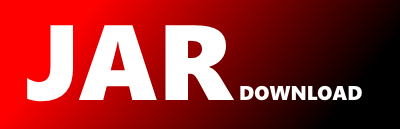
com.zuora.model.CreditMemo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.BillingDocumentStatus;
import com.zuora.model.EInvoiceStatus;
import com.zuora.model.MemoRevenueImpacting;
import com.zuora.model.MemoSourceType;
import com.zuora.model.TaxStatus;
import com.zuora.model.TransferredToAccountingStatus;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* CreditMemo
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreditMemo {
public static final String SERIALIZED_NAME_INTEGRATION_ID_N_S = "IntegrationId__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_ID_N_S)
private String integrationIdNS;
public static final String SERIALIZED_NAME_INTEGRATION_STATUS_N_S = "IntegrationStatus__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_STATUS_N_S)
private String integrationStatusNS;
public static final String SERIALIZED_NAME_ORIGIN_N_S = "Origin__NS";
@SerializedName(SERIALIZED_NAME_ORIGIN_N_S)
private String originNS;
public static final String SERIALIZED_NAME_SYNC_DATE_N_S = "SyncDate__NS";
@SerializedName(SERIALIZED_NAME_SYNC_DATE_N_S)
private String syncDateNS;
public static final String SERIALIZED_NAME_TRANSACTION_N_S = "Transaction__NS";
@SerializedName(SERIALIZED_NAME_TRANSACTION_N_S)
private String transactionNS;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "accountId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "accountNumber";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_APPLIED_AMOUNT = "appliedAmount";
@SerializedName(SERIALIZED_NAME_APPLIED_AMOUNT)
private BigDecimal appliedAmount;
public static final String SERIALIZED_NAME_AUTO_APPLY_UPON_POSTING = "autoApplyUponPosting";
@SerializedName(SERIALIZED_NAME_AUTO_APPLY_UPON_POSTING)
private Boolean autoApplyUponPosting;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT_ID = "billToContactId";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT_ID)
private String billToContactId;
public static final String SERIALIZED_NAME_CANCELLED_BY_ID = "cancelledById";
@SerializedName(SERIALIZED_NAME_CANCELLED_BY_ID)
private String cancelledById;
public static final String SERIALIZED_NAME_CANCELLED_ON = "cancelledOn";
@SerializedName(SERIALIZED_NAME_CANCELLED_ON)
private String cancelledOn;
public static final String SERIALIZED_NAME_COMMENT = "comment";
@SerializedName(SERIALIZED_NAME_COMMENT)
private String comment;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_CREDIT_MEMO_DATE = "creditMemoDate";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_DATE)
private LocalDate creditMemoDate;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_EXCLUDE_FROM_AUTO_APPLY_RULES = "excludeFromAutoApplyRules";
@SerializedName(SERIALIZED_NAME_EXCLUDE_FROM_AUTO_APPLY_RULES)
private Boolean excludeFromAutoApplyRules;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_INVOICE_GROUP_NUMBER = "invoiceGroupNumber";
@SerializedName(SERIALIZED_NAME_INVOICE_GROUP_NUMBER)
private String invoiceGroupNumber;
public static final String SERIALIZED_NAME_REVENUE_IMPACTING = "revenueImpacting";
@SerializedName(SERIALIZED_NAME_REVENUE_IMPACTING)
private MemoRevenueImpacting revenueImpacting;
public static final String SERIALIZED_NAME_LATEST_P_D_F_FILE_ID = "latestPDFFileId";
@SerializedName(SERIALIZED_NAME_LATEST_P_D_F_FILE_ID)
private String latestPDFFileId;
public static final String SERIALIZED_NAME_NUMBER = "number";
@SerializedName(SERIALIZED_NAME_NUMBER)
private String number;
public static final String SERIALIZED_NAME_POSTED_BY_ID = "postedById";
@SerializedName(SERIALIZED_NAME_POSTED_BY_ID)
private String postedById;
public static final String SERIALIZED_NAME_POSTED_ON = "postedOn";
@SerializedName(SERIALIZED_NAME_POSTED_ON)
private String postedOn;
public static final String SERIALIZED_NAME_REASON_CODE = "reasonCode";
@SerializedName(SERIALIZED_NAME_REASON_CODE)
private String reasonCode;
public static final String SERIALIZED_NAME_REFERRED_INVOICE_ID = "referredInvoiceId";
@SerializedName(SERIALIZED_NAME_REFERRED_INVOICE_ID)
private String referredInvoiceId;
public static final String SERIALIZED_NAME_REFUND_AMOUNT = "refundAmount";
@SerializedName(SERIALIZED_NAME_REFUND_AMOUNT)
private BigDecimal refundAmount;
public static final String SERIALIZED_NAME_REVERSED = "reversed";
@SerializedName(SERIALIZED_NAME_REVERSED)
private Boolean reversed;
public static final String SERIALIZED_NAME_SEQUENCE_SET_ID = "sequenceSetId";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_ID)
private String sequenceSetId;
public static final String SERIALIZED_NAME_SOURCE = "source";
@SerializedName(SERIALIZED_NAME_SOURCE)
private String source;
public static final String SERIALIZED_NAME_SOURCE_ID = "sourceId";
@SerializedName(SERIALIZED_NAME_SOURCE_ID)
private String sourceId;
public static final String SERIALIZED_NAME_SOURCE_TYPE = "sourceType";
@SerializedName(SERIALIZED_NAME_SOURCE_TYPE)
private MemoSourceType sourceType;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private BillingDocumentStatus status;
public static final String SERIALIZED_NAME_TARGET_DATE = "targetDate";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public static final String SERIALIZED_NAME_TAX_AMOUNT = "taxAmount";
@SerializedName(SERIALIZED_NAME_TAX_AMOUNT)
private BigDecimal taxAmount;
public static final String SERIALIZED_NAME_TAX_MESSAGE = "taxMessage";
@SerializedName(SERIALIZED_NAME_TAX_MESSAGE)
private String taxMessage;
public static final String SERIALIZED_NAME_TAX_STATUS = "taxStatus";
@SerializedName(SERIALIZED_NAME_TAX_STATUS)
private TaxStatus taxStatus;
public static final String SERIALIZED_NAME_TOTAL_TAX_EXEMPT_AMOUNT = "totalTaxExemptAmount";
@SerializedName(SERIALIZED_NAME_TOTAL_TAX_EXEMPT_AMOUNT)
private BigDecimal totalTaxExemptAmount;
public static final String SERIALIZED_NAME_TRANSFERRED_TO_ACCOUNTING = "transferredToAccounting";
@SerializedName(SERIALIZED_NAME_TRANSFERRED_TO_ACCOUNTING)
private TransferredToAccountingStatus transferredToAccounting;
public static final String SERIALIZED_NAME_UNAPPLIED_AMOUNT = "unappliedAmount";
@SerializedName(SERIALIZED_NAME_UNAPPLIED_AMOUNT)
private BigDecimal unappliedAmount;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public static final String SERIALIZED_NAME_E_INVOICE_STATUS = "eInvoiceStatus";
@SerializedName(SERIALIZED_NAME_E_INVOICE_STATUS)
private EInvoiceStatus eInvoiceStatus;
public static final String SERIALIZED_NAME_E_INVOICE_ERROR_CODE = "eInvoiceErrorCode";
@SerializedName(SERIALIZED_NAME_E_INVOICE_ERROR_CODE)
private String eInvoiceErrorCode;
public static final String SERIALIZED_NAME_E_INVOICE_ERROR_MESSAGE = "eInvoiceErrorMessage";
@SerializedName(SERIALIZED_NAME_E_INVOICE_ERROR_MESSAGE)
private String eInvoiceErrorMessage;
public static final String SERIALIZED_NAME_E_INVOICE_FILE_ID = "eInvoiceFileId";
@SerializedName(SERIALIZED_NAME_E_INVOICE_FILE_ID)
private String eInvoiceFileId;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT_SNAPSHOT_ID = "billToContactSnapshotId";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT_SNAPSHOT_ID)
private String billToContactSnapshotId;
public static final String SERIALIZED_NAME_ORGANIZATION_LABEL = "organizationLabel";
@SerializedName(SERIALIZED_NAME_ORGANIZATION_LABEL)
private String organizationLabel;
public CreditMemo() {
}
public CreditMemo integrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
return this;
}
/**
* ID of the corresponding object in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationIdNS
*/
@javax.annotation.Nullable
public String getIntegrationIdNS() {
return integrationIdNS;
}
public void setIntegrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
}
public CreditMemo integrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
return this;
}
/**
* Status of the credit memo's synchronization with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationStatusNS
*/
@javax.annotation.Nullable
public String getIntegrationStatusNS() {
return integrationStatusNS;
}
public void setIntegrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
}
public CreditMemo originNS(String originNS) {
this.originNS = originNS;
return this;
}
/**
* Origin of the corresponding object in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return originNS
*/
@javax.annotation.Nullable
public String getOriginNS() {
return originNS;
}
public void setOriginNS(String originNS) {
this.originNS = originNS;
}
public CreditMemo syncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
return this;
}
/**
* Date when the credit memo was synchronized with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return syncDateNS
*/
@javax.annotation.Nullable
public String getSyncDateNS() {
return syncDateNS;
}
public void setSyncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
}
public CreditMemo transactionNS(String transactionNS) {
this.transactionNS = transactionNS;
return this;
}
/**
* Related transaction in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return transactionNS
*/
@javax.annotation.Nullable
public String getTransactionNS() {
return transactionNS;
}
public void setTransactionNS(String transactionNS) {
this.transactionNS = transactionNS;
}
public CreditMemo accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* The ID of the customer account associated with the credit memo.
* @return accountId
*/
@javax.annotation.Nullable
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public CreditMemo accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* The number of the account associated with the credit memo.
* @return accountNumber
*/
@javax.annotation.Nullable
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public CreditMemo amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The total amount of the credit memo.
* @return amount
*/
@javax.annotation.Nullable
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public CreditMemo appliedAmount(BigDecimal appliedAmount) {
this.appliedAmount = appliedAmount;
return this;
}
/**
* The applied amount of the credit memo.
* @return appliedAmount
*/
@javax.annotation.Nullable
public BigDecimal getAppliedAmount() {
return appliedAmount;
}
public void setAppliedAmount(BigDecimal appliedAmount) {
this.appliedAmount = appliedAmount;
}
public CreditMemo autoApplyUponPosting(Boolean autoApplyUponPosting) {
this.autoApplyUponPosting = autoApplyUponPosting;
return this;
}
/**
* Whether the credit memo automatically applies to the invoice upon posting.
* @return autoApplyUponPosting
*/
@javax.annotation.Nullable
public Boolean getAutoApplyUponPosting() {
return autoApplyUponPosting;
}
public void setAutoApplyUponPosting(Boolean autoApplyUponPosting) {
this.autoApplyUponPosting = autoApplyUponPosting;
}
public CreditMemo billToContactId(String billToContactId) {
this.billToContactId = billToContactId;
return this;
}
/**
* The ID of the bill-to contact associated with the credit memo. The value of this field is `null` if you have the [Flexible Billing Attributes](https://knowledgecenter.zuora.com/Billing/Subscriptions/Flexible_Billing_Attributes) feature disabled.
* @return billToContactId
*/
@javax.annotation.Nullable
public String getBillToContactId() {
return billToContactId;
}
public void setBillToContactId(String billToContactId) {
this.billToContactId = billToContactId;
}
public CreditMemo cancelledById(String cancelledById) {
this.cancelledById = cancelledById;
return this;
}
/**
* The ID of the Zuora user who cancelled the credit memo.
* @return cancelledById
*/
@javax.annotation.Nullable
public String getCancelledById() {
return cancelledById;
}
public void setCancelledById(String cancelledById) {
this.cancelledById = cancelledById;
}
public CreditMemo cancelledOn(String cancelledOn) {
this.cancelledOn = cancelledOn;
return this;
}
/**
* The date and time when the credit memo was cancelled, in `yyyy-mm-dd hh:mm:ss` format.
* @return cancelledOn
*/
@javax.annotation.Nullable
public String getCancelledOn() {
return cancelledOn;
}
public void setCancelledOn(String cancelledOn) {
this.cancelledOn = cancelledOn;
}
public CreditMemo comment(String comment) {
this.comment = comment;
return this;
}
/**
* Comments about the credit memo.
* @return comment
*/
@javax.annotation.Nullable
public String getComment() {
return comment;
}
public void setComment(String comment) {
this.comment = comment;
}
public CreditMemo createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* The ID of the Zuora user who created the credit memo.
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public CreditMemo createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* The date and time when the credit memo was created, in `yyyy-mm-dd hh:mm:ss` format. For example, 2017-03-01 15:31:10.
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public CreditMemo creditMemoDate(LocalDate creditMemoDate) {
this.creditMemoDate = creditMemoDate;
return this;
}
/**
* The date when the credit memo takes effect, in `yyyy-mm-dd` format. For example, 2017-05-20.
* @return creditMemoDate
*/
@javax.annotation.Nullable
public LocalDate getCreditMemoDate() {
return creditMemoDate;
}
public void setCreditMemoDate(LocalDate creditMemoDate) {
this.creditMemoDate = creditMemoDate;
}
public CreditMemo currency(String currency) {
this.currency = currency;
return this;
}
/**
* A currency defined in the web-based UI administrative settings.
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public CreditMemo excludeFromAutoApplyRules(Boolean excludeFromAutoApplyRules) {
this.excludeFromAutoApplyRules = excludeFromAutoApplyRules;
return this;
}
/**
* Whether the credit memo is excluded from the rule of automatically applying unapplied credit memos to invoices and debit memos during payment runs.
* @return excludeFromAutoApplyRules
*/
@javax.annotation.Nullable
public Boolean getExcludeFromAutoApplyRules() {
return excludeFromAutoApplyRules;
}
public void setExcludeFromAutoApplyRules(Boolean excludeFromAutoApplyRules) {
this.excludeFromAutoApplyRules = excludeFromAutoApplyRules;
}
public CreditMemo id(String id) {
this.id = id;
return this;
}
/**
* The unique ID of the credit memo.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public CreditMemo invoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
return this;
}
/**
* The number of invoice group associated with the credit memo. **Note**: This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_customers_at_subscription_level/Flexible_Billing_Attributes\" target=\"_blank\">Flexible Billing Attributes</a> feature enabled.
* @return invoiceGroupNumber
*/
@javax.annotation.Nullable
public String getInvoiceGroupNumber() {
return invoiceGroupNumber;
}
public void setInvoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
}
public CreditMemo revenueImpacting(MemoRevenueImpacting revenueImpacting) {
this.revenueImpacting = revenueImpacting;
return this;
}
/**
* Get revenueImpacting
* @return revenueImpacting
*/
@javax.annotation.Nullable
public MemoRevenueImpacting getRevenueImpacting() {
return revenueImpacting;
}
public void setRevenueImpacting(MemoRevenueImpacting revenueImpacting) {
this.revenueImpacting = revenueImpacting;
}
public CreditMemo latestPDFFileId(String latestPDFFileId) {
this.latestPDFFileId = latestPDFFileId;
return this;
}
/**
* The ID of the latest PDF file generated for the credit memo.
* @return latestPDFFileId
*/
@javax.annotation.Nullable
public String getLatestPDFFileId() {
return latestPDFFileId;
}
public void setLatestPDFFileId(String latestPDFFileId) {
this.latestPDFFileId = latestPDFFileId;
}
public CreditMemo number(String number) {
this.number = number;
return this;
}
/**
* The unique identification number of the credit memo.
* @return number
*/
@javax.annotation.Nullable
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public CreditMemo postedById(String postedById) {
this.postedById = postedById;
return this;
}
/**
* The ID of the Zuora user who posted the credit memo.
* @return postedById
*/
@javax.annotation.Nullable
public String getPostedById() {
return postedById;
}
public void setPostedById(String postedById) {
this.postedById = postedById;
}
public CreditMemo postedOn(String postedOn) {
this.postedOn = postedOn;
return this;
}
/**
* The date and time when the credit memo was posted, in `yyyy-mm-dd hh:mm:ss` format.
* @return postedOn
*/
@javax.annotation.Nullable
public String getPostedOn() {
return postedOn;
}
public void setPostedOn(String postedOn) {
this.postedOn = postedOn;
}
public CreditMemo reasonCode(String reasonCode) {
this.reasonCode = reasonCode;
return this;
}
/**
* A code identifying the reason for the transaction. The value must be an existing reason code or empty.
* @return reasonCode
*/
@javax.annotation.Nullable
public String getReasonCode() {
return reasonCode;
}
public void setReasonCode(String reasonCode) {
this.reasonCode = reasonCode;
}
public CreditMemo referredInvoiceId(String referredInvoiceId) {
this.referredInvoiceId = referredInvoiceId;
return this;
}
/**
* The ID of a referred invoice.
* @return referredInvoiceId
*/
@javax.annotation.Nullable
public String getReferredInvoiceId() {
return referredInvoiceId;
}
public void setReferredInvoiceId(String referredInvoiceId) {
this.referredInvoiceId = referredInvoiceId;
}
public CreditMemo refundAmount(BigDecimal refundAmount) {
this.refundAmount = refundAmount;
return this;
}
/**
* The amount of the refund on the credit memo.
* @return refundAmount
*/
@javax.annotation.Nullable
public BigDecimal getRefundAmount() {
return refundAmount;
}
public void setRefundAmount(BigDecimal refundAmount) {
this.refundAmount = refundAmount;
}
public CreditMemo reversed(Boolean reversed) {
this.reversed = reversed;
return this;
}
/**
* Whether the credit memo is reversed.
* @return reversed
*/
@javax.annotation.Nullable
public Boolean getReversed() {
return reversed;
}
public void setReversed(Boolean reversed) {
this.reversed = reversed;
}
public CreditMemo sequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
return this;
}
/**
* The ID of the sequence set associated with the credit memo. The value of this field is `null` if you have the [Flexible Billing Attributes](https://knowledgecenter.zuora.com/Billing/Subscriptions/Flexible_Billing_Attributes) feature disabled.
* @return sequenceSetId
*/
@javax.annotation.Nullable
public String getSequenceSetId() {
return sequenceSetId;
}
public void setSequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
}
public CreditMemo source(String source) {
this.source = source;
return this;
}
/**
* The source of the credit memo. Possible values: - `BillRun`: The credit memo is generated by a bill run. - `API`: The credit memo is created by calling the [Invoice and collect](https://www.zuora.com/developer/api-references/api/operation/Post_TransactionInvoicePayment) operation, or by calling the Orders, Order Line Items, or Fulfillments API operations. - `ApiSubscribe`: The credit memo is created by calling the [Create subscription](https://www.zuora.com/developer/api-references/api/operation/Post_Subscription) and [Create account](https://www.zuora.com/developer/api-references/api/operation/Post_Account) operation. - `ApiAmend`: The credit memo is created by calling the [Update subscription](https://www.zuora.com/developer/api-references/api/operation/Put_Subscription) operation. - `AdhocFromPrpc`: The credit memo is created from a product rate plan charge through the Zuora UI or by calling the [Create a credit memo from a charge](https://www.zuora.com/developer/api-references/api/operation/Post_CreditMemoFromPrpc) operation. - `AdhocFromInvoice`: The credit memo is created from an invoice or created by reversing an invoice. You can create a credit memo from an invoice through the Zuora UI or by calling the [Create credit memo from invoice](https://www.zuora.com/developer/api-references/api/operation/Post_CreditMemoFromInvoice) operation. You can create a credit memo by reversing an invoice through the Zuora UI or by calling the [Reverse invoice](https://www.zuora.com/developer/api-references/api/operation/Put_ReverseInvoice) operation.
* @return source
*/
@javax.annotation.Nullable
public String getSource() {
return source;
}
public void setSource(String source) {
this.source = source;
}
public CreditMemo sourceId(String sourceId) {
this.sourceId = sourceId;
return this;
}
/**
* The ID of the credit memo source. If a credit memo is generated from a bill run, the value is the number of the corresponding bill run. Otherwise, the value is `null`.
* @return sourceId
*/
@javax.annotation.Nullable
public String getSourceId() {
return sourceId;
}
public void setSourceId(String sourceId) {
this.sourceId = sourceId;
}
public CreditMemo sourceType(MemoSourceType sourceType) {
this.sourceType = sourceType;
return this;
}
/**
* Get sourceType
* @return sourceType
*/
@javax.annotation.Nullable
public MemoSourceType getSourceType() {
return sourceType;
}
public void setSourceType(MemoSourceType sourceType) {
this.sourceType = sourceType;
}
public CreditMemo status(BillingDocumentStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public BillingDocumentStatus getStatus() {
return status;
}
public void setStatus(BillingDocumentStatus status) {
this.status = status;
}
public CreditMemo targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* The target date for the credit memo, in `yyyy-mm-dd` format. For example, 2017-07-20.
* @return targetDate
*/
@javax.annotation.Nullable
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public CreditMemo taxAmount(BigDecimal taxAmount) {
this.taxAmount = taxAmount;
return this;
}
/**
* The amount of taxation.
* @return taxAmount
*/
@javax.annotation.Nullable
public BigDecimal getTaxAmount() {
return taxAmount;
}
public void setTaxAmount(BigDecimal taxAmount) {
this.taxAmount = taxAmount;
}
public CreditMemo taxMessage(String taxMessage) {
this.taxMessage = taxMessage;
return this;
}
/**
* The message about the status of tax calculation related to the credit memo. If tax calculation fails in one credit memo, this field displays the reason for the failure.
* @return taxMessage
*/
@javax.annotation.Nullable
public String getTaxMessage() {
return taxMessage;
}
public void setTaxMessage(String taxMessage) {
this.taxMessage = taxMessage;
}
public CreditMemo taxStatus(TaxStatus taxStatus) {
this.taxStatus = taxStatus;
return this;
}
/**
* Get taxStatus
* @return taxStatus
*/
@javax.annotation.Nullable
public TaxStatus getTaxStatus() {
return taxStatus;
}
public void setTaxStatus(TaxStatus taxStatus) {
this.taxStatus = taxStatus;
}
public CreditMemo totalTaxExemptAmount(BigDecimal totalTaxExemptAmount) {
this.totalTaxExemptAmount = totalTaxExemptAmount;
return this;
}
/**
* The calculated tax amount excluded due to the exemption.
* @return totalTaxExemptAmount
*/
@javax.annotation.Nullable
public BigDecimal getTotalTaxExemptAmount() {
return totalTaxExemptAmount;
}
public void setTotalTaxExemptAmount(BigDecimal totalTaxExemptAmount) {
this.totalTaxExemptAmount = totalTaxExemptAmount;
}
public CreditMemo transferredToAccounting(TransferredToAccountingStatus transferredToAccounting) {
this.transferredToAccounting = transferredToAccounting;
return this;
}
/**
* Get transferredToAccounting
* @return transferredToAccounting
*/
@javax.annotation.Nullable
public TransferredToAccountingStatus getTransferredToAccounting() {
return transferredToAccounting;
}
public void setTransferredToAccounting(TransferredToAccountingStatus transferredToAccounting) {
this.transferredToAccounting = transferredToAccounting;
}
public CreditMemo unappliedAmount(BigDecimal unappliedAmount) {
this.unappliedAmount = unappliedAmount;
return this;
}
/**
* The unapplied amount of the credit memo.
* @return unappliedAmount
*/
@javax.annotation.Nullable
public BigDecimal getUnappliedAmount() {
return unappliedAmount;
}
public void setUnappliedAmount(BigDecimal unappliedAmount) {
this.unappliedAmount = unappliedAmount;
}
public CreditMemo updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* The ID of the Zuora user who last updated the credit memo.
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public CreditMemo updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* The date and time when the credit memo was last updated, in `yyyy-mm-dd hh:mm:ss` format. For example, 2017-03-02 15:36:10.
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
public CreditMemo eInvoiceStatus(EInvoiceStatus eInvoiceStatus) {
this.eInvoiceStatus = eInvoiceStatus;
return this;
}
/**
* Get eInvoiceStatus
* @return eInvoiceStatus
*/
@javax.annotation.Nullable
public EInvoiceStatus geteInvoiceStatus() {
return eInvoiceStatus;
}
public void seteInvoiceStatus(EInvoiceStatus eInvoiceStatus) {
this.eInvoiceStatus = eInvoiceStatus;
}
public CreditMemo eInvoiceErrorCode(String eInvoiceErrorCode) {
this.eInvoiceErrorCode = eInvoiceErrorCode;
return this;
}
/**
* eInvoiceErrorCode.
* @return eInvoiceErrorCode
*/
@javax.annotation.Nullable
public String geteInvoiceErrorCode() {
return eInvoiceErrorCode;
}
public void seteInvoiceErrorCode(String eInvoiceErrorCode) {
this.eInvoiceErrorCode = eInvoiceErrorCode;
}
public CreditMemo eInvoiceErrorMessage(String eInvoiceErrorMessage) {
this.eInvoiceErrorMessage = eInvoiceErrorMessage;
return this;
}
/**
* eInvoiceErrorMessage.
* @return eInvoiceErrorMessage
*/
@javax.annotation.Nullable
public String geteInvoiceErrorMessage() {
return eInvoiceErrorMessage;
}
public void seteInvoiceErrorMessage(String eInvoiceErrorMessage) {
this.eInvoiceErrorMessage = eInvoiceErrorMessage;
}
public CreditMemo eInvoiceFileId(String eInvoiceFileId) {
this.eInvoiceFileId = eInvoiceFileId;
return this;
}
/**
* eInvoiceFileId.
* @return eInvoiceFileId
*/
@javax.annotation.Nullable
public String geteInvoiceFileId() {
return eInvoiceFileId;
}
public void seteInvoiceFileId(String eInvoiceFileId) {
this.eInvoiceFileId = eInvoiceFileId;
}
public CreditMemo billToContactSnapshotId(String billToContactSnapshotId) {
this.billToContactSnapshotId = billToContactSnapshotId;
return this;
}
/**
* billToContactSnapshotId.
* @return billToContactSnapshotId
*/
@javax.annotation.Nullable
public String getBillToContactSnapshotId() {
return billToContactSnapshotId;
}
public void setBillToContactSnapshotId(String billToContactSnapshotId) {
this.billToContactSnapshotId = billToContactSnapshotId;
}
public CreditMemo organizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
return this;
}
/**
* organization label.
* @return organizationLabel
*/
@javax.annotation.Nullable
public String getOrganizationLabel() {
return organizationLabel;
}
public void setOrganizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreditMemo instance itself
*/
public CreditMemo putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreditMemo creditMemo = (CreditMemo) o;
return Objects.equals(this.integrationIdNS, creditMemo.integrationIdNS) &&
Objects.equals(this.integrationStatusNS, creditMemo.integrationStatusNS) &&
Objects.equals(this.originNS, creditMemo.originNS) &&
Objects.equals(this.syncDateNS, creditMemo.syncDateNS) &&
Objects.equals(this.transactionNS, creditMemo.transactionNS) &&
Objects.equals(this.accountId, creditMemo.accountId) &&
Objects.equals(this.accountNumber, creditMemo.accountNumber) &&
Objects.equals(this.amount, creditMemo.amount) &&
Objects.equals(this.appliedAmount, creditMemo.appliedAmount) &&
Objects.equals(this.autoApplyUponPosting, creditMemo.autoApplyUponPosting) &&
Objects.equals(this.billToContactId, creditMemo.billToContactId) &&
Objects.equals(this.cancelledById, creditMemo.cancelledById) &&
Objects.equals(this.cancelledOn, creditMemo.cancelledOn) &&
Objects.equals(this.comment, creditMemo.comment) &&
Objects.equals(this.createdById, creditMemo.createdById) &&
Objects.equals(this.createdDate, creditMemo.createdDate) &&
Objects.equals(this.creditMemoDate, creditMemo.creditMemoDate) &&
Objects.equals(this.currency, creditMemo.currency) &&
Objects.equals(this.excludeFromAutoApplyRules, creditMemo.excludeFromAutoApplyRules) &&
Objects.equals(this.id, creditMemo.id) &&
Objects.equals(this.invoiceGroupNumber, creditMemo.invoiceGroupNumber) &&
Objects.equals(this.revenueImpacting, creditMemo.revenueImpacting) &&
Objects.equals(this.latestPDFFileId, creditMemo.latestPDFFileId) &&
Objects.equals(this.number, creditMemo.number) &&
Objects.equals(this.postedById, creditMemo.postedById) &&
Objects.equals(this.postedOn, creditMemo.postedOn) &&
Objects.equals(this.reasonCode, creditMemo.reasonCode) &&
Objects.equals(this.referredInvoiceId, creditMemo.referredInvoiceId) &&
Objects.equals(this.refundAmount, creditMemo.refundAmount) &&
Objects.equals(this.reversed, creditMemo.reversed) &&
Objects.equals(this.sequenceSetId, creditMemo.sequenceSetId) &&
Objects.equals(this.source, creditMemo.source) &&
Objects.equals(this.sourceId, creditMemo.sourceId) &&
Objects.equals(this.sourceType, creditMemo.sourceType) &&
Objects.equals(this.status, creditMemo.status) &&
Objects.equals(this.targetDate, creditMemo.targetDate) &&
Objects.equals(this.taxAmount, creditMemo.taxAmount) &&
Objects.equals(this.taxMessage, creditMemo.taxMessage) &&
Objects.equals(this.taxStatus, creditMemo.taxStatus) &&
Objects.equals(this.totalTaxExemptAmount, creditMemo.totalTaxExemptAmount) &&
Objects.equals(this.transferredToAccounting, creditMemo.transferredToAccounting) &&
Objects.equals(this.unappliedAmount, creditMemo.unappliedAmount) &&
Objects.equals(this.updatedById, creditMemo.updatedById) &&
Objects.equals(this.updatedDate, creditMemo.updatedDate) &&
Objects.equals(this.eInvoiceStatus, creditMemo.eInvoiceStatus) &&
Objects.equals(this.eInvoiceErrorCode, creditMemo.eInvoiceErrorCode) &&
Objects.equals(this.eInvoiceErrorMessage, creditMemo.eInvoiceErrorMessage) &&
Objects.equals(this.eInvoiceFileId, creditMemo.eInvoiceFileId) &&
Objects.equals(this.billToContactSnapshotId, creditMemo.billToContactSnapshotId) &&
Objects.equals(this.organizationLabel, creditMemo.organizationLabel)&&
Objects.equals(this.additionalProperties, creditMemo.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(integrationIdNS, integrationStatusNS, originNS, syncDateNS, transactionNS, accountId, accountNumber, amount, appliedAmount, autoApplyUponPosting, billToContactId, cancelledById, cancelledOn, comment, createdById, createdDate, creditMemoDate, currency, excludeFromAutoApplyRules, id, invoiceGroupNumber, revenueImpacting, latestPDFFileId, number, postedById, postedOn, reasonCode, referredInvoiceId, refundAmount, reversed, sequenceSetId, source, sourceId, sourceType, status, targetDate, taxAmount, taxMessage, taxStatus, totalTaxExemptAmount, transferredToAccounting, unappliedAmount, updatedById, updatedDate, eInvoiceStatus, eInvoiceErrorCode, eInvoiceErrorMessage, eInvoiceFileId, billToContactSnapshotId, organizationLabel, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreditMemo {\n");
sb.append(" integrationIdNS: ").append(toIndentedString(integrationIdNS)).append("\n");
sb.append(" integrationStatusNS: ").append(toIndentedString(integrationStatusNS)).append("\n");
sb.append(" originNS: ").append(toIndentedString(originNS)).append("\n");
sb.append(" syncDateNS: ").append(toIndentedString(syncDateNS)).append("\n");
sb.append(" transactionNS: ").append(toIndentedString(transactionNS)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" appliedAmount: ").append(toIndentedString(appliedAmount)).append("\n");
sb.append(" autoApplyUponPosting: ").append(toIndentedString(autoApplyUponPosting)).append("\n");
sb.append(" billToContactId: ").append(toIndentedString(billToContactId)).append("\n");
sb.append(" cancelledById: ").append(toIndentedString(cancelledById)).append("\n");
sb.append(" cancelledOn: ").append(toIndentedString(cancelledOn)).append("\n");
sb.append(" comment: ").append(toIndentedString(comment)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" creditMemoDate: ").append(toIndentedString(creditMemoDate)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" excludeFromAutoApplyRules: ").append(toIndentedString(excludeFromAutoApplyRules)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" invoiceGroupNumber: ").append(toIndentedString(invoiceGroupNumber)).append("\n");
sb.append(" revenueImpacting: ").append(toIndentedString(revenueImpacting)).append("\n");
sb.append(" latestPDFFileId: ").append(toIndentedString(latestPDFFileId)).append("\n");
sb.append(" number: ").append(toIndentedString(number)).append("\n");
sb.append(" postedById: ").append(toIndentedString(postedById)).append("\n");
sb.append(" postedOn: ").append(toIndentedString(postedOn)).append("\n");
sb.append(" reasonCode: ").append(toIndentedString(reasonCode)).append("\n");
sb.append(" referredInvoiceId: ").append(toIndentedString(referredInvoiceId)).append("\n");
sb.append(" refundAmount: ").append(toIndentedString(refundAmount)).append("\n");
sb.append(" reversed: ").append(toIndentedString(reversed)).append("\n");
sb.append(" sequenceSetId: ").append(toIndentedString(sequenceSetId)).append("\n");
sb.append(" source: ").append(toIndentedString(source)).append("\n");
sb.append(" sourceId: ").append(toIndentedString(sourceId)).append("\n");
sb.append(" sourceType: ").append(toIndentedString(sourceType)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" taxAmount: ").append(toIndentedString(taxAmount)).append("\n");
sb.append(" taxMessage: ").append(toIndentedString(taxMessage)).append("\n");
sb.append(" taxStatus: ").append(toIndentedString(taxStatus)).append("\n");
sb.append(" totalTaxExemptAmount: ").append(toIndentedString(totalTaxExemptAmount)).append("\n");
sb.append(" transferredToAccounting: ").append(toIndentedString(transferredToAccounting)).append("\n");
sb.append(" unappliedAmount: ").append(toIndentedString(unappliedAmount)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" eInvoiceStatus: ").append(toIndentedString(eInvoiceStatus)).append("\n");
sb.append(" eInvoiceErrorCode: ").append(toIndentedString(eInvoiceErrorCode)).append("\n");
sb.append(" eInvoiceErrorMessage: ").append(toIndentedString(eInvoiceErrorMessage)).append("\n");
sb.append(" eInvoiceFileId: ").append(toIndentedString(eInvoiceFileId)).append("\n");
sb.append(" billToContactSnapshotId: ").append(toIndentedString(billToContactSnapshotId)).append("\n");
sb.append(" organizationLabel: ").append(toIndentedString(organizationLabel)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("IntegrationId__NS");
openapiFields.add("IntegrationStatus__NS");
openapiFields.add("Origin__NS");
openapiFields.add("SyncDate__NS");
openapiFields.add("Transaction__NS");
openapiFields.add("accountId");
openapiFields.add("accountNumber");
openapiFields.add("amount");
openapiFields.add("appliedAmount");
openapiFields.add("autoApplyUponPosting");
openapiFields.add("billToContactId");
openapiFields.add("cancelledById");
openapiFields.add("cancelledOn");
openapiFields.add("comment");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("creditMemoDate");
openapiFields.add("currency");
openapiFields.add("excludeFromAutoApplyRules");
openapiFields.add("id");
openapiFields.add("invoiceGroupNumber");
openapiFields.add("revenueImpacting");
openapiFields.add("latestPDFFileId");
openapiFields.add("number");
openapiFields.add("postedById");
openapiFields.add("postedOn");
openapiFields.add("reasonCode");
openapiFields.add("referredInvoiceId");
openapiFields.add("refundAmount");
openapiFields.add("reversed");
openapiFields.add("sequenceSetId");
openapiFields.add("source");
openapiFields.add("sourceId");
openapiFields.add("sourceType");
openapiFields.add("status");
openapiFields.add("targetDate");
openapiFields.add("taxAmount");
openapiFields.add("taxMessage");
openapiFields.add("taxStatus");
openapiFields.add("totalTaxExemptAmount");
openapiFields.add("transferredToAccounting");
openapiFields.add("unappliedAmount");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
openapiFields.add("eInvoiceStatus");
openapiFields.add("eInvoiceErrorCode");
openapiFields.add("eInvoiceErrorMessage");
openapiFields.add("eInvoiceFileId");
openapiFields.add("billToContactSnapshotId");
openapiFields.add("organizationLabel");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreditMemo
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreditMemo.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreditMemo is not found in the empty JSON string", CreditMemo.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("IntegrationId__NS") != null && !jsonObj.get("IntegrationId__NS").isJsonNull()) && !jsonObj.get("IntegrationId__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationId__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationId__NS").toString()));
}
if ((jsonObj.get("IntegrationStatus__NS") != null && !jsonObj.get("IntegrationStatus__NS").isJsonNull()) && !jsonObj.get("IntegrationStatus__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationStatus__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationStatus__NS").toString()));
}
if ((jsonObj.get("Origin__NS") != null && !jsonObj.get("Origin__NS").isJsonNull()) && !jsonObj.get("Origin__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Origin__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Origin__NS").toString()));
}
if ((jsonObj.get("SyncDate__NS") != null && !jsonObj.get("SyncDate__NS").isJsonNull()) && !jsonObj.get("SyncDate__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SyncDate__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SyncDate__NS").toString()));
}
if ((jsonObj.get("Transaction__NS") != null && !jsonObj.get("Transaction__NS").isJsonNull()) && !jsonObj.get("Transaction__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Transaction__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Transaction__NS").toString()));
}
if ((jsonObj.get("accountId") != null && !jsonObj.get("accountId").isJsonNull()) && !jsonObj.get("accountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountId").toString()));
}
if ((jsonObj.get("accountNumber") != null && !jsonObj.get("accountNumber").isJsonNull()) && !jsonObj.get("accountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountNumber").toString()));
}
if ((jsonObj.get("billToContactId") != null && !jsonObj.get("billToContactId").isJsonNull()) && !jsonObj.get("billToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billToContactId").toString()));
}
if ((jsonObj.get("cancelledById") != null && !jsonObj.get("cancelledById").isJsonNull()) && !jsonObj.get("cancelledById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cancelledById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cancelledById").toString()));
}
if ((jsonObj.get("cancelledOn") != null && !jsonObj.get("cancelledOn").isJsonNull()) && !jsonObj.get("cancelledOn").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cancelledOn` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cancelledOn").toString()));
}
if ((jsonObj.get("comment") != null && !jsonObj.get("comment").isJsonNull()) && !jsonObj.get("comment").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `comment` to be a primitive type in the JSON string but got `%s`", jsonObj.get("comment").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("invoiceGroupNumber") != null && !jsonObj.get("invoiceGroupNumber").isJsonNull()) && !jsonObj.get("invoiceGroupNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceGroupNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceGroupNumber").toString()));
}
if ((jsonObj.get("revenueImpacting") != null && !jsonObj.get("revenueImpacting").isJsonNull()) && !jsonObj.get("revenueImpacting").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueImpacting` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueImpacting").toString()));
}
// validate the optional field `revenueImpacting`
if (jsonObj.get("revenueImpacting") != null && !jsonObj.get("revenueImpacting").isJsonNull()) {
MemoRevenueImpacting.validateJsonElement(jsonObj.get("revenueImpacting"));
}
if ((jsonObj.get("latestPDFFileId") != null && !jsonObj.get("latestPDFFileId").isJsonNull()) && !jsonObj.get("latestPDFFileId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `latestPDFFileId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("latestPDFFileId").toString()));
}
if ((jsonObj.get("number") != null && !jsonObj.get("number").isJsonNull()) && !jsonObj.get("number").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `number` to be a primitive type in the JSON string but got `%s`", jsonObj.get("number").toString()));
}
if ((jsonObj.get("postedById") != null && !jsonObj.get("postedById").isJsonNull()) && !jsonObj.get("postedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `postedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("postedById").toString()));
}
if ((jsonObj.get("postedOn") != null && !jsonObj.get("postedOn").isJsonNull()) && !jsonObj.get("postedOn").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `postedOn` to be a primitive type in the JSON string but got `%s`", jsonObj.get("postedOn").toString()));
}
if ((jsonObj.get("reasonCode") != null && !jsonObj.get("reasonCode").isJsonNull()) && !jsonObj.get("reasonCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `reasonCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("reasonCode").toString()));
}
if ((jsonObj.get("referredInvoiceId") != null && !jsonObj.get("referredInvoiceId").isJsonNull()) && !jsonObj.get("referredInvoiceId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `referredInvoiceId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("referredInvoiceId").toString()));
}
if ((jsonObj.get("sequenceSetId") != null && !jsonObj.get("sequenceSetId").isJsonNull()) && !jsonObj.get("sequenceSetId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sequenceSetId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sequenceSetId").toString()));
}
if ((jsonObj.get("source") != null && !jsonObj.get("source").isJsonNull()) && !jsonObj.get("source").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `source` to be a primitive type in the JSON string but got `%s`", jsonObj.get("source").toString()));
}
if ((jsonObj.get("sourceId") != null && !jsonObj.get("sourceId").isJsonNull()) && !jsonObj.get("sourceId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sourceId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sourceId").toString()));
}
if ((jsonObj.get("sourceType") != null && !jsonObj.get("sourceType").isJsonNull()) && !jsonObj.get("sourceType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sourceType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sourceType").toString()));
}
// validate the optional field `sourceType`
if (jsonObj.get("sourceType") != null && !jsonObj.get("sourceType").isJsonNull()) {
MemoSourceType.validateJsonElement(jsonObj.get("sourceType"));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
// validate the optional field `status`
if (jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) {
BillingDocumentStatus.validateJsonElement(jsonObj.get("status"));
}
if ((jsonObj.get("taxMessage") != null && !jsonObj.get("taxMessage").isJsonNull()) && !jsonObj.get("taxMessage").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxMessage` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxMessage").toString()));
}
if ((jsonObj.get("taxStatus") != null && !jsonObj.get("taxStatus").isJsonNull()) && !jsonObj.get("taxStatus").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxStatus` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxStatus").toString()));
}
// validate the optional field `taxStatus`
if (jsonObj.get("taxStatus") != null && !jsonObj.get("taxStatus").isJsonNull()) {
TaxStatus.validateJsonElement(jsonObj.get("taxStatus"));
}
if ((jsonObj.get("transferredToAccounting") != null && !jsonObj.get("transferredToAccounting").isJsonNull()) && !jsonObj.get("transferredToAccounting").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `transferredToAccounting` to be a primitive type in the JSON string but got `%s`", jsonObj.get("transferredToAccounting").toString()));
}
// validate the optional field `transferredToAccounting`
if (jsonObj.get("transferredToAccounting") != null && !jsonObj.get("transferredToAccounting").isJsonNull()) {
TransferredToAccountingStatus.validateJsonElement(jsonObj.get("transferredToAccounting"));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
if ((jsonObj.get("eInvoiceStatus") != null && !jsonObj.get("eInvoiceStatus").isJsonNull()) && !jsonObj.get("eInvoiceStatus").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `eInvoiceStatus` to be a primitive type in the JSON string but got `%s`", jsonObj.get("eInvoiceStatus").toString()));
}
// validate the optional field `eInvoiceStatus`
if (jsonObj.get("eInvoiceStatus") != null && !jsonObj.get("eInvoiceStatus").isJsonNull()) {
EInvoiceStatus.validateJsonElement(jsonObj.get("eInvoiceStatus"));
}
if ((jsonObj.get("eInvoiceErrorCode") != null && !jsonObj.get("eInvoiceErrorCode").isJsonNull()) && !jsonObj.get("eInvoiceErrorCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `eInvoiceErrorCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("eInvoiceErrorCode").toString()));
}
if ((jsonObj.get("eInvoiceErrorMessage") != null && !jsonObj.get("eInvoiceErrorMessage").isJsonNull()) && !jsonObj.get("eInvoiceErrorMessage").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `eInvoiceErrorMessage` to be a primitive type in the JSON string but got `%s`", jsonObj.get("eInvoiceErrorMessage").toString()));
}
if ((jsonObj.get("eInvoiceFileId") != null && !jsonObj.get("eInvoiceFileId").isJsonNull()) && !jsonObj.get("eInvoiceFileId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `eInvoiceFileId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("eInvoiceFileId").toString()));
}
if ((jsonObj.get("billToContactSnapshotId") != null && !jsonObj.get("billToContactSnapshotId").isJsonNull()) && !jsonObj.get("billToContactSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billToContactSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billToContactSnapshotId").toString()));
}
if ((jsonObj.get("organizationLabel") != null && !jsonObj.get("organizationLabel").isJsonNull()) && !jsonObj.get("organizationLabel").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `organizationLabel` to be a primitive type in the JSON string but got `%s`", jsonObj.get("organizationLabel").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreditMemo.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreditMemo' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreditMemo.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreditMemo value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreditMemo read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreditMemo instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreditMemo given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreditMemo
* @throws IOException if the JSON string is invalid with respect to CreditMemo
*/
public static CreditMemo fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreditMemo.class);
}
/**
* Convert an instance of CreditMemo to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy