `targetDate` (if the Zuora REST API version is 215.0 or later)
* @return invoiceId
*/
@javax.annotation.Nullable
public String getInvoiceId() {
return invoiceId;
}
public void setInvoiceId(String invoiceId) {
this.invoiceId = invoiceId;
}
public CreateInvoiceCollectRequest invoiceNumber(String invoiceNumber) {
this.invoiceNumber = invoiceNumber;
return this;
}
/**
* The number of an existing invoice for which to collect payment using the account's default payment method. If this value is specified, no new invoice is generated, and the following fields are ignored: - `invoiceDate` and `invoiceTargetDate` (if the Zuora REST API version is 214.0 or earlier) - `documentDate` and `targetDate` (if the Zuora REST API version is 215.0 or later)
* @return invoiceNumber
*/
@javax.annotation.Nullable
public String getInvoiceNumber() {
return invoiceNumber;
}
public void setInvoiceNumber(String invoiceNumber) {
this.invoiceNumber = invoiceNumber;
}
public CreateInvoiceCollectRequest invoiceTargetDate(LocalDate invoiceTargetDate) {
this.invoiceTargetDate = invoiceTargetDate;
return this;
}
/**
* **Note:** This field has been replaced by the `targetDate` field in Zuora REST API version `215.0` and later. The `invoiceTargetDate` field is only available for backward compatibility. The date through which to calculate charges on this account if an invoice is generated, in `yyyy-mm-dd` format. If this field is omitted and `invoiceId` is not specified, the current date is used by default. This field is in Zuora REST API version control. Supported minor versions are `214.0` and earlier.
* @return invoiceTargetDate
*/
@javax.annotation.Nullable
public LocalDate getInvoiceTargetDate() {
return invoiceTargetDate;
}
public void setInvoiceTargetDate(LocalDate invoiceTargetDate) {
this.invoiceTargetDate = invoiceTargetDate;
}
public CreateInvoiceCollectRequest paymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
return this;
}
/**
* The name of the gateway that will be used for the payment. Must be a valid gateway name and the gateway must support the specific payment method. If a value is not specified, the default gateway on the Account will be used.
* @return paymentGateway
*/
@javax.annotation.Nullable
public String getPaymentGateway() {
return paymentGateway;
}
public void setPaymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
}
public CreateInvoiceCollectRequest targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* The date through which to calculate charges on this account if an invoice or a credit memo is generated, in `yyyy-mm-dd` format. If this field is omitted and `invoiceId` is not specified, the current date is used by default. **Note:** The credit memo is only available if you have the Invoice Settlement feature enabled. This field is in Zuora REST API version control. Supported minor versions are `215.0` and later. To use this field in the method, you must set the `zuora-version` parameter to the minor version number in the request header.
* @return targetDate
*/
@javax.annotation.Nullable
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public CreateInvoiceCollectRequest paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
return this;
}
/**
* paymentMethodId.
* @return paymentMethodId
*/
@javax.annotation.Nullable
public String getPaymentMethodId() {
return paymentMethodId;
}
public void setPaymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreateInvoiceCollectRequest instance itself
*/
public CreateInvoiceCollectRequest putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateInvoiceCollectRequest createInvoiceCollectRequest = (CreateInvoiceCollectRequest) o;
return Objects.equals(this.accountKey, createInvoiceCollectRequest.accountKey) &&
Objects.equals(this.documentDate, createInvoiceCollectRequest.documentDate) &&
Objects.equals(this.invoiceDate, createInvoiceCollectRequest.invoiceDate) &&
Objects.equals(this.invoiceId, createInvoiceCollectRequest.invoiceId) &&
Objects.equals(this.invoiceNumber, createInvoiceCollectRequest.invoiceNumber) &&
Objects.equals(this.invoiceTargetDate, createInvoiceCollectRequest.invoiceTargetDate) &&
Objects.equals(this.paymentGateway, createInvoiceCollectRequest.paymentGateway) &&
Objects.equals(this.targetDate, createInvoiceCollectRequest.targetDate) &&
Objects.equals(this.paymentMethodId, createInvoiceCollectRequest.paymentMethodId)&&
Objects.equals(this.additionalProperties, createInvoiceCollectRequest.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(accountKey, documentDate, invoiceDate, invoiceId, invoiceNumber, invoiceTargetDate, paymentGateway, targetDate, paymentMethodId, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateInvoiceCollectRequest {\n");
sb.append(" accountKey: ").append(toIndentedString(accountKey)).append("\n");
sb.append(" documentDate: ").append(toIndentedString(documentDate)).append("\n");
sb.append(" invoiceDate: ").append(toIndentedString(invoiceDate)).append("\n");
sb.append(" invoiceId: ").append(toIndentedString(invoiceId)).append("\n");
sb.append(" invoiceNumber: ").append(toIndentedString(invoiceNumber)).append("\n");
sb.append(" invoiceTargetDate: ").append(toIndentedString(invoiceTargetDate)).append("\n");
sb.append(" paymentGateway: ").append(toIndentedString(paymentGateway)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("accountKey");
openapiFields.add("documentDate");
openapiFields.add("invoiceDate");
openapiFields.add("invoiceId");
openapiFields.add("invoiceNumber");
openapiFields.add("invoiceTargetDate");
openapiFields.add("paymentGateway");
openapiFields.add("targetDate");
openapiFields.add("paymentMethodId");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("accountKey");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreateInvoiceCollectRequest
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreateInvoiceCollectRequest.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreateInvoiceCollectRequest is not found in the empty JSON string", CreateInvoiceCollectRequest.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : CreateInvoiceCollectRequest.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if (!jsonObj.get("accountKey").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountKey` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountKey").toString()));
}
if ((jsonObj.get("invoiceId") != null && !jsonObj.get("invoiceId").isJsonNull()) && !jsonObj.get("invoiceId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceId").toString()));
}
if ((jsonObj.get("invoiceNumber") != null && !jsonObj.get("invoiceNumber").isJsonNull()) && !jsonObj.get("invoiceNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceNumber").toString()));
}
if ((jsonObj.get("paymentGateway") != null && !jsonObj.get("paymentGateway").isJsonNull()) && !jsonObj.get("paymentGateway").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentGateway` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentGateway").toString()));
}
if ((jsonObj.get("paymentMethodId") != null && !jsonObj.get("paymentMethodId").isJsonNull()) && !jsonObj.get("paymentMethodId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentMethodId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentMethodId").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreateInvoiceCollectRequest.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreateInvoiceCollectRequest' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreateInvoiceCollectRequest.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreateInvoiceCollectRequest value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreateInvoiceCollectRequest read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreateInvoiceCollectRequest instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreateInvoiceCollectRequest given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreateInvoiceCollectRequest
* @throws IOException if the JSON string is invalid with respect to CreateInvoiceCollectRequest
*/
public static CreateInvoiceCollectRequest fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreateInvoiceCollectRequest.class);
}
/**
* Convert an instance of CreateInvoiceCollectRequest to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy