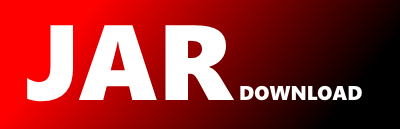
com.zuora.model.CreatePaymentMethodDecryptionRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.CreatePaymentMethodCardholderInfo;
import com.zuora.model.StoredCredentialProfileAction;
import com.zuora.model.StoredCredentialProfileConsentAgreementSrc;
import com.zuora.model.StoredCredentialProfileType;
import java.io.IOException;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* CreatePaymentMethodDecryptionRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreatePaymentMethodDecryptionRequest {
public static final String SERIALIZED_NAME_ACCOUNT_I_D = "accountID";
@SerializedName(SERIALIZED_NAME_ACCOUNT_I_D)
private String accountID;
public static final String SERIALIZED_NAME_CARD_HOLDER_INFO = "cardHolderInfo";
@SerializedName(SERIALIZED_NAME_CARD_HOLDER_INFO)
private CreatePaymentMethodCardholderInfo cardHolderInfo;
public static final String SERIALIZED_NAME_INTEGRATION_TYPE = "integrationType";
@SerializedName(SERIALIZED_NAME_INTEGRATION_TYPE)
private String integrationType;
public static final String SERIALIZED_NAME_INVOICE_ID = "invoiceId";
@SerializedName(SERIALIZED_NAME_INVOICE_ID)
private String invoiceId;
public static final String SERIALIZED_NAME_MERCHANT_I_D = "merchantID";
@SerializedName(SERIALIZED_NAME_MERCHANT_I_D)
private String merchantID;
public static final String SERIALIZED_NAME_MIT_CONSENT_AGREEMENT_SRC = "mitConsentAgreementSrc";
@SerializedName(SERIALIZED_NAME_MIT_CONSENT_AGREEMENT_SRC)
private StoredCredentialProfileConsentAgreementSrc mitConsentAgreementSrc;
public static final String SERIALIZED_NAME_MIT_PROFILE_ACTION = "mitProfileAction";
@SerializedName(SERIALIZED_NAME_MIT_PROFILE_ACTION)
private StoredCredentialProfileAction mitProfileAction;
public static final String SERIALIZED_NAME_MIT_PROFILE_TYPE = "mitProfileType";
@SerializedName(SERIALIZED_NAME_MIT_PROFILE_TYPE)
private StoredCredentialProfileType mitProfileType;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY = "paymentGateway";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY)
private String paymentGateway;
public static final String SERIALIZED_NAME_PAYMENT_TOKEN = "paymentToken";
@SerializedName(SERIALIZED_NAME_PAYMENT_TOKEN)
private Object paymentToken;
public static final String SERIALIZED_NAME_PROCESS_PAYMENT = "processPayment";
@SerializedName(SERIALIZED_NAME_PROCESS_PAYMENT)
private Boolean processPayment;
public CreatePaymentMethodDecryptionRequest() {
}
public CreatePaymentMethodDecryptionRequest accountID(String accountID) {
this.accountID = accountID;
return this;
}
/**
* The ID of the customer account associated with this payment method. To create an orphan payment method that is not associated with any customer account, you do not need to specify this field during creation. However, you must associate the orphan payment method with a customer account within 10 days. Otherwise, this orphan payment method will be deleted.
* @return accountID
*/
@javax.annotation.Nullable
public String getAccountID() {
return accountID;
}
public void setAccountID(String accountID) {
this.accountID = accountID;
}
public CreatePaymentMethodDecryptionRequest cardHolderInfo(CreatePaymentMethodCardholderInfo cardHolderInfo) {
this.cardHolderInfo = cardHolderInfo;
return this;
}
/**
* Get cardHolderInfo
* @return cardHolderInfo
*/
@javax.annotation.Nullable
public CreatePaymentMethodCardholderInfo getCardHolderInfo() {
return cardHolderInfo;
}
public void setCardHolderInfo(CreatePaymentMethodCardholderInfo cardHolderInfo) {
this.cardHolderInfo = cardHolderInfo;
}
public CreatePaymentMethodDecryptionRequest integrationType(String integrationType) {
this.integrationType = integrationType;
return this;
}
/**
* Field to identify the token decryption type. **Note:** The only value at this time is `ApplePay`.
* @return integrationType
*/
@javax.annotation.Nonnull
public String getIntegrationType() {
return integrationType;
}
public void setIntegrationType(String integrationType) {
this.integrationType = integrationType;
}
public CreatePaymentMethodDecryptionRequest invoiceId(String invoiceId) {
this.invoiceId = invoiceId;
return this;
}
/**
* The id of invoice this payment will apply to. **Note:** When `processPayment` is `true`, this field is required. Only one invoice can be paid; for scenarios where you want to pay for multiple invoices, set `processPayment` to `false` and call payment API separately.
* @return invoiceId
*/
@javax.annotation.Nullable
public String getInvoiceId() {
return invoiceId;
}
public void setInvoiceId(String invoiceId) {
this.invoiceId = invoiceId;
}
public CreatePaymentMethodDecryptionRequest merchantID(String merchantID) {
this.merchantID = merchantID;
return this;
}
/**
* The Merchant ID that was configured for use with Apple Pay in the Apple iOS Developer Center.
* @return merchantID
*/
@javax.annotation.Nonnull
public String getMerchantID() {
return merchantID;
}
public void setMerchantID(String merchantID) {
this.merchantID = merchantID;
}
public CreatePaymentMethodDecryptionRequest mitConsentAgreementSrc(StoredCredentialProfileConsentAgreementSrc mitConsentAgreementSrc) {
this.mitConsentAgreementSrc = mitConsentAgreementSrc;
return this;
}
/**
* Get mitConsentAgreementSrc
* @return mitConsentAgreementSrc
*/
@javax.annotation.Nullable
public StoredCredentialProfileConsentAgreementSrc getMitConsentAgreementSrc() {
return mitConsentAgreementSrc;
}
public void setMitConsentAgreementSrc(StoredCredentialProfileConsentAgreementSrc mitConsentAgreementSrc) {
this.mitConsentAgreementSrc = mitConsentAgreementSrc;
}
public CreatePaymentMethodDecryptionRequest mitProfileAction(StoredCredentialProfileAction mitProfileAction) {
this.mitProfileAction = mitProfileAction;
return this;
}
/**
* Get mitProfileAction
* @return mitProfileAction
*/
@javax.annotation.Nullable
public StoredCredentialProfileAction getMitProfileAction() {
return mitProfileAction;
}
public void setMitProfileAction(StoredCredentialProfileAction mitProfileAction) {
this.mitProfileAction = mitProfileAction;
}
public CreatePaymentMethodDecryptionRequest mitProfileType(StoredCredentialProfileType mitProfileType) {
this.mitProfileType = mitProfileType;
return this;
}
/**
* Get mitProfileType
* @return mitProfileType
*/
@javax.annotation.Nullable
public StoredCredentialProfileType getMitProfileType() {
return mitProfileType;
}
public void setMitProfileType(StoredCredentialProfileType mitProfileType) {
this.mitProfileType = mitProfileType;
}
public CreatePaymentMethodDecryptionRequest paymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
return this;
}
/**
* The label name of the gateway instance configured in Zuora that should process the payment. When creating a Payment, this must be a valid gateway instance ID and this gateway must support the specific payment method. If not specified, the default gateway of your Zuora customer account will be used. **Note:** When `processPayment` is `true`, this field is required. When `processPayment` is `false`, the default payment gateway of your Zuora customer account will be used no matter whether a payment gateway instance is specified in the `paymentGateway` field.
* @return paymentGateway
*/
@javax.annotation.Nullable
public String getPaymentGateway() {
return paymentGateway;
}
public void setPaymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
}
public CreatePaymentMethodDecryptionRequest paymentToken(Object paymentToken) {
this.paymentToken = paymentToken;
return this;
}
/**
* The complete JSON Object representing the encrypted payment token payload returned in the response from the Apple Pay session.
* @return paymentToken
*/
@javax.annotation.Nonnull
public Object getPaymentToken() {
return paymentToken;
}
public void setPaymentToken(Object paymentToken) {
this.paymentToken = paymentToken;
}
public CreatePaymentMethodDecryptionRequest processPayment(Boolean processPayment) {
this.processPayment = processPayment;
return this;
}
/**
* A boolean flag to control whether a payment should be processed after creating payment method. The payment amount will be equivalent to the amount the merchant supplied in the ApplePay session. Default is false. If this field is set to `true`, you must specify the `paymentGateway` field with the payment gateway instance name. If this field is set to `false`: - The default payment gateway of your Zuora customer account will be used no matter whether a payment gateway instance is specified in the `paymentGateway` field. - You must select the **Verify new credit card** check box on the gateway instance settings page. Otherwise, the cryptogram will not be sent to the gateway. - A separate subscribe or payment API call is required after this payment method creation call.
* @return processPayment
*/
@javax.annotation.Nullable
public Boolean getProcessPayment() {
return processPayment;
}
public void setProcessPayment(Boolean processPayment) {
this.processPayment = processPayment;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreatePaymentMethodDecryptionRequest instance itself
*/
public CreatePaymentMethodDecryptionRequest putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreatePaymentMethodDecryptionRequest createPaymentMethodDecryptionRequest = (CreatePaymentMethodDecryptionRequest) o;
return Objects.equals(this.accountID, createPaymentMethodDecryptionRequest.accountID) &&
Objects.equals(this.cardHolderInfo, createPaymentMethodDecryptionRequest.cardHolderInfo) &&
Objects.equals(this.integrationType, createPaymentMethodDecryptionRequest.integrationType) &&
Objects.equals(this.invoiceId, createPaymentMethodDecryptionRequest.invoiceId) &&
Objects.equals(this.merchantID, createPaymentMethodDecryptionRequest.merchantID) &&
Objects.equals(this.mitConsentAgreementSrc, createPaymentMethodDecryptionRequest.mitConsentAgreementSrc) &&
Objects.equals(this.mitProfileAction, createPaymentMethodDecryptionRequest.mitProfileAction) &&
Objects.equals(this.mitProfileType, createPaymentMethodDecryptionRequest.mitProfileType) &&
Objects.equals(this.paymentGateway, createPaymentMethodDecryptionRequest.paymentGateway) &&
Objects.equals(this.paymentToken, createPaymentMethodDecryptionRequest.paymentToken) &&
Objects.equals(this.processPayment, createPaymentMethodDecryptionRequest.processPayment)&&
Objects.equals(this.additionalProperties, createPaymentMethodDecryptionRequest.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(accountID, cardHolderInfo, integrationType, invoiceId, merchantID, mitConsentAgreementSrc, mitProfileAction, mitProfileType, paymentGateway, paymentToken, processPayment, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreatePaymentMethodDecryptionRequest {\n");
sb.append(" accountID: ").append(toIndentedString(accountID)).append("\n");
sb.append(" cardHolderInfo: ").append(toIndentedString(cardHolderInfo)).append("\n");
sb.append(" integrationType: ").append(toIndentedString(integrationType)).append("\n");
sb.append(" invoiceId: ").append(toIndentedString(invoiceId)).append("\n");
sb.append(" merchantID: ").append(toIndentedString(merchantID)).append("\n");
sb.append(" mitConsentAgreementSrc: ").append(toIndentedString(mitConsentAgreementSrc)).append("\n");
sb.append(" mitProfileAction: ").append(toIndentedString(mitProfileAction)).append("\n");
sb.append(" mitProfileType: ").append(toIndentedString(mitProfileType)).append("\n");
sb.append(" paymentGateway: ").append(toIndentedString(paymentGateway)).append("\n");
sb.append(" paymentToken: ").append(toIndentedString(paymentToken)).append("\n");
sb.append(" processPayment: ").append(toIndentedString(processPayment)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("accountID");
openapiFields.add("cardHolderInfo");
openapiFields.add("integrationType");
openapiFields.add("invoiceId");
openapiFields.add("merchantID");
openapiFields.add("mitConsentAgreementSrc");
openapiFields.add("mitProfileAction");
openapiFields.add("mitProfileType");
openapiFields.add("paymentGateway");
openapiFields.add("paymentToken");
openapiFields.add("processPayment");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("integrationType");
openapiRequiredFields.add("merchantID");
openapiRequiredFields.add("paymentToken");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreatePaymentMethodDecryptionRequest
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreatePaymentMethodDecryptionRequest.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreatePaymentMethodDecryptionRequest is not found in the empty JSON string", CreatePaymentMethodDecryptionRequest.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : CreatePaymentMethodDecryptionRequest.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("accountID") != null && !jsonObj.get("accountID").isJsonNull()) && !jsonObj.get("accountID").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountID` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountID").toString()));
}
// validate the optional field `cardHolderInfo`
if (jsonObj.get("cardHolderInfo") != null && !jsonObj.get("cardHolderInfo").isJsonNull()) {
CreatePaymentMethodCardholderInfo.validateJsonElement(jsonObj.get("cardHolderInfo"));
}
if (!jsonObj.get("integrationType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `integrationType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("integrationType").toString()));
}
if ((jsonObj.get("invoiceId") != null && !jsonObj.get("invoiceId").isJsonNull()) && !jsonObj.get("invoiceId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceId").toString()));
}
if (!jsonObj.get("merchantID").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `merchantID` to be a primitive type in the JSON string but got `%s`", jsonObj.get("merchantID").toString()));
}
if ((jsonObj.get("mitConsentAgreementSrc") != null && !jsonObj.get("mitConsentAgreementSrc").isJsonNull()) && !jsonObj.get("mitConsentAgreementSrc").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mitConsentAgreementSrc` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mitConsentAgreementSrc").toString()));
}
// validate the optional field `mitConsentAgreementSrc`
if (jsonObj.get("mitConsentAgreementSrc") != null && !jsonObj.get("mitConsentAgreementSrc").isJsonNull()) {
StoredCredentialProfileConsentAgreementSrc.validateJsonElement(jsonObj.get("mitConsentAgreementSrc"));
}
if ((jsonObj.get("mitProfileAction") != null && !jsonObj.get("mitProfileAction").isJsonNull()) && !jsonObj.get("mitProfileAction").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mitProfileAction` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mitProfileAction").toString()));
}
// validate the optional field `mitProfileAction`
if (jsonObj.get("mitProfileAction") != null && !jsonObj.get("mitProfileAction").isJsonNull()) {
StoredCredentialProfileAction.validateJsonElement(jsonObj.get("mitProfileAction"));
}
if ((jsonObj.get("mitProfileType") != null && !jsonObj.get("mitProfileType").isJsonNull()) && !jsonObj.get("mitProfileType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mitProfileType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mitProfileType").toString()));
}
// validate the optional field `mitProfileType`
if (jsonObj.get("mitProfileType") != null && !jsonObj.get("mitProfileType").isJsonNull()) {
StoredCredentialProfileType.validateJsonElement(jsonObj.get("mitProfileType"));
}
if ((jsonObj.get("paymentGateway") != null && !jsonObj.get("paymentGateway").isJsonNull()) && !jsonObj.get("paymentGateway").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentGateway` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentGateway").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreatePaymentMethodDecryptionRequest.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreatePaymentMethodDecryptionRequest' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreatePaymentMethodDecryptionRequest.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreatePaymentMethodDecryptionRequest value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreatePaymentMethodDecryptionRequest read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreatePaymentMethodDecryptionRequest instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreatePaymentMethodDecryptionRequest given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreatePaymentMethodDecryptionRequest
* @throws IOException if the JSON string is invalid with respect to CreatePaymentMethodDecryptionRequest
*/
public static CreatePaymentMethodDecryptionRequest fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreatePaymentMethodDecryptionRequest.class);
}
/**
* Convert an instance of CreatePaymentMethodDecryptionRequest to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy