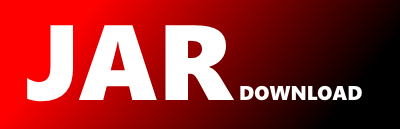
com.zuora.model.CreateOrderCreateSubscription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.CreateOrderRatePlanOverride;
import com.zuora.model.CreateOrderSubscriptionOwnerAccount;
import com.zuora.model.CreateSubscribeToProduct;
import com.zuora.model.OrderActionCreateSubscriptionTerms;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* Information about an order action of type `CreateSubscription`.
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreateOrderCreateSubscription {
public static final String SERIALIZED_NAME_BILL_TO_CONTACT_ID = "billToContactId";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT_ID)
private String billToContactId;
public static final String SERIALIZED_NAME_INVOICE_SEPARATELY = "invoiceSeparately";
@SerializedName(SERIALIZED_NAME_INVOICE_SEPARATELY)
private Boolean invoiceSeparately;
public static final String SERIALIZED_NAME_INVOICE_TEMPLATE_ID = "invoiceTemplateId";
@SerializedName(SERIALIZED_NAME_INVOICE_TEMPLATE_ID)
private String invoiceTemplateId;
public static final String SERIALIZED_NAME_NEW_SUBSCRIPTION_OWNER_ACCOUNT = "newSubscriptionOwnerAccount";
@SerializedName(SERIALIZED_NAME_NEW_SUBSCRIPTION_OWNER_ACCOUNT)
private CreateOrderSubscriptionOwnerAccount newSubscriptionOwnerAccount;
public static final String SERIALIZED_NAME_NOTES = "notes";
@SerializedName(SERIALIZED_NAME_NOTES)
private String notes;
public static final String SERIALIZED_NAME_PAYMENT_TERM = "paymentTerm";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERM)
private String paymentTerm;
public static final String SERIALIZED_NAME_SEQUENCE_SET_ID = "sequenceSetId";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_ID)
private String sequenceSetId;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT_ID = "soldToContactId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT_ID)
private String soldToContactId;
public static final String SERIALIZED_NAME_SHIP_TO_CONTACT_ID = "shipToContactId";
@SerializedName(SERIALIZED_NAME_SHIP_TO_CONTACT_ID)
private String shipToContactId;
public static final String SERIALIZED_NAME_SUBSCRIBE_TO_PRODUCTS = "subscribeToProducts";
@SerializedName(SERIALIZED_NAME_SUBSCRIBE_TO_PRODUCTS)
private List subscribeToProducts;
public static final String SERIALIZED_NAME_SUBSCRIBE_TO_RATE_PLANS = "subscribeToRatePlans";
@SerializedName(SERIALIZED_NAME_SUBSCRIBE_TO_RATE_PLANS)
private List subscribeToRatePlans;
public static final String SERIALIZED_NAME_SUBSCRIPTION_NUMBER = "subscriptionNumber";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_NUMBER)
private String subscriptionNumber;
public static final String SERIALIZED_NAME_SUBSCRIPTION_OWNER_ACCOUNT_NUMBER = "subscriptionOwnerAccountNumber";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_OWNER_ACCOUNT_NUMBER)
private String subscriptionOwnerAccountNumber;
public static final String SERIALIZED_NAME_TERMS = "terms";
@SerializedName(SERIALIZED_NAME_TERMS)
private OrderActionCreateSubscriptionTerms terms;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_INVOICE_GROUP_NUMBER = "invoiceGroupNumber";
@SerializedName(SERIALIZED_NAME_INVOICE_GROUP_NUMBER)
private String invoiceGroupNumber;
public CreateOrderCreateSubscription() {
}
public CreateOrderCreateSubscription billToContactId(String billToContactId) {
this.billToContactId = billToContactId;
return this;
}
/**
* The ID of the bill-to contact associated with the subscription. **Note**: - If you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_customers_at_subscription_level/Flexible_Billing_Attributes\" target=\"_blank\">Flexible Billing Attributes</a> feature disabled, this field is unavailable in the request body and the value of this field is `null` in the response body. - If you have the Flexible Billing Attributes feature enabled, and you do not specify this field in the request or you select **Default Contact from Account** for this field during subscription creation, the value of this field is automatically set to `null` in the response body.
* @return billToContactId
*/
@javax.annotation.Nullable
public String getBillToContactId() {
return billToContactId;
}
public void setBillToContactId(String billToContactId) {
this.billToContactId = billToContactId;
}
public CreateOrderCreateSubscription invoiceSeparately(Boolean invoiceSeparately) {
this.invoiceSeparately = invoiceSeparately;
return this;
}
/**
* Specifies whether the subscription appears on a separate invoice when Zuora generates invoices.
* @return invoiceSeparately
*/
@javax.annotation.Nullable
public Boolean getInvoiceSeparately() {
return invoiceSeparately;
}
public void setInvoiceSeparately(Boolean invoiceSeparately) {
this.invoiceSeparately = invoiceSeparately;
}
public CreateOrderCreateSubscription invoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
return this;
}
/**
* The ID of the invoice template associated with the subscription. **Note**: - If you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_customers_at_subscription_level/Flexible_Billing_Attributes\" target=\"_blank\">Flexible Billing Attributes</a> feature disabled, this field is unavailable in the request body and the value of this field is `null` in the response body. - If you have the Flexible Billing Attributes feature enabled, and you do not specify this field in the request or you select **Default Template from Account** for this field during subscription creation, the value of this field is automatically set to `null` in the response body.
* @return invoiceTemplateId
*/
@javax.annotation.Nullable
public String getInvoiceTemplateId() {
return invoiceTemplateId;
}
public void setInvoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
}
public CreateOrderCreateSubscription newSubscriptionOwnerAccount(CreateOrderSubscriptionOwnerAccount newSubscriptionOwnerAccount) {
this.newSubscriptionOwnerAccount = newSubscriptionOwnerAccount;
return this;
}
/**
* Get newSubscriptionOwnerAccount
* @return newSubscriptionOwnerAccount
*/
@javax.annotation.Nullable
public CreateOrderSubscriptionOwnerAccount getNewSubscriptionOwnerAccount() {
return newSubscriptionOwnerAccount;
}
public void setNewSubscriptionOwnerAccount(CreateOrderSubscriptionOwnerAccount newSubscriptionOwnerAccount) {
this.newSubscriptionOwnerAccount = newSubscriptionOwnerAccount;
}
public CreateOrderCreateSubscription notes(String notes) {
this.notes = notes;
return this;
}
/**
* Notes about the subscription. These notes are only visible to Zuora users.
* @return notes
*/
@javax.annotation.Nullable
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
this.notes = notes;
}
public CreateOrderCreateSubscription paymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
return this;
}
/**
* The name of the payment term associated with the subscription. For example, `Net 30`. The payment term determines the due dates of invoices. **Note**: - If you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_customers_at_subscription_level/Flexible_Billing_Attributes\" target=\"_blank\">Flexible Billing Attributes</a> feature disabled, this field is unavailable in the request body and the value of this field is `null` in the response body. - If you have the Flexible Billing Attributes feature enabled, and you do not specify this field in the request or you select **Default Term from Account** for this field during subscription creation, the value of this field is automatically set to `null` in the response body.
* @return paymentTerm
*/
@javax.annotation.Nullable
public String getPaymentTerm() {
return paymentTerm;
}
public void setPaymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
}
public CreateOrderCreateSubscription sequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
return this;
}
/**
* The ID of the sequence set associated with the subscription. **Note**: - If you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_customers_at_subscription_level/Flexible_Billing_Attributes\" target=\"_blank\">Flexible Billing Attributes</a> feature disabled, this field is unavailable in the request body and the value of this field is `null` in the response body. - If you have the Flexible Billing Attributes feature enabled, and you do not specify this field in the request or you select **Default Set from Account** for this field during subscription creation, the value of this field is automatically set to `null` in the response body.
* @return sequenceSetId
*/
@javax.annotation.Nullable
public String getSequenceSetId() {
return sequenceSetId;
}
public void setSequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
}
public CreateOrderCreateSubscription soldToContactId(String soldToContactId) {
this.soldToContactId = soldToContactId;
return this;
}
/**
* The ID of the sold-to contact associated with the subscription. **Note**: - If you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_customers_at_subscription_level/Flexible_Billing_Attributes\" target=\"_blank\">Flexible Billing Attributes</a> feature disabled, this field is unavailable in the request body and the value of this field is `null` in the response body. - If you have the Flexible Billing Attributes feature enabled, and you do not specify this field in the request or you select **Default Contact from Account** for this field during subscription creation, the value of this field is automatically set to `null` in the response body.
* @return soldToContactId
*/
@javax.annotation.Nullable
public String getSoldToContactId() {
return soldToContactId;
}
public void setSoldToContactId(String soldToContactId) {
this.soldToContactId = soldToContactId;
}
public CreateOrderCreateSubscription shipToContactId(String shipToContactId) {
this.shipToContactId = shipToContactId;
return this;
}
/**
* The ID of the ship-to contact associated with the subscription. it is belong to the subscription owner account
* @return shipToContactId
*/
@javax.annotation.Nullable
public String getShipToContactId() {
return shipToContactId;
}
public void setShipToContactId(String shipToContactId) {
this.shipToContactId = shipToContactId;
}
public CreateOrderCreateSubscription subscribeToProducts(List subscribeToProducts) {
this.subscribeToProducts = subscribeToProducts;
return this;
}
public CreateOrderCreateSubscription addSubscribeToProductsItem(CreateSubscribeToProduct subscribeToProductsItem) {
if (this.subscribeToProducts == null) {
this.subscribeToProducts = new ArrayList<>();
}
this.subscribeToProducts.add(subscribeToProductsItem);
return this;
}
/**
* For a rate plan, the following fields are available: - `chargeOverrides` - `clearingExistingFeatures` - `customFields` - `externallyManagedPlanId` - `newRatePlanId` - `productRatePlanId` - `subscriptionProductFeatures` - `uniqueToken`
* @return subscribeToProducts
*/
@javax.annotation.Nullable
public List getSubscribeToProducts() {
return subscribeToProducts;
}
public void setSubscribeToProducts(List subscribeToProducts) {
this.subscribeToProducts = subscribeToProducts;
}
public CreateOrderCreateSubscription subscribeToRatePlans(List subscribeToRatePlans) {
this.subscribeToRatePlans = subscribeToRatePlans;
return this;
}
public CreateOrderCreateSubscription addSubscribeToRatePlansItem(CreateOrderRatePlanOverride subscribeToRatePlansItem) {
if (this.subscribeToRatePlans == null) {
this.subscribeToRatePlans = new ArrayList<>();
}
this.subscribeToRatePlans.add(subscribeToRatePlansItem);
return this;
}
/**
* List of rate plans associated with the subscription. **Note**: The `subscribeToRatePlans` field has been deprecated, this field is replaced by the `subscribeToProducts` field that supports Rate Plans. In a new order request, you can use either `subscribeToRatePlans` or `subscribeToProducts`, not both.
* @return subscribeToRatePlans
*/
@javax.annotation.Nullable
public List getSubscribeToRatePlans() {
return subscribeToRatePlans;
}
public void setSubscribeToRatePlans(List subscribeToRatePlans) {
this.subscribeToRatePlans = subscribeToRatePlans;
}
public CreateOrderCreateSubscription subscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
return this;
}
/**
* Subscription number of the subscription. For example, A-S00000001. If you do not set this field, Zuora will generate the subscription number.
* @return subscriptionNumber
*/
@javax.annotation.Nullable
public String getSubscriptionNumber() {
return subscriptionNumber;
}
public void setSubscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
}
public CreateOrderCreateSubscription subscriptionOwnerAccountNumber(String subscriptionOwnerAccountNumber) {
this.subscriptionOwnerAccountNumber = subscriptionOwnerAccountNumber;
return this;
}
/**
* Account number of an existing account that will own the subscription. For example, A00000001. If you do not set this field or the `newSubscriptionOwnerAccount` field, the account that owns the order will also own the subscription. Zuora will return an error if you set this field and the `newSubscriptionOwnerAccount` field.
* @return subscriptionOwnerAccountNumber
*/
@javax.annotation.Nullable
public String getSubscriptionOwnerAccountNumber() {
return subscriptionOwnerAccountNumber;
}
public void setSubscriptionOwnerAccountNumber(String subscriptionOwnerAccountNumber) {
this.subscriptionOwnerAccountNumber = subscriptionOwnerAccountNumber;
}
public CreateOrderCreateSubscription terms(OrderActionCreateSubscriptionTerms terms) {
this.terms = terms;
return this;
}
/**
* Get terms
* @return terms
*/
@javax.annotation.Nullable
public OrderActionCreateSubscriptionTerms getTerms() {
return terms;
}
public void setTerms(OrderActionCreateSubscriptionTerms terms) {
this.terms = terms;
}
public CreateOrderCreateSubscription currency(String currency) {
this.currency = currency;
return this;
}
/**
* The code of currency that is used for this subscription. If the currency is not selected, the default currency from the account will be used. All subscriptions in the same order must use the same currency. The currency for a subscription cannot be changed. **Note**: This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Flexible_Billing/Multiple_Currencies\" target=\"_blank\">Multiple Currencies</a> feature enabled.
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public CreateOrderCreateSubscription invoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
return this;
}
/**
* The number of invoice group associated with the subscription. **Note**: This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_customers_at_subscription_level/Flexible_Billing_Attributes\" target=\"_blank\">Flexible Billing Attributes</a> feature enabled.
* @return invoiceGroupNumber
*/
@javax.annotation.Nullable
public String getInvoiceGroupNumber() {
return invoiceGroupNumber;
}
public void setInvoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreateOrderCreateSubscription instance itself
*/
public CreateOrderCreateSubscription putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateOrderCreateSubscription createOrderCreateSubscription = (CreateOrderCreateSubscription) o;
return Objects.equals(this.billToContactId, createOrderCreateSubscription.billToContactId) &&
Objects.equals(this.invoiceSeparately, createOrderCreateSubscription.invoiceSeparately) &&
Objects.equals(this.invoiceTemplateId, createOrderCreateSubscription.invoiceTemplateId) &&
Objects.equals(this.newSubscriptionOwnerAccount, createOrderCreateSubscription.newSubscriptionOwnerAccount) &&
Objects.equals(this.notes, createOrderCreateSubscription.notes) &&
Objects.equals(this.paymentTerm, createOrderCreateSubscription.paymentTerm) &&
Objects.equals(this.sequenceSetId, createOrderCreateSubscription.sequenceSetId) &&
Objects.equals(this.soldToContactId, createOrderCreateSubscription.soldToContactId) &&
Objects.equals(this.shipToContactId, createOrderCreateSubscription.shipToContactId) &&
Objects.equals(this.subscribeToProducts, createOrderCreateSubscription.subscribeToProducts) &&
Objects.equals(this.subscribeToRatePlans, createOrderCreateSubscription.subscribeToRatePlans) &&
Objects.equals(this.subscriptionNumber, createOrderCreateSubscription.subscriptionNumber) &&
Objects.equals(this.subscriptionOwnerAccountNumber, createOrderCreateSubscription.subscriptionOwnerAccountNumber) &&
Objects.equals(this.terms, createOrderCreateSubscription.terms) &&
Objects.equals(this.currency, createOrderCreateSubscription.currency) &&
Objects.equals(this.invoiceGroupNumber, createOrderCreateSubscription.invoiceGroupNumber)&&
Objects.equals(this.additionalProperties, createOrderCreateSubscription.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(billToContactId, invoiceSeparately, invoiceTemplateId, newSubscriptionOwnerAccount, notes, paymentTerm, sequenceSetId, soldToContactId, shipToContactId, subscribeToProducts, subscribeToRatePlans, subscriptionNumber, subscriptionOwnerAccountNumber, terms, currency, invoiceGroupNumber, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateOrderCreateSubscription {\n");
sb.append(" billToContactId: ").append(toIndentedString(billToContactId)).append("\n");
sb.append(" invoiceSeparately: ").append(toIndentedString(invoiceSeparately)).append("\n");
sb.append(" invoiceTemplateId: ").append(toIndentedString(invoiceTemplateId)).append("\n");
sb.append(" newSubscriptionOwnerAccount: ").append(toIndentedString(newSubscriptionOwnerAccount)).append("\n");
sb.append(" notes: ").append(toIndentedString(notes)).append("\n");
sb.append(" paymentTerm: ").append(toIndentedString(paymentTerm)).append("\n");
sb.append(" sequenceSetId: ").append(toIndentedString(sequenceSetId)).append("\n");
sb.append(" soldToContactId: ").append(toIndentedString(soldToContactId)).append("\n");
sb.append(" shipToContactId: ").append(toIndentedString(shipToContactId)).append("\n");
sb.append(" subscribeToProducts: ").append(toIndentedString(subscribeToProducts)).append("\n");
sb.append(" subscribeToRatePlans: ").append(toIndentedString(subscribeToRatePlans)).append("\n");
sb.append(" subscriptionNumber: ").append(toIndentedString(subscriptionNumber)).append("\n");
sb.append(" subscriptionOwnerAccountNumber: ").append(toIndentedString(subscriptionOwnerAccountNumber)).append("\n");
sb.append(" terms: ").append(toIndentedString(terms)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" invoiceGroupNumber: ").append(toIndentedString(invoiceGroupNumber)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("billToContactId");
openapiFields.add("invoiceSeparately");
openapiFields.add("invoiceTemplateId");
openapiFields.add("newSubscriptionOwnerAccount");
openapiFields.add("notes");
openapiFields.add("paymentTerm");
openapiFields.add("sequenceSetId");
openapiFields.add("soldToContactId");
openapiFields.add("shipToContactId");
openapiFields.add("subscribeToProducts");
openapiFields.add("subscribeToRatePlans");
openapiFields.add("subscriptionNumber");
openapiFields.add("subscriptionOwnerAccountNumber");
openapiFields.add("terms");
openapiFields.add("currency");
openapiFields.add("invoiceGroupNumber");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreateOrderCreateSubscription
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreateOrderCreateSubscription.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreateOrderCreateSubscription is not found in the empty JSON string", CreateOrderCreateSubscription.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("billToContactId") != null && !jsonObj.get("billToContactId").isJsonNull()) && !jsonObj.get("billToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billToContactId").toString()));
}
if ((jsonObj.get("invoiceTemplateId") != null && !jsonObj.get("invoiceTemplateId").isJsonNull()) && !jsonObj.get("invoiceTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceTemplateId").toString()));
}
// validate the optional field `newSubscriptionOwnerAccount`
if (jsonObj.get("newSubscriptionOwnerAccount") != null && !jsonObj.get("newSubscriptionOwnerAccount").isJsonNull()) {
CreateOrderSubscriptionOwnerAccount.validateJsonElement(jsonObj.get("newSubscriptionOwnerAccount"));
}
if ((jsonObj.get("notes") != null && !jsonObj.get("notes").isJsonNull()) && !jsonObj.get("notes").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `notes` to be a primitive type in the JSON string but got `%s`", jsonObj.get("notes").toString()));
}
if ((jsonObj.get("paymentTerm") != null && !jsonObj.get("paymentTerm").isJsonNull()) && !jsonObj.get("paymentTerm").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentTerm` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentTerm").toString()));
}
if ((jsonObj.get("sequenceSetId") != null && !jsonObj.get("sequenceSetId").isJsonNull()) && !jsonObj.get("sequenceSetId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sequenceSetId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sequenceSetId").toString()));
}
if ((jsonObj.get("soldToContactId") != null && !jsonObj.get("soldToContactId").isJsonNull()) && !jsonObj.get("soldToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToContactId").toString()));
}
if ((jsonObj.get("shipToContactId") != null && !jsonObj.get("shipToContactId").isJsonNull()) && !jsonObj.get("shipToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `shipToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("shipToContactId").toString()));
}
if (jsonObj.get("subscribeToProducts") != null && !jsonObj.get("subscribeToProducts").isJsonNull()) {
JsonArray jsonArraysubscribeToProducts = jsonObj.getAsJsonArray("subscribeToProducts");
if (jsonArraysubscribeToProducts != null) {
// ensure the json data is an array
if (!jsonObj.get("subscribeToProducts").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `subscribeToProducts` to be an array in the JSON string but got `%s`", jsonObj.get("subscribeToProducts").toString()));
}
// validate the optional field `subscribeToProducts` (array)
for (int i = 0; i < jsonArraysubscribeToProducts.size(); i++) {
CreateSubscribeToProduct.validateJsonElement(jsonArraysubscribeToProducts.get(i));
};
}
}
if (jsonObj.get("subscribeToRatePlans") != null && !jsonObj.get("subscribeToRatePlans").isJsonNull()) {
JsonArray jsonArraysubscribeToRatePlans = jsonObj.getAsJsonArray("subscribeToRatePlans");
if (jsonArraysubscribeToRatePlans != null) {
// ensure the json data is an array
if (!jsonObj.get("subscribeToRatePlans").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `subscribeToRatePlans` to be an array in the JSON string but got `%s`", jsonObj.get("subscribeToRatePlans").toString()));
}
// validate the optional field `subscribeToRatePlans` (array)
for (int i = 0; i < jsonArraysubscribeToRatePlans.size(); i++) {
CreateOrderRatePlanOverride.validateJsonElement(jsonArraysubscribeToRatePlans.get(i));
};
}
}
if ((jsonObj.get("subscriptionNumber") != null && !jsonObj.get("subscriptionNumber").isJsonNull()) && !jsonObj.get("subscriptionNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("subscriptionNumber").toString()));
}
if ((jsonObj.get("subscriptionOwnerAccountNumber") != null && !jsonObj.get("subscriptionOwnerAccountNumber").isJsonNull()) && !jsonObj.get("subscriptionOwnerAccountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionOwnerAccountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("subscriptionOwnerAccountNumber").toString()));
}
// validate the optional field `terms`
if (jsonObj.get("terms") != null && !jsonObj.get("terms").isJsonNull()) {
OrderActionCreateSubscriptionTerms.validateJsonElement(jsonObj.get("terms"));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("invoiceGroupNumber") != null && !jsonObj.get("invoiceGroupNumber").isJsonNull()) && !jsonObj.get("invoiceGroupNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceGroupNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceGroupNumber").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreateOrderCreateSubscription.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreateOrderCreateSubscription' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreateOrderCreateSubscription.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreateOrderCreateSubscription value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreateOrderCreateSubscription read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreateOrderCreateSubscription instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreateOrderCreateSubscription given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreateOrderCreateSubscription
* @throws IOException if the JSON string is invalid with respect to CreateOrderCreateSubscription
*/
public static CreateOrderCreateSubscription fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreateOrderCreateSubscription.class);
}
/**
* Convert an instance of CreateOrderCreateSubscription to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy