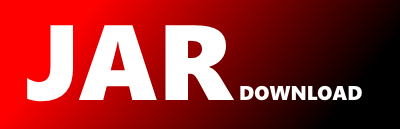
com.zuora.model.CreateRSASignatureRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* CreateRSASignatureRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreateRSASignatureRequest {
public static final String SERIALIZED_NAME_I_B_A_N = "IBAN";
@SerializedName(SERIALIZED_NAME_I_B_A_N)
private String IBAN;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "accountId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_AUTHORIZATION_AMOUNT = "authorizationAmount";
@SerializedName(SERIALIZED_NAME_AUTHORIZATION_AMOUNT)
private BigDecimal authorizationAmount;
public static final String SERIALIZED_NAME_BANK_BRANCH_CODE = "bankBranchCode";
@SerializedName(SERIALIZED_NAME_BANK_BRANCH_CODE)
private String bankBranchCode;
public static final String SERIALIZED_NAME_BANK_CHECK_DIGIT = "bankCheckDigit";
@SerializedName(SERIALIZED_NAME_BANK_CHECK_DIGIT)
private String bankCheckDigit;
public static final String SERIALIZED_NAME_BANK_CITY = "bankCity";
@SerializedName(SERIALIZED_NAME_BANK_CITY)
private String bankCity;
public static final String SERIALIZED_NAME_BANK_POSTAL_CODE = "bankPostalCode";
@SerializedName(SERIALIZED_NAME_BANK_POSTAL_CODE)
private String bankPostalCode;
public static final String SERIALIZED_NAME_BANK_STREET_NAME = "bankStreetName";
@SerializedName(SERIALIZED_NAME_BANK_STREET_NAME)
private String bankStreetName;
public static final String SERIALIZED_NAME_BANK_STREET_NUMBER = "bankStreetNumber";
@SerializedName(SERIALIZED_NAME_BANK_STREET_NUMBER)
private String bankStreetNumber;
public static final String SERIALIZED_NAME_BUSINESS_IDENTIFICATION_CODE = "businessIdentificationCode";
@SerializedName(SERIALIZED_NAME_BUSINESS_IDENTIFICATION_CODE)
private String businessIdentificationCode;
public static final String SERIALIZED_NAME_CITY_BLACK_LIST = "cityBlackList";
@SerializedName(SERIALIZED_NAME_CITY_BLACK_LIST)
private String cityBlackList;
public static final String SERIALIZED_NAME_CITY_WHITE_LIST = "cityWhiteList";
@SerializedName(SERIALIZED_NAME_CITY_WHITE_LIST)
private String cityWhiteList;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_DEVICE_SESSION_ID = "deviceSessionId";
@SerializedName(SERIALIZED_NAME_DEVICE_SESSION_ID)
private String deviceSessionId;
public static final String SERIALIZED_NAME_GATEWAY_NAME = "gatewayName";
@SerializedName(SERIALIZED_NAME_GATEWAY_NAME)
private String gatewayName;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_KEY = "key";
@SerializedName(SERIALIZED_NAME_KEY)
private String key;
public static final String SERIALIZED_NAME_LOCALE = "locale";
@SerializedName(SERIALIZED_NAME_LOCALE)
private String locale;
public static final String SERIALIZED_NAME_MAX_CONSECUTIVE_PAYMENT_FAILURES = "maxConsecutivePaymentFailures";
@SerializedName(SERIALIZED_NAME_MAX_CONSECUTIVE_PAYMENT_FAILURES)
private Integer maxConsecutivePaymentFailures;
public static final String SERIALIZED_NAME_METHOD = "method";
@SerializedName(SERIALIZED_NAME_METHOD)
private String method;
public static final String SERIALIZED_NAME_PAGE_ID = "pageId";
@SerializedName(SERIALIZED_NAME_PAGE_ID)
private String pageId;
public static final String SERIALIZED_NAME_PARAM_GW_OPTIONS_STAR_OPTION_STAR = "param_gwOptions_[*option*]";
@SerializedName(SERIALIZED_NAME_PARAM_GW_OPTIONS_STAR_OPTION_STAR)
private String paramGwOptionsStarOptionStar;
public static final String SERIALIZED_NAME_PARAM_SUPPORTED_TYPES = "param_supportedTypes";
@SerializedName(SERIALIZED_NAME_PARAM_SUPPORTED_TYPES)
private String paramSupportedTypes;
public static final String SERIALIZED_NAME_PASSTHROUGH1_COMMA2_COMMA3_COMMA4_COMMA5 = "passthrough[1,2,3,4,5]";
@SerializedName(SERIALIZED_NAME_PASSTHROUGH1_COMMA2_COMMA3_COMMA4_COMMA5)
private String passthrough1Comma2Comma3Comma4Comma5;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY = "paymentGateway";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY)
private String paymentGateway;
public static final String SERIALIZED_NAME_PAYMENT_RETRY_WINDOW = "paymentRetryWindow";
@SerializedName(SERIALIZED_NAME_PAYMENT_RETRY_WINDOW)
private Integer paymentRetryWindow;
public static final String SERIALIZED_NAME_PM_ID = "pmId";
@SerializedName(SERIALIZED_NAME_PM_ID)
private String pmId;
public static final String SERIALIZED_NAME_SIGNATURE = "signature";
@SerializedName(SERIALIZED_NAME_SIGNATURE)
private String signature;
public static final String SERIALIZED_NAME_SIGNATURE_TYPE = "signatureType";
@SerializedName(SERIALIZED_NAME_SIGNATURE_TYPE)
private String signatureType;
public static final String SERIALIZED_NAME_STYLE = "style";
@SerializedName(SERIALIZED_NAME_STYLE)
private String style;
public static final String SERIALIZED_NAME_SUBMIT_ENABLED = "submitEnabled";
@SerializedName(SERIALIZED_NAME_SUBMIT_ENABLED)
private Boolean submitEnabled;
public static final String SERIALIZED_NAME_TENANT_ID = "tenantId";
@SerializedName(SERIALIZED_NAME_TENANT_ID)
private String tenantId;
public static final String SERIALIZED_NAME_TOKEN = "token";
@SerializedName(SERIALIZED_NAME_TOKEN)
private String token;
public static final String SERIALIZED_NAME_URI = "uri";
@SerializedName(SERIALIZED_NAME_URI)
private String uri;
public static final String SERIALIZED_NAME_USE_DEFAULT_RETRY_RULE = "useDefaultRetryRule";
@SerializedName(SERIALIZED_NAME_USE_DEFAULT_RETRY_RULE)
private Boolean useDefaultRetryRule;
public CreateRSASignatureRequest() {
}
public CreateRSASignatureRequest IBAN(String IBAN) {
this.IBAN = IBAN;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters specific for Bank Transfer - Direct Debit. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return IBAN
*/
@javax.annotation.Nullable
public String getIBAN() {
return IBAN;
}
public void setIBAN(String IBAN) {
this.IBAN = IBAN;
}
public CreateRSASignatureRequest accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return accountId
*/
@javax.annotation.Nullable
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public CreateRSASignatureRequest authorizationAmount(BigDecimal authorizationAmount) {
this.authorizationAmount = authorizationAmount;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return authorizationAmount
*/
@javax.annotation.Nullable
public BigDecimal getAuthorizationAmount() {
return authorizationAmount;
}
public void setAuthorizationAmount(BigDecimal authorizationAmount) {
this.authorizationAmount = authorizationAmount;
}
public CreateRSASignatureRequest bankBranchCode(String bankBranchCode) {
this.bankBranchCode = bankBranchCode;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters specific for Bank Transfer - Direct Debit. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return bankBranchCode
*/
@javax.annotation.Nullable
public String getBankBranchCode() {
return bankBranchCode;
}
public void setBankBranchCode(String bankBranchCode) {
this.bankBranchCode = bankBranchCode;
}
public CreateRSASignatureRequest bankCheckDigit(String bankCheckDigit) {
this.bankCheckDigit = bankCheckDigit;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters specific for Bank Transfer - Direct Debit. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return bankCheckDigit
*/
@javax.annotation.Nullable
public String getBankCheckDigit() {
return bankCheckDigit;
}
public void setBankCheckDigit(String bankCheckDigit) {
this.bankCheckDigit = bankCheckDigit;
}
public CreateRSASignatureRequest bankCity(String bankCity) {
this.bankCity = bankCity;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters specific for Bank Transfer - Direct Debit. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return bankCity
*/
@javax.annotation.Nullable
public String getBankCity() {
return bankCity;
}
public void setBankCity(String bankCity) {
this.bankCity = bankCity;
}
public CreateRSASignatureRequest bankPostalCode(String bankPostalCode) {
this.bankPostalCode = bankPostalCode;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters specific for Bank Transfer - Direct Debit. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return bankPostalCode
*/
@javax.annotation.Nullable
public String getBankPostalCode() {
return bankPostalCode;
}
public void setBankPostalCode(String bankPostalCode) {
this.bankPostalCode = bankPostalCode;
}
public CreateRSASignatureRequest bankStreetName(String bankStreetName) {
this.bankStreetName = bankStreetName;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters specific for Bank Transfer - Direct Debit. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return bankStreetName
*/
@javax.annotation.Nullable
public String getBankStreetName() {
return bankStreetName;
}
public void setBankStreetName(String bankStreetName) {
this.bankStreetName = bankStreetName;
}
public CreateRSASignatureRequest bankStreetNumber(String bankStreetNumber) {
this.bankStreetNumber = bankStreetNumber;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters specific for Bank Transfer - Direct Debit. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return bankStreetNumber
*/
@javax.annotation.Nullable
public String getBankStreetNumber() {
return bankStreetNumber;
}
public void setBankStreetNumber(String bankStreetNumber) {
this.bankStreetNumber = bankStreetNumber;
}
public CreateRSASignatureRequest businessIdentificationCode(String businessIdentificationCode) {
this.businessIdentificationCode = businessIdentificationCode;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters specific for Bank Transfer - Direct Debit. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return businessIdentificationCode
*/
@javax.annotation.Nullable
public String getBusinessIdentificationCode() {
return businessIdentificationCode;
}
public void setBusinessIdentificationCode(String businessIdentificationCode) {
this.businessIdentificationCode = businessIdentificationCode;
}
public CreateRSASignatureRequest cityBlackList(String cityBlackList) {
this.cityBlackList = cityBlackList;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters specific for credit cards. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return cityBlackList
*/
@javax.annotation.Nullable
public String getCityBlackList() {
return cityBlackList;
}
public void setCityBlackList(String cityBlackList) {
this.cityBlackList = cityBlackList;
}
public CreateRSASignatureRequest cityWhiteList(String cityWhiteList) {
this.cityWhiteList = cityWhiteList;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters specific for credit cards. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return cityWhiteList
*/
@javax.annotation.Nullable
public String getCityWhiteList() {
return cityWhiteList;
}
public void setCityWhiteList(String cityWhiteList) {
this.cityWhiteList = cityWhiteList;
}
public CreateRSASignatureRequest currency(String currency) {
this.currency = currency;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public CreateRSASignatureRequest deviceSessionId(String deviceSessionId) {
this.deviceSessionId = deviceSessionId;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return deviceSessionId
*/
@javax.annotation.Nullable
public String getDeviceSessionId() {
return deviceSessionId;
}
public void setDeviceSessionId(String deviceSessionId) {
this.deviceSessionId = deviceSessionId;
}
public CreateRSASignatureRequest gatewayName(String gatewayName) {
this.gatewayName = gatewayName;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return gatewayName
*/
@javax.annotation.Nullable
public String getGatewayName() {
return gatewayName;
}
public void setGatewayName(String gatewayName) {
this.gatewayName = gatewayName;
}
public CreateRSASignatureRequest id(String id) {
this.id = id;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public CreateRSASignatureRequest key(String key) {
this.key = key;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return key
*/
@javax.annotation.Nullable
public String getKey() {
return key;
}
public void setKey(String key) {
this.key = key;
}
public CreateRSASignatureRequest locale(String locale) {
this.locale = locale;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return locale
*/
@javax.annotation.Nullable
public String getLocale() {
return locale;
}
public void setLocale(String locale) {
this.locale = locale;
}
public CreateRSASignatureRequest maxConsecutivePaymentFailures(Integer maxConsecutivePaymentFailures) {
this.maxConsecutivePaymentFailures = maxConsecutivePaymentFailures;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return maxConsecutivePaymentFailures
*/
@javax.annotation.Nullable
public Integer getMaxConsecutivePaymentFailures() {
return maxConsecutivePaymentFailures;
}
public void setMaxConsecutivePaymentFailures(Integer maxConsecutivePaymentFailures) {
this.maxConsecutivePaymentFailures = maxConsecutivePaymentFailures;
}
public CreateRSASignatureRequest method(String method) {
this.method = method;
return this;
}
/**
* The type of the request. Set it to Post.
* @return method
*/
@javax.annotation.Nonnull
public String getMethod() {
return method;
}
public void setMethod(String method) {
this.method = method;
}
public CreateRSASignatureRequest pageId(String pageId) {
this.pageId = pageId;
return this;
}
/**
* The page id of your Payment Pages 2.0 form. Click **Show Page Id** next to the Payment Page name in the Hosted Page List to retrieve the page id.
* @return pageId
*/
@javax.annotation.Nonnull
public String getPageId() {
return pageId;
}
public void setPageId(String pageId) {
this.pageId = pageId;
}
public CreateRSASignatureRequest paramGwOptionsStarOptionStar(String paramGwOptionsStarOptionStar) {
this.paramGwOptionsStarOptionStar = paramGwOptionsStarOptionStar;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return paramGwOptionsStarOptionStar
*/
@javax.annotation.Nullable
public String getParamGwOptionsStarOptionStar() {
return paramGwOptionsStarOptionStar;
}
public void setParamGwOptionsStarOptionStar(String paramGwOptionsStarOptionStar) {
this.paramGwOptionsStarOptionStar = paramGwOptionsStarOptionStar;
}
public CreateRSASignatureRequest paramSupportedTypes(String paramSupportedTypes) {
this.paramSupportedTypes = paramSupportedTypes;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters specific for credit cards. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return paramSupportedTypes
*/
@javax.annotation.Nullable
public String getParamSupportedTypes() {
return paramSupportedTypes;
}
public void setParamSupportedTypes(String paramSupportedTypes) {
this.paramSupportedTypes = paramSupportedTypes;
}
public CreateRSASignatureRequest passthrough1Comma2Comma3Comma4Comma5(String passthrough1Comma2Comma3Comma4Comma5) {
this.passthrough1Comma2Comma3Comma4Comma5 = passthrough1Comma2Comma3Comma4Comma5;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details. Note: Although up to 15 passthrough parameters can be supported when passing in your client parameters, only the first 5 parameters are used for signature generation and validation.
* @return passthrough1Comma2Comma3Comma4Comma5
*/
@javax.annotation.Nullable
public String getPassthrough1Comma2Comma3Comma4Comma5() {
return passthrough1Comma2Comma3Comma4Comma5;
}
public void setPassthrough1Comma2Comma3Comma4Comma5(String passthrough1Comma2Comma3Comma4Comma5) {
this.passthrough1Comma2Comma3Comma4Comma5 = passthrough1Comma2Comma3Comma4Comma5;
}
public CreateRSASignatureRequest paymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return paymentGateway
*/
@javax.annotation.Nullable
public String getPaymentGateway() {
return paymentGateway;
}
public void setPaymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
}
public CreateRSASignatureRequest paymentRetryWindow(Integer paymentRetryWindow) {
this.paymentRetryWindow = paymentRetryWindow;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return paymentRetryWindow
*/
@javax.annotation.Nullable
public Integer getPaymentRetryWindow() {
return paymentRetryWindow;
}
public void setPaymentRetryWindow(Integer paymentRetryWindow) {
this.paymentRetryWindow = paymentRetryWindow;
}
public CreateRSASignatureRequest pmId(String pmId) {
this.pmId = pmId;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters specific for credit cards. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return pmId
*/
@javax.annotation.Nullable
public String getPmId() {
return pmId;
}
public void setPmId(String pmId) {
this.pmId = pmId;
}
public CreateRSASignatureRequest signature(String signature) {
this.signature = signature;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return signature
*/
@javax.annotation.Nullable
public String getSignature() {
return signature;
}
public void setSignature(String signature) {
this.signature = signature;
}
public CreateRSASignatureRequest signatureType(String signatureType) {
this.signatureType = signatureType;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return signatureType
*/
@javax.annotation.Nullable
public String getSignatureType() {
return signatureType;
}
public void setSignatureType(String signatureType) {
this.signatureType = signatureType;
}
public CreateRSASignatureRequest style(String style) {
this.style = style;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return style
*/
@javax.annotation.Nullable
public String getStyle() {
return style;
}
public void setStyle(String style) {
this.style = style;
}
public CreateRSASignatureRequest submitEnabled(Boolean submitEnabled) {
this.submitEnabled = submitEnabled;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return submitEnabled
*/
@javax.annotation.Nullable
public Boolean getSubmitEnabled() {
return submitEnabled;
}
public void setSubmitEnabled(Boolean submitEnabled) {
this.submitEnabled = submitEnabled;
}
public CreateRSASignatureRequest tenantId(String tenantId) {
this.tenantId = tenantId;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return tenantId
*/
@javax.annotation.Nullable
public String getTenantId() {
return tenantId;
}
public void setTenantId(String tenantId) {
this.tenantId = tenantId;
}
public CreateRSASignatureRequest token(String token) {
this.token = token;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return token
*/
@javax.annotation.Nullable
public String getToken() {
return token;
}
public void setToken(String token) {
this.token = token;
}
public CreateRSASignatureRequest uri(String uri) {
this.uri = uri;
return this;
}
/**
* The URL that the Payment Page will be served from. * For US Cloud 1 Production environment: Use https://na.zuora.com/apps/PublicHostedPageLite.do * For US Cloud 1 Sandbox environment: Use https://sandbox.na.zuora.com/apps/PublicHostedPageLite.d * For US Cloud 2 Production environment: Use https://www.zuora.com/apps/PublicHostedPageLite.do * For US Cloud 2 API Sandbox environment: Use https://apisandbox.zuora.com/apps/PublicHostedPageLite.do * For US Central Sandbox environment: Use https://test.zuora.com/apps/PublicHostedPageLite.do * For EU Cloud Production environment: Use https://eu.zuora.com/apps/PublicHostedPageLite.do * For EU Cloud Sandbox environment: Use https://sandbox.eu.zuora.com/apps/PublicHostedPageLite.do * For EU Central Sandbox environment: Use https://test.eu.zuora.com/apps/PublicHostedPageLite.do
* @return uri
*/
@javax.annotation.Nonnull
public String getUri() {
return uri;
}
public void setUri(String uri) {
this.uri = uri;
}
public CreateRSASignatureRequest useDefaultRetryRule(Boolean useDefaultRetryRule) {
this.useDefaultRetryRule = useDefaultRetryRule;
return this;
}
/**
* An optional client parameter that can be used for validating client-side HPM parameters. See [Client parameters for Payment Pages 2.0](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/J_Client_Parameters_for_Payment_Pages_2.0) and [Validate client-side HPM parameters](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/LA_Hosted_Payment_Pages/B_Payment_Pages_2.0/F_Generate_the_Digital_Signature_for_Payment_Pages_2.0#Validate_Client-side_HPM_Parameters) for details.
* @return useDefaultRetryRule
*/
@javax.annotation.Nullable
public Boolean getUseDefaultRetryRule() {
return useDefaultRetryRule;
}
public void setUseDefaultRetryRule(Boolean useDefaultRetryRule) {
this.useDefaultRetryRule = useDefaultRetryRule;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreateRSASignatureRequest instance itself
*/
public CreateRSASignatureRequest putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateRSASignatureRequest createRSASignatureRequest = (CreateRSASignatureRequest) o;
return Objects.equals(this.IBAN, createRSASignatureRequest.IBAN) &&
Objects.equals(this.accountId, createRSASignatureRequest.accountId) &&
Objects.equals(this.authorizationAmount, createRSASignatureRequest.authorizationAmount) &&
Objects.equals(this.bankBranchCode, createRSASignatureRequest.bankBranchCode) &&
Objects.equals(this.bankCheckDigit, createRSASignatureRequest.bankCheckDigit) &&
Objects.equals(this.bankCity, createRSASignatureRequest.bankCity) &&
Objects.equals(this.bankPostalCode, createRSASignatureRequest.bankPostalCode) &&
Objects.equals(this.bankStreetName, createRSASignatureRequest.bankStreetName) &&
Objects.equals(this.bankStreetNumber, createRSASignatureRequest.bankStreetNumber) &&
Objects.equals(this.businessIdentificationCode, createRSASignatureRequest.businessIdentificationCode) &&
Objects.equals(this.cityBlackList, createRSASignatureRequest.cityBlackList) &&
Objects.equals(this.cityWhiteList, createRSASignatureRequest.cityWhiteList) &&
Objects.equals(this.currency, createRSASignatureRequest.currency) &&
Objects.equals(this.deviceSessionId, createRSASignatureRequest.deviceSessionId) &&
Objects.equals(this.gatewayName, createRSASignatureRequest.gatewayName) &&
Objects.equals(this.id, createRSASignatureRequest.id) &&
Objects.equals(this.key, createRSASignatureRequest.key) &&
Objects.equals(this.locale, createRSASignatureRequest.locale) &&
Objects.equals(this.maxConsecutivePaymentFailures, createRSASignatureRequest.maxConsecutivePaymentFailures) &&
Objects.equals(this.method, createRSASignatureRequest.method) &&
Objects.equals(this.pageId, createRSASignatureRequest.pageId) &&
Objects.equals(this.paramGwOptionsStarOptionStar, createRSASignatureRequest.paramGwOptionsStarOptionStar) &&
Objects.equals(this.paramSupportedTypes, createRSASignatureRequest.paramSupportedTypes) &&
Objects.equals(this.passthrough1Comma2Comma3Comma4Comma5, createRSASignatureRequest.passthrough1Comma2Comma3Comma4Comma5) &&
Objects.equals(this.paymentGateway, createRSASignatureRequest.paymentGateway) &&
Objects.equals(this.paymentRetryWindow, createRSASignatureRequest.paymentRetryWindow) &&
Objects.equals(this.pmId, createRSASignatureRequest.pmId) &&
Objects.equals(this.signature, createRSASignatureRequest.signature) &&
Objects.equals(this.signatureType, createRSASignatureRequest.signatureType) &&
Objects.equals(this.style, createRSASignatureRequest.style) &&
Objects.equals(this.submitEnabled, createRSASignatureRequest.submitEnabled) &&
Objects.equals(this.tenantId, createRSASignatureRequest.tenantId) &&
Objects.equals(this.token, createRSASignatureRequest.token) &&
Objects.equals(this.uri, createRSASignatureRequest.uri) &&
Objects.equals(this.useDefaultRetryRule, createRSASignatureRequest.useDefaultRetryRule)&&
Objects.equals(this.additionalProperties, createRSASignatureRequest.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(IBAN, accountId, authorizationAmount, bankBranchCode, bankCheckDigit, bankCity, bankPostalCode, bankStreetName, bankStreetNumber, businessIdentificationCode, cityBlackList, cityWhiteList, currency, deviceSessionId, gatewayName, id, key, locale, maxConsecutivePaymentFailures, method, pageId, paramGwOptionsStarOptionStar, paramSupportedTypes, passthrough1Comma2Comma3Comma4Comma5, paymentGateway, paymentRetryWindow, pmId, signature, signatureType, style, submitEnabled, tenantId, token, uri, useDefaultRetryRule, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateRSASignatureRequest {\n");
sb.append(" IBAN: ").append(toIndentedString(IBAN)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" authorizationAmount: ").append(toIndentedString(authorizationAmount)).append("\n");
sb.append(" bankBranchCode: ").append(toIndentedString(bankBranchCode)).append("\n");
sb.append(" bankCheckDigit: ").append(toIndentedString(bankCheckDigit)).append("\n");
sb.append(" bankCity: ").append(toIndentedString(bankCity)).append("\n");
sb.append(" bankPostalCode: ").append(toIndentedString(bankPostalCode)).append("\n");
sb.append(" bankStreetName: ").append(toIndentedString(bankStreetName)).append("\n");
sb.append(" bankStreetNumber: ").append(toIndentedString(bankStreetNumber)).append("\n");
sb.append(" businessIdentificationCode: ").append(toIndentedString(businessIdentificationCode)).append("\n");
sb.append(" cityBlackList: ").append(toIndentedString(cityBlackList)).append("\n");
sb.append(" cityWhiteList: ").append(toIndentedString(cityWhiteList)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" deviceSessionId: ").append(toIndentedString(deviceSessionId)).append("\n");
sb.append(" gatewayName: ").append(toIndentedString(gatewayName)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" key: ").append(toIndentedString(key)).append("\n");
sb.append(" locale: ").append(toIndentedString(locale)).append("\n");
sb.append(" maxConsecutivePaymentFailures: ").append(toIndentedString(maxConsecutivePaymentFailures)).append("\n");
sb.append(" method: ").append(toIndentedString(method)).append("\n");
sb.append(" pageId: ").append(toIndentedString(pageId)).append("\n");
sb.append(" paramGwOptionsStarOptionStar: ").append(toIndentedString(paramGwOptionsStarOptionStar)).append("\n");
sb.append(" paramSupportedTypes: ").append(toIndentedString(paramSupportedTypes)).append("\n");
sb.append(" passthrough1Comma2Comma3Comma4Comma5: ").append(toIndentedString(passthrough1Comma2Comma3Comma4Comma5)).append("\n");
sb.append(" paymentGateway: ").append(toIndentedString(paymentGateway)).append("\n");
sb.append(" paymentRetryWindow: ").append(toIndentedString(paymentRetryWindow)).append("\n");
sb.append(" pmId: ").append(toIndentedString(pmId)).append("\n");
sb.append(" signature: ").append(toIndentedString(signature)).append("\n");
sb.append(" signatureType: ").append(toIndentedString(signatureType)).append("\n");
sb.append(" style: ").append(toIndentedString(style)).append("\n");
sb.append(" submitEnabled: ").append(toIndentedString(submitEnabled)).append("\n");
sb.append(" tenantId: ").append(toIndentedString(tenantId)).append("\n");
sb.append(" token: ").append(toIndentedString(token)).append("\n");
sb.append(" uri: ").append(toIndentedString(uri)).append("\n");
sb.append(" useDefaultRetryRule: ").append(toIndentedString(useDefaultRetryRule)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("IBAN");
openapiFields.add("accountId");
openapiFields.add("authorizationAmount");
openapiFields.add("bankBranchCode");
openapiFields.add("bankCheckDigit");
openapiFields.add("bankCity");
openapiFields.add("bankPostalCode");
openapiFields.add("bankStreetName");
openapiFields.add("bankStreetNumber");
openapiFields.add("businessIdentificationCode");
openapiFields.add("cityBlackList");
openapiFields.add("cityWhiteList");
openapiFields.add("currency");
openapiFields.add("deviceSessionId");
openapiFields.add("gatewayName");
openapiFields.add("id");
openapiFields.add("key");
openapiFields.add("locale");
openapiFields.add("maxConsecutivePaymentFailures");
openapiFields.add("method");
openapiFields.add("pageId");
openapiFields.add("param_gwOptions_[*option*]");
openapiFields.add("param_supportedTypes");
openapiFields.add("passthrough[1,2,3,4,5]");
openapiFields.add("paymentGateway");
openapiFields.add("paymentRetryWindow");
openapiFields.add("pmId");
openapiFields.add("signature");
openapiFields.add("signatureType");
openapiFields.add("style");
openapiFields.add("submitEnabled");
openapiFields.add("tenantId");
openapiFields.add("token");
openapiFields.add("uri");
openapiFields.add("useDefaultRetryRule");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("method");
openapiRequiredFields.add("pageId");
openapiRequiredFields.add("uri");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreateRSASignatureRequest
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreateRSASignatureRequest.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreateRSASignatureRequest is not found in the empty JSON string", CreateRSASignatureRequest.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : CreateRSASignatureRequest.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("IBAN") != null && !jsonObj.get("IBAN").isJsonNull()) && !jsonObj.get("IBAN").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IBAN` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IBAN").toString()));
}
if ((jsonObj.get("accountId") != null && !jsonObj.get("accountId").isJsonNull()) && !jsonObj.get("accountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountId").toString()));
}
if ((jsonObj.get("bankBranchCode") != null && !jsonObj.get("bankBranchCode").isJsonNull()) && !jsonObj.get("bankBranchCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankBranchCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankBranchCode").toString()));
}
if ((jsonObj.get("bankCheckDigit") != null && !jsonObj.get("bankCheckDigit").isJsonNull()) && !jsonObj.get("bankCheckDigit").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankCheckDigit` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankCheckDigit").toString()));
}
if ((jsonObj.get("bankCity") != null && !jsonObj.get("bankCity").isJsonNull()) && !jsonObj.get("bankCity").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankCity` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankCity").toString()));
}
if ((jsonObj.get("bankPostalCode") != null && !jsonObj.get("bankPostalCode").isJsonNull()) && !jsonObj.get("bankPostalCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankPostalCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankPostalCode").toString()));
}
if ((jsonObj.get("bankStreetName") != null && !jsonObj.get("bankStreetName").isJsonNull()) && !jsonObj.get("bankStreetName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankStreetName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankStreetName").toString()));
}
if ((jsonObj.get("bankStreetNumber") != null && !jsonObj.get("bankStreetNumber").isJsonNull()) && !jsonObj.get("bankStreetNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankStreetNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankStreetNumber").toString()));
}
if ((jsonObj.get("businessIdentificationCode") != null && !jsonObj.get("businessIdentificationCode").isJsonNull()) && !jsonObj.get("businessIdentificationCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `businessIdentificationCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("businessIdentificationCode").toString()));
}
if ((jsonObj.get("cityBlackList") != null && !jsonObj.get("cityBlackList").isJsonNull()) && !jsonObj.get("cityBlackList").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cityBlackList` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cityBlackList").toString()));
}
if ((jsonObj.get("cityWhiteList") != null && !jsonObj.get("cityWhiteList").isJsonNull()) && !jsonObj.get("cityWhiteList").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cityWhiteList` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cityWhiteList").toString()));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("deviceSessionId") != null && !jsonObj.get("deviceSessionId").isJsonNull()) && !jsonObj.get("deviceSessionId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `deviceSessionId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("deviceSessionId").toString()));
}
if ((jsonObj.get("gatewayName") != null && !jsonObj.get("gatewayName").isJsonNull()) && !jsonObj.get("gatewayName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayName").toString()));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("key") != null && !jsonObj.get("key").isJsonNull()) && !jsonObj.get("key").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `key` to be a primitive type in the JSON string but got `%s`", jsonObj.get("key").toString()));
}
if ((jsonObj.get("locale") != null && !jsonObj.get("locale").isJsonNull()) && !jsonObj.get("locale").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `locale` to be a primitive type in the JSON string but got `%s`", jsonObj.get("locale").toString()));
}
if (!jsonObj.get("method").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `method` to be a primitive type in the JSON string but got `%s`", jsonObj.get("method").toString()));
}
if (!jsonObj.get("pageId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `pageId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("pageId").toString()));
}
if ((jsonObj.get("param_gwOptions_[*option*]") != null && !jsonObj.get("param_gwOptions_[*option*]").isJsonNull()) && !jsonObj.get("param_gwOptions_[*option*]").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `param_gwOptions_[*option*]` to be a primitive type in the JSON string but got `%s`", jsonObj.get("param_gwOptions_[*option*]").toString()));
}
if ((jsonObj.get("param_supportedTypes") != null && !jsonObj.get("param_supportedTypes").isJsonNull()) && !jsonObj.get("param_supportedTypes").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `param_supportedTypes` to be a primitive type in the JSON string but got `%s`", jsonObj.get("param_supportedTypes").toString()));
}
if ((jsonObj.get("passthrough[1,2,3,4,5]") != null && !jsonObj.get("passthrough[1,2,3,4,5]").isJsonNull()) && !jsonObj.get("passthrough[1,2,3,4,5]").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `passthrough[1,2,3,4,5]` to be a primitive type in the JSON string but got `%s`", jsonObj.get("passthrough[1,2,3,4,5]").toString()));
}
if ((jsonObj.get("paymentGateway") != null && !jsonObj.get("paymentGateway").isJsonNull()) && !jsonObj.get("paymentGateway").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentGateway` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentGateway").toString()));
}
if ((jsonObj.get("pmId") != null && !jsonObj.get("pmId").isJsonNull()) && !jsonObj.get("pmId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `pmId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("pmId").toString()));
}
if ((jsonObj.get("signature") != null && !jsonObj.get("signature").isJsonNull()) && !jsonObj.get("signature").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `signature` to be a primitive type in the JSON string but got `%s`", jsonObj.get("signature").toString()));
}
if ((jsonObj.get("signatureType") != null && !jsonObj.get("signatureType").isJsonNull()) && !jsonObj.get("signatureType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `signatureType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("signatureType").toString()));
}
if ((jsonObj.get("style") != null && !jsonObj.get("style").isJsonNull()) && !jsonObj.get("style").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `style` to be a primitive type in the JSON string but got `%s`", jsonObj.get("style").toString()));
}
if ((jsonObj.get("tenantId") != null && !jsonObj.get("tenantId").isJsonNull()) && !jsonObj.get("tenantId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `tenantId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("tenantId").toString()));
}
if ((jsonObj.get("token") != null && !jsonObj.get("token").isJsonNull()) && !jsonObj.get("token").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `token` to be a primitive type in the JSON string but got `%s`", jsonObj.get("token").toString()));
}
if (!jsonObj.get("uri").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `uri` to be a primitive type in the JSON string but got `%s`", jsonObj.get("uri").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreateRSASignatureRequest.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreateRSASignatureRequest' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreateRSASignatureRequest.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreateRSASignatureRequest value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreateRSASignatureRequest read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreateRSASignatureRequest instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreateRSASignatureRequest given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreateRSASignatureRequest
* @throws IOException if the JSON string is invalid with respect to CreateRSASignatureRequest
*/
public static CreateRSASignatureRequest fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreateRSASignatureRequest.class);
}
/**
* Convert an instance of CreateRSASignatureRequest to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy