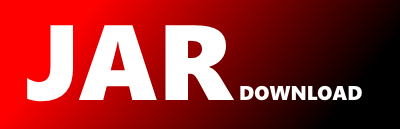
com.zuora.model.CustomObjectCustomFieldDefinitionUpdate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.CustomObjectCustomFieldDefinitionUpdateOrigin;
import java.io.IOException;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* The custom field definition in the custom object
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CustomObjectCustomFieldDefinitionUpdate {
public static final String SERIALIZED_NAME_DEFAULT = "default";
@SerializedName(SERIALIZED_NAME_DEFAULT)
private String _default;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_DISPLAY_NAME = "displayName";
@SerializedName(SERIALIZED_NAME_DISPLAY_NAME)
private Boolean displayName;
public static final String SERIALIZED_NAME_FORMAT = "format";
@SerializedName(SERIALIZED_NAME_FORMAT)
private String format;
public static final String SERIALIZED_NAME_LABEL = "label";
@SerializedName(SERIALIZED_NAME_LABEL)
private String label;
public static final String SERIALIZED_NAME_MAX_LENGTH = "maxLength";
@SerializedName(SERIALIZED_NAME_MAX_LENGTH)
private Integer maxLength;
public static final String SERIALIZED_NAME_MULTISELECT = "multiselect";
@SerializedName(SERIALIZED_NAME_MULTISELECT)
private Boolean multiselect;
public static final String SERIALIZED_NAME_ORIGIN = "origin";
@SerializedName(SERIALIZED_NAME_ORIGIN)
private CustomObjectCustomFieldDefinitionUpdateOrigin origin;
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private String type;
public CustomObjectCustomFieldDefinitionUpdate() {
}
public CustomObjectCustomFieldDefinitionUpdate _default(String _default) {
this._default = _default;
return this;
}
/**
* Applicable if the `type` of the action is `updateField`
* @return _default
*/
@javax.annotation.Nullable
public String getDefault() {
return _default;
}
public void setDefault(String _default) {
this._default = _default;
}
public CustomObjectCustomFieldDefinitionUpdate description(String description) {
this.description = description;
return this;
}
/**
* Applicable if the `type` of the action is `updateField`
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public CustomObjectCustomFieldDefinitionUpdate displayName(Boolean displayName) {
this.displayName = displayName;
return this;
}
/**
* Indicates whether to use this field as the display name of the custom object when being linked to another custom object. This field applies only to the Text custom field type: - The `type` field is `string`. - The `enum` field is not specified.
* @return displayName
*/
@javax.annotation.Nullable
public Boolean getDisplayName() {
return displayName;
}
public void setDisplayName(Boolean displayName) {
this.displayName = displayName;
}
public CustomObjectCustomFieldDefinitionUpdate format(String format) {
this.format = format;
return this;
}
/**
* The data format of the custom field
* @return format
*/
@javax.annotation.Nullable
public String getFormat() {
return format;
}
public void setFormat(String format) {
this.format = format;
}
public CustomObjectCustomFieldDefinitionUpdate label(String label) {
this.label = label;
return this;
}
/**
* The UI label of the custom field
* @return label
*/
@javax.annotation.Nullable
public String getLabel() {
return label;
}
public void setLabel(String label) {
this.label = label;
}
public CustomObjectCustomFieldDefinitionUpdate maxLength(Integer maxLength) {
this.maxLength = maxLength;
return this;
}
/**
* The maximum length of string that can be stored in the custom field. This field applies only to the following custom field types: - Text: - The `type` field is `string`. - The `format` field is not specified or is `url`. - The `enum` field is not specified. - Picklist: - The `type` field is `string`. - The `enum` field is specified. - The `multiselect` field is not specified or is `false`. - Multiselect: - The `type` field is `string`. - The `enum` field is specified. - The `multiselect` field is `true`. If the custom field is filterable, the value of `maxLength` must be 512 or less.
* @return maxLength
*/
@javax.annotation.Nullable
public Integer getMaxLength() {
return maxLength;
}
public void setMaxLength(Integer maxLength) {
this.maxLength = maxLength;
}
public CustomObjectCustomFieldDefinitionUpdate multiselect(Boolean multiselect) {
this.multiselect = multiselect;
return this;
}
/**
* Indicates whether this is a multiselect custom field. This field applies only to the creation of Picklist or Multiselect custom fields: - The action `type` field is `addField`. - The definition `type` field is `string`. - The `maxLength` field is specified. - The `enum` field is specified.
* @return multiselect
*/
@javax.annotation.Nullable
public Boolean getMultiselect() {
return multiselect;
}
public void setMultiselect(Boolean multiselect) {
this.multiselect = multiselect;
}
public CustomObjectCustomFieldDefinitionUpdate origin(CustomObjectCustomFieldDefinitionUpdateOrigin origin) {
this.origin = origin;
return this;
}
/**
* Get origin
* @return origin
*/
@javax.annotation.Nullable
public CustomObjectCustomFieldDefinitionUpdateOrigin getOrigin() {
return origin;
}
public void setOrigin(CustomObjectCustomFieldDefinitionUpdateOrigin origin) {
this.origin = origin;
}
public CustomObjectCustomFieldDefinitionUpdate type(String type) {
this.type = type;
return this;
}
/**
* The data type of the custom field
* @return type
*/
@javax.annotation.Nullable
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CustomObjectCustomFieldDefinitionUpdate instance itself
*/
public CustomObjectCustomFieldDefinitionUpdate putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CustomObjectCustomFieldDefinitionUpdate customObjectCustomFieldDefinitionUpdate = (CustomObjectCustomFieldDefinitionUpdate) o;
return Objects.equals(this._default, customObjectCustomFieldDefinitionUpdate._default) &&
Objects.equals(this.description, customObjectCustomFieldDefinitionUpdate.description) &&
Objects.equals(this.displayName, customObjectCustomFieldDefinitionUpdate.displayName) &&
Objects.equals(this.format, customObjectCustomFieldDefinitionUpdate.format) &&
Objects.equals(this.label, customObjectCustomFieldDefinitionUpdate.label) &&
Objects.equals(this.maxLength, customObjectCustomFieldDefinitionUpdate.maxLength) &&
Objects.equals(this.multiselect, customObjectCustomFieldDefinitionUpdate.multiselect) &&
Objects.equals(this.origin, customObjectCustomFieldDefinitionUpdate.origin) &&
Objects.equals(this.type, customObjectCustomFieldDefinitionUpdate.type)&&
Objects.equals(this.additionalProperties, customObjectCustomFieldDefinitionUpdate.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(_default, description, displayName, format, label, maxLength, multiselect, origin, type, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CustomObjectCustomFieldDefinitionUpdate {\n");
sb.append(" _default: ").append(toIndentedString(_default)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" displayName: ").append(toIndentedString(displayName)).append("\n");
sb.append(" format: ").append(toIndentedString(format)).append("\n");
sb.append(" label: ").append(toIndentedString(label)).append("\n");
sb.append(" maxLength: ").append(toIndentedString(maxLength)).append("\n");
sb.append(" multiselect: ").append(toIndentedString(multiselect)).append("\n");
sb.append(" origin: ").append(toIndentedString(origin)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("default");
openapiFields.add("description");
openapiFields.add("displayName");
openapiFields.add("format");
openapiFields.add("label");
openapiFields.add("maxLength");
openapiFields.add("multiselect");
openapiFields.add("origin");
openapiFields.add("type");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CustomObjectCustomFieldDefinitionUpdate
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CustomObjectCustomFieldDefinitionUpdate.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CustomObjectCustomFieldDefinitionUpdate is not found in the empty JSON string", CustomObjectCustomFieldDefinitionUpdate.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("default") != null && !jsonObj.get("default").isJsonNull()) && !jsonObj.get("default").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `default` to be a primitive type in the JSON string but got `%s`", jsonObj.get("default").toString()));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if ((jsonObj.get("format") != null && !jsonObj.get("format").isJsonNull()) && !jsonObj.get("format").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `format` to be a primitive type in the JSON string but got `%s`", jsonObj.get("format").toString()));
}
if ((jsonObj.get("label") != null && !jsonObj.get("label").isJsonNull()) && !jsonObj.get("label").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `label` to be a primitive type in the JSON string but got `%s`", jsonObj.get("label").toString()));
}
if ((jsonObj.get("origin") != null && !jsonObj.get("origin").isJsonNull()) && !jsonObj.get("origin").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `origin` to be a primitive type in the JSON string but got `%s`", jsonObj.get("origin").toString()));
}
// validate the optional field `origin`
if (jsonObj.get("origin") != null && !jsonObj.get("origin").isJsonNull()) {
CustomObjectCustomFieldDefinitionUpdateOrigin.validateJsonElement(jsonObj.get("origin"));
}
if ((jsonObj.get("type") != null && !jsonObj.get("type").isJsonNull()) && !jsonObj.get("type").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `type` to be a primitive type in the JSON string but got `%s`", jsonObj.get("type").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CustomObjectCustomFieldDefinitionUpdate.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CustomObjectCustomFieldDefinitionUpdate' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CustomObjectCustomFieldDefinitionUpdate.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CustomObjectCustomFieldDefinitionUpdate value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CustomObjectCustomFieldDefinitionUpdate read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CustomObjectCustomFieldDefinitionUpdate instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CustomObjectCustomFieldDefinitionUpdate given an JSON string
*
* @param jsonString JSON string
* @return An instance of CustomObjectCustomFieldDefinitionUpdate
* @throws IOException if the JSON string is invalid with respect to CustomObjectCustomFieldDefinitionUpdate
*/
public static CustomObjectCustomFieldDefinitionUpdate fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CustomObjectCustomFieldDefinitionUpdate.class);
}
/**
* Convert an instance of CustomObjectCustomFieldDefinitionUpdate to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy