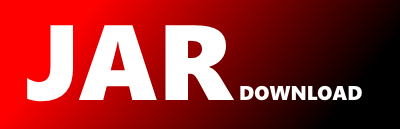
com.zuora.model.DebitMemoResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.BillingDocumentStatus;
import com.zuora.model.EInvoiceStatus;
import com.zuora.model.FailedReason;
import com.zuora.model.MemoSourceType;
import com.zuora.model.TaxStatus;
import com.zuora.model.TransferredToAccountingStatus;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* DebitMemoResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class DebitMemoResponse {
public static final String SERIALIZED_NAME_PROCESS_ID = "processId";
@SerializedName(SERIALIZED_NAME_PROCESS_ID)
private String processId;
public static final String SERIALIZED_NAME_REQUEST_ID = "requestId";
@SerializedName(SERIALIZED_NAME_REQUEST_ID)
private String requestId;
public static final String SERIALIZED_NAME_REASONS = "reasons";
@SerializedName(SERIALIZED_NAME_REASONS)
private List reasons;
public static final String SERIALIZED_NAME_SUCCESS = "success";
@SerializedName(SERIALIZED_NAME_SUCCESS)
private Boolean success;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "accountId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "accountNumber";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_AUTO_PAY = "autoPay";
@SerializedName(SERIALIZED_NAME_AUTO_PAY)
private Boolean autoPay;
public static final String SERIALIZED_NAME_BALANCE = "balance";
@SerializedName(SERIALIZED_NAME_BALANCE)
private BigDecimal balance;
public static final String SERIALIZED_NAME_BE_APPLIED_AMOUNT = "beAppliedAmount";
@SerializedName(SERIALIZED_NAME_BE_APPLIED_AMOUNT)
private BigDecimal beAppliedAmount;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT_ID = "billToContactId";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT_ID)
private String billToContactId;
public static final String SERIALIZED_NAME_CANCELLED_BY_ID = "cancelledById";
@SerializedName(SERIALIZED_NAME_CANCELLED_BY_ID)
private String cancelledById;
public static final String SERIALIZED_NAME_CANCELLED_ON = "cancelledOn";
@SerializedName(SERIALIZED_NAME_CANCELLED_ON)
private String cancelledOn;
public static final String SERIALIZED_NAME_COMMENT = "comment";
@SerializedName(SERIALIZED_NAME_COMMENT)
private String comment;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_DEBIT_MEMO_DATE = "debitMemoDate";
@SerializedName(SERIALIZED_NAME_DEBIT_MEMO_DATE)
private LocalDate debitMemoDate;
public static final String SERIALIZED_NAME_DUE_DATE = "dueDate";
@SerializedName(SERIALIZED_NAME_DUE_DATE)
private LocalDate dueDate;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_INVOICE_GROUP_NUMBER = "invoiceGroupNumber";
@SerializedName(SERIALIZED_NAME_INVOICE_GROUP_NUMBER)
private String invoiceGroupNumber;
public static final String SERIALIZED_NAME_LATEST_P_D_F_FILE_ID = "latestPDFFileId";
@SerializedName(SERIALIZED_NAME_LATEST_P_D_F_FILE_ID)
private String latestPDFFileId;
public static final String SERIALIZED_NAME_NUMBER = "number";
@SerializedName(SERIALIZED_NAME_NUMBER)
private String number;
public static final String SERIALIZED_NAME_PAYMENT_TERM = "paymentTerm";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERM)
private String paymentTerm;
public static final String SERIALIZED_NAME_POSTED_BY_ID = "postedById";
@SerializedName(SERIALIZED_NAME_POSTED_BY_ID)
private String postedById;
public static final String SERIALIZED_NAME_POSTED_ON = "postedOn";
@SerializedName(SERIALIZED_NAME_POSTED_ON)
private String postedOn;
public static final String SERIALIZED_NAME_REASON_CODE = "reasonCode";
@SerializedName(SERIALIZED_NAME_REASON_CODE)
private String reasonCode;
public static final String SERIALIZED_NAME_REFERRED_CREDIT_MEMO_ID = "referredCreditMemoId";
@SerializedName(SERIALIZED_NAME_REFERRED_CREDIT_MEMO_ID)
private String referredCreditMemoId;
public static final String SERIALIZED_NAME_REFERRED_INVOICE_ID = "referredInvoiceId";
@SerializedName(SERIALIZED_NAME_REFERRED_INVOICE_ID)
private String referredInvoiceId;
public static final String SERIALIZED_NAME_SEQUENCE_SET_ID = "sequenceSetId";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_ID)
private String sequenceSetId;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT_ID = "soldToContactId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT_ID)
private String soldToContactId;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT_SNAPSHOT_ID = "soldToContactSnapshotId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT_SNAPSHOT_ID)
private String soldToContactSnapshotId;
public static final String SERIALIZED_NAME_SOURCE_TYPE = "sourceType";
@SerializedName(SERIALIZED_NAME_SOURCE_TYPE)
private MemoSourceType sourceType;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private BillingDocumentStatus status;
public static final String SERIALIZED_NAME_TARGET_DATE = "targetDate";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public static final String SERIALIZED_NAME_TAX_AMOUNT = "taxAmount";
@SerializedName(SERIALIZED_NAME_TAX_AMOUNT)
private BigDecimal taxAmount;
public static final String SERIALIZED_NAME_TAX_MESSAGE = "taxMessage";
@SerializedName(SERIALIZED_NAME_TAX_MESSAGE)
private String taxMessage;
public static final String SERIALIZED_NAME_TAX_STATUS = "taxStatus";
@SerializedName(SERIALIZED_NAME_TAX_STATUS)
private TaxStatus taxStatus;
public static final String SERIALIZED_NAME_TOTAL_TAX_EXEMPT_AMOUNT = "totalTaxExemptAmount";
@SerializedName(SERIALIZED_NAME_TOTAL_TAX_EXEMPT_AMOUNT)
private BigDecimal totalTaxExemptAmount;
public static final String SERIALIZED_NAME_TRANSFERRED_TO_ACCOUNTING = "transferredToAccounting";
@SerializedName(SERIALIZED_NAME_TRANSFERRED_TO_ACCOUNTING)
private TransferredToAccountingStatus transferredToAccounting;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public static final String SERIALIZED_NAME_E_INVOICE_STATUS = "eInvoiceStatus";
@SerializedName(SERIALIZED_NAME_E_INVOICE_STATUS)
private EInvoiceStatus eInvoiceStatus;
public static final String SERIALIZED_NAME_E_INVOICE_ERROR_CODE = "eInvoiceErrorCode";
@SerializedName(SERIALIZED_NAME_E_INVOICE_ERROR_CODE)
private String eInvoiceErrorCode;
public static final String SERIALIZED_NAME_E_INVOICE_ERROR_MESSAGE = "eInvoiceErrorMessage";
@SerializedName(SERIALIZED_NAME_E_INVOICE_ERROR_MESSAGE)
private String eInvoiceErrorMessage;
public static final String SERIALIZED_NAME_E_INVOICE_FILE_ID = "eInvoiceFileId";
@SerializedName(SERIALIZED_NAME_E_INVOICE_FILE_ID)
private String eInvoiceFileId;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT_SNAPSHOT_ID = "billToContactSnapshotId";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT_SNAPSHOT_ID)
private String billToContactSnapshotId;
public static final String SERIALIZED_NAME_ORGANIZATION_LABEL = "organizationLabel";
@SerializedName(SERIALIZED_NAME_ORGANIZATION_LABEL)
private String organizationLabel;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_INTEGRATION_ID_N_S = "IntegrationId__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_ID_N_S)
private String integrationIdNS;
public static final String SERIALIZED_NAME_INTEGRATION_STATUS_N_S = "IntegrationStatus__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_STATUS_N_S)
private String integrationStatusNS;
public static final String SERIALIZED_NAME_SYNC_DATE_N_S = "SyncDate__NS";
@SerializedName(SERIALIZED_NAME_SYNC_DATE_N_S)
private String syncDateNS;
public DebitMemoResponse() {
}
public DebitMemoResponse processId(String processId) {
this.processId = processId;
return this;
}
/**
* The Id of the process that handle the operation.
* @return processId
*/
@javax.annotation.Nullable
public String getProcessId() {
return processId;
}
public void setProcessId(String processId) {
this.processId = processId;
}
public DebitMemoResponse requestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* Unique request identifier. If you need to contact us about a specific request, providing the request identifier will ensure the fastest possible resolution.
* @return requestId
*/
@javax.annotation.Nullable
public String getRequestId() {
return requestId;
}
public void setRequestId(String requestId) {
this.requestId = requestId;
}
public DebitMemoResponse reasons(List reasons) {
this.reasons = reasons;
return this;
}
public DebitMemoResponse addReasonsItem(FailedReason reasonsItem) {
if (this.reasons == null) {
this.reasons = new ArrayList<>();
}
this.reasons.add(reasonsItem);
return this;
}
/**
* Get reasons
* @return reasons
*/
@javax.annotation.Nullable
public List getReasons() {
return reasons;
}
public void setReasons(List reasons) {
this.reasons = reasons;
}
public DebitMemoResponse success(Boolean success) {
this.success = success;
return this;
}
/**
* Indicates whether the call succeeded.
* @return success
*/
@javax.annotation.Nullable
public Boolean getSuccess() {
return success;
}
public void setSuccess(Boolean success) {
this.success = success;
}
public DebitMemoResponse accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* The ID of the customer account associated with the debit memo.
* @return accountId
*/
@javax.annotation.Nullable
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public DebitMemoResponse accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* The number of the customer account associated with the debit memo.
* @return accountNumber
*/
@javax.annotation.Nullable
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public DebitMemoResponse amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The total amount of the debit memo.
* @return amount
*/
@javax.annotation.Nullable
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public DebitMemoResponse autoPay(Boolean autoPay) {
this.autoPay = autoPay;
return this;
}
/**
* Whether debit memos are automatically picked up for processing in the corresponding payment run. By default, debit memos are automatically picked up for processing in the corresponding payment run.
* @return autoPay
*/
@javax.annotation.Nullable
public Boolean getAutoPay() {
return autoPay;
}
public void setAutoPay(Boolean autoPay) {
this.autoPay = autoPay;
}
public DebitMemoResponse balance(BigDecimal balance) {
this.balance = balance;
return this;
}
/**
* The balance of the debit memo.
* @return balance
*/
@javax.annotation.Nullable
public BigDecimal getBalance() {
return balance;
}
public void setBalance(BigDecimal balance) {
this.balance = balance;
}
public DebitMemoResponse beAppliedAmount(BigDecimal beAppliedAmount) {
this.beAppliedAmount = beAppliedAmount;
return this;
}
/**
* The applied amount of the debit memo.
* @return beAppliedAmount
*/
@javax.annotation.Nullable
public BigDecimal getBeAppliedAmount() {
return beAppliedAmount;
}
public void setBeAppliedAmount(BigDecimal beAppliedAmount) {
this.beAppliedAmount = beAppliedAmount;
}
public DebitMemoResponse billToContactId(String billToContactId) {
this.billToContactId = billToContactId;
return this;
}
/**
* The ID of the bill-to contact associated with the debit memo. The value of this field is `null` if you have the [Flexible Billing Attributes](https://knowledgecenter.zuora.com/Billing/Subscriptions/Flexible_Billing_Attributes) feature disabled.
* @return billToContactId
*/
@javax.annotation.Nullable
public String getBillToContactId() {
return billToContactId;
}
public void setBillToContactId(String billToContactId) {
this.billToContactId = billToContactId;
}
public DebitMemoResponse cancelledById(String cancelledById) {
this.cancelledById = cancelledById;
return this;
}
/**
* The ID of the Zuora user who cancelled the debit memo.
* @return cancelledById
*/
@javax.annotation.Nullable
public String getCancelledById() {
return cancelledById;
}
public void setCancelledById(String cancelledById) {
this.cancelledById = cancelledById;
}
public DebitMemoResponse cancelledOn(String cancelledOn) {
this.cancelledOn = cancelledOn;
return this;
}
/**
* The date and time when the debit memo was cancelled, in `yyyy-mm-dd hh:mm:ss` format.
* @return cancelledOn
*/
@javax.annotation.Nullable
public String getCancelledOn() {
return cancelledOn;
}
public void setCancelledOn(String cancelledOn) {
this.cancelledOn = cancelledOn;
}
public DebitMemoResponse comment(String comment) {
this.comment = comment;
return this;
}
/**
* Comments about the debit memo.
* @return comment
*/
@javax.annotation.Nullable
public String getComment() {
return comment;
}
public void setComment(String comment) {
this.comment = comment;
}
public DebitMemoResponse createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* The ID of the Zuora user who created the debit memo.
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public DebitMemoResponse createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* The date and time when the debit memo was created, in `yyyy-mm-dd hh:mm:ss` format. For example, 2017-03-01 15:31:10.
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public DebitMemoResponse debitMemoDate(LocalDate debitMemoDate) {
this.debitMemoDate = debitMemoDate;
return this;
}
/**
* The date when the debit memo takes effect, in `yyyy-mm-dd` format. For example, 2017-05-20.
* @return debitMemoDate
*/
@javax.annotation.Nullable
public LocalDate getDebitMemoDate() {
return debitMemoDate;
}
public void setDebitMemoDate(LocalDate debitMemoDate) {
this.debitMemoDate = debitMemoDate;
}
public DebitMemoResponse dueDate(LocalDate dueDate) {
this.dueDate = dueDate;
return this;
}
/**
* The date by which the payment for the debit memo is due, in `yyyy-mm-dd` format.
* @return dueDate
*/
@javax.annotation.Nullable
public LocalDate getDueDate() {
return dueDate;
}
public void setDueDate(LocalDate dueDate) {
this.dueDate = dueDate;
}
public DebitMemoResponse id(String id) {
this.id = id;
return this;
}
/**
* The unique ID of the debit memo.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public DebitMemoResponse invoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
return this;
}
/**
* The number of invoice group associated with the debit memo. **Note**: This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_customers_at_subscription_level/Flexible_Billing_Attributes\" target=\"_blank\">Flexible Billing Attributes</a> feature enabled.
* @return invoiceGroupNumber
*/
@javax.annotation.Nullable
public String getInvoiceGroupNumber() {
return invoiceGroupNumber;
}
public void setInvoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
}
public DebitMemoResponse latestPDFFileId(String latestPDFFileId) {
this.latestPDFFileId = latestPDFFileId;
return this;
}
/**
* The ID of the latest PDF file generated for the debit memo.
* @return latestPDFFileId
*/
@javax.annotation.Nullable
public String getLatestPDFFileId() {
return latestPDFFileId;
}
public void setLatestPDFFileId(String latestPDFFileId) {
this.latestPDFFileId = latestPDFFileId;
}
public DebitMemoResponse number(String number) {
this.number = number;
return this;
}
/**
* The unique identification number of the debit memo.
* @return number
*/
@javax.annotation.Nullable
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public DebitMemoResponse paymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
return this;
}
/**
* The name of the payment term associated with the debit memo. The value of this field is `null` if you have the [Flexible Billing Attributes](https://knowledgecenter.zuora.com/Billing/Subscriptions/Flexible_Billing_Attributes) feature disabled.
* @return paymentTerm
*/
@javax.annotation.Nullable
public String getPaymentTerm() {
return paymentTerm;
}
public void setPaymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
}
public DebitMemoResponse postedById(String postedById) {
this.postedById = postedById;
return this;
}
/**
* The ID of the Zuora user who posted the debit memo.
* @return postedById
*/
@javax.annotation.Nullable
public String getPostedById() {
return postedById;
}
public void setPostedById(String postedById) {
this.postedById = postedById;
}
public DebitMemoResponse postedOn(String postedOn) {
this.postedOn = postedOn;
return this;
}
/**
* The date and time when the debit memo was posted, in `yyyy-mm-dd hh:mm:ss` format.
* @return postedOn
*/
@javax.annotation.Nullable
public String getPostedOn() {
return postedOn;
}
public void setPostedOn(String postedOn) {
this.postedOn = postedOn;
}
public DebitMemoResponse reasonCode(String reasonCode) {
this.reasonCode = reasonCode;
return this;
}
/**
* A code identifying the reason for the transaction. The value must be an existing reason code or empty.
* @return reasonCode
*/
@javax.annotation.Nullable
public String getReasonCode() {
return reasonCode;
}
public void setReasonCode(String reasonCode) {
this.reasonCode = reasonCode;
}
public DebitMemoResponse referredCreditMemoId(String referredCreditMemoId) {
this.referredCreditMemoId = referredCreditMemoId;
return this;
}
/**
* The ID of the credit memo from which the debit memo was created.
* @return referredCreditMemoId
*/
@javax.annotation.Nullable
public String getReferredCreditMemoId() {
return referredCreditMemoId;
}
public void setReferredCreditMemoId(String referredCreditMemoId) {
this.referredCreditMemoId = referredCreditMemoId;
}
public DebitMemoResponse referredInvoiceId(String referredInvoiceId) {
this.referredInvoiceId = referredInvoiceId;
return this;
}
/**
* The ID of a referred invoice.
* @return referredInvoiceId
*/
@javax.annotation.Nullable
public String getReferredInvoiceId() {
return referredInvoiceId;
}
public void setReferredInvoiceId(String referredInvoiceId) {
this.referredInvoiceId = referredInvoiceId;
}
public DebitMemoResponse sequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
return this;
}
/**
* The ID of the sequence set associated with the debit memo. The value of this field is `null` if you have the [Flexible Billing Attributes](https://knowledgecenter.zuora.com/Billing/Subscriptions/Flexible_Billing_Attributes) feature disabled.
* @return sequenceSetId
*/
@javax.annotation.Nullable
public String getSequenceSetId() {
return sequenceSetId;
}
public void setSequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
}
public DebitMemoResponse soldToContactId(String soldToContactId) {
this.soldToContactId = soldToContactId;
return this;
}
/**
* The ID of the sold-to contact associated with the debit memo. The value of this field is `null` if you have the [Flexible Billing Attributes](https://knowledgecenter.zuora.com/Billing/Subscriptions/Flexible_Billing_Attributes) feature disabled.
* @return soldToContactId
*/
@javax.annotation.Nullable
public String getSoldToContactId() {
return soldToContactId;
}
public void setSoldToContactId(String soldToContactId) {
this.soldToContactId = soldToContactId;
}
public DebitMemoResponse soldToContactSnapshotId(String soldToContactSnapshotId) {
this.soldToContactSnapshotId = soldToContactSnapshotId;
return this;
}
/**
* The ID of the sold-to contact snapshot associated with the debit memo. The value of this field is `null` if you have the [Flexible Billing Attributes](https://knowledgecenter.zuora.com/Billing/Subscriptions/Flexible_Billing_Attributes) feature disabled.
* @return soldToContactSnapshotId
*/
@javax.annotation.Nullable
public String getSoldToContactSnapshotId() {
return soldToContactSnapshotId;
}
public void setSoldToContactSnapshotId(String soldToContactSnapshotId) {
this.soldToContactSnapshotId = soldToContactSnapshotId;
}
public DebitMemoResponse sourceType(MemoSourceType sourceType) {
this.sourceType = sourceType;
return this;
}
/**
* Get sourceType
* @return sourceType
*/
@javax.annotation.Nullable
public MemoSourceType getSourceType() {
return sourceType;
}
public void setSourceType(MemoSourceType sourceType) {
this.sourceType = sourceType;
}
public DebitMemoResponse status(BillingDocumentStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public BillingDocumentStatus getStatus() {
return status;
}
public void setStatus(BillingDocumentStatus status) {
this.status = status;
}
public DebitMemoResponse targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* The target date for the debit memo, in `yyyy-mm-dd` format. For example, 2017-07-20.
* @return targetDate
*/
@javax.annotation.Nullable
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public DebitMemoResponse taxAmount(BigDecimal taxAmount) {
this.taxAmount = taxAmount;
return this;
}
/**
* The amount of taxation.
* @return taxAmount
*/
@javax.annotation.Nullable
public BigDecimal getTaxAmount() {
return taxAmount;
}
public void setTaxAmount(BigDecimal taxAmount) {
this.taxAmount = taxAmount;
}
public DebitMemoResponse taxMessage(String taxMessage) {
this.taxMessage = taxMessage;
return this;
}
/**
* The message about the status of tax calculation related to the debit memo. If tax calculation fails in one debit memo, this field displays the reason for the failure.
* @return taxMessage
*/
@javax.annotation.Nullable
public String getTaxMessage() {
return taxMessage;
}
public void setTaxMessage(String taxMessage) {
this.taxMessage = taxMessage;
}
public DebitMemoResponse taxStatus(TaxStatus taxStatus) {
this.taxStatus = taxStatus;
return this;
}
/**
* Get taxStatus
* @return taxStatus
*/
@javax.annotation.Nullable
public TaxStatus getTaxStatus() {
return taxStatus;
}
public void setTaxStatus(TaxStatus taxStatus) {
this.taxStatus = taxStatus;
}
public DebitMemoResponse totalTaxExemptAmount(BigDecimal totalTaxExemptAmount) {
this.totalTaxExemptAmount = totalTaxExemptAmount;
return this;
}
/**
* The calculated tax amount excluded due to the exemption.
* @return totalTaxExemptAmount
*/
@javax.annotation.Nullable
public BigDecimal getTotalTaxExemptAmount() {
return totalTaxExemptAmount;
}
public void setTotalTaxExemptAmount(BigDecimal totalTaxExemptAmount) {
this.totalTaxExemptAmount = totalTaxExemptAmount;
}
public DebitMemoResponse transferredToAccounting(TransferredToAccountingStatus transferredToAccounting) {
this.transferredToAccounting = transferredToAccounting;
return this;
}
/**
* Get transferredToAccounting
* @return transferredToAccounting
*/
@javax.annotation.Nullable
public TransferredToAccountingStatus getTransferredToAccounting() {
return transferredToAccounting;
}
public void setTransferredToAccounting(TransferredToAccountingStatus transferredToAccounting) {
this.transferredToAccounting = transferredToAccounting;
}
public DebitMemoResponse updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* The ID of the Zuora user who last updated the debit memo.
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public DebitMemoResponse updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* The date and time when the debit memo was last updated, in `yyyy-mm-dd hh:mm:ss` format. For example, 2017-03-02 15:31:10.
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
public DebitMemoResponse eInvoiceStatus(EInvoiceStatus eInvoiceStatus) {
this.eInvoiceStatus = eInvoiceStatus;
return this;
}
/**
* Get eInvoiceStatus
* @return eInvoiceStatus
*/
@javax.annotation.Nullable
public EInvoiceStatus geteInvoiceStatus() {
return eInvoiceStatus;
}
public void seteInvoiceStatus(EInvoiceStatus eInvoiceStatus) {
this.eInvoiceStatus = eInvoiceStatus;
}
public DebitMemoResponse eInvoiceErrorCode(String eInvoiceErrorCode) {
this.eInvoiceErrorCode = eInvoiceErrorCode;
return this;
}
/**
* eInvoiceErrorCode.
* @return eInvoiceErrorCode
*/
@javax.annotation.Nullable
public String geteInvoiceErrorCode() {
return eInvoiceErrorCode;
}
public void seteInvoiceErrorCode(String eInvoiceErrorCode) {
this.eInvoiceErrorCode = eInvoiceErrorCode;
}
public DebitMemoResponse eInvoiceErrorMessage(String eInvoiceErrorMessage) {
this.eInvoiceErrorMessage = eInvoiceErrorMessage;
return this;
}
/**
* eInvoiceErrorMessage.
* @return eInvoiceErrorMessage
*/
@javax.annotation.Nullable
public String geteInvoiceErrorMessage() {
return eInvoiceErrorMessage;
}
public void seteInvoiceErrorMessage(String eInvoiceErrorMessage) {
this.eInvoiceErrorMessage = eInvoiceErrorMessage;
}
public DebitMemoResponse eInvoiceFileId(String eInvoiceFileId) {
this.eInvoiceFileId = eInvoiceFileId;
return this;
}
/**
* eInvoiceFileId.
* @return eInvoiceFileId
*/
@javax.annotation.Nullable
public String geteInvoiceFileId() {
return eInvoiceFileId;
}
public void seteInvoiceFileId(String eInvoiceFileId) {
this.eInvoiceFileId = eInvoiceFileId;
}
public DebitMemoResponse billToContactSnapshotId(String billToContactSnapshotId) {
this.billToContactSnapshotId = billToContactSnapshotId;
return this;
}
/**
* billToContactSnapshotId.
* @return billToContactSnapshotId
*/
@javax.annotation.Nullable
public String getBillToContactSnapshotId() {
return billToContactSnapshotId;
}
public void setBillToContactSnapshotId(String billToContactSnapshotId) {
this.billToContactSnapshotId = billToContactSnapshotId;
}
public DebitMemoResponse organizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
return this;
}
/**
* organization label.
* @return organizationLabel
*/
@javax.annotation.Nullable
public String getOrganizationLabel() {
return organizationLabel;
}
public void setOrganizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
}
public DebitMemoResponse currency(String currency) {
this.currency = currency;
return this;
}
/**
* Currency code.
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public DebitMemoResponse integrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
return this;
}
/**
* ID of the corresponding object in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationIdNS
*/
@javax.annotation.Nullable
public String getIntegrationIdNS() {
return integrationIdNS;
}
public void setIntegrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
}
public DebitMemoResponse integrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
return this;
}
/**
* Status of the debit memo's synchronization with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationStatusNS
*/
@javax.annotation.Nullable
public String getIntegrationStatusNS() {
return integrationStatusNS;
}
public void setIntegrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
}
public DebitMemoResponse syncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
return this;
}
/**
* Date when the debit memo was synchronized with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return syncDateNS
*/
@javax.annotation.Nullable
public String getSyncDateNS() {
return syncDateNS;
}
public void setSyncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the DebitMemoResponse instance itself
*/
public DebitMemoResponse putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DebitMemoResponse debitMemoResponse = (DebitMemoResponse) o;
return Objects.equals(this.processId, debitMemoResponse.processId) &&
Objects.equals(this.requestId, debitMemoResponse.requestId) &&
Objects.equals(this.reasons, debitMemoResponse.reasons) &&
Objects.equals(this.success, debitMemoResponse.success) &&
Objects.equals(this.accountId, debitMemoResponse.accountId) &&
Objects.equals(this.accountNumber, debitMemoResponse.accountNumber) &&
Objects.equals(this.amount, debitMemoResponse.amount) &&
Objects.equals(this.autoPay, debitMemoResponse.autoPay) &&
Objects.equals(this.balance, debitMemoResponse.balance) &&
Objects.equals(this.beAppliedAmount, debitMemoResponse.beAppliedAmount) &&
Objects.equals(this.billToContactId, debitMemoResponse.billToContactId) &&
Objects.equals(this.cancelledById, debitMemoResponse.cancelledById) &&
Objects.equals(this.cancelledOn, debitMemoResponse.cancelledOn) &&
Objects.equals(this.comment, debitMemoResponse.comment) &&
Objects.equals(this.createdById, debitMemoResponse.createdById) &&
Objects.equals(this.createdDate, debitMemoResponse.createdDate) &&
Objects.equals(this.debitMemoDate, debitMemoResponse.debitMemoDate) &&
Objects.equals(this.dueDate, debitMemoResponse.dueDate) &&
Objects.equals(this.id, debitMemoResponse.id) &&
Objects.equals(this.invoiceGroupNumber, debitMemoResponse.invoiceGroupNumber) &&
Objects.equals(this.latestPDFFileId, debitMemoResponse.latestPDFFileId) &&
Objects.equals(this.number, debitMemoResponse.number) &&
Objects.equals(this.paymentTerm, debitMemoResponse.paymentTerm) &&
Objects.equals(this.postedById, debitMemoResponse.postedById) &&
Objects.equals(this.postedOn, debitMemoResponse.postedOn) &&
Objects.equals(this.reasonCode, debitMemoResponse.reasonCode) &&
Objects.equals(this.referredCreditMemoId, debitMemoResponse.referredCreditMemoId) &&
Objects.equals(this.referredInvoiceId, debitMemoResponse.referredInvoiceId) &&
Objects.equals(this.sequenceSetId, debitMemoResponse.sequenceSetId) &&
Objects.equals(this.soldToContactId, debitMemoResponse.soldToContactId) &&
Objects.equals(this.soldToContactSnapshotId, debitMemoResponse.soldToContactSnapshotId) &&
Objects.equals(this.sourceType, debitMemoResponse.sourceType) &&
Objects.equals(this.status, debitMemoResponse.status) &&
Objects.equals(this.targetDate, debitMemoResponse.targetDate) &&
Objects.equals(this.taxAmount, debitMemoResponse.taxAmount) &&
Objects.equals(this.taxMessage, debitMemoResponse.taxMessage) &&
Objects.equals(this.taxStatus, debitMemoResponse.taxStatus) &&
Objects.equals(this.totalTaxExemptAmount, debitMemoResponse.totalTaxExemptAmount) &&
Objects.equals(this.transferredToAccounting, debitMemoResponse.transferredToAccounting) &&
Objects.equals(this.updatedById, debitMemoResponse.updatedById) &&
Objects.equals(this.updatedDate, debitMemoResponse.updatedDate) &&
Objects.equals(this.eInvoiceStatus, debitMemoResponse.eInvoiceStatus) &&
Objects.equals(this.eInvoiceErrorCode, debitMemoResponse.eInvoiceErrorCode) &&
Objects.equals(this.eInvoiceErrorMessage, debitMemoResponse.eInvoiceErrorMessage) &&
Objects.equals(this.eInvoiceFileId, debitMemoResponse.eInvoiceFileId) &&
Objects.equals(this.billToContactSnapshotId, debitMemoResponse.billToContactSnapshotId) &&
Objects.equals(this.organizationLabel, debitMemoResponse.organizationLabel) &&
Objects.equals(this.currency, debitMemoResponse.currency) &&
Objects.equals(this.integrationIdNS, debitMemoResponse.integrationIdNS) &&
Objects.equals(this.integrationStatusNS, debitMemoResponse.integrationStatusNS) &&
Objects.equals(this.syncDateNS, debitMemoResponse.syncDateNS)&&
Objects.equals(this.additionalProperties, debitMemoResponse.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(processId, requestId, reasons, success, accountId, accountNumber, amount, autoPay, balance, beAppliedAmount, billToContactId, cancelledById, cancelledOn, comment, createdById, createdDate, debitMemoDate, dueDate, id, invoiceGroupNumber, latestPDFFileId, number, paymentTerm, postedById, postedOn, reasonCode, referredCreditMemoId, referredInvoiceId, sequenceSetId, soldToContactId, soldToContactSnapshotId, sourceType, status, targetDate, taxAmount, taxMessage, taxStatus, totalTaxExemptAmount, transferredToAccounting, updatedById, updatedDate, eInvoiceStatus, eInvoiceErrorCode, eInvoiceErrorMessage, eInvoiceFileId, billToContactSnapshotId, organizationLabel, currency, integrationIdNS, integrationStatusNS, syncDateNS, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DebitMemoResponse {\n");
sb.append(" processId: ").append(toIndentedString(processId)).append("\n");
sb.append(" requestId: ").append(toIndentedString(requestId)).append("\n");
sb.append(" reasons: ").append(toIndentedString(reasons)).append("\n");
sb.append(" success: ").append(toIndentedString(success)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" autoPay: ").append(toIndentedString(autoPay)).append("\n");
sb.append(" balance: ").append(toIndentedString(balance)).append("\n");
sb.append(" beAppliedAmount: ").append(toIndentedString(beAppliedAmount)).append("\n");
sb.append(" billToContactId: ").append(toIndentedString(billToContactId)).append("\n");
sb.append(" cancelledById: ").append(toIndentedString(cancelledById)).append("\n");
sb.append(" cancelledOn: ").append(toIndentedString(cancelledOn)).append("\n");
sb.append(" comment: ").append(toIndentedString(comment)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" debitMemoDate: ").append(toIndentedString(debitMemoDate)).append("\n");
sb.append(" dueDate: ").append(toIndentedString(dueDate)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" invoiceGroupNumber: ").append(toIndentedString(invoiceGroupNumber)).append("\n");
sb.append(" latestPDFFileId: ").append(toIndentedString(latestPDFFileId)).append("\n");
sb.append(" number: ").append(toIndentedString(number)).append("\n");
sb.append(" paymentTerm: ").append(toIndentedString(paymentTerm)).append("\n");
sb.append(" postedById: ").append(toIndentedString(postedById)).append("\n");
sb.append(" postedOn: ").append(toIndentedString(postedOn)).append("\n");
sb.append(" reasonCode: ").append(toIndentedString(reasonCode)).append("\n");
sb.append(" referredCreditMemoId: ").append(toIndentedString(referredCreditMemoId)).append("\n");
sb.append(" referredInvoiceId: ").append(toIndentedString(referredInvoiceId)).append("\n");
sb.append(" sequenceSetId: ").append(toIndentedString(sequenceSetId)).append("\n");
sb.append(" soldToContactId: ").append(toIndentedString(soldToContactId)).append("\n");
sb.append(" soldToContactSnapshotId: ").append(toIndentedString(soldToContactSnapshotId)).append("\n");
sb.append(" sourceType: ").append(toIndentedString(sourceType)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" taxAmount: ").append(toIndentedString(taxAmount)).append("\n");
sb.append(" taxMessage: ").append(toIndentedString(taxMessage)).append("\n");
sb.append(" taxStatus: ").append(toIndentedString(taxStatus)).append("\n");
sb.append(" totalTaxExemptAmount: ").append(toIndentedString(totalTaxExemptAmount)).append("\n");
sb.append(" transferredToAccounting: ").append(toIndentedString(transferredToAccounting)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" eInvoiceStatus: ").append(toIndentedString(eInvoiceStatus)).append("\n");
sb.append(" eInvoiceErrorCode: ").append(toIndentedString(eInvoiceErrorCode)).append("\n");
sb.append(" eInvoiceErrorMessage: ").append(toIndentedString(eInvoiceErrorMessage)).append("\n");
sb.append(" eInvoiceFileId: ").append(toIndentedString(eInvoiceFileId)).append("\n");
sb.append(" billToContactSnapshotId: ").append(toIndentedString(billToContactSnapshotId)).append("\n");
sb.append(" organizationLabel: ").append(toIndentedString(organizationLabel)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" integrationIdNS: ").append(toIndentedString(integrationIdNS)).append("\n");
sb.append(" integrationStatusNS: ").append(toIndentedString(integrationStatusNS)).append("\n");
sb.append(" syncDateNS: ").append(toIndentedString(syncDateNS)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("processId");
openapiFields.add("requestId");
openapiFields.add("reasons");
openapiFields.add("success");
openapiFields.add("accountId");
openapiFields.add("accountNumber");
openapiFields.add("amount");
openapiFields.add("autoPay");
openapiFields.add("balance");
openapiFields.add("beAppliedAmount");
openapiFields.add("billToContactId");
openapiFields.add("cancelledById");
openapiFields.add("cancelledOn");
openapiFields.add("comment");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("debitMemoDate");
openapiFields.add("dueDate");
openapiFields.add("id");
openapiFields.add("invoiceGroupNumber");
openapiFields.add("latestPDFFileId");
openapiFields.add("number");
openapiFields.add("paymentTerm");
openapiFields.add("postedById");
openapiFields.add("postedOn");
openapiFields.add("reasonCode");
openapiFields.add("referredCreditMemoId");
openapiFields.add("referredInvoiceId");
openapiFields.add("sequenceSetId");
openapiFields.add("soldToContactId");
openapiFields.add("soldToContactSnapshotId");
openapiFields.add("sourceType");
openapiFields.add("status");
openapiFields.add("targetDate");
openapiFields.add("taxAmount");
openapiFields.add("taxMessage");
openapiFields.add("taxStatus");
openapiFields.add("totalTaxExemptAmount");
openapiFields.add("transferredToAccounting");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
openapiFields.add("eInvoiceStatus");
openapiFields.add("eInvoiceErrorCode");
openapiFields.add("eInvoiceErrorMessage");
openapiFields.add("eInvoiceFileId");
openapiFields.add("billToContactSnapshotId");
openapiFields.add("organizationLabel");
openapiFields.add("currency");
openapiFields.add("IntegrationId__NS");
openapiFields.add("IntegrationStatus__NS");
openapiFields.add("SyncDate__NS");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to DebitMemoResponse
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!DebitMemoResponse.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in DebitMemoResponse is not found in the empty JSON string", DebitMemoResponse.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("processId") != null && !jsonObj.get("processId").isJsonNull()) && !jsonObj.get("processId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `processId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("processId").toString()));
}
if ((jsonObj.get("requestId") != null && !jsonObj.get("requestId").isJsonNull()) && !jsonObj.get("requestId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `requestId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("requestId").toString()));
}
if (jsonObj.get("reasons") != null && !jsonObj.get("reasons").isJsonNull()) {
JsonArray jsonArrayreasons = jsonObj.getAsJsonArray("reasons");
if (jsonArrayreasons != null) {
// ensure the json data is an array
if (!jsonObj.get("reasons").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `reasons` to be an array in the JSON string but got `%s`", jsonObj.get("reasons").toString()));
}
// validate the optional field `reasons` (array)
for (int i = 0; i < jsonArrayreasons.size(); i++) {
FailedReason.validateJsonElement(jsonArrayreasons.get(i));
};
}
}
if ((jsonObj.get("accountId") != null && !jsonObj.get("accountId").isJsonNull()) && !jsonObj.get("accountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountId").toString()));
}
if ((jsonObj.get("accountNumber") != null && !jsonObj.get("accountNumber").isJsonNull()) && !jsonObj.get("accountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountNumber").toString()));
}
if ((jsonObj.get("billToContactId") != null && !jsonObj.get("billToContactId").isJsonNull()) && !jsonObj.get("billToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billToContactId").toString()));
}
if ((jsonObj.get("cancelledById") != null && !jsonObj.get("cancelledById").isJsonNull()) && !jsonObj.get("cancelledById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cancelledById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cancelledById").toString()));
}
if ((jsonObj.get("cancelledOn") != null && !jsonObj.get("cancelledOn").isJsonNull()) && !jsonObj.get("cancelledOn").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cancelledOn` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cancelledOn").toString()));
}
if ((jsonObj.get("comment") != null && !jsonObj.get("comment").isJsonNull()) && !jsonObj.get("comment").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `comment` to be a primitive type in the JSON string but got `%s`", jsonObj.get("comment").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("invoiceGroupNumber") != null && !jsonObj.get("invoiceGroupNumber").isJsonNull()) && !jsonObj.get("invoiceGroupNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceGroupNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceGroupNumber").toString()));
}
if ((jsonObj.get("latestPDFFileId") != null && !jsonObj.get("latestPDFFileId").isJsonNull()) && !jsonObj.get("latestPDFFileId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `latestPDFFileId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("latestPDFFileId").toString()));
}
if ((jsonObj.get("number") != null && !jsonObj.get("number").isJsonNull()) && !jsonObj.get("number").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `number` to be a primitive type in the JSON string but got `%s`", jsonObj.get("number").toString()));
}
if ((jsonObj.get("paymentTerm") != null && !jsonObj.get("paymentTerm").isJsonNull()) && !jsonObj.get("paymentTerm").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentTerm` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentTerm").toString()));
}
if ((jsonObj.get("postedById") != null && !jsonObj.get("postedById").isJsonNull()) && !jsonObj.get("postedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `postedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("postedById").toString()));
}
if ((jsonObj.get("postedOn") != null && !jsonObj.get("postedOn").isJsonNull()) && !jsonObj.get("postedOn").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `postedOn` to be a primitive type in the JSON string but got `%s`", jsonObj.get("postedOn").toString()));
}
if ((jsonObj.get("reasonCode") != null && !jsonObj.get("reasonCode").isJsonNull()) && !jsonObj.get("reasonCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `reasonCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("reasonCode").toString()));
}
if ((jsonObj.get("referredCreditMemoId") != null && !jsonObj.get("referredCreditMemoId").isJsonNull()) && !jsonObj.get("referredCreditMemoId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `referredCreditMemoId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("referredCreditMemoId").toString()));
}
if ((jsonObj.get("referredInvoiceId") != null && !jsonObj.get("referredInvoiceId").isJsonNull()) && !jsonObj.get("referredInvoiceId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `referredInvoiceId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("referredInvoiceId").toString()));
}
if ((jsonObj.get("sequenceSetId") != null && !jsonObj.get("sequenceSetId").isJsonNull()) && !jsonObj.get("sequenceSetId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sequenceSetId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sequenceSetId").toString()));
}
if ((jsonObj.get("soldToContactId") != null && !jsonObj.get("soldToContactId").isJsonNull()) && !jsonObj.get("soldToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToContactId").toString()));
}
if ((jsonObj.get("soldToContactSnapshotId") != null && !jsonObj.get("soldToContactSnapshotId").isJsonNull()) && !jsonObj.get("soldToContactSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToContactSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToContactSnapshotId").toString()));
}
if ((jsonObj.get("sourceType") != null && !jsonObj.get("sourceType").isJsonNull()) && !jsonObj.get("sourceType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sourceType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sourceType").toString()));
}
// validate the optional field `sourceType`
if (jsonObj.get("sourceType") != null && !jsonObj.get("sourceType").isJsonNull()) {
MemoSourceType.validateJsonElement(jsonObj.get("sourceType"));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
// validate the optional field `status`
if (jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) {
BillingDocumentStatus.validateJsonElement(jsonObj.get("status"));
}
if ((jsonObj.get("taxMessage") != null && !jsonObj.get("taxMessage").isJsonNull()) && !jsonObj.get("taxMessage").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxMessage` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxMessage").toString()));
}
if ((jsonObj.get("taxStatus") != null && !jsonObj.get("taxStatus").isJsonNull()) && !jsonObj.get("taxStatus").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxStatus` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxStatus").toString()));
}
// validate the optional field `taxStatus`
if (jsonObj.get("taxStatus") != null && !jsonObj.get("taxStatus").isJsonNull()) {
TaxStatus.validateJsonElement(jsonObj.get("taxStatus"));
}
if ((jsonObj.get("transferredToAccounting") != null && !jsonObj.get("transferredToAccounting").isJsonNull()) && !jsonObj.get("transferredToAccounting").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `transferredToAccounting` to be a primitive type in the JSON string but got `%s`", jsonObj.get("transferredToAccounting").toString()));
}
// validate the optional field `transferredToAccounting`
if (jsonObj.get("transferredToAccounting") != null && !jsonObj.get("transferredToAccounting").isJsonNull()) {
TransferredToAccountingStatus.validateJsonElement(jsonObj.get("transferredToAccounting"));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
if ((jsonObj.get("eInvoiceStatus") != null && !jsonObj.get("eInvoiceStatus").isJsonNull()) && !jsonObj.get("eInvoiceStatus").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `eInvoiceStatus` to be a primitive type in the JSON string but got `%s`", jsonObj.get("eInvoiceStatus").toString()));
}
// validate the optional field `eInvoiceStatus`
if (jsonObj.get("eInvoiceStatus") != null && !jsonObj.get("eInvoiceStatus").isJsonNull()) {
EInvoiceStatus.validateJsonElement(jsonObj.get("eInvoiceStatus"));
}
if ((jsonObj.get("eInvoiceErrorCode") != null && !jsonObj.get("eInvoiceErrorCode").isJsonNull()) && !jsonObj.get("eInvoiceErrorCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `eInvoiceErrorCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("eInvoiceErrorCode").toString()));
}
if ((jsonObj.get("eInvoiceErrorMessage") != null && !jsonObj.get("eInvoiceErrorMessage").isJsonNull()) && !jsonObj.get("eInvoiceErrorMessage").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `eInvoiceErrorMessage` to be a primitive type in the JSON string but got `%s`", jsonObj.get("eInvoiceErrorMessage").toString()));
}
if ((jsonObj.get("eInvoiceFileId") != null && !jsonObj.get("eInvoiceFileId").isJsonNull()) && !jsonObj.get("eInvoiceFileId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `eInvoiceFileId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("eInvoiceFileId").toString()));
}
if ((jsonObj.get("billToContactSnapshotId") != null && !jsonObj.get("billToContactSnapshotId").isJsonNull()) && !jsonObj.get("billToContactSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billToContactSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billToContactSnapshotId").toString()));
}
if ((jsonObj.get("organizationLabel") != null && !jsonObj.get("organizationLabel").isJsonNull()) && !jsonObj.get("organizationLabel").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `organizationLabel` to be a primitive type in the JSON string but got `%s`", jsonObj.get("organizationLabel").toString()));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("IntegrationId__NS") != null && !jsonObj.get("IntegrationId__NS").isJsonNull()) && !jsonObj.get("IntegrationId__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationId__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationId__NS").toString()));
}
if ((jsonObj.get("IntegrationStatus__NS") != null && !jsonObj.get("IntegrationStatus__NS").isJsonNull()) && !jsonObj.get("IntegrationStatus__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationStatus__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationStatus__NS").toString()));
}
if ((jsonObj.get("SyncDate__NS") != null && !jsonObj.get("SyncDate__NS").isJsonNull()) && !jsonObj.get("SyncDate__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SyncDate__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SyncDate__NS").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!DebitMemoResponse.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'DebitMemoResponse' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(DebitMemoResponse.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, DebitMemoResponse value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public DebitMemoResponse read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
DebitMemoResponse instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of DebitMemoResponse given an JSON string
*
* @param jsonString JSON string
* @return An instance of DebitMemoResponse
* @throws IOException if the JSON string is invalid with respect to DebitMemoResponse
*/
public static DebitMemoResponse fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, DebitMemoResponse.class);
}
/**
* Convert an instance of DebitMemoResponse to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy