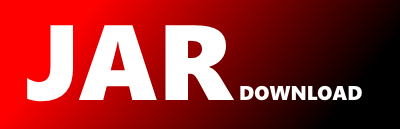
com.zuora.model.CreateSubscriptionRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.CreateSubscriptionRatePlan;
import java.io.IOException;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* CreateSubscriptionRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreateSubscriptionRequest {
public static final String SERIALIZED_NAME_CPQ_BUNDLE_JSON_ID_Q_T = "CpqBundleJsonId__QT";
@SerializedName(SERIALIZED_NAME_CPQ_BUNDLE_JSON_ID_Q_T)
private String cpqBundleJsonIdQT;
public static final String SERIALIZED_NAME_OPPORTUNITY_CLOSE_DATE_Q_T = "OpportunityCloseDate__QT";
@SerializedName(SERIALIZED_NAME_OPPORTUNITY_CLOSE_DATE_Q_T)
private LocalDate opportunityCloseDateQT;
public static final String SERIALIZED_NAME_OPPORTUNITY_NAME_Q_T = "OpportunityName__QT";
@SerializedName(SERIALIZED_NAME_OPPORTUNITY_NAME_Q_T)
private String opportunityNameQT;
public static final String SERIALIZED_NAME_QUOTE_BUSINESS_TYPE_Q_T = "QuoteBusinessType__QT";
@SerializedName(SERIALIZED_NAME_QUOTE_BUSINESS_TYPE_Q_T)
private String quoteBusinessTypeQT;
public static final String SERIALIZED_NAME_QUOTE_NUMBER_Q_T = "QuoteNumber__QT";
@SerializedName(SERIALIZED_NAME_QUOTE_NUMBER_Q_T)
private String quoteNumberQT;
public static final String SERIALIZED_NAME_QUOTE_TYPE_Q_T = "QuoteType__QT";
@SerializedName(SERIALIZED_NAME_QUOTE_TYPE_Q_T)
private String quoteTypeQT;
public static final String SERIALIZED_NAME_INTEGRATION_ID_N_S = "IntegrationId__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_ID_N_S)
private String integrationIdNS;
public static final String SERIALIZED_NAME_INTEGRATION_STATUS_N_S = "IntegrationStatus__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_STATUS_N_S)
private String integrationStatusNS;
public static final String SERIALIZED_NAME_PROJECT_N_S = "Project__NS";
@SerializedName(SERIALIZED_NAME_PROJECT_N_S)
private String projectNS;
public static final String SERIALIZED_NAME_SALES_ORDER_N_S = "SalesOrder__NS";
@SerializedName(SERIALIZED_NAME_SALES_ORDER_N_S)
private String salesOrderNS;
public static final String SERIALIZED_NAME_SYNC_DATE_N_S = "SyncDate__NS";
@SerializedName(SERIALIZED_NAME_SYNC_DATE_N_S)
private String syncDateNS;
public static final String SERIALIZED_NAME_ACCOUNT_KEY = "accountKey";
@SerializedName(SERIALIZED_NAME_ACCOUNT_KEY)
private String accountKey;
public static final String SERIALIZED_NAME_APPLICATION_ORDER = "applicationOrder";
@SerializedName(SERIALIZED_NAME_APPLICATION_ORDER)
private List applicationOrder;
public static final String SERIALIZED_NAME_APPLY_CREDIT = "applyCredit";
@SerializedName(SERIALIZED_NAME_APPLY_CREDIT)
private Boolean applyCredit;
public static final String SERIALIZED_NAME_APPLY_CREDIT_BALANCE = "applyCreditBalance";
@SerializedName(SERIALIZED_NAME_APPLY_CREDIT_BALANCE)
private Boolean applyCreditBalance;
public static final String SERIALIZED_NAME_AUTO_RENEW = "autoRenew";
@SerializedName(SERIALIZED_NAME_AUTO_RENEW)
private Boolean autoRenew = false;
public static final String SERIALIZED_NAME_COLLECT = "collect";
@SerializedName(SERIALIZED_NAME_COLLECT)
private Boolean collect;
public static final String SERIALIZED_NAME_CONTRACT_EFFECTIVE_DATE = "contractEffectiveDate";
@SerializedName(SERIALIZED_NAME_CONTRACT_EFFECTIVE_DATE)
private LocalDate contractEffectiveDate;
public static final String SERIALIZED_NAME_CREDIT_MEMO_REASON_CODE = "creditMemoReasonCode";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_REASON_CODE)
private String creditMemoReasonCode;
public static final String SERIALIZED_NAME_CUSTOMER_ACCEPTANCE_DATE = "customerAcceptanceDate";
@SerializedName(SERIALIZED_NAME_CUSTOMER_ACCEPTANCE_DATE)
private LocalDate customerAcceptanceDate;
public static final String SERIALIZED_NAME_DOCUMENT_DATE = "documentDate";
@SerializedName(SERIALIZED_NAME_DOCUMENT_DATE)
private LocalDate documentDate;
/**
* An enum field on the Subscription object to indicate the name of a third-party store. This field is used to represent subscriptions created through third-party stores.
*/
@JsonAdapter(ExternallyManagedByEnum.Adapter.class)
public enum ExternallyManagedByEnum {
AMAZON("Amazon"),
APPLE("Apple"),
GOOGLE("Google"),
ROKU("Roku");
private String value;
ExternallyManagedByEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ExternallyManagedByEnum fromValue(String value) {
for (ExternallyManagedByEnum b : ExternallyManagedByEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ExternallyManagedByEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ExternallyManagedByEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ExternallyManagedByEnum.fromValue(value);
}
}
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
String value = jsonElement.getAsString();
ExternallyManagedByEnum.fromValue(value);
}
}
public static final String SERIALIZED_NAME_EXTERNALLY_MANAGED_BY = "externallyManagedBy";
@SerializedName(SERIALIZED_NAME_EXTERNALLY_MANAGED_BY)
private ExternallyManagedByEnum externallyManagedBy;
public static final String SERIALIZED_NAME_GATEWAY_ID = "gatewayId";
@SerializedName(SERIALIZED_NAME_GATEWAY_ID)
private String gatewayId;
public static final String SERIALIZED_NAME_INITIAL_TERM = "initialTerm";
@SerializedName(SERIALIZED_NAME_INITIAL_TERM)
private Long initialTerm;
public static final String SERIALIZED_NAME_INITIAL_TERM_PERIOD_TYPE = "initialTermPeriodType";
@SerializedName(SERIALIZED_NAME_INITIAL_TERM_PERIOD_TYPE)
private String initialTermPeriodType;
public static final String SERIALIZED_NAME_INVOICE = "invoice";
@Deprecated
@SerializedName(SERIALIZED_NAME_INVOICE)
private Boolean invoice;
public static final String SERIALIZED_NAME_INVOICE_COLLECT = "invoiceCollect";
@Deprecated
@SerializedName(SERIALIZED_NAME_INVOICE_COLLECT)
private Boolean invoiceCollect;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_KEY = "invoiceOwnerAccountKey";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_KEY)
private String invoiceOwnerAccountKey;
public static final String SERIALIZED_NAME_INVOICE_SEPARATELY = "invoiceSeparately";
@SerializedName(SERIALIZED_NAME_INVOICE_SEPARATELY)
private Boolean invoiceSeparately;
public static final String SERIALIZED_NAME_INVOICE_TARGET_DATE = "invoiceTargetDate";
@Deprecated
@SerializedName(SERIALIZED_NAME_INVOICE_TARGET_DATE)
private LocalDate invoiceTargetDate;
public static final String SERIALIZED_NAME_LAST_BOOKING_DATE = "lastBookingDate";
@SerializedName(SERIALIZED_NAME_LAST_BOOKING_DATE)
private LocalDate lastBookingDate;
public static final String SERIALIZED_NAME_NOTES = "notes";
@SerializedName(SERIALIZED_NAME_NOTES)
private String notes;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "paymentMethodId";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_PREPAYMENT = "prepayment";
@SerializedName(SERIALIZED_NAME_PREPAYMENT)
private Boolean prepayment;
public static final String SERIALIZED_NAME_RENEWAL_SETTING = "renewalSetting";
@SerializedName(SERIALIZED_NAME_RENEWAL_SETTING)
private String renewalSetting;
public static final String SERIALIZED_NAME_RENEWAL_TERM = "renewalTerm";
@SerializedName(SERIALIZED_NAME_RENEWAL_TERM)
private Long renewalTerm;
public static final String SERIALIZED_NAME_RENEWAL_TERM_PERIOD_TYPE = "renewalTermPeriodType";
@SerializedName(SERIALIZED_NAME_RENEWAL_TERM_PERIOD_TYPE)
private String renewalTermPeriodType;
public static final String SERIALIZED_NAME_RUN_BILLING = "runBilling";
@SerializedName(SERIALIZED_NAME_RUN_BILLING)
private Boolean runBilling;
public static final String SERIALIZED_NAME_SERVICE_ACTIVATION_DATE = "serviceActivationDate";
@SerializedName(SERIALIZED_NAME_SERVICE_ACTIVATION_DATE)
private LocalDate serviceActivationDate;
public static final String SERIALIZED_NAME_SUBSCRIBE_TO_RATE_PLANS = "subscribeToRatePlans";
@SerializedName(SERIALIZED_NAME_SUBSCRIBE_TO_RATE_PLANS)
private List subscribeToRatePlans;
public static final String SERIALIZED_NAME_SUBSCRIPTION_NUMBER = "subscriptionNumber";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_NUMBER)
private String subscriptionNumber;
public static final String SERIALIZED_NAME_TARGET_DATE = "targetDate";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public static final String SERIALIZED_NAME_TERM_START_DATE = "termStartDate";
@SerializedName(SERIALIZED_NAME_TERM_START_DATE)
private LocalDate termStartDate;
public static final String SERIALIZED_NAME_TERM_TYPE = "termType";
@SerializedName(SERIALIZED_NAME_TERM_TYPE)
private String termType;
public CreateSubscriptionRequest() {
}
public CreateSubscriptionRequest cpqBundleJsonIdQT(String cpqBundleJsonIdQT) {
this.cpqBundleJsonIdQT = cpqBundleJsonIdQT;
return this;
}
/**
* The Bundle product structures from Zuora Quotes if you utilize Bundling in Salesforce. Do not change the value in this field.
* @return cpqBundleJsonIdQT
*/
@javax.annotation.Nullable
public String getCpqBundleJsonIdQT() {
return cpqBundleJsonIdQT;
}
public void setCpqBundleJsonIdQT(String cpqBundleJsonIdQT) {
this.cpqBundleJsonIdQT = cpqBundleJsonIdQT;
}
public CreateSubscriptionRequest opportunityCloseDateQT(LocalDate opportunityCloseDateQT) {
this.opportunityCloseDateQT = opportunityCloseDateQT;
return this;
}
/**
* The closing date of the Opportunity. This field is used in Zuora data sources to report on Subscription metrics. If the subscription originated from Zuora Quotes, the value is populated with the value from Zuora Quotes.
* @return opportunityCloseDateQT
*/
@javax.annotation.Nullable
public LocalDate getOpportunityCloseDateQT() {
return opportunityCloseDateQT;
}
public void setOpportunityCloseDateQT(LocalDate opportunityCloseDateQT) {
this.opportunityCloseDateQT = opportunityCloseDateQT;
}
public CreateSubscriptionRequest opportunityNameQT(String opportunityNameQT) {
this.opportunityNameQT = opportunityNameQT;
return this;
}
/**
* The unique identifier of the Opportunity. This field is used in Zuora data sources to report on Subscription metrics. If the subscription originated from Zuora Quotes, the value is populated with the value from Zuora Quotes.
* @return opportunityNameQT
*/
@javax.annotation.Nullable
public String getOpportunityNameQT() {
return opportunityNameQT;
}
public void setOpportunityNameQT(String opportunityNameQT) {
this.opportunityNameQT = opportunityNameQT;
}
public CreateSubscriptionRequest quoteBusinessTypeQT(String quoteBusinessTypeQT) {
this.quoteBusinessTypeQT = quoteBusinessTypeQT;
return this;
}
/**
* The specific identifier for the type of business transaction the Quote represents such as New, Upsell, Downsell, Renewal or Churn. This field is used in Zuora data sources to report on Subscription metrics. If the subscription originated from Zuora Quotes, the value is populated with the value from Zuora Quotes.
* @return quoteBusinessTypeQT
*/
@javax.annotation.Nullable
public String getQuoteBusinessTypeQT() {
return quoteBusinessTypeQT;
}
public void setQuoteBusinessTypeQT(String quoteBusinessTypeQT) {
this.quoteBusinessTypeQT = quoteBusinessTypeQT;
}
public CreateSubscriptionRequest quoteNumberQT(String quoteNumberQT) {
this.quoteNumberQT = quoteNumberQT;
return this;
}
/**
* The unique identifier of the Quote. This field is used in Zuora data sources to report on Subscription metrics. If the subscription originated from Zuora Quotes, the value is populated with the value from Zuora Quotes.
* @return quoteNumberQT
*/
@javax.annotation.Nullable
public String getQuoteNumberQT() {
return quoteNumberQT;
}
public void setQuoteNumberQT(String quoteNumberQT) {
this.quoteNumberQT = quoteNumberQT;
}
public CreateSubscriptionRequest quoteTypeQT(String quoteTypeQT) {
this.quoteTypeQT = quoteTypeQT;
return this;
}
/**
* The Quote type that represents the subscription lifecycle stage such as New, Amendment, Renew or Cancel. This field is used in Zuora data sources to report on Subscription metrics. If the subscription originated from Zuora Quotes, the value is populated with the value from Zuora Quotes.
* @return quoteTypeQT
*/
@javax.annotation.Nullable
public String getQuoteTypeQT() {
return quoteTypeQT;
}
public void setQuoteTypeQT(String quoteTypeQT) {
this.quoteTypeQT = quoteTypeQT;
}
public CreateSubscriptionRequest integrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
return this;
}
/**
* ID of the corresponding object in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationIdNS
*/
@javax.annotation.Nullable
public String getIntegrationIdNS() {
return integrationIdNS;
}
public void setIntegrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
}
public CreateSubscriptionRequest integrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
return this;
}
/**
* Status of the subscription's synchronization with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationStatusNS
*/
@javax.annotation.Nullable
public String getIntegrationStatusNS() {
return integrationStatusNS;
}
public void setIntegrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
}
public CreateSubscriptionRequest projectNS(String projectNS) {
this.projectNS = projectNS;
return this;
}
/**
* The NetSuite project that the subscription was created from. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return projectNS
*/
@javax.annotation.Nullable
public String getProjectNS() {
return projectNS;
}
public void setProjectNS(String projectNS) {
this.projectNS = projectNS;
}
public CreateSubscriptionRequest salesOrderNS(String salesOrderNS) {
this.salesOrderNS = salesOrderNS;
return this;
}
/**
* The NetSuite sales order than the subscription was created from. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return salesOrderNS
*/
@javax.annotation.Nullable
public String getSalesOrderNS() {
return salesOrderNS;
}
public void setSalesOrderNS(String salesOrderNS) {
this.salesOrderNS = salesOrderNS;
}
public CreateSubscriptionRequest syncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
return this;
}
/**
* Date when the subscription was synchronized with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return syncDateNS
*/
@javax.annotation.Nullable
public String getSyncDateNS() {
return syncDateNS;
}
public void setSyncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
}
public CreateSubscriptionRequest accountKey(String accountKey) {
this.accountKey = accountKey;
return this;
}
/**
* Customer account number or ID
* @return accountKey
*/
@javax.annotation.Nonnull
public String getAccountKey() {
return accountKey;
}
public void setAccountKey(String accountKey) {
this.accountKey = accountKey;
}
public CreateSubscriptionRequest applicationOrder(List applicationOrder) {
this.applicationOrder = applicationOrder;
return this;
}
public CreateSubscriptionRequest addApplicationOrderItem(String applicationOrderItem) {
if (this.applicationOrder == null) {
this.applicationOrder = new ArrayList<>();
}
this.applicationOrder.add(applicationOrderItem);
return this;
}
/**
* The priority order to apply credit memos and/or unapplied payments to an invoice. Possible item values are: `CreditMemo`, `UnappliedPayment`. **Note:** - This field is valid only if the `applyCredit` field is set to `true`. - If no value is specified for this field, the default priority order is used, [\"CreditMemo\", \"UnappliedPayment\"], to apply credit memos first and then apply unapplied payments. - If only one item is specified, only the items of the spedified type are applied to invoices. For example, if the value is `[\"CreditMemo\"]`, only credit memos are used to apply to invoices.
* @return applicationOrder
*/
@javax.annotation.Nullable
public List getApplicationOrder() {
return applicationOrder;
}
public void setApplicationOrder(List applicationOrder) {
this.applicationOrder = applicationOrder;
}
public CreateSubscriptionRequest applyCredit(Boolean applyCredit) {
this.applyCredit = applyCredit;
return this;
}
/**
* If the value is true, the credit memo or unapplied payment on the order account will be automatically applied to the invoices generated by this order. The credit memo generated by this order will not be automatically applied to any invoices. **Note:** This field is only available if you have [Invoice Settlement](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement) enabled. The Invoice Settlement feature is generally available as of Zuora Billing Release 296 (March 2021). This feature includes Unapplied Payments, Credit and Debit Memo, and Invoice Item Settlement. If you want to enable Invoice Settlement, see [Invoice Settlement Enablement and Checklist Guide](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement/Invoice_Settlement_Migration_Checklist_and_Guide) for more information.
* @return applyCredit
*/
@javax.annotation.Nullable
public Boolean getApplyCredit() {
return applyCredit;
}
public void setApplyCredit(Boolean applyCredit) {
this.applyCredit = applyCredit;
}
public CreateSubscriptionRequest applyCreditBalance(Boolean applyCreditBalance) {
this.applyCreditBalance = applyCreditBalance;
return this;
}
/**
* Whether to automatically apply a credit balance to an invoice. If the value is `true`, the credit balance is applied to the invoice. If the value is `false`, no action is taken. To view the credit balance adjustment, retrieve the details of the invoice using the Get Invoices method. Prerequisite: `invoice` must be `true`. **Note:** - If you are using the field `invoiceCollect` rather than the field `invoice`, the `invoiceCollect` value must be `true`. - This field is deprecated if you have the Invoice Settlement feature enabled.
* @return applyCreditBalance
*/
@javax.annotation.Nullable
public Boolean getApplyCreditBalance() {
return applyCreditBalance;
}
public void setApplyCreditBalance(Boolean applyCreditBalance) {
this.applyCreditBalance = applyCreditBalance;
}
public CreateSubscriptionRequest autoRenew(Boolean autoRenew) {
this.autoRenew = autoRenew;
return this;
}
/**
* If true, this subscription automatically renews at the end of the subscription term. This field is only required if the `termType` field is set to `TERMED`.
* @return autoRenew
*/
@javax.annotation.Nullable
public Boolean getAutoRenew() {
return autoRenew;
}
public void setAutoRenew(Boolean autoRenew) {
this.autoRenew = autoRenew;
}
public CreateSubscriptionRequest collect(Boolean collect) {
this.collect = collect;
return this;
}
/**
* Collects an automatic payment for a subscription. The collection generated in this operation is only for this subscription, not for the entire customer account. If the value is `true`, the automatic payment is collected. If the value is `false`, no action is taken. Prerequisite: The `invoice` or `runBilling` field must be `true`. **Note**: This field is only available if you set the `zuora-version` request header to `196.0` or later.
* @return collect
*/
@javax.annotation.Nullable
public Boolean getCollect() {
return collect;
}
public void setCollect(Boolean collect) {
this.collect = collect;
}
public CreateSubscriptionRequest contractEffectiveDate(LocalDate contractEffectiveDate) {
this.contractEffectiveDate = contractEffectiveDate;
return this;
}
/**
* Effective contract date for this subscription, as yyyy-mm-dd
* @return contractEffectiveDate
*/
@javax.annotation.Nonnull
public LocalDate getContractEffectiveDate() {
return contractEffectiveDate;
}
public void setContractEffectiveDate(LocalDate contractEffectiveDate) {
this.contractEffectiveDate = contractEffectiveDate;
}
public CreateSubscriptionRequest creditMemoReasonCode(String creditMemoReasonCode) {
this.creditMemoReasonCode = creditMemoReasonCode;
return this;
}
/**
* A code identifying the reason for the credit memo transaction that is generated by the request. The value must be an existing reason code. If you do not pass the field or pass the field with empty value, Zuora uses the default reason code.
* @return creditMemoReasonCode
*/
@javax.annotation.Nullable
public String getCreditMemoReasonCode() {
return creditMemoReasonCode;
}
public void setCreditMemoReasonCode(String creditMemoReasonCode) {
this.creditMemoReasonCode = creditMemoReasonCode;
}
public CreateSubscriptionRequest customerAcceptanceDate(LocalDate customerAcceptanceDate) {
this.customerAcceptanceDate = customerAcceptanceDate;
return this;
}
/**
* The date on which the services or products within a subscription have been accepted by the customer, as yyyy-mm-dd. Default value is dependent on the value of other fields. See **Notes** section for more details.
* @return customerAcceptanceDate
*/
@javax.annotation.Nullable
public LocalDate getCustomerAcceptanceDate() {
return customerAcceptanceDate;
}
public void setCustomerAcceptanceDate(LocalDate customerAcceptanceDate) {
this.customerAcceptanceDate = customerAcceptanceDate;
}
public CreateSubscriptionRequest documentDate(LocalDate documentDate) {
this.documentDate = documentDate;
return this;
}
/**
* The date of the billing document, in `yyyy-mm-dd` format. It represents the invoice date for invoices, credit memo date for credit memos, and debit memo date for debit memos. - If this field is specified, the specified date is used as the billing document date. - If this field is not specified, the date specified in the `targetDate` is used as the billing document date.
* @return documentDate
*/
@javax.annotation.Nullable
public LocalDate getDocumentDate() {
return documentDate;
}
public void setDocumentDate(LocalDate documentDate) {
this.documentDate = documentDate;
}
public CreateSubscriptionRequest externallyManagedBy(ExternallyManagedByEnum externallyManagedBy) {
this.externallyManagedBy = externallyManagedBy;
return this;
}
/**
* An enum field on the Subscription object to indicate the name of a third-party store. This field is used to represent subscriptions created through third-party stores.
* @return externallyManagedBy
*/
@javax.annotation.Nullable
public ExternallyManagedByEnum getExternallyManagedBy() {
return externallyManagedBy;
}
public void setExternallyManagedBy(ExternallyManagedByEnum externallyManagedBy) {
this.externallyManagedBy = externallyManagedBy;
}
public CreateSubscriptionRequest gatewayId(String gatewayId) {
this.gatewayId = gatewayId;
return this;
}
/**
* The ID of the payment gateway instance. For example, `2c92c0f86078c4d5016091674bcc3e92`.
* @return gatewayId
*/
@javax.annotation.Nullable
public String getGatewayId() {
return gatewayId;
}
public void setGatewayId(String gatewayId) {
this.gatewayId = gatewayId;
}
public CreateSubscriptionRequest initialTerm(Long initialTerm) {
this.initialTerm = initialTerm;
return this;
}
/**
* The length of the period for the first subscription term. If `termType` is `TERMED`, then this field is required, and the value must be greater than `0`. If `termType` is `EVERGREEN`, this field is ignored.
* @return initialTerm
*/
@javax.annotation.Nullable
public Long getInitialTerm() {
return initialTerm;
}
public void setInitialTerm(Long initialTerm) {
this.initialTerm = initialTerm;
}
public CreateSubscriptionRequest initialTermPeriodType(String initialTermPeriodType) {
this.initialTermPeriodType = initialTermPeriodType;
return this;
}
/**
* The period type for the first subscription term. This field is used with the `InitialTerm` field to specify the initial subscription term. Values are: * `Month` (default) * `Year` * `Day` * `Week`
* @return initialTermPeriodType
*/
@javax.annotation.Nullable
public String getInitialTermPeriodType() {
return initialTermPeriodType;
}
public void setInitialTermPeriodType(String initialTermPeriodType) {
this.initialTermPeriodType = initialTermPeriodType;
}
@Deprecated
public CreateSubscriptionRequest invoice(Boolean invoice) {
this.invoice = invoice;
return this;
}
/**
* **Note:** This field has been replaced by the `runBilling` field. The `invoice` field is only available for backward compatibility. Creates an invoice for a subscription. The invoice generated in this operation is only for this subscription, not for the entire customer account. If the value is `true`, an invoice is created. If the value is `false`, no action is taken. The default value is `true`. This field is in Zuora REST API version control. Supported minor versions are `196.0` and `207.0`. To use this field in the method, you must set the zuora-version parameter to the minor version number in the request header.
* @return invoice
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public Boolean getInvoice() {
return invoice;
}
@Deprecated
public void setInvoice(Boolean invoice) {
this.invoice = invoice;
}
@Deprecated
public CreateSubscriptionRequest invoiceCollect(Boolean invoiceCollect) {
this.invoiceCollect = invoiceCollect;
return this;
}
/**
* **Note:** This field has been replaced by the `invoice` field and the `collect` field. `invoiceCollect` is available only for backward compatibility. If this field is set to `true`, an invoice is generated and payment collected automatically during the subscription process. If `false`, no invoicing or payment takes place. The invoice generated in this operation is only for this subscription, not for the entire customer account. **Note**: This field is only available if you set the `zuora-version` request header to `186.0`, `187.0`, `188.0`, or `189.0`.
* @return invoiceCollect
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public Boolean getInvoiceCollect() {
return invoiceCollect;
}
@Deprecated
public void setInvoiceCollect(Boolean invoiceCollect) {
this.invoiceCollect = invoiceCollect;
}
public CreateSubscriptionRequest invoiceOwnerAccountKey(String invoiceOwnerAccountKey) {
this.invoiceOwnerAccountKey = invoiceOwnerAccountKey;
return this;
}
/**
* Invoice owner account number or ID. **Note:** This feature is in **Limited Availability**. If you wish to have access to the feature, submit a request at [Zuora Global Support](http://support.zuora.com/).
* @return invoiceOwnerAccountKey
*/
@javax.annotation.Nullable
public String getInvoiceOwnerAccountKey() {
return invoiceOwnerAccountKey;
}
public void setInvoiceOwnerAccountKey(String invoiceOwnerAccountKey) {
this.invoiceOwnerAccountKey = invoiceOwnerAccountKey;
}
public CreateSubscriptionRequest invoiceSeparately(Boolean invoiceSeparately) {
this.invoiceSeparately = invoiceSeparately;
return this;
}
/**
* Separates a single subscription from other subscriptions and invoices the charge independently. If the value is `true`, the subscription is billed separately from other subscriptions. If the value is `false`, the subscription is included with other subscriptions in the account invoice. The default value is `false`. Prerequisite: The default subscription setting Enable Subscriptions to be Invoiced Separately must be set to Yes.
* @return invoiceSeparately
*/
@javax.annotation.Nullable
public Boolean getInvoiceSeparately() {
return invoiceSeparately;
}
public void setInvoiceSeparately(Boolean invoiceSeparately) {
this.invoiceSeparately = invoiceSeparately;
}
@Deprecated
public CreateSubscriptionRequest invoiceTargetDate(LocalDate invoiceTargetDate) {
this.invoiceTargetDate = invoiceTargetDate;
return this;
}
/**
* **Note:** This field has been replaced by the `targetDate` field. The `invoiceTargetDate` field is only available for backward compatibility. Date through which to calculate charges if an invoice is generated, as yyyy-mm-dd. Default is current date. This field is in Zuora REST API version control. Supported minor versions are `207.0` and earlier.
* @return invoiceTargetDate
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public LocalDate getInvoiceTargetDate() {
return invoiceTargetDate;
}
@Deprecated
public void setInvoiceTargetDate(LocalDate invoiceTargetDate) {
this.invoiceTargetDate = invoiceTargetDate;
}
public CreateSubscriptionRequest lastBookingDate(LocalDate lastBookingDate) {
this.lastBookingDate = lastBookingDate;
return this;
}
/**
* The last booking date of the subscription object. This field is writable only when the subscription is newly created as a first version subscription. You can override the date value when creating a subscription through the Subscribe and Amend API or the subscription creation UI (non-Orders). Otherwise, the default value `today` is set per the user's timezone. The value of this field is as follows: * For a new subscription created by the [Subscribe and Amend APIs](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/Orders_Harmonization/Orders_Migration_Guidance#Subscribe_and_Amend_APIs_to_Migrate), this field has the value of the subscription creation date. * For a subscription changed by an amendment, this field has the value of the amendment booking date. * For a subscription created or changed by an order, this field has the value of the order date.
* @return lastBookingDate
*/
@javax.annotation.Nullable
public LocalDate getLastBookingDate() {
return lastBookingDate;
}
public void setLastBookingDate(LocalDate lastBookingDate) {
this.lastBookingDate = lastBookingDate;
}
public CreateSubscriptionRequest notes(String notes) {
this.notes = notes;
return this;
}
/**
* String of up to 500 characters.
* @return notes
*/
@javax.annotation.Nullable
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
this.notes = notes;
}
public CreateSubscriptionRequest paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
return this;
}
/**
* The ID of the payment method used for the payment.
* @return paymentMethodId
*/
@javax.annotation.Nullable
public String getPaymentMethodId() {
return paymentMethodId;
}
public void setPaymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
}
public CreateSubscriptionRequest prepayment(Boolean prepayment) {
this.prepayment = prepayment;
return this;
}
/**
* Indicates whether the subscription will consume the reserved payment amount of the customer account. See [Prepaid Cash with Drawdown](https://knowledgecenter.zuora.com/Zuora_Billing/Billing_and_Invoicing/JA_Advanced_Consumption_Billing/Prepaid_Cash_with_Drawdown) for more information.
* @return prepayment
*/
@javax.annotation.Nullable
public Boolean getPrepayment() {
return prepayment;
}
public void setPrepayment(Boolean prepayment) {
this.prepayment = prepayment;
}
public CreateSubscriptionRequest renewalSetting(String renewalSetting) {
this.renewalSetting = renewalSetting;
return this;
}
/**
* Specifies whether a termed subscription will remain termed or change to evergreen when it is renewed. Values: * `RENEW_WITH_SPECIFIC_TERM` (default) * `RENEW_TO_EVERGREEN`
* @return renewalSetting
*/
@javax.annotation.Nullable
public String getRenewalSetting() {
return renewalSetting;
}
public void setRenewalSetting(String renewalSetting) {
this.renewalSetting = renewalSetting;
}
public CreateSubscriptionRequest renewalTerm(Long renewalTerm) {
this.renewalTerm = renewalTerm;
return this;
}
/**
* The length of the period for the subscription renewal term. Default is `0`.
* @return renewalTerm
*/
@javax.annotation.Nonnull
public Long getRenewalTerm() {
return renewalTerm;
}
public void setRenewalTerm(Long renewalTerm) {
this.renewalTerm = renewalTerm;
}
public CreateSubscriptionRequest renewalTermPeriodType(String renewalTermPeriodType) {
this.renewalTermPeriodType = renewalTermPeriodType;
return this;
}
/**
* The period type for the subscription renewal term. This field is used with the `renewalTerm` field to specify the subscription renewal term. Values are: * `Month` (default) * `Year` * `Day` * `Week`
* @return renewalTermPeriodType
*/
@javax.annotation.Nullable
public String getRenewalTermPeriodType() {
return renewalTermPeriodType;
}
public void setRenewalTermPeriodType(String renewalTermPeriodType) {
this.renewalTermPeriodType = renewalTermPeriodType;
}
public CreateSubscriptionRequest runBilling(Boolean runBilling) {
this.runBilling = runBilling;
return this;
}
/**
* Creates an invoice for a subscription. If you have the Invoice Settlement feature enabled, a credit memo might also be created based on the [invoice and credit memo generation rule](https://knowledgecenter.zuora.com/CB_Billing/Invoice_Settlement/Credit_and_Debit_Memos/Rules_for_Generating_Invoices_and_Credit_Memos). The billing documents generated in this operation is only for this subscription, not for the entire customer account. Possible values: - `true`: An invoice is created. If you have the Invoice Settlement feature enabled, a credit memo might also be created. - `false`: No invoice is created. **Note:** This field is in Zuora REST API version control. Supported minor versions are `211.0` or later. To use this field in the method, you must set the `zuora-version` parameter to the minor version number in the request header.
* @return runBilling
*/
@javax.annotation.Nullable
public Boolean getRunBilling() {
return runBilling;
}
public void setRunBilling(Boolean runBilling) {
this.runBilling = runBilling;
}
public CreateSubscriptionRequest serviceActivationDate(LocalDate serviceActivationDate) {
this.serviceActivationDate = serviceActivationDate;
return this;
}
/**
* The date on which the services or products within a subscription have been activated and access has been provided to the customer, as yyyy-mm-dd. Default value is dependent on the value of other fields. See **Notes** section for more details.
* @return serviceActivationDate
*/
@javax.annotation.Nullable
public LocalDate getServiceActivationDate() {
return serviceActivationDate;
}
public void setServiceActivationDate(LocalDate serviceActivationDate) {
this.serviceActivationDate = serviceActivationDate;
}
public CreateSubscriptionRequest subscribeToRatePlans(List subscribeToRatePlans) {
this.subscribeToRatePlans = subscribeToRatePlans;
return this;
}
public CreateSubscriptionRequest addSubscribeToRatePlansItem(CreateSubscriptionRatePlan subscribeToRatePlansItem) {
if (this.subscribeToRatePlans == null) {
this.subscribeToRatePlans = new ArrayList<>();
}
this.subscribeToRatePlans.add(subscribeToRatePlansItem);
return this;
}
/**
* Container for one or more rate plans for this subscription.
* @return subscribeToRatePlans
*/
@javax.annotation.Nonnull
public List getSubscribeToRatePlans() {
return subscribeToRatePlans;
}
public void setSubscribeToRatePlans(List subscribeToRatePlans) {
this.subscribeToRatePlans = subscribeToRatePlans;
}
public CreateSubscriptionRequest subscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
return this;
}
/**
* Subscription Number. The value can be up to 1000 characters. If you do not specify a subscription number when creating a subscription, Zuora will generate a subscription number automatically. If the account is created successfully, the subscription number is returned in the `subscriptionNumber` response field.
* @return subscriptionNumber
*/
@javax.annotation.Nullable
public String getSubscriptionNumber() {
return subscriptionNumber;
}
public void setSubscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
}
public CreateSubscriptionRequest targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* Date through which to calculate charges if an invoice or a credit memo is generated, as yyyy-mm-dd. Default is current date. **Note:** The credit memo is only available if you have the Invoice Settlement feature enabled. This field is in Zuora REST API version control. Supported minor versions are `211.0` and later. To use this field in the method, you must set the `zuora-version` parameter to the minor version number in the request header.
* @return targetDate
*/
@javax.annotation.Nullable
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public CreateSubscriptionRequest termStartDate(LocalDate termStartDate) {
this.termStartDate = termStartDate;
return this;
}
/**
* The date on which the subscription term begins, as yyyy-mm-dd. If this is a renewal subscription, this date is different from the subscription start date.
* @return termStartDate
*/
@javax.annotation.Nullable
public LocalDate getTermStartDate() {
return termStartDate;
}
public void setTermStartDate(LocalDate termStartDate) {
this.termStartDate = termStartDate;
}
public CreateSubscriptionRequest termType(String termType) {
this.termType = termType;
return this;
}
/**
* Possible values are: `TERMED`, `EVERGREEN`.
* @return termType
*/
@javax.annotation.Nonnull
public String getTermType() {
return termType;
}
public void setTermType(String termType) {
this.termType = termType;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreateSubscriptionRequest instance itself
*/
public CreateSubscriptionRequest putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateSubscriptionRequest createSubscriptionRequest = (CreateSubscriptionRequest) o;
return Objects.equals(this.cpqBundleJsonIdQT, createSubscriptionRequest.cpqBundleJsonIdQT) &&
Objects.equals(this.opportunityCloseDateQT, createSubscriptionRequest.opportunityCloseDateQT) &&
Objects.equals(this.opportunityNameQT, createSubscriptionRequest.opportunityNameQT) &&
Objects.equals(this.quoteBusinessTypeQT, createSubscriptionRequest.quoteBusinessTypeQT) &&
Objects.equals(this.quoteNumberQT, createSubscriptionRequest.quoteNumberQT) &&
Objects.equals(this.quoteTypeQT, createSubscriptionRequest.quoteTypeQT) &&
Objects.equals(this.integrationIdNS, createSubscriptionRequest.integrationIdNS) &&
Objects.equals(this.integrationStatusNS, createSubscriptionRequest.integrationStatusNS) &&
Objects.equals(this.projectNS, createSubscriptionRequest.projectNS) &&
Objects.equals(this.salesOrderNS, createSubscriptionRequest.salesOrderNS) &&
Objects.equals(this.syncDateNS, createSubscriptionRequest.syncDateNS) &&
Objects.equals(this.accountKey, createSubscriptionRequest.accountKey) &&
Objects.equals(this.applicationOrder, createSubscriptionRequest.applicationOrder) &&
Objects.equals(this.applyCredit, createSubscriptionRequest.applyCredit) &&
Objects.equals(this.applyCreditBalance, createSubscriptionRequest.applyCreditBalance) &&
Objects.equals(this.autoRenew, createSubscriptionRequest.autoRenew) &&
Objects.equals(this.collect, createSubscriptionRequest.collect) &&
Objects.equals(this.contractEffectiveDate, createSubscriptionRequest.contractEffectiveDate) &&
Objects.equals(this.creditMemoReasonCode, createSubscriptionRequest.creditMemoReasonCode) &&
Objects.equals(this.customerAcceptanceDate, createSubscriptionRequest.customerAcceptanceDate) &&
Objects.equals(this.documentDate, createSubscriptionRequest.documentDate) &&
Objects.equals(this.externallyManagedBy, createSubscriptionRequest.externallyManagedBy) &&
Objects.equals(this.gatewayId, createSubscriptionRequest.gatewayId) &&
Objects.equals(this.initialTerm, createSubscriptionRequest.initialTerm) &&
Objects.equals(this.initialTermPeriodType, createSubscriptionRequest.initialTermPeriodType) &&
Objects.equals(this.invoice, createSubscriptionRequest.invoice) &&
Objects.equals(this.invoiceCollect, createSubscriptionRequest.invoiceCollect) &&
Objects.equals(this.invoiceOwnerAccountKey, createSubscriptionRequest.invoiceOwnerAccountKey) &&
Objects.equals(this.invoiceSeparately, createSubscriptionRequest.invoiceSeparately) &&
Objects.equals(this.invoiceTargetDate, createSubscriptionRequest.invoiceTargetDate) &&
Objects.equals(this.lastBookingDate, createSubscriptionRequest.lastBookingDate) &&
Objects.equals(this.notes, createSubscriptionRequest.notes) &&
Objects.equals(this.paymentMethodId, createSubscriptionRequest.paymentMethodId) &&
Objects.equals(this.prepayment, createSubscriptionRequest.prepayment) &&
Objects.equals(this.renewalSetting, createSubscriptionRequest.renewalSetting) &&
Objects.equals(this.renewalTerm, createSubscriptionRequest.renewalTerm) &&
Objects.equals(this.renewalTermPeriodType, createSubscriptionRequest.renewalTermPeriodType) &&
Objects.equals(this.runBilling, createSubscriptionRequest.runBilling) &&
Objects.equals(this.serviceActivationDate, createSubscriptionRequest.serviceActivationDate) &&
Objects.equals(this.subscribeToRatePlans, createSubscriptionRequest.subscribeToRatePlans) &&
Objects.equals(this.subscriptionNumber, createSubscriptionRequest.subscriptionNumber) &&
Objects.equals(this.targetDate, createSubscriptionRequest.targetDate) &&
Objects.equals(this.termStartDate, createSubscriptionRequest.termStartDate) &&
Objects.equals(this.termType, createSubscriptionRequest.termType)&&
Objects.equals(this.additionalProperties, createSubscriptionRequest.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(cpqBundleJsonIdQT, opportunityCloseDateQT, opportunityNameQT, quoteBusinessTypeQT, quoteNumberQT, quoteTypeQT, integrationIdNS, integrationStatusNS, projectNS, salesOrderNS, syncDateNS, accountKey, applicationOrder, applyCredit, applyCreditBalance, autoRenew, collect, contractEffectiveDate, creditMemoReasonCode, customerAcceptanceDate, documentDate, externallyManagedBy, gatewayId, initialTerm, initialTermPeriodType, invoice, invoiceCollect, invoiceOwnerAccountKey, invoiceSeparately, invoiceTargetDate, lastBookingDate, notes, paymentMethodId, prepayment, renewalSetting, renewalTerm, renewalTermPeriodType, runBilling, serviceActivationDate, subscribeToRatePlans, subscriptionNumber, targetDate, termStartDate, termType, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateSubscriptionRequest {\n");
sb.append(" cpqBundleJsonIdQT: ").append(toIndentedString(cpqBundleJsonIdQT)).append("\n");
sb.append(" opportunityCloseDateQT: ").append(toIndentedString(opportunityCloseDateQT)).append("\n");
sb.append(" opportunityNameQT: ").append(toIndentedString(opportunityNameQT)).append("\n");
sb.append(" quoteBusinessTypeQT: ").append(toIndentedString(quoteBusinessTypeQT)).append("\n");
sb.append(" quoteNumberQT: ").append(toIndentedString(quoteNumberQT)).append("\n");
sb.append(" quoteTypeQT: ").append(toIndentedString(quoteTypeQT)).append("\n");
sb.append(" integrationIdNS: ").append(toIndentedString(integrationIdNS)).append("\n");
sb.append(" integrationStatusNS: ").append(toIndentedString(integrationStatusNS)).append("\n");
sb.append(" projectNS: ").append(toIndentedString(projectNS)).append("\n");
sb.append(" salesOrderNS: ").append(toIndentedString(salesOrderNS)).append("\n");
sb.append(" syncDateNS: ").append(toIndentedString(syncDateNS)).append("\n");
sb.append(" accountKey: ").append(toIndentedString(accountKey)).append("\n");
sb.append(" applicationOrder: ").append(toIndentedString(applicationOrder)).append("\n");
sb.append(" applyCredit: ").append(toIndentedString(applyCredit)).append("\n");
sb.append(" applyCreditBalance: ").append(toIndentedString(applyCreditBalance)).append("\n");
sb.append(" autoRenew: ").append(toIndentedString(autoRenew)).append("\n");
sb.append(" collect: ").append(toIndentedString(collect)).append("\n");
sb.append(" contractEffectiveDate: ").append(toIndentedString(contractEffectiveDate)).append("\n");
sb.append(" creditMemoReasonCode: ").append(toIndentedString(creditMemoReasonCode)).append("\n");
sb.append(" customerAcceptanceDate: ").append(toIndentedString(customerAcceptanceDate)).append("\n");
sb.append(" documentDate: ").append(toIndentedString(documentDate)).append("\n");
sb.append(" externallyManagedBy: ").append(toIndentedString(externallyManagedBy)).append("\n");
sb.append(" gatewayId: ").append(toIndentedString(gatewayId)).append("\n");
sb.append(" initialTerm: ").append(toIndentedString(initialTerm)).append("\n");
sb.append(" initialTermPeriodType: ").append(toIndentedString(initialTermPeriodType)).append("\n");
sb.append(" invoice: ").append(toIndentedString(invoice)).append("\n");
sb.append(" invoiceCollect: ").append(toIndentedString(invoiceCollect)).append("\n");
sb.append(" invoiceOwnerAccountKey: ").append(toIndentedString(invoiceOwnerAccountKey)).append("\n");
sb.append(" invoiceSeparately: ").append(toIndentedString(invoiceSeparately)).append("\n");
sb.append(" invoiceTargetDate: ").append(toIndentedString(invoiceTargetDate)).append("\n");
sb.append(" lastBookingDate: ").append(toIndentedString(lastBookingDate)).append("\n");
sb.append(" notes: ").append(toIndentedString(notes)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" prepayment: ").append(toIndentedString(prepayment)).append("\n");
sb.append(" renewalSetting: ").append(toIndentedString(renewalSetting)).append("\n");
sb.append(" renewalTerm: ").append(toIndentedString(renewalTerm)).append("\n");
sb.append(" renewalTermPeriodType: ").append(toIndentedString(renewalTermPeriodType)).append("\n");
sb.append(" runBilling: ").append(toIndentedString(runBilling)).append("\n");
sb.append(" serviceActivationDate: ").append(toIndentedString(serviceActivationDate)).append("\n");
sb.append(" subscribeToRatePlans: ").append(toIndentedString(subscribeToRatePlans)).append("\n");
sb.append(" subscriptionNumber: ").append(toIndentedString(subscriptionNumber)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" termStartDate: ").append(toIndentedString(termStartDate)).append("\n");
sb.append(" termType: ").append(toIndentedString(termType)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("CpqBundleJsonId__QT");
openapiFields.add("OpportunityCloseDate__QT");
openapiFields.add("OpportunityName__QT");
openapiFields.add("QuoteBusinessType__QT");
openapiFields.add("QuoteNumber__QT");
openapiFields.add("QuoteType__QT");
openapiFields.add("IntegrationId__NS");
openapiFields.add("IntegrationStatus__NS");
openapiFields.add("Project__NS");
openapiFields.add("SalesOrder__NS");
openapiFields.add("SyncDate__NS");
openapiFields.add("accountKey");
openapiFields.add("applicationOrder");
openapiFields.add("applyCredit");
openapiFields.add("applyCreditBalance");
openapiFields.add("autoRenew");
openapiFields.add("collect");
openapiFields.add("contractEffectiveDate");
openapiFields.add("creditMemoReasonCode");
openapiFields.add("customerAcceptanceDate");
openapiFields.add("documentDate");
openapiFields.add("externallyManagedBy");
openapiFields.add("gatewayId");
openapiFields.add("initialTerm");
openapiFields.add("initialTermPeriodType");
openapiFields.add("invoice");
openapiFields.add("invoiceCollect");
openapiFields.add("invoiceOwnerAccountKey");
openapiFields.add("invoiceSeparately");
openapiFields.add("invoiceTargetDate");
openapiFields.add("lastBookingDate");
openapiFields.add("notes");
openapiFields.add("paymentMethodId");
openapiFields.add("prepayment");
openapiFields.add("renewalSetting");
openapiFields.add("renewalTerm");
openapiFields.add("renewalTermPeriodType");
openapiFields.add("runBilling");
openapiFields.add("serviceActivationDate");
openapiFields.add("subscribeToRatePlans");
openapiFields.add("subscriptionNumber");
openapiFields.add("targetDate");
openapiFields.add("termStartDate");
openapiFields.add("termType");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("accountKey");
openapiRequiredFields.add("contractEffectiveDate");
openapiRequiredFields.add("renewalTerm");
openapiRequiredFields.add("subscribeToRatePlans");
openapiRequiredFields.add("termType");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreateSubscriptionRequest
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreateSubscriptionRequest.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreateSubscriptionRequest is not found in the empty JSON string", CreateSubscriptionRequest.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : CreateSubscriptionRequest.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("CpqBundleJsonId__QT") != null && !jsonObj.get("CpqBundleJsonId__QT").isJsonNull()) && !jsonObj.get("CpqBundleJsonId__QT").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `CpqBundleJsonId__QT` to be a primitive type in the JSON string but got `%s`", jsonObj.get("CpqBundleJsonId__QT").toString()));
}
if ((jsonObj.get("OpportunityName__QT") != null && !jsonObj.get("OpportunityName__QT").isJsonNull()) && !jsonObj.get("OpportunityName__QT").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `OpportunityName__QT` to be a primitive type in the JSON string but got `%s`", jsonObj.get("OpportunityName__QT").toString()));
}
if ((jsonObj.get("QuoteBusinessType__QT") != null && !jsonObj.get("QuoteBusinessType__QT").isJsonNull()) && !jsonObj.get("QuoteBusinessType__QT").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `QuoteBusinessType__QT` to be a primitive type in the JSON string but got `%s`", jsonObj.get("QuoteBusinessType__QT").toString()));
}
if ((jsonObj.get("QuoteNumber__QT") != null && !jsonObj.get("QuoteNumber__QT").isJsonNull()) && !jsonObj.get("QuoteNumber__QT").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `QuoteNumber__QT` to be a primitive type in the JSON string but got `%s`", jsonObj.get("QuoteNumber__QT").toString()));
}
if ((jsonObj.get("QuoteType__QT") != null && !jsonObj.get("QuoteType__QT").isJsonNull()) && !jsonObj.get("QuoteType__QT").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `QuoteType__QT` to be a primitive type in the JSON string but got `%s`", jsonObj.get("QuoteType__QT").toString()));
}
if ((jsonObj.get("IntegrationId__NS") != null && !jsonObj.get("IntegrationId__NS").isJsonNull()) && !jsonObj.get("IntegrationId__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationId__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationId__NS").toString()));
}
if ((jsonObj.get("IntegrationStatus__NS") != null && !jsonObj.get("IntegrationStatus__NS").isJsonNull()) && !jsonObj.get("IntegrationStatus__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationStatus__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationStatus__NS").toString()));
}
if ((jsonObj.get("Project__NS") != null && !jsonObj.get("Project__NS").isJsonNull()) && !jsonObj.get("Project__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Project__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Project__NS").toString()));
}
if ((jsonObj.get("SalesOrder__NS") != null && !jsonObj.get("SalesOrder__NS").isJsonNull()) && !jsonObj.get("SalesOrder__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SalesOrder__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SalesOrder__NS").toString()));
}
if ((jsonObj.get("SyncDate__NS") != null && !jsonObj.get("SyncDate__NS").isJsonNull()) && !jsonObj.get("SyncDate__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SyncDate__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SyncDate__NS").toString()));
}
if (!jsonObj.get("accountKey").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountKey` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountKey").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("applicationOrder") != null && !jsonObj.get("applicationOrder").isJsonNull() && !jsonObj.get("applicationOrder").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `applicationOrder` to be an array in the JSON string but got `%s`", jsonObj.get("applicationOrder").toString()));
}
if ((jsonObj.get("creditMemoReasonCode") != null && !jsonObj.get("creditMemoReasonCode").isJsonNull()) && !jsonObj.get("creditMemoReasonCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creditMemoReasonCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creditMemoReasonCode").toString()));
}
if ((jsonObj.get("externallyManagedBy") != null && !jsonObj.get("externallyManagedBy").isJsonNull()) && !jsonObj.get("externallyManagedBy").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `externallyManagedBy` to be a primitive type in the JSON string but got `%s`", jsonObj.get("externallyManagedBy").toString()));
}
// validate the optional field `externallyManagedBy`
if (jsonObj.get("externallyManagedBy") != null && !jsonObj.get("externallyManagedBy").isJsonNull()) {
ExternallyManagedByEnum.validateJsonElement(jsonObj.get("externallyManagedBy"));
}
if ((jsonObj.get("gatewayId") != null && !jsonObj.get("gatewayId").isJsonNull()) && !jsonObj.get("gatewayId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayId").toString()));
}
if ((jsonObj.get("initialTermPeriodType") != null && !jsonObj.get("initialTermPeriodType").isJsonNull()) && !jsonObj.get("initialTermPeriodType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `initialTermPeriodType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("initialTermPeriodType").toString()));
}
if ((jsonObj.get("invoiceOwnerAccountKey") != null && !jsonObj.get("invoiceOwnerAccountKey").isJsonNull()) && !jsonObj.get("invoiceOwnerAccountKey").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceOwnerAccountKey` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceOwnerAccountKey").toString()));
}
if ((jsonObj.get("notes") != null && !jsonObj.get("notes").isJsonNull()) && !jsonObj.get("notes").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `notes` to be a primitive type in the JSON string but got `%s`", jsonObj.get("notes").toString()));
}
if ((jsonObj.get("paymentMethodId") != null && !jsonObj.get("paymentMethodId").isJsonNull()) && !jsonObj.get("paymentMethodId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentMethodId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentMethodId").toString()));
}
if ((jsonObj.get("renewalSetting") != null && !jsonObj.get("renewalSetting").isJsonNull()) && !jsonObj.get("renewalSetting").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `renewalSetting` to be a primitive type in the JSON string but got `%s`", jsonObj.get("renewalSetting").toString()));
}
if ((jsonObj.get("renewalTermPeriodType") != null && !jsonObj.get("renewalTermPeriodType").isJsonNull()) && !jsonObj.get("renewalTermPeriodType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `renewalTermPeriodType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("renewalTermPeriodType").toString()));
}
// ensure the json data is an array
if (!jsonObj.get("subscribeToRatePlans").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `subscribeToRatePlans` to be an array in the JSON string but got `%s`", jsonObj.get("subscribeToRatePlans").toString()));
}
JsonArray jsonArraysubscribeToRatePlans = jsonObj.getAsJsonArray("subscribeToRatePlans");
// validate the required field `subscribeToRatePlans` (array)
for (int i = 0; i < jsonArraysubscribeToRatePlans.size(); i++) {
CreateSubscriptionRatePlan.validateJsonElement(jsonArraysubscribeToRatePlans.get(i));
};
if ((jsonObj.get("subscriptionNumber") != null && !jsonObj.get("subscriptionNumber").isJsonNull()) && !jsonObj.get("subscriptionNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("subscriptionNumber").toString()));
}
if (!jsonObj.get("termType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `termType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("termType").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreateSubscriptionRequest.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreateSubscriptionRequest' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreateSubscriptionRequest.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreateSubscriptionRequest value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreateSubscriptionRequest read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreateSubscriptionRequest instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreateSubscriptionRequest given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreateSubscriptionRequest
* @throws IOException if the JSON string is invalid with respect to CreateSubscriptionRequest
*/
public static CreateSubscriptionRequest fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreateSubscriptionRequest.class);
}
/**
* Convert an instance of CreateSubscriptionRequest to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy