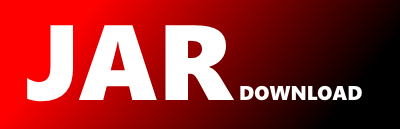
com.zuora.model.CreatePaymentRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.CreatePaymentDebitMemoApplication;
import com.zuora.model.CreatePaymentInvoiceApplication;
import com.zuora.model.GatewayOptions;
import com.zuora.model.MitTransactionSource;
import com.zuora.model.PaymentRequestFinanceInformation;
import com.zuora.model.PaymentSchedulePaymentOptionFields;
import com.zuora.model.PaymentType;
import com.zuora.model.PaymentWithCustomRates;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* CreatePaymentRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreatePaymentRequest {
public static final String SERIALIZED_NAME_ACCOUNT_ID = "accountId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "accountNumber";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private Double amount;
public static final String SERIALIZED_NAME_AUTH_TRANSACTION_ID = "authTransactionId";
@SerializedName(SERIALIZED_NAME_AUTH_TRANSACTION_ID)
private String authTransactionId;
public static final String SERIALIZED_NAME_COMMENT = "comment";
@SerializedName(SERIALIZED_NAME_COMMENT)
private String comment;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_CUSTOM_RATES = "customRates";
@SerializedName(SERIALIZED_NAME_CUSTOM_RATES)
private List customRates;
public static final String SERIALIZED_NAME_DEBIT_MEMOS = "debitMemos";
@SerializedName(SERIALIZED_NAME_DEBIT_MEMOS)
private List debitMemos;
public static final String SERIALIZED_NAME_EFFECTIVE_DATE = "effectiveDate";
@SerializedName(SERIALIZED_NAME_EFFECTIVE_DATE)
private String effectiveDate;
public static final String SERIALIZED_NAME_FINANCE_INFORMATION = "financeInformation";
@SerializedName(SERIALIZED_NAME_FINANCE_INFORMATION)
private PaymentRequestFinanceInformation financeInformation;
public static final String SERIALIZED_NAME_GATEWAY_ID = "gatewayId";
@SerializedName(SERIALIZED_NAME_GATEWAY_ID)
private String gatewayId;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY_NUMBER = "paymentGatewayNumber";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY_NUMBER)
private String paymentGatewayNumber;
public static final String SERIALIZED_NAME_GATEWAY_OPTIONS = "gatewayOptions";
@SerializedName(SERIALIZED_NAME_GATEWAY_OPTIONS)
private GatewayOptions gatewayOptions;
public static final String SERIALIZED_NAME_GATEWAY_ORDER_ID = "gatewayOrderId";
@SerializedName(SERIALIZED_NAME_GATEWAY_ORDER_ID)
private String gatewayOrderId;
public static final String SERIALIZED_NAME_INVOICES = "invoices";
@SerializedName(SERIALIZED_NAME_INVOICES)
private List invoices;
public static final String SERIALIZED_NAME_MIT_TRANSACTION_SOURCE = "mitTransactionSource";
@SerializedName(SERIALIZED_NAME_MIT_TRANSACTION_SOURCE)
private MitTransactionSource mitTransactionSource;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "paymentMethodId";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_TYPE = "paymentMethodType";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_TYPE)
private String paymentMethodType;
public static final String SERIALIZED_NAME_PAYMENT_OPTION = "paymentOption";
@SerializedName(SERIALIZED_NAME_PAYMENT_OPTION)
private List paymentOption;
public static final String SERIALIZED_NAME_PAYMENT_SCHEDULE_KEY = "paymentScheduleKey";
@SerializedName(SERIALIZED_NAME_PAYMENT_SCHEDULE_KEY)
private String paymentScheduleKey;
public static final String SERIALIZED_NAME_PREPAYMENT = "prepayment";
@SerializedName(SERIALIZED_NAME_PREPAYMENT)
private Boolean prepayment;
public static final String SERIALIZED_NAME_REFERENCE_ID = "referenceId";
@SerializedName(SERIALIZED_NAME_REFERENCE_ID)
private String referenceId;
public static final String SERIALIZED_NAME_SOFT_DESCRIPTOR = "softDescriptor";
@SerializedName(SERIALIZED_NAME_SOFT_DESCRIPTOR)
private String softDescriptor;
public static final String SERIALIZED_NAME_SOFT_DESCRIPTOR_PHONE = "softDescriptorPhone";
@SerializedName(SERIALIZED_NAME_SOFT_DESCRIPTOR_PHONE)
private String softDescriptorPhone;
public static final String SERIALIZED_NAME_STANDALONE = "standalone";
@SerializedName(SERIALIZED_NAME_STANDALONE)
private Boolean standalone;
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private PaymentType type;
public CreatePaymentRequest() {
}
public CreatePaymentRequest accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* The ID of the customer account that the payment is created for.
* @return accountId
*/
@javax.annotation.Nullable
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public CreatePaymentRequest accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* The number of the customer account that the payment is created for, such as `A00000001`. You can specify either `accountNumber` or `accountId` for a customer account. If both of them are specified, they must refer to the same customer account.
* @return accountNumber
*/
@javax.annotation.Nullable
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public CreatePaymentRequest amount(Double amount) {
this.amount = amount;
return this;
}
/**
* The total amount of the payment.
* @return amount
*/
@javax.annotation.Nonnull
public Double getAmount() {
return amount;
}
public void setAmount(Double amount) {
this.amount = amount;
}
public CreatePaymentRequest authTransactionId(String authTransactionId) {
this.authTransactionId = authTransactionId;
return this;
}
/**
* The authorization transaction ID from the payment gateway. Use this field for electronic payments, such as credit cards. When you create a payment for capturing the authorized funds, it is highly recommended to pass in the gatewayOrderId that you used when authorizing the funds by using the [Create authorization](https://www.zuora.com/developer/api-references/api/operation/Post_CreateAuthorization) operation, together with the `authTransactionId` field. The following payment gateways support this field: - Adyen Integration v2.0 - CyberSource 1.28 - CyberSource 1.97 - CyberSource 2.0 - Chase Paymentech Orbital - Ingenico ePayments - SlimPay - Stripe v2 - Verifi Global Payment Gateway - WePay Payment Gateway Integration
* @return authTransactionId
*/
@javax.annotation.Nullable
public String getAuthTransactionId() {
return authTransactionId;
}
public void setAuthTransactionId(String authTransactionId) {
this.authTransactionId = authTransactionId;
}
public CreatePaymentRequest comment(String comment) {
this.comment = comment;
return this;
}
/**
* Additional information related to the payment.
* @return comment
*/
@javax.annotation.Nullable
public String getComment() {
return comment;
}
public void setComment(String comment) {
this.comment = comment;
}
public CreatePaymentRequest currency(String currency) {
this.currency = currency;
return this;
}
/**
* When Standalone Payment is not enabled, the `currency` of the payment must be the same as the payment currency defined in the customer account settings through Zuora UI. When Standalone Payment is enabled and `standalone` is `true`, the `currency` of the standalone payment can be different from the payment currency defined in the customer account settings. The amount will not be summed up to the account balance or key metrics regardless of currency.
* @return currency
*/
@javax.annotation.Nonnull
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public CreatePaymentRequest customRates(List customRates) {
this.customRates = customRates;
return this;
}
public CreatePaymentRequest addCustomRatesItem(PaymentWithCustomRates customRatesItem) {
if (this.customRates == null) {
this.customRates = new ArrayList<>();
}
this.customRates.add(customRatesItem);
return this;
}
/**
* It contains Home currency and Reporting currency custom rates currencies. The maximum number of items is 2 (you can pass the Home currency item or Reporting currency item or both). **Note**: The API custom rate feature is permission controlled.
* @return customRates
*/
@javax.annotation.Nullable
public List getCustomRates() {
return customRates;
}
public void setCustomRates(List customRates) {
this.customRates = customRates;
}
public CreatePaymentRequest debitMemos(List debitMemos) {
this.debitMemos = debitMemos;
return this;
}
public CreatePaymentRequest addDebitMemosItem(CreatePaymentDebitMemoApplication debitMemosItem) {
if (this.debitMemos == null) {
this.debitMemos = new ArrayList<>();
}
this.debitMemos.add(debitMemosItem);
return this;
}
/**
* Container for debit memos. The maximum number of debit memos is 1,000.
* @return debitMemos
*/
@javax.annotation.Nullable
public List getDebitMemos() {
return debitMemos;
}
public void setDebitMemos(List debitMemos) {
this.debitMemos = debitMemos;
}
public CreatePaymentRequest effectiveDate(String effectiveDate) {
this.effectiveDate = effectiveDate;
return this;
}
/**
* The date when the payment takes effect, in `yyyy-mm-dd` format. **Note:** - This field is required for only electronic payments. It's an optional field for external payments. - When specified, this field must be set to the date of today.
* @return effectiveDate
*/
@javax.annotation.Nullable
public String getEffectiveDate() {
return effectiveDate;
}
public void setEffectiveDate(String effectiveDate) {
this.effectiveDate = effectiveDate;
}
public CreatePaymentRequest financeInformation(PaymentRequestFinanceInformation financeInformation) {
this.financeInformation = financeInformation;
return this;
}
/**
* Get financeInformation
* @return financeInformation
*/
@javax.annotation.Nullable
public PaymentRequestFinanceInformation getFinanceInformation() {
return financeInformation;
}
public void setFinanceInformation(PaymentRequestFinanceInformation financeInformation) {
this.financeInformation = financeInformation;
}
public CreatePaymentRequest gatewayId(String gatewayId) {
this.gatewayId = gatewayId;
return this;
}
/**
* The ID of the gateway instance that processes the payment. The ID must be a valid gateway instance ID and this gateway must support the specific payment method. - When creating electronic payments, this field is required. - When creating external payments, this field is optional.
* @return gatewayId
*/
@javax.annotation.Nullable
public String getGatewayId() {
return gatewayId;
}
public void setGatewayId(String gatewayId) {
this.gatewayId = gatewayId;
}
public CreatePaymentRequest paymentGatewayNumber(String paymentGatewayNumber) {
this.paymentGatewayNumber = paymentGatewayNumber;
return this;
}
/**
* The natural key for the payment gateway.
* @return paymentGatewayNumber
*/
@javax.annotation.Nullable
public String getPaymentGatewayNumber() {
return paymentGatewayNumber;
}
public void setPaymentGatewayNumber(String paymentGatewayNumber) {
this.paymentGatewayNumber = paymentGatewayNumber;
}
public CreatePaymentRequest gatewayOptions(GatewayOptions gatewayOptions) {
this.gatewayOptions = gatewayOptions;
return this;
}
/**
* Get gatewayOptions
* @return gatewayOptions
*/
@javax.annotation.Nullable
public GatewayOptions getGatewayOptions() {
return gatewayOptions;
}
public void setGatewayOptions(GatewayOptions gatewayOptions) {
this.gatewayOptions = gatewayOptions;
}
public CreatePaymentRequest gatewayOrderId(String gatewayOrderId) {
this.gatewayOrderId = gatewayOrderId;
return this;
}
/**
* A merchant-specified natural key value that can be passed to the electronic payment gateway when a payment is created. If not specified, the payment number will be passed in instead. Gateways check duplicates on the gateway order ID to ensure that the merchant do not accidentally enter the same transaction twice. This ID can also be used to do reconciliation and tie the payment to a natural key in external systems. The source of this ID varies by merchant. Some merchants use their shopping cart order IDs, and others use something different. Merchants use this ID to track transactions in their eCommerce systems. When you create a payment for capturing the authorized funds, it is highly recommended to pass in the gatewayOrderId that you used when authorizing the funds by using the [Create authorization](https://www.zuora.com/developer/api-references/api/operation/Post_CreateAuthorization) operation, together with the `authTransactionId` field.
* @return gatewayOrderId
*/
@javax.annotation.Nullable
public String getGatewayOrderId() {
return gatewayOrderId;
}
public void setGatewayOrderId(String gatewayOrderId) {
this.gatewayOrderId = gatewayOrderId;
}
public CreatePaymentRequest invoices(List invoices) {
this.invoices = invoices;
return this;
}
public CreatePaymentRequest addInvoicesItem(CreatePaymentInvoiceApplication invoicesItem) {
if (this.invoices == null) {
this.invoices = new ArrayList<>();
}
this.invoices.add(invoicesItem);
return this;
}
/**
* Container for invoices. The maximum number of invoices is 1,000.
* @return invoices
*/
@javax.annotation.Nullable
public List getInvoices() {
return invoices;
}
public void setInvoices(List invoices) {
this.invoices = invoices;
}
public CreatePaymentRequest mitTransactionSource(MitTransactionSource mitTransactionSource) {
this.mitTransactionSource = mitTransactionSource;
return this;
}
/**
* Get mitTransactionSource
* @return mitTransactionSource
*/
@javax.annotation.Nullable
public MitTransactionSource getMitTransactionSource() {
return mitTransactionSource;
}
public void setMitTransactionSource(MitTransactionSource mitTransactionSource) {
this.mitTransactionSource = mitTransactionSource;
}
public CreatePaymentRequest paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
return this;
}
/**
* The unique ID of the payment method that the customer used to make the payment. If no payment method ID is specified in the request body, the default payment method for the customer account is used automatically. If the default payment method is different from the type of payments that you want to create, an error occurs.
* @return paymentMethodId
*/
@javax.annotation.Nullable
public String getPaymentMethodId() {
return paymentMethodId;
}
public void setPaymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
}
public CreatePaymentRequest paymentMethodType(String paymentMethodType) {
this.paymentMethodType = paymentMethodType;
return this;
}
/**
* The type of the payment method that the customer used to make the payment. Specify this value when you are creating an external payment method. If both `paymentMethodType` and `paymentMethodId` are specified, only the `paymentMethodId` value is used to create the payment.
* @return paymentMethodType
*/
@javax.annotation.Nullable
public String getPaymentMethodType() {
return paymentMethodType;
}
public void setPaymentMethodType(String paymentMethodType) {
this.paymentMethodType = paymentMethodType;
}
public CreatePaymentRequest paymentOption(List paymentOption) {
this.paymentOption = paymentOption;
return this;
}
public CreatePaymentRequest addPaymentOptionItem(PaymentSchedulePaymentOptionFields paymentOptionItem) {
if (this.paymentOption == null) {
this.paymentOption = new ArrayList<>();
}
this.paymentOption.add(paymentOptionItem);
return this;
}
/**
* Container for the paymentOption items, which describe the transactional level rules for processing payments. Currently, only the Gateway Options type is supported. Here is an example: ``` \"paymentOption\": [ { \"type\": \"GatewayOptions\", \"detail\": { \"SecCode\":\"WEB\" } } ] ``` `paymentOption` of the payment schedule takes precedence over `paymentOption` of the payment schedule item. You can use this field or the `gatewayOptions` field to pass the Gateway Options fields supported by a payment gateway. However, the Gateway Options fields passed through the `paymentOption` field will be stored in the Payment Option object and can be easily retrieved. To enable this field, submit a request at [Zuora Global Support](https://support.zuora.com/). This field is only available if `zuora-version` is set to 337.0 or later.
* @return paymentOption
*/
@javax.annotation.Nullable
public List getPaymentOption() {
return paymentOption;
}
public void setPaymentOption(List paymentOption) {
this.paymentOption = paymentOption;
}
public CreatePaymentRequest paymentScheduleKey(String paymentScheduleKey) {
this.paymentScheduleKey = paymentScheduleKey;
return this;
}
/**
* The unique ID or the number of the payment schedule to be linked with the payment. See [Link payments to payment schedules](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Payment_Schedules/Link_payments_with_payment_schedules) for more information.
* @return paymentScheduleKey
*/
@javax.annotation.Nullable
public String getPaymentScheduleKey() {
return paymentScheduleKey;
}
public void setPaymentScheduleKey(String paymentScheduleKey) {
this.paymentScheduleKey = paymentScheduleKey;
}
public CreatePaymentRequest prepayment(Boolean prepayment) {
this.prepayment = prepayment;
return this;
}
/**
* Indicates whether the payment will be used as a reserved payment. See [Prepaid Cash with Drawdown](https://knowledgecenter.zuora.com/Zuora_Billing/Billing_and_Invoicing/JA_Advanced_Consumption_Billing/Prepaid_Cash_with_Drawdown) for more information.
* @return prepayment
*/
@javax.annotation.Nullable
public Boolean getPrepayment() {
return prepayment;
}
public void setPrepayment(Boolean prepayment) {
this.prepayment = prepayment;
}
public CreatePaymentRequest referenceId(String referenceId) {
this.referenceId = referenceId;
return this;
}
/**
* The transaction ID returned by the payment gateway. Use this field to reconcile payments between your gateway and Zuora Payments.
* @return referenceId
*/
@javax.annotation.Nullable
public String getReferenceId() {
return referenceId;
}
public void setReferenceId(String referenceId) {
this.referenceId = referenceId;
}
public CreatePaymentRequest softDescriptor(String softDescriptor) {
this.softDescriptor = softDescriptor;
return this;
}
/**
* A payment gateway-specific field that maps to Zuora for the gateways, Orbital, Vantiv and Verifi.
* @return softDescriptor
*/
@javax.annotation.Nullable
public String getSoftDescriptor() {
return softDescriptor;
}
public void setSoftDescriptor(String softDescriptor) {
this.softDescriptor = softDescriptor;
}
public CreatePaymentRequest softDescriptorPhone(String softDescriptorPhone) {
this.softDescriptorPhone = softDescriptorPhone;
return this;
}
/**
* A payment gateway-specific field that maps to Zuora for the gateways, Orbital, Vantiv and Verifi.
* @return softDescriptorPhone
*/
@javax.annotation.Nullable
public String getSoftDescriptorPhone() {
return softDescriptorPhone;
}
public void setSoftDescriptorPhone(String softDescriptorPhone) {
this.softDescriptorPhone = softDescriptorPhone;
}
public CreatePaymentRequest standalone(Boolean standalone) {
this.standalone = standalone;
return this;
}
/**
* This field is only available if support for standalone payments is enabled. Specify `true` to create a standalone payment that will be processed in Zuora through Zuora gateway integration but will be settled outside of Zuora. When `standalone` is set to `true`: - `accountId`, `amount`, `currency`, and `type` are required. - `type` must be `Electronic`. - `currency` of the payment can be different from the payment currency in the customer account settings. - The amount will not be summed up into the account balance and key metrics regardless of the payment currency. - No settlement data will be created. - Either the applied amount or the unapplied amount of the payment is zero. - The standalone payment cannot be applied, unapplied, or transferred. Specify `false` to create an ordinary payment that will be created, processed, and settled in Zuora. The `currency` of an ordinary payment must be the same as the currency in the customer account settings.
* @return standalone
*/
@javax.annotation.Nullable
public Boolean getStandalone() {
return standalone;
}
public void setStandalone(Boolean standalone) {
this.standalone = standalone;
}
public CreatePaymentRequest type(PaymentType type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
*/
@javax.annotation.Nonnull
public PaymentType getType() {
return type;
}
public void setType(PaymentType type) {
this.type = type;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreatePaymentRequest instance itself
*/
public CreatePaymentRequest putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreatePaymentRequest createPaymentRequest = (CreatePaymentRequest) o;
return Objects.equals(this.accountId, createPaymentRequest.accountId) &&
Objects.equals(this.accountNumber, createPaymentRequest.accountNumber) &&
Objects.equals(this.amount, createPaymentRequest.amount) &&
Objects.equals(this.authTransactionId, createPaymentRequest.authTransactionId) &&
Objects.equals(this.comment, createPaymentRequest.comment) &&
Objects.equals(this.currency, createPaymentRequest.currency) &&
Objects.equals(this.customRates, createPaymentRequest.customRates) &&
Objects.equals(this.debitMemos, createPaymentRequest.debitMemos) &&
Objects.equals(this.effectiveDate, createPaymentRequest.effectiveDate) &&
Objects.equals(this.financeInformation, createPaymentRequest.financeInformation) &&
Objects.equals(this.gatewayId, createPaymentRequest.gatewayId) &&
Objects.equals(this.paymentGatewayNumber, createPaymentRequest.paymentGatewayNumber) &&
Objects.equals(this.gatewayOptions, createPaymentRequest.gatewayOptions) &&
Objects.equals(this.gatewayOrderId, createPaymentRequest.gatewayOrderId) &&
Objects.equals(this.invoices, createPaymentRequest.invoices) &&
Objects.equals(this.mitTransactionSource, createPaymentRequest.mitTransactionSource) &&
Objects.equals(this.paymentMethodId, createPaymentRequest.paymentMethodId) &&
Objects.equals(this.paymentMethodType, createPaymentRequest.paymentMethodType) &&
Objects.equals(this.paymentOption, createPaymentRequest.paymentOption) &&
Objects.equals(this.paymentScheduleKey, createPaymentRequest.paymentScheduleKey) &&
Objects.equals(this.prepayment, createPaymentRequest.prepayment) &&
Objects.equals(this.referenceId, createPaymentRequest.referenceId) &&
Objects.equals(this.softDescriptor, createPaymentRequest.softDescriptor) &&
Objects.equals(this.softDescriptorPhone, createPaymentRequest.softDescriptorPhone) &&
Objects.equals(this.standalone, createPaymentRequest.standalone) &&
Objects.equals(this.type, createPaymentRequest.type)&&
Objects.equals(this.additionalProperties, createPaymentRequest.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(accountId, accountNumber, amount, authTransactionId, comment, currency, customRates, debitMemos, effectiveDate, financeInformation, gatewayId, paymentGatewayNumber, gatewayOptions, gatewayOrderId, invoices, mitTransactionSource, paymentMethodId, paymentMethodType, paymentOption, paymentScheduleKey, prepayment, referenceId, softDescriptor, softDescriptorPhone, standalone, type, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreatePaymentRequest {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" authTransactionId: ").append(toIndentedString(authTransactionId)).append("\n");
sb.append(" comment: ").append(toIndentedString(comment)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" customRates: ").append(toIndentedString(customRates)).append("\n");
sb.append(" debitMemos: ").append(toIndentedString(debitMemos)).append("\n");
sb.append(" effectiveDate: ").append(toIndentedString(effectiveDate)).append("\n");
sb.append(" financeInformation: ").append(toIndentedString(financeInformation)).append("\n");
sb.append(" gatewayId: ").append(toIndentedString(gatewayId)).append("\n");
sb.append(" paymentGatewayNumber: ").append(toIndentedString(paymentGatewayNumber)).append("\n");
sb.append(" gatewayOptions: ").append(toIndentedString(gatewayOptions)).append("\n");
sb.append(" gatewayOrderId: ").append(toIndentedString(gatewayOrderId)).append("\n");
sb.append(" invoices: ").append(toIndentedString(invoices)).append("\n");
sb.append(" mitTransactionSource: ").append(toIndentedString(mitTransactionSource)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" paymentMethodType: ").append(toIndentedString(paymentMethodType)).append("\n");
sb.append(" paymentOption: ").append(toIndentedString(paymentOption)).append("\n");
sb.append(" paymentScheduleKey: ").append(toIndentedString(paymentScheduleKey)).append("\n");
sb.append(" prepayment: ").append(toIndentedString(prepayment)).append("\n");
sb.append(" referenceId: ").append(toIndentedString(referenceId)).append("\n");
sb.append(" softDescriptor: ").append(toIndentedString(softDescriptor)).append("\n");
sb.append(" softDescriptorPhone: ").append(toIndentedString(softDescriptorPhone)).append("\n");
sb.append(" standalone: ").append(toIndentedString(standalone)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("accountId");
openapiFields.add("accountNumber");
openapiFields.add("amount");
openapiFields.add("authTransactionId");
openapiFields.add("comment");
openapiFields.add("currency");
openapiFields.add("customRates");
openapiFields.add("debitMemos");
openapiFields.add("effectiveDate");
openapiFields.add("financeInformation");
openapiFields.add("gatewayId");
openapiFields.add("paymentGatewayNumber");
openapiFields.add("gatewayOptions");
openapiFields.add("gatewayOrderId");
openapiFields.add("invoices");
openapiFields.add("mitTransactionSource");
openapiFields.add("paymentMethodId");
openapiFields.add("paymentMethodType");
openapiFields.add("paymentOption");
openapiFields.add("paymentScheduleKey");
openapiFields.add("prepayment");
openapiFields.add("referenceId");
openapiFields.add("softDescriptor");
openapiFields.add("softDescriptorPhone");
openapiFields.add("standalone");
openapiFields.add("type");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("amount");
openapiRequiredFields.add("currency");
openapiRequiredFields.add("type");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreatePaymentRequest
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreatePaymentRequest.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreatePaymentRequest is not found in the empty JSON string", CreatePaymentRequest.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : CreatePaymentRequest.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("accountId") != null && !jsonObj.get("accountId").isJsonNull()) && !jsonObj.get("accountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountId").toString()));
}
if ((jsonObj.get("accountNumber") != null && !jsonObj.get("accountNumber").isJsonNull()) && !jsonObj.get("accountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountNumber").toString()));
}
if ((jsonObj.get("authTransactionId") != null && !jsonObj.get("authTransactionId").isJsonNull()) && !jsonObj.get("authTransactionId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `authTransactionId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("authTransactionId").toString()));
}
if ((jsonObj.get("comment") != null && !jsonObj.get("comment").isJsonNull()) && !jsonObj.get("comment").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `comment` to be a primitive type in the JSON string but got `%s`", jsonObj.get("comment").toString()));
}
if (!jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if (jsonObj.get("customRates") != null && !jsonObj.get("customRates").isJsonNull()) {
JsonArray jsonArraycustomRates = jsonObj.getAsJsonArray("customRates");
if (jsonArraycustomRates != null) {
// ensure the json data is an array
if (!jsonObj.get("customRates").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `customRates` to be an array in the JSON string but got `%s`", jsonObj.get("customRates").toString()));
}
// validate the optional field `customRates` (array)
for (int i = 0; i < jsonArraycustomRates.size(); i++) {
PaymentWithCustomRates.validateJsonElement(jsonArraycustomRates.get(i));
};
}
}
if (jsonObj.get("debitMemos") != null && !jsonObj.get("debitMemos").isJsonNull()) {
JsonArray jsonArraydebitMemos = jsonObj.getAsJsonArray("debitMemos");
if (jsonArraydebitMemos != null) {
// ensure the json data is an array
if (!jsonObj.get("debitMemos").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `debitMemos` to be an array in the JSON string but got `%s`", jsonObj.get("debitMemos").toString()));
}
// validate the optional field `debitMemos` (array)
for (int i = 0; i < jsonArraydebitMemos.size(); i++) {
CreatePaymentDebitMemoApplication.validateJsonElement(jsonArraydebitMemos.get(i));
};
}
}
if ((jsonObj.get("effectiveDate") != null && !jsonObj.get("effectiveDate").isJsonNull()) && !jsonObj.get("effectiveDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `effectiveDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("effectiveDate").toString()));
}
// validate the optional field `financeInformation`
if (jsonObj.get("financeInformation") != null && !jsonObj.get("financeInformation").isJsonNull()) {
PaymentRequestFinanceInformation.validateJsonElement(jsonObj.get("financeInformation"));
}
if ((jsonObj.get("gatewayId") != null && !jsonObj.get("gatewayId").isJsonNull()) && !jsonObj.get("gatewayId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayId").toString()));
}
if ((jsonObj.get("paymentGatewayNumber") != null && !jsonObj.get("paymentGatewayNumber").isJsonNull()) && !jsonObj.get("paymentGatewayNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentGatewayNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentGatewayNumber").toString()));
}
// validate the optional field `gatewayOptions`
if (jsonObj.get("gatewayOptions") != null && !jsonObj.get("gatewayOptions").isJsonNull()) {
GatewayOptions.validateJsonElement(jsonObj.get("gatewayOptions"));
}
if ((jsonObj.get("gatewayOrderId") != null && !jsonObj.get("gatewayOrderId").isJsonNull()) && !jsonObj.get("gatewayOrderId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayOrderId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayOrderId").toString()));
}
if (jsonObj.get("invoices") != null && !jsonObj.get("invoices").isJsonNull()) {
JsonArray jsonArrayinvoices = jsonObj.getAsJsonArray("invoices");
if (jsonArrayinvoices != null) {
// ensure the json data is an array
if (!jsonObj.get("invoices").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `invoices` to be an array in the JSON string but got `%s`", jsonObj.get("invoices").toString()));
}
// validate the optional field `invoices` (array)
for (int i = 0; i < jsonArrayinvoices.size(); i++) {
CreatePaymentInvoiceApplication.validateJsonElement(jsonArrayinvoices.get(i));
};
}
}
if ((jsonObj.get("mitTransactionSource") != null && !jsonObj.get("mitTransactionSource").isJsonNull()) && !jsonObj.get("mitTransactionSource").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mitTransactionSource` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mitTransactionSource").toString()));
}
// validate the optional field `mitTransactionSource`
if (jsonObj.get("mitTransactionSource") != null && !jsonObj.get("mitTransactionSource").isJsonNull()) {
MitTransactionSource.validateJsonElement(jsonObj.get("mitTransactionSource"));
}
if ((jsonObj.get("paymentMethodId") != null && !jsonObj.get("paymentMethodId").isJsonNull()) && !jsonObj.get("paymentMethodId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentMethodId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentMethodId").toString()));
}
if ((jsonObj.get("paymentMethodType") != null && !jsonObj.get("paymentMethodType").isJsonNull()) && !jsonObj.get("paymentMethodType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentMethodType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentMethodType").toString()));
}
if (jsonObj.get("paymentOption") != null && !jsonObj.get("paymentOption").isJsonNull()) {
JsonArray jsonArraypaymentOption = jsonObj.getAsJsonArray("paymentOption");
if (jsonArraypaymentOption != null) {
// ensure the json data is an array
if (!jsonObj.get("paymentOption").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentOption` to be an array in the JSON string but got `%s`", jsonObj.get("paymentOption").toString()));
}
// validate the optional field `paymentOption` (array)
for (int i = 0; i < jsonArraypaymentOption.size(); i++) {
PaymentSchedulePaymentOptionFields.validateJsonElement(jsonArraypaymentOption.get(i));
};
}
}
if ((jsonObj.get("paymentScheduleKey") != null && !jsonObj.get("paymentScheduleKey").isJsonNull()) && !jsonObj.get("paymentScheduleKey").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentScheduleKey` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentScheduleKey").toString()));
}
if ((jsonObj.get("referenceId") != null && !jsonObj.get("referenceId").isJsonNull()) && !jsonObj.get("referenceId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `referenceId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("referenceId").toString()));
}
if ((jsonObj.get("softDescriptor") != null && !jsonObj.get("softDescriptor").isJsonNull()) && !jsonObj.get("softDescriptor").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `softDescriptor` to be a primitive type in the JSON string but got `%s`", jsonObj.get("softDescriptor").toString()));
}
if ((jsonObj.get("softDescriptorPhone") != null && !jsonObj.get("softDescriptorPhone").isJsonNull()) && !jsonObj.get("softDescriptorPhone").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `softDescriptorPhone` to be a primitive type in the JSON string but got `%s`", jsonObj.get("softDescriptorPhone").toString()));
}
if (!jsonObj.get("type").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `type` to be a primitive type in the JSON string but got `%s`", jsonObj.get("type").toString()));
}
// validate the required field `type`
PaymentType.validateJsonElement(jsonObj.get("type"));
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreatePaymentRequest.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreatePaymentRequest' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreatePaymentRequest.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreatePaymentRequest value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreatePaymentRequest read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreatePaymentRequest instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreatePaymentRequest given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreatePaymentRequest
* @throws IOException if the JSON string is invalid with respect to CreatePaymentRequest
*/
public static CreatePaymentRequest fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreatePaymentRequest.class);
}
/**
* Convert an instance of CreatePaymentRequest to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy