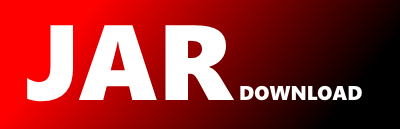
com.zuora.model.CreateOrderResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.CreateOrderResponseOrderLineItems;
import com.zuora.model.CreateOrderResponseRamps;
import com.zuora.model.CreateOrderResponseRefunds;
import com.zuora.model.CreateOrderResponseSubscriptions;
import com.zuora.model.CreateOrderResponseWriteOff;
import com.zuora.model.FailedReason;
import com.zuora.model.OrderStatus;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* CreateOrderResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreateOrderResponse {
public static final String SERIALIZED_NAME_PROCESS_ID = "processId";
@SerializedName(SERIALIZED_NAME_PROCESS_ID)
private String processId;
public static final String SERIALIZED_NAME_REQUEST_ID = "requestId";
@SerializedName(SERIALIZED_NAME_REQUEST_ID)
private String requestId;
public static final String SERIALIZED_NAME_REASONS = "reasons";
@SerializedName(SERIALIZED_NAME_REASONS)
private List reasons;
public static final String SERIALIZED_NAME_SUCCESS = "success";
@SerializedName(SERIALIZED_NAME_SUCCESS)
private Boolean success;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "accountId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "accountNumber";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_CREDIT_MEMO_IDS = "creditMemoIds";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_IDS)
private List creditMemoIds;
public static final String SERIALIZED_NAME_CREDIT_MEMO_NUMBERS = "creditMemoNumbers";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_NUMBERS)
private List creditMemoNumbers;
public static final String SERIALIZED_NAME_INVOICE_IDS = "invoiceIds";
@SerializedName(SERIALIZED_NAME_INVOICE_IDS)
private List invoiceIds;
public static final String SERIALIZED_NAME_INVOICE_NUMBERS = "invoiceNumbers";
@SerializedName(SERIALIZED_NAME_INVOICE_NUMBERS)
private List invoiceNumbers;
public static final String SERIALIZED_NAME_ORDER_ID = "orderId";
@SerializedName(SERIALIZED_NAME_ORDER_ID)
private String orderId;
public static final String SERIALIZED_NAME_ORDER_LINE_ITEMS = "orderLineItems";
@SerializedName(SERIALIZED_NAME_ORDER_LINE_ITEMS)
private List orderLineItems;
public static final String SERIALIZED_NAME_ORDER_NUMBER = "orderNumber";
@SerializedName(SERIALIZED_NAME_ORDER_NUMBER)
private String orderNumber;
public static final String SERIALIZED_NAME_PAID_AMOUNT = "paidAmount";
@SerializedName(SERIALIZED_NAME_PAID_AMOUNT)
private String paidAmount;
public static final String SERIALIZED_NAME_PAYMENT_ID = "paymentId";
@SerializedName(SERIALIZED_NAME_PAYMENT_ID)
private String paymentId;
public static final String SERIALIZED_NAME_PAYMENT_NUMBER = "paymentNumber";
@SerializedName(SERIALIZED_NAME_PAYMENT_NUMBER)
private String paymentNumber;
public static final String SERIALIZED_NAME_RAMPS = "ramps";
@SerializedName(SERIALIZED_NAME_RAMPS)
private List ramps;
public static final String SERIALIZED_NAME_REFUNDS = "refunds";
@SerializedName(SERIALIZED_NAME_REFUNDS)
private List refunds;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private OrderStatus status;
public static final String SERIALIZED_NAME_SUBSCRIPTION_IDS = "subscriptionIds";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_IDS)
private List subscriptionIds;
public static final String SERIALIZED_NAME_SUBSCRIPTION_NUMBERS = "subscriptionNumbers";
@Deprecated
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_NUMBERS)
private List subscriptionNumbers;
public static final String SERIALIZED_NAME_SUBSCRIPTIONS = "subscriptions";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTIONS)
private List subscriptions;
public static final String SERIALIZED_NAME_WRITE_OFF = "writeOff";
@SerializedName(SERIALIZED_NAME_WRITE_OFF)
private List writeOff;
public CreateOrderResponse() {
}
public CreateOrderResponse processId(String processId) {
this.processId = processId;
return this;
}
/**
* The Id of the process that handle the operation.
* @return processId
*/
@javax.annotation.Nullable
public String getProcessId() {
return processId;
}
public void setProcessId(String processId) {
this.processId = processId;
}
public CreateOrderResponse requestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* Unique request identifier. If you need to contact us about a specific request, providing the request identifier will ensure the fastest possible resolution.
* @return requestId
*/
@javax.annotation.Nullable
public String getRequestId() {
return requestId;
}
public void setRequestId(String requestId) {
this.requestId = requestId;
}
public CreateOrderResponse reasons(List reasons) {
this.reasons = reasons;
return this;
}
public CreateOrderResponse addReasonsItem(FailedReason reasonsItem) {
if (this.reasons == null) {
this.reasons = new ArrayList<>();
}
this.reasons.add(reasonsItem);
return this;
}
/**
* Get reasons
* @return reasons
*/
@javax.annotation.Nullable
public List getReasons() {
return reasons;
}
public void setReasons(List reasons) {
this.reasons = reasons;
}
public CreateOrderResponse success(Boolean success) {
this.success = success;
return this;
}
/**
* Indicates whether the call succeeded.
* @return success
*/
@javax.annotation.Nullable
public Boolean getSuccess() {
return success;
}
public void setSuccess(Boolean success) {
this.success = success;
}
public CreateOrderResponse accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* The account ID for the order. This field is returned instead of the `accountNumber` field if the `returnIds` query parameter is set to `true`.
* @return accountId
*/
@javax.annotation.Nullable
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public CreateOrderResponse accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* The account number for the order.
* @return accountNumber
*/
@javax.annotation.Nullable
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public CreateOrderResponse creditMemoIds(List creditMemoIds) {
this.creditMemoIds = creditMemoIds;
return this;
}
public CreateOrderResponse addCreditMemoIdsItem(String creditMemoIdsItem) {
if (this.creditMemoIds == null) {
this.creditMemoIds = new ArrayList<>();
}
this.creditMemoIds.add(creditMemoIdsItem);
return this;
}
/**
* An array of the credit memo IDs generated in this order request. The credit memo is only available if you have the Invoice Settlement feature enabled. This field is returned instead of the `creditMemoNumbers` field if the `returnIds` query parameter is set to `true`.
* @return creditMemoIds
*/
@javax.annotation.Nullable
public List getCreditMemoIds() {
return creditMemoIds;
}
public void setCreditMemoIds(List creditMemoIds) {
this.creditMemoIds = creditMemoIds;
}
public CreateOrderResponse creditMemoNumbers(List creditMemoNumbers) {
this.creditMemoNumbers = creditMemoNumbers;
return this;
}
public CreateOrderResponse addCreditMemoNumbersItem(String creditMemoNumbersItem) {
if (this.creditMemoNumbers == null) {
this.creditMemoNumbers = new ArrayList<>();
}
this.creditMemoNumbers.add(creditMemoNumbersItem);
return this;
}
/**
* An array of the credit memo numbers generated in this order request. The credit memo is only available if you have the Invoice Settlement feature enabled.
* @return creditMemoNumbers
*/
@javax.annotation.Nullable
public List getCreditMemoNumbers() {
return creditMemoNumbers;
}
public void setCreditMemoNumbers(List creditMemoNumbers) {
this.creditMemoNumbers = creditMemoNumbers;
}
public CreateOrderResponse invoiceIds(List invoiceIds) {
this.invoiceIds = invoiceIds;
return this;
}
public CreateOrderResponse addInvoiceIdsItem(String invoiceIdsItem) {
if (this.invoiceIds == null) {
this.invoiceIds = new ArrayList<>();
}
this.invoiceIds.add(invoiceIdsItem);
return this;
}
/**
* An array of the invoice IDs generated in this order request. Normally it includes one invoice ID only, but can include multiple items when a subscription was tagged as invoice separately. This field is returned instead of the `invoiceNumbers` field if the `returnIds` query parameter is set to `true`.
* @return invoiceIds
*/
@javax.annotation.Nullable
public List getInvoiceIds() {
return invoiceIds;
}
public void setInvoiceIds(List invoiceIds) {
this.invoiceIds = invoiceIds;
}
public CreateOrderResponse invoiceNumbers(List invoiceNumbers) {
this.invoiceNumbers = invoiceNumbers;
return this;
}
public CreateOrderResponse addInvoiceNumbersItem(String invoiceNumbersItem) {
if (this.invoiceNumbers == null) {
this.invoiceNumbers = new ArrayList<>();
}
this.invoiceNumbers.add(invoiceNumbersItem);
return this;
}
/**
* An array of the invoice numbers generated in this order request. Normally it includes one invoice number only, but can include multiple items when a subscription was tagged as invoice separately.
* @return invoiceNumbers
*/
@javax.annotation.Nullable
public List getInvoiceNumbers() {
return invoiceNumbers;
}
public void setInvoiceNumbers(List invoiceNumbers) {
this.invoiceNumbers = invoiceNumbers;
}
public CreateOrderResponse orderId(String orderId) {
this.orderId = orderId;
return this;
}
/**
* The ID of the order created. This field is returned instead of the `orderNumber` field if the `returnIds` query parameter is set to `true`.
* @return orderId
*/
@javax.annotation.Nullable
public String getOrderId() {
return orderId;
}
public void setOrderId(String orderId) {
this.orderId = orderId;
}
public CreateOrderResponse orderLineItems(List orderLineItems) {
this.orderLineItems = orderLineItems;
return this;
}
public CreateOrderResponse addOrderLineItemsItem(CreateOrderResponseOrderLineItems orderLineItemsItem) {
if (this.orderLineItems == null) {
this.orderLineItems = new ArrayList<>();
}
this.orderLineItems.add(orderLineItemsItem);
return this;
}
/**
* Get orderLineItems
* @return orderLineItems
*/
@javax.annotation.Nullable
public List getOrderLineItems() {
return orderLineItems;
}
public void setOrderLineItems(List orderLineItems) {
this.orderLineItems = orderLineItems;
}
public CreateOrderResponse orderNumber(String orderNumber) {
this.orderNumber = orderNumber;
return this;
}
/**
* The order number of the order created.
* @return orderNumber
*/
@javax.annotation.Nullable
public String getOrderNumber() {
return orderNumber;
}
public void setOrderNumber(String orderNumber) {
this.orderNumber = orderNumber;
}
public CreateOrderResponse paidAmount(String paidAmount) {
this.paidAmount = paidAmount;
return this;
}
/**
* The total amount collected in this order request.
* @return paidAmount
*/
@javax.annotation.Nullable
public String getPaidAmount() {
return paidAmount;
}
public void setPaidAmount(String paidAmount) {
this.paidAmount = paidAmount;
}
public CreateOrderResponse paymentId(String paymentId) {
this.paymentId = paymentId;
return this;
}
/**
* The payment Id that is collected in this order request. This field is returned instead of the `paymentNumber` field if the `returnIds` query parameter is set to `true`.
* @return paymentId
*/
@javax.annotation.Nullable
public String getPaymentId() {
return paymentId;
}
public void setPaymentId(String paymentId) {
this.paymentId = paymentId;
}
public CreateOrderResponse paymentNumber(String paymentNumber) {
this.paymentNumber = paymentNumber;
return this;
}
/**
* The payment number that is collected in this order request.
* @return paymentNumber
*/
@javax.annotation.Nullable
public String getPaymentNumber() {
return paymentNumber;
}
public void setPaymentNumber(String paymentNumber) {
this.paymentNumber = paymentNumber;
}
public CreateOrderResponse ramps(List ramps) {
this.ramps = ramps;
return this;
}
public CreateOrderResponse addRampsItem(CreateOrderResponseRamps rampsItem) {
if (this.ramps == null) {
this.ramps = new ArrayList<>();
}
this.ramps.add(rampsItem);
return this;
}
/**
* **Note**: This field is only available if you have the Ramps feature enabled. The [Orders](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/AA_Overview_of_Orders) feature must be enabled before you can access the [Ramps](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/Ramps_and_Ramp_Metrics/A_Overview_of_Ramps_and_Ramp_Metrics) feature. The Ramps feature is available for customers with Enterprise and Nine editions by default. If you are a Growth customer, see [Zuora Editions](https://knowledgecenter.zuora.com/BB_Introducing_Z_Business/C_Zuora_Editions) for pricing information coming October 2020. The ramp definitions created by this order request.
* @return ramps
*/
@javax.annotation.Nullable
public List getRamps() {
return ramps;
}
public void setRamps(List ramps) {
this.ramps = ramps;
}
public CreateOrderResponse refunds(List refunds) {
this.refunds = refunds;
return this;
}
public CreateOrderResponse addRefundsItem(CreateOrderResponseRefunds refundsItem) {
if (this.refunds == null) {
this.refunds = new ArrayList<>();
}
this.refunds.add(refundsItem);
return this;
}
/**
* Get refunds
* @return refunds
*/
@javax.annotation.Nullable
public List getRefunds() {
return refunds;
}
public void setRefunds(List refunds) {
this.refunds = refunds;
}
public CreateOrderResponse status(OrderStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public OrderStatus getStatus() {
return status;
}
public void setStatus(OrderStatus status) {
this.status = status;
}
public CreateOrderResponse subscriptionIds(List subscriptionIds) {
this.subscriptionIds = subscriptionIds;
return this;
}
public CreateOrderResponse addSubscriptionIdsItem(String subscriptionIdsItem) {
if (this.subscriptionIds == null) {
this.subscriptionIds = new ArrayList<>();
}
this.subscriptionIds.add(subscriptionIdsItem);
return this;
}
/**
* Container for the subscription IDs of the subscriptions in an order. This field is returned if the `returnIds` query parameter is set to `true`.
* @return subscriptionIds
*/
@javax.annotation.Nullable
public List getSubscriptionIds() {
return subscriptionIds;
}
public void setSubscriptionIds(List subscriptionIds) {
this.subscriptionIds = subscriptionIds;
}
@Deprecated
public CreateOrderResponse subscriptionNumbers(List subscriptionNumbers) {
this.subscriptionNumbers = subscriptionNumbers;
return this;
}
public CreateOrderResponse addSubscriptionNumbersItem(String subscriptionNumbersItem) {
if (this.subscriptionNumbers == null) {
this.subscriptionNumbers = new ArrayList<>();
}
this.subscriptionNumbers.add(subscriptionNumbersItem);
return this;
}
/**
* Container for the subscription numbers of the subscriptions in an order. Subscriptions in the response are displayed in the same sequence as the subscriptions defined in the request. This field is in Zuora REST API version control. Supported minor versions are `206.0` and earlier.
* @return subscriptionNumbers
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public List getSubscriptionNumbers() {
return subscriptionNumbers;
}
@Deprecated
public void setSubscriptionNumbers(List subscriptionNumbers) {
this.subscriptionNumbers = subscriptionNumbers;
}
public CreateOrderResponse subscriptions(List subscriptions) {
this.subscriptions = subscriptions;
return this;
}
public CreateOrderResponse addSubscriptionsItem(CreateOrderResponseSubscriptions subscriptionsItem) {
if (this.subscriptions == null) {
this.subscriptions = new ArrayList<>();
}
this.subscriptions.add(subscriptionsItem);
return this;
}
/**
* **Note:** This field is in Zuora REST API version control. Supported minor versions are 223.0 or later. To use this field in the method, you must set the `zuora-version` parameter to the minor version number in the request header. Container for the subscription numbers and statuses in an order.
* @return subscriptions
*/
@javax.annotation.Nullable
public List getSubscriptions() {
return subscriptions;
}
public void setSubscriptions(List subscriptions) {
this.subscriptions = subscriptions;
}
public CreateOrderResponse writeOff(List writeOff) {
this.writeOff = writeOff;
return this;
}
public CreateOrderResponse addWriteOffItem(CreateOrderResponseWriteOff writeOffItem) {
if (this.writeOff == null) {
this.writeOff = new ArrayList<>();
}
this.writeOff.add(writeOffItem);
return this;
}
/**
* Get writeOff
* @return writeOff
*/
@javax.annotation.Nullable
public List getWriteOff() {
return writeOff;
}
public void setWriteOff(List writeOff) {
this.writeOff = writeOff;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreateOrderResponse instance itself
*/
public CreateOrderResponse putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateOrderResponse createOrderResponse = (CreateOrderResponse) o;
return Objects.equals(this.processId, createOrderResponse.processId) &&
Objects.equals(this.requestId, createOrderResponse.requestId) &&
Objects.equals(this.reasons, createOrderResponse.reasons) &&
Objects.equals(this.success, createOrderResponse.success) &&
Objects.equals(this.accountId, createOrderResponse.accountId) &&
Objects.equals(this.accountNumber, createOrderResponse.accountNumber) &&
Objects.equals(this.creditMemoIds, createOrderResponse.creditMemoIds) &&
Objects.equals(this.creditMemoNumbers, createOrderResponse.creditMemoNumbers) &&
Objects.equals(this.invoiceIds, createOrderResponse.invoiceIds) &&
Objects.equals(this.invoiceNumbers, createOrderResponse.invoiceNumbers) &&
Objects.equals(this.orderId, createOrderResponse.orderId) &&
Objects.equals(this.orderLineItems, createOrderResponse.orderLineItems) &&
Objects.equals(this.orderNumber, createOrderResponse.orderNumber) &&
Objects.equals(this.paidAmount, createOrderResponse.paidAmount) &&
Objects.equals(this.paymentId, createOrderResponse.paymentId) &&
Objects.equals(this.paymentNumber, createOrderResponse.paymentNumber) &&
Objects.equals(this.ramps, createOrderResponse.ramps) &&
Objects.equals(this.refunds, createOrderResponse.refunds) &&
Objects.equals(this.status, createOrderResponse.status) &&
Objects.equals(this.subscriptionIds, createOrderResponse.subscriptionIds) &&
Objects.equals(this.subscriptionNumbers, createOrderResponse.subscriptionNumbers) &&
Objects.equals(this.subscriptions, createOrderResponse.subscriptions) &&
Objects.equals(this.writeOff, createOrderResponse.writeOff)&&
Objects.equals(this.additionalProperties, createOrderResponse.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(processId, requestId, reasons, success, accountId, accountNumber, creditMemoIds, creditMemoNumbers, invoiceIds, invoiceNumbers, orderId, orderLineItems, orderNumber, paidAmount, paymentId, paymentNumber, ramps, refunds, status, subscriptionIds, subscriptionNumbers, subscriptions, writeOff, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateOrderResponse {\n");
sb.append(" processId: ").append(toIndentedString(processId)).append("\n");
sb.append(" requestId: ").append(toIndentedString(requestId)).append("\n");
sb.append(" reasons: ").append(toIndentedString(reasons)).append("\n");
sb.append(" success: ").append(toIndentedString(success)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" creditMemoIds: ").append(toIndentedString(creditMemoIds)).append("\n");
sb.append(" creditMemoNumbers: ").append(toIndentedString(creditMemoNumbers)).append("\n");
sb.append(" invoiceIds: ").append(toIndentedString(invoiceIds)).append("\n");
sb.append(" invoiceNumbers: ").append(toIndentedString(invoiceNumbers)).append("\n");
sb.append(" orderId: ").append(toIndentedString(orderId)).append("\n");
sb.append(" orderLineItems: ").append(toIndentedString(orderLineItems)).append("\n");
sb.append(" orderNumber: ").append(toIndentedString(orderNumber)).append("\n");
sb.append(" paidAmount: ").append(toIndentedString(paidAmount)).append("\n");
sb.append(" paymentId: ").append(toIndentedString(paymentId)).append("\n");
sb.append(" paymentNumber: ").append(toIndentedString(paymentNumber)).append("\n");
sb.append(" ramps: ").append(toIndentedString(ramps)).append("\n");
sb.append(" refunds: ").append(toIndentedString(refunds)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" subscriptionIds: ").append(toIndentedString(subscriptionIds)).append("\n");
sb.append(" subscriptionNumbers: ").append(toIndentedString(subscriptionNumbers)).append("\n");
sb.append(" subscriptions: ").append(toIndentedString(subscriptions)).append("\n");
sb.append(" writeOff: ").append(toIndentedString(writeOff)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("processId");
openapiFields.add("requestId");
openapiFields.add("reasons");
openapiFields.add("success");
openapiFields.add("accountId");
openapiFields.add("accountNumber");
openapiFields.add("creditMemoIds");
openapiFields.add("creditMemoNumbers");
openapiFields.add("invoiceIds");
openapiFields.add("invoiceNumbers");
openapiFields.add("orderId");
openapiFields.add("orderLineItems");
openapiFields.add("orderNumber");
openapiFields.add("paidAmount");
openapiFields.add("paymentId");
openapiFields.add("paymentNumber");
openapiFields.add("ramps");
openapiFields.add("refunds");
openapiFields.add("status");
openapiFields.add("subscriptionIds");
openapiFields.add("subscriptionNumbers");
openapiFields.add("subscriptions");
openapiFields.add("writeOff");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreateOrderResponse
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreateOrderResponse.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreateOrderResponse is not found in the empty JSON string", CreateOrderResponse.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("processId") != null && !jsonObj.get("processId").isJsonNull()) && !jsonObj.get("processId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `processId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("processId").toString()));
}
if ((jsonObj.get("requestId") != null && !jsonObj.get("requestId").isJsonNull()) && !jsonObj.get("requestId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `requestId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("requestId").toString()));
}
if (jsonObj.get("reasons") != null && !jsonObj.get("reasons").isJsonNull()) {
JsonArray jsonArrayreasons = jsonObj.getAsJsonArray("reasons");
if (jsonArrayreasons != null) {
// ensure the json data is an array
if (!jsonObj.get("reasons").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `reasons` to be an array in the JSON string but got `%s`", jsonObj.get("reasons").toString()));
}
// validate the optional field `reasons` (array)
for (int i = 0; i < jsonArrayreasons.size(); i++) {
FailedReason.validateJsonElement(jsonArrayreasons.get(i));
};
}
}
if ((jsonObj.get("accountId") != null && !jsonObj.get("accountId").isJsonNull()) && !jsonObj.get("accountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountId").toString()));
}
if ((jsonObj.get("accountNumber") != null && !jsonObj.get("accountNumber").isJsonNull()) && !jsonObj.get("accountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountNumber").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("creditMemoIds") != null && !jsonObj.get("creditMemoIds").isJsonNull() && !jsonObj.get("creditMemoIds").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `creditMemoIds` to be an array in the JSON string but got `%s`", jsonObj.get("creditMemoIds").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("creditMemoNumbers") != null && !jsonObj.get("creditMemoNumbers").isJsonNull() && !jsonObj.get("creditMemoNumbers").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `creditMemoNumbers` to be an array in the JSON string but got `%s`", jsonObj.get("creditMemoNumbers").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("invoiceIds") != null && !jsonObj.get("invoiceIds").isJsonNull() && !jsonObj.get("invoiceIds").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceIds` to be an array in the JSON string but got `%s`", jsonObj.get("invoiceIds").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("invoiceNumbers") != null && !jsonObj.get("invoiceNumbers").isJsonNull() && !jsonObj.get("invoiceNumbers").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceNumbers` to be an array in the JSON string but got `%s`", jsonObj.get("invoiceNumbers").toString()));
}
if ((jsonObj.get("orderId") != null && !jsonObj.get("orderId").isJsonNull()) && !jsonObj.get("orderId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `orderId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("orderId").toString()));
}
if (jsonObj.get("orderLineItems") != null && !jsonObj.get("orderLineItems").isJsonNull()) {
JsonArray jsonArrayorderLineItems = jsonObj.getAsJsonArray("orderLineItems");
if (jsonArrayorderLineItems != null) {
// ensure the json data is an array
if (!jsonObj.get("orderLineItems").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `orderLineItems` to be an array in the JSON string but got `%s`", jsonObj.get("orderLineItems").toString()));
}
// validate the optional field `orderLineItems` (array)
for (int i = 0; i < jsonArrayorderLineItems.size(); i++) {
CreateOrderResponseOrderLineItems.validateJsonElement(jsonArrayorderLineItems.get(i));
};
}
}
if ((jsonObj.get("orderNumber") != null && !jsonObj.get("orderNumber").isJsonNull()) && !jsonObj.get("orderNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `orderNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("orderNumber").toString()));
}
if ((jsonObj.get("paidAmount") != null && !jsonObj.get("paidAmount").isJsonNull()) && !jsonObj.get("paidAmount").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paidAmount` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paidAmount").toString()));
}
if ((jsonObj.get("paymentId") != null && !jsonObj.get("paymentId").isJsonNull()) && !jsonObj.get("paymentId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentId").toString()));
}
if ((jsonObj.get("paymentNumber") != null && !jsonObj.get("paymentNumber").isJsonNull()) && !jsonObj.get("paymentNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentNumber").toString()));
}
if (jsonObj.get("ramps") != null && !jsonObj.get("ramps").isJsonNull()) {
JsonArray jsonArrayramps = jsonObj.getAsJsonArray("ramps");
if (jsonArrayramps != null) {
// ensure the json data is an array
if (!jsonObj.get("ramps").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `ramps` to be an array in the JSON string but got `%s`", jsonObj.get("ramps").toString()));
}
// validate the optional field `ramps` (array)
for (int i = 0; i < jsonArrayramps.size(); i++) {
CreateOrderResponseRamps.validateJsonElement(jsonArrayramps.get(i));
};
}
}
if (jsonObj.get("refunds") != null && !jsonObj.get("refunds").isJsonNull()) {
JsonArray jsonArrayrefunds = jsonObj.getAsJsonArray("refunds");
if (jsonArrayrefunds != null) {
// ensure the json data is an array
if (!jsonObj.get("refunds").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `refunds` to be an array in the JSON string but got `%s`", jsonObj.get("refunds").toString()));
}
// validate the optional field `refunds` (array)
for (int i = 0; i < jsonArrayrefunds.size(); i++) {
CreateOrderResponseRefunds.validateJsonElement(jsonArrayrefunds.get(i));
};
}
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
// validate the optional field `status`
if (jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) {
OrderStatus.validateJsonElement(jsonObj.get("status"));
}
// ensure the optional json data is an array if present
if (jsonObj.get("subscriptionIds") != null && !jsonObj.get("subscriptionIds").isJsonNull() && !jsonObj.get("subscriptionIds").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionIds` to be an array in the JSON string but got `%s`", jsonObj.get("subscriptionIds").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("subscriptionNumbers") != null && !jsonObj.get("subscriptionNumbers").isJsonNull() && !jsonObj.get("subscriptionNumbers").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionNumbers` to be an array in the JSON string but got `%s`", jsonObj.get("subscriptionNumbers").toString()));
}
if (jsonObj.get("subscriptions") != null && !jsonObj.get("subscriptions").isJsonNull()) {
JsonArray jsonArraysubscriptions = jsonObj.getAsJsonArray("subscriptions");
if (jsonArraysubscriptions != null) {
// ensure the json data is an array
if (!jsonObj.get("subscriptions").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptions` to be an array in the JSON string but got `%s`", jsonObj.get("subscriptions").toString()));
}
// validate the optional field `subscriptions` (array)
for (int i = 0; i < jsonArraysubscriptions.size(); i++) {
CreateOrderResponseSubscriptions.validateJsonElement(jsonArraysubscriptions.get(i));
};
}
}
if (jsonObj.get("writeOff") != null && !jsonObj.get("writeOff").isJsonNull()) {
JsonArray jsonArraywriteOff = jsonObj.getAsJsonArray("writeOff");
if (jsonArraywriteOff != null) {
// ensure the json data is an array
if (!jsonObj.get("writeOff").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `writeOff` to be an array in the JSON string but got `%s`", jsonObj.get("writeOff").toString()));
}
// validate the optional field `writeOff` (array)
for (int i = 0; i < jsonArraywriteOff.size(); i++) {
CreateOrderResponseWriteOff.validateJsonElement(jsonArraywriteOff.get(i));
};
}
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreateOrderResponse.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreateOrderResponse' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreateOrderResponse.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreateOrderResponse value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreateOrderResponse read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreateOrderResponse instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreateOrderResponse given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreateOrderResponse
* @throws IOException if the JSON string is invalid with respect to CreateOrderResponse
*/
public static CreateOrderResponse fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreateOrderResponse.class);
}
/**
* Convert an instance of CreateOrderResponse to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy