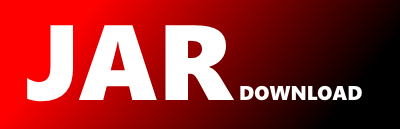
com.zuora.model.ExpandedAccount Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.ExpandedContact;
import com.zuora.model.ExpandedCreditMemo;
import com.zuora.model.ExpandedDebitMemo;
import com.zuora.model.ExpandedInvoice;
import com.zuora.model.ExpandedPayment;
import com.zuora.model.ExpandedPaymentMethod;
import com.zuora.model.ExpandedRefund;
import com.zuora.model.ExpandedSubscription;
import com.zuora.model.ExpandedUsage;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* ExpandedAccount
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class ExpandedAccount {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "accountNumber";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_ADDITIONAL_EMAIL_ADDRESSES = "additionalEmailAddresses";
@SerializedName(SERIALIZED_NAME_ADDITIONAL_EMAIL_ADDRESSES)
private String additionalEmailAddresses;
public static final String SERIALIZED_NAME_ALLOW_INVOICE_EDIT = "allowInvoiceEdit";
@SerializedName(SERIALIZED_NAME_ALLOW_INVOICE_EDIT)
private Boolean allowInvoiceEdit;
public static final String SERIALIZED_NAME_AUTO_PAY = "autoPay";
@SerializedName(SERIALIZED_NAME_AUTO_PAY)
private Boolean autoPay;
public static final String SERIALIZED_NAME_BALANCE = "balance";
@SerializedName(SERIALIZED_NAME_BALANCE)
private BigDecimal balance;
public static final String SERIALIZED_NAME_BATCH = "batch";
@SerializedName(SERIALIZED_NAME_BATCH)
private String batch;
public static final String SERIALIZED_NAME_BCD_SETTING_OPTION = "bcdSettingOption";
@SerializedName(SERIALIZED_NAME_BCD_SETTING_OPTION)
private String bcdSettingOption;
public static final String SERIALIZED_NAME_BILL_CYCLE_DAY = "billCycleDay";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_DAY)
private Long billCycleDay;
public static final String SERIALIZED_NAME_BILL_TO_ID = "billToId";
@SerializedName(SERIALIZED_NAME_BILL_TO_ID)
private String billToId;
public static final String SERIALIZED_NAME_COMMUNICATION_PROFILE_ID = "communicationProfileId";
@SerializedName(SERIALIZED_NAME_COMMUNICATION_PROFILE_ID)
private String communicationProfileId;
public static final String SERIALIZED_NAME_CREDIT_BALANCE = "creditBalance";
@SerializedName(SERIALIZED_NAME_CREDIT_BALANCE)
private BigDecimal creditBalance;
public static final String SERIALIZED_NAME_CRM_ID = "crmId";
@SerializedName(SERIALIZED_NAME_CRM_ID)
private String crmId;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_CUSTOMER_SERVICE_REP_NAME = "customerServiceRepName";
@SerializedName(SERIALIZED_NAME_CUSTOMER_SERVICE_REP_NAME)
private String customerServiceRepName;
public static final String SERIALIZED_NAME_DEFAULT_PAYMENT_METHOD_ID = "defaultPaymentMethodId";
@SerializedName(SERIALIZED_NAME_DEFAULT_PAYMENT_METHOD_ID)
private String defaultPaymentMethodId;
public static final String SERIALIZED_NAME_E_INVOICE_PROFILE_ID = "eInvoiceProfileId";
@SerializedName(SERIALIZED_NAME_E_INVOICE_PROFILE_ID)
private String eInvoiceProfileId;
public static final String SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_EMAIL = "invoiceDeliveryPrefsEmail";
@SerializedName(SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_EMAIL)
private Boolean invoiceDeliveryPrefsEmail;
public static final String SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_PRINT = "invoiceDeliveryPrefsPrint";
@SerializedName(SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_PRINT)
private Boolean invoiceDeliveryPrefsPrint;
public static final String SERIALIZED_NAME_INVOICE_TEMPLATE_ID = "invoiceTemplateId";
@SerializedName(SERIALIZED_NAME_INVOICE_TEMPLATE_ID)
private String invoiceTemplateId;
public static final String SERIALIZED_NAME_LAST_INVOICE_DATE = "lastInvoiceDate";
@SerializedName(SERIALIZED_NAME_LAST_INVOICE_DATE)
private LocalDate lastInvoiceDate;
public static final String SERIALIZED_NAME_LAST_METRICS_UPDATE = "lastMetricsUpdate";
@SerializedName(SERIALIZED_NAME_LAST_METRICS_UPDATE)
private String lastMetricsUpdate;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_NOTES = "notes";
@SerializedName(SERIALIZED_NAME_NOTES)
private String notes;
public static final String SERIALIZED_NAME_ORGANIZATION_ID = "organizationId";
@SerializedName(SERIALIZED_NAME_ORGANIZATION_ID)
private String organizationId;
public static final String SERIALIZED_NAME_PARENT_ID = "parentId";
@SerializedName(SERIALIZED_NAME_PARENT_ID)
private String parentId;
public static final String SERIALIZED_NAME_PARTNER_ACCOUNT = "partnerAccount";
@SerializedName(SERIALIZED_NAME_PARTNER_ACCOUNT)
private Boolean partnerAccount;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_CASCADING_CONSENT = "paymentMethodCascadingConsent";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_CASCADING_CONSENT)
private Boolean paymentMethodCascadingConsent;
public static final String SERIALIZED_NAME_PURCHASE_ORDER_NUMBER = "purchaseOrderNumber";
@SerializedName(SERIALIZED_NAME_PURCHASE_ORDER_NUMBER)
private String purchaseOrderNumber;
public static final String SERIALIZED_NAME_SALES_REP_NAME = "salesRepName";
@SerializedName(SERIALIZED_NAME_SALES_REP_NAME)
private String salesRepName;
public static final String SERIALIZED_NAME_SEQUENCE_SET_ID = "sequenceSetId";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_ID)
private String sequenceSetId;
public static final String SERIALIZED_NAME_SOLD_TO_ID = "soldToId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_ID)
private String soldToId;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private String status;
public static final String SERIALIZED_NAME_TAX_COMPANY_CODE = "taxCompanyCode";
@SerializedName(SERIALIZED_NAME_TAX_COMPANY_CODE)
private String taxCompanyCode;
public static final String SERIALIZED_NAME_TAX_EXEMPT_CERTIFICATE_I_D = "taxExemptCertificateID";
@SerializedName(SERIALIZED_NAME_TAX_EXEMPT_CERTIFICATE_I_D)
private String taxExemptCertificateID;
public static final String SERIALIZED_NAME_TAX_EXEMPT_CERTIFICATE_TYPE = "taxExemptCertificateType";
@SerializedName(SERIALIZED_NAME_TAX_EXEMPT_CERTIFICATE_TYPE)
private String taxExemptCertificateType;
public static final String SERIALIZED_NAME_TAX_EXEMPT_DESCRIPTION = "taxExemptDescription";
@SerializedName(SERIALIZED_NAME_TAX_EXEMPT_DESCRIPTION)
private String taxExemptDescription;
public static final String SERIALIZED_NAME_TAX_EXEMPT_EFFECTIVE_DATE = "taxExemptEffectiveDate";
@SerializedName(SERIALIZED_NAME_TAX_EXEMPT_EFFECTIVE_DATE)
private LocalDate taxExemptEffectiveDate;
public static final String SERIALIZED_NAME_TAX_EXEMPT_ENTITY_USE_CODE = "taxExemptEntityUseCode";
@SerializedName(SERIALIZED_NAME_TAX_EXEMPT_ENTITY_USE_CODE)
private String taxExemptEntityUseCode;
public static final String SERIALIZED_NAME_TAX_EXEMPT_EXPIRATION_DATE = "taxExemptExpirationDate";
@SerializedName(SERIALIZED_NAME_TAX_EXEMPT_EXPIRATION_DATE)
private LocalDate taxExemptExpirationDate;
public static final String SERIALIZED_NAME_TAX_EXEMPT_ISSUING_JURISDICTION = "taxExemptIssuingJurisdiction";
@SerializedName(SERIALIZED_NAME_TAX_EXEMPT_ISSUING_JURISDICTION)
private String taxExemptIssuingJurisdiction;
public static final String SERIALIZED_NAME_TAX_EXEMPT_STATUS = "taxExemptStatus";
@SerializedName(SERIALIZED_NAME_TAX_EXEMPT_STATUS)
private String taxExemptStatus;
public static final String SERIALIZED_NAME_TOTAL_INVOICE_BALANCE = "totalInvoiceBalance";
@SerializedName(SERIALIZED_NAME_TOTAL_INVOICE_BALANCE)
private BigDecimal totalInvoiceBalance;
public static final String SERIALIZED_NAME_UNAPPLIED_BALANCE = "unappliedBalance";
@SerializedName(SERIALIZED_NAME_UNAPPLIED_BALANCE)
private BigDecimal unappliedBalance;
public static final String SERIALIZED_NAME_V_A_T_ID = "vATId";
@SerializedName(SERIALIZED_NAME_V_A_T_ID)
private String vATId;
public static final String SERIALIZED_NAME_MRR = "mrr";
@SerializedName(SERIALIZED_NAME_MRR)
private BigDecimal mrr;
public static final String SERIALIZED_NAME_TOTAL_DEBIT_MEMO_BALANCE = "totalDebitMemoBalance";
@SerializedName(SERIALIZED_NAME_TOTAL_DEBIT_MEMO_BALANCE)
private BigDecimal totalDebitMemoBalance;
public static final String SERIALIZED_NAME_UNAPPLIED_CREDIT_MEMO_AMOUNT = "unappliedCreditMemoAmount";
@SerializedName(SERIALIZED_NAME_UNAPPLIED_CREDIT_MEMO_AMOUNT)
private BigDecimal unappliedCreditMemoAmount;
public static final String SERIALIZED_NAME_CREDIT_MEMO_TEMPLATE_ID = "creditMemoTemplateId";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_TEMPLATE_ID)
private String creditMemoTemplateId;
public static final String SERIALIZED_NAME_DEBIT_MEMO_TEMPLATE_ID = "debitMemoTemplateId";
@SerializedName(SERIALIZED_NAME_DEBIT_MEMO_TEMPLATE_ID)
private String debitMemoTemplateId;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY = "paymentGateway";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY)
private String paymentGateway;
public static final String SERIALIZED_NAME_PAYMENT_TERM = "paymentTerm";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERM)
private String paymentTerm;
public static final String SERIALIZED_NAME_BILL_TO = "billTo";
@SerializedName(SERIALIZED_NAME_BILL_TO)
private ExpandedContact billTo;
public static final String SERIALIZED_NAME_SOLD_TO = "soldTo";
@SerializedName(SERIALIZED_NAME_SOLD_TO)
private ExpandedContact soldTo;
public static final String SERIALIZED_NAME_DEFAULT_PAYMENT_METHOD = "defaultPaymentMethod";
@SerializedName(SERIALIZED_NAME_DEFAULT_PAYMENT_METHOD)
private ExpandedPaymentMethod defaultPaymentMethod;
public static final String SERIALIZED_NAME_SUBSCRIPTIONS = "subscriptions";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTIONS)
private List subscriptions;
public static final String SERIALIZED_NAME_PAYMENTS = "payments";
@SerializedName(SERIALIZED_NAME_PAYMENTS)
private List payments;
public static final String SERIALIZED_NAME_REFUNDS = "refunds";
@SerializedName(SERIALIZED_NAME_REFUNDS)
private List refunds;
public static final String SERIALIZED_NAME_CREDIT_MEMOS = "creditMemos";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMOS)
private List creditMemos;
public static final String SERIALIZED_NAME_DEBIT_MEMOS = "debitMemos";
@SerializedName(SERIALIZED_NAME_DEBIT_MEMOS)
private List debitMemos;
public static final String SERIALIZED_NAME_INVOICES = "invoices";
@SerializedName(SERIALIZED_NAME_INVOICES)
private List invoices;
public static final String SERIALIZED_NAME_USAGES = "usages";
@SerializedName(SERIALIZED_NAME_USAGES)
private List usages;
public static final String SERIALIZED_NAME_PAYMENT_METHODS = "paymentMethods";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHODS)
private List paymentMethods;
public ExpandedAccount() {
}
public ExpandedAccount id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public ExpandedAccount createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* Get createdById
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public ExpandedAccount createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* Get createdDate
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public ExpandedAccount updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* Get updatedById
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public ExpandedAccount updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* Get updatedDate
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
public ExpandedAccount accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Get accountNumber
* @return accountNumber
*/
@javax.annotation.Nullable
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public ExpandedAccount additionalEmailAddresses(String additionalEmailAddresses) {
this.additionalEmailAddresses = additionalEmailAddresses;
return this;
}
/**
* Get additionalEmailAddresses
* @return additionalEmailAddresses
*/
@javax.annotation.Nullable
public String getAdditionalEmailAddresses() {
return additionalEmailAddresses;
}
public void setAdditionalEmailAddresses(String additionalEmailAddresses) {
this.additionalEmailAddresses = additionalEmailAddresses;
}
public ExpandedAccount allowInvoiceEdit(Boolean allowInvoiceEdit) {
this.allowInvoiceEdit = allowInvoiceEdit;
return this;
}
/**
* Get allowInvoiceEdit
* @return allowInvoiceEdit
*/
@javax.annotation.Nullable
public Boolean getAllowInvoiceEdit() {
return allowInvoiceEdit;
}
public void setAllowInvoiceEdit(Boolean allowInvoiceEdit) {
this.allowInvoiceEdit = allowInvoiceEdit;
}
public ExpandedAccount autoPay(Boolean autoPay) {
this.autoPay = autoPay;
return this;
}
/**
* Get autoPay
* @return autoPay
*/
@javax.annotation.Nullable
public Boolean getAutoPay() {
return autoPay;
}
public void setAutoPay(Boolean autoPay) {
this.autoPay = autoPay;
}
public ExpandedAccount balance(BigDecimal balance) {
this.balance = balance;
return this;
}
/**
* Get balance
* @return balance
*/
@javax.annotation.Nullable
public BigDecimal getBalance() {
return balance;
}
public void setBalance(BigDecimal balance) {
this.balance = balance;
}
public ExpandedAccount batch(String batch) {
this.batch = batch;
return this;
}
/**
* Get batch
* @return batch
*/
@javax.annotation.Nullable
public String getBatch() {
return batch;
}
public void setBatch(String batch) {
this.batch = batch;
}
public ExpandedAccount bcdSettingOption(String bcdSettingOption) {
this.bcdSettingOption = bcdSettingOption;
return this;
}
/**
* Get bcdSettingOption
* @return bcdSettingOption
*/
@javax.annotation.Nullable
public String getBcdSettingOption() {
return bcdSettingOption;
}
public void setBcdSettingOption(String bcdSettingOption) {
this.bcdSettingOption = bcdSettingOption;
}
public ExpandedAccount billCycleDay(Long billCycleDay) {
this.billCycleDay = billCycleDay;
return this;
}
/**
* Get billCycleDay
* @return billCycleDay
*/
@javax.annotation.Nullable
public Long getBillCycleDay() {
return billCycleDay;
}
public void setBillCycleDay(Long billCycleDay) {
this.billCycleDay = billCycleDay;
}
public ExpandedAccount billToId(String billToId) {
this.billToId = billToId;
return this;
}
/**
* Get billToId
* @return billToId
*/
@javax.annotation.Nullable
public String getBillToId() {
return billToId;
}
public void setBillToId(String billToId) {
this.billToId = billToId;
}
public ExpandedAccount communicationProfileId(String communicationProfileId) {
this.communicationProfileId = communicationProfileId;
return this;
}
/**
* Get communicationProfileId
* @return communicationProfileId
*/
@javax.annotation.Nullable
public String getCommunicationProfileId() {
return communicationProfileId;
}
public void setCommunicationProfileId(String communicationProfileId) {
this.communicationProfileId = communicationProfileId;
}
public ExpandedAccount creditBalance(BigDecimal creditBalance) {
this.creditBalance = creditBalance;
return this;
}
/**
* Get creditBalance
* @return creditBalance
*/
@javax.annotation.Nullable
public BigDecimal getCreditBalance() {
return creditBalance;
}
public void setCreditBalance(BigDecimal creditBalance) {
this.creditBalance = creditBalance;
}
public ExpandedAccount crmId(String crmId) {
this.crmId = crmId;
return this;
}
/**
* Get crmId
* @return crmId
*/
@javax.annotation.Nullable
public String getCrmId() {
return crmId;
}
public void setCrmId(String crmId) {
this.crmId = crmId;
}
public ExpandedAccount currency(String currency) {
this.currency = currency;
return this;
}
/**
* Get currency
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public ExpandedAccount customerServiceRepName(String customerServiceRepName) {
this.customerServiceRepName = customerServiceRepName;
return this;
}
/**
* Get customerServiceRepName
* @return customerServiceRepName
*/
@javax.annotation.Nullable
public String getCustomerServiceRepName() {
return customerServiceRepName;
}
public void setCustomerServiceRepName(String customerServiceRepName) {
this.customerServiceRepName = customerServiceRepName;
}
public ExpandedAccount defaultPaymentMethodId(String defaultPaymentMethodId) {
this.defaultPaymentMethodId = defaultPaymentMethodId;
return this;
}
/**
* Get defaultPaymentMethodId
* @return defaultPaymentMethodId
*/
@javax.annotation.Nullable
public String getDefaultPaymentMethodId() {
return defaultPaymentMethodId;
}
public void setDefaultPaymentMethodId(String defaultPaymentMethodId) {
this.defaultPaymentMethodId = defaultPaymentMethodId;
}
public ExpandedAccount eInvoiceProfileId(String eInvoiceProfileId) {
this.eInvoiceProfileId = eInvoiceProfileId;
return this;
}
/**
* Get eInvoiceProfileId
* @return eInvoiceProfileId
*/
@javax.annotation.Nullable
public String geteInvoiceProfileId() {
return eInvoiceProfileId;
}
public void seteInvoiceProfileId(String eInvoiceProfileId) {
this.eInvoiceProfileId = eInvoiceProfileId;
}
public ExpandedAccount invoiceDeliveryPrefsEmail(Boolean invoiceDeliveryPrefsEmail) {
this.invoiceDeliveryPrefsEmail = invoiceDeliveryPrefsEmail;
return this;
}
/**
* Get invoiceDeliveryPrefsEmail
* @return invoiceDeliveryPrefsEmail
*/
@javax.annotation.Nullable
public Boolean getInvoiceDeliveryPrefsEmail() {
return invoiceDeliveryPrefsEmail;
}
public void setInvoiceDeliveryPrefsEmail(Boolean invoiceDeliveryPrefsEmail) {
this.invoiceDeliveryPrefsEmail = invoiceDeliveryPrefsEmail;
}
public ExpandedAccount invoiceDeliveryPrefsPrint(Boolean invoiceDeliveryPrefsPrint) {
this.invoiceDeliveryPrefsPrint = invoiceDeliveryPrefsPrint;
return this;
}
/**
* Get invoiceDeliveryPrefsPrint
* @return invoiceDeliveryPrefsPrint
*/
@javax.annotation.Nullable
public Boolean getInvoiceDeliveryPrefsPrint() {
return invoiceDeliveryPrefsPrint;
}
public void setInvoiceDeliveryPrefsPrint(Boolean invoiceDeliveryPrefsPrint) {
this.invoiceDeliveryPrefsPrint = invoiceDeliveryPrefsPrint;
}
public ExpandedAccount invoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
return this;
}
/**
* Get invoiceTemplateId
* @return invoiceTemplateId
*/
@javax.annotation.Nullable
public String getInvoiceTemplateId() {
return invoiceTemplateId;
}
public void setInvoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
}
public ExpandedAccount lastInvoiceDate(LocalDate lastInvoiceDate) {
this.lastInvoiceDate = lastInvoiceDate;
return this;
}
/**
* Get lastInvoiceDate
* @return lastInvoiceDate
*/
@javax.annotation.Nullable
public LocalDate getLastInvoiceDate() {
return lastInvoiceDate;
}
public void setLastInvoiceDate(LocalDate lastInvoiceDate) {
this.lastInvoiceDate = lastInvoiceDate;
}
public ExpandedAccount lastMetricsUpdate(String lastMetricsUpdate) {
this.lastMetricsUpdate = lastMetricsUpdate;
return this;
}
/**
* Get lastMetricsUpdate
* @return lastMetricsUpdate
*/
@javax.annotation.Nullable
public String getLastMetricsUpdate() {
return lastMetricsUpdate;
}
public void setLastMetricsUpdate(String lastMetricsUpdate) {
this.lastMetricsUpdate = lastMetricsUpdate;
}
public ExpandedAccount name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
*/
@javax.annotation.Nullable
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public ExpandedAccount notes(String notes) {
this.notes = notes;
return this;
}
/**
* Get notes
* @return notes
*/
@javax.annotation.Nullable
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
this.notes = notes;
}
public ExpandedAccount organizationId(String organizationId) {
this.organizationId = organizationId;
return this;
}
/**
* Get organizationId
* @return organizationId
*/
@javax.annotation.Nullable
public String getOrganizationId() {
return organizationId;
}
public void setOrganizationId(String organizationId) {
this.organizationId = organizationId;
}
public ExpandedAccount parentId(String parentId) {
this.parentId = parentId;
return this;
}
/**
* Get parentId
* @return parentId
*/
@javax.annotation.Nullable
public String getParentId() {
return parentId;
}
public void setParentId(String parentId) {
this.parentId = parentId;
}
public ExpandedAccount partnerAccount(Boolean partnerAccount) {
this.partnerAccount = partnerAccount;
return this;
}
/**
* Get partnerAccount
* @return partnerAccount
*/
@javax.annotation.Nullable
public Boolean getPartnerAccount() {
return partnerAccount;
}
public void setPartnerAccount(Boolean partnerAccount) {
this.partnerAccount = partnerAccount;
}
public ExpandedAccount paymentMethodCascadingConsent(Boolean paymentMethodCascadingConsent) {
this.paymentMethodCascadingConsent = paymentMethodCascadingConsent;
return this;
}
/**
* Get paymentMethodCascadingConsent
* @return paymentMethodCascadingConsent
*/
@javax.annotation.Nullable
public Boolean getPaymentMethodCascadingConsent() {
return paymentMethodCascadingConsent;
}
public void setPaymentMethodCascadingConsent(Boolean paymentMethodCascadingConsent) {
this.paymentMethodCascadingConsent = paymentMethodCascadingConsent;
}
public ExpandedAccount purchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
return this;
}
/**
* Get purchaseOrderNumber
* @return purchaseOrderNumber
*/
@javax.annotation.Nullable
public String getPurchaseOrderNumber() {
return purchaseOrderNumber;
}
public void setPurchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
}
public ExpandedAccount salesRepName(String salesRepName) {
this.salesRepName = salesRepName;
return this;
}
/**
* Get salesRepName
* @return salesRepName
*/
@javax.annotation.Nullable
public String getSalesRepName() {
return salesRepName;
}
public void setSalesRepName(String salesRepName) {
this.salesRepName = salesRepName;
}
public ExpandedAccount sequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
return this;
}
/**
* Get sequenceSetId
* @return sequenceSetId
*/
@javax.annotation.Nullable
public String getSequenceSetId() {
return sequenceSetId;
}
public void setSequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
}
public ExpandedAccount soldToId(String soldToId) {
this.soldToId = soldToId;
return this;
}
/**
* Get soldToId
* @return soldToId
*/
@javax.annotation.Nullable
public String getSoldToId() {
return soldToId;
}
public void setSoldToId(String soldToId) {
this.soldToId = soldToId;
}
public ExpandedAccount status(String status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public ExpandedAccount taxCompanyCode(String taxCompanyCode) {
this.taxCompanyCode = taxCompanyCode;
return this;
}
/**
* Get taxCompanyCode
* @return taxCompanyCode
*/
@javax.annotation.Nullable
public String getTaxCompanyCode() {
return taxCompanyCode;
}
public void setTaxCompanyCode(String taxCompanyCode) {
this.taxCompanyCode = taxCompanyCode;
}
public ExpandedAccount taxExemptCertificateID(String taxExemptCertificateID) {
this.taxExemptCertificateID = taxExemptCertificateID;
return this;
}
/**
* Get taxExemptCertificateID
* @return taxExemptCertificateID
*/
@javax.annotation.Nullable
public String getTaxExemptCertificateID() {
return taxExemptCertificateID;
}
public void setTaxExemptCertificateID(String taxExemptCertificateID) {
this.taxExemptCertificateID = taxExemptCertificateID;
}
public ExpandedAccount taxExemptCertificateType(String taxExemptCertificateType) {
this.taxExemptCertificateType = taxExemptCertificateType;
return this;
}
/**
* Get taxExemptCertificateType
* @return taxExemptCertificateType
*/
@javax.annotation.Nullable
public String getTaxExemptCertificateType() {
return taxExemptCertificateType;
}
public void setTaxExemptCertificateType(String taxExemptCertificateType) {
this.taxExemptCertificateType = taxExemptCertificateType;
}
public ExpandedAccount taxExemptDescription(String taxExemptDescription) {
this.taxExemptDescription = taxExemptDescription;
return this;
}
/**
* Get taxExemptDescription
* @return taxExemptDescription
*/
@javax.annotation.Nullable
public String getTaxExemptDescription() {
return taxExemptDescription;
}
public void setTaxExemptDescription(String taxExemptDescription) {
this.taxExemptDescription = taxExemptDescription;
}
public ExpandedAccount taxExemptEffectiveDate(LocalDate taxExemptEffectiveDate) {
this.taxExemptEffectiveDate = taxExemptEffectiveDate;
return this;
}
/**
* Get taxExemptEffectiveDate
* @return taxExemptEffectiveDate
*/
@javax.annotation.Nullable
public LocalDate getTaxExemptEffectiveDate() {
return taxExemptEffectiveDate;
}
public void setTaxExemptEffectiveDate(LocalDate taxExemptEffectiveDate) {
this.taxExemptEffectiveDate = taxExemptEffectiveDate;
}
public ExpandedAccount taxExemptEntityUseCode(String taxExemptEntityUseCode) {
this.taxExemptEntityUseCode = taxExemptEntityUseCode;
return this;
}
/**
* Get taxExemptEntityUseCode
* @return taxExemptEntityUseCode
*/
@javax.annotation.Nullable
public String getTaxExemptEntityUseCode() {
return taxExemptEntityUseCode;
}
public void setTaxExemptEntityUseCode(String taxExemptEntityUseCode) {
this.taxExemptEntityUseCode = taxExemptEntityUseCode;
}
public ExpandedAccount taxExemptExpirationDate(LocalDate taxExemptExpirationDate) {
this.taxExemptExpirationDate = taxExemptExpirationDate;
return this;
}
/**
* Get taxExemptExpirationDate
* @return taxExemptExpirationDate
*/
@javax.annotation.Nullable
public LocalDate getTaxExemptExpirationDate() {
return taxExemptExpirationDate;
}
public void setTaxExemptExpirationDate(LocalDate taxExemptExpirationDate) {
this.taxExemptExpirationDate = taxExemptExpirationDate;
}
public ExpandedAccount taxExemptIssuingJurisdiction(String taxExemptIssuingJurisdiction) {
this.taxExemptIssuingJurisdiction = taxExemptIssuingJurisdiction;
return this;
}
/**
* Get taxExemptIssuingJurisdiction
* @return taxExemptIssuingJurisdiction
*/
@javax.annotation.Nullable
public String getTaxExemptIssuingJurisdiction() {
return taxExemptIssuingJurisdiction;
}
public void setTaxExemptIssuingJurisdiction(String taxExemptIssuingJurisdiction) {
this.taxExemptIssuingJurisdiction = taxExemptIssuingJurisdiction;
}
public ExpandedAccount taxExemptStatus(String taxExemptStatus) {
this.taxExemptStatus = taxExemptStatus;
return this;
}
/**
* Get taxExemptStatus
* @return taxExemptStatus
*/
@javax.annotation.Nullable
public String getTaxExemptStatus() {
return taxExemptStatus;
}
public void setTaxExemptStatus(String taxExemptStatus) {
this.taxExemptStatus = taxExemptStatus;
}
public ExpandedAccount totalInvoiceBalance(BigDecimal totalInvoiceBalance) {
this.totalInvoiceBalance = totalInvoiceBalance;
return this;
}
/**
* Get totalInvoiceBalance
* @return totalInvoiceBalance
*/
@javax.annotation.Nullable
public BigDecimal getTotalInvoiceBalance() {
return totalInvoiceBalance;
}
public void setTotalInvoiceBalance(BigDecimal totalInvoiceBalance) {
this.totalInvoiceBalance = totalInvoiceBalance;
}
public ExpandedAccount unappliedBalance(BigDecimal unappliedBalance) {
this.unappliedBalance = unappliedBalance;
return this;
}
/**
* Get unappliedBalance
* @return unappliedBalance
*/
@javax.annotation.Nullable
public BigDecimal getUnappliedBalance() {
return unappliedBalance;
}
public void setUnappliedBalance(BigDecimal unappliedBalance) {
this.unappliedBalance = unappliedBalance;
}
public ExpandedAccount vATId(String vATId) {
this.vATId = vATId;
return this;
}
/**
* Get vATId
* @return vATId
*/
@javax.annotation.Nullable
public String getvATId() {
return vATId;
}
public void setvATId(String vATId) {
this.vATId = vATId;
}
public ExpandedAccount mrr(BigDecimal mrr) {
this.mrr = mrr;
return this;
}
/**
* Get mrr
* @return mrr
*/
@javax.annotation.Nullable
public BigDecimal getMrr() {
return mrr;
}
public void setMrr(BigDecimal mrr) {
this.mrr = mrr;
}
public ExpandedAccount totalDebitMemoBalance(BigDecimal totalDebitMemoBalance) {
this.totalDebitMemoBalance = totalDebitMemoBalance;
return this;
}
/**
* Get totalDebitMemoBalance
* @return totalDebitMemoBalance
*/
@javax.annotation.Nullable
public BigDecimal getTotalDebitMemoBalance() {
return totalDebitMemoBalance;
}
public void setTotalDebitMemoBalance(BigDecimal totalDebitMemoBalance) {
this.totalDebitMemoBalance = totalDebitMemoBalance;
}
public ExpandedAccount unappliedCreditMemoAmount(BigDecimal unappliedCreditMemoAmount) {
this.unappliedCreditMemoAmount = unappliedCreditMemoAmount;
return this;
}
/**
* Get unappliedCreditMemoAmount
* @return unappliedCreditMemoAmount
*/
@javax.annotation.Nullable
public BigDecimal getUnappliedCreditMemoAmount() {
return unappliedCreditMemoAmount;
}
public void setUnappliedCreditMemoAmount(BigDecimal unappliedCreditMemoAmount) {
this.unappliedCreditMemoAmount = unappliedCreditMemoAmount;
}
public ExpandedAccount creditMemoTemplateId(String creditMemoTemplateId) {
this.creditMemoTemplateId = creditMemoTemplateId;
return this;
}
/**
* Get creditMemoTemplateId
* @return creditMemoTemplateId
*/
@javax.annotation.Nullable
public String getCreditMemoTemplateId() {
return creditMemoTemplateId;
}
public void setCreditMemoTemplateId(String creditMemoTemplateId) {
this.creditMemoTemplateId = creditMemoTemplateId;
}
public ExpandedAccount debitMemoTemplateId(String debitMemoTemplateId) {
this.debitMemoTemplateId = debitMemoTemplateId;
return this;
}
/**
* Get debitMemoTemplateId
* @return debitMemoTemplateId
*/
@javax.annotation.Nullable
public String getDebitMemoTemplateId() {
return debitMemoTemplateId;
}
public void setDebitMemoTemplateId(String debitMemoTemplateId) {
this.debitMemoTemplateId = debitMemoTemplateId;
}
public ExpandedAccount paymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
return this;
}
/**
* Get paymentGateway
* @return paymentGateway
*/
@javax.annotation.Nullable
public String getPaymentGateway() {
return paymentGateway;
}
public void setPaymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
}
public ExpandedAccount paymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
return this;
}
/**
* Get paymentTerm
* @return paymentTerm
*/
@javax.annotation.Nullable
public String getPaymentTerm() {
return paymentTerm;
}
public void setPaymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
}
public ExpandedAccount billTo(ExpandedContact billTo) {
this.billTo = billTo;
return this;
}
/**
* Get billTo
* @return billTo
*/
@javax.annotation.Nullable
public ExpandedContact getBillTo() {
return billTo;
}
public void setBillTo(ExpandedContact billTo) {
this.billTo = billTo;
}
public ExpandedAccount soldTo(ExpandedContact soldTo) {
this.soldTo = soldTo;
return this;
}
/**
* Get soldTo
* @return soldTo
*/
@javax.annotation.Nullable
public ExpandedContact getSoldTo() {
return soldTo;
}
public void setSoldTo(ExpandedContact soldTo) {
this.soldTo = soldTo;
}
public ExpandedAccount defaultPaymentMethod(ExpandedPaymentMethod defaultPaymentMethod) {
this.defaultPaymentMethod = defaultPaymentMethod;
return this;
}
/**
* Get defaultPaymentMethod
* @return defaultPaymentMethod
*/
@javax.annotation.Nullable
public ExpandedPaymentMethod getDefaultPaymentMethod() {
return defaultPaymentMethod;
}
public void setDefaultPaymentMethod(ExpandedPaymentMethod defaultPaymentMethod) {
this.defaultPaymentMethod = defaultPaymentMethod;
}
public ExpandedAccount subscriptions(List subscriptions) {
this.subscriptions = subscriptions;
return this;
}
public ExpandedAccount addSubscriptionsItem(ExpandedSubscription subscriptionsItem) {
if (this.subscriptions == null) {
this.subscriptions = new ArrayList<>();
}
this.subscriptions.add(subscriptionsItem);
return this;
}
/**
* Get subscriptions
* @return subscriptions
*/
@javax.annotation.Nullable
public List getSubscriptions() {
return subscriptions;
}
public void setSubscriptions(List subscriptions) {
this.subscriptions = subscriptions;
}
public ExpandedAccount payments(List payments) {
this.payments = payments;
return this;
}
public ExpandedAccount addPaymentsItem(ExpandedPayment paymentsItem) {
if (this.payments == null) {
this.payments = new ArrayList<>();
}
this.payments.add(paymentsItem);
return this;
}
/**
* Get payments
* @return payments
*/
@javax.annotation.Nullable
public List getPayments() {
return payments;
}
public void setPayments(List payments) {
this.payments = payments;
}
public ExpandedAccount refunds(List refunds) {
this.refunds = refunds;
return this;
}
public ExpandedAccount addRefundsItem(ExpandedRefund refundsItem) {
if (this.refunds == null) {
this.refunds = new ArrayList<>();
}
this.refunds.add(refundsItem);
return this;
}
/**
* Get refunds
* @return refunds
*/
@javax.annotation.Nullable
public List getRefunds() {
return refunds;
}
public void setRefunds(List refunds) {
this.refunds = refunds;
}
public ExpandedAccount creditMemos(List creditMemos) {
this.creditMemos = creditMemos;
return this;
}
public ExpandedAccount addCreditMemosItem(ExpandedCreditMemo creditMemosItem) {
if (this.creditMemos == null) {
this.creditMemos = new ArrayList<>();
}
this.creditMemos.add(creditMemosItem);
return this;
}
/**
* Get creditMemos
* @return creditMemos
*/
@javax.annotation.Nullable
public List getCreditMemos() {
return creditMemos;
}
public void setCreditMemos(List creditMemos) {
this.creditMemos = creditMemos;
}
public ExpandedAccount debitMemos(List debitMemos) {
this.debitMemos = debitMemos;
return this;
}
public ExpandedAccount addDebitMemosItem(ExpandedDebitMemo debitMemosItem) {
if (this.debitMemos == null) {
this.debitMemos = new ArrayList<>();
}
this.debitMemos.add(debitMemosItem);
return this;
}
/**
* Get debitMemos
* @return debitMemos
*/
@javax.annotation.Nullable
public List getDebitMemos() {
return debitMemos;
}
public void setDebitMemos(List debitMemos) {
this.debitMemos = debitMemos;
}
public ExpandedAccount invoices(List invoices) {
this.invoices = invoices;
return this;
}
public ExpandedAccount addInvoicesItem(ExpandedInvoice invoicesItem) {
if (this.invoices == null) {
this.invoices = new ArrayList<>();
}
this.invoices.add(invoicesItem);
return this;
}
/**
* Get invoices
* @return invoices
*/
@javax.annotation.Nullable
public List getInvoices() {
return invoices;
}
public void setInvoices(List invoices) {
this.invoices = invoices;
}
public ExpandedAccount usages(List usages) {
this.usages = usages;
return this;
}
public ExpandedAccount addUsagesItem(ExpandedUsage usagesItem) {
if (this.usages == null) {
this.usages = new ArrayList<>();
}
this.usages.add(usagesItem);
return this;
}
/**
* Get usages
* @return usages
*/
@javax.annotation.Nullable
public List getUsages() {
return usages;
}
public void setUsages(List usages) {
this.usages = usages;
}
public ExpandedAccount paymentMethods(List paymentMethods) {
this.paymentMethods = paymentMethods;
return this;
}
public ExpandedAccount addPaymentMethodsItem(ExpandedPaymentMethod paymentMethodsItem) {
if (this.paymentMethods == null) {
this.paymentMethods = new ArrayList<>();
}
this.paymentMethods.add(paymentMethodsItem);
return this;
}
/**
* Get paymentMethods
* @return paymentMethods
*/
@javax.annotation.Nullable
public List getPaymentMethods() {
return paymentMethods;
}
public void setPaymentMethods(List paymentMethods) {
this.paymentMethods = paymentMethods;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the ExpandedAccount instance itself
*/
public ExpandedAccount putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ExpandedAccount expandedAccount = (ExpandedAccount) o;
return Objects.equals(this.id, expandedAccount.id) &&
Objects.equals(this.createdById, expandedAccount.createdById) &&
Objects.equals(this.createdDate, expandedAccount.createdDate) &&
Objects.equals(this.updatedById, expandedAccount.updatedById) &&
Objects.equals(this.updatedDate, expandedAccount.updatedDate) &&
Objects.equals(this.accountNumber, expandedAccount.accountNumber) &&
Objects.equals(this.additionalEmailAddresses, expandedAccount.additionalEmailAddresses) &&
Objects.equals(this.allowInvoiceEdit, expandedAccount.allowInvoiceEdit) &&
Objects.equals(this.autoPay, expandedAccount.autoPay) &&
Objects.equals(this.balance, expandedAccount.balance) &&
Objects.equals(this.batch, expandedAccount.batch) &&
Objects.equals(this.bcdSettingOption, expandedAccount.bcdSettingOption) &&
Objects.equals(this.billCycleDay, expandedAccount.billCycleDay) &&
Objects.equals(this.billToId, expandedAccount.billToId) &&
Objects.equals(this.communicationProfileId, expandedAccount.communicationProfileId) &&
Objects.equals(this.creditBalance, expandedAccount.creditBalance) &&
Objects.equals(this.crmId, expandedAccount.crmId) &&
Objects.equals(this.currency, expandedAccount.currency) &&
Objects.equals(this.customerServiceRepName, expandedAccount.customerServiceRepName) &&
Objects.equals(this.defaultPaymentMethodId, expandedAccount.defaultPaymentMethodId) &&
Objects.equals(this.eInvoiceProfileId, expandedAccount.eInvoiceProfileId) &&
Objects.equals(this.invoiceDeliveryPrefsEmail, expandedAccount.invoiceDeliveryPrefsEmail) &&
Objects.equals(this.invoiceDeliveryPrefsPrint, expandedAccount.invoiceDeliveryPrefsPrint) &&
Objects.equals(this.invoiceTemplateId, expandedAccount.invoiceTemplateId) &&
Objects.equals(this.lastInvoiceDate, expandedAccount.lastInvoiceDate) &&
Objects.equals(this.lastMetricsUpdate, expandedAccount.lastMetricsUpdate) &&
Objects.equals(this.name, expandedAccount.name) &&
Objects.equals(this.notes, expandedAccount.notes) &&
Objects.equals(this.organizationId, expandedAccount.organizationId) &&
Objects.equals(this.parentId, expandedAccount.parentId) &&
Objects.equals(this.partnerAccount, expandedAccount.partnerAccount) &&
Objects.equals(this.paymentMethodCascadingConsent, expandedAccount.paymentMethodCascadingConsent) &&
Objects.equals(this.purchaseOrderNumber, expandedAccount.purchaseOrderNumber) &&
Objects.equals(this.salesRepName, expandedAccount.salesRepName) &&
Objects.equals(this.sequenceSetId, expandedAccount.sequenceSetId) &&
Objects.equals(this.soldToId, expandedAccount.soldToId) &&
Objects.equals(this.status, expandedAccount.status) &&
Objects.equals(this.taxCompanyCode, expandedAccount.taxCompanyCode) &&
Objects.equals(this.taxExemptCertificateID, expandedAccount.taxExemptCertificateID) &&
Objects.equals(this.taxExemptCertificateType, expandedAccount.taxExemptCertificateType) &&
Objects.equals(this.taxExemptDescription, expandedAccount.taxExemptDescription) &&
Objects.equals(this.taxExemptEffectiveDate, expandedAccount.taxExemptEffectiveDate) &&
Objects.equals(this.taxExemptEntityUseCode, expandedAccount.taxExemptEntityUseCode) &&
Objects.equals(this.taxExemptExpirationDate, expandedAccount.taxExemptExpirationDate) &&
Objects.equals(this.taxExemptIssuingJurisdiction, expandedAccount.taxExemptIssuingJurisdiction) &&
Objects.equals(this.taxExemptStatus, expandedAccount.taxExemptStatus) &&
Objects.equals(this.totalInvoiceBalance, expandedAccount.totalInvoiceBalance) &&
Objects.equals(this.unappliedBalance, expandedAccount.unappliedBalance) &&
Objects.equals(this.vATId, expandedAccount.vATId) &&
Objects.equals(this.mrr, expandedAccount.mrr) &&
Objects.equals(this.totalDebitMemoBalance, expandedAccount.totalDebitMemoBalance) &&
Objects.equals(this.unappliedCreditMemoAmount, expandedAccount.unappliedCreditMemoAmount) &&
Objects.equals(this.creditMemoTemplateId, expandedAccount.creditMemoTemplateId) &&
Objects.equals(this.debitMemoTemplateId, expandedAccount.debitMemoTemplateId) &&
Objects.equals(this.paymentGateway, expandedAccount.paymentGateway) &&
Objects.equals(this.paymentTerm, expandedAccount.paymentTerm) &&
Objects.equals(this.billTo, expandedAccount.billTo) &&
Objects.equals(this.soldTo, expandedAccount.soldTo) &&
Objects.equals(this.defaultPaymentMethod, expandedAccount.defaultPaymentMethod) &&
Objects.equals(this.subscriptions, expandedAccount.subscriptions) &&
Objects.equals(this.payments, expandedAccount.payments) &&
Objects.equals(this.refunds, expandedAccount.refunds) &&
Objects.equals(this.creditMemos, expandedAccount.creditMemos) &&
Objects.equals(this.debitMemos, expandedAccount.debitMemos) &&
Objects.equals(this.invoices, expandedAccount.invoices) &&
Objects.equals(this.usages, expandedAccount.usages) &&
Objects.equals(this.paymentMethods, expandedAccount.paymentMethods)&&
Objects.equals(this.additionalProperties, expandedAccount.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(id, createdById, createdDate, updatedById, updatedDate, accountNumber, additionalEmailAddresses, allowInvoiceEdit, autoPay, balance, batch, bcdSettingOption, billCycleDay, billToId, communicationProfileId, creditBalance, crmId, currency, customerServiceRepName, defaultPaymentMethodId, eInvoiceProfileId, invoiceDeliveryPrefsEmail, invoiceDeliveryPrefsPrint, invoiceTemplateId, lastInvoiceDate, lastMetricsUpdate, name, notes, organizationId, parentId, partnerAccount, paymentMethodCascadingConsent, purchaseOrderNumber, salesRepName, sequenceSetId, soldToId, status, taxCompanyCode, taxExemptCertificateID, taxExemptCertificateType, taxExemptDescription, taxExemptEffectiveDate, taxExemptEntityUseCode, taxExemptExpirationDate, taxExemptIssuingJurisdiction, taxExemptStatus, totalInvoiceBalance, unappliedBalance, vATId, mrr, totalDebitMemoBalance, unappliedCreditMemoAmount, creditMemoTemplateId, debitMemoTemplateId, paymentGateway, paymentTerm, billTo, soldTo, defaultPaymentMethod, subscriptions, payments, refunds, creditMemos, debitMemos, invoices, usages, paymentMethods, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ExpandedAccount {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" additionalEmailAddresses: ").append(toIndentedString(additionalEmailAddresses)).append("\n");
sb.append(" allowInvoiceEdit: ").append(toIndentedString(allowInvoiceEdit)).append("\n");
sb.append(" autoPay: ").append(toIndentedString(autoPay)).append("\n");
sb.append(" balance: ").append(toIndentedString(balance)).append("\n");
sb.append(" batch: ").append(toIndentedString(batch)).append("\n");
sb.append(" bcdSettingOption: ").append(toIndentedString(bcdSettingOption)).append("\n");
sb.append(" billCycleDay: ").append(toIndentedString(billCycleDay)).append("\n");
sb.append(" billToId: ").append(toIndentedString(billToId)).append("\n");
sb.append(" communicationProfileId: ").append(toIndentedString(communicationProfileId)).append("\n");
sb.append(" creditBalance: ").append(toIndentedString(creditBalance)).append("\n");
sb.append(" crmId: ").append(toIndentedString(crmId)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" customerServiceRepName: ").append(toIndentedString(customerServiceRepName)).append("\n");
sb.append(" defaultPaymentMethodId: ").append(toIndentedString(defaultPaymentMethodId)).append("\n");
sb.append(" eInvoiceProfileId: ").append(toIndentedString(eInvoiceProfileId)).append("\n");
sb.append(" invoiceDeliveryPrefsEmail: ").append(toIndentedString(invoiceDeliveryPrefsEmail)).append("\n");
sb.append(" invoiceDeliveryPrefsPrint: ").append(toIndentedString(invoiceDeliveryPrefsPrint)).append("\n");
sb.append(" invoiceTemplateId: ").append(toIndentedString(invoiceTemplateId)).append("\n");
sb.append(" lastInvoiceDate: ").append(toIndentedString(lastInvoiceDate)).append("\n");
sb.append(" lastMetricsUpdate: ").append(toIndentedString(lastMetricsUpdate)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" notes: ").append(toIndentedString(notes)).append("\n");
sb.append(" organizationId: ").append(toIndentedString(organizationId)).append("\n");
sb.append(" parentId: ").append(toIndentedString(parentId)).append("\n");
sb.append(" partnerAccount: ").append(toIndentedString(partnerAccount)).append("\n");
sb.append(" paymentMethodCascadingConsent: ").append(toIndentedString(paymentMethodCascadingConsent)).append("\n");
sb.append(" purchaseOrderNumber: ").append(toIndentedString(purchaseOrderNumber)).append("\n");
sb.append(" salesRepName: ").append(toIndentedString(salesRepName)).append("\n");
sb.append(" sequenceSetId: ").append(toIndentedString(sequenceSetId)).append("\n");
sb.append(" soldToId: ").append(toIndentedString(soldToId)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" taxCompanyCode: ").append(toIndentedString(taxCompanyCode)).append("\n");
sb.append(" taxExemptCertificateID: ").append(toIndentedString(taxExemptCertificateID)).append("\n");
sb.append(" taxExemptCertificateType: ").append(toIndentedString(taxExemptCertificateType)).append("\n");
sb.append(" taxExemptDescription: ").append(toIndentedString(taxExemptDescription)).append("\n");
sb.append(" taxExemptEffectiveDate: ").append(toIndentedString(taxExemptEffectiveDate)).append("\n");
sb.append(" taxExemptEntityUseCode: ").append(toIndentedString(taxExemptEntityUseCode)).append("\n");
sb.append(" taxExemptExpirationDate: ").append(toIndentedString(taxExemptExpirationDate)).append("\n");
sb.append(" taxExemptIssuingJurisdiction: ").append(toIndentedString(taxExemptIssuingJurisdiction)).append("\n");
sb.append(" taxExemptStatus: ").append(toIndentedString(taxExemptStatus)).append("\n");
sb.append(" totalInvoiceBalance: ").append(toIndentedString(totalInvoiceBalance)).append("\n");
sb.append(" unappliedBalance: ").append(toIndentedString(unappliedBalance)).append("\n");
sb.append(" vATId: ").append(toIndentedString(vATId)).append("\n");
sb.append(" mrr: ").append(toIndentedString(mrr)).append("\n");
sb.append(" totalDebitMemoBalance: ").append(toIndentedString(totalDebitMemoBalance)).append("\n");
sb.append(" unappliedCreditMemoAmount: ").append(toIndentedString(unappliedCreditMemoAmount)).append("\n");
sb.append(" creditMemoTemplateId: ").append(toIndentedString(creditMemoTemplateId)).append("\n");
sb.append(" debitMemoTemplateId: ").append(toIndentedString(debitMemoTemplateId)).append("\n");
sb.append(" paymentGateway: ").append(toIndentedString(paymentGateway)).append("\n");
sb.append(" paymentTerm: ").append(toIndentedString(paymentTerm)).append("\n");
sb.append(" billTo: ").append(toIndentedString(billTo)).append("\n");
sb.append(" soldTo: ").append(toIndentedString(soldTo)).append("\n");
sb.append(" defaultPaymentMethod: ").append(toIndentedString(defaultPaymentMethod)).append("\n");
sb.append(" subscriptions: ").append(toIndentedString(subscriptions)).append("\n");
sb.append(" payments: ").append(toIndentedString(payments)).append("\n");
sb.append(" refunds: ").append(toIndentedString(refunds)).append("\n");
sb.append(" creditMemos: ").append(toIndentedString(creditMemos)).append("\n");
sb.append(" debitMemos: ").append(toIndentedString(debitMemos)).append("\n");
sb.append(" invoices: ").append(toIndentedString(invoices)).append("\n");
sb.append(" usages: ").append(toIndentedString(usages)).append("\n");
sb.append(" paymentMethods: ").append(toIndentedString(paymentMethods)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("id");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
openapiFields.add("accountNumber");
openapiFields.add("additionalEmailAddresses");
openapiFields.add("allowInvoiceEdit");
openapiFields.add("autoPay");
openapiFields.add("balance");
openapiFields.add("batch");
openapiFields.add("bcdSettingOption");
openapiFields.add("billCycleDay");
openapiFields.add("billToId");
openapiFields.add("communicationProfileId");
openapiFields.add("creditBalance");
openapiFields.add("crmId");
openapiFields.add("currency");
openapiFields.add("customerServiceRepName");
openapiFields.add("defaultPaymentMethodId");
openapiFields.add("eInvoiceProfileId");
openapiFields.add("invoiceDeliveryPrefsEmail");
openapiFields.add("invoiceDeliveryPrefsPrint");
openapiFields.add("invoiceTemplateId");
openapiFields.add("lastInvoiceDate");
openapiFields.add("lastMetricsUpdate");
openapiFields.add("name");
openapiFields.add("notes");
openapiFields.add("organizationId");
openapiFields.add("parentId");
openapiFields.add("partnerAccount");
openapiFields.add("paymentMethodCascadingConsent");
openapiFields.add("purchaseOrderNumber");
openapiFields.add("salesRepName");
openapiFields.add("sequenceSetId");
openapiFields.add("soldToId");
openapiFields.add("status");
openapiFields.add("taxCompanyCode");
openapiFields.add("taxExemptCertificateID");
openapiFields.add("taxExemptCertificateType");
openapiFields.add("taxExemptDescription");
openapiFields.add("taxExemptEffectiveDate");
openapiFields.add("taxExemptEntityUseCode");
openapiFields.add("taxExemptExpirationDate");
openapiFields.add("taxExemptIssuingJurisdiction");
openapiFields.add("taxExemptStatus");
openapiFields.add("totalInvoiceBalance");
openapiFields.add("unappliedBalance");
openapiFields.add("vATId");
openapiFields.add("mrr");
openapiFields.add("totalDebitMemoBalance");
openapiFields.add("unappliedCreditMemoAmount");
openapiFields.add("creditMemoTemplateId");
openapiFields.add("debitMemoTemplateId");
openapiFields.add("paymentGateway");
openapiFields.add("paymentTerm");
openapiFields.add("billTo");
openapiFields.add("soldTo");
openapiFields.add("defaultPaymentMethod");
openapiFields.add("subscriptions");
openapiFields.add("payments");
openapiFields.add("refunds");
openapiFields.add("creditMemos");
openapiFields.add("debitMemos");
openapiFields.add("invoices");
openapiFields.add("usages");
openapiFields.add("paymentMethods");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to ExpandedAccount
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!ExpandedAccount.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in ExpandedAccount is not found in the empty JSON string", ExpandedAccount.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
if ((jsonObj.get("accountNumber") != null && !jsonObj.get("accountNumber").isJsonNull()) && !jsonObj.get("accountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountNumber").toString()));
}
if ((jsonObj.get("additionalEmailAddresses") != null && !jsonObj.get("additionalEmailAddresses").isJsonNull()) && !jsonObj.get("additionalEmailAddresses").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `additionalEmailAddresses` to be a primitive type in the JSON string but got `%s`", jsonObj.get("additionalEmailAddresses").toString()));
}
if ((jsonObj.get("batch") != null && !jsonObj.get("batch").isJsonNull()) && !jsonObj.get("batch").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `batch` to be a primitive type in the JSON string but got `%s`", jsonObj.get("batch").toString()));
}
if ((jsonObj.get("bcdSettingOption") != null && !jsonObj.get("bcdSettingOption").isJsonNull()) && !jsonObj.get("bcdSettingOption").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bcdSettingOption` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bcdSettingOption").toString()));
}
if ((jsonObj.get("billToId") != null && !jsonObj.get("billToId").isJsonNull()) && !jsonObj.get("billToId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billToId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billToId").toString()));
}
if ((jsonObj.get("communicationProfileId") != null && !jsonObj.get("communicationProfileId").isJsonNull()) && !jsonObj.get("communicationProfileId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `communicationProfileId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("communicationProfileId").toString()));
}
if ((jsonObj.get("crmId") != null && !jsonObj.get("crmId").isJsonNull()) && !jsonObj.get("crmId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `crmId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("crmId").toString()));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("customerServiceRepName") != null && !jsonObj.get("customerServiceRepName").isJsonNull()) && !jsonObj.get("customerServiceRepName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `customerServiceRepName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("customerServiceRepName").toString()));
}
if ((jsonObj.get("defaultPaymentMethodId") != null && !jsonObj.get("defaultPaymentMethodId").isJsonNull()) && !jsonObj.get("defaultPaymentMethodId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `defaultPaymentMethodId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("defaultPaymentMethodId").toString()));
}
if ((jsonObj.get("eInvoiceProfileId") != null && !jsonObj.get("eInvoiceProfileId").isJsonNull()) && !jsonObj.get("eInvoiceProfileId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `eInvoiceProfileId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("eInvoiceProfileId").toString()));
}
if ((jsonObj.get("invoiceTemplateId") != null && !jsonObj.get("invoiceTemplateId").isJsonNull()) && !jsonObj.get("invoiceTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceTemplateId").toString()));
}
if ((jsonObj.get("lastMetricsUpdate") != null && !jsonObj.get("lastMetricsUpdate").isJsonNull()) && !jsonObj.get("lastMetricsUpdate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `lastMetricsUpdate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("lastMetricsUpdate").toString()));
}
if ((jsonObj.get("name") != null && !jsonObj.get("name").isJsonNull()) && !jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if ((jsonObj.get("notes") != null && !jsonObj.get("notes").isJsonNull()) && !jsonObj.get("notes").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `notes` to be a primitive type in the JSON string but got `%s`", jsonObj.get("notes").toString()));
}
if ((jsonObj.get("organizationId") != null && !jsonObj.get("organizationId").isJsonNull()) && !jsonObj.get("organizationId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `organizationId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("organizationId").toString()));
}
if ((jsonObj.get("parentId") != null && !jsonObj.get("parentId").isJsonNull()) && !jsonObj.get("parentId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `parentId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("parentId").toString()));
}
if ((jsonObj.get("purchaseOrderNumber") != null && !jsonObj.get("purchaseOrderNumber").isJsonNull()) && !jsonObj.get("purchaseOrderNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `purchaseOrderNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("purchaseOrderNumber").toString()));
}
if ((jsonObj.get("salesRepName") != null && !jsonObj.get("salesRepName").isJsonNull()) && !jsonObj.get("salesRepName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `salesRepName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("salesRepName").toString()));
}
if ((jsonObj.get("sequenceSetId") != null && !jsonObj.get("sequenceSetId").isJsonNull()) && !jsonObj.get("sequenceSetId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sequenceSetId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sequenceSetId").toString()));
}
if ((jsonObj.get("soldToId") != null && !jsonObj.get("soldToId").isJsonNull()) && !jsonObj.get("soldToId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToId").toString()));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
if ((jsonObj.get("taxCompanyCode") != null && !jsonObj.get("taxCompanyCode").isJsonNull()) && !jsonObj.get("taxCompanyCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxCompanyCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxCompanyCode").toString()));
}
if ((jsonObj.get("taxExemptCertificateID") != null && !jsonObj.get("taxExemptCertificateID").isJsonNull()) && !jsonObj.get("taxExemptCertificateID").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxExemptCertificateID` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxExemptCertificateID").toString()));
}
if ((jsonObj.get("taxExemptCertificateType") != null && !jsonObj.get("taxExemptCertificateType").isJsonNull()) && !jsonObj.get("taxExemptCertificateType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxExemptCertificateType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxExemptCertificateType").toString()));
}
if ((jsonObj.get("taxExemptDescription") != null && !jsonObj.get("taxExemptDescription").isJsonNull()) && !jsonObj.get("taxExemptDescription").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxExemptDescription` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxExemptDescription").toString()));
}
if ((jsonObj.get("taxExemptEntityUseCode") != null && !jsonObj.get("taxExemptEntityUseCode").isJsonNull()) && !jsonObj.get("taxExemptEntityUseCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxExemptEntityUseCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxExemptEntityUseCode").toString()));
}
if ((jsonObj.get("taxExemptIssuingJurisdiction") != null && !jsonObj.get("taxExemptIssuingJurisdiction").isJsonNull()) && !jsonObj.get("taxExemptIssuingJurisdiction").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxExemptIssuingJurisdiction` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxExemptIssuingJurisdiction").toString()));
}
if ((jsonObj.get("taxExemptStatus") != null && !jsonObj.get("taxExemptStatus").isJsonNull()) && !jsonObj.get("taxExemptStatus").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxExemptStatus` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxExemptStatus").toString()));
}
if ((jsonObj.get("vATId") != null && !jsonObj.get("vATId").isJsonNull()) && !jsonObj.get("vATId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `vATId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("vATId").toString()));
}
if ((jsonObj.get("creditMemoTemplateId") != null && !jsonObj.get("creditMemoTemplateId").isJsonNull()) && !jsonObj.get("creditMemoTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creditMemoTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creditMemoTemplateId").toString()));
}
if ((jsonObj.get("debitMemoTemplateId") != null && !jsonObj.get("debitMemoTemplateId").isJsonNull()) && !jsonObj.get("debitMemoTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `debitMemoTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("debitMemoTemplateId").toString()));
}
if ((jsonObj.get("paymentGateway") != null && !jsonObj.get("paymentGateway").isJsonNull()) && !jsonObj.get("paymentGateway").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentGateway` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentGateway").toString()));
}
if ((jsonObj.get("paymentTerm") != null && !jsonObj.get("paymentTerm").isJsonNull()) && !jsonObj.get("paymentTerm").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentTerm` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentTerm").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("subscriptions") != null && !jsonObj.get("subscriptions").isJsonNull() && !jsonObj.get("subscriptions").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptions` to be an array in the JSON string but got `%s`", jsonObj.get("subscriptions").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("payments") != null && !jsonObj.get("payments").isJsonNull() && !jsonObj.get("payments").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `payments` to be an array in the JSON string but got `%s`", jsonObj.get("payments").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("refunds") != null && !jsonObj.get("refunds").isJsonNull() && !jsonObj.get("refunds").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `refunds` to be an array in the JSON string but got `%s`", jsonObj.get("refunds").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("creditMemos") != null && !jsonObj.get("creditMemos").isJsonNull() && !jsonObj.get("creditMemos").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `creditMemos` to be an array in the JSON string but got `%s`", jsonObj.get("creditMemos").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("debitMemos") != null && !jsonObj.get("debitMemos").isJsonNull() && !jsonObj.get("debitMemos").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `debitMemos` to be an array in the JSON string but got `%s`", jsonObj.get("debitMemos").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("invoices") != null && !jsonObj.get("invoices").isJsonNull() && !jsonObj.get("invoices").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `invoices` to be an array in the JSON string but got `%s`", jsonObj.get("invoices").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("usages") != null && !jsonObj.get("usages").isJsonNull() && !jsonObj.get("usages").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `usages` to be an array in the JSON string but got `%s`", jsonObj.get("usages").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("paymentMethods") != null && !jsonObj.get("paymentMethods").isJsonNull() && !jsonObj.get("paymentMethods").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentMethods` to be an array in the JSON string but got `%s`", jsonObj.get("paymentMethods").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!ExpandedAccount.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'ExpandedAccount' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(ExpandedAccount.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, ExpandedAccount value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public ExpandedAccount read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
ExpandedAccount instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of ExpandedAccount given an JSON string
*
* @param jsonString JSON string
* @return An instance of ExpandedAccount
* @throws IOException if the JSON string is invalid with respect to ExpandedAccount
*/
public static ExpandedAccount fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, ExpandedAccount.class);
}
/**
* Convert an instance of ExpandedAccount to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy