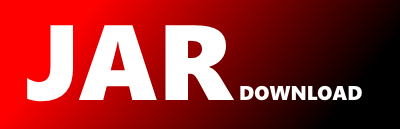
com.zuora.model.ExpandedContact Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* ExpandedContact
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class ExpandedContact {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "accountId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ADDRESS1 = "address1";
@SerializedName(SERIALIZED_NAME_ADDRESS1)
private String address1;
public static final String SERIALIZED_NAME_ADDRESS2 = "address2";
@SerializedName(SERIALIZED_NAME_ADDRESS2)
private String address2;
public static final String SERIALIZED_NAME_CITY = "city";
@SerializedName(SERIALIZED_NAME_CITY)
private String city;
public static final String SERIALIZED_NAME_COUNTRY = "country";
@SerializedName(SERIALIZED_NAME_COUNTRY)
private String country;
public static final String SERIALIZED_NAME_COUNTY = "county";
@SerializedName(SERIALIZED_NAME_COUNTY)
private String county;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_FAX = "fax";
@SerializedName(SERIALIZED_NAME_FAX)
private String fax;
public static final String SERIALIZED_NAME_FIRST_NAME = "firstName";
@SerializedName(SERIALIZED_NAME_FIRST_NAME)
private String firstName;
public static final String SERIALIZED_NAME_HOME_PHONE = "homePhone";
@SerializedName(SERIALIZED_NAME_HOME_PHONE)
private String homePhone;
public static final String SERIALIZED_NAME_LAST_NAME = "lastName";
@SerializedName(SERIALIZED_NAME_LAST_NAME)
private String lastName;
public static final String SERIALIZED_NAME_MOBILE_PHONE = "mobilePhone";
@SerializedName(SERIALIZED_NAME_MOBILE_PHONE)
private String mobilePhone;
public static final String SERIALIZED_NAME_NICK_NAME = "nickName";
@SerializedName(SERIALIZED_NAME_NICK_NAME)
private String nickName;
public static final String SERIALIZED_NAME_OTHER_PHONE = "otherPhone";
@SerializedName(SERIALIZED_NAME_OTHER_PHONE)
private String otherPhone;
public static final String SERIALIZED_NAME_OTHER_PHONE_TYPE = "otherPhoneType";
@SerializedName(SERIALIZED_NAME_OTHER_PHONE_TYPE)
private String otherPhoneType;
public static final String SERIALIZED_NAME_PERSONAL_EMAIL = "personalEmail";
@SerializedName(SERIALIZED_NAME_PERSONAL_EMAIL)
private String personalEmail;
public static final String SERIALIZED_NAME_POSTAL_CODE = "postalCode";
@SerializedName(SERIALIZED_NAME_POSTAL_CODE)
private String postalCode;
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private String state;
public static final String SERIALIZED_NAME_TAX_REGION = "taxRegion";
@SerializedName(SERIALIZED_NAME_TAX_REGION)
private String taxRegion;
public static final String SERIALIZED_NAME_WORK_EMAIL = "workEmail";
@SerializedName(SERIALIZED_NAME_WORK_EMAIL)
private String workEmail;
public static final String SERIALIZED_NAME_WORK_PHONE = "workPhone";
@SerializedName(SERIALIZED_NAME_WORK_PHONE)
private String workPhone;
public ExpandedContact() {
}
public ExpandedContact id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public ExpandedContact createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* Get createdById
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public ExpandedContact createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* Get createdDate
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public ExpandedContact updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* Get updatedById
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public ExpandedContact updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* Get updatedDate
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
public ExpandedContact accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Get accountId
* @return accountId
*/
@javax.annotation.Nullable
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public ExpandedContact address1(String address1) {
this.address1 = address1;
return this;
}
/**
* Get address1
* @return address1
*/
@javax.annotation.Nullable
public String getAddress1() {
return address1;
}
public void setAddress1(String address1) {
this.address1 = address1;
}
public ExpandedContact address2(String address2) {
this.address2 = address2;
return this;
}
/**
* Get address2
* @return address2
*/
@javax.annotation.Nullable
public String getAddress2() {
return address2;
}
public void setAddress2(String address2) {
this.address2 = address2;
}
public ExpandedContact city(String city) {
this.city = city;
return this;
}
/**
* Get city
* @return city
*/
@javax.annotation.Nullable
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public ExpandedContact country(String country) {
this.country = country;
return this;
}
/**
* Get country
* @return country
*/
@javax.annotation.Nullable
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
public ExpandedContact county(String county) {
this.county = county;
return this;
}
/**
* Get county
* @return county
*/
@javax.annotation.Nullable
public String getCounty() {
return county;
}
public void setCounty(String county) {
this.county = county;
}
public ExpandedContact description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public ExpandedContact fax(String fax) {
this.fax = fax;
return this;
}
/**
* Get fax
* @return fax
*/
@javax.annotation.Nullable
public String getFax() {
return fax;
}
public void setFax(String fax) {
this.fax = fax;
}
public ExpandedContact firstName(String firstName) {
this.firstName = firstName;
return this;
}
/**
* Get firstName
* @return firstName
*/
@javax.annotation.Nullable
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public ExpandedContact homePhone(String homePhone) {
this.homePhone = homePhone;
return this;
}
/**
* Get homePhone
* @return homePhone
*/
@javax.annotation.Nullable
public String getHomePhone() {
return homePhone;
}
public void setHomePhone(String homePhone) {
this.homePhone = homePhone;
}
public ExpandedContact lastName(String lastName) {
this.lastName = lastName;
return this;
}
/**
* Get lastName
* @return lastName
*/
@javax.annotation.Nullable
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public ExpandedContact mobilePhone(String mobilePhone) {
this.mobilePhone = mobilePhone;
return this;
}
/**
* Get mobilePhone
* @return mobilePhone
*/
@javax.annotation.Nullable
public String getMobilePhone() {
return mobilePhone;
}
public void setMobilePhone(String mobilePhone) {
this.mobilePhone = mobilePhone;
}
public ExpandedContact nickName(String nickName) {
this.nickName = nickName;
return this;
}
/**
* Get nickName
* @return nickName
*/
@javax.annotation.Nullable
public String getNickName() {
return nickName;
}
public void setNickName(String nickName) {
this.nickName = nickName;
}
public ExpandedContact otherPhone(String otherPhone) {
this.otherPhone = otherPhone;
return this;
}
/**
* Get otherPhone
* @return otherPhone
*/
@javax.annotation.Nullable
public String getOtherPhone() {
return otherPhone;
}
public void setOtherPhone(String otherPhone) {
this.otherPhone = otherPhone;
}
public ExpandedContact otherPhoneType(String otherPhoneType) {
this.otherPhoneType = otherPhoneType;
return this;
}
/**
* Get otherPhoneType
* @return otherPhoneType
*/
@javax.annotation.Nullable
public String getOtherPhoneType() {
return otherPhoneType;
}
public void setOtherPhoneType(String otherPhoneType) {
this.otherPhoneType = otherPhoneType;
}
public ExpandedContact personalEmail(String personalEmail) {
this.personalEmail = personalEmail;
return this;
}
/**
* Get personalEmail
* @return personalEmail
*/
@javax.annotation.Nullable
public String getPersonalEmail() {
return personalEmail;
}
public void setPersonalEmail(String personalEmail) {
this.personalEmail = personalEmail;
}
public ExpandedContact postalCode(String postalCode) {
this.postalCode = postalCode;
return this;
}
/**
* Get postalCode
* @return postalCode
*/
@javax.annotation.Nullable
public String getPostalCode() {
return postalCode;
}
public void setPostalCode(String postalCode) {
this.postalCode = postalCode;
}
public ExpandedContact state(String state) {
this.state = state;
return this;
}
/**
* Get state
* @return state
*/
@javax.annotation.Nullable
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public ExpandedContact taxRegion(String taxRegion) {
this.taxRegion = taxRegion;
return this;
}
/**
* Get taxRegion
* @return taxRegion
*/
@javax.annotation.Nullable
public String getTaxRegion() {
return taxRegion;
}
public void setTaxRegion(String taxRegion) {
this.taxRegion = taxRegion;
}
public ExpandedContact workEmail(String workEmail) {
this.workEmail = workEmail;
return this;
}
/**
* Get workEmail
* @return workEmail
*/
@javax.annotation.Nullable
public String getWorkEmail() {
return workEmail;
}
public void setWorkEmail(String workEmail) {
this.workEmail = workEmail;
}
public ExpandedContact workPhone(String workPhone) {
this.workPhone = workPhone;
return this;
}
/**
* Get workPhone
* @return workPhone
*/
@javax.annotation.Nullable
public String getWorkPhone() {
return workPhone;
}
public void setWorkPhone(String workPhone) {
this.workPhone = workPhone;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the ExpandedContact instance itself
*/
public ExpandedContact putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ExpandedContact expandedContact = (ExpandedContact) o;
return Objects.equals(this.id, expandedContact.id) &&
Objects.equals(this.createdById, expandedContact.createdById) &&
Objects.equals(this.createdDate, expandedContact.createdDate) &&
Objects.equals(this.updatedById, expandedContact.updatedById) &&
Objects.equals(this.updatedDate, expandedContact.updatedDate) &&
Objects.equals(this.accountId, expandedContact.accountId) &&
Objects.equals(this.address1, expandedContact.address1) &&
Objects.equals(this.address2, expandedContact.address2) &&
Objects.equals(this.city, expandedContact.city) &&
Objects.equals(this.country, expandedContact.country) &&
Objects.equals(this.county, expandedContact.county) &&
Objects.equals(this.description, expandedContact.description) &&
Objects.equals(this.fax, expandedContact.fax) &&
Objects.equals(this.firstName, expandedContact.firstName) &&
Objects.equals(this.homePhone, expandedContact.homePhone) &&
Objects.equals(this.lastName, expandedContact.lastName) &&
Objects.equals(this.mobilePhone, expandedContact.mobilePhone) &&
Objects.equals(this.nickName, expandedContact.nickName) &&
Objects.equals(this.otherPhone, expandedContact.otherPhone) &&
Objects.equals(this.otherPhoneType, expandedContact.otherPhoneType) &&
Objects.equals(this.personalEmail, expandedContact.personalEmail) &&
Objects.equals(this.postalCode, expandedContact.postalCode) &&
Objects.equals(this.state, expandedContact.state) &&
Objects.equals(this.taxRegion, expandedContact.taxRegion) &&
Objects.equals(this.workEmail, expandedContact.workEmail) &&
Objects.equals(this.workPhone, expandedContact.workPhone)&&
Objects.equals(this.additionalProperties, expandedContact.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(id, createdById, createdDate, updatedById, updatedDate, accountId, address1, address2, city, country, county, description, fax, firstName, homePhone, lastName, mobilePhone, nickName, otherPhone, otherPhoneType, personalEmail, postalCode, state, taxRegion, workEmail, workPhone, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ExpandedContact {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" address1: ").append(toIndentedString(address1)).append("\n");
sb.append(" address2: ").append(toIndentedString(address2)).append("\n");
sb.append(" city: ").append(toIndentedString(city)).append("\n");
sb.append(" country: ").append(toIndentedString(country)).append("\n");
sb.append(" county: ").append(toIndentedString(county)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" fax: ").append(toIndentedString(fax)).append("\n");
sb.append(" firstName: ").append(toIndentedString(firstName)).append("\n");
sb.append(" homePhone: ").append(toIndentedString(homePhone)).append("\n");
sb.append(" lastName: ").append(toIndentedString(lastName)).append("\n");
sb.append(" mobilePhone: ").append(toIndentedString(mobilePhone)).append("\n");
sb.append(" nickName: ").append(toIndentedString(nickName)).append("\n");
sb.append(" otherPhone: ").append(toIndentedString(otherPhone)).append("\n");
sb.append(" otherPhoneType: ").append(toIndentedString(otherPhoneType)).append("\n");
sb.append(" personalEmail: ").append(toIndentedString(personalEmail)).append("\n");
sb.append(" postalCode: ").append(toIndentedString(postalCode)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" taxRegion: ").append(toIndentedString(taxRegion)).append("\n");
sb.append(" workEmail: ").append(toIndentedString(workEmail)).append("\n");
sb.append(" workPhone: ").append(toIndentedString(workPhone)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("id");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
openapiFields.add("accountId");
openapiFields.add("address1");
openapiFields.add("address2");
openapiFields.add("city");
openapiFields.add("country");
openapiFields.add("county");
openapiFields.add("description");
openapiFields.add("fax");
openapiFields.add("firstName");
openapiFields.add("homePhone");
openapiFields.add("lastName");
openapiFields.add("mobilePhone");
openapiFields.add("nickName");
openapiFields.add("otherPhone");
openapiFields.add("otherPhoneType");
openapiFields.add("personalEmail");
openapiFields.add("postalCode");
openapiFields.add("state");
openapiFields.add("taxRegion");
openapiFields.add("workEmail");
openapiFields.add("workPhone");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to ExpandedContact
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!ExpandedContact.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in ExpandedContact is not found in the empty JSON string", ExpandedContact.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
if ((jsonObj.get("accountId") != null && !jsonObj.get("accountId").isJsonNull()) && !jsonObj.get("accountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountId").toString()));
}
if ((jsonObj.get("address1") != null && !jsonObj.get("address1").isJsonNull()) && !jsonObj.get("address1").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `address1` to be a primitive type in the JSON string but got `%s`", jsonObj.get("address1").toString()));
}
if ((jsonObj.get("address2") != null && !jsonObj.get("address2").isJsonNull()) && !jsonObj.get("address2").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `address2` to be a primitive type in the JSON string but got `%s`", jsonObj.get("address2").toString()));
}
if ((jsonObj.get("city") != null && !jsonObj.get("city").isJsonNull()) && !jsonObj.get("city").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `city` to be a primitive type in the JSON string but got `%s`", jsonObj.get("city").toString()));
}
if ((jsonObj.get("country") != null && !jsonObj.get("country").isJsonNull()) && !jsonObj.get("country").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `country` to be a primitive type in the JSON string but got `%s`", jsonObj.get("country").toString()));
}
if ((jsonObj.get("county") != null && !jsonObj.get("county").isJsonNull()) && !jsonObj.get("county").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `county` to be a primitive type in the JSON string but got `%s`", jsonObj.get("county").toString()));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if ((jsonObj.get("fax") != null && !jsonObj.get("fax").isJsonNull()) && !jsonObj.get("fax").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `fax` to be a primitive type in the JSON string but got `%s`", jsonObj.get("fax").toString()));
}
if ((jsonObj.get("firstName") != null && !jsonObj.get("firstName").isJsonNull()) && !jsonObj.get("firstName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `firstName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("firstName").toString()));
}
if ((jsonObj.get("homePhone") != null && !jsonObj.get("homePhone").isJsonNull()) && !jsonObj.get("homePhone").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `homePhone` to be a primitive type in the JSON string but got `%s`", jsonObj.get("homePhone").toString()));
}
if ((jsonObj.get("lastName") != null && !jsonObj.get("lastName").isJsonNull()) && !jsonObj.get("lastName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `lastName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("lastName").toString()));
}
if ((jsonObj.get("mobilePhone") != null && !jsonObj.get("mobilePhone").isJsonNull()) && !jsonObj.get("mobilePhone").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mobilePhone` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mobilePhone").toString()));
}
if ((jsonObj.get("nickName") != null && !jsonObj.get("nickName").isJsonNull()) && !jsonObj.get("nickName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `nickName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("nickName").toString()));
}
if ((jsonObj.get("otherPhone") != null && !jsonObj.get("otherPhone").isJsonNull()) && !jsonObj.get("otherPhone").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `otherPhone` to be a primitive type in the JSON string but got `%s`", jsonObj.get("otherPhone").toString()));
}
if ((jsonObj.get("otherPhoneType") != null && !jsonObj.get("otherPhoneType").isJsonNull()) && !jsonObj.get("otherPhoneType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `otherPhoneType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("otherPhoneType").toString()));
}
if ((jsonObj.get("personalEmail") != null && !jsonObj.get("personalEmail").isJsonNull()) && !jsonObj.get("personalEmail").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `personalEmail` to be a primitive type in the JSON string but got `%s`", jsonObj.get("personalEmail").toString()));
}
if ((jsonObj.get("postalCode") != null && !jsonObj.get("postalCode").isJsonNull()) && !jsonObj.get("postalCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `postalCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("postalCode").toString()));
}
if ((jsonObj.get("state") != null && !jsonObj.get("state").isJsonNull()) && !jsonObj.get("state").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `state` to be a primitive type in the JSON string but got `%s`", jsonObj.get("state").toString()));
}
if ((jsonObj.get("taxRegion") != null && !jsonObj.get("taxRegion").isJsonNull()) && !jsonObj.get("taxRegion").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxRegion` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxRegion").toString()));
}
if ((jsonObj.get("workEmail") != null && !jsonObj.get("workEmail").isJsonNull()) && !jsonObj.get("workEmail").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `workEmail` to be a primitive type in the JSON string but got `%s`", jsonObj.get("workEmail").toString()));
}
if ((jsonObj.get("workPhone") != null && !jsonObj.get("workPhone").isJsonNull()) && !jsonObj.get("workPhone").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `workPhone` to be a primitive type in the JSON string but got `%s`", jsonObj.get("workPhone").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!ExpandedContact.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'ExpandedContact' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(ExpandedContact.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, ExpandedContact value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public ExpandedContact read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
ExpandedContact instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of ExpandedContact given an JSON string
*
* @param jsonString JSON string
* @return An instance of ExpandedContact
* @throws IOException if the JSON string is invalid with respect to ExpandedContact
*/
public static ExpandedContact fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, ExpandedContact.class);
}
/**
* Convert an instance of ExpandedContact to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy