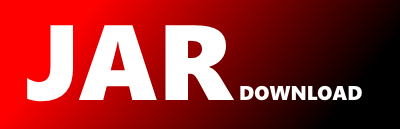
com.zuora.model.ExpandedInvoice Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.ExpandedAccount;
import com.zuora.model.ExpandedContact;
import com.zuora.model.ExpandedInvoiceItem;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* ExpandedInvoice
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class ExpandedInvoice {
public static final String SERIALIZED_NAME_ACCOUNT_ID = "accountId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ADJUSTMENT_AMOUNT = "adjustmentAmount";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_AMOUNT)
private BigDecimal adjustmentAmount;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_AMOUNT_WITHOUT_TAX = "amountWithoutTax";
@SerializedName(SERIALIZED_NAME_AMOUNT_WITHOUT_TAX)
private BigDecimal amountWithoutTax;
public static final String SERIALIZED_NAME_AUTO_PAY = "autoPay";
@SerializedName(SERIALIZED_NAME_AUTO_PAY)
private Boolean autoPay;
public static final String SERIALIZED_NAME_BALANCE = "balance";
@SerializedName(SERIALIZED_NAME_BALANCE)
private BigDecimal balance;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT_ID = "billToContactId";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT_ID)
private String billToContactId;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT_SNAPSHOT_ID = "billToContactSnapshotId";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT_SNAPSHOT_ID)
private String billToContactSnapshotId;
public static final String SERIALIZED_NAME_COMMENTS = "comments";
@SerializedName(SERIALIZED_NAME_COMMENTS)
private String comments;
public static final String SERIALIZED_NAME_CREDIT_BALANCE_ADJUSTMENT_AMOUNT = "creditBalanceAdjustmentAmount";
@SerializedName(SERIALIZED_NAME_CREDIT_BALANCE_ADJUSTMENT_AMOUNT)
private BigDecimal creditBalanceAdjustmentAmount;
public static final String SERIALIZED_NAME_CREDIT_MEMO_AMOUNT = "creditMemoAmount";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_AMOUNT)
private BigDecimal creditMemoAmount;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_DUE_DATE = "dueDate";
@SerializedName(SERIALIZED_NAME_DUE_DATE)
private LocalDate dueDate;
public static final String SERIALIZED_NAME_INCLUDES_ONE_TIME = "includesOneTime";
@SerializedName(SERIALIZED_NAME_INCLUDES_ONE_TIME)
private Boolean includesOneTime;
public static final String SERIALIZED_NAME_INCLUDES_RECURRING = "includesRecurring";
@SerializedName(SERIALIZED_NAME_INCLUDES_RECURRING)
private Boolean includesRecurring;
public static final String SERIALIZED_NAME_INCLUDES_USAGE = "includesUsage";
@SerializedName(SERIALIZED_NAME_INCLUDES_USAGE)
private Boolean includesUsage;
public static final String SERIALIZED_NAME_INVOICE_DATE = "invoiceDate";
@SerializedName(SERIALIZED_NAME_INVOICE_DATE)
private LocalDate invoiceDate;
public static final String SERIALIZED_NAME_INVOICE_GROUP_NUMBER = "invoiceGroupNumber";
@SerializedName(SERIALIZED_NAME_INVOICE_GROUP_NUMBER)
private String invoiceGroupNumber;
public static final String SERIALIZED_NAME_INVOICE_NUMBER = "invoiceNumber";
@SerializedName(SERIALIZED_NAME_INVOICE_NUMBER)
private String invoiceNumber;
public static final String SERIALIZED_NAME_LAST_EMAIL_SENT_DATE = "lastEmailSentDate";
@SerializedName(SERIALIZED_NAME_LAST_EMAIL_SENT_DATE)
private String lastEmailSentDate;
public static final String SERIALIZED_NAME_ORGANIZATION_ID = "organizationId";
@SerializedName(SERIALIZED_NAME_ORGANIZATION_ID)
private String organizationId;
public static final String SERIALIZED_NAME_PAYMENT_AMOUNT = "paymentAmount";
@SerializedName(SERIALIZED_NAME_PAYMENT_AMOUNT)
private BigDecimal paymentAmount;
public static final String SERIALIZED_NAME_POSTED_BY = "postedBy";
@SerializedName(SERIALIZED_NAME_POSTED_BY)
private String postedBy;
public static final String SERIALIZED_NAME_POSTED_DATE = "postedDate";
@SerializedName(SERIALIZED_NAME_POSTED_DATE)
private String postedDate;
public static final String SERIALIZED_NAME_REFUND_AMOUNT = "refundAmount";
@SerializedName(SERIALIZED_NAME_REFUND_AMOUNT)
private BigDecimal refundAmount;
public static final String SERIALIZED_NAME_REVERSED = "reversed";
@SerializedName(SERIALIZED_NAME_REVERSED)
private Boolean reversed;
public static final String SERIALIZED_NAME_SEQUENCE_SET_ID = "sequenceSetId";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_ID)
private String sequenceSetId;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT_ID = "soldToContactId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT_ID)
private String soldToContactId;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT_SNAPSHOT_ID = "soldToContactSnapshotId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT_SNAPSHOT_ID)
private String soldToContactSnapshotId;
public static final String SERIALIZED_NAME_SOURCE = "source";
@SerializedName(SERIALIZED_NAME_SOURCE)
private String source;
public static final String SERIALIZED_NAME_SOURCE_ID = "sourceId";
@SerializedName(SERIALIZED_NAME_SOURCE_ID)
private String sourceId;
public static final String SERIALIZED_NAME_SOURCE_TYPE = "sourceType";
@SerializedName(SERIALIZED_NAME_SOURCE_TYPE)
private String sourceType;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private String status;
public static final String SERIALIZED_NAME_TARGET_DATE = "targetDate";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public static final String SERIALIZED_NAME_TAX_AMOUNT = "taxAmount";
@SerializedName(SERIALIZED_NAME_TAX_AMOUNT)
private BigDecimal taxAmount;
public static final String SERIALIZED_NAME_TAX_EXEMPT_AMOUNT = "taxExemptAmount";
@SerializedName(SERIALIZED_NAME_TAX_EXEMPT_AMOUNT)
private BigDecimal taxExemptAmount;
public static final String SERIALIZED_NAME_TAX_STATUS = "taxStatus";
@SerializedName(SERIALIZED_NAME_TAX_STATUS)
private String taxStatus;
public static final String SERIALIZED_NAME_TAX_MESSAGE = "taxMessage";
@SerializedName(SERIALIZED_NAME_TAX_MESSAGE)
private String taxMessage;
public static final String SERIALIZED_NAME_TEMPLATE_ID = "templateId";
@SerializedName(SERIALIZED_NAME_TEMPLATE_ID)
private String templateId;
public static final String SERIALIZED_NAME_TRANSFERRED_TO_ACCOUNTING = "transferredToAccounting";
@SerializedName(SERIALIZED_NAME_TRANSFERRED_TO_ACCOUNTING)
private String transferredToAccounting;
public static final String SERIALIZED_NAME_E_INVOICE_STATUS = "eInvoiceStatus";
@SerializedName(SERIALIZED_NAME_E_INVOICE_STATUS)
private String eInvoiceStatus;
public static final String SERIALIZED_NAME_E_INVOICE_FILE_ID = "eInvoiceFileId";
@SerializedName(SERIALIZED_NAME_E_INVOICE_FILE_ID)
private String eInvoiceFileId;
public static final String SERIALIZED_NAME_E_INVOICE_ERROR_CODE = "eInvoiceErrorCode";
@SerializedName(SERIALIZED_NAME_E_INVOICE_ERROR_CODE)
private String eInvoiceErrorCode;
public static final String SERIALIZED_NAME_E_INVOICE_ERROR_MESSAGE = "eInvoiceErrorMessage";
@SerializedName(SERIALIZED_NAME_E_INVOICE_ERROR_MESSAGE)
private String eInvoiceErrorMessage;
public static final String SERIALIZED_NAME_PAYMENT_LINK = "paymentLink";
@SerializedName(SERIALIZED_NAME_PAYMENT_LINK)
private String paymentLink;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public static final String SERIALIZED_NAME_PAYMENT_TERM = "paymentTerm";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERM)
private String paymentTerm;
public static final String SERIALIZED_NAME_ACCOUNT = "account";
@SerializedName(SERIALIZED_NAME_ACCOUNT)
private ExpandedAccount account;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT = "billToContact";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT)
private ExpandedContact billToContact;
public static final String SERIALIZED_NAME_INVOICE_ITEMS = "invoiceItems";
@SerializedName(SERIALIZED_NAME_INVOICE_ITEMS)
private List invoiceItems;
public ExpandedInvoice() {
}
public ExpandedInvoice accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Get accountId
* @return accountId
*/
@javax.annotation.Nullable
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public ExpandedInvoice adjustmentAmount(BigDecimal adjustmentAmount) {
this.adjustmentAmount = adjustmentAmount;
return this;
}
/**
* Get adjustmentAmount
* @return adjustmentAmount
*/
@javax.annotation.Nullable
public BigDecimal getAdjustmentAmount() {
return adjustmentAmount;
}
public void setAdjustmentAmount(BigDecimal adjustmentAmount) {
this.adjustmentAmount = adjustmentAmount;
}
public ExpandedInvoice amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* Get amount
* @return amount
*/
@javax.annotation.Nullable
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public ExpandedInvoice amountWithoutTax(BigDecimal amountWithoutTax) {
this.amountWithoutTax = amountWithoutTax;
return this;
}
/**
* Get amountWithoutTax
* @return amountWithoutTax
*/
@javax.annotation.Nullable
public BigDecimal getAmountWithoutTax() {
return amountWithoutTax;
}
public void setAmountWithoutTax(BigDecimal amountWithoutTax) {
this.amountWithoutTax = amountWithoutTax;
}
public ExpandedInvoice autoPay(Boolean autoPay) {
this.autoPay = autoPay;
return this;
}
/**
* Get autoPay
* @return autoPay
*/
@javax.annotation.Nullable
public Boolean getAutoPay() {
return autoPay;
}
public void setAutoPay(Boolean autoPay) {
this.autoPay = autoPay;
}
public ExpandedInvoice balance(BigDecimal balance) {
this.balance = balance;
return this;
}
/**
* Get balance
* @return balance
*/
@javax.annotation.Nullable
public BigDecimal getBalance() {
return balance;
}
public void setBalance(BigDecimal balance) {
this.balance = balance;
}
public ExpandedInvoice billToContactId(String billToContactId) {
this.billToContactId = billToContactId;
return this;
}
/**
* Get billToContactId
* @return billToContactId
*/
@javax.annotation.Nullable
public String getBillToContactId() {
return billToContactId;
}
public void setBillToContactId(String billToContactId) {
this.billToContactId = billToContactId;
}
public ExpandedInvoice billToContactSnapshotId(String billToContactSnapshotId) {
this.billToContactSnapshotId = billToContactSnapshotId;
return this;
}
/**
* Get billToContactSnapshotId
* @return billToContactSnapshotId
*/
@javax.annotation.Nullable
public String getBillToContactSnapshotId() {
return billToContactSnapshotId;
}
public void setBillToContactSnapshotId(String billToContactSnapshotId) {
this.billToContactSnapshotId = billToContactSnapshotId;
}
public ExpandedInvoice comments(String comments) {
this.comments = comments;
return this;
}
/**
* Get comments
* @return comments
*/
@javax.annotation.Nullable
public String getComments() {
return comments;
}
public void setComments(String comments) {
this.comments = comments;
}
public ExpandedInvoice creditBalanceAdjustmentAmount(BigDecimal creditBalanceAdjustmentAmount) {
this.creditBalanceAdjustmentAmount = creditBalanceAdjustmentAmount;
return this;
}
/**
* Get creditBalanceAdjustmentAmount
* @return creditBalanceAdjustmentAmount
*/
@javax.annotation.Nullable
public BigDecimal getCreditBalanceAdjustmentAmount() {
return creditBalanceAdjustmentAmount;
}
public void setCreditBalanceAdjustmentAmount(BigDecimal creditBalanceAdjustmentAmount) {
this.creditBalanceAdjustmentAmount = creditBalanceAdjustmentAmount;
}
public ExpandedInvoice creditMemoAmount(BigDecimal creditMemoAmount) {
this.creditMemoAmount = creditMemoAmount;
return this;
}
/**
* Get creditMemoAmount
* @return creditMemoAmount
*/
@javax.annotation.Nullable
public BigDecimal getCreditMemoAmount() {
return creditMemoAmount;
}
public void setCreditMemoAmount(BigDecimal creditMemoAmount) {
this.creditMemoAmount = creditMemoAmount;
}
public ExpandedInvoice currency(String currency) {
this.currency = currency;
return this;
}
/**
* Get currency
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public ExpandedInvoice dueDate(LocalDate dueDate) {
this.dueDate = dueDate;
return this;
}
/**
* Get dueDate
* @return dueDate
*/
@javax.annotation.Nullable
public LocalDate getDueDate() {
return dueDate;
}
public void setDueDate(LocalDate dueDate) {
this.dueDate = dueDate;
}
public ExpandedInvoice includesOneTime(Boolean includesOneTime) {
this.includesOneTime = includesOneTime;
return this;
}
/**
* Get includesOneTime
* @return includesOneTime
*/
@javax.annotation.Nullable
public Boolean getIncludesOneTime() {
return includesOneTime;
}
public void setIncludesOneTime(Boolean includesOneTime) {
this.includesOneTime = includesOneTime;
}
public ExpandedInvoice includesRecurring(Boolean includesRecurring) {
this.includesRecurring = includesRecurring;
return this;
}
/**
* Get includesRecurring
* @return includesRecurring
*/
@javax.annotation.Nullable
public Boolean getIncludesRecurring() {
return includesRecurring;
}
public void setIncludesRecurring(Boolean includesRecurring) {
this.includesRecurring = includesRecurring;
}
public ExpandedInvoice includesUsage(Boolean includesUsage) {
this.includesUsage = includesUsage;
return this;
}
/**
* Get includesUsage
* @return includesUsage
*/
@javax.annotation.Nullable
public Boolean getIncludesUsage() {
return includesUsage;
}
public void setIncludesUsage(Boolean includesUsage) {
this.includesUsage = includesUsage;
}
public ExpandedInvoice invoiceDate(LocalDate invoiceDate) {
this.invoiceDate = invoiceDate;
return this;
}
/**
* Get invoiceDate
* @return invoiceDate
*/
@javax.annotation.Nullable
public LocalDate getInvoiceDate() {
return invoiceDate;
}
public void setInvoiceDate(LocalDate invoiceDate) {
this.invoiceDate = invoiceDate;
}
public ExpandedInvoice invoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
return this;
}
/**
* Get invoiceGroupNumber
* @return invoiceGroupNumber
*/
@javax.annotation.Nullable
public String getInvoiceGroupNumber() {
return invoiceGroupNumber;
}
public void setInvoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
}
public ExpandedInvoice invoiceNumber(String invoiceNumber) {
this.invoiceNumber = invoiceNumber;
return this;
}
/**
* Get invoiceNumber
* @return invoiceNumber
*/
@javax.annotation.Nullable
public String getInvoiceNumber() {
return invoiceNumber;
}
public void setInvoiceNumber(String invoiceNumber) {
this.invoiceNumber = invoiceNumber;
}
public ExpandedInvoice lastEmailSentDate(String lastEmailSentDate) {
this.lastEmailSentDate = lastEmailSentDate;
return this;
}
/**
* Get lastEmailSentDate
* @return lastEmailSentDate
*/
@javax.annotation.Nullable
public String getLastEmailSentDate() {
return lastEmailSentDate;
}
public void setLastEmailSentDate(String lastEmailSentDate) {
this.lastEmailSentDate = lastEmailSentDate;
}
public ExpandedInvoice organizationId(String organizationId) {
this.organizationId = organizationId;
return this;
}
/**
* Get organizationId
* @return organizationId
*/
@javax.annotation.Nullable
public String getOrganizationId() {
return organizationId;
}
public void setOrganizationId(String organizationId) {
this.organizationId = organizationId;
}
public ExpandedInvoice paymentAmount(BigDecimal paymentAmount) {
this.paymentAmount = paymentAmount;
return this;
}
/**
* Get paymentAmount
* @return paymentAmount
*/
@javax.annotation.Nullable
public BigDecimal getPaymentAmount() {
return paymentAmount;
}
public void setPaymentAmount(BigDecimal paymentAmount) {
this.paymentAmount = paymentAmount;
}
public ExpandedInvoice postedBy(String postedBy) {
this.postedBy = postedBy;
return this;
}
/**
* Get postedBy
* @return postedBy
*/
@javax.annotation.Nullable
public String getPostedBy() {
return postedBy;
}
public void setPostedBy(String postedBy) {
this.postedBy = postedBy;
}
public ExpandedInvoice postedDate(String postedDate) {
this.postedDate = postedDate;
return this;
}
/**
* Get postedDate
* @return postedDate
*/
@javax.annotation.Nullable
public String getPostedDate() {
return postedDate;
}
public void setPostedDate(String postedDate) {
this.postedDate = postedDate;
}
public ExpandedInvoice refundAmount(BigDecimal refundAmount) {
this.refundAmount = refundAmount;
return this;
}
/**
* Get refundAmount
* @return refundAmount
*/
@javax.annotation.Nullable
public BigDecimal getRefundAmount() {
return refundAmount;
}
public void setRefundAmount(BigDecimal refundAmount) {
this.refundAmount = refundAmount;
}
public ExpandedInvoice reversed(Boolean reversed) {
this.reversed = reversed;
return this;
}
/**
* Get reversed
* @return reversed
*/
@javax.annotation.Nullable
public Boolean getReversed() {
return reversed;
}
public void setReversed(Boolean reversed) {
this.reversed = reversed;
}
public ExpandedInvoice sequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
return this;
}
/**
* Get sequenceSetId
* @return sequenceSetId
*/
@javax.annotation.Nullable
public String getSequenceSetId() {
return sequenceSetId;
}
public void setSequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
}
public ExpandedInvoice soldToContactId(String soldToContactId) {
this.soldToContactId = soldToContactId;
return this;
}
/**
* Get soldToContactId
* @return soldToContactId
*/
@javax.annotation.Nullable
public String getSoldToContactId() {
return soldToContactId;
}
public void setSoldToContactId(String soldToContactId) {
this.soldToContactId = soldToContactId;
}
public ExpandedInvoice soldToContactSnapshotId(String soldToContactSnapshotId) {
this.soldToContactSnapshotId = soldToContactSnapshotId;
return this;
}
/**
* Get soldToContactSnapshotId
* @return soldToContactSnapshotId
*/
@javax.annotation.Nullable
public String getSoldToContactSnapshotId() {
return soldToContactSnapshotId;
}
public void setSoldToContactSnapshotId(String soldToContactSnapshotId) {
this.soldToContactSnapshotId = soldToContactSnapshotId;
}
public ExpandedInvoice source(String source) {
this.source = source;
return this;
}
/**
* Get source
* @return source
*/
@javax.annotation.Nullable
public String getSource() {
return source;
}
public void setSource(String source) {
this.source = source;
}
public ExpandedInvoice sourceId(String sourceId) {
this.sourceId = sourceId;
return this;
}
/**
* Get sourceId
* @return sourceId
*/
@javax.annotation.Nullable
public String getSourceId() {
return sourceId;
}
public void setSourceId(String sourceId) {
this.sourceId = sourceId;
}
public ExpandedInvoice sourceType(String sourceType) {
this.sourceType = sourceType;
return this;
}
/**
* Get sourceType
* @return sourceType
*/
@javax.annotation.Nullable
public String getSourceType() {
return sourceType;
}
public void setSourceType(String sourceType) {
this.sourceType = sourceType;
}
public ExpandedInvoice status(String status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public ExpandedInvoice targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* Get targetDate
* @return targetDate
*/
@javax.annotation.Nullable
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public ExpandedInvoice taxAmount(BigDecimal taxAmount) {
this.taxAmount = taxAmount;
return this;
}
/**
* Get taxAmount
* @return taxAmount
*/
@javax.annotation.Nullable
public BigDecimal getTaxAmount() {
return taxAmount;
}
public void setTaxAmount(BigDecimal taxAmount) {
this.taxAmount = taxAmount;
}
public ExpandedInvoice taxExemptAmount(BigDecimal taxExemptAmount) {
this.taxExemptAmount = taxExemptAmount;
return this;
}
/**
* Get taxExemptAmount
* @return taxExemptAmount
*/
@javax.annotation.Nullable
public BigDecimal getTaxExemptAmount() {
return taxExemptAmount;
}
public void setTaxExemptAmount(BigDecimal taxExemptAmount) {
this.taxExemptAmount = taxExemptAmount;
}
public ExpandedInvoice taxStatus(String taxStatus) {
this.taxStatus = taxStatus;
return this;
}
/**
* Get taxStatus
* @return taxStatus
*/
@javax.annotation.Nullable
public String getTaxStatus() {
return taxStatus;
}
public void setTaxStatus(String taxStatus) {
this.taxStatus = taxStatus;
}
public ExpandedInvoice taxMessage(String taxMessage) {
this.taxMessage = taxMessage;
return this;
}
/**
* Get taxMessage
* @return taxMessage
*/
@javax.annotation.Nullable
public String getTaxMessage() {
return taxMessage;
}
public void setTaxMessage(String taxMessage) {
this.taxMessage = taxMessage;
}
public ExpandedInvoice templateId(String templateId) {
this.templateId = templateId;
return this;
}
/**
* Get templateId
* @return templateId
*/
@javax.annotation.Nullable
public String getTemplateId() {
return templateId;
}
public void setTemplateId(String templateId) {
this.templateId = templateId;
}
public ExpandedInvoice transferredToAccounting(String transferredToAccounting) {
this.transferredToAccounting = transferredToAccounting;
return this;
}
/**
* Get transferredToAccounting
* @return transferredToAccounting
*/
@javax.annotation.Nullable
public String getTransferredToAccounting() {
return transferredToAccounting;
}
public void setTransferredToAccounting(String transferredToAccounting) {
this.transferredToAccounting = transferredToAccounting;
}
public ExpandedInvoice eInvoiceStatus(String eInvoiceStatus) {
this.eInvoiceStatus = eInvoiceStatus;
return this;
}
/**
* Get eInvoiceStatus
* @return eInvoiceStatus
*/
@javax.annotation.Nullable
public String geteInvoiceStatus() {
return eInvoiceStatus;
}
public void seteInvoiceStatus(String eInvoiceStatus) {
this.eInvoiceStatus = eInvoiceStatus;
}
public ExpandedInvoice eInvoiceFileId(String eInvoiceFileId) {
this.eInvoiceFileId = eInvoiceFileId;
return this;
}
/**
* Get eInvoiceFileId
* @return eInvoiceFileId
*/
@javax.annotation.Nullable
public String geteInvoiceFileId() {
return eInvoiceFileId;
}
public void seteInvoiceFileId(String eInvoiceFileId) {
this.eInvoiceFileId = eInvoiceFileId;
}
public ExpandedInvoice eInvoiceErrorCode(String eInvoiceErrorCode) {
this.eInvoiceErrorCode = eInvoiceErrorCode;
return this;
}
/**
* Get eInvoiceErrorCode
* @return eInvoiceErrorCode
*/
@javax.annotation.Nullable
public String geteInvoiceErrorCode() {
return eInvoiceErrorCode;
}
public void seteInvoiceErrorCode(String eInvoiceErrorCode) {
this.eInvoiceErrorCode = eInvoiceErrorCode;
}
public ExpandedInvoice eInvoiceErrorMessage(String eInvoiceErrorMessage) {
this.eInvoiceErrorMessage = eInvoiceErrorMessage;
return this;
}
/**
* Get eInvoiceErrorMessage
* @return eInvoiceErrorMessage
*/
@javax.annotation.Nullable
public String geteInvoiceErrorMessage() {
return eInvoiceErrorMessage;
}
public void seteInvoiceErrorMessage(String eInvoiceErrorMessage) {
this.eInvoiceErrorMessage = eInvoiceErrorMessage;
}
public ExpandedInvoice paymentLink(String paymentLink) {
this.paymentLink = paymentLink;
return this;
}
/**
* Get paymentLink
* @return paymentLink
*/
@javax.annotation.Nullable
public String getPaymentLink() {
return paymentLink;
}
public void setPaymentLink(String paymentLink) {
this.paymentLink = paymentLink;
}
public ExpandedInvoice id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public ExpandedInvoice createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* Get createdById
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public ExpandedInvoice createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* Get createdDate
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public ExpandedInvoice updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* Get updatedById
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public ExpandedInvoice updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* Get updatedDate
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
public ExpandedInvoice paymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
return this;
}
/**
* Get paymentTerm
* @return paymentTerm
*/
@javax.annotation.Nullable
public String getPaymentTerm() {
return paymentTerm;
}
public void setPaymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
}
public ExpandedInvoice account(ExpandedAccount account) {
this.account = account;
return this;
}
/**
* Get account
* @return account
*/
@javax.annotation.Nullable
public ExpandedAccount getAccount() {
return account;
}
public void setAccount(ExpandedAccount account) {
this.account = account;
}
public ExpandedInvoice billToContact(ExpandedContact billToContact) {
this.billToContact = billToContact;
return this;
}
/**
* Get billToContact
* @return billToContact
*/
@javax.annotation.Nullable
public ExpandedContact getBillToContact() {
return billToContact;
}
public void setBillToContact(ExpandedContact billToContact) {
this.billToContact = billToContact;
}
public ExpandedInvoice invoiceItems(List invoiceItems) {
this.invoiceItems = invoiceItems;
return this;
}
public ExpandedInvoice addInvoiceItemsItem(ExpandedInvoiceItem invoiceItemsItem) {
if (this.invoiceItems == null) {
this.invoiceItems = new ArrayList<>();
}
this.invoiceItems.add(invoiceItemsItem);
return this;
}
/**
* Get invoiceItems
* @return invoiceItems
*/
@javax.annotation.Nullable
public List getInvoiceItems() {
return invoiceItems;
}
public void setInvoiceItems(List invoiceItems) {
this.invoiceItems = invoiceItems;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the ExpandedInvoice instance itself
*/
public ExpandedInvoice putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ExpandedInvoice expandedInvoice = (ExpandedInvoice) o;
return Objects.equals(this.accountId, expandedInvoice.accountId) &&
Objects.equals(this.adjustmentAmount, expandedInvoice.adjustmentAmount) &&
Objects.equals(this.amount, expandedInvoice.amount) &&
Objects.equals(this.amountWithoutTax, expandedInvoice.amountWithoutTax) &&
Objects.equals(this.autoPay, expandedInvoice.autoPay) &&
Objects.equals(this.balance, expandedInvoice.balance) &&
Objects.equals(this.billToContactId, expandedInvoice.billToContactId) &&
Objects.equals(this.billToContactSnapshotId, expandedInvoice.billToContactSnapshotId) &&
Objects.equals(this.comments, expandedInvoice.comments) &&
Objects.equals(this.creditBalanceAdjustmentAmount, expandedInvoice.creditBalanceAdjustmentAmount) &&
Objects.equals(this.creditMemoAmount, expandedInvoice.creditMemoAmount) &&
Objects.equals(this.currency, expandedInvoice.currency) &&
Objects.equals(this.dueDate, expandedInvoice.dueDate) &&
Objects.equals(this.includesOneTime, expandedInvoice.includesOneTime) &&
Objects.equals(this.includesRecurring, expandedInvoice.includesRecurring) &&
Objects.equals(this.includesUsage, expandedInvoice.includesUsage) &&
Objects.equals(this.invoiceDate, expandedInvoice.invoiceDate) &&
Objects.equals(this.invoiceGroupNumber, expandedInvoice.invoiceGroupNumber) &&
Objects.equals(this.invoiceNumber, expandedInvoice.invoiceNumber) &&
Objects.equals(this.lastEmailSentDate, expandedInvoice.lastEmailSentDate) &&
Objects.equals(this.organizationId, expandedInvoice.organizationId) &&
Objects.equals(this.paymentAmount, expandedInvoice.paymentAmount) &&
Objects.equals(this.postedBy, expandedInvoice.postedBy) &&
Objects.equals(this.postedDate, expandedInvoice.postedDate) &&
Objects.equals(this.refundAmount, expandedInvoice.refundAmount) &&
Objects.equals(this.reversed, expandedInvoice.reversed) &&
Objects.equals(this.sequenceSetId, expandedInvoice.sequenceSetId) &&
Objects.equals(this.soldToContactId, expandedInvoice.soldToContactId) &&
Objects.equals(this.soldToContactSnapshotId, expandedInvoice.soldToContactSnapshotId) &&
Objects.equals(this.source, expandedInvoice.source) &&
Objects.equals(this.sourceId, expandedInvoice.sourceId) &&
Objects.equals(this.sourceType, expandedInvoice.sourceType) &&
Objects.equals(this.status, expandedInvoice.status) &&
Objects.equals(this.targetDate, expandedInvoice.targetDate) &&
Objects.equals(this.taxAmount, expandedInvoice.taxAmount) &&
Objects.equals(this.taxExemptAmount, expandedInvoice.taxExemptAmount) &&
Objects.equals(this.taxStatus, expandedInvoice.taxStatus) &&
Objects.equals(this.taxMessage, expandedInvoice.taxMessage) &&
Objects.equals(this.templateId, expandedInvoice.templateId) &&
Objects.equals(this.transferredToAccounting, expandedInvoice.transferredToAccounting) &&
Objects.equals(this.eInvoiceStatus, expandedInvoice.eInvoiceStatus) &&
Objects.equals(this.eInvoiceFileId, expandedInvoice.eInvoiceFileId) &&
Objects.equals(this.eInvoiceErrorCode, expandedInvoice.eInvoiceErrorCode) &&
Objects.equals(this.eInvoiceErrorMessage, expandedInvoice.eInvoiceErrorMessage) &&
Objects.equals(this.paymentLink, expandedInvoice.paymentLink) &&
Objects.equals(this.id, expandedInvoice.id) &&
Objects.equals(this.createdById, expandedInvoice.createdById) &&
Objects.equals(this.createdDate, expandedInvoice.createdDate) &&
Objects.equals(this.updatedById, expandedInvoice.updatedById) &&
Objects.equals(this.updatedDate, expandedInvoice.updatedDate) &&
Objects.equals(this.paymentTerm, expandedInvoice.paymentTerm) &&
Objects.equals(this.account, expandedInvoice.account) &&
Objects.equals(this.billToContact, expandedInvoice.billToContact) &&
Objects.equals(this.invoiceItems, expandedInvoice.invoiceItems)&&
Objects.equals(this.additionalProperties, expandedInvoice.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(accountId, adjustmentAmount, amount, amountWithoutTax, autoPay, balance, billToContactId, billToContactSnapshotId, comments, creditBalanceAdjustmentAmount, creditMemoAmount, currency, dueDate, includesOneTime, includesRecurring, includesUsage, invoiceDate, invoiceGroupNumber, invoiceNumber, lastEmailSentDate, organizationId, paymentAmount, postedBy, postedDate, refundAmount, reversed, sequenceSetId, soldToContactId, soldToContactSnapshotId, source, sourceId, sourceType, status, targetDate, taxAmount, taxExemptAmount, taxStatus, taxMessage, templateId, transferredToAccounting, eInvoiceStatus, eInvoiceFileId, eInvoiceErrorCode, eInvoiceErrorMessage, paymentLink, id, createdById, createdDate, updatedById, updatedDate, paymentTerm, account, billToContact, invoiceItems, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ExpandedInvoice {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" adjustmentAmount: ").append(toIndentedString(adjustmentAmount)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" amountWithoutTax: ").append(toIndentedString(amountWithoutTax)).append("\n");
sb.append(" autoPay: ").append(toIndentedString(autoPay)).append("\n");
sb.append(" balance: ").append(toIndentedString(balance)).append("\n");
sb.append(" billToContactId: ").append(toIndentedString(billToContactId)).append("\n");
sb.append(" billToContactSnapshotId: ").append(toIndentedString(billToContactSnapshotId)).append("\n");
sb.append(" comments: ").append(toIndentedString(comments)).append("\n");
sb.append(" creditBalanceAdjustmentAmount: ").append(toIndentedString(creditBalanceAdjustmentAmount)).append("\n");
sb.append(" creditMemoAmount: ").append(toIndentedString(creditMemoAmount)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" dueDate: ").append(toIndentedString(dueDate)).append("\n");
sb.append(" includesOneTime: ").append(toIndentedString(includesOneTime)).append("\n");
sb.append(" includesRecurring: ").append(toIndentedString(includesRecurring)).append("\n");
sb.append(" includesUsage: ").append(toIndentedString(includesUsage)).append("\n");
sb.append(" invoiceDate: ").append(toIndentedString(invoiceDate)).append("\n");
sb.append(" invoiceGroupNumber: ").append(toIndentedString(invoiceGroupNumber)).append("\n");
sb.append(" invoiceNumber: ").append(toIndentedString(invoiceNumber)).append("\n");
sb.append(" lastEmailSentDate: ").append(toIndentedString(lastEmailSentDate)).append("\n");
sb.append(" organizationId: ").append(toIndentedString(organizationId)).append("\n");
sb.append(" paymentAmount: ").append(toIndentedString(paymentAmount)).append("\n");
sb.append(" postedBy: ").append(toIndentedString(postedBy)).append("\n");
sb.append(" postedDate: ").append(toIndentedString(postedDate)).append("\n");
sb.append(" refundAmount: ").append(toIndentedString(refundAmount)).append("\n");
sb.append(" reversed: ").append(toIndentedString(reversed)).append("\n");
sb.append(" sequenceSetId: ").append(toIndentedString(sequenceSetId)).append("\n");
sb.append(" soldToContactId: ").append(toIndentedString(soldToContactId)).append("\n");
sb.append(" soldToContactSnapshotId: ").append(toIndentedString(soldToContactSnapshotId)).append("\n");
sb.append(" source: ").append(toIndentedString(source)).append("\n");
sb.append(" sourceId: ").append(toIndentedString(sourceId)).append("\n");
sb.append(" sourceType: ").append(toIndentedString(sourceType)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" taxAmount: ").append(toIndentedString(taxAmount)).append("\n");
sb.append(" taxExemptAmount: ").append(toIndentedString(taxExemptAmount)).append("\n");
sb.append(" taxStatus: ").append(toIndentedString(taxStatus)).append("\n");
sb.append(" taxMessage: ").append(toIndentedString(taxMessage)).append("\n");
sb.append(" templateId: ").append(toIndentedString(templateId)).append("\n");
sb.append(" transferredToAccounting: ").append(toIndentedString(transferredToAccounting)).append("\n");
sb.append(" eInvoiceStatus: ").append(toIndentedString(eInvoiceStatus)).append("\n");
sb.append(" eInvoiceFileId: ").append(toIndentedString(eInvoiceFileId)).append("\n");
sb.append(" eInvoiceErrorCode: ").append(toIndentedString(eInvoiceErrorCode)).append("\n");
sb.append(" eInvoiceErrorMessage: ").append(toIndentedString(eInvoiceErrorMessage)).append("\n");
sb.append(" paymentLink: ").append(toIndentedString(paymentLink)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" paymentTerm: ").append(toIndentedString(paymentTerm)).append("\n");
sb.append(" account: ").append(toIndentedString(account)).append("\n");
sb.append(" billToContact: ").append(toIndentedString(billToContact)).append("\n");
sb.append(" invoiceItems: ").append(toIndentedString(invoiceItems)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("accountId");
openapiFields.add("adjustmentAmount");
openapiFields.add("amount");
openapiFields.add("amountWithoutTax");
openapiFields.add("autoPay");
openapiFields.add("balance");
openapiFields.add("billToContactId");
openapiFields.add("billToContactSnapshotId");
openapiFields.add("comments");
openapiFields.add("creditBalanceAdjustmentAmount");
openapiFields.add("creditMemoAmount");
openapiFields.add("currency");
openapiFields.add("dueDate");
openapiFields.add("includesOneTime");
openapiFields.add("includesRecurring");
openapiFields.add("includesUsage");
openapiFields.add("invoiceDate");
openapiFields.add("invoiceGroupNumber");
openapiFields.add("invoiceNumber");
openapiFields.add("lastEmailSentDate");
openapiFields.add("organizationId");
openapiFields.add("paymentAmount");
openapiFields.add("postedBy");
openapiFields.add("postedDate");
openapiFields.add("refundAmount");
openapiFields.add("reversed");
openapiFields.add("sequenceSetId");
openapiFields.add("soldToContactId");
openapiFields.add("soldToContactSnapshotId");
openapiFields.add("source");
openapiFields.add("sourceId");
openapiFields.add("sourceType");
openapiFields.add("status");
openapiFields.add("targetDate");
openapiFields.add("taxAmount");
openapiFields.add("taxExemptAmount");
openapiFields.add("taxStatus");
openapiFields.add("taxMessage");
openapiFields.add("templateId");
openapiFields.add("transferredToAccounting");
openapiFields.add("eInvoiceStatus");
openapiFields.add("eInvoiceFileId");
openapiFields.add("eInvoiceErrorCode");
openapiFields.add("eInvoiceErrorMessage");
openapiFields.add("paymentLink");
openapiFields.add("id");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
openapiFields.add("paymentTerm");
openapiFields.add("account");
openapiFields.add("billToContact");
openapiFields.add("invoiceItems");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to ExpandedInvoice
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!ExpandedInvoice.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in ExpandedInvoice is not found in the empty JSON string", ExpandedInvoice.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("accountId") != null && !jsonObj.get("accountId").isJsonNull()) && !jsonObj.get("accountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountId").toString()));
}
if ((jsonObj.get("billToContactId") != null && !jsonObj.get("billToContactId").isJsonNull()) && !jsonObj.get("billToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billToContactId").toString()));
}
if ((jsonObj.get("billToContactSnapshotId") != null && !jsonObj.get("billToContactSnapshotId").isJsonNull()) && !jsonObj.get("billToContactSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billToContactSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billToContactSnapshotId").toString()));
}
if ((jsonObj.get("comments") != null && !jsonObj.get("comments").isJsonNull()) && !jsonObj.get("comments").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `comments` to be a primitive type in the JSON string but got `%s`", jsonObj.get("comments").toString()));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("invoiceGroupNumber") != null && !jsonObj.get("invoiceGroupNumber").isJsonNull()) && !jsonObj.get("invoiceGroupNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceGroupNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceGroupNumber").toString()));
}
if ((jsonObj.get("invoiceNumber") != null && !jsonObj.get("invoiceNumber").isJsonNull()) && !jsonObj.get("invoiceNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceNumber").toString()));
}
if ((jsonObj.get("lastEmailSentDate") != null && !jsonObj.get("lastEmailSentDate").isJsonNull()) && !jsonObj.get("lastEmailSentDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `lastEmailSentDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("lastEmailSentDate").toString()));
}
if ((jsonObj.get("organizationId") != null && !jsonObj.get("organizationId").isJsonNull()) && !jsonObj.get("organizationId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `organizationId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("organizationId").toString()));
}
if ((jsonObj.get("postedBy") != null && !jsonObj.get("postedBy").isJsonNull()) && !jsonObj.get("postedBy").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `postedBy` to be a primitive type in the JSON string but got `%s`", jsonObj.get("postedBy").toString()));
}
if ((jsonObj.get("postedDate") != null && !jsonObj.get("postedDate").isJsonNull()) && !jsonObj.get("postedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `postedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("postedDate").toString()));
}
if ((jsonObj.get("sequenceSetId") != null && !jsonObj.get("sequenceSetId").isJsonNull()) && !jsonObj.get("sequenceSetId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sequenceSetId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sequenceSetId").toString()));
}
if ((jsonObj.get("soldToContactId") != null && !jsonObj.get("soldToContactId").isJsonNull()) && !jsonObj.get("soldToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToContactId").toString()));
}
if ((jsonObj.get("soldToContactSnapshotId") != null && !jsonObj.get("soldToContactSnapshotId").isJsonNull()) && !jsonObj.get("soldToContactSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToContactSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToContactSnapshotId").toString()));
}
if ((jsonObj.get("source") != null && !jsonObj.get("source").isJsonNull()) && !jsonObj.get("source").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `source` to be a primitive type in the JSON string but got `%s`", jsonObj.get("source").toString()));
}
if ((jsonObj.get("sourceId") != null && !jsonObj.get("sourceId").isJsonNull()) && !jsonObj.get("sourceId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sourceId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sourceId").toString()));
}
if ((jsonObj.get("sourceType") != null && !jsonObj.get("sourceType").isJsonNull()) && !jsonObj.get("sourceType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sourceType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sourceType").toString()));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
if ((jsonObj.get("taxStatus") != null && !jsonObj.get("taxStatus").isJsonNull()) && !jsonObj.get("taxStatus").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxStatus` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxStatus").toString()));
}
if ((jsonObj.get("taxMessage") != null && !jsonObj.get("taxMessage").isJsonNull()) && !jsonObj.get("taxMessage").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxMessage` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxMessage").toString()));
}
if ((jsonObj.get("templateId") != null && !jsonObj.get("templateId").isJsonNull()) && !jsonObj.get("templateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `templateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("templateId").toString()));
}
if ((jsonObj.get("transferredToAccounting") != null && !jsonObj.get("transferredToAccounting").isJsonNull()) && !jsonObj.get("transferredToAccounting").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `transferredToAccounting` to be a primitive type in the JSON string but got `%s`", jsonObj.get("transferredToAccounting").toString()));
}
if ((jsonObj.get("eInvoiceStatus") != null && !jsonObj.get("eInvoiceStatus").isJsonNull()) && !jsonObj.get("eInvoiceStatus").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `eInvoiceStatus` to be a primitive type in the JSON string but got `%s`", jsonObj.get("eInvoiceStatus").toString()));
}
if ((jsonObj.get("eInvoiceFileId") != null && !jsonObj.get("eInvoiceFileId").isJsonNull()) && !jsonObj.get("eInvoiceFileId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `eInvoiceFileId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("eInvoiceFileId").toString()));
}
if ((jsonObj.get("eInvoiceErrorCode") != null && !jsonObj.get("eInvoiceErrorCode").isJsonNull()) && !jsonObj.get("eInvoiceErrorCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `eInvoiceErrorCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("eInvoiceErrorCode").toString()));
}
if ((jsonObj.get("eInvoiceErrorMessage") != null && !jsonObj.get("eInvoiceErrorMessage").isJsonNull()) && !jsonObj.get("eInvoiceErrorMessage").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `eInvoiceErrorMessage` to be a primitive type in the JSON string but got `%s`", jsonObj.get("eInvoiceErrorMessage").toString()));
}
if ((jsonObj.get("paymentLink") != null && !jsonObj.get("paymentLink").isJsonNull()) && !jsonObj.get("paymentLink").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentLink` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentLink").toString()));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
if ((jsonObj.get("paymentTerm") != null && !jsonObj.get("paymentTerm").isJsonNull()) && !jsonObj.get("paymentTerm").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentTerm` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentTerm").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("invoiceItems") != null && !jsonObj.get("invoiceItems").isJsonNull() && !jsonObj.get("invoiceItems").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceItems` to be an array in the JSON string but got `%s`", jsonObj.get("invoiceItems").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!ExpandedInvoice.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'ExpandedInvoice' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(ExpandedInvoice.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, ExpandedInvoice value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public ExpandedInvoice read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
ExpandedInvoice instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of ExpandedInvoice given an JSON string
*
* @param jsonString JSON string
* @return An instance of ExpandedInvoice
* @throws IOException if the JSON string is invalid with respect to ExpandedInvoice
*/
public static ExpandedInvoice fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, ExpandedInvoice.class);
}
/**
* Convert an instance of ExpandedInvoice to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy