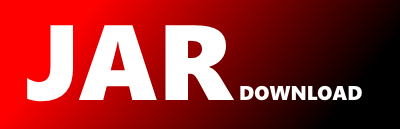
com.zuora.model.CreateOrderProductRatePlanOverride Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.ChargeOverride;
import com.zuora.model.CreateOrderRatePlanFeatureOverride;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* Information about an order action of type `addProduct`.
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreateOrderProductRatePlanOverride {
public static final String SERIALIZED_NAME_CHARGE_OVERRIDES = "chargeOverrides";
@SerializedName(SERIALIZED_NAME_CHARGE_OVERRIDES)
private List chargeOverrides;
public static final String SERIALIZED_NAME_CLEARING_EXISTING_FEATURES = "clearingExistingFeatures";
@SerializedName(SERIALIZED_NAME_CLEARING_EXISTING_FEATURES)
private Boolean clearingExistingFeatures;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "customFields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
private Map customFields;
public static final String SERIALIZED_NAME_EXTERNAL_CATALOG_PLAN_ID = "externalCatalogPlanId";
@SerializedName(SERIALIZED_NAME_EXTERNAL_CATALOG_PLAN_ID)
private String externalCatalogPlanId;
public static final String SERIALIZED_NAME_EXTERNALLY_MANAGED_PLAN_ID = "externallyManagedPlanId";
@SerializedName(SERIALIZED_NAME_EXTERNALLY_MANAGED_PLAN_ID)
private String externallyManagedPlanId;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_ID = "productRatePlanId";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_ID)
private String productRatePlanId;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_NUMBER = "productRatePlanNumber";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_NUMBER)
private String productRatePlanNumber;
public static final String SERIALIZED_NAME_SUBSCRIPTION_PRODUCT_FEATURES = "subscriptionProductFeatures";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_PRODUCT_FEATURES)
private List subscriptionProductFeatures;
public static final String SERIALIZED_NAME_UNIQUE_TOKEN = "uniqueToken";
@SerializedName(SERIALIZED_NAME_UNIQUE_TOKEN)
private String uniqueToken;
public CreateOrderProductRatePlanOverride() {
}
public CreateOrderProductRatePlanOverride chargeOverrides(List chargeOverrides) {
this.chargeOverrides = chargeOverrides;
return this;
}
public CreateOrderProductRatePlanOverride addChargeOverridesItem(ChargeOverride chargeOverridesItem) {
if (this.chargeOverrides == null) {
this.chargeOverrides = new ArrayList<>();
}
this.chargeOverrides.add(chargeOverridesItem);
return this;
}
/**
* List of charges associated with the rate plan.
* @return chargeOverrides
*/
@javax.annotation.Nullable
public List getChargeOverrides() {
return chargeOverrides;
}
public void setChargeOverrides(List chargeOverrides) {
this.chargeOverrides = chargeOverrides;
}
public CreateOrderProductRatePlanOverride clearingExistingFeatures(Boolean clearingExistingFeatures) {
this.clearingExistingFeatures = clearingExistingFeatures;
return this;
}
/**
* Specifies whether all features in the rate plan will be cleared.
* @return clearingExistingFeatures
*/
@javax.annotation.Nullable
public Boolean getClearingExistingFeatures() {
return clearingExistingFeatures;
}
public void setClearingExistingFeatures(Boolean clearingExistingFeatures) {
this.clearingExistingFeatures = clearingExistingFeatures;
}
public CreateOrderProductRatePlanOverride customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public CreateOrderProductRatePlanOverride putCustomFieldsItem(String key, Object customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap<>();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Container for custom fields of the Rate Plan object. The custom fields of the Rate Plan object are used when rate plans are subscribed.
* @return customFields
*/
@javax.annotation.Nullable
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public CreateOrderProductRatePlanOverride externalCatalogPlanId(String externalCatalogPlanId) {
this.externalCatalogPlanId = externalCatalogPlanId;
return this;
}
/**
* An external ID of the product rate plan to be added. You can use this field to specify a product rate plan that is imported from an external system. The value of the `externalCatalogPlanId` field must match one of the values that are predefined in the `externallyManagedPlanIds` field on a product rate plan. **Note:** If both `externalCatalogPlanId` and `productRatePlanId` are provided. They must point to the same product rate plan. Otherwise, the request would fail.
* @return externalCatalogPlanId
*/
@javax.annotation.Nullable
public String getExternalCatalogPlanId() {
return externalCatalogPlanId;
}
public void setExternalCatalogPlanId(String externalCatalogPlanId) {
this.externalCatalogPlanId = externalCatalogPlanId;
}
public CreateOrderProductRatePlanOverride externallyManagedPlanId(String externallyManagedPlanId) {
this.externallyManagedPlanId = externallyManagedPlanId;
return this;
}
/**
* Indicates the unique identifier for the rate plan purchased on a third-party store. This field is used to represent a subscription rate plan created through third-party stores.
* @return externallyManagedPlanId
*/
@javax.annotation.Nullable
public String getExternallyManagedPlanId() {
return externallyManagedPlanId;
}
public void setExternallyManagedPlanId(String externallyManagedPlanId) {
this.externallyManagedPlanId = externallyManagedPlanId;
}
public CreateOrderProductRatePlanOverride productRatePlanId(String productRatePlanId) {
this.productRatePlanId = productRatePlanId;
return this;
}
/**
* Internal identifier of the product rate plan that the rate plan is based on.
* @return productRatePlanId
*/
@javax.annotation.Nullable
public String getProductRatePlanId() {
return productRatePlanId;
}
public void setProductRatePlanId(String productRatePlanId) {
this.productRatePlanId = productRatePlanId;
}
public CreateOrderProductRatePlanOverride productRatePlanNumber(String productRatePlanNumber) {
this.productRatePlanNumber = productRatePlanNumber;
return this;
}
/**
* Number of a product rate plan for this subscription.
* @return productRatePlanNumber
*/
@javax.annotation.Nullable
public String getProductRatePlanNumber() {
return productRatePlanNumber;
}
public void setProductRatePlanNumber(String productRatePlanNumber) {
this.productRatePlanNumber = productRatePlanNumber;
}
public CreateOrderProductRatePlanOverride subscriptionProductFeatures(List subscriptionProductFeatures) {
this.subscriptionProductFeatures = subscriptionProductFeatures;
return this;
}
public CreateOrderProductRatePlanOverride addSubscriptionProductFeaturesItem(CreateOrderRatePlanFeatureOverride subscriptionProductFeaturesItem) {
if (this.subscriptionProductFeatures == null) {
this.subscriptionProductFeatures = new ArrayList<>();
}
this.subscriptionProductFeatures.add(subscriptionProductFeaturesItem);
return this;
}
/**
* List of features associated with the rate plan. The system compares the `subscriptionProductFeatures` and `featureId` fields in the request with the counterpart fields in a rate plan. The comparison results are as follows: * If there is no `subscriptionProductFeatures` field or the field is empty, features in the rate plan remain unchanged. But if the `clearingExistingFeatures` field is additionally set to true, all features in the rate plan are cleared. * If the `subscriptionProductFeatures` field contains the `featureId` nested fields, as well as the optional `description` and `customFields` nested fields, the features indicated by the featureId nested fields in the request overwrite all features in the rate plan.
* @return subscriptionProductFeatures
*/
@javax.annotation.Nullable
public List getSubscriptionProductFeatures() {
return subscriptionProductFeatures;
}
public void setSubscriptionProductFeatures(List subscriptionProductFeatures) {
this.subscriptionProductFeatures = subscriptionProductFeatures;
}
public CreateOrderProductRatePlanOverride uniqueToken(String uniqueToken) {
this.uniqueToken = uniqueToken;
return this;
}
/**
* Unique identifier for the rate plan. This identifier enables you to refer to the rate plan before the rate plan has an internal identifier in Zuora. For instance, suppose that you want to use a single order to add a product to a subscription and later update the same product. When you add the product, you can set a unique identifier for the rate plan. Then when you update the product, you can use the same unique identifier to specify which rate plan to modify.
* @return uniqueToken
*/
@javax.annotation.Nullable
public String getUniqueToken() {
return uniqueToken;
}
public void setUniqueToken(String uniqueToken) {
this.uniqueToken = uniqueToken;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreateOrderProductRatePlanOverride instance itself
*/
public CreateOrderProductRatePlanOverride putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateOrderProductRatePlanOverride createOrderProductRatePlanOverride = (CreateOrderProductRatePlanOverride) o;
return Objects.equals(this.chargeOverrides, createOrderProductRatePlanOverride.chargeOverrides) &&
Objects.equals(this.clearingExistingFeatures, createOrderProductRatePlanOverride.clearingExistingFeatures) &&
Objects.equals(this.customFields, createOrderProductRatePlanOverride.customFields) &&
Objects.equals(this.externalCatalogPlanId, createOrderProductRatePlanOverride.externalCatalogPlanId) &&
Objects.equals(this.externallyManagedPlanId, createOrderProductRatePlanOverride.externallyManagedPlanId) &&
Objects.equals(this.productRatePlanId, createOrderProductRatePlanOverride.productRatePlanId) &&
Objects.equals(this.productRatePlanNumber, createOrderProductRatePlanOverride.productRatePlanNumber) &&
Objects.equals(this.subscriptionProductFeatures, createOrderProductRatePlanOverride.subscriptionProductFeatures) &&
Objects.equals(this.uniqueToken, createOrderProductRatePlanOverride.uniqueToken)&&
Objects.equals(this.additionalProperties, createOrderProductRatePlanOverride.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(chargeOverrides, clearingExistingFeatures, customFields, externalCatalogPlanId, externallyManagedPlanId, productRatePlanId, productRatePlanNumber, subscriptionProductFeatures, uniqueToken, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateOrderProductRatePlanOverride {\n");
sb.append(" chargeOverrides: ").append(toIndentedString(chargeOverrides)).append("\n");
sb.append(" clearingExistingFeatures: ").append(toIndentedString(clearingExistingFeatures)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" externalCatalogPlanId: ").append(toIndentedString(externalCatalogPlanId)).append("\n");
sb.append(" externallyManagedPlanId: ").append(toIndentedString(externallyManagedPlanId)).append("\n");
sb.append(" productRatePlanId: ").append(toIndentedString(productRatePlanId)).append("\n");
sb.append(" productRatePlanNumber: ").append(toIndentedString(productRatePlanNumber)).append("\n");
sb.append(" subscriptionProductFeatures: ").append(toIndentedString(subscriptionProductFeatures)).append("\n");
sb.append(" uniqueToken: ").append(toIndentedString(uniqueToken)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("chargeOverrides");
openapiFields.add("clearingExistingFeatures");
openapiFields.add("customFields");
openapiFields.add("externalCatalogPlanId");
openapiFields.add("externallyManagedPlanId");
openapiFields.add("productRatePlanId");
openapiFields.add("productRatePlanNumber");
openapiFields.add("subscriptionProductFeatures");
openapiFields.add("uniqueToken");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreateOrderProductRatePlanOverride
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreateOrderProductRatePlanOverride.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreateOrderProductRatePlanOverride is not found in the empty JSON string", CreateOrderProductRatePlanOverride.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if (jsonObj.get("chargeOverrides") != null && !jsonObj.get("chargeOverrides").isJsonNull()) {
JsonArray jsonArraychargeOverrides = jsonObj.getAsJsonArray("chargeOverrides");
if (jsonArraychargeOverrides != null) {
// ensure the json data is an array
if (!jsonObj.get("chargeOverrides").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeOverrides` to be an array in the JSON string but got `%s`", jsonObj.get("chargeOverrides").toString()));
}
// validate the optional field `chargeOverrides` (array)
for (int i = 0; i < jsonArraychargeOverrides.size(); i++) {
ChargeOverride.validateJsonElement(jsonArraychargeOverrides.get(i));
};
}
}
if ((jsonObj.get("externalCatalogPlanId") != null && !jsonObj.get("externalCatalogPlanId").isJsonNull()) && !jsonObj.get("externalCatalogPlanId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `externalCatalogPlanId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("externalCatalogPlanId").toString()));
}
if ((jsonObj.get("externallyManagedPlanId") != null && !jsonObj.get("externallyManagedPlanId").isJsonNull()) && !jsonObj.get("externallyManagedPlanId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `externallyManagedPlanId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("externallyManagedPlanId").toString()));
}
if ((jsonObj.get("productRatePlanId") != null && !jsonObj.get("productRatePlanId").isJsonNull()) && !jsonObj.get("productRatePlanId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productRatePlanId").toString()));
}
if ((jsonObj.get("productRatePlanNumber") != null && !jsonObj.get("productRatePlanNumber").isJsonNull()) && !jsonObj.get("productRatePlanNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productRatePlanNumber").toString()));
}
if (jsonObj.get("subscriptionProductFeatures") != null && !jsonObj.get("subscriptionProductFeatures").isJsonNull()) {
JsonArray jsonArraysubscriptionProductFeatures = jsonObj.getAsJsonArray("subscriptionProductFeatures");
if (jsonArraysubscriptionProductFeatures != null) {
// ensure the json data is an array
if (!jsonObj.get("subscriptionProductFeatures").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionProductFeatures` to be an array in the JSON string but got `%s`", jsonObj.get("subscriptionProductFeatures").toString()));
}
// validate the optional field `subscriptionProductFeatures` (array)
for (int i = 0; i < jsonArraysubscriptionProductFeatures.size(); i++) {
CreateOrderRatePlanFeatureOverride.validateJsonElement(jsonArraysubscriptionProductFeatures.get(i));
};
}
}
if ((jsonObj.get("uniqueToken") != null && !jsonObj.get("uniqueToken").isJsonNull()) && !jsonObj.get("uniqueToken").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `uniqueToken` to be a primitive type in the JSON string but got `%s`", jsonObj.get("uniqueToken").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreateOrderProductRatePlanOverride.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreateOrderProductRatePlanOverride' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreateOrderProductRatePlanOverride.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreateOrderProductRatePlanOverride value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreateOrderProductRatePlanOverride read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreateOrderProductRatePlanOverride instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreateOrderProductRatePlanOverride given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreateOrderProductRatePlanOverride
* @throws IOException if the JSON string is invalid with respect to CreateOrderProductRatePlanOverride
*/
public static CreateOrderProductRatePlanOverride fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreateOrderProductRatePlanOverride.class);
}
/**
* Convert an instance of CreateOrderProductRatePlanOverride to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy