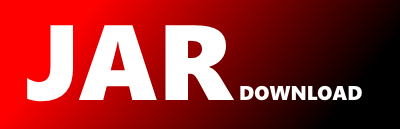
com.zuora.model.CreateOrderSubscriptionOwnerAccount Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.CreateAccountContact;
import com.zuora.model.CreateAccountPaymentMethod;
import com.zuora.model.CreditCard;
import com.zuora.model.TaxInfo;
import java.io.IOException;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* Information about a new account that will own the subscription. Only available if you have enabled the Owner Transfer feature. **Note:** The Owner Transfer feature is in **Limited Availability**. If you wish to have access to the feature, submit a request at [Zuora Global Support](http://support.zuora.com/). If you do not set this field or the `subscriptionOwnerAccountNumber` field, the account that owns the order will also own the subscription. Zuora will return an error if you set this field and the `subscriptionOwnerAccountNumber` field.
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreateOrderSubscriptionOwnerAccount {
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "accountNumber";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_AUTO_PAY = "autoPay";
@SerializedName(SERIALIZED_NAME_AUTO_PAY)
private Boolean autoPay;
public static final String SERIALIZED_NAME_BATCH = "batch";
@SerializedName(SERIALIZED_NAME_BATCH)
private String batch;
public static final String SERIALIZED_NAME_BILL_CYCLE_DAY = "billCycleDay";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_DAY)
private Integer billCycleDay;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT = "billToContact";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT)
private CreateAccountContact billToContact;
public static final String SERIALIZED_NAME_COMMUNICATION_PROFILE_ID = "communicationProfileId";
@SerializedName(SERIALIZED_NAME_COMMUNICATION_PROFILE_ID)
private String communicationProfileId;
public static final String SERIALIZED_NAME_CREDIT_CARD = "creditCard";
@SerializedName(SERIALIZED_NAME_CREDIT_CARD)
private CreditCard creditCard;
public static final String SERIALIZED_NAME_CRM_ID = "crmId";
@SerializedName(SERIALIZED_NAME_CRM_ID)
private String crmId;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "customFields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
private Map customFields;
public static final String SERIALIZED_NAME_HPM_CREDIT_CARD_PAYMENT_METHOD_ID = "hpmCreditCardPaymentMethodId";
@SerializedName(SERIALIZED_NAME_HPM_CREDIT_CARD_PAYMENT_METHOD_ID)
private String hpmCreditCardPaymentMethodId;
public static final String SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_EMAIL = "invoiceDeliveryPrefsEmail";
@SerializedName(SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_EMAIL)
private Boolean invoiceDeliveryPrefsEmail;
public static final String SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_PRINT = "invoiceDeliveryPrefsPrint";
@SerializedName(SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_PRINT)
private Boolean invoiceDeliveryPrefsPrint;
public static final String SERIALIZED_NAME_INVOICE_TEMPLATE_ID = "invoiceTemplateId";
@SerializedName(SERIALIZED_NAME_INVOICE_TEMPLATE_ID)
private String invoiceTemplateId;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_NOTES = "notes";
@SerializedName(SERIALIZED_NAME_NOTES)
private String notes;
public static final String SERIALIZED_NAME_PARENT_ID = "parentId";
@SerializedName(SERIALIZED_NAME_PARENT_ID)
private String parentId;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY = "paymentGateway";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY)
private String paymentGateway;
public static final String SERIALIZED_NAME_PAYMENT_METHOD = "paymentMethod";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD)
private CreateAccountPaymentMethod paymentMethod;
public static final String SERIALIZED_NAME_PAYMENT_TERM = "paymentTerm";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERM)
private String paymentTerm;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT = "soldToContact";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT)
private CreateAccountContact soldToContact;
public static final String SERIALIZED_NAME_TAX_INFO = "taxInfo";
@SerializedName(SERIALIZED_NAME_TAX_INFO)
private TaxInfo taxInfo;
public CreateOrderSubscriptionOwnerAccount() {
}
public CreateOrderSubscriptionOwnerAccount accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Account number. For example, A00000001.
* @return accountNumber
*/
@javax.annotation.Nullable
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public CreateOrderSubscriptionOwnerAccount autoPay(Boolean autoPay) {
this.autoPay = autoPay;
return this;
}
/**
* Specifies whether future payments are automatically billed when they are due.
* @return autoPay
*/
@javax.annotation.Nullable
public Boolean getAutoPay() {
return autoPay;
}
public void setAutoPay(Boolean autoPay) {
this.autoPay = autoPay;
}
public CreateOrderSubscriptionOwnerAccount batch(String batch) {
this.batch = batch;
return this;
}
/**
* Name of the billing batch that the account belongs to. For example, Batch1.
* @return batch
*/
@javax.annotation.Nullable
public String getBatch() {
return batch;
}
public void setBatch(String batch) {
this.batch = batch;
}
public CreateOrderSubscriptionOwnerAccount billCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
return this;
}
/**
* Day of the month that the account prefers billing periods to begin on. If set to 0, the bill cycle day will be set as \"AutoSet\".
* minimum: 0
* maximum: 31
* @return billCycleDay
*/
@javax.annotation.Nonnull
public Integer getBillCycleDay() {
return billCycleDay;
}
public void setBillCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
}
public CreateOrderSubscriptionOwnerAccount billToContact(CreateAccountContact billToContact) {
this.billToContact = billToContact;
return this;
}
/**
* Get billToContact
* @return billToContact
*/
@javax.annotation.Nonnull
public CreateAccountContact getBillToContact() {
return billToContact;
}
public void setBillToContact(CreateAccountContact billToContact) {
this.billToContact = billToContact;
}
public CreateOrderSubscriptionOwnerAccount communicationProfileId(String communicationProfileId) {
this.communicationProfileId = communicationProfileId;
return this;
}
/**
* Internal identifier of the communication profile that Zuora uses when sending notifications to the account's contacts.
* @return communicationProfileId
*/
@javax.annotation.Nullable
public String getCommunicationProfileId() {
return communicationProfileId;
}
public void setCommunicationProfileId(String communicationProfileId) {
this.communicationProfileId = communicationProfileId;
}
public CreateOrderSubscriptionOwnerAccount creditCard(CreditCard creditCard) {
this.creditCard = creditCard;
return this;
}
/**
* Get creditCard
* @return creditCard
*/
@javax.annotation.Nullable
public CreditCard getCreditCard() {
return creditCard;
}
public void setCreditCard(CreditCard creditCard) {
this.creditCard = creditCard;
}
public CreateOrderSubscriptionOwnerAccount crmId(String crmId) {
this.crmId = crmId;
return this;
}
/**
* External identifier of the account in a CRM system.
* @return crmId
*/
@javax.annotation.Nullable
public String getCrmId() {
return crmId;
}
public void setCrmId(String crmId) {
this.crmId = crmId;
}
public CreateOrderSubscriptionOwnerAccount currency(String currency) {
this.currency = currency;
return this;
}
/**
* ISO 3-letter currency code (uppercase). For example, USD.
* @return currency
*/
@javax.annotation.Nonnull
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public CreateOrderSubscriptionOwnerAccount customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public CreateOrderSubscriptionOwnerAccount putCustomFieldsItem(String key, Object customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap<>();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Container for custom fields of an Account object.
* @return customFields
*/
@javax.annotation.Nullable
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public CreateOrderSubscriptionOwnerAccount hpmCreditCardPaymentMethodId(String hpmCreditCardPaymentMethodId) {
this.hpmCreditCardPaymentMethodId = hpmCreditCardPaymentMethodId;
return this;
}
/**
* The ID of the payment method associated with this account. The payment method specified for this field will be set as the default payment method of the account. If the `autoPay` field is set to `true`, you must provide the credit card payment method ID for either this field or the `creditCard` field, but not both. For the Credit Card Reference Transaction payment method, you can specify the payment method ID in this field or use the `paymentMethod` field to create a CC Reference Transaction payment method for an account.
* @return hpmCreditCardPaymentMethodId
*/
@javax.annotation.Nullable
public String getHpmCreditCardPaymentMethodId() {
return hpmCreditCardPaymentMethodId;
}
public void setHpmCreditCardPaymentMethodId(String hpmCreditCardPaymentMethodId) {
this.hpmCreditCardPaymentMethodId = hpmCreditCardPaymentMethodId;
}
public CreateOrderSubscriptionOwnerAccount invoiceDeliveryPrefsEmail(Boolean invoiceDeliveryPrefsEmail) {
this.invoiceDeliveryPrefsEmail = invoiceDeliveryPrefsEmail;
return this;
}
/**
* Specifies whether to turn on the invoice delivery method 'Email' for the new account. Values are: * `true` (default). Turn on the invoice delivery method 'Email' for the new account. * `false`. Turn off the invoice delivery method 'Email' for the new account.
* @return invoiceDeliveryPrefsEmail
*/
@javax.annotation.Nullable
public Boolean getInvoiceDeliveryPrefsEmail() {
return invoiceDeliveryPrefsEmail;
}
public void setInvoiceDeliveryPrefsEmail(Boolean invoiceDeliveryPrefsEmail) {
this.invoiceDeliveryPrefsEmail = invoiceDeliveryPrefsEmail;
}
public CreateOrderSubscriptionOwnerAccount invoiceDeliveryPrefsPrint(Boolean invoiceDeliveryPrefsPrint) {
this.invoiceDeliveryPrefsPrint = invoiceDeliveryPrefsPrint;
return this;
}
/**
* Specifies whether to turn on the invoice delivery method 'Print' for the new account. Values are: * `true`. Turn on the invoice delivery method 'Print' for the new account. * `false` (default). Turn off the invoice delivery method 'Print' for the new account.
* @return invoiceDeliveryPrefsPrint
*/
@javax.annotation.Nullable
public Boolean getInvoiceDeliveryPrefsPrint() {
return invoiceDeliveryPrefsPrint;
}
public void setInvoiceDeliveryPrefsPrint(Boolean invoiceDeliveryPrefsPrint) {
this.invoiceDeliveryPrefsPrint = invoiceDeliveryPrefsPrint;
}
public CreateOrderSubscriptionOwnerAccount invoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
return this;
}
/**
* Internal identifier of the invoice template that Zuora uses when generating invoices for the account.
* @return invoiceTemplateId
*/
@javax.annotation.Nullable
public String getInvoiceTemplateId() {
return invoiceTemplateId;
}
public void setInvoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
}
public CreateOrderSubscriptionOwnerAccount name(String name) {
this.name = name;
return this;
}
/**
* Account name.
* @return name
*/
@javax.annotation.Nonnull
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public CreateOrderSubscriptionOwnerAccount notes(String notes) {
this.notes = notes;
return this;
}
/**
* Notes about the account. These notes are only visible to Zuora users.
* @return notes
*/
@javax.annotation.Nullable
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
this.notes = notes;
}
public CreateOrderSubscriptionOwnerAccount parentId(String parentId) {
this.parentId = parentId;
return this;
}
/**
* Identifier of the parent customer account for this Account object. Use this field if you have <a href=\"https://knowledgecenter.zuora.com/Billing/Subscriptions/Customer_Accounts/A_Customer_Account_Introduction#Customer_Hierarchy\" target=\"_blank\">Customer Hierarchy</a> enabled.
* @return parentId
*/
@javax.annotation.Nullable
public String getParentId() {
return parentId;
}
public void setParentId(String parentId) {
this.parentId = parentId;
}
public CreateOrderSubscriptionOwnerAccount paymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
return this;
}
/**
* The payment gateway that Zuora uses when processing electronic payments and refunds for the account. If you do not specify this field or if the value of this field is null, Zuora uses your default payment gateway.
* @return paymentGateway
*/
@javax.annotation.Nullable
public String getPaymentGateway() {
return paymentGateway;
}
public void setPaymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
}
public CreateOrderSubscriptionOwnerAccount paymentMethod(CreateAccountPaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
return this;
}
/**
* Get paymentMethod
* @return paymentMethod
*/
@javax.annotation.Nullable
public CreateAccountPaymentMethod getPaymentMethod() {
return paymentMethod;
}
public void setPaymentMethod(CreateAccountPaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
}
public CreateOrderSubscriptionOwnerAccount paymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
return this;
}
/**
* Name of the payment term associated with the account. For example, \"Net 30\". The payment term determines the due dates of invoices.
* @return paymentTerm
*/
@javax.annotation.Nullable
public String getPaymentTerm() {
return paymentTerm;
}
public void setPaymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
}
public CreateOrderSubscriptionOwnerAccount soldToContact(CreateAccountContact soldToContact) {
this.soldToContact = soldToContact;
return this;
}
/**
* Get soldToContact
* @return soldToContact
*/
@javax.annotation.Nullable
public CreateAccountContact getSoldToContact() {
return soldToContact;
}
public void setSoldToContact(CreateAccountContact soldToContact) {
this.soldToContact = soldToContact;
}
public CreateOrderSubscriptionOwnerAccount taxInfo(TaxInfo taxInfo) {
this.taxInfo = taxInfo;
return this;
}
/**
* Get taxInfo
* @return taxInfo
*/
@javax.annotation.Nullable
public TaxInfo getTaxInfo() {
return taxInfo;
}
public void setTaxInfo(TaxInfo taxInfo) {
this.taxInfo = taxInfo;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreateOrderSubscriptionOwnerAccount instance itself
*/
public CreateOrderSubscriptionOwnerAccount putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateOrderSubscriptionOwnerAccount createOrderSubscriptionOwnerAccount = (CreateOrderSubscriptionOwnerAccount) o;
return Objects.equals(this.accountNumber, createOrderSubscriptionOwnerAccount.accountNumber) &&
Objects.equals(this.autoPay, createOrderSubscriptionOwnerAccount.autoPay) &&
Objects.equals(this.batch, createOrderSubscriptionOwnerAccount.batch) &&
Objects.equals(this.billCycleDay, createOrderSubscriptionOwnerAccount.billCycleDay) &&
Objects.equals(this.billToContact, createOrderSubscriptionOwnerAccount.billToContact) &&
Objects.equals(this.communicationProfileId, createOrderSubscriptionOwnerAccount.communicationProfileId) &&
Objects.equals(this.creditCard, createOrderSubscriptionOwnerAccount.creditCard) &&
Objects.equals(this.crmId, createOrderSubscriptionOwnerAccount.crmId) &&
Objects.equals(this.currency, createOrderSubscriptionOwnerAccount.currency) &&
Objects.equals(this.customFields, createOrderSubscriptionOwnerAccount.customFields) &&
Objects.equals(this.hpmCreditCardPaymentMethodId, createOrderSubscriptionOwnerAccount.hpmCreditCardPaymentMethodId) &&
Objects.equals(this.invoiceDeliveryPrefsEmail, createOrderSubscriptionOwnerAccount.invoiceDeliveryPrefsEmail) &&
Objects.equals(this.invoiceDeliveryPrefsPrint, createOrderSubscriptionOwnerAccount.invoiceDeliveryPrefsPrint) &&
Objects.equals(this.invoiceTemplateId, createOrderSubscriptionOwnerAccount.invoiceTemplateId) &&
Objects.equals(this.name, createOrderSubscriptionOwnerAccount.name) &&
Objects.equals(this.notes, createOrderSubscriptionOwnerAccount.notes) &&
Objects.equals(this.parentId, createOrderSubscriptionOwnerAccount.parentId) &&
Objects.equals(this.paymentGateway, createOrderSubscriptionOwnerAccount.paymentGateway) &&
Objects.equals(this.paymentMethod, createOrderSubscriptionOwnerAccount.paymentMethod) &&
Objects.equals(this.paymentTerm, createOrderSubscriptionOwnerAccount.paymentTerm) &&
Objects.equals(this.soldToContact, createOrderSubscriptionOwnerAccount.soldToContact) &&
Objects.equals(this.taxInfo, createOrderSubscriptionOwnerAccount.taxInfo)&&
Objects.equals(this.additionalProperties, createOrderSubscriptionOwnerAccount.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(accountNumber, autoPay, batch, billCycleDay, billToContact, communicationProfileId, creditCard, crmId, currency, customFields, hpmCreditCardPaymentMethodId, invoiceDeliveryPrefsEmail, invoiceDeliveryPrefsPrint, invoiceTemplateId, name, notes, parentId, paymentGateway, paymentMethod, paymentTerm, soldToContact, taxInfo, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateOrderSubscriptionOwnerAccount {\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" autoPay: ").append(toIndentedString(autoPay)).append("\n");
sb.append(" batch: ").append(toIndentedString(batch)).append("\n");
sb.append(" billCycleDay: ").append(toIndentedString(billCycleDay)).append("\n");
sb.append(" billToContact: ").append(toIndentedString(billToContact)).append("\n");
sb.append(" communicationProfileId: ").append(toIndentedString(communicationProfileId)).append("\n");
sb.append(" creditCard: ").append(toIndentedString(creditCard)).append("\n");
sb.append(" crmId: ").append(toIndentedString(crmId)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" hpmCreditCardPaymentMethodId: ").append(toIndentedString(hpmCreditCardPaymentMethodId)).append("\n");
sb.append(" invoiceDeliveryPrefsEmail: ").append(toIndentedString(invoiceDeliveryPrefsEmail)).append("\n");
sb.append(" invoiceDeliveryPrefsPrint: ").append(toIndentedString(invoiceDeliveryPrefsPrint)).append("\n");
sb.append(" invoiceTemplateId: ").append(toIndentedString(invoiceTemplateId)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" notes: ").append(toIndentedString(notes)).append("\n");
sb.append(" parentId: ").append(toIndentedString(parentId)).append("\n");
sb.append(" paymentGateway: ").append(toIndentedString(paymentGateway)).append("\n");
sb.append(" paymentMethod: ").append(toIndentedString(paymentMethod)).append("\n");
sb.append(" paymentTerm: ").append(toIndentedString(paymentTerm)).append("\n");
sb.append(" soldToContact: ").append(toIndentedString(soldToContact)).append("\n");
sb.append(" taxInfo: ").append(toIndentedString(taxInfo)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("accountNumber");
openapiFields.add("autoPay");
openapiFields.add("batch");
openapiFields.add("billCycleDay");
openapiFields.add("billToContact");
openapiFields.add("communicationProfileId");
openapiFields.add("creditCard");
openapiFields.add("crmId");
openapiFields.add("currency");
openapiFields.add("customFields");
openapiFields.add("hpmCreditCardPaymentMethodId");
openapiFields.add("invoiceDeliveryPrefsEmail");
openapiFields.add("invoiceDeliveryPrefsPrint");
openapiFields.add("invoiceTemplateId");
openapiFields.add("name");
openapiFields.add("notes");
openapiFields.add("parentId");
openapiFields.add("paymentGateway");
openapiFields.add("paymentMethod");
openapiFields.add("paymentTerm");
openapiFields.add("soldToContact");
openapiFields.add("taxInfo");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("billCycleDay");
openapiRequiredFields.add("billToContact");
openapiRequiredFields.add("currency");
openapiRequiredFields.add("name");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreateOrderSubscriptionOwnerAccount
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreateOrderSubscriptionOwnerAccount.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreateOrderSubscriptionOwnerAccount is not found in the empty JSON string", CreateOrderSubscriptionOwnerAccount.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : CreateOrderSubscriptionOwnerAccount.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("accountNumber") != null && !jsonObj.get("accountNumber").isJsonNull()) && !jsonObj.get("accountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountNumber").toString()));
}
if ((jsonObj.get("batch") != null && !jsonObj.get("batch").isJsonNull()) && !jsonObj.get("batch").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `batch` to be a primitive type in the JSON string but got `%s`", jsonObj.get("batch").toString()));
}
// validate the required field `billToContact`
CreateAccountContact.validateJsonElement(jsonObj.get("billToContact"));
if ((jsonObj.get("communicationProfileId") != null && !jsonObj.get("communicationProfileId").isJsonNull()) && !jsonObj.get("communicationProfileId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `communicationProfileId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("communicationProfileId").toString()));
}
// validate the optional field `creditCard`
if (jsonObj.get("creditCard") != null && !jsonObj.get("creditCard").isJsonNull()) {
CreditCard.validateJsonElement(jsonObj.get("creditCard"));
}
if ((jsonObj.get("crmId") != null && !jsonObj.get("crmId").isJsonNull()) && !jsonObj.get("crmId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `crmId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("crmId").toString()));
}
if (!jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("hpmCreditCardPaymentMethodId") != null && !jsonObj.get("hpmCreditCardPaymentMethodId").isJsonNull()) && !jsonObj.get("hpmCreditCardPaymentMethodId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `hpmCreditCardPaymentMethodId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("hpmCreditCardPaymentMethodId").toString()));
}
if ((jsonObj.get("invoiceTemplateId") != null && !jsonObj.get("invoiceTemplateId").isJsonNull()) && !jsonObj.get("invoiceTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceTemplateId").toString()));
}
if (!jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if ((jsonObj.get("notes") != null && !jsonObj.get("notes").isJsonNull()) && !jsonObj.get("notes").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `notes` to be a primitive type in the JSON string but got `%s`", jsonObj.get("notes").toString()));
}
if ((jsonObj.get("parentId") != null && !jsonObj.get("parentId").isJsonNull()) && !jsonObj.get("parentId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `parentId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("parentId").toString()));
}
if ((jsonObj.get("paymentGateway") != null && !jsonObj.get("paymentGateway").isJsonNull()) && !jsonObj.get("paymentGateway").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentGateway` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentGateway").toString()));
}
// validate the optional field `paymentMethod`
if (jsonObj.get("paymentMethod") != null && !jsonObj.get("paymentMethod").isJsonNull()) {
CreateAccountPaymentMethod.validateJsonElement(jsonObj.get("paymentMethod"));
}
if ((jsonObj.get("paymentTerm") != null && !jsonObj.get("paymentTerm").isJsonNull()) && !jsonObj.get("paymentTerm").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentTerm` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentTerm").toString()));
}
// validate the optional field `soldToContact`
if (jsonObj.get("soldToContact") != null && !jsonObj.get("soldToContact").isJsonNull()) {
CreateAccountContact.validateJsonElement(jsonObj.get("soldToContact"));
}
// validate the optional field `taxInfo`
if (jsonObj.get("taxInfo") != null && !jsonObj.get("taxInfo").isJsonNull()) {
TaxInfo.validateJsonElement(jsonObj.get("taxInfo"));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreateOrderSubscriptionOwnerAccount.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreateOrderSubscriptionOwnerAccount' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreateOrderSubscriptionOwnerAccount.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreateOrderSubscriptionOwnerAccount value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreateOrderSubscriptionOwnerAccount read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreateOrderSubscriptionOwnerAccount instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreateOrderSubscriptionOwnerAccount given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreateOrderSubscriptionOwnerAccount
* @throws IOException if the JSON string is invalid with respect to CreateOrderSubscriptionOwnerAccount
*/
public static CreateOrderSubscriptionOwnerAccount fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreateOrderSubscriptionOwnerAccount.class);
}
/**
* Convert an instance of CreateOrderSubscriptionOwnerAccount to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy