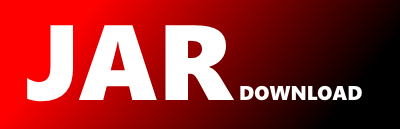
com.zuora.model.ExpandedOrderLineItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.ExpandedInvoiceItem;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* ExpandedOrderLineItem
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class ExpandedOrderLineItem {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public static final String SERIALIZED_NAME_ACCOUNTING_CODE = "accountingCode";
@SerializedName(SERIALIZED_NAME_ACCOUNTING_CODE)
private String accountingCode;
public static final String SERIALIZED_NAME_BILL_TARGET_DATE = "billTargetDate";
@SerializedName(SERIALIZED_NAME_BILL_TARGET_DATE)
private LocalDate billTargetDate;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_AMOUNT_PER_UNIT = "amountPerUnit";
@SerializedName(SERIALIZED_NAME_AMOUNT_PER_UNIT)
private BigDecimal amountPerUnit;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_AMOUNT_WITHOUT_TAX = "amountWithoutTax";
@SerializedName(SERIALIZED_NAME_AMOUNT_WITHOUT_TAX)
private BigDecimal amountWithoutTax;
public static final String SERIALIZED_NAME_INVOICE_GROUP_NUMBER = "invoiceGroupNumber";
@SerializedName(SERIALIZED_NAME_INVOICE_GROUP_NUMBER)
private String invoiceGroupNumber;
public static final String SERIALIZED_NAME_ITEM_NAME = "itemName";
@SerializedName(SERIALIZED_NAME_ITEM_NAME)
private String itemName;
public static final String SERIALIZED_NAME_ITEM_NUMBER = "itemNumber";
@SerializedName(SERIALIZED_NAME_ITEM_NUMBER)
private String itemNumber;
public static final String SERIALIZED_NAME_ITEM_STATE = "itemState";
@SerializedName(SERIALIZED_NAME_ITEM_STATE)
private String itemState;
public static final String SERIALIZED_NAME_ITEM_TYPE = "itemType";
@SerializedName(SERIALIZED_NAME_ITEM_TYPE)
private String itemType;
public static final String SERIALIZED_NAME_LIST_PRICE_PER_UNIT = "listPricePerUnit";
@SerializedName(SERIALIZED_NAME_LIST_PRICE_PER_UNIT)
private BigDecimal listPricePerUnit;
public static final String SERIALIZED_NAME_LIST_PRICE = "listPrice";
@SerializedName(SERIALIZED_NAME_LIST_PRICE)
private BigDecimal listPrice;
public static final String SERIALIZED_NAME_ORDER_ID = "orderId";
@SerializedName(SERIALIZED_NAME_ORDER_ID)
private String orderId;
public static final String SERIALIZED_NAME_PRODUCT_CODE = "productCode";
@SerializedName(SERIALIZED_NAME_PRODUCT_CODE)
private String productCode;
public static final String SERIALIZED_NAME_PURCHASE_ORDER_NUMBER = "purchaseOrderNumber";
@SerializedName(SERIALIZED_NAME_PURCHASE_ORDER_NUMBER)
private String purchaseOrderNumber;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_RULE = "revenueRecognitionRule";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_RULE)
private String revenueRecognitionRule;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_ID = "productRatePlanChargeId";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_ID)
private String productRatePlanChargeId;
public static final String SERIALIZED_NAME_BILL_TO_ID = "billToId";
@SerializedName(SERIALIZED_NAME_BILL_TO_ID)
private String billToId;
public static final String SERIALIZED_NAME_BILL_TO_SNAPSHOT_ID = "billToSnapshotId";
@SerializedName(SERIALIZED_NAME_BILL_TO_SNAPSHOT_ID)
private String billToSnapshotId;
public static final String SERIALIZED_NAME_SOLD_TO = "soldTo";
@SerializedName(SERIALIZED_NAME_SOLD_TO)
private String soldTo;
public static final String SERIALIZED_NAME_SOLD_TO_SNAPSHOT_ID = "soldToSnapshotId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_SNAPSHOT_ID)
private String soldToSnapshotId;
public static final String SERIALIZED_NAME_SOLD_TO_ORDER_CONTACT_ID = "soldToOrderContactId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_ORDER_CONTACT_ID)
private String soldToOrderContactId;
public static final String SERIALIZED_NAME_OWNER_ACCOUNT_ID = "ownerAccountId";
@SerializedName(SERIALIZED_NAME_OWNER_ACCOUNT_ID)
private String ownerAccountId;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_ID = "invoiceOwnerAccountId";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_ID)
private String invoiceOwnerAccountId;
public static final String SERIALIZED_NAME_TAX_CODE = "taxCode";
@SerializedName(SERIALIZED_NAME_TAX_CODE)
private String taxCode;
public static final String SERIALIZED_NAME_TAX_MODE = "taxMode";
@SerializedName(SERIALIZED_NAME_TAX_MODE)
private String taxMode;
public static final String SERIALIZED_NAME_TRANSACTION_DATE = "transactionDate";
@SerializedName(SERIALIZED_NAME_TRANSACTION_DATE)
private LocalDate transactionDate;
public static final String SERIALIZED_NAME_U_O_M = "uOM";
@SerializedName(SERIALIZED_NAME_U_O_M)
private String uOM;
public static final String SERIALIZED_NAME_RELATED_SUBSCRIPTION_NUMBER = "relatedSubscriptionNumber";
@SerializedName(SERIALIZED_NAME_RELATED_SUBSCRIPTION_NUMBER)
private String relatedSubscriptionNumber;
public static final String SERIALIZED_NAME_TRANSACTION_START_DATE = "transactionStartDate";
@SerializedName(SERIALIZED_NAME_TRANSACTION_START_DATE)
private LocalDate transactionStartDate;
public static final String SERIALIZED_NAME_TRANSACTION_END_DATE = "transactionEndDate";
@SerializedName(SERIALIZED_NAME_TRANSACTION_END_DATE)
private LocalDate transactionEndDate;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING = "excludeItemBookingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBookingFromRevenueAccounting;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING = "excludeItemBillingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBillingFromRevenueAccounting;
public static final String SERIALIZED_NAME_IS_ALLOCATION_ELIGIBLE = "isAllocationEligible";
@SerializedName(SERIALIZED_NAME_IS_ALLOCATION_ELIGIBLE)
private Boolean isAllocationEligible;
public static final String SERIALIZED_NAME_IS_UNBILLED = "isUnbilled";
@SerializedName(SERIALIZED_NAME_IS_UNBILLED)
private Boolean isUnbilled;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_TIMING = "revenueRecognitionTiming";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_TIMING)
private String revenueRecognitionTiming;
public static final String SERIALIZED_NAME_REVENUE_AMORTIZATION_METHOD = "revenueAmortizationMethod";
@SerializedName(SERIALIZED_NAME_REVENUE_AMORTIZATION_METHOD)
private String revenueAmortizationMethod;
public static final String SERIALIZED_NAME_ORIGINAL_ORDER_DATE = "originalOrderDate";
@SerializedName(SERIALIZED_NAME_ORIGINAL_ORDER_DATE)
private LocalDate originalOrderDate;
public static final String SERIALIZED_NAME_AMENDED_BY_ORDER_ON = "amendedByOrderOn";
@SerializedName(SERIALIZED_NAME_AMENDED_BY_ORDER_ON)
private LocalDate amendedByOrderOn;
public static final String SERIALIZED_NAME_ITEM_CATEGORY = "itemCategory";
@SerializedName(SERIALIZED_NAME_ITEM_CATEGORY)
private String itemCategory;
public static final String SERIALIZED_NAME_ORIGINAL_ORDER_ID = "originalOrderId";
@SerializedName(SERIALIZED_NAME_ORIGINAL_ORDER_ID)
private String originalOrderId;
public static final String SERIALIZED_NAME_ORIGINAL_ORDER_NUMBER = "originalOrderNumber";
@SerializedName(SERIALIZED_NAME_ORIGINAL_ORDER_NUMBER)
private String originalOrderNumber;
public static final String SERIALIZED_NAME_ORIGINAL_ORDER_LINE_ITEM_ID = "originalOrderLineItemId";
@SerializedName(SERIALIZED_NAME_ORIGINAL_ORDER_LINE_ITEM_ID)
private String originalOrderLineItemId;
public static final String SERIALIZED_NAME_ORIGINAL_ORDER_LINE_ITEM_NUMBER = "originalOrderLineItemNumber";
@SerializedName(SERIALIZED_NAME_ORIGINAL_ORDER_LINE_ITEM_NUMBER)
private String originalOrderLineItemNumber;
public static final String SERIALIZED_NAME_QUANTITY_FULFILLED = "quantityFulfilled";
@SerializedName(SERIALIZED_NAME_QUANTITY_FULFILLED)
private BigDecimal quantityFulfilled;
public static final String SERIALIZED_NAME_QUANTITY_PENDING_FULFILLMENT = "quantityPendingFulfillment";
@SerializedName(SERIALIZED_NAME_QUANTITY_PENDING_FULFILLMENT)
private BigDecimal quantityPendingFulfillment;
public static final String SERIALIZED_NAME_QUANTITY_AVAILABLE_FOR_RETURN = "quantityAvailableForReturn";
@SerializedName(SERIALIZED_NAME_QUANTITY_AVAILABLE_FOR_RETURN)
private BigDecimal quantityAvailableForReturn;
public static final String SERIALIZED_NAME_REQUIRES_FULFILLMENT = "requiresFulfillment";
@SerializedName(SERIALIZED_NAME_REQUIRES_FULFILLMENT)
private Boolean requiresFulfillment;
public static final String SERIALIZED_NAME_BILLING_RULE = "billingRule";
@SerializedName(SERIALIZED_NAME_BILLING_RULE)
private String billingRule;
public static final String SERIALIZED_NAME_INLINE_DISCOUNT_PER_UNIT = "inlineDiscountPerUnit";
@SerializedName(SERIALIZED_NAME_INLINE_DISCOUNT_PER_UNIT)
private BigDecimal inlineDiscountPerUnit;
public static final String SERIALIZED_NAME_INLINE_DISCOUNT_TYPE = "inlineDiscountType";
@SerializedName(SERIALIZED_NAME_INLINE_DISCOUNT_TYPE)
private String inlineDiscountType;
public static final String SERIALIZED_NAME_DISCOUNT = "discount";
@SerializedName(SERIALIZED_NAME_DISCOUNT)
private BigDecimal discount;
public static final String SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE_ID = "recognizedRevenueAccountingCodeId";
@SerializedName(SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE_ID)
private String recognizedRevenueAccountingCodeId;
public static final String SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE_ID = "deferredRevenueAccountingCodeId";
@SerializedName(SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE_ID)
private String deferredRevenueAccountingCodeId;
public static final String SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNTING_CODE_ID = "contractAssetAccountingCodeId";
@SerializedName(SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNTING_CODE_ID)
private String contractAssetAccountingCodeId;
public static final String SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNTING_CODE_ID = "contractLiabilityAccountingCodeId";
@SerializedName(SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNTING_CODE_ID)
private String contractLiabilityAccountingCodeId;
public static final String SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNTING_CODE_ID = "contractRecognizedRevenueAccountingCodeId";
@SerializedName(SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNTING_CODE_ID)
private String contractRecognizedRevenueAccountingCodeId;
public static final String SERIALIZED_NAME_UNBILLED_RECEIVABLES_ACCOUNTING_CODE_ID = "unbilledReceivablesAccountingCodeId";
@SerializedName(SERIALIZED_NAME_UNBILLED_RECEIVABLES_ACCOUNTING_CODE_ID)
private String unbilledReceivablesAccountingCodeId;
public static final String SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNTING_CODE_ID = "adjustmentRevenueAccountingCodeId";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNTING_CODE_ID)
private String adjustmentRevenueAccountingCodeId;
public static final String SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNTING_CODE_ID = "adjustmentLiabilityAccountingCodeId";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNTING_CODE_ID)
private String adjustmentLiabilityAccountingCodeId;
public static final String SERIALIZED_NAME_INVOICE_ITEMS = "invoiceItems";
@SerializedName(SERIALIZED_NAME_INVOICE_ITEMS)
private List invoiceItems;
public ExpandedOrderLineItem() {
}
public ExpandedOrderLineItem id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public ExpandedOrderLineItem createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* Get createdById
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public ExpandedOrderLineItem createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* Get createdDate
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public ExpandedOrderLineItem updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* Get updatedById
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public ExpandedOrderLineItem updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* Get updatedDate
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
public ExpandedOrderLineItem accountingCode(String accountingCode) {
this.accountingCode = accountingCode;
return this;
}
/**
* Get accountingCode
* @return accountingCode
*/
@javax.annotation.Nullable
public String getAccountingCode() {
return accountingCode;
}
public void setAccountingCode(String accountingCode) {
this.accountingCode = accountingCode;
}
public ExpandedOrderLineItem billTargetDate(LocalDate billTargetDate) {
this.billTargetDate = billTargetDate;
return this;
}
/**
* Get billTargetDate
* @return billTargetDate
*/
@javax.annotation.Nullable
public LocalDate getBillTargetDate() {
return billTargetDate;
}
public void setBillTargetDate(LocalDate billTargetDate) {
this.billTargetDate = billTargetDate;
}
public ExpandedOrderLineItem currency(String currency) {
this.currency = currency;
return this;
}
/**
* Get currency
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public ExpandedOrderLineItem amountPerUnit(BigDecimal amountPerUnit) {
this.amountPerUnit = amountPerUnit;
return this;
}
/**
* Get amountPerUnit
* @return amountPerUnit
*/
@javax.annotation.Nullable
public BigDecimal getAmountPerUnit() {
return amountPerUnit;
}
public void setAmountPerUnit(BigDecimal amountPerUnit) {
this.amountPerUnit = amountPerUnit;
}
public ExpandedOrderLineItem description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public ExpandedOrderLineItem amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* Get amount
* @return amount
*/
@javax.annotation.Nullable
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public ExpandedOrderLineItem amountWithoutTax(BigDecimal amountWithoutTax) {
this.amountWithoutTax = amountWithoutTax;
return this;
}
/**
* Get amountWithoutTax
* @return amountWithoutTax
*/
@javax.annotation.Nullable
public BigDecimal getAmountWithoutTax() {
return amountWithoutTax;
}
public void setAmountWithoutTax(BigDecimal amountWithoutTax) {
this.amountWithoutTax = amountWithoutTax;
}
public ExpandedOrderLineItem invoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
return this;
}
/**
* Get invoiceGroupNumber
* @return invoiceGroupNumber
*/
@javax.annotation.Nullable
public String getInvoiceGroupNumber() {
return invoiceGroupNumber;
}
public void setInvoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
}
public ExpandedOrderLineItem itemName(String itemName) {
this.itemName = itemName;
return this;
}
/**
* Get itemName
* @return itemName
*/
@javax.annotation.Nullable
public String getItemName() {
return itemName;
}
public void setItemName(String itemName) {
this.itemName = itemName;
}
public ExpandedOrderLineItem itemNumber(String itemNumber) {
this.itemNumber = itemNumber;
return this;
}
/**
* Get itemNumber
* @return itemNumber
*/
@javax.annotation.Nullable
public String getItemNumber() {
return itemNumber;
}
public void setItemNumber(String itemNumber) {
this.itemNumber = itemNumber;
}
public ExpandedOrderLineItem itemState(String itemState) {
this.itemState = itemState;
return this;
}
/**
* Get itemState
* @return itemState
*/
@javax.annotation.Nullable
public String getItemState() {
return itemState;
}
public void setItemState(String itemState) {
this.itemState = itemState;
}
public ExpandedOrderLineItem itemType(String itemType) {
this.itemType = itemType;
return this;
}
/**
* Get itemType
* @return itemType
*/
@javax.annotation.Nullable
public String getItemType() {
return itemType;
}
public void setItemType(String itemType) {
this.itemType = itemType;
}
public ExpandedOrderLineItem listPricePerUnit(BigDecimal listPricePerUnit) {
this.listPricePerUnit = listPricePerUnit;
return this;
}
/**
* Get listPricePerUnit
* @return listPricePerUnit
*/
@javax.annotation.Nullable
public BigDecimal getListPricePerUnit() {
return listPricePerUnit;
}
public void setListPricePerUnit(BigDecimal listPricePerUnit) {
this.listPricePerUnit = listPricePerUnit;
}
public ExpandedOrderLineItem listPrice(BigDecimal listPrice) {
this.listPrice = listPrice;
return this;
}
/**
* Get listPrice
* @return listPrice
*/
@javax.annotation.Nullable
public BigDecimal getListPrice() {
return listPrice;
}
public void setListPrice(BigDecimal listPrice) {
this.listPrice = listPrice;
}
public ExpandedOrderLineItem orderId(String orderId) {
this.orderId = orderId;
return this;
}
/**
* Get orderId
* @return orderId
*/
@javax.annotation.Nullable
public String getOrderId() {
return orderId;
}
public void setOrderId(String orderId) {
this.orderId = orderId;
}
public ExpandedOrderLineItem productCode(String productCode) {
this.productCode = productCode;
return this;
}
/**
* Get productCode
* @return productCode
*/
@javax.annotation.Nullable
public String getProductCode() {
return productCode;
}
public void setProductCode(String productCode) {
this.productCode = productCode;
}
public ExpandedOrderLineItem purchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
return this;
}
/**
* Get purchaseOrderNumber
* @return purchaseOrderNumber
*/
@javax.annotation.Nullable
public String getPurchaseOrderNumber() {
return purchaseOrderNumber;
}
public void setPurchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
}
public ExpandedOrderLineItem quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* Get quantity
* @return quantity
*/
@javax.annotation.Nullable
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public ExpandedOrderLineItem revenueRecognitionRule(String revenueRecognitionRule) {
this.revenueRecognitionRule = revenueRecognitionRule;
return this;
}
/**
* Get revenueRecognitionRule
* @return revenueRecognitionRule
*/
@javax.annotation.Nullable
public String getRevenueRecognitionRule() {
return revenueRecognitionRule;
}
public void setRevenueRecognitionRule(String revenueRecognitionRule) {
this.revenueRecognitionRule = revenueRecognitionRule;
}
public ExpandedOrderLineItem productRatePlanChargeId(String productRatePlanChargeId) {
this.productRatePlanChargeId = productRatePlanChargeId;
return this;
}
/**
* Get productRatePlanChargeId
* @return productRatePlanChargeId
*/
@javax.annotation.Nullable
public String getProductRatePlanChargeId() {
return productRatePlanChargeId;
}
public void setProductRatePlanChargeId(String productRatePlanChargeId) {
this.productRatePlanChargeId = productRatePlanChargeId;
}
public ExpandedOrderLineItem billToId(String billToId) {
this.billToId = billToId;
return this;
}
/**
* Get billToId
* @return billToId
*/
@javax.annotation.Nullable
public String getBillToId() {
return billToId;
}
public void setBillToId(String billToId) {
this.billToId = billToId;
}
public ExpandedOrderLineItem billToSnapshotId(String billToSnapshotId) {
this.billToSnapshotId = billToSnapshotId;
return this;
}
/**
* Get billToSnapshotId
* @return billToSnapshotId
*/
@javax.annotation.Nullable
public String getBillToSnapshotId() {
return billToSnapshotId;
}
public void setBillToSnapshotId(String billToSnapshotId) {
this.billToSnapshotId = billToSnapshotId;
}
public ExpandedOrderLineItem soldTo(String soldTo) {
this.soldTo = soldTo;
return this;
}
/**
* Get soldTo
* @return soldTo
*/
@javax.annotation.Nullable
public String getSoldTo() {
return soldTo;
}
public void setSoldTo(String soldTo) {
this.soldTo = soldTo;
}
public ExpandedOrderLineItem soldToSnapshotId(String soldToSnapshotId) {
this.soldToSnapshotId = soldToSnapshotId;
return this;
}
/**
* Get soldToSnapshotId
* @return soldToSnapshotId
*/
@javax.annotation.Nullable
public String getSoldToSnapshotId() {
return soldToSnapshotId;
}
public void setSoldToSnapshotId(String soldToSnapshotId) {
this.soldToSnapshotId = soldToSnapshotId;
}
public ExpandedOrderLineItem soldToOrderContactId(String soldToOrderContactId) {
this.soldToOrderContactId = soldToOrderContactId;
return this;
}
/**
* Get soldToOrderContactId
* @return soldToOrderContactId
*/
@javax.annotation.Nullable
public String getSoldToOrderContactId() {
return soldToOrderContactId;
}
public void setSoldToOrderContactId(String soldToOrderContactId) {
this.soldToOrderContactId = soldToOrderContactId;
}
public ExpandedOrderLineItem ownerAccountId(String ownerAccountId) {
this.ownerAccountId = ownerAccountId;
return this;
}
/**
* Get ownerAccountId
* @return ownerAccountId
*/
@javax.annotation.Nullable
public String getOwnerAccountId() {
return ownerAccountId;
}
public void setOwnerAccountId(String ownerAccountId) {
this.ownerAccountId = ownerAccountId;
}
public ExpandedOrderLineItem invoiceOwnerAccountId(String invoiceOwnerAccountId) {
this.invoiceOwnerAccountId = invoiceOwnerAccountId;
return this;
}
/**
* Get invoiceOwnerAccountId
* @return invoiceOwnerAccountId
*/
@javax.annotation.Nullable
public String getInvoiceOwnerAccountId() {
return invoiceOwnerAccountId;
}
public void setInvoiceOwnerAccountId(String invoiceOwnerAccountId) {
this.invoiceOwnerAccountId = invoiceOwnerAccountId;
}
public ExpandedOrderLineItem taxCode(String taxCode) {
this.taxCode = taxCode;
return this;
}
/**
* Get taxCode
* @return taxCode
*/
@javax.annotation.Nullable
public String getTaxCode() {
return taxCode;
}
public void setTaxCode(String taxCode) {
this.taxCode = taxCode;
}
public ExpandedOrderLineItem taxMode(String taxMode) {
this.taxMode = taxMode;
return this;
}
/**
* Get taxMode
* @return taxMode
*/
@javax.annotation.Nullable
public String getTaxMode() {
return taxMode;
}
public void setTaxMode(String taxMode) {
this.taxMode = taxMode;
}
public ExpandedOrderLineItem transactionDate(LocalDate transactionDate) {
this.transactionDate = transactionDate;
return this;
}
/**
* Get transactionDate
* @return transactionDate
*/
@javax.annotation.Nullable
public LocalDate getTransactionDate() {
return transactionDate;
}
public void setTransactionDate(LocalDate transactionDate) {
this.transactionDate = transactionDate;
}
public ExpandedOrderLineItem uOM(String uOM) {
this.uOM = uOM;
return this;
}
/**
* Get uOM
* @return uOM
*/
@javax.annotation.Nullable
public String getuOM() {
return uOM;
}
public void setuOM(String uOM) {
this.uOM = uOM;
}
public ExpandedOrderLineItem relatedSubscriptionNumber(String relatedSubscriptionNumber) {
this.relatedSubscriptionNumber = relatedSubscriptionNumber;
return this;
}
/**
* Get relatedSubscriptionNumber
* @return relatedSubscriptionNumber
*/
@javax.annotation.Nullable
public String getRelatedSubscriptionNumber() {
return relatedSubscriptionNumber;
}
public void setRelatedSubscriptionNumber(String relatedSubscriptionNumber) {
this.relatedSubscriptionNumber = relatedSubscriptionNumber;
}
public ExpandedOrderLineItem transactionStartDate(LocalDate transactionStartDate) {
this.transactionStartDate = transactionStartDate;
return this;
}
/**
* Get transactionStartDate
* @return transactionStartDate
*/
@javax.annotation.Nullable
public LocalDate getTransactionStartDate() {
return transactionStartDate;
}
public void setTransactionStartDate(LocalDate transactionStartDate) {
this.transactionStartDate = transactionStartDate;
}
public ExpandedOrderLineItem transactionEndDate(LocalDate transactionEndDate) {
this.transactionEndDate = transactionEndDate;
return this;
}
/**
* Get transactionEndDate
* @return transactionEndDate
*/
@javax.annotation.Nullable
public LocalDate getTransactionEndDate() {
return transactionEndDate;
}
public void setTransactionEndDate(LocalDate transactionEndDate) {
this.transactionEndDate = transactionEndDate;
}
public ExpandedOrderLineItem excludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
return this;
}
/**
* Get excludeItemBookingFromRevenueAccounting
* @return excludeItemBookingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBookingFromRevenueAccounting() {
return excludeItemBookingFromRevenueAccounting;
}
public void setExcludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
}
public ExpandedOrderLineItem excludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
return this;
}
/**
* Get excludeItemBillingFromRevenueAccounting
* @return excludeItemBillingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBillingFromRevenueAccounting() {
return excludeItemBillingFromRevenueAccounting;
}
public void setExcludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
}
public ExpandedOrderLineItem isAllocationEligible(Boolean isAllocationEligible) {
this.isAllocationEligible = isAllocationEligible;
return this;
}
/**
* Get isAllocationEligible
* @return isAllocationEligible
*/
@javax.annotation.Nullable
public Boolean getIsAllocationEligible() {
return isAllocationEligible;
}
public void setIsAllocationEligible(Boolean isAllocationEligible) {
this.isAllocationEligible = isAllocationEligible;
}
public ExpandedOrderLineItem isUnbilled(Boolean isUnbilled) {
this.isUnbilled = isUnbilled;
return this;
}
/**
* Get isUnbilled
* @return isUnbilled
*/
@javax.annotation.Nullable
public Boolean getIsUnbilled() {
return isUnbilled;
}
public void setIsUnbilled(Boolean isUnbilled) {
this.isUnbilled = isUnbilled;
}
public ExpandedOrderLineItem revenueRecognitionTiming(String revenueRecognitionTiming) {
this.revenueRecognitionTiming = revenueRecognitionTiming;
return this;
}
/**
* Get revenueRecognitionTiming
* @return revenueRecognitionTiming
*/
@javax.annotation.Nullable
public String getRevenueRecognitionTiming() {
return revenueRecognitionTiming;
}
public void setRevenueRecognitionTiming(String revenueRecognitionTiming) {
this.revenueRecognitionTiming = revenueRecognitionTiming;
}
public ExpandedOrderLineItem revenueAmortizationMethod(String revenueAmortizationMethod) {
this.revenueAmortizationMethod = revenueAmortizationMethod;
return this;
}
/**
* Get revenueAmortizationMethod
* @return revenueAmortizationMethod
*/
@javax.annotation.Nullable
public String getRevenueAmortizationMethod() {
return revenueAmortizationMethod;
}
public void setRevenueAmortizationMethod(String revenueAmortizationMethod) {
this.revenueAmortizationMethod = revenueAmortizationMethod;
}
public ExpandedOrderLineItem originalOrderDate(LocalDate originalOrderDate) {
this.originalOrderDate = originalOrderDate;
return this;
}
/**
* Get originalOrderDate
* @return originalOrderDate
*/
@javax.annotation.Nullable
public LocalDate getOriginalOrderDate() {
return originalOrderDate;
}
public void setOriginalOrderDate(LocalDate originalOrderDate) {
this.originalOrderDate = originalOrderDate;
}
public ExpandedOrderLineItem amendedByOrderOn(LocalDate amendedByOrderOn) {
this.amendedByOrderOn = amendedByOrderOn;
return this;
}
/**
* Get amendedByOrderOn
* @return amendedByOrderOn
*/
@javax.annotation.Nullable
public LocalDate getAmendedByOrderOn() {
return amendedByOrderOn;
}
public void setAmendedByOrderOn(LocalDate amendedByOrderOn) {
this.amendedByOrderOn = amendedByOrderOn;
}
public ExpandedOrderLineItem itemCategory(String itemCategory) {
this.itemCategory = itemCategory;
return this;
}
/**
* Get itemCategory
* @return itemCategory
*/
@javax.annotation.Nullable
public String getItemCategory() {
return itemCategory;
}
public void setItemCategory(String itemCategory) {
this.itemCategory = itemCategory;
}
public ExpandedOrderLineItem originalOrderId(String originalOrderId) {
this.originalOrderId = originalOrderId;
return this;
}
/**
* Get originalOrderId
* @return originalOrderId
*/
@javax.annotation.Nullable
public String getOriginalOrderId() {
return originalOrderId;
}
public void setOriginalOrderId(String originalOrderId) {
this.originalOrderId = originalOrderId;
}
public ExpandedOrderLineItem originalOrderNumber(String originalOrderNumber) {
this.originalOrderNumber = originalOrderNumber;
return this;
}
/**
* Get originalOrderNumber
* @return originalOrderNumber
*/
@javax.annotation.Nullable
public String getOriginalOrderNumber() {
return originalOrderNumber;
}
public void setOriginalOrderNumber(String originalOrderNumber) {
this.originalOrderNumber = originalOrderNumber;
}
public ExpandedOrderLineItem originalOrderLineItemId(String originalOrderLineItemId) {
this.originalOrderLineItemId = originalOrderLineItemId;
return this;
}
/**
* Get originalOrderLineItemId
* @return originalOrderLineItemId
*/
@javax.annotation.Nullable
public String getOriginalOrderLineItemId() {
return originalOrderLineItemId;
}
public void setOriginalOrderLineItemId(String originalOrderLineItemId) {
this.originalOrderLineItemId = originalOrderLineItemId;
}
public ExpandedOrderLineItem originalOrderLineItemNumber(String originalOrderLineItemNumber) {
this.originalOrderLineItemNumber = originalOrderLineItemNumber;
return this;
}
/**
* Get originalOrderLineItemNumber
* @return originalOrderLineItemNumber
*/
@javax.annotation.Nullable
public String getOriginalOrderLineItemNumber() {
return originalOrderLineItemNumber;
}
public void setOriginalOrderLineItemNumber(String originalOrderLineItemNumber) {
this.originalOrderLineItemNumber = originalOrderLineItemNumber;
}
public ExpandedOrderLineItem quantityFulfilled(BigDecimal quantityFulfilled) {
this.quantityFulfilled = quantityFulfilled;
return this;
}
/**
* Get quantityFulfilled
* @return quantityFulfilled
*/
@javax.annotation.Nullable
public BigDecimal getQuantityFulfilled() {
return quantityFulfilled;
}
public void setQuantityFulfilled(BigDecimal quantityFulfilled) {
this.quantityFulfilled = quantityFulfilled;
}
public ExpandedOrderLineItem quantityPendingFulfillment(BigDecimal quantityPendingFulfillment) {
this.quantityPendingFulfillment = quantityPendingFulfillment;
return this;
}
/**
* Get quantityPendingFulfillment
* @return quantityPendingFulfillment
*/
@javax.annotation.Nullable
public BigDecimal getQuantityPendingFulfillment() {
return quantityPendingFulfillment;
}
public void setQuantityPendingFulfillment(BigDecimal quantityPendingFulfillment) {
this.quantityPendingFulfillment = quantityPendingFulfillment;
}
public ExpandedOrderLineItem quantityAvailableForReturn(BigDecimal quantityAvailableForReturn) {
this.quantityAvailableForReturn = quantityAvailableForReturn;
return this;
}
/**
* Get quantityAvailableForReturn
* @return quantityAvailableForReturn
*/
@javax.annotation.Nullable
public BigDecimal getQuantityAvailableForReturn() {
return quantityAvailableForReturn;
}
public void setQuantityAvailableForReturn(BigDecimal quantityAvailableForReturn) {
this.quantityAvailableForReturn = quantityAvailableForReturn;
}
public ExpandedOrderLineItem requiresFulfillment(Boolean requiresFulfillment) {
this.requiresFulfillment = requiresFulfillment;
return this;
}
/**
* Get requiresFulfillment
* @return requiresFulfillment
*/
@javax.annotation.Nullable
public Boolean getRequiresFulfillment() {
return requiresFulfillment;
}
public void setRequiresFulfillment(Boolean requiresFulfillment) {
this.requiresFulfillment = requiresFulfillment;
}
public ExpandedOrderLineItem billingRule(String billingRule) {
this.billingRule = billingRule;
return this;
}
/**
* Get billingRule
* @return billingRule
*/
@javax.annotation.Nullable
public String getBillingRule() {
return billingRule;
}
public void setBillingRule(String billingRule) {
this.billingRule = billingRule;
}
public ExpandedOrderLineItem inlineDiscountPerUnit(BigDecimal inlineDiscountPerUnit) {
this.inlineDiscountPerUnit = inlineDiscountPerUnit;
return this;
}
/**
* Get inlineDiscountPerUnit
* @return inlineDiscountPerUnit
*/
@javax.annotation.Nullable
public BigDecimal getInlineDiscountPerUnit() {
return inlineDiscountPerUnit;
}
public void setInlineDiscountPerUnit(BigDecimal inlineDiscountPerUnit) {
this.inlineDiscountPerUnit = inlineDiscountPerUnit;
}
public ExpandedOrderLineItem inlineDiscountType(String inlineDiscountType) {
this.inlineDiscountType = inlineDiscountType;
return this;
}
/**
* Get inlineDiscountType
* @return inlineDiscountType
*/
@javax.annotation.Nullable
public String getInlineDiscountType() {
return inlineDiscountType;
}
public void setInlineDiscountType(String inlineDiscountType) {
this.inlineDiscountType = inlineDiscountType;
}
public ExpandedOrderLineItem discount(BigDecimal discount) {
this.discount = discount;
return this;
}
/**
* Get discount
* @return discount
*/
@javax.annotation.Nullable
public BigDecimal getDiscount() {
return discount;
}
public void setDiscount(BigDecimal discount) {
this.discount = discount;
}
public ExpandedOrderLineItem recognizedRevenueAccountingCodeId(String recognizedRevenueAccountingCodeId) {
this.recognizedRevenueAccountingCodeId = recognizedRevenueAccountingCodeId;
return this;
}
/**
* Get recognizedRevenueAccountingCodeId
* @return recognizedRevenueAccountingCodeId
*/
@javax.annotation.Nullable
public String getRecognizedRevenueAccountingCodeId() {
return recognizedRevenueAccountingCodeId;
}
public void setRecognizedRevenueAccountingCodeId(String recognizedRevenueAccountingCodeId) {
this.recognizedRevenueAccountingCodeId = recognizedRevenueAccountingCodeId;
}
public ExpandedOrderLineItem deferredRevenueAccountingCodeId(String deferredRevenueAccountingCodeId) {
this.deferredRevenueAccountingCodeId = deferredRevenueAccountingCodeId;
return this;
}
/**
* Get deferredRevenueAccountingCodeId
* @return deferredRevenueAccountingCodeId
*/
@javax.annotation.Nullable
public String getDeferredRevenueAccountingCodeId() {
return deferredRevenueAccountingCodeId;
}
public void setDeferredRevenueAccountingCodeId(String deferredRevenueAccountingCodeId) {
this.deferredRevenueAccountingCodeId = deferredRevenueAccountingCodeId;
}
public ExpandedOrderLineItem contractAssetAccountingCodeId(String contractAssetAccountingCodeId) {
this.contractAssetAccountingCodeId = contractAssetAccountingCodeId;
return this;
}
/**
* Get contractAssetAccountingCodeId
* @return contractAssetAccountingCodeId
*/
@javax.annotation.Nullable
public String getContractAssetAccountingCodeId() {
return contractAssetAccountingCodeId;
}
public void setContractAssetAccountingCodeId(String contractAssetAccountingCodeId) {
this.contractAssetAccountingCodeId = contractAssetAccountingCodeId;
}
public ExpandedOrderLineItem contractLiabilityAccountingCodeId(String contractLiabilityAccountingCodeId) {
this.contractLiabilityAccountingCodeId = contractLiabilityAccountingCodeId;
return this;
}
/**
* Get contractLiabilityAccountingCodeId
* @return contractLiabilityAccountingCodeId
*/
@javax.annotation.Nullable
public String getContractLiabilityAccountingCodeId() {
return contractLiabilityAccountingCodeId;
}
public void setContractLiabilityAccountingCodeId(String contractLiabilityAccountingCodeId) {
this.contractLiabilityAccountingCodeId = contractLiabilityAccountingCodeId;
}
public ExpandedOrderLineItem contractRecognizedRevenueAccountingCodeId(String contractRecognizedRevenueAccountingCodeId) {
this.contractRecognizedRevenueAccountingCodeId = contractRecognizedRevenueAccountingCodeId;
return this;
}
/**
* Get contractRecognizedRevenueAccountingCodeId
* @return contractRecognizedRevenueAccountingCodeId
*/
@javax.annotation.Nullable
public String getContractRecognizedRevenueAccountingCodeId() {
return contractRecognizedRevenueAccountingCodeId;
}
public void setContractRecognizedRevenueAccountingCodeId(String contractRecognizedRevenueAccountingCodeId) {
this.contractRecognizedRevenueAccountingCodeId = contractRecognizedRevenueAccountingCodeId;
}
public ExpandedOrderLineItem unbilledReceivablesAccountingCodeId(String unbilledReceivablesAccountingCodeId) {
this.unbilledReceivablesAccountingCodeId = unbilledReceivablesAccountingCodeId;
return this;
}
/**
* Get unbilledReceivablesAccountingCodeId
* @return unbilledReceivablesAccountingCodeId
*/
@javax.annotation.Nullable
public String getUnbilledReceivablesAccountingCodeId() {
return unbilledReceivablesAccountingCodeId;
}
public void setUnbilledReceivablesAccountingCodeId(String unbilledReceivablesAccountingCodeId) {
this.unbilledReceivablesAccountingCodeId = unbilledReceivablesAccountingCodeId;
}
public ExpandedOrderLineItem adjustmentRevenueAccountingCodeId(String adjustmentRevenueAccountingCodeId) {
this.adjustmentRevenueAccountingCodeId = adjustmentRevenueAccountingCodeId;
return this;
}
/**
* Get adjustmentRevenueAccountingCodeId
* @return adjustmentRevenueAccountingCodeId
*/
@javax.annotation.Nullable
public String getAdjustmentRevenueAccountingCodeId() {
return adjustmentRevenueAccountingCodeId;
}
public void setAdjustmentRevenueAccountingCodeId(String adjustmentRevenueAccountingCodeId) {
this.adjustmentRevenueAccountingCodeId = adjustmentRevenueAccountingCodeId;
}
public ExpandedOrderLineItem adjustmentLiabilityAccountingCodeId(String adjustmentLiabilityAccountingCodeId) {
this.adjustmentLiabilityAccountingCodeId = adjustmentLiabilityAccountingCodeId;
return this;
}
/**
* Get adjustmentLiabilityAccountingCodeId
* @return adjustmentLiabilityAccountingCodeId
*/
@javax.annotation.Nullable
public String getAdjustmentLiabilityAccountingCodeId() {
return adjustmentLiabilityAccountingCodeId;
}
public void setAdjustmentLiabilityAccountingCodeId(String adjustmentLiabilityAccountingCodeId) {
this.adjustmentLiabilityAccountingCodeId = adjustmentLiabilityAccountingCodeId;
}
public ExpandedOrderLineItem invoiceItems(List invoiceItems) {
this.invoiceItems = invoiceItems;
return this;
}
public ExpandedOrderLineItem addInvoiceItemsItem(ExpandedInvoiceItem invoiceItemsItem) {
if (this.invoiceItems == null) {
this.invoiceItems = new ArrayList<>();
}
this.invoiceItems.add(invoiceItemsItem);
return this;
}
/**
* Get invoiceItems
* @return invoiceItems
*/
@javax.annotation.Nullable
public List getInvoiceItems() {
return invoiceItems;
}
public void setInvoiceItems(List invoiceItems) {
this.invoiceItems = invoiceItems;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the ExpandedOrderLineItem instance itself
*/
public ExpandedOrderLineItem putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ExpandedOrderLineItem expandedOrderLineItem = (ExpandedOrderLineItem) o;
return Objects.equals(this.id, expandedOrderLineItem.id) &&
Objects.equals(this.createdById, expandedOrderLineItem.createdById) &&
Objects.equals(this.createdDate, expandedOrderLineItem.createdDate) &&
Objects.equals(this.updatedById, expandedOrderLineItem.updatedById) &&
Objects.equals(this.updatedDate, expandedOrderLineItem.updatedDate) &&
Objects.equals(this.accountingCode, expandedOrderLineItem.accountingCode) &&
Objects.equals(this.billTargetDate, expandedOrderLineItem.billTargetDate) &&
Objects.equals(this.currency, expandedOrderLineItem.currency) &&
Objects.equals(this.amountPerUnit, expandedOrderLineItem.amountPerUnit) &&
Objects.equals(this.description, expandedOrderLineItem.description) &&
Objects.equals(this.amount, expandedOrderLineItem.amount) &&
Objects.equals(this.amountWithoutTax, expandedOrderLineItem.amountWithoutTax) &&
Objects.equals(this.invoiceGroupNumber, expandedOrderLineItem.invoiceGroupNumber) &&
Objects.equals(this.itemName, expandedOrderLineItem.itemName) &&
Objects.equals(this.itemNumber, expandedOrderLineItem.itemNumber) &&
Objects.equals(this.itemState, expandedOrderLineItem.itemState) &&
Objects.equals(this.itemType, expandedOrderLineItem.itemType) &&
Objects.equals(this.listPricePerUnit, expandedOrderLineItem.listPricePerUnit) &&
Objects.equals(this.listPrice, expandedOrderLineItem.listPrice) &&
Objects.equals(this.orderId, expandedOrderLineItem.orderId) &&
Objects.equals(this.productCode, expandedOrderLineItem.productCode) &&
Objects.equals(this.purchaseOrderNumber, expandedOrderLineItem.purchaseOrderNumber) &&
Objects.equals(this.quantity, expandedOrderLineItem.quantity) &&
Objects.equals(this.revenueRecognitionRule, expandedOrderLineItem.revenueRecognitionRule) &&
Objects.equals(this.productRatePlanChargeId, expandedOrderLineItem.productRatePlanChargeId) &&
Objects.equals(this.billToId, expandedOrderLineItem.billToId) &&
Objects.equals(this.billToSnapshotId, expandedOrderLineItem.billToSnapshotId) &&
Objects.equals(this.soldTo, expandedOrderLineItem.soldTo) &&
Objects.equals(this.soldToSnapshotId, expandedOrderLineItem.soldToSnapshotId) &&
Objects.equals(this.soldToOrderContactId, expandedOrderLineItem.soldToOrderContactId) &&
Objects.equals(this.ownerAccountId, expandedOrderLineItem.ownerAccountId) &&
Objects.equals(this.invoiceOwnerAccountId, expandedOrderLineItem.invoiceOwnerAccountId) &&
Objects.equals(this.taxCode, expandedOrderLineItem.taxCode) &&
Objects.equals(this.taxMode, expandedOrderLineItem.taxMode) &&
Objects.equals(this.transactionDate, expandedOrderLineItem.transactionDate) &&
Objects.equals(this.uOM, expandedOrderLineItem.uOM) &&
Objects.equals(this.relatedSubscriptionNumber, expandedOrderLineItem.relatedSubscriptionNumber) &&
Objects.equals(this.transactionStartDate, expandedOrderLineItem.transactionStartDate) &&
Objects.equals(this.transactionEndDate, expandedOrderLineItem.transactionEndDate) &&
Objects.equals(this.excludeItemBookingFromRevenueAccounting, expandedOrderLineItem.excludeItemBookingFromRevenueAccounting) &&
Objects.equals(this.excludeItemBillingFromRevenueAccounting, expandedOrderLineItem.excludeItemBillingFromRevenueAccounting) &&
Objects.equals(this.isAllocationEligible, expandedOrderLineItem.isAllocationEligible) &&
Objects.equals(this.isUnbilled, expandedOrderLineItem.isUnbilled) &&
Objects.equals(this.revenueRecognitionTiming, expandedOrderLineItem.revenueRecognitionTiming) &&
Objects.equals(this.revenueAmortizationMethod, expandedOrderLineItem.revenueAmortizationMethod) &&
Objects.equals(this.originalOrderDate, expandedOrderLineItem.originalOrderDate) &&
Objects.equals(this.amendedByOrderOn, expandedOrderLineItem.amendedByOrderOn) &&
Objects.equals(this.itemCategory, expandedOrderLineItem.itemCategory) &&
Objects.equals(this.originalOrderId, expandedOrderLineItem.originalOrderId) &&
Objects.equals(this.originalOrderNumber, expandedOrderLineItem.originalOrderNumber) &&
Objects.equals(this.originalOrderLineItemId, expandedOrderLineItem.originalOrderLineItemId) &&
Objects.equals(this.originalOrderLineItemNumber, expandedOrderLineItem.originalOrderLineItemNumber) &&
Objects.equals(this.quantityFulfilled, expandedOrderLineItem.quantityFulfilled) &&
Objects.equals(this.quantityPendingFulfillment, expandedOrderLineItem.quantityPendingFulfillment) &&
Objects.equals(this.quantityAvailableForReturn, expandedOrderLineItem.quantityAvailableForReturn) &&
Objects.equals(this.requiresFulfillment, expandedOrderLineItem.requiresFulfillment) &&
Objects.equals(this.billingRule, expandedOrderLineItem.billingRule) &&
Objects.equals(this.inlineDiscountPerUnit, expandedOrderLineItem.inlineDiscountPerUnit) &&
Objects.equals(this.inlineDiscountType, expandedOrderLineItem.inlineDiscountType) &&
Objects.equals(this.discount, expandedOrderLineItem.discount) &&
Objects.equals(this.recognizedRevenueAccountingCodeId, expandedOrderLineItem.recognizedRevenueAccountingCodeId) &&
Objects.equals(this.deferredRevenueAccountingCodeId, expandedOrderLineItem.deferredRevenueAccountingCodeId) &&
Objects.equals(this.contractAssetAccountingCodeId, expandedOrderLineItem.contractAssetAccountingCodeId) &&
Objects.equals(this.contractLiabilityAccountingCodeId, expandedOrderLineItem.contractLiabilityAccountingCodeId) &&
Objects.equals(this.contractRecognizedRevenueAccountingCodeId, expandedOrderLineItem.contractRecognizedRevenueAccountingCodeId) &&
Objects.equals(this.unbilledReceivablesAccountingCodeId, expandedOrderLineItem.unbilledReceivablesAccountingCodeId) &&
Objects.equals(this.adjustmentRevenueAccountingCodeId, expandedOrderLineItem.adjustmentRevenueAccountingCodeId) &&
Objects.equals(this.adjustmentLiabilityAccountingCodeId, expandedOrderLineItem.adjustmentLiabilityAccountingCodeId) &&
Objects.equals(this.invoiceItems, expandedOrderLineItem.invoiceItems)&&
Objects.equals(this.additionalProperties, expandedOrderLineItem.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(id, createdById, createdDate, updatedById, updatedDate, accountingCode, billTargetDate, currency, amountPerUnit, description, amount, amountWithoutTax, invoiceGroupNumber, itemName, itemNumber, itemState, itemType, listPricePerUnit, listPrice, orderId, productCode, purchaseOrderNumber, quantity, revenueRecognitionRule, productRatePlanChargeId, billToId, billToSnapshotId, soldTo, soldToSnapshotId, soldToOrderContactId, ownerAccountId, invoiceOwnerAccountId, taxCode, taxMode, transactionDate, uOM, relatedSubscriptionNumber, transactionStartDate, transactionEndDate, excludeItemBookingFromRevenueAccounting, excludeItemBillingFromRevenueAccounting, isAllocationEligible, isUnbilled, revenueRecognitionTiming, revenueAmortizationMethod, originalOrderDate, amendedByOrderOn, itemCategory, originalOrderId, originalOrderNumber, originalOrderLineItemId, originalOrderLineItemNumber, quantityFulfilled, quantityPendingFulfillment, quantityAvailableForReturn, requiresFulfillment, billingRule, inlineDiscountPerUnit, inlineDiscountType, discount, recognizedRevenueAccountingCodeId, deferredRevenueAccountingCodeId, contractAssetAccountingCodeId, contractLiabilityAccountingCodeId, contractRecognizedRevenueAccountingCodeId, unbilledReceivablesAccountingCodeId, adjustmentRevenueAccountingCodeId, adjustmentLiabilityAccountingCodeId, invoiceItems, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ExpandedOrderLineItem {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" accountingCode: ").append(toIndentedString(accountingCode)).append("\n");
sb.append(" billTargetDate: ").append(toIndentedString(billTargetDate)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" amountPerUnit: ").append(toIndentedString(amountPerUnit)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" amountWithoutTax: ").append(toIndentedString(amountWithoutTax)).append("\n");
sb.append(" invoiceGroupNumber: ").append(toIndentedString(invoiceGroupNumber)).append("\n");
sb.append(" itemName: ").append(toIndentedString(itemName)).append("\n");
sb.append(" itemNumber: ").append(toIndentedString(itemNumber)).append("\n");
sb.append(" itemState: ").append(toIndentedString(itemState)).append("\n");
sb.append(" itemType: ").append(toIndentedString(itemType)).append("\n");
sb.append(" listPricePerUnit: ").append(toIndentedString(listPricePerUnit)).append("\n");
sb.append(" listPrice: ").append(toIndentedString(listPrice)).append("\n");
sb.append(" orderId: ").append(toIndentedString(orderId)).append("\n");
sb.append(" productCode: ").append(toIndentedString(productCode)).append("\n");
sb.append(" purchaseOrderNumber: ").append(toIndentedString(purchaseOrderNumber)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" revenueRecognitionRule: ").append(toIndentedString(revenueRecognitionRule)).append("\n");
sb.append(" productRatePlanChargeId: ").append(toIndentedString(productRatePlanChargeId)).append("\n");
sb.append(" billToId: ").append(toIndentedString(billToId)).append("\n");
sb.append(" billToSnapshotId: ").append(toIndentedString(billToSnapshotId)).append("\n");
sb.append(" soldTo: ").append(toIndentedString(soldTo)).append("\n");
sb.append(" soldToSnapshotId: ").append(toIndentedString(soldToSnapshotId)).append("\n");
sb.append(" soldToOrderContactId: ").append(toIndentedString(soldToOrderContactId)).append("\n");
sb.append(" ownerAccountId: ").append(toIndentedString(ownerAccountId)).append("\n");
sb.append(" invoiceOwnerAccountId: ").append(toIndentedString(invoiceOwnerAccountId)).append("\n");
sb.append(" taxCode: ").append(toIndentedString(taxCode)).append("\n");
sb.append(" taxMode: ").append(toIndentedString(taxMode)).append("\n");
sb.append(" transactionDate: ").append(toIndentedString(transactionDate)).append("\n");
sb.append(" uOM: ").append(toIndentedString(uOM)).append("\n");
sb.append(" relatedSubscriptionNumber: ").append(toIndentedString(relatedSubscriptionNumber)).append("\n");
sb.append(" transactionStartDate: ").append(toIndentedString(transactionStartDate)).append("\n");
sb.append(" transactionEndDate: ").append(toIndentedString(transactionEndDate)).append("\n");
sb.append(" excludeItemBookingFromRevenueAccounting: ").append(toIndentedString(excludeItemBookingFromRevenueAccounting)).append("\n");
sb.append(" excludeItemBillingFromRevenueAccounting: ").append(toIndentedString(excludeItemBillingFromRevenueAccounting)).append("\n");
sb.append(" isAllocationEligible: ").append(toIndentedString(isAllocationEligible)).append("\n");
sb.append(" isUnbilled: ").append(toIndentedString(isUnbilled)).append("\n");
sb.append(" revenueRecognitionTiming: ").append(toIndentedString(revenueRecognitionTiming)).append("\n");
sb.append(" revenueAmortizationMethod: ").append(toIndentedString(revenueAmortizationMethod)).append("\n");
sb.append(" originalOrderDate: ").append(toIndentedString(originalOrderDate)).append("\n");
sb.append(" amendedByOrderOn: ").append(toIndentedString(amendedByOrderOn)).append("\n");
sb.append(" itemCategory: ").append(toIndentedString(itemCategory)).append("\n");
sb.append(" originalOrderId: ").append(toIndentedString(originalOrderId)).append("\n");
sb.append(" originalOrderNumber: ").append(toIndentedString(originalOrderNumber)).append("\n");
sb.append(" originalOrderLineItemId: ").append(toIndentedString(originalOrderLineItemId)).append("\n");
sb.append(" originalOrderLineItemNumber: ").append(toIndentedString(originalOrderLineItemNumber)).append("\n");
sb.append(" quantityFulfilled: ").append(toIndentedString(quantityFulfilled)).append("\n");
sb.append(" quantityPendingFulfillment: ").append(toIndentedString(quantityPendingFulfillment)).append("\n");
sb.append(" quantityAvailableForReturn: ").append(toIndentedString(quantityAvailableForReturn)).append("\n");
sb.append(" requiresFulfillment: ").append(toIndentedString(requiresFulfillment)).append("\n");
sb.append(" billingRule: ").append(toIndentedString(billingRule)).append("\n");
sb.append(" inlineDiscountPerUnit: ").append(toIndentedString(inlineDiscountPerUnit)).append("\n");
sb.append(" inlineDiscountType: ").append(toIndentedString(inlineDiscountType)).append("\n");
sb.append(" discount: ").append(toIndentedString(discount)).append("\n");
sb.append(" recognizedRevenueAccountingCodeId: ").append(toIndentedString(recognizedRevenueAccountingCodeId)).append("\n");
sb.append(" deferredRevenueAccountingCodeId: ").append(toIndentedString(deferredRevenueAccountingCodeId)).append("\n");
sb.append(" contractAssetAccountingCodeId: ").append(toIndentedString(contractAssetAccountingCodeId)).append("\n");
sb.append(" contractLiabilityAccountingCodeId: ").append(toIndentedString(contractLiabilityAccountingCodeId)).append("\n");
sb.append(" contractRecognizedRevenueAccountingCodeId: ").append(toIndentedString(contractRecognizedRevenueAccountingCodeId)).append("\n");
sb.append(" unbilledReceivablesAccountingCodeId: ").append(toIndentedString(unbilledReceivablesAccountingCodeId)).append("\n");
sb.append(" adjustmentRevenueAccountingCodeId: ").append(toIndentedString(adjustmentRevenueAccountingCodeId)).append("\n");
sb.append(" adjustmentLiabilityAccountingCodeId: ").append(toIndentedString(adjustmentLiabilityAccountingCodeId)).append("\n");
sb.append(" invoiceItems: ").append(toIndentedString(invoiceItems)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("id");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
openapiFields.add("accountingCode");
openapiFields.add("billTargetDate");
openapiFields.add("currency");
openapiFields.add("amountPerUnit");
openapiFields.add("description");
openapiFields.add("amount");
openapiFields.add("amountWithoutTax");
openapiFields.add("invoiceGroupNumber");
openapiFields.add("itemName");
openapiFields.add("itemNumber");
openapiFields.add("itemState");
openapiFields.add("itemType");
openapiFields.add("listPricePerUnit");
openapiFields.add("listPrice");
openapiFields.add("orderId");
openapiFields.add("productCode");
openapiFields.add("purchaseOrderNumber");
openapiFields.add("quantity");
openapiFields.add("revenueRecognitionRule");
openapiFields.add("productRatePlanChargeId");
openapiFields.add("billToId");
openapiFields.add("billToSnapshotId");
openapiFields.add("soldTo");
openapiFields.add("soldToSnapshotId");
openapiFields.add("soldToOrderContactId");
openapiFields.add("ownerAccountId");
openapiFields.add("invoiceOwnerAccountId");
openapiFields.add("taxCode");
openapiFields.add("taxMode");
openapiFields.add("transactionDate");
openapiFields.add("uOM");
openapiFields.add("relatedSubscriptionNumber");
openapiFields.add("transactionStartDate");
openapiFields.add("transactionEndDate");
openapiFields.add("excludeItemBookingFromRevenueAccounting");
openapiFields.add("excludeItemBillingFromRevenueAccounting");
openapiFields.add("isAllocationEligible");
openapiFields.add("isUnbilled");
openapiFields.add("revenueRecognitionTiming");
openapiFields.add("revenueAmortizationMethod");
openapiFields.add("originalOrderDate");
openapiFields.add("amendedByOrderOn");
openapiFields.add("itemCategory");
openapiFields.add("originalOrderId");
openapiFields.add("originalOrderNumber");
openapiFields.add("originalOrderLineItemId");
openapiFields.add("originalOrderLineItemNumber");
openapiFields.add("quantityFulfilled");
openapiFields.add("quantityPendingFulfillment");
openapiFields.add("quantityAvailableForReturn");
openapiFields.add("requiresFulfillment");
openapiFields.add("billingRule");
openapiFields.add("inlineDiscountPerUnit");
openapiFields.add("inlineDiscountType");
openapiFields.add("discount");
openapiFields.add("recognizedRevenueAccountingCodeId");
openapiFields.add("deferredRevenueAccountingCodeId");
openapiFields.add("contractAssetAccountingCodeId");
openapiFields.add("contractLiabilityAccountingCodeId");
openapiFields.add("contractRecognizedRevenueAccountingCodeId");
openapiFields.add("unbilledReceivablesAccountingCodeId");
openapiFields.add("adjustmentRevenueAccountingCodeId");
openapiFields.add("adjustmentLiabilityAccountingCodeId");
openapiFields.add("invoiceItems");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to ExpandedOrderLineItem
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!ExpandedOrderLineItem.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in ExpandedOrderLineItem is not found in the empty JSON string", ExpandedOrderLineItem.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
if ((jsonObj.get("accountingCode") != null && !jsonObj.get("accountingCode").isJsonNull()) && !jsonObj.get("accountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountingCode").toString()));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if ((jsonObj.get("invoiceGroupNumber") != null && !jsonObj.get("invoiceGroupNumber").isJsonNull()) && !jsonObj.get("invoiceGroupNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceGroupNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceGroupNumber").toString()));
}
if ((jsonObj.get("itemName") != null && !jsonObj.get("itemName").isJsonNull()) && !jsonObj.get("itemName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `itemName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("itemName").toString()));
}
if ((jsonObj.get("itemNumber") != null && !jsonObj.get("itemNumber").isJsonNull()) && !jsonObj.get("itemNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `itemNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("itemNumber").toString()));
}
if ((jsonObj.get("itemState") != null && !jsonObj.get("itemState").isJsonNull()) && !jsonObj.get("itemState").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `itemState` to be a primitive type in the JSON string but got `%s`", jsonObj.get("itemState").toString()));
}
if ((jsonObj.get("itemType") != null && !jsonObj.get("itemType").isJsonNull()) && !jsonObj.get("itemType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `itemType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("itemType").toString()));
}
if ((jsonObj.get("orderId") != null && !jsonObj.get("orderId").isJsonNull()) && !jsonObj.get("orderId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `orderId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("orderId").toString()));
}
if ((jsonObj.get("productCode") != null && !jsonObj.get("productCode").isJsonNull()) && !jsonObj.get("productCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productCode").toString()));
}
if ((jsonObj.get("purchaseOrderNumber") != null && !jsonObj.get("purchaseOrderNumber").isJsonNull()) && !jsonObj.get("purchaseOrderNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `purchaseOrderNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("purchaseOrderNumber").toString()));
}
if ((jsonObj.get("revenueRecognitionRule") != null && !jsonObj.get("revenueRecognitionRule").isJsonNull()) && !jsonObj.get("revenueRecognitionRule").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueRecognitionRule` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueRecognitionRule").toString()));
}
if ((jsonObj.get("productRatePlanChargeId") != null && !jsonObj.get("productRatePlanChargeId").isJsonNull()) && !jsonObj.get("productRatePlanChargeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanChargeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productRatePlanChargeId").toString()));
}
if ((jsonObj.get("billToId") != null && !jsonObj.get("billToId").isJsonNull()) && !jsonObj.get("billToId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billToId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billToId").toString()));
}
if ((jsonObj.get("billToSnapshotId") != null && !jsonObj.get("billToSnapshotId").isJsonNull()) && !jsonObj.get("billToSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billToSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billToSnapshotId").toString()));
}
if ((jsonObj.get("soldTo") != null && !jsonObj.get("soldTo").isJsonNull()) && !jsonObj.get("soldTo").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldTo` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldTo").toString()));
}
if ((jsonObj.get("soldToSnapshotId") != null && !jsonObj.get("soldToSnapshotId").isJsonNull()) && !jsonObj.get("soldToSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToSnapshotId").toString()));
}
if ((jsonObj.get("soldToOrderContactId") != null && !jsonObj.get("soldToOrderContactId").isJsonNull()) && !jsonObj.get("soldToOrderContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToOrderContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToOrderContactId").toString()));
}
if ((jsonObj.get("ownerAccountId") != null && !jsonObj.get("ownerAccountId").isJsonNull()) && !jsonObj.get("ownerAccountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ownerAccountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ownerAccountId").toString()));
}
if ((jsonObj.get("invoiceOwnerAccountId") != null && !jsonObj.get("invoiceOwnerAccountId").isJsonNull()) && !jsonObj.get("invoiceOwnerAccountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceOwnerAccountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceOwnerAccountId").toString()));
}
if ((jsonObj.get("taxCode") != null && !jsonObj.get("taxCode").isJsonNull()) && !jsonObj.get("taxCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxCode").toString()));
}
if ((jsonObj.get("taxMode") != null && !jsonObj.get("taxMode").isJsonNull()) && !jsonObj.get("taxMode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxMode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxMode").toString()));
}
if ((jsonObj.get("uOM") != null && !jsonObj.get("uOM").isJsonNull()) && !jsonObj.get("uOM").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `uOM` to be a primitive type in the JSON string but got `%s`", jsonObj.get("uOM").toString()));
}
if ((jsonObj.get("relatedSubscriptionNumber") != null && !jsonObj.get("relatedSubscriptionNumber").isJsonNull()) && !jsonObj.get("relatedSubscriptionNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `relatedSubscriptionNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("relatedSubscriptionNumber").toString()));
}
if ((jsonObj.get("revenueRecognitionTiming") != null && !jsonObj.get("revenueRecognitionTiming").isJsonNull()) && !jsonObj.get("revenueRecognitionTiming").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueRecognitionTiming` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueRecognitionTiming").toString()));
}
if ((jsonObj.get("revenueAmortizationMethod") != null && !jsonObj.get("revenueAmortizationMethod").isJsonNull()) && !jsonObj.get("revenueAmortizationMethod").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueAmortizationMethod` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueAmortizationMethod").toString()));
}
if ((jsonObj.get("itemCategory") != null && !jsonObj.get("itemCategory").isJsonNull()) && !jsonObj.get("itemCategory").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `itemCategory` to be a primitive type in the JSON string but got `%s`", jsonObj.get("itemCategory").toString()));
}
if ((jsonObj.get("originalOrderId") != null && !jsonObj.get("originalOrderId").isJsonNull()) && !jsonObj.get("originalOrderId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `originalOrderId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("originalOrderId").toString()));
}
if ((jsonObj.get("originalOrderNumber") != null && !jsonObj.get("originalOrderNumber").isJsonNull()) && !jsonObj.get("originalOrderNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `originalOrderNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("originalOrderNumber").toString()));
}
if ((jsonObj.get("originalOrderLineItemId") != null && !jsonObj.get("originalOrderLineItemId").isJsonNull()) && !jsonObj.get("originalOrderLineItemId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `originalOrderLineItemId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("originalOrderLineItemId").toString()));
}
if ((jsonObj.get("originalOrderLineItemNumber") != null && !jsonObj.get("originalOrderLineItemNumber").isJsonNull()) && !jsonObj.get("originalOrderLineItemNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `originalOrderLineItemNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("originalOrderLineItemNumber").toString()));
}
if ((jsonObj.get("billingRule") != null && !jsonObj.get("billingRule").isJsonNull()) && !jsonObj.get("billingRule").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billingRule` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billingRule").toString()));
}
if ((jsonObj.get("inlineDiscountType") != null && !jsonObj.get("inlineDiscountType").isJsonNull()) && !jsonObj.get("inlineDiscountType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `inlineDiscountType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("inlineDiscountType").toString()));
}
if ((jsonObj.get("recognizedRevenueAccountingCodeId") != null && !jsonObj.get("recognizedRevenueAccountingCodeId").isJsonNull()) && !jsonObj.get("recognizedRevenueAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `recognizedRevenueAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("recognizedRevenueAccountingCodeId").toString()));
}
if ((jsonObj.get("deferredRevenueAccountingCodeId") != null && !jsonObj.get("deferredRevenueAccountingCodeId").isJsonNull()) && !jsonObj.get("deferredRevenueAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `deferredRevenueAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("deferredRevenueAccountingCodeId").toString()));
}
if ((jsonObj.get("contractAssetAccountingCodeId") != null && !jsonObj.get("contractAssetAccountingCodeId").isJsonNull()) && !jsonObj.get("contractAssetAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractAssetAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractAssetAccountingCodeId").toString()));
}
if ((jsonObj.get("contractLiabilityAccountingCodeId") != null && !jsonObj.get("contractLiabilityAccountingCodeId").isJsonNull()) && !jsonObj.get("contractLiabilityAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractLiabilityAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractLiabilityAccountingCodeId").toString()));
}
if ((jsonObj.get("contractRecognizedRevenueAccountingCodeId") != null && !jsonObj.get("contractRecognizedRevenueAccountingCodeId").isJsonNull()) && !jsonObj.get("contractRecognizedRevenueAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractRecognizedRevenueAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractRecognizedRevenueAccountingCodeId").toString()));
}
if ((jsonObj.get("unbilledReceivablesAccountingCodeId") != null && !jsonObj.get("unbilledReceivablesAccountingCodeId").isJsonNull()) && !jsonObj.get("unbilledReceivablesAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `unbilledReceivablesAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("unbilledReceivablesAccountingCodeId").toString()));
}
if ((jsonObj.get("adjustmentRevenueAccountingCodeId") != null && !jsonObj.get("adjustmentRevenueAccountingCodeId").isJsonNull()) && !jsonObj.get("adjustmentRevenueAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `adjustmentRevenueAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("adjustmentRevenueAccountingCodeId").toString()));
}
if ((jsonObj.get("adjustmentLiabilityAccountingCodeId") != null && !jsonObj.get("adjustmentLiabilityAccountingCodeId").isJsonNull()) && !jsonObj.get("adjustmentLiabilityAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `adjustmentLiabilityAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("adjustmentLiabilityAccountingCodeId").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("invoiceItems") != null && !jsonObj.get("invoiceItems").isJsonNull() && !jsonObj.get("invoiceItems").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceItems` to be an array in the JSON string but got `%s`", jsonObj.get("invoiceItems").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!ExpandedOrderLineItem.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'ExpandedOrderLineItem' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(ExpandedOrderLineItem.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, ExpandedOrderLineItem value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public ExpandedOrderLineItem read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
ExpandedOrderLineItem instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of ExpandedOrderLineItem given an JSON string
*
* @param jsonString JSON string
* @return An instance of ExpandedOrderLineItem
* @throws IOException if the JSON string is invalid with respect to ExpandedOrderLineItem
*/
public static ExpandedOrderLineItem fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, ExpandedOrderLineItem.class);
}
/**
* Convert an instance of ExpandedOrderLineItem to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy