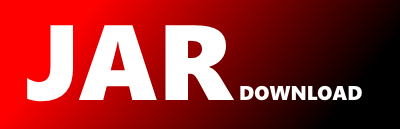
com.zuora.model.ExpandedDebitMemoItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.ExpandedRatePlanCharge;
import com.zuora.model.ExpandedSubscription;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* ExpandedDebitMemoItem
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class ExpandedDebitMemoItem {
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_AMOUNT_WITHOUT_TAX = "amountWithoutTax";
@SerializedName(SERIALIZED_NAME_AMOUNT_WITHOUT_TAX)
private BigDecimal amountWithoutTax;
public static final String SERIALIZED_NAME_APPLIED_TO_ITEM_ID = "appliedToItemId";
@SerializedName(SERIALIZED_NAME_APPLIED_TO_ITEM_ID)
private String appliedToItemId;
public static final String SERIALIZED_NAME_APPLIED_TO_OTHERS_AMOUNT = "appliedToOthersAmount";
@SerializedName(SERIALIZED_NAME_APPLIED_TO_OTHERS_AMOUNT)
private BigDecimal appliedToOthersAmount;
public static final String SERIALIZED_NAME_BE_APPLIED_BY_OTHERS_AMOUNT = "beAppliedByOthersAmount";
@SerializedName(SERIALIZED_NAME_BE_APPLIED_BY_OTHERS_AMOUNT)
private BigDecimal beAppliedByOthersAmount;
public static final String SERIALIZED_NAME_CHARGE_DATE = "chargeDate";
@SerializedName(SERIALIZED_NAME_CHARGE_DATE)
private String chargeDate;
public static final String SERIALIZED_NAME_CREDIT_MEMO_ITEM_ID = "creditMemoItemId";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_ITEM_ID)
private String creditMemoItemId;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING = "excludeItemBillingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBillingFromRevenueAccounting;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_ITEM_SOLD_TO_CONTACT_ID = "itemSoldToContactId";
@SerializedName(SERIALIZED_NAME_ITEM_SOLD_TO_CONTACT_ID)
private String itemSoldToContactId;
public static final String SERIALIZED_NAME_ITEM_SOLD_TO_CONTACT_SNAPSHOT_ID = "itemSoldToContactSnapshotId";
@SerializedName(SERIALIZED_NAME_ITEM_SOLD_TO_CONTACT_SNAPSHOT_ID)
private String itemSoldToContactSnapshotId;
public static final String SERIALIZED_NAME_PROCESSING_TYPE = "processingType";
@SerializedName(SERIALIZED_NAME_PROCESSING_TYPE)
private String processingType;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_SERVICE_END_DATE = "serviceEndDate";
@SerializedName(SERIALIZED_NAME_SERVICE_END_DATE)
private LocalDate serviceEndDate;
public static final String SERIALIZED_NAME_SERVICE_START_DATE = "serviceStartDate";
@SerializedName(SERIALIZED_NAME_SERVICE_START_DATE)
private LocalDate serviceStartDate;
public static final String SERIALIZED_NAME_SKU = "sku";
@SerializedName(SERIALIZED_NAME_SKU)
private String sku;
public static final String SERIALIZED_NAME_SOURCE_ITEM_TYPE = "sourceItemType";
@SerializedName(SERIALIZED_NAME_SOURCE_ITEM_TYPE)
private String sourceItemType;
public static final String SERIALIZED_NAME_CHARGE_NAME = "chargeName";
@SerializedName(SERIALIZED_NAME_CHARGE_NAME)
private String chargeName;
public static final String SERIALIZED_NAME_CHARGE_NUMBER = "chargeNumber";
@SerializedName(SERIALIZED_NAME_CHARGE_NUMBER)
private String chargeNumber;
public static final String SERIALIZED_NAME_TAX_AMOUNT = "taxAmount";
@SerializedName(SERIALIZED_NAME_TAX_AMOUNT)
private BigDecimal taxAmount;
public static final String SERIALIZED_NAME_TAX_CODE_NAME = "taxCodeName";
@SerializedName(SERIALIZED_NAME_TAX_CODE_NAME)
private String taxCodeName;
public static final String SERIALIZED_NAME_TAX_EXEMPT_AMOUNT = "taxExemptAmount";
@SerializedName(SERIALIZED_NAME_TAX_EXEMPT_AMOUNT)
private BigDecimal taxExemptAmount;
public static final String SERIALIZED_NAME_TAX_MODE = "taxMode";
@SerializedName(SERIALIZED_NAME_TAX_MODE)
private String taxMode;
public static final String SERIALIZED_NAME_UNIT_OF_MEASURE = "unitOfMeasure";
@SerializedName(SERIALIZED_NAME_UNIT_OF_MEASURE)
private String unitOfMeasure;
public static final String SERIALIZED_NAME_UNIT_PRICE = "unitPrice";
@SerializedName(SERIALIZED_NAME_UNIT_PRICE)
private BigDecimal unitPrice;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ID = "subscriptionId";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ID)
private String subscriptionId;
public static final String SERIALIZED_NAME_SUBSCRIPTION_NUMBER = "subscriptionNumber";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_NUMBER)
private String subscriptionNumber;
public static final String SERIALIZED_NAME_INVOICE_ITEM_ID = "invoiceItemId";
@SerializedName(SERIALIZED_NAME_INVOICE_ITEM_ID)
private String invoiceItemId;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_ID = "productRatePlanChargeId";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_ID)
private String productRatePlanChargeId;
public static final String SERIALIZED_NAME_RATE_PLAN_CHARGE_ID = "ratePlanChargeId";
@SerializedName(SERIALIZED_NAME_RATE_PLAN_CHARGE_ID)
private String ratePlanChargeId;
public static final String SERIALIZED_NAME_DEBIT_MEMO_ID = "debitMemoId";
@SerializedName(SERIALIZED_NAME_DEBIT_MEMO_ID)
private String debitMemoId;
public static final String SERIALIZED_NAME_BALANCE = "balance";
@SerializedName(SERIALIZED_NAME_BALANCE)
private BigDecimal balance;
public static final String SERIALIZED_NAME_REFLECT_DISCOUNT_IN_NET_AMOUNT = "reflectDiscountInNetAmount";
@SerializedName(SERIALIZED_NAME_REFLECT_DISCOUNT_IN_NET_AMOUNT)
private Boolean reflectDiscountInNetAmount;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public static final String SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE_ID = "recognizedRevenueAccountingCodeId";
@SerializedName(SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE_ID)
private String recognizedRevenueAccountingCodeId;
public static final String SERIALIZED_NAME_ACCOUNT_RECEIVABLE_ACCOUNTING_CODE_ID = "accountReceivableAccountingCodeId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_RECEIVABLE_ACCOUNTING_CODE_ID)
private String accountReceivableAccountingCodeId;
public static final String SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE_ID = "deferredRevenueAccountingCodeId";
@SerializedName(SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE_ID)
private String deferredRevenueAccountingCodeId;
public static final String SERIALIZED_NAME_JOURNAL_ENTRY_ID = "journalEntryId";
@SerializedName(SERIALIZED_NAME_JOURNAL_ENTRY_ID)
private String journalEntryId;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT_SNAPSHOT_ID = "soldToContactSnapshotId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT_SNAPSHOT_ID)
private String soldToContactSnapshotId;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT_SNAPSHOT_ID = "billToContactSnapshotId";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT_SNAPSHOT_ID)
private String billToContactSnapshotId;
public static final String SERIALIZED_NAME_SUBSCRIPTION = "subscription";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION)
private ExpandedSubscription subscription;
public static final String SERIALIZED_NAME_RATE_PLAN_CHARGE = "ratePlanCharge";
@SerializedName(SERIALIZED_NAME_RATE_PLAN_CHARGE)
private ExpandedRatePlanCharge ratePlanCharge;
public ExpandedDebitMemoItem() {
}
public ExpandedDebitMemoItem amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* Get amount
* @return amount
*/
@javax.annotation.Nullable
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public ExpandedDebitMemoItem amountWithoutTax(BigDecimal amountWithoutTax) {
this.amountWithoutTax = amountWithoutTax;
return this;
}
/**
* Get amountWithoutTax
* @return amountWithoutTax
*/
@javax.annotation.Nullable
public BigDecimal getAmountWithoutTax() {
return amountWithoutTax;
}
public void setAmountWithoutTax(BigDecimal amountWithoutTax) {
this.amountWithoutTax = amountWithoutTax;
}
public ExpandedDebitMemoItem appliedToItemId(String appliedToItemId) {
this.appliedToItemId = appliedToItemId;
return this;
}
/**
* Get appliedToItemId
* @return appliedToItemId
*/
@javax.annotation.Nullable
public String getAppliedToItemId() {
return appliedToItemId;
}
public void setAppliedToItemId(String appliedToItemId) {
this.appliedToItemId = appliedToItemId;
}
public ExpandedDebitMemoItem appliedToOthersAmount(BigDecimal appliedToOthersAmount) {
this.appliedToOthersAmount = appliedToOthersAmount;
return this;
}
/**
* Get appliedToOthersAmount
* @return appliedToOthersAmount
*/
@javax.annotation.Nullable
public BigDecimal getAppliedToOthersAmount() {
return appliedToOthersAmount;
}
public void setAppliedToOthersAmount(BigDecimal appliedToOthersAmount) {
this.appliedToOthersAmount = appliedToOthersAmount;
}
public ExpandedDebitMemoItem beAppliedByOthersAmount(BigDecimal beAppliedByOthersAmount) {
this.beAppliedByOthersAmount = beAppliedByOthersAmount;
return this;
}
/**
* Get beAppliedByOthersAmount
* @return beAppliedByOthersAmount
*/
@javax.annotation.Nullable
public BigDecimal getBeAppliedByOthersAmount() {
return beAppliedByOthersAmount;
}
public void setBeAppliedByOthersAmount(BigDecimal beAppliedByOthersAmount) {
this.beAppliedByOthersAmount = beAppliedByOthersAmount;
}
public ExpandedDebitMemoItem chargeDate(String chargeDate) {
this.chargeDate = chargeDate;
return this;
}
/**
* Get chargeDate
* @return chargeDate
*/
@javax.annotation.Nullable
public String getChargeDate() {
return chargeDate;
}
public void setChargeDate(String chargeDate) {
this.chargeDate = chargeDate;
}
public ExpandedDebitMemoItem creditMemoItemId(String creditMemoItemId) {
this.creditMemoItemId = creditMemoItemId;
return this;
}
/**
* Get creditMemoItemId
* @return creditMemoItemId
*/
@javax.annotation.Nullable
public String getCreditMemoItemId() {
return creditMemoItemId;
}
public void setCreditMemoItemId(String creditMemoItemId) {
this.creditMemoItemId = creditMemoItemId;
}
public ExpandedDebitMemoItem excludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
return this;
}
/**
* Get excludeItemBillingFromRevenueAccounting
* @return excludeItemBillingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBillingFromRevenueAccounting() {
return excludeItemBillingFromRevenueAccounting;
}
public void setExcludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
}
public ExpandedDebitMemoItem description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public ExpandedDebitMemoItem itemSoldToContactId(String itemSoldToContactId) {
this.itemSoldToContactId = itemSoldToContactId;
return this;
}
/**
* Get itemSoldToContactId
* @return itemSoldToContactId
*/
@javax.annotation.Nullable
public String getItemSoldToContactId() {
return itemSoldToContactId;
}
public void setItemSoldToContactId(String itemSoldToContactId) {
this.itemSoldToContactId = itemSoldToContactId;
}
public ExpandedDebitMemoItem itemSoldToContactSnapshotId(String itemSoldToContactSnapshotId) {
this.itemSoldToContactSnapshotId = itemSoldToContactSnapshotId;
return this;
}
/**
* Get itemSoldToContactSnapshotId
* @return itemSoldToContactSnapshotId
*/
@javax.annotation.Nullable
public String getItemSoldToContactSnapshotId() {
return itemSoldToContactSnapshotId;
}
public void setItemSoldToContactSnapshotId(String itemSoldToContactSnapshotId) {
this.itemSoldToContactSnapshotId = itemSoldToContactSnapshotId;
}
public ExpandedDebitMemoItem processingType(String processingType) {
this.processingType = processingType;
return this;
}
/**
* Get processingType
* @return processingType
*/
@javax.annotation.Nullable
public String getProcessingType() {
return processingType;
}
public void setProcessingType(String processingType) {
this.processingType = processingType;
}
public ExpandedDebitMemoItem quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* Get quantity
* @return quantity
*/
@javax.annotation.Nullable
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public ExpandedDebitMemoItem serviceEndDate(LocalDate serviceEndDate) {
this.serviceEndDate = serviceEndDate;
return this;
}
/**
* Get serviceEndDate
* @return serviceEndDate
*/
@javax.annotation.Nullable
public LocalDate getServiceEndDate() {
return serviceEndDate;
}
public void setServiceEndDate(LocalDate serviceEndDate) {
this.serviceEndDate = serviceEndDate;
}
public ExpandedDebitMemoItem serviceStartDate(LocalDate serviceStartDate) {
this.serviceStartDate = serviceStartDate;
return this;
}
/**
* Get serviceStartDate
* @return serviceStartDate
*/
@javax.annotation.Nullable
public LocalDate getServiceStartDate() {
return serviceStartDate;
}
public void setServiceStartDate(LocalDate serviceStartDate) {
this.serviceStartDate = serviceStartDate;
}
public ExpandedDebitMemoItem sku(String sku) {
this.sku = sku;
return this;
}
/**
* Get sku
* @return sku
*/
@javax.annotation.Nullable
public String getSku() {
return sku;
}
public void setSku(String sku) {
this.sku = sku;
}
public ExpandedDebitMemoItem sourceItemType(String sourceItemType) {
this.sourceItemType = sourceItemType;
return this;
}
/**
* Get sourceItemType
* @return sourceItemType
*/
@javax.annotation.Nullable
public String getSourceItemType() {
return sourceItemType;
}
public void setSourceItemType(String sourceItemType) {
this.sourceItemType = sourceItemType;
}
public ExpandedDebitMemoItem chargeName(String chargeName) {
this.chargeName = chargeName;
return this;
}
/**
* Get chargeName
* @return chargeName
*/
@javax.annotation.Nullable
public String getChargeName() {
return chargeName;
}
public void setChargeName(String chargeName) {
this.chargeName = chargeName;
}
public ExpandedDebitMemoItem chargeNumber(String chargeNumber) {
this.chargeNumber = chargeNumber;
return this;
}
/**
* Get chargeNumber
* @return chargeNumber
*/
@javax.annotation.Nullable
public String getChargeNumber() {
return chargeNumber;
}
public void setChargeNumber(String chargeNumber) {
this.chargeNumber = chargeNumber;
}
public ExpandedDebitMemoItem taxAmount(BigDecimal taxAmount) {
this.taxAmount = taxAmount;
return this;
}
/**
* Get taxAmount
* @return taxAmount
*/
@javax.annotation.Nullable
public BigDecimal getTaxAmount() {
return taxAmount;
}
public void setTaxAmount(BigDecimal taxAmount) {
this.taxAmount = taxAmount;
}
public ExpandedDebitMemoItem taxCodeName(String taxCodeName) {
this.taxCodeName = taxCodeName;
return this;
}
/**
* Get taxCodeName
* @return taxCodeName
*/
@javax.annotation.Nullable
public String getTaxCodeName() {
return taxCodeName;
}
public void setTaxCodeName(String taxCodeName) {
this.taxCodeName = taxCodeName;
}
public ExpandedDebitMemoItem taxExemptAmount(BigDecimal taxExemptAmount) {
this.taxExemptAmount = taxExemptAmount;
return this;
}
/**
* Get taxExemptAmount
* @return taxExemptAmount
*/
@javax.annotation.Nullable
public BigDecimal getTaxExemptAmount() {
return taxExemptAmount;
}
public void setTaxExemptAmount(BigDecimal taxExemptAmount) {
this.taxExemptAmount = taxExemptAmount;
}
public ExpandedDebitMemoItem taxMode(String taxMode) {
this.taxMode = taxMode;
return this;
}
/**
* Get taxMode
* @return taxMode
*/
@javax.annotation.Nullable
public String getTaxMode() {
return taxMode;
}
public void setTaxMode(String taxMode) {
this.taxMode = taxMode;
}
public ExpandedDebitMemoItem unitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
return this;
}
/**
* Get unitOfMeasure
* @return unitOfMeasure
*/
@javax.annotation.Nullable
public String getUnitOfMeasure() {
return unitOfMeasure;
}
public void setUnitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
}
public ExpandedDebitMemoItem unitPrice(BigDecimal unitPrice) {
this.unitPrice = unitPrice;
return this;
}
/**
* Get unitPrice
* @return unitPrice
*/
@javax.annotation.Nullable
public BigDecimal getUnitPrice() {
return unitPrice;
}
public void setUnitPrice(BigDecimal unitPrice) {
this.unitPrice = unitPrice;
}
public ExpandedDebitMemoItem subscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/**
* Get subscriptionId
* @return subscriptionId
*/
@javax.annotation.Nullable
public String getSubscriptionId() {
return subscriptionId;
}
public void setSubscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
}
public ExpandedDebitMemoItem subscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
return this;
}
/**
* Get subscriptionNumber
* @return subscriptionNumber
*/
@javax.annotation.Nullable
public String getSubscriptionNumber() {
return subscriptionNumber;
}
public void setSubscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
}
public ExpandedDebitMemoItem invoiceItemId(String invoiceItemId) {
this.invoiceItemId = invoiceItemId;
return this;
}
/**
* Get invoiceItemId
* @return invoiceItemId
*/
@javax.annotation.Nullable
public String getInvoiceItemId() {
return invoiceItemId;
}
public void setInvoiceItemId(String invoiceItemId) {
this.invoiceItemId = invoiceItemId;
}
public ExpandedDebitMemoItem productRatePlanChargeId(String productRatePlanChargeId) {
this.productRatePlanChargeId = productRatePlanChargeId;
return this;
}
/**
* Get productRatePlanChargeId
* @return productRatePlanChargeId
*/
@javax.annotation.Nullable
public String getProductRatePlanChargeId() {
return productRatePlanChargeId;
}
public void setProductRatePlanChargeId(String productRatePlanChargeId) {
this.productRatePlanChargeId = productRatePlanChargeId;
}
public ExpandedDebitMemoItem ratePlanChargeId(String ratePlanChargeId) {
this.ratePlanChargeId = ratePlanChargeId;
return this;
}
/**
* Get ratePlanChargeId
* @return ratePlanChargeId
*/
@javax.annotation.Nullable
public String getRatePlanChargeId() {
return ratePlanChargeId;
}
public void setRatePlanChargeId(String ratePlanChargeId) {
this.ratePlanChargeId = ratePlanChargeId;
}
public ExpandedDebitMemoItem debitMemoId(String debitMemoId) {
this.debitMemoId = debitMemoId;
return this;
}
/**
* Get debitMemoId
* @return debitMemoId
*/
@javax.annotation.Nullable
public String getDebitMemoId() {
return debitMemoId;
}
public void setDebitMemoId(String debitMemoId) {
this.debitMemoId = debitMemoId;
}
public ExpandedDebitMemoItem balance(BigDecimal balance) {
this.balance = balance;
return this;
}
/**
* Get balance
* @return balance
*/
@javax.annotation.Nullable
public BigDecimal getBalance() {
return balance;
}
public void setBalance(BigDecimal balance) {
this.balance = balance;
}
public ExpandedDebitMemoItem reflectDiscountInNetAmount(Boolean reflectDiscountInNetAmount) {
this.reflectDiscountInNetAmount = reflectDiscountInNetAmount;
return this;
}
/**
* Get reflectDiscountInNetAmount
* @return reflectDiscountInNetAmount
*/
@javax.annotation.Nullable
public Boolean getReflectDiscountInNetAmount() {
return reflectDiscountInNetAmount;
}
public void setReflectDiscountInNetAmount(Boolean reflectDiscountInNetAmount) {
this.reflectDiscountInNetAmount = reflectDiscountInNetAmount;
}
public ExpandedDebitMemoItem id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public ExpandedDebitMemoItem createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* Get createdById
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public ExpandedDebitMemoItem createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* Get createdDate
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public ExpandedDebitMemoItem updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* Get updatedById
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public ExpandedDebitMemoItem updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* Get updatedDate
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
public ExpandedDebitMemoItem recognizedRevenueAccountingCodeId(String recognizedRevenueAccountingCodeId) {
this.recognizedRevenueAccountingCodeId = recognizedRevenueAccountingCodeId;
return this;
}
/**
* Get recognizedRevenueAccountingCodeId
* @return recognizedRevenueAccountingCodeId
*/
@javax.annotation.Nullable
public String getRecognizedRevenueAccountingCodeId() {
return recognizedRevenueAccountingCodeId;
}
public void setRecognizedRevenueAccountingCodeId(String recognizedRevenueAccountingCodeId) {
this.recognizedRevenueAccountingCodeId = recognizedRevenueAccountingCodeId;
}
public ExpandedDebitMemoItem accountReceivableAccountingCodeId(String accountReceivableAccountingCodeId) {
this.accountReceivableAccountingCodeId = accountReceivableAccountingCodeId;
return this;
}
/**
* Get accountReceivableAccountingCodeId
* @return accountReceivableAccountingCodeId
*/
@javax.annotation.Nullable
public String getAccountReceivableAccountingCodeId() {
return accountReceivableAccountingCodeId;
}
public void setAccountReceivableAccountingCodeId(String accountReceivableAccountingCodeId) {
this.accountReceivableAccountingCodeId = accountReceivableAccountingCodeId;
}
public ExpandedDebitMemoItem deferredRevenueAccountingCodeId(String deferredRevenueAccountingCodeId) {
this.deferredRevenueAccountingCodeId = deferredRevenueAccountingCodeId;
return this;
}
/**
* Get deferredRevenueAccountingCodeId
* @return deferredRevenueAccountingCodeId
*/
@javax.annotation.Nullable
public String getDeferredRevenueAccountingCodeId() {
return deferredRevenueAccountingCodeId;
}
public void setDeferredRevenueAccountingCodeId(String deferredRevenueAccountingCodeId) {
this.deferredRevenueAccountingCodeId = deferredRevenueAccountingCodeId;
}
public ExpandedDebitMemoItem journalEntryId(String journalEntryId) {
this.journalEntryId = journalEntryId;
return this;
}
/**
* Get journalEntryId
* @return journalEntryId
*/
@javax.annotation.Nullable
public String getJournalEntryId() {
return journalEntryId;
}
public void setJournalEntryId(String journalEntryId) {
this.journalEntryId = journalEntryId;
}
public ExpandedDebitMemoItem soldToContactSnapshotId(String soldToContactSnapshotId) {
this.soldToContactSnapshotId = soldToContactSnapshotId;
return this;
}
/**
* Get soldToContactSnapshotId
* @return soldToContactSnapshotId
*/
@javax.annotation.Nullable
public String getSoldToContactSnapshotId() {
return soldToContactSnapshotId;
}
public void setSoldToContactSnapshotId(String soldToContactSnapshotId) {
this.soldToContactSnapshotId = soldToContactSnapshotId;
}
public ExpandedDebitMemoItem billToContactSnapshotId(String billToContactSnapshotId) {
this.billToContactSnapshotId = billToContactSnapshotId;
return this;
}
/**
* Get billToContactSnapshotId
* @return billToContactSnapshotId
*/
@javax.annotation.Nullable
public String getBillToContactSnapshotId() {
return billToContactSnapshotId;
}
public void setBillToContactSnapshotId(String billToContactSnapshotId) {
this.billToContactSnapshotId = billToContactSnapshotId;
}
public ExpandedDebitMemoItem subscription(ExpandedSubscription subscription) {
this.subscription = subscription;
return this;
}
/**
* Get subscription
* @return subscription
*/
@javax.annotation.Nullable
public ExpandedSubscription getSubscription() {
return subscription;
}
public void setSubscription(ExpandedSubscription subscription) {
this.subscription = subscription;
}
public ExpandedDebitMemoItem ratePlanCharge(ExpandedRatePlanCharge ratePlanCharge) {
this.ratePlanCharge = ratePlanCharge;
return this;
}
/**
* Get ratePlanCharge
* @return ratePlanCharge
*/
@javax.annotation.Nullable
public ExpandedRatePlanCharge getRatePlanCharge() {
return ratePlanCharge;
}
public void setRatePlanCharge(ExpandedRatePlanCharge ratePlanCharge) {
this.ratePlanCharge = ratePlanCharge;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the ExpandedDebitMemoItem instance itself
*/
public ExpandedDebitMemoItem putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ExpandedDebitMemoItem expandedDebitMemoItem = (ExpandedDebitMemoItem) o;
return Objects.equals(this.amount, expandedDebitMemoItem.amount) &&
Objects.equals(this.amountWithoutTax, expandedDebitMemoItem.amountWithoutTax) &&
Objects.equals(this.appliedToItemId, expandedDebitMemoItem.appliedToItemId) &&
Objects.equals(this.appliedToOthersAmount, expandedDebitMemoItem.appliedToOthersAmount) &&
Objects.equals(this.beAppliedByOthersAmount, expandedDebitMemoItem.beAppliedByOthersAmount) &&
Objects.equals(this.chargeDate, expandedDebitMemoItem.chargeDate) &&
Objects.equals(this.creditMemoItemId, expandedDebitMemoItem.creditMemoItemId) &&
Objects.equals(this.excludeItemBillingFromRevenueAccounting, expandedDebitMemoItem.excludeItemBillingFromRevenueAccounting) &&
Objects.equals(this.description, expandedDebitMemoItem.description) &&
Objects.equals(this.itemSoldToContactId, expandedDebitMemoItem.itemSoldToContactId) &&
Objects.equals(this.itemSoldToContactSnapshotId, expandedDebitMemoItem.itemSoldToContactSnapshotId) &&
Objects.equals(this.processingType, expandedDebitMemoItem.processingType) &&
Objects.equals(this.quantity, expandedDebitMemoItem.quantity) &&
Objects.equals(this.serviceEndDate, expandedDebitMemoItem.serviceEndDate) &&
Objects.equals(this.serviceStartDate, expandedDebitMemoItem.serviceStartDate) &&
Objects.equals(this.sku, expandedDebitMemoItem.sku) &&
Objects.equals(this.sourceItemType, expandedDebitMemoItem.sourceItemType) &&
Objects.equals(this.chargeName, expandedDebitMemoItem.chargeName) &&
Objects.equals(this.chargeNumber, expandedDebitMemoItem.chargeNumber) &&
Objects.equals(this.taxAmount, expandedDebitMemoItem.taxAmount) &&
Objects.equals(this.taxCodeName, expandedDebitMemoItem.taxCodeName) &&
Objects.equals(this.taxExemptAmount, expandedDebitMemoItem.taxExemptAmount) &&
Objects.equals(this.taxMode, expandedDebitMemoItem.taxMode) &&
Objects.equals(this.unitOfMeasure, expandedDebitMemoItem.unitOfMeasure) &&
Objects.equals(this.unitPrice, expandedDebitMemoItem.unitPrice) &&
Objects.equals(this.subscriptionId, expandedDebitMemoItem.subscriptionId) &&
Objects.equals(this.subscriptionNumber, expandedDebitMemoItem.subscriptionNumber) &&
Objects.equals(this.invoiceItemId, expandedDebitMemoItem.invoiceItemId) &&
Objects.equals(this.productRatePlanChargeId, expandedDebitMemoItem.productRatePlanChargeId) &&
Objects.equals(this.ratePlanChargeId, expandedDebitMemoItem.ratePlanChargeId) &&
Objects.equals(this.debitMemoId, expandedDebitMemoItem.debitMemoId) &&
Objects.equals(this.balance, expandedDebitMemoItem.balance) &&
Objects.equals(this.reflectDiscountInNetAmount, expandedDebitMemoItem.reflectDiscountInNetAmount) &&
Objects.equals(this.id, expandedDebitMemoItem.id) &&
Objects.equals(this.createdById, expandedDebitMemoItem.createdById) &&
Objects.equals(this.createdDate, expandedDebitMemoItem.createdDate) &&
Objects.equals(this.updatedById, expandedDebitMemoItem.updatedById) &&
Objects.equals(this.updatedDate, expandedDebitMemoItem.updatedDate) &&
Objects.equals(this.recognizedRevenueAccountingCodeId, expandedDebitMemoItem.recognizedRevenueAccountingCodeId) &&
Objects.equals(this.accountReceivableAccountingCodeId, expandedDebitMemoItem.accountReceivableAccountingCodeId) &&
Objects.equals(this.deferredRevenueAccountingCodeId, expandedDebitMemoItem.deferredRevenueAccountingCodeId) &&
Objects.equals(this.journalEntryId, expandedDebitMemoItem.journalEntryId) &&
Objects.equals(this.soldToContactSnapshotId, expandedDebitMemoItem.soldToContactSnapshotId) &&
Objects.equals(this.billToContactSnapshotId, expandedDebitMemoItem.billToContactSnapshotId) &&
Objects.equals(this.subscription, expandedDebitMemoItem.subscription) &&
Objects.equals(this.ratePlanCharge, expandedDebitMemoItem.ratePlanCharge)&&
Objects.equals(this.additionalProperties, expandedDebitMemoItem.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(amount, amountWithoutTax, appliedToItemId, appliedToOthersAmount, beAppliedByOthersAmount, chargeDate, creditMemoItemId, excludeItemBillingFromRevenueAccounting, description, itemSoldToContactId, itemSoldToContactSnapshotId, processingType, quantity, serviceEndDate, serviceStartDate, sku, sourceItemType, chargeName, chargeNumber, taxAmount, taxCodeName, taxExemptAmount, taxMode, unitOfMeasure, unitPrice, subscriptionId, subscriptionNumber, invoiceItemId, productRatePlanChargeId, ratePlanChargeId, debitMemoId, balance, reflectDiscountInNetAmount, id, createdById, createdDate, updatedById, updatedDate, recognizedRevenueAccountingCodeId, accountReceivableAccountingCodeId, deferredRevenueAccountingCodeId, journalEntryId, soldToContactSnapshotId, billToContactSnapshotId, subscription, ratePlanCharge, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ExpandedDebitMemoItem {\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" amountWithoutTax: ").append(toIndentedString(amountWithoutTax)).append("\n");
sb.append(" appliedToItemId: ").append(toIndentedString(appliedToItemId)).append("\n");
sb.append(" appliedToOthersAmount: ").append(toIndentedString(appliedToOthersAmount)).append("\n");
sb.append(" beAppliedByOthersAmount: ").append(toIndentedString(beAppliedByOthersAmount)).append("\n");
sb.append(" chargeDate: ").append(toIndentedString(chargeDate)).append("\n");
sb.append(" creditMemoItemId: ").append(toIndentedString(creditMemoItemId)).append("\n");
sb.append(" excludeItemBillingFromRevenueAccounting: ").append(toIndentedString(excludeItemBillingFromRevenueAccounting)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" itemSoldToContactId: ").append(toIndentedString(itemSoldToContactId)).append("\n");
sb.append(" itemSoldToContactSnapshotId: ").append(toIndentedString(itemSoldToContactSnapshotId)).append("\n");
sb.append(" processingType: ").append(toIndentedString(processingType)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" serviceEndDate: ").append(toIndentedString(serviceEndDate)).append("\n");
sb.append(" serviceStartDate: ").append(toIndentedString(serviceStartDate)).append("\n");
sb.append(" sku: ").append(toIndentedString(sku)).append("\n");
sb.append(" sourceItemType: ").append(toIndentedString(sourceItemType)).append("\n");
sb.append(" chargeName: ").append(toIndentedString(chargeName)).append("\n");
sb.append(" chargeNumber: ").append(toIndentedString(chargeNumber)).append("\n");
sb.append(" taxAmount: ").append(toIndentedString(taxAmount)).append("\n");
sb.append(" taxCodeName: ").append(toIndentedString(taxCodeName)).append("\n");
sb.append(" taxExemptAmount: ").append(toIndentedString(taxExemptAmount)).append("\n");
sb.append(" taxMode: ").append(toIndentedString(taxMode)).append("\n");
sb.append(" unitOfMeasure: ").append(toIndentedString(unitOfMeasure)).append("\n");
sb.append(" unitPrice: ").append(toIndentedString(unitPrice)).append("\n");
sb.append(" subscriptionId: ").append(toIndentedString(subscriptionId)).append("\n");
sb.append(" subscriptionNumber: ").append(toIndentedString(subscriptionNumber)).append("\n");
sb.append(" invoiceItemId: ").append(toIndentedString(invoiceItemId)).append("\n");
sb.append(" productRatePlanChargeId: ").append(toIndentedString(productRatePlanChargeId)).append("\n");
sb.append(" ratePlanChargeId: ").append(toIndentedString(ratePlanChargeId)).append("\n");
sb.append(" debitMemoId: ").append(toIndentedString(debitMemoId)).append("\n");
sb.append(" balance: ").append(toIndentedString(balance)).append("\n");
sb.append(" reflectDiscountInNetAmount: ").append(toIndentedString(reflectDiscountInNetAmount)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" recognizedRevenueAccountingCodeId: ").append(toIndentedString(recognizedRevenueAccountingCodeId)).append("\n");
sb.append(" accountReceivableAccountingCodeId: ").append(toIndentedString(accountReceivableAccountingCodeId)).append("\n");
sb.append(" deferredRevenueAccountingCodeId: ").append(toIndentedString(deferredRevenueAccountingCodeId)).append("\n");
sb.append(" journalEntryId: ").append(toIndentedString(journalEntryId)).append("\n");
sb.append(" soldToContactSnapshotId: ").append(toIndentedString(soldToContactSnapshotId)).append("\n");
sb.append(" billToContactSnapshotId: ").append(toIndentedString(billToContactSnapshotId)).append("\n");
sb.append(" subscription: ").append(toIndentedString(subscription)).append("\n");
sb.append(" ratePlanCharge: ").append(toIndentedString(ratePlanCharge)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("amount");
openapiFields.add("amountWithoutTax");
openapiFields.add("appliedToItemId");
openapiFields.add("appliedToOthersAmount");
openapiFields.add("beAppliedByOthersAmount");
openapiFields.add("chargeDate");
openapiFields.add("creditMemoItemId");
openapiFields.add("excludeItemBillingFromRevenueAccounting");
openapiFields.add("description");
openapiFields.add("itemSoldToContactId");
openapiFields.add("itemSoldToContactSnapshotId");
openapiFields.add("processingType");
openapiFields.add("quantity");
openapiFields.add("serviceEndDate");
openapiFields.add("serviceStartDate");
openapiFields.add("sku");
openapiFields.add("sourceItemType");
openapiFields.add("chargeName");
openapiFields.add("chargeNumber");
openapiFields.add("taxAmount");
openapiFields.add("taxCodeName");
openapiFields.add("taxExemptAmount");
openapiFields.add("taxMode");
openapiFields.add("unitOfMeasure");
openapiFields.add("unitPrice");
openapiFields.add("subscriptionId");
openapiFields.add("subscriptionNumber");
openapiFields.add("invoiceItemId");
openapiFields.add("productRatePlanChargeId");
openapiFields.add("ratePlanChargeId");
openapiFields.add("debitMemoId");
openapiFields.add("balance");
openapiFields.add("reflectDiscountInNetAmount");
openapiFields.add("id");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
openapiFields.add("recognizedRevenueAccountingCodeId");
openapiFields.add("accountReceivableAccountingCodeId");
openapiFields.add("deferredRevenueAccountingCodeId");
openapiFields.add("journalEntryId");
openapiFields.add("soldToContactSnapshotId");
openapiFields.add("billToContactSnapshotId");
openapiFields.add("subscription");
openapiFields.add("ratePlanCharge");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to ExpandedDebitMemoItem
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!ExpandedDebitMemoItem.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in ExpandedDebitMemoItem is not found in the empty JSON string", ExpandedDebitMemoItem.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("appliedToItemId") != null && !jsonObj.get("appliedToItemId").isJsonNull()) && !jsonObj.get("appliedToItemId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `appliedToItemId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("appliedToItemId").toString()));
}
if ((jsonObj.get("chargeDate") != null && !jsonObj.get("chargeDate").isJsonNull()) && !jsonObj.get("chargeDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeDate").toString()));
}
if ((jsonObj.get("creditMemoItemId") != null && !jsonObj.get("creditMemoItemId").isJsonNull()) && !jsonObj.get("creditMemoItemId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creditMemoItemId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creditMemoItemId").toString()));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if ((jsonObj.get("itemSoldToContactId") != null && !jsonObj.get("itemSoldToContactId").isJsonNull()) && !jsonObj.get("itemSoldToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `itemSoldToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("itemSoldToContactId").toString()));
}
if ((jsonObj.get("itemSoldToContactSnapshotId") != null && !jsonObj.get("itemSoldToContactSnapshotId").isJsonNull()) && !jsonObj.get("itemSoldToContactSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `itemSoldToContactSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("itemSoldToContactSnapshotId").toString()));
}
if ((jsonObj.get("processingType") != null && !jsonObj.get("processingType").isJsonNull()) && !jsonObj.get("processingType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `processingType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("processingType").toString()));
}
if ((jsonObj.get("sku") != null && !jsonObj.get("sku").isJsonNull()) && !jsonObj.get("sku").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sku` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sku").toString()));
}
if ((jsonObj.get("sourceItemType") != null && !jsonObj.get("sourceItemType").isJsonNull()) && !jsonObj.get("sourceItemType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sourceItemType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sourceItemType").toString()));
}
if ((jsonObj.get("chargeName") != null && !jsonObj.get("chargeName").isJsonNull()) && !jsonObj.get("chargeName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeName").toString()));
}
if ((jsonObj.get("chargeNumber") != null && !jsonObj.get("chargeNumber").isJsonNull()) && !jsonObj.get("chargeNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeNumber").toString()));
}
if ((jsonObj.get("taxCodeName") != null && !jsonObj.get("taxCodeName").isJsonNull()) && !jsonObj.get("taxCodeName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxCodeName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxCodeName").toString()));
}
if ((jsonObj.get("taxMode") != null && !jsonObj.get("taxMode").isJsonNull()) && !jsonObj.get("taxMode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxMode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxMode").toString()));
}
if ((jsonObj.get("unitOfMeasure") != null && !jsonObj.get("unitOfMeasure").isJsonNull()) && !jsonObj.get("unitOfMeasure").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `unitOfMeasure` to be a primitive type in the JSON string but got `%s`", jsonObj.get("unitOfMeasure").toString()));
}
if ((jsonObj.get("subscriptionId") != null && !jsonObj.get("subscriptionId").isJsonNull()) && !jsonObj.get("subscriptionId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("subscriptionId").toString()));
}
if ((jsonObj.get("subscriptionNumber") != null && !jsonObj.get("subscriptionNumber").isJsonNull()) && !jsonObj.get("subscriptionNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("subscriptionNumber").toString()));
}
if ((jsonObj.get("invoiceItemId") != null && !jsonObj.get("invoiceItemId").isJsonNull()) && !jsonObj.get("invoiceItemId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceItemId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceItemId").toString()));
}
if ((jsonObj.get("productRatePlanChargeId") != null && !jsonObj.get("productRatePlanChargeId").isJsonNull()) && !jsonObj.get("productRatePlanChargeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanChargeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productRatePlanChargeId").toString()));
}
if ((jsonObj.get("ratePlanChargeId") != null && !jsonObj.get("ratePlanChargeId").isJsonNull()) && !jsonObj.get("ratePlanChargeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ratePlanChargeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ratePlanChargeId").toString()));
}
if ((jsonObj.get("debitMemoId") != null && !jsonObj.get("debitMemoId").isJsonNull()) && !jsonObj.get("debitMemoId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `debitMemoId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("debitMemoId").toString()));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
if ((jsonObj.get("recognizedRevenueAccountingCodeId") != null && !jsonObj.get("recognizedRevenueAccountingCodeId").isJsonNull()) && !jsonObj.get("recognizedRevenueAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `recognizedRevenueAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("recognizedRevenueAccountingCodeId").toString()));
}
if ((jsonObj.get("accountReceivableAccountingCodeId") != null && !jsonObj.get("accountReceivableAccountingCodeId").isJsonNull()) && !jsonObj.get("accountReceivableAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountReceivableAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountReceivableAccountingCodeId").toString()));
}
if ((jsonObj.get("deferredRevenueAccountingCodeId") != null && !jsonObj.get("deferredRevenueAccountingCodeId").isJsonNull()) && !jsonObj.get("deferredRevenueAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `deferredRevenueAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("deferredRevenueAccountingCodeId").toString()));
}
if ((jsonObj.get("journalEntryId") != null && !jsonObj.get("journalEntryId").isJsonNull()) && !jsonObj.get("journalEntryId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `journalEntryId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("journalEntryId").toString()));
}
if ((jsonObj.get("soldToContactSnapshotId") != null && !jsonObj.get("soldToContactSnapshotId").isJsonNull()) && !jsonObj.get("soldToContactSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToContactSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToContactSnapshotId").toString()));
}
if ((jsonObj.get("billToContactSnapshotId") != null && !jsonObj.get("billToContactSnapshotId").isJsonNull()) && !jsonObj.get("billToContactSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billToContactSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billToContactSnapshotId").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!ExpandedDebitMemoItem.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'ExpandedDebitMemoItem' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(ExpandedDebitMemoItem.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, ExpandedDebitMemoItem value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public ExpandedDebitMemoItem read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
ExpandedDebitMemoItem instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of ExpandedDebitMemoItem given an JSON string
*
* @param jsonString JSON string
* @return An instance of ExpandedDebitMemoItem
* @throws IOException if the JSON string is invalid with respect to ExpandedDebitMemoItem
*/
public static ExpandedDebitMemoItem fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, ExpandedDebitMemoItem.class);
}
/**
* Convert an instance of ExpandedDebitMemoItem to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy