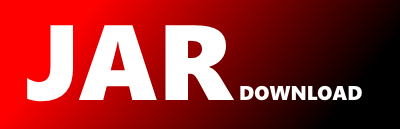
com.zuora.model.CreateSubscriptionComponent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.ChargeModelConfigurationForSubscription;
import com.zuora.model.Tier;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* CreateSubscriptionComponent
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreateSubscriptionComponent {
public static final String SERIALIZED_NAME_AMENDED_BY_ORDER_ON = "amendedByOrderOn";
@SerializedName(SERIALIZED_NAME_AMENDED_BY_ORDER_ON)
private String amendedByOrderOn;
public static final String SERIALIZED_NAME_APPLY_DISCOUNT_TO = "applyDiscountTo";
@SerializedName(SERIALIZED_NAME_APPLY_DISCOUNT_TO)
private String applyDiscountTo;
public static final String SERIALIZED_NAME_BILL_CYCLE_DAY = "billCycleDay";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_DAY)
private String billCycleDay;
public static final String SERIALIZED_NAME_BILL_CYCLE_TYPE = "billCycleType";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_TYPE)
private String billCycleType;
public static final String SERIALIZED_NAME_BILLING_PERIOD = "billingPeriod";
@SerializedName(SERIALIZED_NAME_BILLING_PERIOD)
private String billingPeriod;
public static final String SERIALIZED_NAME_BILLING_PERIOD_ALIGNMENT = "billingPeriodAlignment";
@SerializedName(SERIALIZED_NAME_BILLING_PERIOD_ALIGNMENT)
private String billingPeriodAlignment;
public static final String SERIALIZED_NAME_BILLING_TIMING = "billingTiming";
@SerializedName(SERIALIZED_NAME_BILLING_TIMING)
private String billingTiming;
public static final String SERIALIZED_NAME_CHARGE_MODEL_CONFIGURATION = "chargeModelConfiguration";
@SerializedName(SERIALIZED_NAME_CHARGE_MODEL_CONFIGURATION)
private ChargeModelConfigurationForSubscription chargeModelConfiguration;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_DISCOUNT_AMOUNT = "discountAmount";
@SerializedName(SERIALIZED_NAME_DISCOUNT_AMOUNT)
private BigDecimal discountAmount;
public static final String SERIALIZED_NAME_DISCOUNT_LEVEL = "discountLevel";
@SerializedName(SERIALIZED_NAME_DISCOUNT_LEVEL)
private String discountLevel;
public static final String SERIALIZED_NAME_DISCOUNT_PERCENTAGE = "discountPercentage";
@SerializedName(SERIALIZED_NAME_DISCOUNT_PERCENTAGE)
private BigDecimal discountPercentage;
public static final String SERIALIZED_NAME_END_DATE_CONDITION = "endDateCondition";
@SerializedName(SERIALIZED_NAME_END_DATE_CONDITION)
private String endDateCondition;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING = "excludeItemBillingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBillingFromRevenueAccounting;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING = "excludeItemBookingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBookingFromRevenueAccounting;
public static final String SERIALIZED_NAME_INCLUDED_UNITS = "includedUnits";
@SerializedName(SERIALIZED_NAME_INCLUDED_UNITS)
private BigDecimal includedUnits;
public static final String SERIALIZED_NAME_IS_ALLOCATION_ELIGIBLE = "isAllocationEligible";
@SerializedName(SERIALIZED_NAME_IS_ALLOCATION_ELIGIBLE)
private Boolean isAllocationEligible;
public static final String SERIALIZED_NAME_IS_UNBILLED = "isUnbilled";
@SerializedName(SERIALIZED_NAME_IS_UNBILLED)
private Boolean isUnbilled;
public static final String SERIALIZED_NAME_LIST_PRICE_BASE = "listPriceBase";
@SerializedName(SERIALIZED_NAME_LIST_PRICE_BASE)
private String listPriceBase;
public static final String SERIALIZED_NAME_NUMBER = "number";
@SerializedName(SERIALIZED_NAME_NUMBER)
private String number;
public static final String SERIALIZED_NAME_NUMBER_OF_PERIODS = "numberOfPeriods";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_PERIODS)
private Long numberOfPeriods;
public static final String SERIALIZED_NAME_ORIGINAL_ORDER_DATE = "originalOrderDate";
@SerializedName(SERIALIZED_NAME_ORIGINAL_ORDER_DATE)
private LocalDate originalOrderDate;
public static final String SERIALIZED_NAME_OVERAGE_PRICE = "overagePrice";
@SerializedName(SERIALIZED_NAME_OVERAGE_PRICE)
private BigDecimal overagePrice;
public static final String SERIALIZED_NAME_OVERAGE_UNUSED_UNITS_CREDIT_OPTION = "overageUnusedUnitsCreditOption";
@SerializedName(SERIALIZED_NAME_OVERAGE_UNUSED_UNITS_CREDIT_OPTION)
private String overageUnusedUnitsCreditOption;
public static final String SERIALIZED_NAME_PRICE = "price";
@SerializedName(SERIALIZED_NAME_PRICE)
private BigDecimal price;
public static final String SERIALIZED_NAME_PRICE_CHANGE_OPTION = "priceChangeOption";
@SerializedName(SERIALIZED_NAME_PRICE_CHANGE_OPTION)
private String priceChangeOption;
public static final String SERIALIZED_NAME_PRICE_INCREASE_PERCENTAGE = "priceIncreasePercentage";
@SerializedName(SERIALIZED_NAME_PRICE_INCREASE_PERCENTAGE)
private BigDecimal priceIncreasePercentage;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_ID = "productRatePlanChargeId";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_ID)
private String productRatePlanChargeId;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_NUMBER = "productRatePlanChargeNumber";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_NUMBER)
private String productRatePlanChargeNumber;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_RATING_GROUP = "ratingGroup";
@SerializedName(SERIALIZED_NAME_RATING_GROUP)
private String ratingGroup;
public static final String SERIALIZED_NAME_SPECIFIC_BILLING_PERIOD = "specificBillingPeriod";
@SerializedName(SERIALIZED_NAME_SPECIFIC_BILLING_PERIOD)
private Long specificBillingPeriod;
public static final String SERIALIZED_NAME_SPECIFIC_END_DATE = "specificEndDate";
@SerializedName(SERIALIZED_NAME_SPECIFIC_END_DATE)
private LocalDate specificEndDate;
public static final String SERIALIZED_NAME_SPECIFIC_LIST_PRICE_BASE = "specificListPriceBase";
@SerializedName(SERIALIZED_NAME_SPECIFIC_LIST_PRICE_BASE)
private Integer specificListPriceBase;
public static final String SERIALIZED_NAME_TIERS = "tiers";
@SerializedName(SERIALIZED_NAME_TIERS)
private List tiers;
public static final String SERIALIZED_NAME_TRIGGER_DATE = "triggerDate";
@SerializedName(SERIALIZED_NAME_TRIGGER_DATE)
private LocalDate triggerDate;
public static final String SERIALIZED_NAME_TRIGGER_EVENT = "triggerEvent";
@SerializedName(SERIALIZED_NAME_TRIGGER_EVENT)
private String triggerEvent;
public static final String SERIALIZED_NAME_UNUSED_UNITS_CREDIT_RATES = "unusedUnitsCreditRates";
@SerializedName(SERIALIZED_NAME_UNUSED_UNITS_CREDIT_RATES)
private BigDecimal unusedUnitsCreditRates;
public static final String SERIALIZED_NAME_UP_TO_PERIODS = "upToPeriods";
@SerializedName(SERIALIZED_NAME_UP_TO_PERIODS)
private Long upToPeriods;
public static final String SERIALIZED_NAME_UP_TO_PERIODS_TYPE = "upToPeriodsType";
@SerializedName(SERIALIZED_NAME_UP_TO_PERIODS_TYPE)
private String upToPeriodsType;
public static final String SERIALIZED_NAME_WEEKLY_BILL_CYCLE_DAY = "weeklyBillCycleDay";
@SerializedName(SERIALIZED_NAME_WEEKLY_BILL_CYCLE_DAY)
private String weeklyBillCycleDay;
public CreateSubscriptionComponent() {
}
public CreateSubscriptionComponent amendedByOrderOn(String amendedByOrderOn) {
this.amendedByOrderOn = amendedByOrderOn;
return this;
}
/**
* The date when the rate plan charge is amended through an order or amendment. This field is to standardize the booking date information to increase audit ability and traceability of data between Zuora Billing and Zuora Revenue. It is mapped as the booking date for a sale order line in Zuora Revenue.
* @return amendedByOrderOn
*/
@javax.annotation.Nullable
public String getAmendedByOrderOn() {
return amendedByOrderOn;
}
public void setAmendedByOrderOn(String amendedByOrderOn) {
this.amendedByOrderOn = amendedByOrderOn;
}
public CreateSubscriptionComponent applyDiscountTo(String applyDiscountTo) {
this.applyDiscountTo = applyDiscountTo;
return this;
}
/**
* Specifies the type of charges that you want a specific discount to apply to. Values: * `ONETIME` * `RECURRING` * `USAGE` * `ONETIMERECURRING` * `ONETIMEUSAGE` * `RECURRINGUSAGE` * `ONETIMERECURRINGUSAGE`
* @return applyDiscountTo
*/
@javax.annotation.Nullable
public String getApplyDiscountTo() {
return applyDiscountTo;
}
public void setApplyDiscountTo(String applyDiscountTo) {
this.applyDiscountTo = applyDiscountTo;
}
public CreateSubscriptionComponent billCycleDay(String billCycleDay) {
this.billCycleDay = billCycleDay;
return this;
}
/**
* Sets the bill cycle day (BCD) for the charge. The BCD determines which day of the month the customer is billed. Values: `1`-`31`
* @return billCycleDay
*/
@javax.annotation.Nullable
public String getBillCycleDay() {
return billCycleDay;
}
public void setBillCycleDay(String billCycleDay) {
this.billCycleDay = billCycleDay;
}
public CreateSubscriptionComponent billCycleType(String billCycleType) {
this.billCycleType = billCycleType;
return this;
}
/**
* Specifies how to determine the billing day for the charge. When this field is set to `SpecificDayofMonth`, set the `BillCycleDay` field. When this field is set to `SpecificDayofWeek`, set the `weeklyBillCycleDay` field. Values: * `DefaultFromCustomer` * `SpecificDayofMonth` * `SubscriptionStartDay` * `ChargeTriggerDay` * `SpecificDayofWeek`
* @return billCycleType
*/
@javax.annotation.Nullable
public String getBillCycleType() {
return billCycleType;
}
public void setBillCycleType(String billCycleType) {
this.billCycleType = billCycleType;
}
public CreateSubscriptionComponent billingPeriod(String billingPeriod) {
this.billingPeriod = billingPeriod;
return this;
}
/**
* Billing period for the charge. The start day of the billing period is also called the bill cycle day (BCD). Values: * `Month` * `Quarter` * `Semi_Annual` * `Annual` * `Eighteen_Months` * `Two_Years` * `Three_Years` * `Five_Years` * `Specific_Months` * `Subscription_Term` * `Week` * `Specific_Weeks`
* @return billingPeriod
*/
@javax.annotation.Nullable
public String getBillingPeriod() {
return billingPeriod;
}
public void setBillingPeriod(String billingPeriod) {
this.billingPeriod = billingPeriod;
}
public CreateSubscriptionComponent billingPeriodAlignment(String billingPeriodAlignment) {
this.billingPeriodAlignment = billingPeriodAlignment;
return this;
}
/**
* Aligns charges within the same subscription if multiple charges begin on different dates. Values: * `AlignToCharge` * `AlignToSubscriptionStart` * `AlignToTermStart`
* @return billingPeriodAlignment
*/
@javax.annotation.Nullable
public String getBillingPeriodAlignment() {
return billingPeriodAlignment;
}
public void setBillingPeriodAlignment(String billingPeriodAlignment) {
this.billingPeriodAlignment = billingPeriodAlignment;
}
public CreateSubscriptionComponent billingTiming(String billingTiming) {
this.billingTiming = billingTiming;
return this;
}
/**
* Billing timing for the charge for recurring charge types. Not avaliable for one time, usage, and discount charges. Values: * `IN_ADVANCE` (default) * `IN_ARREARS`
* @return billingTiming
*/
@javax.annotation.Nullable
public String getBillingTiming() {
return billingTiming;
}
public void setBillingTiming(String billingTiming) {
this.billingTiming = billingTiming;
}
public CreateSubscriptionComponent chargeModelConfiguration(ChargeModelConfigurationForSubscription chargeModelConfiguration) {
this.chargeModelConfiguration = chargeModelConfiguration;
return this;
}
/**
* Get chargeModelConfiguration
* @return chargeModelConfiguration
*/
@javax.annotation.Nullable
public ChargeModelConfigurationForSubscription getChargeModelConfiguration() {
return chargeModelConfiguration;
}
public void setChargeModelConfiguration(ChargeModelConfigurationForSubscription chargeModelConfiguration) {
this.chargeModelConfiguration = chargeModelConfiguration;
}
public CreateSubscriptionComponent description(String description) {
this.description = description;
return this;
}
/**
* Description of the charge.
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public CreateSubscriptionComponent discountAmount(BigDecimal discountAmount) {
this.discountAmount = discountAmount;
return this;
}
/**
* Specifies the amount of fixed-amount discount.
* @return discountAmount
*/
@javax.annotation.Nullable
public BigDecimal getDiscountAmount() {
return discountAmount;
}
public void setDiscountAmount(BigDecimal discountAmount) {
this.discountAmount = discountAmount;
}
public CreateSubscriptionComponent discountLevel(String discountLevel) {
this.discountLevel = discountLevel;
return this;
}
/**
* Specifies if the discount applies to the product rate plan only, the entire subscription, or to any activity in the account. Values: * `rateplan` * `subscription` * `account`
* @return discountLevel
*/
@javax.annotation.Nullable
public String getDiscountLevel() {
return discountLevel;
}
public void setDiscountLevel(String discountLevel) {
this.discountLevel = discountLevel;
}
public CreateSubscriptionComponent discountPercentage(BigDecimal discountPercentage) {
this.discountPercentage = discountPercentage;
return this;
}
/**
* Percentage of discount for a percentage discount.
* @return discountPercentage
*/
@javax.annotation.Nullable
public BigDecimal getDiscountPercentage() {
return discountPercentage;
}
public void setDiscountPercentage(BigDecimal discountPercentage) {
this.discountPercentage = discountPercentage;
}
public CreateSubscriptionComponent endDateCondition(String endDateCondition) {
this.endDateCondition = endDateCondition;
return this;
}
/**
* Defines when the charge ends after the charge trigger date. If the subscription ends before the charge end date, the charge ends when the subscription ends. But if the subscription end date is subsequently changed through a Renewal, or Terms and Conditions amendment, the charge will end on the charge end date. Values: * `Subscription_End` * `Fixed_Period` * `Specific_End_Date` * `One_Time`
* @return endDateCondition
*/
@javax.annotation.Nullable
public String getEndDateCondition() {
return endDateCondition;
}
public void setEndDateCondition(String endDateCondition) {
this.endDateCondition = endDateCondition;
}
public CreateSubscriptionComponent excludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
return this;
}
/**
* The flag to exclude rate plan charge related invoice items, invoice item adjustments, credit memo items, and debit memo items from revenue accounting. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return excludeItemBillingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBillingFromRevenueAccounting() {
return excludeItemBillingFromRevenueAccounting;
}
public void setExcludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
}
public CreateSubscriptionComponent excludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
return this;
}
/**
* The flag to exclude rate plan charges from revenue accounting. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return excludeItemBookingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBookingFromRevenueAccounting() {
return excludeItemBookingFromRevenueAccounting;
}
public void setExcludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
}
public CreateSubscriptionComponent includedUnits(BigDecimal includedUnits) {
this.includedUnits = includedUnits;
return this;
}
/**
* Specifies the number of units in the base set of units for this charge. Must be >=`0`.
* @return includedUnits
*/
@javax.annotation.Nullable
public BigDecimal getIncludedUnits() {
return includedUnits;
}
public void setIncludedUnits(BigDecimal includedUnits) {
this.includedUnits = includedUnits;
}
public CreateSubscriptionComponent isAllocationEligible(Boolean isAllocationEligible) {
this.isAllocationEligible = isAllocationEligible;
return this;
}
/**
* This field is used to identify if the charge segment is allocation eligible in revenue recognition. **Note**: This feature is in the **Early Adopter** phase. If you want to use the feature, submit a request at <a href=\"https://support.zuora.com/\" target=\"_blank\">Zuora Global Support</a>, and we will evaluate whether the feature is suitable for your use cases.
* @return isAllocationEligible
*/
@javax.annotation.Nullable
public Boolean getIsAllocationEligible() {
return isAllocationEligible;
}
public void setIsAllocationEligible(Boolean isAllocationEligible) {
this.isAllocationEligible = isAllocationEligible;
}
public CreateSubscriptionComponent isUnbilled(Boolean isUnbilled) {
this.isUnbilled = isUnbilled;
return this;
}
/**
* This field is used to dictate how to perform the accounting during revenue recognition. **Note**: This feature is in the **Early Adopter** phase. If you want to use the feature, submit a request at <a href=\"https://support.zuora.com/\" target=\"_blank\">Zuora Global Support</a>, and we will evaluate whether the feature is suitable for your use cases.
* @return isUnbilled
*/
@javax.annotation.Nullable
public Boolean getIsUnbilled() {
return isUnbilled;
}
public void setIsUnbilled(Boolean isUnbilled) {
this.isUnbilled = isUnbilled;
}
public CreateSubscriptionComponent listPriceBase(String listPriceBase) {
this.listPriceBase = listPriceBase;
return this;
}
/**
* The list price base for the product rate plan charge. Values: * `Per_Billing_Period` * `Per_Month` * `Per_Week` * `Per_Year` * `Per_Specific_Months`
* @return listPriceBase
*/
@javax.annotation.Nullable
public String getListPriceBase() {
return listPriceBase;
}
public void setListPriceBase(String listPriceBase) {
this.listPriceBase = listPriceBase;
}
public CreateSubscriptionComponent number(String number) {
this.number = number;
return this;
}
/**
* Unique number that identifies the charge. Max 50 characters. System-generated if not provided.
* @return number
*/
@javax.annotation.Nullable
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public CreateSubscriptionComponent numberOfPeriods(Long numberOfPeriods) {
this.numberOfPeriods = numberOfPeriods;
return this;
}
/**
* Specifies the number of periods to use when calculating charges in an overage smoothing charge model.
* @return numberOfPeriods
*/
@javax.annotation.Nullable
public Long getNumberOfPeriods() {
return numberOfPeriods;
}
public void setNumberOfPeriods(Long numberOfPeriods) {
this.numberOfPeriods = numberOfPeriods;
}
public CreateSubscriptionComponent originalOrderDate(LocalDate originalOrderDate) {
this.originalOrderDate = originalOrderDate;
return this;
}
/**
* The date when the rate plan charge is created through an order or amendment. This field is not updatable. This field is to standardize the booking date information to increase audit ability and traceability of data between Zuora Billing and Zuora Revenue. It is mapped as the booking date for a sale order line in Zuora Revenue.
* @return originalOrderDate
*/
@javax.annotation.Nullable
public LocalDate getOriginalOrderDate() {
return originalOrderDate;
}
public void setOriginalOrderDate(LocalDate originalOrderDate) {
this.originalOrderDate = originalOrderDate;
}
public CreateSubscriptionComponent overagePrice(BigDecimal overagePrice) {
this.overagePrice = overagePrice;
return this;
}
/**
* Price for units over the allowed amount.
* @return overagePrice
*/
@javax.annotation.Nullable
public BigDecimal getOveragePrice() {
return overagePrice;
}
public void setOveragePrice(BigDecimal overagePrice) {
this.overagePrice = overagePrice;
}
public CreateSubscriptionComponent overageUnusedUnitsCreditOption(String overageUnusedUnitsCreditOption) {
this.overageUnusedUnitsCreditOption = overageUnusedUnitsCreditOption;
return this;
}
/**
* Determines whether to credit the customer with unused units of usage. Values: * `NoCredit` * `CreditBySpecificRate`
* @return overageUnusedUnitsCreditOption
*/
@javax.annotation.Nullable
public String getOverageUnusedUnitsCreditOption() {
return overageUnusedUnitsCreditOption;
}
public void setOverageUnusedUnitsCreditOption(String overageUnusedUnitsCreditOption) {
this.overageUnusedUnitsCreditOption = overageUnusedUnitsCreditOption;
}
public CreateSubscriptionComponent price(BigDecimal price) {
this.price = price;
return this;
}
/**
* Price for units in the subscription rate plan.
* @return price
*/
@javax.annotation.Nullable
public BigDecimal getPrice() {
return price;
}
public void setPrice(BigDecimal price) {
this.price = price;
}
public CreateSubscriptionComponent priceChangeOption(String priceChangeOption) {
this.priceChangeOption = priceChangeOption;
return this;
}
/**
* Applies an automatic price change when a termed subscription is renewed. The Billing Admin setting **Enable Automatic Price Change When Subscriptions are Renewed?** must be set to Yes to use this field. Values: * `NoChange` (default) * `SpecificPercentageValue` * `UseLatestProductCatalogPricing`
* @return priceChangeOption
*/
@javax.annotation.Nullable
public String getPriceChangeOption() {
return priceChangeOption;
}
public void setPriceChangeOption(String priceChangeOption) {
this.priceChangeOption = priceChangeOption;
}
public CreateSubscriptionComponent priceIncreasePercentage(BigDecimal priceIncreasePercentage) {
this.priceIncreasePercentage = priceIncreasePercentage;
return this;
}
/**
* Specifies the percentage to increase or decrease the price of a termed subscription's renewal. Required if you set the `PriceChangeOption` field to `SpecificPercentageValue`. Value must be a decimal between `-100` and `100`.
* @return priceIncreasePercentage
*/
@javax.annotation.Nullable
public BigDecimal getPriceIncreasePercentage() {
return priceIncreasePercentage;
}
public void setPriceIncreasePercentage(BigDecimal priceIncreasePercentage) {
this.priceIncreasePercentage = priceIncreasePercentage;
}
public CreateSubscriptionComponent productRatePlanChargeId(String productRatePlanChargeId) {
this.productRatePlanChargeId = productRatePlanChargeId;
return this;
}
/**
* ID of a product rate-plan charge for this subscription.
* @return productRatePlanChargeId
*/
@javax.annotation.Nonnull
public String getProductRatePlanChargeId() {
return productRatePlanChargeId;
}
public void setProductRatePlanChargeId(String productRatePlanChargeId) {
this.productRatePlanChargeId = productRatePlanChargeId;
}
public CreateSubscriptionComponent productRatePlanChargeNumber(String productRatePlanChargeNumber) {
this.productRatePlanChargeNumber = productRatePlanChargeNumber;
return this;
}
/**
* Number of a product rate-plan charge for this subscription.
* @return productRatePlanChargeNumber
*/
@javax.annotation.Nullable
public String getProductRatePlanChargeNumber() {
return productRatePlanChargeNumber;
}
public void setProductRatePlanChargeNumber(String productRatePlanChargeNumber) {
this.productRatePlanChargeNumber = productRatePlanChargeNumber;
}
public CreateSubscriptionComponent quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* Number of units. Must be a decimal >=`0`. When using `chargeOverrides` for creating subscriptions with recurring charge types, the `quantity` field must be populated when the charge model is \"Tiered Pricing\" or \"Volume Pricing\". It is not required for \"Flat Fee Pricing\" charge model.
* @return quantity
*/
@javax.annotation.Nullable
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public CreateSubscriptionComponent ratingGroup(String ratingGroup) {
this.ratingGroup = ratingGroup;
return this;
}
/**
* Specifies a rating group based on which usage records are rated. Possible values: - `ByBillingPeriod` (default): The rating is based on all the usages in a billing period. - `ByUsageStartDate`: The rating is based on all the usages on the same usage start date. - `ByUsageRecord`: The rating is based on each usage record. - `ByUsageUpload`: The rating is based on all the usages in a uploaded usage file (`.xls` or `.csv`). - `ByGroupId`: The rating is based on all the usages in a custom group. **Note:** - The `ByBillingPeriod` value can be applied for all charge models. - The `ByUsageStartDate`, `ByUsageRecord`, and `ByUsageUpload` values can only be applied for per unit, volume pricing, and tiered pricing charge models. - The `ByGroupId` value is only available if you have the Active Rating feature enabled. - Use this field only for Usage charges. One-Time Charges and Recurring Charges return `NULL`.
* @return ratingGroup
*/
@javax.annotation.Nullable
public String getRatingGroup() {
return ratingGroup;
}
public void setRatingGroup(String ratingGroup) {
this.ratingGroup = ratingGroup;
}
public CreateSubscriptionComponent specificBillingPeriod(Long specificBillingPeriod) {
this.specificBillingPeriod = specificBillingPeriod;
return this;
}
/**
* Specifies the number of month or week for the charges billing period. Required if you set the value of the `billingPeriod` field to `Specific_Months` or `Specific_Weeks`.
* @return specificBillingPeriod
*/
@javax.annotation.Nullable
public Long getSpecificBillingPeriod() {
return specificBillingPeriod;
}
public void setSpecificBillingPeriod(Long specificBillingPeriod) {
this.specificBillingPeriod = specificBillingPeriod;
}
public CreateSubscriptionComponent specificEndDate(LocalDate specificEndDate) {
this.specificEndDate = specificEndDate;
return this;
}
/**
* Defines when the charge ends after the charge trigger date. **note:** * This field is only applicable when the `endDateCondition` field is set to `Specific_End_Date`. * If the subscription ends before the specific end date, the charge ends when the subscription ends. But if the subscription end date is subsequently changed through a Renewal, or Terms and Conditions amendment, the charge will end on the specific end date.
* @return specificEndDate
*/
@javax.annotation.Nullable
public LocalDate getSpecificEndDate() {
return specificEndDate;
}
public void setSpecificEndDate(LocalDate specificEndDate) {
this.specificEndDate = specificEndDate;
}
public CreateSubscriptionComponent specificListPriceBase(Integer specificListPriceBase) {
this.specificListPriceBase = specificListPriceBase;
return this;
}
/**
* The number of months for the list price base of the charge. This field is required if you set the value of the `listPriceBase` field to `Per_Specific_Months`. **Note**: - This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Billing/Subscriptions/Product_Catalog/I_Annual_List_Price\" target=\"_blank\">Annual List Price</a> feature enabled. - The value of this field is `null` if you do not set the value of the `listPriceBase` field to `Per_Specific_Months`.
* minimum: 1
* maximum: 200
* @return specificListPriceBase
*/
@javax.annotation.Nullable
public Integer getSpecificListPriceBase() {
return specificListPriceBase;
}
public void setSpecificListPriceBase(Integer specificListPriceBase) {
this.specificListPriceBase = specificListPriceBase;
}
public CreateSubscriptionComponent tiers(List tiers) {
this.tiers = tiers;
return this;
}
public CreateSubscriptionComponent addTiersItem(Tier tiersItem) {
if (this.tiers == null) {
this.tiers = new ArrayList<>();
}
this.tiers.add(tiersItem);
return this;
}
/**
* Container for Volume, Tiered, or Tiered with Overage charge models. Supports the following charge types: * One-time * Recurring * Usage-based
* @return tiers
*/
@javax.annotation.Nullable
public List getTiers() {
return tiers;
}
public void setTiers(List tiers) {
this.tiers = tiers;
}
public CreateSubscriptionComponent triggerDate(LocalDate triggerDate) {
this.triggerDate = triggerDate;
return this;
}
/**
* Specifies when to start billing the customer for the charge. Required if the `triggerEvent` field is set to `USD`.
* @return triggerDate
*/
@javax.annotation.Nullable
public LocalDate getTriggerDate() {
return triggerDate;
}
public void setTriggerDate(LocalDate triggerDate) {
this.triggerDate = triggerDate;
}
public CreateSubscriptionComponent triggerEvent(String triggerEvent) {
this.triggerEvent = triggerEvent;
return this;
}
/**
* Specifies when to start billing the customer for the charge. Values: * `UCE` * `USA` * `UCA` * `USD`
* @return triggerEvent
*/
@javax.annotation.Nullable
public String getTriggerEvent() {
return triggerEvent;
}
public void setTriggerEvent(String triggerEvent) {
this.triggerEvent = triggerEvent;
}
public CreateSubscriptionComponent unusedUnitsCreditRates(BigDecimal unusedUnitsCreditRates) {
this.unusedUnitsCreditRates = unusedUnitsCreditRates;
return this;
}
/**
* Specifies the rate to credit a customer for unused units of usage. This field applies only for overage charge models when the `OverageUnusedUnitsCreditOption` field is set to `CreditBySpecificRate`.
* @return unusedUnitsCreditRates
*/
@javax.annotation.Nullable
public BigDecimal getUnusedUnitsCreditRates() {
return unusedUnitsCreditRates;
}
public void setUnusedUnitsCreditRates(BigDecimal unusedUnitsCreditRates) {
this.unusedUnitsCreditRates = unusedUnitsCreditRates;
}
public CreateSubscriptionComponent upToPeriods(Long upToPeriods) {
this.upToPeriods = upToPeriods;
return this;
}
/**
* Specifies the length of the period during which the charge is active. If this period ends before the subscription ends, the charge ends when this period ends. **Note:** You must use this field together with the `upToPeriodsType` field to specify the time period. * This field is applicable only when the `endDateCondition` field is set to `Fixed_Period`. * If the subscription end date is subsequently changed through a Renewal, or Terms and Conditions amendment, the charge end date will change accordingly up to the original period end.
* @return upToPeriods
*/
@javax.annotation.Nullable
public Long getUpToPeriods() {
return upToPeriods;
}
public void setUpToPeriods(Long upToPeriods) {
this.upToPeriods = upToPeriods;
}
public CreateSubscriptionComponent upToPeriodsType(String upToPeriodsType) {
this.upToPeriodsType = upToPeriodsType;
return this;
}
/**
* The period type used to define when the charge ends. Values: * `Billing_Periods` * `Days` * `Weeks` * `Months` * `Years` You must use this field together with the `upToPeriods` field to specify the time period. This field is applicable only when the `endDateCondition` field is set to `Fixed_Period`.
* @return upToPeriodsType
*/
@javax.annotation.Nullable
public String getUpToPeriodsType() {
return upToPeriodsType;
}
public void setUpToPeriodsType(String upToPeriodsType) {
this.upToPeriodsType = upToPeriodsType;
}
public CreateSubscriptionComponent weeklyBillCycleDay(String weeklyBillCycleDay) {
this.weeklyBillCycleDay = weeklyBillCycleDay;
return this;
}
/**
* Specifies which day of the week is the bill cycle day (BCD) for the charge. Values: * `Sunday` * `Monday` * `Tuesday` * `Wednesday` * `Thursday` * `Friday` * `Saturday`
* @return weeklyBillCycleDay
*/
@javax.annotation.Nullable
public String getWeeklyBillCycleDay() {
return weeklyBillCycleDay;
}
public void setWeeklyBillCycleDay(String weeklyBillCycleDay) {
this.weeklyBillCycleDay = weeklyBillCycleDay;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreateSubscriptionComponent instance itself
*/
public CreateSubscriptionComponent putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateSubscriptionComponent createSubscriptionComponent = (CreateSubscriptionComponent) o;
return Objects.equals(this.amendedByOrderOn, createSubscriptionComponent.amendedByOrderOn) &&
Objects.equals(this.applyDiscountTo, createSubscriptionComponent.applyDiscountTo) &&
Objects.equals(this.billCycleDay, createSubscriptionComponent.billCycleDay) &&
Objects.equals(this.billCycleType, createSubscriptionComponent.billCycleType) &&
Objects.equals(this.billingPeriod, createSubscriptionComponent.billingPeriod) &&
Objects.equals(this.billingPeriodAlignment, createSubscriptionComponent.billingPeriodAlignment) &&
Objects.equals(this.billingTiming, createSubscriptionComponent.billingTiming) &&
Objects.equals(this.chargeModelConfiguration, createSubscriptionComponent.chargeModelConfiguration) &&
Objects.equals(this.description, createSubscriptionComponent.description) &&
Objects.equals(this.discountAmount, createSubscriptionComponent.discountAmount) &&
Objects.equals(this.discountLevel, createSubscriptionComponent.discountLevel) &&
Objects.equals(this.discountPercentage, createSubscriptionComponent.discountPercentage) &&
Objects.equals(this.endDateCondition, createSubscriptionComponent.endDateCondition) &&
Objects.equals(this.excludeItemBillingFromRevenueAccounting, createSubscriptionComponent.excludeItemBillingFromRevenueAccounting) &&
Objects.equals(this.excludeItemBookingFromRevenueAccounting, createSubscriptionComponent.excludeItemBookingFromRevenueAccounting) &&
Objects.equals(this.includedUnits, createSubscriptionComponent.includedUnits) &&
Objects.equals(this.isAllocationEligible, createSubscriptionComponent.isAllocationEligible) &&
Objects.equals(this.isUnbilled, createSubscriptionComponent.isUnbilled) &&
Objects.equals(this.listPriceBase, createSubscriptionComponent.listPriceBase) &&
Objects.equals(this.number, createSubscriptionComponent.number) &&
Objects.equals(this.numberOfPeriods, createSubscriptionComponent.numberOfPeriods) &&
Objects.equals(this.originalOrderDate, createSubscriptionComponent.originalOrderDate) &&
Objects.equals(this.overagePrice, createSubscriptionComponent.overagePrice) &&
Objects.equals(this.overageUnusedUnitsCreditOption, createSubscriptionComponent.overageUnusedUnitsCreditOption) &&
Objects.equals(this.price, createSubscriptionComponent.price) &&
Objects.equals(this.priceChangeOption, createSubscriptionComponent.priceChangeOption) &&
Objects.equals(this.priceIncreasePercentage, createSubscriptionComponent.priceIncreasePercentage) &&
Objects.equals(this.productRatePlanChargeId, createSubscriptionComponent.productRatePlanChargeId) &&
Objects.equals(this.productRatePlanChargeNumber, createSubscriptionComponent.productRatePlanChargeNumber) &&
Objects.equals(this.quantity, createSubscriptionComponent.quantity) &&
Objects.equals(this.ratingGroup, createSubscriptionComponent.ratingGroup) &&
Objects.equals(this.specificBillingPeriod, createSubscriptionComponent.specificBillingPeriod) &&
Objects.equals(this.specificEndDate, createSubscriptionComponent.specificEndDate) &&
Objects.equals(this.specificListPriceBase, createSubscriptionComponent.specificListPriceBase) &&
Objects.equals(this.tiers, createSubscriptionComponent.tiers) &&
Objects.equals(this.triggerDate, createSubscriptionComponent.triggerDate) &&
Objects.equals(this.triggerEvent, createSubscriptionComponent.triggerEvent) &&
Objects.equals(this.unusedUnitsCreditRates, createSubscriptionComponent.unusedUnitsCreditRates) &&
Objects.equals(this.upToPeriods, createSubscriptionComponent.upToPeriods) &&
Objects.equals(this.upToPeriodsType, createSubscriptionComponent.upToPeriodsType) &&
Objects.equals(this.weeklyBillCycleDay, createSubscriptionComponent.weeklyBillCycleDay)&&
Objects.equals(this.additionalProperties, createSubscriptionComponent.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(amendedByOrderOn, applyDiscountTo, billCycleDay, billCycleType, billingPeriod, billingPeriodAlignment, billingTiming, chargeModelConfiguration, description, discountAmount, discountLevel, discountPercentage, endDateCondition, excludeItemBillingFromRevenueAccounting, excludeItemBookingFromRevenueAccounting, includedUnits, isAllocationEligible, isUnbilled, listPriceBase, number, numberOfPeriods, originalOrderDate, overagePrice, overageUnusedUnitsCreditOption, price, priceChangeOption, priceIncreasePercentage, productRatePlanChargeId, productRatePlanChargeNumber, quantity, ratingGroup, specificBillingPeriod, specificEndDate, specificListPriceBase, tiers, triggerDate, triggerEvent, unusedUnitsCreditRates, upToPeriods, upToPeriodsType, weeklyBillCycleDay, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateSubscriptionComponent {\n");
sb.append(" amendedByOrderOn: ").append(toIndentedString(amendedByOrderOn)).append("\n");
sb.append(" applyDiscountTo: ").append(toIndentedString(applyDiscountTo)).append("\n");
sb.append(" billCycleDay: ").append(toIndentedString(billCycleDay)).append("\n");
sb.append(" billCycleType: ").append(toIndentedString(billCycleType)).append("\n");
sb.append(" billingPeriod: ").append(toIndentedString(billingPeriod)).append("\n");
sb.append(" billingPeriodAlignment: ").append(toIndentedString(billingPeriodAlignment)).append("\n");
sb.append(" billingTiming: ").append(toIndentedString(billingTiming)).append("\n");
sb.append(" chargeModelConfiguration: ").append(toIndentedString(chargeModelConfiguration)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" discountAmount: ").append(toIndentedString(discountAmount)).append("\n");
sb.append(" discountLevel: ").append(toIndentedString(discountLevel)).append("\n");
sb.append(" discountPercentage: ").append(toIndentedString(discountPercentage)).append("\n");
sb.append(" endDateCondition: ").append(toIndentedString(endDateCondition)).append("\n");
sb.append(" excludeItemBillingFromRevenueAccounting: ").append(toIndentedString(excludeItemBillingFromRevenueAccounting)).append("\n");
sb.append(" excludeItemBookingFromRevenueAccounting: ").append(toIndentedString(excludeItemBookingFromRevenueAccounting)).append("\n");
sb.append(" includedUnits: ").append(toIndentedString(includedUnits)).append("\n");
sb.append(" isAllocationEligible: ").append(toIndentedString(isAllocationEligible)).append("\n");
sb.append(" isUnbilled: ").append(toIndentedString(isUnbilled)).append("\n");
sb.append(" listPriceBase: ").append(toIndentedString(listPriceBase)).append("\n");
sb.append(" number: ").append(toIndentedString(number)).append("\n");
sb.append(" numberOfPeriods: ").append(toIndentedString(numberOfPeriods)).append("\n");
sb.append(" originalOrderDate: ").append(toIndentedString(originalOrderDate)).append("\n");
sb.append(" overagePrice: ").append(toIndentedString(overagePrice)).append("\n");
sb.append(" overageUnusedUnitsCreditOption: ").append(toIndentedString(overageUnusedUnitsCreditOption)).append("\n");
sb.append(" price: ").append(toIndentedString(price)).append("\n");
sb.append(" priceChangeOption: ").append(toIndentedString(priceChangeOption)).append("\n");
sb.append(" priceIncreasePercentage: ").append(toIndentedString(priceIncreasePercentage)).append("\n");
sb.append(" productRatePlanChargeId: ").append(toIndentedString(productRatePlanChargeId)).append("\n");
sb.append(" productRatePlanChargeNumber: ").append(toIndentedString(productRatePlanChargeNumber)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" ratingGroup: ").append(toIndentedString(ratingGroup)).append("\n");
sb.append(" specificBillingPeriod: ").append(toIndentedString(specificBillingPeriod)).append("\n");
sb.append(" specificEndDate: ").append(toIndentedString(specificEndDate)).append("\n");
sb.append(" specificListPriceBase: ").append(toIndentedString(specificListPriceBase)).append("\n");
sb.append(" tiers: ").append(toIndentedString(tiers)).append("\n");
sb.append(" triggerDate: ").append(toIndentedString(triggerDate)).append("\n");
sb.append(" triggerEvent: ").append(toIndentedString(triggerEvent)).append("\n");
sb.append(" unusedUnitsCreditRates: ").append(toIndentedString(unusedUnitsCreditRates)).append("\n");
sb.append(" upToPeriods: ").append(toIndentedString(upToPeriods)).append("\n");
sb.append(" upToPeriodsType: ").append(toIndentedString(upToPeriodsType)).append("\n");
sb.append(" weeklyBillCycleDay: ").append(toIndentedString(weeklyBillCycleDay)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("amendedByOrderOn");
openapiFields.add("applyDiscountTo");
openapiFields.add("billCycleDay");
openapiFields.add("billCycleType");
openapiFields.add("billingPeriod");
openapiFields.add("billingPeriodAlignment");
openapiFields.add("billingTiming");
openapiFields.add("chargeModelConfiguration");
openapiFields.add("description");
openapiFields.add("discountAmount");
openapiFields.add("discountLevel");
openapiFields.add("discountPercentage");
openapiFields.add("endDateCondition");
openapiFields.add("excludeItemBillingFromRevenueAccounting");
openapiFields.add("excludeItemBookingFromRevenueAccounting");
openapiFields.add("includedUnits");
openapiFields.add("isAllocationEligible");
openapiFields.add("isUnbilled");
openapiFields.add("listPriceBase");
openapiFields.add("number");
openapiFields.add("numberOfPeriods");
openapiFields.add("originalOrderDate");
openapiFields.add("overagePrice");
openapiFields.add("overageUnusedUnitsCreditOption");
openapiFields.add("price");
openapiFields.add("priceChangeOption");
openapiFields.add("priceIncreasePercentage");
openapiFields.add("productRatePlanChargeId");
openapiFields.add("productRatePlanChargeNumber");
openapiFields.add("quantity");
openapiFields.add("ratingGroup");
openapiFields.add("specificBillingPeriod");
openapiFields.add("specificEndDate");
openapiFields.add("specificListPriceBase");
openapiFields.add("tiers");
openapiFields.add("triggerDate");
openapiFields.add("triggerEvent");
openapiFields.add("unusedUnitsCreditRates");
openapiFields.add("upToPeriods");
openapiFields.add("upToPeriodsType");
openapiFields.add("weeklyBillCycleDay");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("productRatePlanChargeId");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreateSubscriptionComponent
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreateSubscriptionComponent.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreateSubscriptionComponent is not found in the empty JSON string", CreateSubscriptionComponent.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : CreateSubscriptionComponent.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("amendedByOrderOn") != null && !jsonObj.get("amendedByOrderOn").isJsonNull()) && !jsonObj.get("amendedByOrderOn").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `amendedByOrderOn` to be a primitive type in the JSON string but got `%s`", jsonObj.get("amendedByOrderOn").toString()));
}
if ((jsonObj.get("applyDiscountTo") != null && !jsonObj.get("applyDiscountTo").isJsonNull()) && !jsonObj.get("applyDiscountTo").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `applyDiscountTo` to be a primitive type in the JSON string but got `%s`", jsonObj.get("applyDiscountTo").toString()));
}
if ((jsonObj.get("billCycleDay") != null && !jsonObj.get("billCycleDay").isJsonNull()) && !jsonObj.get("billCycleDay").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billCycleDay` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billCycleDay").toString()));
}
if ((jsonObj.get("billCycleType") != null && !jsonObj.get("billCycleType").isJsonNull()) && !jsonObj.get("billCycleType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billCycleType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billCycleType").toString()));
}
if ((jsonObj.get("billingPeriod") != null && !jsonObj.get("billingPeriod").isJsonNull()) && !jsonObj.get("billingPeriod").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billingPeriod` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billingPeriod").toString()));
}
if ((jsonObj.get("billingPeriodAlignment") != null && !jsonObj.get("billingPeriodAlignment").isJsonNull()) && !jsonObj.get("billingPeriodAlignment").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billingPeriodAlignment` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billingPeriodAlignment").toString()));
}
if ((jsonObj.get("billingTiming") != null && !jsonObj.get("billingTiming").isJsonNull()) && !jsonObj.get("billingTiming").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billingTiming` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billingTiming").toString()));
}
// validate the optional field `chargeModelConfiguration`
if (jsonObj.get("chargeModelConfiguration") != null && !jsonObj.get("chargeModelConfiguration").isJsonNull()) {
ChargeModelConfigurationForSubscription.validateJsonElement(jsonObj.get("chargeModelConfiguration"));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if ((jsonObj.get("discountLevel") != null && !jsonObj.get("discountLevel").isJsonNull()) && !jsonObj.get("discountLevel").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `discountLevel` to be a primitive type in the JSON string but got `%s`", jsonObj.get("discountLevel").toString()));
}
if ((jsonObj.get("endDateCondition") != null && !jsonObj.get("endDateCondition").isJsonNull()) && !jsonObj.get("endDateCondition").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `endDateCondition` to be a primitive type in the JSON string but got `%s`", jsonObj.get("endDateCondition").toString()));
}
if ((jsonObj.get("listPriceBase") != null && !jsonObj.get("listPriceBase").isJsonNull()) && !jsonObj.get("listPriceBase").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `listPriceBase` to be a primitive type in the JSON string but got `%s`", jsonObj.get("listPriceBase").toString()));
}
if ((jsonObj.get("number") != null && !jsonObj.get("number").isJsonNull()) && !jsonObj.get("number").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `number` to be a primitive type in the JSON string but got `%s`", jsonObj.get("number").toString()));
}
if ((jsonObj.get("overageUnusedUnitsCreditOption") != null && !jsonObj.get("overageUnusedUnitsCreditOption").isJsonNull()) && !jsonObj.get("overageUnusedUnitsCreditOption").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `overageUnusedUnitsCreditOption` to be a primitive type in the JSON string but got `%s`", jsonObj.get("overageUnusedUnitsCreditOption").toString()));
}
if ((jsonObj.get("priceChangeOption") != null && !jsonObj.get("priceChangeOption").isJsonNull()) && !jsonObj.get("priceChangeOption").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `priceChangeOption` to be a primitive type in the JSON string but got `%s`", jsonObj.get("priceChangeOption").toString()));
}
if (!jsonObj.get("productRatePlanChargeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanChargeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productRatePlanChargeId").toString()));
}
if ((jsonObj.get("productRatePlanChargeNumber") != null && !jsonObj.get("productRatePlanChargeNumber").isJsonNull()) && !jsonObj.get("productRatePlanChargeNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanChargeNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productRatePlanChargeNumber").toString()));
}
if ((jsonObj.get("ratingGroup") != null && !jsonObj.get("ratingGroup").isJsonNull()) && !jsonObj.get("ratingGroup").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ratingGroup` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ratingGroup").toString()));
}
if (jsonObj.get("tiers") != null && !jsonObj.get("tiers").isJsonNull()) {
JsonArray jsonArraytiers = jsonObj.getAsJsonArray("tiers");
if (jsonArraytiers != null) {
// ensure the json data is an array
if (!jsonObj.get("tiers").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `tiers` to be an array in the JSON string but got `%s`", jsonObj.get("tiers").toString()));
}
// validate the optional field `tiers` (array)
for (int i = 0; i < jsonArraytiers.size(); i++) {
Tier.validateJsonElement(jsonArraytiers.get(i));
};
}
}
if ((jsonObj.get("triggerEvent") != null && !jsonObj.get("triggerEvent").isJsonNull()) && !jsonObj.get("triggerEvent").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `triggerEvent` to be a primitive type in the JSON string but got `%s`", jsonObj.get("triggerEvent").toString()));
}
if ((jsonObj.get("upToPeriodsType") != null && !jsonObj.get("upToPeriodsType").isJsonNull()) && !jsonObj.get("upToPeriodsType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `upToPeriodsType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("upToPeriodsType").toString()));
}
if ((jsonObj.get("weeklyBillCycleDay") != null && !jsonObj.get("weeklyBillCycleDay").isJsonNull()) && !jsonObj.get("weeklyBillCycleDay").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `weeklyBillCycleDay` to be a primitive type in the JSON string but got `%s`", jsonObj.get("weeklyBillCycleDay").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreateSubscriptionComponent.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreateSubscriptionComponent' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreateSubscriptionComponent.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreateSubscriptionComponent value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreateSubscriptionComponent read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreateSubscriptionComponent instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreateSubscriptionComponent given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreateSubscriptionComponent
* @throws IOException if the JSON string is invalid with respect to CreateSubscriptionComponent
*/
public static CreateSubscriptionComponent fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreateSubscriptionComponent.class);
}
/**
* Convert an instance of CreateSubscriptionComponent to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy