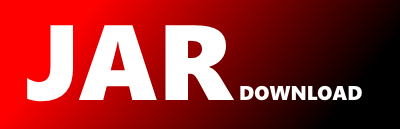
com.zuora.model.DebitMemoItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.BillingDocumentItemProcessingType;
import com.zuora.model.BillingDocumentItemSourceType;
import com.zuora.model.DebitMemoItemTaxationItems;
import com.zuora.model.GetDebitMemoItemFinanceInformation;
import com.zuora.model.GetDebitMemoTaxItem;
import com.zuora.model.TaxMode;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* DebitMemoItem
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class DebitMemoItem {
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_AMOUNT_WITHOUT_TAX = "amountWithoutTax";
@SerializedName(SERIALIZED_NAME_AMOUNT_WITHOUT_TAX)
private BigDecimal amountWithoutTax;
public static final String SERIALIZED_NAME_APPLIED_TO_ITEM_ID = "appliedToItemId";
@SerializedName(SERIALIZED_NAME_APPLIED_TO_ITEM_ID)
private String appliedToItemId;
public static final String SERIALIZED_NAME_BALANCE = "balance";
@SerializedName(SERIALIZED_NAME_BALANCE)
private BigDecimal balance;
public static final String SERIALIZED_NAME_BE_APPLIED_AMOUNT = "beAppliedAmount";
@SerializedName(SERIALIZED_NAME_BE_APPLIED_AMOUNT)
private BigDecimal beAppliedAmount;
public static final String SERIALIZED_NAME_COMMENT = "comment";
@Deprecated
@SerializedName(SERIALIZED_NAME_COMMENT)
private String comment;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING = "excludeItemBillingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBillingFromRevenueAccounting;
public static final String SERIALIZED_NAME_FINANCE_INFORMATION = "financeInformation";
@SerializedName(SERIALIZED_NAME_FINANCE_INFORMATION)
private GetDebitMemoItemFinanceInformation financeInformation;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_PROCESSING_TYPE = "processingType";
@SerializedName(SERIALIZED_NAME_PROCESSING_TYPE)
private BillingDocumentItemProcessingType processingType;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_REFLECT_DISCOUNT_IN_NET_AMOUNT = "reflectDiscountInNetAmount";
@SerializedName(SERIALIZED_NAME_REFLECT_DISCOUNT_IN_NET_AMOUNT)
private Boolean reflectDiscountInNetAmount;
public static final String SERIALIZED_NAME_SERVICE_END_DATE = "serviceEndDate";
@SerializedName(SERIALIZED_NAME_SERVICE_END_DATE)
private LocalDate serviceEndDate;
public static final String SERIALIZED_NAME_SERVICE_START_DATE = "serviceStartDate";
@SerializedName(SERIALIZED_NAME_SERVICE_START_DATE)
private LocalDate serviceStartDate;
public static final String SERIALIZED_NAME_SHIP_TO_CONTACT_ID = "shipToContactId";
@SerializedName(SERIALIZED_NAME_SHIP_TO_CONTACT_ID)
private String shipToContactId;
public static final String SERIALIZED_NAME_SKU = "sku";
@SerializedName(SERIALIZED_NAME_SKU)
private String sku;
public static final String SERIALIZED_NAME_SKU_NAME = "skuName";
@SerializedName(SERIALIZED_NAME_SKU_NAME)
private String skuName;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT_ID = "soldToContactId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT_ID)
private String soldToContactId;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT_SNAPSHOT_ID = "soldToContactSnapshotId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT_SNAPSHOT_ID)
private String soldToContactSnapshotId;
public static final String SERIALIZED_NAME_SOURCE_ITEM_ID = "sourceItemId";
@SerializedName(SERIALIZED_NAME_SOURCE_ITEM_ID)
private String sourceItemId;
public static final String SERIALIZED_NAME_SOURCE_ITEM_TYPE = "sourceItemType";
@SerializedName(SERIALIZED_NAME_SOURCE_ITEM_TYPE)
private BillingDocumentItemSourceType sourceItemType;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ID = "subscriptionId";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ID)
private String subscriptionId;
public static final String SERIALIZED_NAME_TAX_ITEMS = "taxItems";
@Deprecated
@SerializedName(SERIALIZED_NAME_TAX_ITEMS)
private List taxItems;
public static final String SERIALIZED_NAME_TAX_MODE = "taxMode";
@SerializedName(SERIALIZED_NAME_TAX_MODE)
private TaxMode taxMode;
public static final String SERIALIZED_NAME_TAXATION_ITEMS = "taxationItems";
@SerializedName(SERIALIZED_NAME_TAXATION_ITEMS)
private DebitMemoItemTaxationItems taxationItems;
public static final String SERIALIZED_NAME_UNIT_OF_MEASURE = "unitOfMeasure";
@SerializedName(SERIALIZED_NAME_UNIT_OF_MEASURE)
private String unitOfMeasure;
public static final String SERIALIZED_NAME_UNIT_PRICE = "unitPrice";
@SerializedName(SERIALIZED_NAME_UNIT_PRICE)
private BigDecimal unitPrice;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public DebitMemoItem() {
}
public DebitMemoItem amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The amount of the debit memo item. For tax-inclusive debit memo items, the amount indicates the debit memo item amount including tax. For tax-exclusive debit memo items, the amount indicates the debit memo item amount excluding tax.
* @return amount
*/
@javax.annotation.Nullable
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public DebitMemoItem amountWithoutTax(BigDecimal amountWithoutTax) {
this.amountWithoutTax = amountWithoutTax;
return this;
}
/**
* The debit memo item amount excluding tax.
* @return amountWithoutTax
*/
@javax.annotation.Nullable
public BigDecimal getAmountWithoutTax() {
return amountWithoutTax;
}
public void setAmountWithoutTax(BigDecimal amountWithoutTax) {
this.amountWithoutTax = amountWithoutTax;
}
public DebitMemoItem appliedToItemId(String appliedToItemId) {
this.appliedToItemId = appliedToItemId;
return this;
}
/**
* The parent debit memo item that this debit memo items is applied to if this item is discount.
* @return appliedToItemId
*/
@javax.annotation.Nullable
public String getAppliedToItemId() {
return appliedToItemId;
}
public void setAppliedToItemId(String appliedToItemId) {
this.appliedToItemId = appliedToItemId;
}
public DebitMemoItem balance(BigDecimal balance) {
this.balance = balance;
return this;
}
/**
* The balance of the debit memo item.
* @return balance
*/
@javax.annotation.Nullable
public BigDecimal getBalance() {
return balance;
}
public void setBalance(BigDecimal balance) {
this.balance = balance;
}
public DebitMemoItem beAppliedAmount(BigDecimal beAppliedAmount) {
this.beAppliedAmount = beAppliedAmount;
return this;
}
/**
* The applied amount of the debit memo item.
* @return beAppliedAmount
*/
@javax.annotation.Nullable
public BigDecimal getBeAppliedAmount() {
return beAppliedAmount;
}
public void setBeAppliedAmount(BigDecimal beAppliedAmount) {
this.beAppliedAmount = beAppliedAmount;
}
@Deprecated
public DebitMemoItem comment(String comment) {
this.comment = comment;
return this;
}
/**
* Comments about the debit memo item. **Note**: This field is not available if you set the `zuora-version` request header to `257.0` or later.
* @return comment
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public String getComment() {
return comment;
}
@Deprecated
public void setComment(String comment) {
this.comment = comment;
}
public DebitMemoItem createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* The ID of the Zuora user who created the debit memo item.
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public DebitMemoItem createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* The date and time when the debit memo item was created, in `yyyy-mm-dd hh:mm:ss` format. For example, 2017-03-01 15:31:10.
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public DebitMemoItem description(String description) {
this.description = description;
return this;
}
/**
* The description of the debit memo item. **Note**: This field is only available if you set the `zuora-version` request header to `257.0` or later.
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public DebitMemoItem excludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
return this;
}
/**
* The flag to exclude the debit memo item from revenue accounting. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return excludeItemBillingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBillingFromRevenueAccounting() {
return excludeItemBillingFromRevenueAccounting;
}
public void setExcludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
}
public DebitMemoItem financeInformation(GetDebitMemoItemFinanceInformation financeInformation) {
this.financeInformation = financeInformation;
return this;
}
/**
* Get financeInformation
* @return financeInformation
*/
@javax.annotation.Nullable
public GetDebitMemoItemFinanceInformation getFinanceInformation() {
return financeInformation;
}
public void setFinanceInformation(GetDebitMemoItemFinanceInformation financeInformation) {
this.financeInformation = financeInformation;
}
public DebitMemoItem id(String id) {
this.id = id;
return this;
}
/**
* The ID of the debit memo item.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public DebitMemoItem processingType(BillingDocumentItemProcessingType processingType) {
this.processingType = processingType;
return this;
}
/**
* Get processingType
* @return processingType
*/
@javax.annotation.Nullable
public BillingDocumentItemProcessingType getProcessingType() {
return processingType;
}
public void setProcessingType(BillingDocumentItemProcessingType processingType) {
this.processingType = processingType;
}
public DebitMemoItem quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* The number of units for the debit memo item.
* @return quantity
*/
@javax.annotation.Nullable
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public DebitMemoItem reflectDiscountInNetAmount(Boolean reflectDiscountInNetAmount) {
this.reflectDiscountInNetAmount = reflectDiscountInNetAmount;
return this;
}
/**
* The flag to reflect Discount in Apply To Charge Net Amount.
* @return reflectDiscountInNetAmount
*/
@javax.annotation.Nullable
public Boolean getReflectDiscountInNetAmount() {
return reflectDiscountInNetAmount;
}
public void setReflectDiscountInNetAmount(Boolean reflectDiscountInNetAmount) {
this.reflectDiscountInNetAmount = reflectDiscountInNetAmount;
}
public DebitMemoItem serviceEndDate(LocalDate serviceEndDate) {
this.serviceEndDate = serviceEndDate;
return this;
}
/**
* The end date of the service period associated with this debit memo item. Service ends one second before the date specified in this field.
* @return serviceEndDate
*/
@javax.annotation.Nullable
public LocalDate getServiceEndDate() {
return serviceEndDate;
}
public void setServiceEndDate(LocalDate serviceEndDate) {
this.serviceEndDate = serviceEndDate;
}
public DebitMemoItem serviceStartDate(LocalDate serviceStartDate) {
this.serviceStartDate = serviceStartDate;
return this;
}
/**
* The start date of the service period associated with this debit memo item. If the associated charge is a one-time fee, this date is the date of that charge.
* @return serviceStartDate
*/
@javax.annotation.Nullable
public LocalDate getServiceStartDate() {
return serviceStartDate;
}
public void setServiceStartDate(LocalDate serviceStartDate) {
this.serviceStartDate = serviceStartDate;
}
public DebitMemoItem shipToContactId(String shipToContactId) {
this.shipToContactId = shipToContactId;
return this;
}
/**
* The ID of the ship-to contact associated with the debit memo item. The value of this field is `null` if you have the [Flexible Billing Attributes](https://knowledgecenter.zuora.com/Billing/Subscriptions/Flexible_Billing_Attributes) feature disabled.
* @return shipToContactId
*/
@javax.annotation.Nullable
public String getShipToContactId() {
return shipToContactId;
}
public void setShipToContactId(String shipToContactId) {
this.shipToContactId = shipToContactId;
}
public DebitMemoItem sku(String sku) {
this.sku = sku;
return this;
}
/**
* The SKU for the product associated with the debit memo item.
* @return sku
*/
@javax.annotation.Nullable
public String getSku() {
return sku;
}
public void setSku(String sku) {
this.sku = sku;
}
public DebitMemoItem skuName(String skuName) {
this.skuName = skuName;
return this;
}
/**
* The name of the SKU.
* @return skuName
*/
@javax.annotation.Nullable
public String getSkuName() {
return skuName;
}
public void setSkuName(String skuName) {
this.skuName = skuName;
}
public DebitMemoItem soldToContactId(String soldToContactId) {
this.soldToContactId = soldToContactId;
return this;
}
/**
* The ID of the sold-to contact associated with the debit memo item. The value of this field is `null` if you have the [Flexible Billing Attributes](https://knowledgecenter.zuora.com/Billing/Subscriptions/Flexible_Billing_Attributes) feature disabled.
* @return soldToContactId
*/
@javax.annotation.Nullable
public String getSoldToContactId() {
return soldToContactId;
}
public void setSoldToContactId(String soldToContactId) {
this.soldToContactId = soldToContactId;
}
public DebitMemoItem soldToContactSnapshotId(String soldToContactSnapshotId) {
this.soldToContactSnapshotId = soldToContactSnapshotId;
return this;
}
/**
* The ID of the sold-to contact snapshot associated with the invoice item. The value of this field is `null` if you have the [Flexible Billing Attributes](https://knowledgecenter.zuora.com/Billing/Subscriptions/Flexible_Billing_Attributes) feature disabled.
* @return soldToContactSnapshotId
*/
@javax.annotation.Nullable
public String getSoldToContactSnapshotId() {
return soldToContactSnapshotId;
}
public void setSoldToContactSnapshotId(String soldToContactSnapshotId) {
this.soldToContactSnapshotId = soldToContactSnapshotId;
}
public DebitMemoItem sourceItemId(String sourceItemId) {
this.sourceItemId = sourceItemId;
return this;
}
/**
* The ID of the source item.
* @return sourceItemId
*/
@javax.annotation.Nullable
public String getSourceItemId() {
return sourceItemId;
}
public void setSourceItemId(String sourceItemId) {
this.sourceItemId = sourceItemId;
}
public DebitMemoItem sourceItemType(BillingDocumentItemSourceType sourceItemType) {
this.sourceItemType = sourceItemType;
return this;
}
/**
* Get sourceItemType
* @return sourceItemType
*/
@javax.annotation.Nullable
public BillingDocumentItemSourceType getSourceItemType() {
return sourceItemType;
}
public void setSourceItemType(BillingDocumentItemSourceType sourceItemType) {
this.sourceItemType = sourceItemType;
}
public DebitMemoItem subscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/**
* The ID of the subscription associated with the debit memo item.
* @return subscriptionId
*/
@javax.annotation.Nullable
public String getSubscriptionId() {
return subscriptionId;
}
public void setSubscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
}
@Deprecated
public DebitMemoItem taxItems(List taxItems) {
this.taxItems = taxItems;
return this;
}
public DebitMemoItem addTaxItemsItem(GetDebitMemoTaxItem taxItemsItem) {
if (this.taxItems == null) {
this.taxItems = new ArrayList<>();
}
this.taxItems.add(taxItemsItem);
return this;
}
/**
* Container for the taxation items of the debit memo item. **Note**: This field is not available if you set the `zuora-version` request header to `239.0` or later.
* @return taxItems
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public List getTaxItems() {
return taxItems;
}
@Deprecated
public void setTaxItems(List taxItems) {
this.taxItems = taxItems;
}
public DebitMemoItem taxMode(TaxMode taxMode) {
this.taxMode = taxMode;
return this;
}
/**
* Get taxMode
* @return taxMode
*/
@javax.annotation.Nullable
public TaxMode getTaxMode() {
return taxMode;
}
public void setTaxMode(TaxMode taxMode) {
this.taxMode = taxMode;
}
public DebitMemoItem taxationItems(DebitMemoItemTaxationItems taxationItems) {
this.taxationItems = taxationItems;
return this;
}
/**
* Get taxationItems
* @return taxationItems
*/
@javax.annotation.Nullable
public DebitMemoItemTaxationItems getTaxationItems() {
return taxationItems;
}
public void setTaxationItems(DebitMemoItemTaxationItems taxationItems) {
this.taxationItems = taxationItems;
}
public DebitMemoItem unitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
return this;
}
/**
* The units to measure usage.
* @return unitOfMeasure
*/
@javax.annotation.Nullable
public String getUnitOfMeasure() {
return unitOfMeasure;
}
public void setUnitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
}
public DebitMemoItem unitPrice(BigDecimal unitPrice) {
this.unitPrice = unitPrice;
return this;
}
/**
* The per-unit price of the debit memo item.
* @return unitPrice
*/
@javax.annotation.Nullable
public BigDecimal getUnitPrice() {
return unitPrice;
}
public void setUnitPrice(BigDecimal unitPrice) {
this.unitPrice = unitPrice;
}
public DebitMemoItem updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* The ID of the Zuora user who last updated the debit memo item.
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public DebitMemoItem updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* The date and time when the debit memo item was last updated, in `yyyy-mm-dd hh:mm:ss` format. For example, 2017-03-02 15:36:10.
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the DebitMemoItem instance itself
*/
public DebitMemoItem putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DebitMemoItem debitMemoItem = (DebitMemoItem) o;
return Objects.equals(this.amount, debitMemoItem.amount) &&
Objects.equals(this.amountWithoutTax, debitMemoItem.amountWithoutTax) &&
Objects.equals(this.appliedToItemId, debitMemoItem.appliedToItemId) &&
Objects.equals(this.balance, debitMemoItem.balance) &&
Objects.equals(this.beAppliedAmount, debitMemoItem.beAppliedAmount) &&
Objects.equals(this.comment, debitMemoItem.comment) &&
Objects.equals(this.createdById, debitMemoItem.createdById) &&
Objects.equals(this.createdDate, debitMemoItem.createdDate) &&
Objects.equals(this.description, debitMemoItem.description) &&
Objects.equals(this.excludeItemBillingFromRevenueAccounting, debitMemoItem.excludeItemBillingFromRevenueAccounting) &&
Objects.equals(this.financeInformation, debitMemoItem.financeInformation) &&
Objects.equals(this.id, debitMemoItem.id) &&
Objects.equals(this.processingType, debitMemoItem.processingType) &&
Objects.equals(this.quantity, debitMemoItem.quantity) &&
Objects.equals(this.reflectDiscountInNetAmount, debitMemoItem.reflectDiscountInNetAmount) &&
Objects.equals(this.serviceEndDate, debitMemoItem.serviceEndDate) &&
Objects.equals(this.serviceStartDate, debitMemoItem.serviceStartDate) &&
Objects.equals(this.shipToContactId, debitMemoItem.shipToContactId) &&
Objects.equals(this.sku, debitMemoItem.sku) &&
Objects.equals(this.skuName, debitMemoItem.skuName) &&
Objects.equals(this.soldToContactId, debitMemoItem.soldToContactId) &&
Objects.equals(this.soldToContactSnapshotId, debitMemoItem.soldToContactSnapshotId) &&
Objects.equals(this.sourceItemId, debitMemoItem.sourceItemId) &&
Objects.equals(this.sourceItemType, debitMemoItem.sourceItemType) &&
Objects.equals(this.subscriptionId, debitMemoItem.subscriptionId) &&
Objects.equals(this.taxItems, debitMemoItem.taxItems) &&
Objects.equals(this.taxMode, debitMemoItem.taxMode) &&
Objects.equals(this.taxationItems, debitMemoItem.taxationItems) &&
Objects.equals(this.unitOfMeasure, debitMemoItem.unitOfMeasure) &&
Objects.equals(this.unitPrice, debitMemoItem.unitPrice) &&
Objects.equals(this.updatedById, debitMemoItem.updatedById) &&
Objects.equals(this.updatedDate, debitMemoItem.updatedDate)&&
Objects.equals(this.additionalProperties, debitMemoItem.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(amount, amountWithoutTax, appliedToItemId, balance, beAppliedAmount, comment, createdById, createdDate, description, excludeItemBillingFromRevenueAccounting, financeInformation, id, processingType, quantity, reflectDiscountInNetAmount, serviceEndDate, serviceStartDate, shipToContactId, sku, skuName, soldToContactId, soldToContactSnapshotId, sourceItemId, sourceItemType, subscriptionId, taxItems, taxMode, taxationItems, unitOfMeasure, unitPrice, updatedById, updatedDate, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DebitMemoItem {\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" amountWithoutTax: ").append(toIndentedString(amountWithoutTax)).append("\n");
sb.append(" appliedToItemId: ").append(toIndentedString(appliedToItemId)).append("\n");
sb.append(" balance: ").append(toIndentedString(balance)).append("\n");
sb.append(" beAppliedAmount: ").append(toIndentedString(beAppliedAmount)).append("\n");
sb.append(" comment: ").append(toIndentedString(comment)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" excludeItemBillingFromRevenueAccounting: ").append(toIndentedString(excludeItemBillingFromRevenueAccounting)).append("\n");
sb.append(" financeInformation: ").append(toIndentedString(financeInformation)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" processingType: ").append(toIndentedString(processingType)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" reflectDiscountInNetAmount: ").append(toIndentedString(reflectDiscountInNetAmount)).append("\n");
sb.append(" serviceEndDate: ").append(toIndentedString(serviceEndDate)).append("\n");
sb.append(" serviceStartDate: ").append(toIndentedString(serviceStartDate)).append("\n");
sb.append(" shipToContactId: ").append(toIndentedString(shipToContactId)).append("\n");
sb.append(" sku: ").append(toIndentedString(sku)).append("\n");
sb.append(" skuName: ").append(toIndentedString(skuName)).append("\n");
sb.append(" soldToContactId: ").append(toIndentedString(soldToContactId)).append("\n");
sb.append(" soldToContactSnapshotId: ").append(toIndentedString(soldToContactSnapshotId)).append("\n");
sb.append(" sourceItemId: ").append(toIndentedString(sourceItemId)).append("\n");
sb.append(" sourceItemType: ").append(toIndentedString(sourceItemType)).append("\n");
sb.append(" subscriptionId: ").append(toIndentedString(subscriptionId)).append("\n");
sb.append(" taxItems: ").append(toIndentedString(taxItems)).append("\n");
sb.append(" taxMode: ").append(toIndentedString(taxMode)).append("\n");
sb.append(" taxationItems: ").append(toIndentedString(taxationItems)).append("\n");
sb.append(" unitOfMeasure: ").append(toIndentedString(unitOfMeasure)).append("\n");
sb.append(" unitPrice: ").append(toIndentedString(unitPrice)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("amount");
openapiFields.add("amountWithoutTax");
openapiFields.add("appliedToItemId");
openapiFields.add("balance");
openapiFields.add("beAppliedAmount");
openapiFields.add("comment");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("description");
openapiFields.add("excludeItemBillingFromRevenueAccounting");
openapiFields.add("financeInformation");
openapiFields.add("id");
openapiFields.add("processingType");
openapiFields.add("quantity");
openapiFields.add("reflectDiscountInNetAmount");
openapiFields.add("serviceEndDate");
openapiFields.add("serviceStartDate");
openapiFields.add("shipToContactId");
openapiFields.add("sku");
openapiFields.add("skuName");
openapiFields.add("soldToContactId");
openapiFields.add("soldToContactSnapshotId");
openapiFields.add("sourceItemId");
openapiFields.add("sourceItemType");
openapiFields.add("subscriptionId");
openapiFields.add("taxItems");
openapiFields.add("taxMode");
openapiFields.add("taxationItems");
openapiFields.add("unitOfMeasure");
openapiFields.add("unitPrice");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to DebitMemoItem
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!DebitMemoItem.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in DebitMemoItem is not found in the empty JSON string", DebitMemoItem.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("appliedToItemId") != null && !jsonObj.get("appliedToItemId").isJsonNull()) && !jsonObj.get("appliedToItemId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `appliedToItemId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("appliedToItemId").toString()));
}
if ((jsonObj.get("comment") != null && !jsonObj.get("comment").isJsonNull()) && !jsonObj.get("comment").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `comment` to be a primitive type in the JSON string but got `%s`", jsonObj.get("comment").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
// validate the optional field `financeInformation`
if (jsonObj.get("financeInformation") != null && !jsonObj.get("financeInformation").isJsonNull()) {
GetDebitMemoItemFinanceInformation.validateJsonElement(jsonObj.get("financeInformation"));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("processingType") != null && !jsonObj.get("processingType").isJsonNull()) && !jsonObj.get("processingType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `processingType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("processingType").toString()));
}
// validate the optional field `processingType`
if (jsonObj.get("processingType") != null && !jsonObj.get("processingType").isJsonNull()) {
BillingDocumentItemProcessingType.validateJsonElement(jsonObj.get("processingType"));
}
if ((jsonObj.get("shipToContactId") != null && !jsonObj.get("shipToContactId").isJsonNull()) && !jsonObj.get("shipToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `shipToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("shipToContactId").toString()));
}
if ((jsonObj.get("sku") != null && !jsonObj.get("sku").isJsonNull()) && !jsonObj.get("sku").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sku` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sku").toString()));
}
if ((jsonObj.get("skuName") != null && !jsonObj.get("skuName").isJsonNull()) && !jsonObj.get("skuName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `skuName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("skuName").toString()));
}
if ((jsonObj.get("soldToContactId") != null && !jsonObj.get("soldToContactId").isJsonNull()) && !jsonObj.get("soldToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToContactId").toString()));
}
if ((jsonObj.get("soldToContactSnapshotId") != null && !jsonObj.get("soldToContactSnapshotId").isJsonNull()) && !jsonObj.get("soldToContactSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToContactSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToContactSnapshotId").toString()));
}
if ((jsonObj.get("sourceItemId") != null && !jsonObj.get("sourceItemId").isJsonNull()) && !jsonObj.get("sourceItemId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sourceItemId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sourceItemId").toString()));
}
if ((jsonObj.get("sourceItemType") != null && !jsonObj.get("sourceItemType").isJsonNull()) && !jsonObj.get("sourceItemType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sourceItemType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sourceItemType").toString()));
}
// validate the optional field `sourceItemType`
if (jsonObj.get("sourceItemType") != null && !jsonObj.get("sourceItemType").isJsonNull()) {
BillingDocumentItemSourceType.validateJsonElement(jsonObj.get("sourceItemType"));
}
if ((jsonObj.get("subscriptionId") != null && !jsonObj.get("subscriptionId").isJsonNull()) && !jsonObj.get("subscriptionId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("subscriptionId").toString()));
}
if (jsonObj.get("taxItems") != null && !jsonObj.get("taxItems").isJsonNull()) {
JsonArray jsonArraytaxItems = jsonObj.getAsJsonArray("taxItems");
if (jsonArraytaxItems != null) {
// ensure the json data is an array
if (!jsonObj.get("taxItems").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `taxItems` to be an array in the JSON string but got `%s`", jsonObj.get("taxItems").toString()));
}
// validate the optional field `taxItems` (array)
for (int i = 0; i < jsonArraytaxItems.size(); i++) {
GetDebitMemoTaxItem.validateJsonElement(jsonArraytaxItems.get(i));
};
}
}
if ((jsonObj.get("taxMode") != null && !jsonObj.get("taxMode").isJsonNull()) && !jsonObj.get("taxMode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxMode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxMode").toString()));
}
// validate the optional field `taxMode`
if (jsonObj.get("taxMode") != null && !jsonObj.get("taxMode").isJsonNull()) {
TaxMode.validateJsonElement(jsonObj.get("taxMode"));
}
// validate the optional field `taxationItems`
if (jsonObj.get("taxationItems") != null && !jsonObj.get("taxationItems").isJsonNull()) {
DebitMemoItemTaxationItems.validateJsonElement(jsonObj.get("taxationItems"));
}
if ((jsonObj.get("unitOfMeasure") != null && !jsonObj.get("unitOfMeasure").isJsonNull()) && !jsonObj.get("unitOfMeasure").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `unitOfMeasure` to be a primitive type in the JSON string but got `%s`", jsonObj.get("unitOfMeasure").toString()));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!DebitMemoItem.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'DebitMemoItem' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(DebitMemoItem.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, DebitMemoItem value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public DebitMemoItem read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
DebitMemoItem instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of DebitMemoItem given an JSON string
*
* @param jsonString JSON string
* @return An instance of DebitMemoItem
* @throws IOException if the JSON string is invalid with respect to DebitMemoItem
*/
public static DebitMemoItem fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, DebitMemoItem.class);
}
/**
* Convert an instance of DebitMemoItem to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy