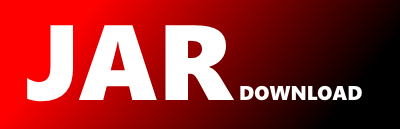
com.zuora.model.ExpandedBillingRun Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.time.LocalDate;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* ExpandedBillingRun
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class ExpandedBillingRun {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public static final String SERIALIZED_NAME_BILLING_RUN_NUMBER = "billingRunNumber";
@SerializedName(SERIALIZED_NAME_BILLING_RUN_NUMBER)
private String billingRunNumber;
public static final String SERIALIZED_NAME_BILLING_RUN_TYPE = "billingRunType";
@SerializedName(SERIALIZED_NAME_BILLING_RUN_TYPE)
private String billingRunType;
public static final String SERIALIZED_NAME_END_DATE = "endDate";
@SerializedName(SERIALIZED_NAME_END_DATE)
private String endDate;
public static final String SERIALIZED_NAME_ERROR_MESSAGE = "errorMessage";
@SerializedName(SERIALIZED_NAME_ERROR_MESSAGE)
private String errorMessage;
public static final String SERIALIZED_NAME_EXECUTED_DATE = "executedDate";
@SerializedName(SERIALIZED_NAME_EXECUTED_DATE)
private String executedDate;
public static final String SERIALIZED_NAME_INVOICE_DATE = "invoiceDate";
@SerializedName(SERIALIZED_NAME_INVOICE_DATE)
private LocalDate invoiceDate;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_NUMBER_OF_ACCOUNTS = "numberOfAccounts";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_ACCOUNTS)
private Long numberOfAccounts;
public static final String SERIALIZED_NAME_NUMBER_OF_INVOICES = "numberOfInvoices";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_INVOICES)
private Long numberOfInvoices;
public static final String SERIALIZED_NAME_NUMBER_OF_CREDIT_MEMOS = "numberOfCreditMemos";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_CREDIT_MEMOS)
private Long numberOfCreditMemos;
public static final String SERIALIZED_NAME_POSTED_DATE = "postedDate";
@SerializedName(SERIALIZED_NAME_POSTED_DATE)
private String postedDate;
public static final String SERIALIZED_NAME_START_DATE = "startDate";
@SerializedName(SERIALIZED_NAME_START_DATE)
private String startDate;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private String status;
public static final String SERIALIZED_NAME_TARGET_DATE = "targetDate";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public static final String SERIALIZED_NAME_TARGET_TYPE = "targetType";
@SerializedName(SERIALIZED_NAME_TARGET_TYPE)
private String targetType;
public static final String SERIALIZED_NAME_TOTAL_TIME = "totalTime";
@SerializedName(SERIALIZED_NAME_TOTAL_TIME)
private Long totalTime;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "accountId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_BILL_CYCLE_DAY = "billCycleDay";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_DAY)
private String billCycleDay;
public static final String SERIALIZED_NAME_BATCHES = "batches";
@SerializedName(SERIALIZED_NAME_BATCHES)
private String batches;
public static final String SERIALIZED_NAME_NO_EMAIL_FOR_ZERO_AMOUNT_INVOICE = "noEmailForZeroAmountInvoice";
@SerializedName(SERIALIZED_NAME_NO_EMAIL_FOR_ZERO_AMOUNT_INVOICE)
private Boolean noEmailForZeroAmountInvoice;
public static final String SERIALIZED_NAME_AUTO_POST = "autoPost";
@SerializedName(SERIALIZED_NAME_AUTO_POST)
private Boolean autoPost;
public static final String SERIALIZED_NAME_AUTO_EMAIL = "autoEmail";
@SerializedName(SERIALIZED_NAME_AUTO_EMAIL)
private Boolean autoEmail;
public static final String SERIALIZED_NAME_AUTO_RENEWAL = "autoRenewal";
@SerializedName(SERIALIZED_NAME_AUTO_RENEWAL)
private Boolean autoRenewal;
public static final String SERIALIZED_NAME_INVOICES_EMAILED = "invoicesEmailed";
@SerializedName(SERIALIZED_NAME_INVOICES_EMAILED)
private Boolean invoicesEmailed;
public static final String SERIALIZED_NAME_LAST_EMAIL_SENT_TIME = "lastEmailSentTime";
@SerializedName(SERIALIZED_NAME_LAST_EMAIL_SENT_TIME)
private String lastEmailSentTime;
public static final String SERIALIZED_NAME_TARGET_DATE_OFF_SET = "targetDateOffSet";
@SerializedName(SERIALIZED_NAME_TARGET_DATE_OFF_SET)
private Integer targetDateOffSet;
public static final String SERIALIZED_NAME_INVOICE_DATE_OFF_SET = "invoiceDateOffSet";
@SerializedName(SERIALIZED_NAME_INVOICE_DATE_OFF_SET)
private Integer invoiceDateOffSet;
public static final String SERIALIZED_NAME_CHARGE_TYPE_TO_EXCLUDE = "chargeTypeToExclude";
@SerializedName(SERIALIZED_NAME_CHARGE_TYPE_TO_EXCLUDE)
private String chargeTypeToExclude;
public static final String SERIALIZED_NAME_SCHEDULED_EXECUTION_TIME = "scheduledExecutionTime";
@SerializedName(SERIALIZED_NAME_SCHEDULED_EXECUTION_TIME)
private String scheduledExecutionTime;
public static final String SERIALIZED_NAME_TARGET_DATE_MONTH_OFFSET = "targetDateMonthOffset";
@SerializedName(SERIALIZED_NAME_TARGET_DATE_MONTH_OFFSET)
private Integer targetDateMonthOffset;
public static final String SERIALIZED_NAME_TARGET_DATE_DAY_OF_MONTH = "targetDateDayOfMonth";
@SerializedName(SERIALIZED_NAME_TARGET_DATE_DAY_OF_MONTH)
private String targetDateDayOfMonth;
public static final String SERIALIZED_NAME_INVOICE_DATE_MONTH_OFFSET = "invoiceDateMonthOffset";
@SerializedName(SERIALIZED_NAME_INVOICE_DATE_MONTH_OFFSET)
private Integer invoiceDateMonthOffset;
public static final String SERIALIZED_NAME_INVOICE_DATE_DAY_OF_MONTH = "invoiceDateDayOfMonth";
@SerializedName(SERIALIZED_NAME_INVOICE_DATE_DAY_OF_MONTH)
private String invoiceDateDayOfMonth;
public static final String SERIALIZED_NAME_REPEAT_TYPE = "repeatType";
@SerializedName(SERIALIZED_NAME_REPEAT_TYPE)
private String repeatType;
public static final String SERIALIZED_NAME_REPEAT_FROM = "repeatFrom";
@SerializedName(SERIALIZED_NAME_REPEAT_FROM)
private String repeatFrom;
public static final String SERIALIZED_NAME_REPEAT_TO = "repeatTo";
@SerializedName(SERIALIZED_NAME_REPEAT_TO)
private String repeatTo;
public static final String SERIALIZED_NAME_RUN_TIME = "runTime";
@SerializedName(SERIALIZED_NAME_RUN_TIME)
private Integer runTime;
public static final String SERIALIZED_NAME_TIME_ZONE = "timeZone";
@SerializedName(SERIALIZED_NAME_TIME_ZONE)
private String timeZone;
public static final String SERIALIZED_NAME_MONTHLY_ON_DAY = "monthlyOnDay";
@SerializedName(SERIALIZED_NAME_MONTHLY_ON_DAY)
private String monthlyOnDay;
public static final String SERIALIZED_NAME_WEEKLY_ON_DAY = "weeklyOnDay";
@SerializedName(SERIALIZED_NAME_WEEKLY_ON_DAY)
private String weeklyOnDay;
public ExpandedBillingRun() {
}
public ExpandedBillingRun id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public ExpandedBillingRun createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* Get createdById
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public ExpandedBillingRun createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* Get createdDate
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public ExpandedBillingRun updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* Get updatedById
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public ExpandedBillingRun updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* Get updatedDate
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
public ExpandedBillingRun billingRunNumber(String billingRunNumber) {
this.billingRunNumber = billingRunNumber;
return this;
}
/**
* Get billingRunNumber
* @return billingRunNumber
*/
@javax.annotation.Nullable
public String getBillingRunNumber() {
return billingRunNumber;
}
public void setBillingRunNumber(String billingRunNumber) {
this.billingRunNumber = billingRunNumber;
}
public ExpandedBillingRun billingRunType(String billingRunType) {
this.billingRunType = billingRunType;
return this;
}
/**
* Get billingRunType
* @return billingRunType
*/
@javax.annotation.Nullable
public String getBillingRunType() {
return billingRunType;
}
public void setBillingRunType(String billingRunType) {
this.billingRunType = billingRunType;
}
public ExpandedBillingRun endDate(String endDate) {
this.endDate = endDate;
return this;
}
/**
* Get endDate
* @return endDate
*/
@javax.annotation.Nullable
public String getEndDate() {
return endDate;
}
public void setEndDate(String endDate) {
this.endDate = endDate;
}
public ExpandedBillingRun errorMessage(String errorMessage) {
this.errorMessage = errorMessage;
return this;
}
/**
* Get errorMessage
* @return errorMessage
*/
@javax.annotation.Nullable
public String getErrorMessage() {
return errorMessage;
}
public void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
public ExpandedBillingRun executedDate(String executedDate) {
this.executedDate = executedDate;
return this;
}
/**
* Get executedDate
* @return executedDate
*/
@javax.annotation.Nullable
public String getExecutedDate() {
return executedDate;
}
public void setExecutedDate(String executedDate) {
this.executedDate = executedDate;
}
public ExpandedBillingRun invoiceDate(LocalDate invoiceDate) {
this.invoiceDate = invoiceDate;
return this;
}
/**
* Get invoiceDate
* @return invoiceDate
*/
@javax.annotation.Nullable
public LocalDate getInvoiceDate() {
return invoiceDate;
}
public void setInvoiceDate(LocalDate invoiceDate) {
this.invoiceDate = invoiceDate;
}
public ExpandedBillingRun name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
*/
@javax.annotation.Nullable
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public ExpandedBillingRun numberOfAccounts(Long numberOfAccounts) {
this.numberOfAccounts = numberOfAccounts;
return this;
}
/**
* Get numberOfAccounts
* @return numberOfAccounts
*/
@javax.annotation.Nullable
public Long getNumberOfAccounts() {
return numberOfAccounts;
}
public void setNumberOfAccounts(Long numberOfAccounts) {
this.numberOfAccounts = numberOfAccounts;
}
public ExpandedBillingRun numberOfInvoices(Long numberOfInvoices) {
this.numberOfInvoices = numberOfInvoices;
return this;
}
/**
* Get numberOfInvoices
* @return numberOfInvoices
*/
@javax.annotation.Nullable
public Long getNumberOfInvoices() {
return numberOfInvoices;
}
public void setNumberOfInvoices(Long numberOfInvoices) {
this.numberOfInvoices = numberOfInvoices;
}
public ExpandedBillingRun numberOfCreditMemos(Long numberOfCreditMemos) {
this.numberOfCreditMemos = numberOfCreditMemos;
return this;
}
/**
* Get numberOfCreditMemos
* @return numberOfCreditMemos
*/
@javax.annotation.Nullable
public Long getNumberOfCreditMemos() {
return numberOfCreditMemos;
}
public void setNumberOfCreditMemos(Long numberOfCreditMemos) {
this.numberOfCreditMemos = numberOfCreditMemos;
}
public ExpandedBillingRun postedDate(String postedDate) {
this.postedDate = postedDate;
return this;
}
/**
* Get postedDate
* @return postedDate
*/
@javax.annotation.Nullable
public String getPostedDate() {
return postedDate;
}
public void setPostedDate(String postedDate) {
this.postedDate = postedDate;
}
public ExpandedBillingRun startDate(String startDate) {
this.startDate = startDate;
return this;
}
/**
* Get startDate
* @return startDate
*/
@javax.annotation.Nullable
public String getStartDate() {
return startDate;
}
public void setStartDate(String startDate) {
this.startDate = startDate;
}
public ExpandedBillingRun status(String status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public ExpandedBillingRun targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* Get targetDate
* @return targetDate
*/
@javax.annotation.Nullable
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public ExpandedBillingRun targetType(String targetType) {
this.targetType = targetType;
return this;
}
/**
* Get targetType
* @return targetType
*/
@javax.annotation.Nullable
public String getTargetType() {
return targetType;
}
public void setTargetType(String targetType) {
this.targetType = targetType;
}
public ExpandedBillingRun totalTime(Long totalTime) {
this.totalTime = totalTime;
return this;
}
/**
* Get totalTime
* @return totalTime
*/
@javax.annotation.Nullable
public Long getTotalTime() {
return totalTime;
}
public void setTotalTime(Long totalTime) {
this.totalTime = totalTime;
}
public ExpandedBillingRun accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Get accountId
* @return accountId
*/
@javax.annotation.Nullable
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public ExpandedBillingRun billCycleDay(String billCycleDay) {
this.billCycleDay = billCycleDay;
return this;
}
/**
* Get billCycleDay
* @return billCycleDay
*/
@javax.annotation.Nullable
public String getBillCycleDay() {
return billCycleDay;
}
public void setBillCycleDay(String billCycleDay) {
this.billCycleDay = billCycleDay;
}
public ExpandedBillingRun batches(String batches) {
this.batches = batches;
return this;
}
/**
* Get batches
* @return batches
*/
@javax.annotation.Nullable
public String getBatches() {
return batches;
}
public void setBatches(String batches) {
this.batches = batches;
}
public ExpandedBillingRun noEmailForZeroAmountInvoice(Boolean noEmailForZeroAmountInvoice) {
this.noEmailForZeroAmountInvoice = noEmailForZeroAmountInvoice;
return this;
}
/**
* Get noEmailForZeroAmountInvoice
* @return noEmailForZeroAmountInvoice
*/
@javax.annotation.Nullable
public Boolean getNoEmailForZeroAmountInvoice() {
return noEmailForZeroAmountInvoice;
}
public void setNoEmailForZeroAmountInvoice(Boolean noEmailForZeroAmountInvoice) {
this.noEmailForZeroAmountInvoice = noEmailForZeroAmountInvoice;
}
public ExpandedBillingRun autoPost(Boolean autoPost) {
this.autoPost = autoPost;
return this;
}
/**
* Get autoPost
* @return autoPost
*/
@javax.annotation.Nullable
public Boolean getAutoPost() {
return autoPost;
}
public void setAutoPost(Boolean autoPost) {
this.autoPost = autoPost;
}
public ExpandedBillingRun autoEmail(Boolean autoEmail) {
this.autoEmail = autoEmail;
return this;
}
/**
* Get autoEmail
* @return autoEmail
*/
@javax.annotation.Nullable
public Boolean getAutoEmail() {
return autoEmail;
}
public void setAutoEmail(Boolean autoEmail) {
this.autoEmail = autoEmail;
}
public ExpandedBillingRun autoRenewal(Boolean autoRenewal) {
this.autoRenewal = autoRenewal;
return this;
}
/**
* Get autoRenewal
* @return autoRenewal
*/
@javax.annotation.Nullable
public Boolean getAutoRenewal() {
return autoRenewal;
}
public void setAutoRenewal(Boolean autoRenewal) {
this.autoRenewal = autoRenewal;
}
public ExpandedBillingRun invoicesEmailed(Boolean invoicesEmailed) {
this.invoicesEmailed = invoicesEmailed;
return this;
}
/**
* Get invoicesEmailed
* @return invoicesEmailed
*/
@javax.annotation.Nullable
public Boolean getInvoicesEmailed() {
return invoicesEmailed;
}
public void setInvoicesEmailed(Boolean invoicesEmailed) {
this.invoicesEmailed = invoicesEmailed;
}
public ExpandedBillingRun lastEmailSentTime(String lastEmailSentTime) {
this.lastEmailSentTime = lastEmailSentTime;
return this;
}
/**
* Get lastEmailSentTime
* @return lastEmailSentTime
*/
@javax.annotation.Nullable
public String getLastEmailSentTime() {
return lastEmailSentTime;
}
public void setLastEmailSentTime(String lastEmailSentTime) {
this.lastEmailSentTime = lastEmailSentTime;
}
public ExpandedBillingRun targetDateOffSet(Integer targetDateOffSet) {
this.targetDateOffSet = targetDateOffSet;
return this;
}
/**
* Get targetDateOffSet
* @return targetDateOffSet
*/
@javax.annotation.Nullable
public Integer getTargetDateOffSet() {
return targetDateOffSet;
}
public void setTargetDateOffSet(Integer targetDateOffSet) {
this.targetDateOffSet = targetDateOffSet;
}
public ExpandedBillingRun invoiceDateOffSet(Integer invoiceDateOffSet) {
this.invoiceDateOffSet = invoiceDateOffSet;
return this;
}
/**
* Get invoiceDateOffSet
* @return invoiceDateOffSet
*/
@javax.annotation.Nullable
public Integer getInvoiceDateOffSet() {
return invoiceDateOffSet;
}
public void setInvoiceDateOffSet(Integer invoiceDateOffSet) {
this.invoiceDateOffSet = invoiceDateOffSet;
}
public ExpandedBillingRun chargeTypeToExclude(String chargeTypeToExclude) {
this.chargeTypeToExclude = chargeTypeToExclude;
return this;
}
/**
* Get chargeTypeToExclude
* @return chargeTypeToExclude
*/
@javax.annotation.Nullable
public String getChargeTypeToExclude() {
return chargeTypeToExclude;
}
public void setChargeTypeToExclude(String chargeTypeToExclude) {
this.chargeTypeToExclude = chargeTypeToExclude;
}
public ExpandedBillingRun scheduledExecutionTime(String scheduledExecutionTime) {
this.scheduledExecutionTime = scheduledExecutionTime;
return this;
}
/**
* Get scheduledExecutionTime
* @return scheduledExecutionTime
*/
@javax.annotation.Nullable
public String getScheduledExecutionTime() {
return scheduledExecutionTime;
}
public void setScheduledExecutionTime(String scheduledExecutionTime) {
this.scheduledExecutionTime = scheduledExecutionTime;
}
public ExpandedBillingRun targetDateMonthOffset(Integer targetDateMonthOffset) {
this.targetDateMonthOffset = targetDateMonthOffset;
return this;
}
/**
* Get targetDateMonthOffset
* @return targetDateMonthOffset
*/
@javax.annotation.Nullable
public Integer getTargetDateMonthOffset() {
return targetDateMonthOffset;
}
public void setTargetDateMonthOffset(Integer targetDateMonthOffset) {
this.targetDateMonthOffset = targetDateMonthOffset;
}
public ExpandedBillingRun targetDateDayOfMonth(String targetDateDayOfMonth) {
this.targetDateDayOfMonth = targetDateDayOfMonth;
return this;
}
/**
* Get targetDateDayOfMonth
* @return targetDateDayOfMonth
*/
@javax.annotation.Nullable
public String getTargetDateDayOfMonth() {
return targetDateDayOfMonth;
}
public void setTargetDateDayOfMonth(String targetDateDayOfMonth) {
this.targetDateDayOfMonth = targetDateDayOfMonth;
}
public ExpandedBillingRun invoiceDateMonthOffset(Integer invoiceDateMonthOffset) {
this.invoiceDateMonthOffset = invoiceDateMonthOffset;
return this;
}
/**
* Get invoiceDateMonthOffset
* @return invoiceDateMonthOffset
*/
@javax.annotation.Nullable
public Integer getInvoiceDateMonthOffset() {
return invoiceDateMonthOffset;
}
public void setInvoiceDateMonthOffset(Integer invoiceDateMonthOffset) {
this.invoiceDateMonthOffset = invoiceDateMonthOffset;
}
public ExpandedBillingRun invoiceDateDayOfMonth(String invoiceDateDayOfMonth) {
this.invoiceDateDayOfMonth = invoiceDateDayOfMonth;
return this;
}
/**
* Get invoiceDateDayOfMonth
* @return invoiceDateDayOfMonth
*/
@javax.annotation.Nullable
public String getInvoiceDateDayOfMonth() {
return invoiceDateDayOfMonth;
}
public void setInvoiceDateDayOfMonth(String invoiceDateDayOfMonth) {
this.invoiceDateDayOfMonth = invoiceDateDayOfMonth;
}
public ExpandedBillingRun repeatType(String repeatType) {
this.repeatType = repeatType;
return this;
}
/**
* Get repeatType
* @return repeatType
*/
@javax.annotation.Nullable
public String getRepeatType() {
return repeatType;
}
public void setRepeatType(String repeatType) {
this.repeatType = repeatType;
}
public ExpandedBillingRun repeatFrom(String repeatFrom) {
this.repeatFrom = repeatFrom;
return this;
}
/**
* Get repeatFrom
* @return repeatFrom
*/
@javax.annotation.Nullable
public String getRepeatFrom() {
return repeatFrom;
}
public void setRepeatFrom(String repeatFrom) {
this.repeatFrom = repeatFrom;
}
public ExpandedBillingRun repeatTo(String repeatTo) {
this.repeatTo = repeatTo;
return this;
}
/**
* Get repeatTo
* @return repeatTo
*/
@javax.annotation.Nullable
public String getRepeatTo() {
return repeatTo;
}
public void setRepeatTo(String repeatTo) {
this.repeatTo = repeatTo;
}
public ExpandedBillingRun runTime(Integer runTime) {
this.runTime = runTime;
return this;
}
/**
* Get runTime
* @return runTime
*/
@javax.annotation.Nullable
public Integer getRunTime() {
return runTime;
}
public void setRunTime(Integer runTime) {
this.runTime = runTime;
}
public ExpandedBillingRun timeZone(String timeZone) {
this.timeZone = timeZone;
return this;
}
/**
* Get timeZone
* @return timeZone
*/
@javax.annotation.Nullable
public String getTimeZone() {
return timeZone;
}
public void setTimeZone(String timeZone) {
this.timeZone = timeZone;
}
public ExpandedBillingRun monthlyOnDay(String monthlyOnDay) {
this.monthlyOnDay = monthlyOnDay;
return this;
}
/**
* Get monthlyOnDay
* @return monthlyOnDay
*/
@javax.annotation.Nullable
public String getMonthlyOnDay() {
return monthlyOnDay;
}
public void setMonthlyOnDay(String monthlyOnDay) {
this.monthlyOnDay = monthlyOnDay;
}
public ExpandedBillingRun weeklyOnDay(String weeklyOnDay) {
this.weeklyOnDay = weeklyOnDay;
return this;
}
/**
* Get weeklyOnDay
* @return weeklyOnDay
*/
@javax.annotation.Nullable
public String getWeeklyOnDay() {
return weeklyOnDay;
}
public void setWeeklyOnDay(String weeklyOnDay) {
this.weeklyOnDay = weeklyOnDay;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the ExpandedBillingRun instance itself
*/
public ExpandedBillingRun putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ExpandedBillingRun expandedBillingRun = (ExpandedBillingRun) o;
return Objects.equals(this.id, expandedBillingRun.id) &&
Objects.equals(this.createdById, expandedBillingRun.createdById) &&
Objects.equals(this.createdDate, expandedBillingRun.createdDate) &&
Objects.equals(this.updatedById, expandedBillingRun.updatedById) &&
Objects.equals(this.updatedDate, expandedBillingRun.updatedDate) &&
Objects.equals(this.billingRunNumber, expandedBillingRun.billingRunNumber) &&
Objects.equals(this.billingRunType, expandedBillingRun.billingRunType) &&
Objects.equals(this.endDate, expandedBillingRun.endDate) &&
Objects.equals(this.errorMessage, expandedBillingRun.errorMessage) &&
Objects.equals(this.executedDate, expandedBillingRun.executedDate) &&
Objects.equals(this.invoiceDate, expandedBillingRun.invoiceDate) &&
Objects.equals(this.name, expandedBillingRun.name) &&
Objects.equals(this.numberOfAccounts, expandedBillingRun.numberOfAccounts) &&
Objects.equals(this.numberOfInvoices, expandedBillingRun.numberOfInvoices) &&
Objects.equals(this.numberOfCreditMemos, expandedBillingRun.numberOfCreditMemos) &&
Objects.equals(this.postedDate, expandedBillingRun.postedDate) &&
Objects.equals(this.startDate, expandedBillingRun.startDate) &&
Objects.equals(this.status, expandedBillingRun.status) &&
Objects.equals(this.targetDate, expandedBillingRun.targetDate) &&
Objects.equals(this.targetType, expandedBillingRun.targetType) &&
Objects.equals(this.totalTime, expandedBillingRun.totalTime) &&
Objects.equals(this.accountId, expandedBillingRun.accountId) &&
Objects.equals(this.billCycleDay, expandedBillingRun.billCycleDay) &&
Objects.equals(this.batches, expandedBillingRun.batches) &&
Objects.equals(this.noEmailForZeroAmountInvoice, expandedBillingRun.noEmailForZeroAmountInvoice) &&
Objects.equals(this.autoPost, expandedBillingRun.autoPost) &&
Objects.equals(this.autoEmail, expandedBillingRun.autoEmail) &&
Objects.equals(this.autoRenewal, expandedBillingRun.autoRenewal) &&
Objects.equals(this.invoicesEmailed, expandedBillingRun.invoicesEmailed) &&
Objects.equals(this.lastEmailSentTime, expandedBillingRun.lastEmailSentTime) &&
Objects.equals(this.targetDateOffSet, expandedBillingRun.targetDateOffSet) &&
Objects.equals(this.invoiceDateOffSet, expandedBillingRun.invoiceDateOffSet) &&
Objects.equals(this.chargeTypeToExclude, expandedBillingRun.chargeTypeToExclude) &&
Objects.equals(this.scheduledExecutionTime, expandedBillingRun.scheduledExecutionTime) &&
Objects.equals(this.targetDateMonthOffset, expandedBillingRun.targetDateMonthOffset) &&
Objects.equals(this.targetDateDayOfMonth, expandedBillingRun.targetDateDayOfMonth) &&
Objects.equals(this.invoiceDateMonthOffset, expandedBillingRun.invoiceDateMonthOffset) &&
Objects.equals(this.invoiceDateDayOfMonth, expandedBillingRun.invoiceDateDayOfMonth) &&
Objects.equals(this.repeatType, expandedBillingRun.repeatType) &&
Objects.equals(this.repeatFrom, expandedBillingRun.repeatFrom) &&
Objects.equals(this.repeatTo, expandedBillingRun.repeatTo) &&
Objects.equals(this.runTime, expandedBillingRun.runTime) &&
Objects.equals(this.timeZone, expandedBillingRun.timeZone) &&
Objects.equals(this.monthlyOnDay, expandedBillingRun.monthlyOnDay) &&
Objects.equals(this.weeklyOnDay, expandedBillingRun.weeklyOnDay)&&
Objects.equals(this.additionalProperties, expandedBillingRun.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(id, createdById, createdDate, updatedById, updatedDate, billingRunNumber, billingRunType, endDate, errorMessage, executedDate, invoiceDate, name, numberOfAccounts, numberOfInvoices, numberOfCreditMemos, postedDate, startDate, status, targetDate, targetType, totalTime, accountId, billCycleDay, batches, noEmailForZeroAmountInvoice, autoPost, autoEmail, autoRenewal, invoicesEmailed, lastEmailSentTime, targetDateOffSet, invoiceDateOffSet, chargeTypeToExclude, scheduledExecutionTime, targetDateMonthOffset, targetDateDayOfMonth, invoiceDateMonthOffset, invoiceDateDayOfMonth, repeatType, repeatFrom, repeatTo, runTime, timeZone, monthlyOnDay, weeklyOnDay, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ExpandedBillingRun {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" billingRunNumber: ").append(toIndentedString(billingRunNumber)).append("\n");
sb.append(" billingRunType: ").append(toIndentedString(billingRunType)).append("\n");
sb.append(" endDate: ").append(toIndentedString(endDate)).append("\n");
sb.append(" errorMessage: ").append(toIndentedString(errorMessage)).append("\n");
sb.append(" executedDate: ").append(toIndentedString(executedDate)).append("\n");
sb.append(" invoiceDate: ").append(toIndentedString(invoiceDate)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" numberOfAccounts: ").append(toIndentedString(numberOfAccounts)).append("\n");
sb.append(" numberOfInvoices: ").append(toIndentedString(numberOfInvoices)).append("\n");
sb.append(" numberOfCreditMemos: ").append(toIndentedString(numberOfCreditMemos)).append("\n");
sb.append(" postedDate: ").append(toIndentedString(postedDate)).append("\n");
sb.append(" startDate: ").append(toIndentedString(startDate)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" targetType: ").append(toIndentedString(targetType)).append("\n");
sb.append(" totalTime: ").append(toIndentedString(totalTime)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" billCycleDay: ").append(toIndentedString(billCycleDay)).append("\n");
sb.append(" batches: ").append(toIndentedString(batches)).append("\n");
sb.append(" noEmailForZeroAmountInvoice: ").append(toIndentedString(noEmailForZeroAmountInvoice)).append("\n");
sb.append(" autoPost: ").append(toIndentedString(autoPost)).append("\n");
sb.append(" autoEmail: ").append(toIndentedString(autoEmail)).append("\n");
sb.append(" autoRenewal: ").append(toIndentedString(autoRenewal)).append("\n");
sb.append(" invoicesEmailed: ").append(toIndentedString(invoicesEmailed)).append("\n");
sb.append(" lastEmailSentTime: ").append(toIndentedString(lastEmailSentTime)).append("\n");
sb.append(" targetDateOffSet: ").append(toIndentedString(targetDateOffSet)).append("\n");
sb.append(" invoiceDateOffSet: ").append(toIndentedString(invoiceDateOffSet)).append("\n");
sb.append(" chargeTypeToExclude: ").append(toIndentedString(chargeTypeToExclude)).append("\n");
sb.append(" scheduledExecutionTime: ").append(toIndentedString(scheduledExecutionTime)).append("\n");
sb.append(" targetDateMonthOffset: ").append(toIndentedString(targetDateMonthOffset)).append("\n");
sb.append(" targetDateDayOfMonth: ").append(toIndentedString(targetDateDayOfMonth)).append("\n");
sb.append(" invoiceDateMonthOffset: ").append(toIndentedString(invoiceDateMonthOffset)).append("\n");
sb.append(" invoiceDateDayOfMonth: ").append(toIndentedString(invoiceDateDayOfMonth)).append("\n");
sb.append(" repeatType: ").append(toIndentedString(repeatType)).append("\n");
sb.append(" repeatFrom: ").append(toIndentedString(repeatFrom)).append("\n");
sb.append(" repeatTo: ").append(toIndentedString(repeatTo)).append("\n");
sb.append(" runTime: ").append(toIndentedString(runTime)).append("\n");
sb.append(" timeZone: ").append(toIndentedString(timeZone)).append("\n");
sb.append(" monthlyOnDay: ").append(toIndentedString(monthlyOnDay)).append("\n");
sb.append(" weeklyOnDay: ").append(toIndentedString(weeklyOnDay)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("id");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
openapiFields.add("billingRunNumber");
openapiFields.add("billingRunType");
openapiFields.add("endDate");
openapiFields.add("errorMessage");
openapiFields.add("executedDate");
openapiFields.add("invoiceDate");
openapiFields.add("name");
openapiFields.add("numberOfAccounts");
openapiFields.add("numberOfInvoices");
openapiFields.add("numberOfCreditMemos");
openapiFields.add("postedDate");
openapiFields.add("startDate");
openapiFields.add("status");
openapiFields.add("targetDate");
openapiFields.add("targetType");
openapiFields.add("totalTime");
openapiFields.add("accountId");
openapiFields.add("billCycleDay");
openapiFields.add("batches");
openapiFields.add("noEmailForZeroAmountInvoice");
openapiFields.add("autoPost");
openapiFields.add("autoEmail");
openapiFields.add("autoRenewal");
openapiFields.add("invoicesEmailed");
openapiFields.add("lastEmailSentTime");
openapiFields.add("targetDateOffSet");
openapiFields.add("invoiceDateOffSet");
openapiFields.add("chargeTypeToExclude");
openapiFields.add("scheduledExecutionTime");
openapiFields.add("targetDateMonthOffset");
openapiFields.add("targetDateDayOfMonth");
openapiFields.add("invoiceDateMonthOffset");
openapiFields.add("invoiceDateDayOfMonth");
openapiFields.add("repeatType");
openapiFields.add("repeatFrom");
openapiFields.add("repeatTo");
openapiFields.add("runTime");
openapiFields.add("timeZone");
openapiFields.add("monthlyOnDay");
openapiFields.add("weeklyOnDay");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to ExpandedBillingRun
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!ExpandedBillingRun.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in ExpandedBillingRun is not found in the empty JSON string", ExpandedBillingRun.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
if ((jsonObj.get("billingRunNumber") != null && !jsonObj.get("billingRunNumber").isJsonNull()) && !jsonObj.get("billingRunNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billingRunNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billingRunNumber").toString()));
}
if ((jsonObj.get("billingRunType") != null && !jsonObj.get("billingRunType").isJsonNull()) && !jsonObj.get("billingRunType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billingRunType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billingRunType").toString()));
}
if ((jsonObj.get("endDate") != null && !jsonObj.get("endDate").isJsonNull()) && !jsonObj.get("endDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `endDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("endDate").toString()));
}
if ((jsonObj.get("errorMessage") != null && !jsonObj.get("errorMessage").isJsonNull()) && !jsonObj.get("errorMessage").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `errorMessage` to be a primitive type in the JSON string but got `%s`", jsonObj.get("errorMessage").toString()));
}
if ((jsonObj.get("executedDate") != null && !jsonObj.get("executedDate").isJsonNull()) && !jsonObj.get("executedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `executedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("executedDate").toString()));
}
if ((jsonObj.get("name") != null && !jsonObj.get("name").isJsonNull()) && !jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if ((jsonObj.get("postedDate") != null && !jsonObj.get("postedDate").isJsonNull()) && !jsonObj.get("postedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `postedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("postedDate").toString()));
}
if ((jsonObj.get("startDate") != null && !jsonObj.get("startDate").isJsonNull()) && !jsonObj.get("startDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `startDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("startDate").toString()));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
if ((jsonObj.get("targetType") != null && !jsonObj.get("targetType").isJsonNull()) && !jsonObj.get("targetType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `targetType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("targetType").toString()));
}
if ((jsonObj.get("accountId") != null && !jsonObj.get("accountId").isJsonNull()) && !jsonObj.get("accountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountId").toString()));
}
if ((jsonObj.get("billCycleDay") != null && !jsonObj.get("billCycleDay").isJsonNull()) && !jsonObj.get("billCycleDay").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billCycleDay` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billCycleDay").toString()));
}
if ((jsonObj.get("batches") != null && !jsonObj.get("batches").isJsonNull()) && !jsonObj.get("batches").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `batches` to be a primitive type in the JSON string but got `%s`", jsonObj.get("batches").toString()));
}
if ((jsonObj.get("lastEmailSentTime") != null && !jsonObj.get("lastEmailSentTime").isJsonNull()) && !jsonObj.get("lastEmailSentTime").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `lastEmailSentTime` to be a primitive type in the JSON string but got `%s`", jsonObj.get("lastEmailSentTime").toString()));
}
if ((jsonObj.get("chargeTypeToExclude") != null && !jsonObj.get("chargeTypeToExclude").isJsonNull()) && !jsonObj.get("chargeTypeToExclude").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeTypeToExclude` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeTypeToExclude").toString()));
}
if ((jsonObj.get("scheduledExecutionTime") != null && !jsonObj.get("scheduledExecutionTime").isJsonNull()) && !jsonObj.get("scheduledExecutionTime").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `scheduledExecutionTime` to be a primitive type in the JSON string but got `%s`", jsonObj.get("scheduledExecutionTime").toString()));
}
if ((jsonObj.get("targetDateDayOfMonth") != null && !jsonObj.get("targetDateDayOfMonth").isJsonNull()) && !jsonObj.get("targetDateDayOfMonth").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `targetDateDayOfMonth` to be a primitive type in the JSON string but got `%s`", jsonObj.get("targetDateDayOfMonth").toString()));
}
if ((jsonObj.get("invoiceDateDayOfMonth") != null && !jsonObj.get("invoiceDateDayOfMonth").isJsonNull()) && !jsonObj.get("invoiceDateDayOfMonth").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceDateDayOfMonth` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceDateDayOfMonth").toString()));
}
if ((jsonObj.get("repeatType") != null && !jsonObj.get("repeatType").isJsonNull()) && !jsonObj.get("repeatType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `repeatType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("repeatType").toString()));
}
if ((jsonObj.get("repeatFrom") != null && !jsonObj.get("repeatFrom").isJsonNull()) && !jsonObj.get("repeatFrom").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `repeatFrom` to be a primitive type in the JSON string but got `%s`", jsonObj.get("repeatFrom").toString()));
}
if ((jsonObj.get("repeatTo") != null && !jsonObj.get("repeatTo").isJsonNull()) && !jsonObj.get("repeatTo").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `repeatTo` to be a primitive type in the JSON string but got `%s`", jsonObj.get("repeatTo").toString()));
}
if ((jsonObj.get("timeZone") != null && !jsonObj.get("timeZone").isJsonNull()) && !jsonObj.get("timeZone").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `timeZone` to be a primitive type in the JSON string but got `%s`", jsonObj.get("timeZone").toString()));
}
if ((jsonObj.get("monthlyOnDay") != null && !jsonObj.get("monthlyOnDay").isJsonNull()) && !jsonObj.get("monthlyOnDay").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `monthlyOnDay` to be a primitive type in the JSON string but got `%s`", jsonObj.get("monthlyOnDay").toString()));
}
if ((jsonObj.get("weeklyOnDay") != null && !jsonObj.get("weeklyOnDay").isJsonNull()) && !jsonObj.get("weeklyOnDay").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `weeklyOnDay` to be a primitive type in the JSON string but got `%s`", jsonObj.get("weeklyOnDay").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!ExpandedBillingRun.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'ExpandedBillingRun' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(ExpandedBillingRun.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, ExpandedBillingRun value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public ExpandedBillingRun read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
ExpandedBillingRun instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of ExpandedBillingRun given an JSON string
*
* @param jsonString JSON string
* @return An instance of ExpandedBillingRun
* @throws IOException if the JSON string is invalid with respect to ExpandedBillingRun
*/
public static ExpandedBillingRun fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, ExpandedBillingRun.class);
}
/**
* Convert an instance of ExpandedBillingRun to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy