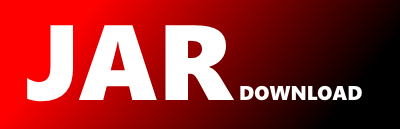
com.zuora.model.ExpandedDailyConsumptionSummary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* ExpandedDailyConsumptionSummary
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class ExpandedDailyConsumptionSummary {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_ORIGIN_CHARGE_ID = "originChargeId";
@SerializedName(SERIALIZED_NAME_ORIGIN_CHARGE_ID)
private String originChargeId;
public static final String SERIALIZED_NAME_CHARGE_SEGMENT_NUMBER = "chargeSegmentNumber";
@SerializedName(SERIALIZED_NAME_CHARGE_SEGMENT_NUMBER)
private Integer chargeSegmentNumber;
public static final String SERIALIZED_NAME_FUND_ID = "fundId";
@SerializedName(SERIALIZED_NAME_FUND_ID)
private String fundId;
public static final String SERIALIZED_NAME_DAILY_CONSUMPTION_AMOUNT = "dailyConsumptionAmount";
@SerializedName(SERIALIZED_NAME_DAILY_CONSUMPTION_AMOUNT)
private BigDecimal dailyConsumptionAmount;
public static final String SERIALIZED_NAME_DAILY_TOTAL_QUANTITY = "dailyTotalQuantity";
@SerializedName(SERIALIZED_NAME_DAILY_TOTAL_QUANTITY)
private BigDecimal dailyTotalQuantity;
public static final String SERIALIZED_NAME_TRANSACTION_DATE = "transactionDate";
@SerializedName(SERIALIZED_NAME_TRANSACTION_DATE)
private LocalDate transactionDate;
public static final String SERIALIZED_NAME_RATING_RESULT_ID = "ratingResultId";
@SerializedName(SERIALIZED_NAME_RATING_RESULT_ID)
private String ratingResultId;
public static final String SERIALIZED_NAME_DAILY_GROUP_SUMMARY_ID = "dailyGroupSummaryId";
@SerializedName(SERIALIZED_NAME_DAILY_GROUP_SUMMARY_ID)
private String dailyGroupSummaryId;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public static final String SERIALIZED_NAME_DRAWDOWN_ORIGIN_CHARGE_ID = "drawdownOriginChargeId";
@SerializedName(SERIALIZED_NAME_DRAWDOWN_ORIGIN_CHARGE_ID)
private String drawdownOriginChargeId;
public static final String SERIALIZED_NAME_DRAWDOWN_CHARGE_SEGMENT_NUMBER = "drawdownChargeSegmentNumber";
@SerializedName(SERIALIZED_NAME_DRAWDOWN_CHARGE_SEGMENT_NUMBER)
private Integer drawdownChargeSegmentNumber;
public static final String SERIALIZED_NAME_DRAWDOWN_RECOGNIZED_REVENUE_GL_ACCOUNT_NUMBER = "drawdownRecognizedRevenueGlAccountNumber";
@SerializedName(SERIALIZED_NAME_DRAWDOWN_RECOGNIZED_REVENUE_GL_ACCOUNT_NUMBER)
private String drawdownRecognizedRevenueGlAccountNumber;
public static final String SERIALIZED_NAME_DRAWDOWN_ADJUSTMENT_REVENUE_GL_ACCOUNT_NUMBER = "drawdownAdjustmentRevenueGlAccountNumber";
@SerializedName(SERIALIZED_NAME_DRAWDOWN_ADJUSTMENT_REVENUE_GL_ACCOUNT_NUMBER)
private String drawdownAdjustmentRevenueGlAccountNumber;
public static final String SERIALIZED_NAME_USAGE_CHARGE_NAME = "usageChargeName";
@SerializedName(SERIALIZED_NAME_USAGE_CHARGE_NAME)
private String usageChargeName;
public static final String SERIALIZED_NAME_USAGE_CHARGE_NUMBER = "usageChargeNumber";
@SerializedName(SERIALIZED_NAME_USAGE_CHARGE_NUMBER)
private String usageChargeNumber;
public ExpandedDailyConsumptionSummary() {
}
public ExpandedDailyConsumptionSummary id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public ExpandedDailyConsumptionSummary originChargeId(String originChargeId) {
this.originChargeId = originChargeId;
return this;
}
/**
* Get originChargeId
* @return originChargeId
*/
@javax.annotation.Nullable
public String getOriginChargeId() {
return originChargeId;
}
public void setOriginChargeId(String originChargeId) {
this.originChargeId = originChargeId;
}
public ExpandedDailyConsumptionSummary chargeSegmentNumber(Integer chargeSegmentNumber) {
this.chargeSegmentNumber = chargeSegmentNumber;
return this;
}
/**
* Get chargeSegmentNumber
* @return chargeSegmentNumber
*/
@javax.annotation.Nullable
public Integer getChargeSegmentNumber() {
return chargeSegmentNumber;
}
public void setChargeSegmentNumber(Integer chargeSegmentNumber) {
this.chargeSegmentNumber = chargeSegmentNumber;
}
public ExpandedDailyConsumptionSummary fundId(String fundId) {
this.fundId = fundId;
return this;
}
/**
* Get fundId
* @return fundId
*/
@javax.annotation.Nullable
public String getFundId() {
return fundId;
}
public void setFundId(String fundId) {
this.fundId = fundId;
}
public ExpandedDailyConsumptionSummary dailyConsumptionAmount(BigDecimal dailyConsumptionAmount) {
this.dailyConsumptionAmount = dailyConsumptionAmount;
return this;
}
/**
* Get dailyConsumptionAmount
* @return dailyConsumptionAmount
*/
@javax.annotation.Nullable
public BigDecimal getDailyConsumptionAmount() {
return dailyConsumptionAmount;
}
public void setDailyConsumptionAmount(BigDecimal dailyConsumptionAmount) {
this.dailyConsumptionAmount = dailyConsumptionAmount;
}
public ExpandedDailyConsumptionSummary dailyTotalQuantity(BigDecimal dailyTotalQuantity) {
this.dailyTotalQuantity = dailyTotalQuantity;
return this;
}
/**
* Get dailyTotalQuantity
* @return dailyTotalQuantity
*/
@javax.annotation.Nullable
public BigDecimal getDailyTotalQuantity() {
return dailyTotalQuantity;
}
public void setDailyTotalQuantity(BigDecimal dailyTotalQuantity) {
this.dailyTotalQuantity = dailyTotalQuantity;
}
public ExpandedDailyConsumptionSummary transactionDate(LocalDate transactionDate) {
this.transactionDate = transactionDate;
return this;
}
/**
* Get transactionDate
* @return transactionDate
*/
@javax.annotation.Nullable
public LocalDate getTransactionDate() {
return transactionDate;
}
public void setTransactionDate(LocalDate transactionDate) {
this.transactionDate = transactionDate;
}
public ExpandedDailyConsumptionSummary ratingResultId(String ratingResultId) {
this.ratingResultId = ratingResultId;
return this;
}
/**
* Get ratingResultId
* @return ratingResultId
*/
@javax.annotation.Nullable
public String getRatingResultId() {
return ratingResultId;
}
public void setRatingResultId(String ratingResultId) {
this.ratingResultId = ratingResultId;
}
public ExpandedDailyConsumptionSummary dailyGroupSummaryId(String dailyGroupSummaryId) {
this.dailyGroupSummaryId = dailyGroupSummaryId;
return this;
}
/**
* Get dailyGroupSummaryId
* @return dailyGroupSummaryId
*/
@javax.annotation.Nullable
public String getDailyGroupSummaryId() {
return dailyGroupSummaryId;
}
public void setDailyGroupSummaryId(String dailyGroupSummaryId) {
this.dailyGroupSummaryId = dailyGroupSummaryId;
}
public ExpandedDailyConsumptionSummary createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* Get createdById
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public ExpandedDailyConsumptionSummary createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* Get createdDate
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public ExpandedDailyConsumptionSummary updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* Get updatedById
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public ExpandedDailyConsumptionSummary updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* Get updatedDate
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
public ExpandedDailyConsumptionSummary drawdownOriginChargeId(String drawdownOriginChargeId) {
this.drawdownOriginChargeId = drawdownOriginChargeId;
return this;
}
/**
* Get drawdownOriginChargeId
* @return drawdownOriginChargeId
*/
@javax.annotation.Nullable
public String getDrawdownOriginChargeId() {
return drawdownOriginChargeId;
}
public void setDrawdownOriginChargeId(String drawdownOriginChargeId) {
this.drawdownOriginChargeId = drawdownOriginChargeId;
}
public ExpandedDailyConsumptionSummary drawdownChargeSegmentNumber(Integer drawdownChargeSegmentNumber) {
this.drawdownChargeSegmentNumber = drawdownChargeSegmentNumber;
return this;
}
/**
* Get drawdownChargeSegmentNumber
* @return drawdownChargeSegmentNumber
*/
@javax.annotation.Nullable
public Integer getDrawdownChargeSegmentNumber() {
return drawdownChargeSegmentNumber;
}
public void setDrawdownChargeSegmentNumber(Integer drawdownChargeSegmentNumber) {
this.drawdownChargeSegmentNumber = drawdownChargeSegmentNumber;
}
public ExpandedDailyConsumptionSummary drawdownRecognizedRevenueGlAccountNumber(String drawdownRecognizedRevenueGlAccountNumber) {
this.drawdownRecognizedRevenueGlAccountNumber = drawdownRecognizedRevenueGlAccountNumber;
return this;
}
/**
* Get drawdownRecognizedRevenueGlAccountNumber
* @return drawdownRecognizedRevenueGlAccountNumber
*/
@javax.annotation.Nullable
public String getDrawdownRecognizedRevenueGlAccountNumber() {
return drawdownRecognizedRevenueGlAccountNumber;
}
public void setDrawdownRecognizedRevenueGlAccountNumber(String drawdownRecognizedRevenueGlAccountNumber) {
this.drawdownRecognizedRevenueGlAccountNumber = drawdownRecognizedRevenueGlAccountNumber;
}
public ExpandedDailyConsumptionSummary drawdownAdjustmentRevenueGlAccountNumber(String drawdownAdjustmentRevenueGlAccountNumber) {
this.drawdownAdjustmentRevenueGlAccountNumber = drawdownAdjustmentRevenueGlAccountNumber;
return this;
}
/**
* Get drawdownAdjustmentRevenueGlAccountNumber
* @return drawdownAdjustmentRevenueGlAccountNumber
*/
@javax.annotation.Nullable
public String getDrawdownAdjustmentRevenueGlAccountNumber() {
return drawdownAdjustmentRevenueGlAccountNumber;
}
public void setDrawdownAdjustmentRevenueGlAccountNumber(String drawdownAdjustmentRevenueGlAccountNumber) {
this.drawdownAdjustmentRevenueGlAccountNumber = drawdownAdjustmentRevenueGlAccountNumber;
}
public ExpandedDailyConsumptionSummary usageChargeName(String usageChargeName) {
this.usageChargeName = usageChargeName;
return this;
}
/**
* Get usageChargeName
* @return usageChargeName
*/
@javax.annotation.Nullable
public String getUsageChargeName() {
return usageChargeName;
}
public void setUsageChargeName(String usageChargeName) {
this.usageChargeName = usageChargeName;
}
public ExpandedDailyConsumptionSummary usageChargeNumber(String usageChargeNumber) {
this.usageChargeNumber = usageChargeNumber;
return this;
}
/**
* Get usageChargeNumber
* @return usageChargeNumber
*/
@javax.annotation.Nullable
public String getUsageChargeNumber() {
return usageChargeNumber;
}
public void setUsageChargeNumber(String usageChargeNumber) {
this.usageChargeNumber = usageChargeNumber;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the ExpandedDailyConsumptionSummary instance itself
*/
public ExpandedDailyConsumptionSummary putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ExpandedDailyConsumptionSummary expandedDailyConsumptionSummary = (ExpandedDailyConsumptionSummary) o;
return Objects.equals(this.id, expandedDailyConsumptionSummary.id) &&
Objects.equals(this.originChargeId, expandedDailyConsumptionSummary.originChargeId) &&
Objects.equals(this.chargeSegmentNumber, expandedDailyConsumptionSummary.chargeSegmentNumber) &&
Objects.equals(this.fundId, expandedDailyConsumptionSummary.fundId) &&
Objects.equals(this.dailyConsumptionAmount, expandedDailyConsumptionSummary.dailyConsumptionAmount) &&
Objects.equals(this.dailyTotalQuantity, expandedDailyConsumptionSummary.dailyTotalQuantity) &&
Objects.equals(this.transactionDate, expandedDailyConsumptionSummary.transactionDate) &&
Objects.equals(this.ratingResultId, expandedDailyConsumptionSummary.ratingResultId) &&
Objects.equals(this.dailyGroupSummaryId, expandedDailyConsumptionSummary.dailyGroupSummaryId) &&
Objects.equals(this.createdById, expandedDailyConsumptionSummary.createdById) &&
Objects.equals(this.createdDate, expandedDailyConsumptionSummary.createdDate) &&
Objects.equals(this.updatedById, expandedDailyConsumptionSummary.updatedById) &&
Objects.equals(this.updatedDate, expandedDailyConsumptionSummary.updatedDate) &&
Objects.equals(this.drawdownOriginChargeId, expandedDailyConsumptionSummary.drawdownOriginChargeId) &&
Objects.equals(this.drawdownChargeSegmentNumber, expandedDailyConsumptionSummary.drawdownChargeSegmentNumber) &&
Objects.equals(this.drawdownRecognizedRevenueGlAccountNumber, expandedDailyConsumptionSummary.drawdownRecognizedRevenueGlAccountNumber) &&
Objects.equals(this.drawdownAdjustmentRevenueGlAccountNumber, expandedDailyConsumptionSummary.drawdownAdjustmentRevenueGlAccountNumber) &&
Objects.equals(this.usageChargeName, expandedDailyConsumptionSummary.usageChargeName) &&
Objects.equals(this.usageChargeNumber, expandedDailyConsumptionSummary.usageChargeNumber)&&
Objects.equals(this.additionalProperties, expandedDailyConsumptionSummary.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(id, originChargeId, chargeSegmentNumber, fundId, dailyConsumptionAmount, dailyTotalQuantity, transactionDate, ratingResultId, dailyGroupSummaryId, createdById, createdDate, updatedById, updatedDate, drawdownOriginChargeId, drawdownChargeSegmentNumber, drawdownRecognizedRevenueGlAccountNumber, drawdownAdjustmentRevenueGlAccountNumber, usageChargeName, usageChargeNumber, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ExpandedDailyConsumptionSummary {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" originChargeId: ").append(toIndentedString(originChargeId)).append("\n");
sb.append(" chargeSegmentNumber: ").append(toIndentedString(chargeSegmentNumber)).append("\n");
sb.append(" fundId: ").append(toIndentedString(fundId)).append("\n");
sb.append(" dailyConsumptionAmount: ").append(toIndentedString(dailyConsumptionAmount)).append("\n");
sb.append(" dailyTotalQuantity: ").append(toIndentedString(dailyTotalQuantity)).append("\n");
sb.append(" transactionDate: ").append(toIndentedString(transactionDate)).append("\n");
sb.append(" ratingResultId: ").append(toIndentedString(ratingResultId)).append("\n");
sb.append(" dailyGroupSummaryId: ").append(toIndentedString(dailyGroupSummaryId)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" drawdownOriginChargeId: ").append(toIndentedString(drawdownOriginChargeId)).append("\n");
sb.append(" drawdownChargeSegmentNumber: ").append(toIndentedString(drawdownChargeSegmentNumber)).append("\n");
sb.append(" drawdownRecognizedRevenueGlAccountNumber: ").append(toIndentedString(drawdownRecognizedRevenueGlAccountNumber)).append("\n");
sb.append(" drawdownAdjustmentRevenueGlAccountNumber: ").append(toIndentedString(drawdownAdjustmentRevenueGlAccountNumber)).append("\n");
sb.append(" usageChargeName: ").append(toIndentedString(usageChargeName)).append("\n");
sb.append(" usageChargeNumber: ").append(toIndentedString(usageChargeNumber)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("id");
openapiFields.add("originChargeId");
openapiFields.add("chargeSegmentNumber");
openapiFields.add("fundId");
openapiFields.add("dailyConsumptionAmount");
openapiFields.add("dailyTotalQuantity");
openapiFields.add("transactionDate");
openapiFields.add("ratingResultId");
openapiFields.add("dailyGroupSummaryId");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
openapiFields.add("drawdownOriginChargeId");
openapiFields.add("drawdownChargeSegmentNumber");
openapiFields.add("drawdownRecognizedRevenueGlAccountNumber");
openapiFields.add("drawdownAdjustmentRevenueGlAccountNumber");
openapiFields.add("usageChargeName");
openapiFields.add("usageChargeNumber");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to ExpandedDailyConsumptionSummary
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!ExpandedDailyConsumptionSummary.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in ExpandedDailyConsumptionSummary is not found in the empty JSON string", ExpandedDailyConsumptionSummary.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("originChargeId") != null && !jsonObj.get("originChargeId").isJsonNull()) && !jsonObj.get("originChargeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `originChargeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("originChargeId").toString()));
}
if ((jsonObj.get("fundId") != null && !jsonObj.get("fundId").isJsonNull()) && !jsonObj.get("fundId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `fundId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("fundId").toString()));
}
if ((jsonObj.get("ratingResultId") != null && !jsonObj.get("ratingResultId").isJsonNull()) && !jsonObj.get("ratingResultId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ratingResultId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ratingResultId").toString()));
}
if ((jsonObj.get("dailyGroupSummaryId") != null && !jsonObj.get("dailyGroupSummaryId").isJsonNull()) && !jsonObj.get("dailyGroupSummaryId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `dailyGroupSummaryId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("dailyGroupSummaryId").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
if ((jsonObj.get("drawdownOriginChargeId") != null && !jsonObj.get("drawdownOriginChargeId").isJsonNull()) && !jsonObj.get("drawdownOriginChargeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `drawdownOriginChargeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("drawdownOriginChargeId").toString()));
}
if ((jsonObj.get("drawdownRecognizedRevenueGlAccountNumber") != null && !jsonObj.get("drawdownRecognizedRevenueGlAccountNumber").isJsonNull()) && !jsonObj.get("drawdownRecognizedRevenueGlAccountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `drawdownRecognizedRevenueGlAccountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("drawdownRecognizedRevenueGlAccountNumber").toString()));
}
if ((jsonObj.get("drawdownAdjustmentRevenueGlAccountNumber") != null && !jsonObj.get("drawdownAdjustmentRevenueGlAccountNumber").isJsonNull()) && !jsonObj.get("drawdownAdjustmentRevenueGlAccountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `drawdownAdjustmentRevenueGlAccountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("drawdownAdjustmentRevenueGlAccountNumber").toString()));
}
if ((jsonObj.get("usageChargeName") != null && !jsonObj.get("usageChargeName").isJsonNull()) && !jsonObj.get("usageChargeName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `usageChargeName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("usageChargeName").toString()));
}
if ((jsonObj.get("usageChargeNumber") != null && !jsonObj.get("usageChargeNumber").isJsonNull()) && !jsonObj.get("usageChargeNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `usageChargeNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("usageChargeNumber").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!ExpandedDailyConsumptionSummary.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'ExpandedDailyConsumptionSummary' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(ExpandedDailyConsumptionSummary.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, ExpandedDailyConsumptionSummary value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public ExpandedDailyConsumptionSummary read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
ExpandedDailyConsumptionSummary instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of ExpandedDailyConsumptionSummary given an JSON string
*
* @param jsonString JSON string
* @return An instance of ExpandedDailyConsumptionSummary
* @throws IOException if the JSON string is invalid with respect to ExpandedDailyConsumptionSummary
*/
public static ExpandedDailyConsumptionSummary fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, ExpandedDailyConsumptionSummary.class);
}
/**
* Convert an instance of ExpandedDailyConsumptionSummary to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy