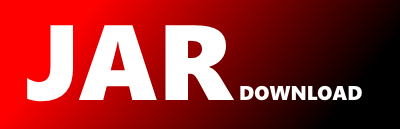
com.zuora.model.ExpandedPayment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.ExpandedAccount;
import com.zuora.model.ExpandedPaymentApplication;
import com.zuora.model.ExpandedPaymentMethod;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* ExpandedPayment
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class ExpandedPayment {
public static final String SERIALIZED_NAME_ACCOUNT_ID = "accountId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNTING_CODE = "accountingCode";
@SerializedName(SERIALIZED_NAME_ACCOUNTING_CODE)
private String accountingCode;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_APPLIED_AMOUNT = "appliedAmount";
@SerializedName(SERIALIZED_NAME_APPLIED_AMOUNT)
private BigDecimal appliedAmount;
public static final String SERIALIZED_NAME_APPLIED_CREDIT_BALANCE_AMOUNT = "appliedCreditBalanceAmount";
@SerializedName(SERIALIZED_NAME_APPLIED_CREDIT_BALANCE_AMOUNT)
private BigDecimal appliedCreditBalanceAmount;
public static final String SERIALIZED_NAME_AUTH_TRANSACTION_ID = "authTransactionId";
@SerializedName(SERIALIZED_NAME_AUTH_TRANSACTION_ID)
private String authTransactionId;
public static final String SERIALIZED_NAME_BANK_IDENTIFICATION_NUMBER = "bankIdentificationNumber";
@SerializedName(SERIALIZED_NAME_BANK_IDENTIFICATION_NUMBER)
private String bankIdentificationNumber;
public static final String SERIALIZED_NAME_CANCELLED_ON = "cancelledOn";
@SerializedName(SERIALIZED_NAME_CANCELLED_ON)
private String cancelledOn;
public static final String SERIALIZED_NAME_COMMENT = "comment";
@SerializedName(SERIALIZED_NAME_COMMENT)
private String comment;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_EFFECTIVE_DATE = "effectiveDate";
@SerializedName(SERIALIZED_NAME_EFFECTIVE_DATE)
private LocalDate effectiveDate;
public static final String SERIALIZED_NAME_GATEWAY_ORDER_ID = "gatewayOrderId";
@SerializedName(SERIALIZED_NAME_GATEWAY_ORDER_ID)
private String gatewayOrderId;
public static final String SERIALIZED_NAME_GATEWAY_RECONCILIATION_REASON = "gatewayReconciliationReason";
@SerializedName(SERIALIZED_NAME_GATEWAY_RECONCILIATION_REASON)
private String gatewayReconciliationReason;
public static final String SERIALIZED_NAME_GATEWAY_RECONCILIATION_STATUS = "gatewayReconciliationStatus";
@SerializedName(SERIALIZED_NAME_GATEWAY_RECONCILIATION_STATUS)
private String gatewayReconciliationStatus;
public static final String SERIALIZED_NAME_GATEWAY_RESPONSE = "gatewayResponse";
@SerializedName(SERIALIZED_NAME_GATEWAY_RESPONSE)
private String gatewayResponse;
public static final String SERIALIZED_NAME_GATEWAY_RESPONSE_CODE = "gatewayResponseCode";
@SerializedName(SERIALIZED_NAME_GATEWAY_RESPONSE_CODE)
private String gatewayResponseCode;
public static final String SERIALIZED_NAME_GATEWAY_STATE = "gatewayState";
@SerializedName(SERIALIZED_NAME_GATEWAY_STATE)
private String gatewayState;
public static final String SERIALIZED_NAME_GATEWAY_TRANSACTION_STATE = "gatewayTransactionState";
@SerializedName(SERIALIZED_NAME_GATEWAY_TRANSACTION_STATE)
private String gatewayTransactionState;
public static final String SERIALIZED_NAME_IS_STANDALONE = "isStandalone";
@SerializedName(SERIALIZED_NAME_IS_STANDALONE)
private Boolean isStandalone;
public static final String SERIALIZED_NAME_MARKED_FOR_SUBMISSION_ON = "markedForSubmissionOn";
@SerializedName(SERIALIZED_NAME_MARKED_FOR_SUBMISSION_ON)
private String markedForSubmissionOn;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "paymentMethodId";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_SNAPSHOT_ID = "paymentMethodSnapshotId";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_SNAPSHOT_ID)
private String paymentMethodSnapshotId;
public static final String SERIALIZED_NAME_PAYMENT_OPTION_ID = "paymentOptionId";
@SerializedName(SERIALIZED_NAME_PAYMENT_OPTION_ID)
private String paymentOptionId;
public static final String SERIALIZED_NAME_PAYMENT_NUMBER = "paymentNumber";
@SerializedName(SERIALIZED_NAME_PAYMENT_NUMBER)
private String paymentNumber;
public static final String SERIALIZED_NAME_PAYOUT_ID = "payoutId";
@SerializedName(SERIALIZED_NAME_PAYOUT_ID)
private String payoutId;
public static final String SERIALIZED_NAME_PREPAYMENT = "prepayment";
@SerializedName(SERIALIZED_NAME_PREPAYMENT)
private Boolean prepayment;
public static final String SERIALIZED_NAME_REFERENCED_PAYMENT_I_D = "referencedPaymentID";
@SerializedName(SERIALIZED_NAME_REFERENCED_PAYMENT_I_D)
private String referencedPaymentID;
public static final String SERIALIZED_NAME_REFERENCE_ID = "referenceId";
@SerializedName(SERIALIZED_NAME_REFERENCE_ID)
private String referenceId;
public static final String SERIALIZED_NAME_REFUND_AMOUNT = "refundAmount";
@SerializedName(SERIALIZED_NAME_REFUND_AMOUNT)
private BigDecimal refundAmount;
public static final String SERIALIZED_NAME_SECOND_PAYMENT_REFERENCE_ID = "secondPaymentReferenceId";
@SerializedName(SERIALIZED_NAME_SECOND_PAYMENT_REFERENCE_ID)
private String secondPaymentReferenceId;
public static final String SERIALIZED_NAME_SETTLED_ON = "settledOn";
@SerializedName(SERIALIZED_NAME_SETTLED_ON)
private String settledOn;
public static final String SERIALIZED_NAME_SOFT_DESCRIPTOR = "softDescriptor";
@SerializedName(SERIALIZED_NAME_SOFT_DESCRIPTOR)
private String softDescriptor;
public static final String SERIALIZED_NAME_SOFT_DESCRIPTOR_PHONE = "softDescriptorPhone";
@SerializedName(SERIALIZED_NAME_SOFT_DESCRIPTOR_PHONE)
private String softDescriptorPhone;
public static final String SERIALIZED_NAME_SOURCE = "source";
@SerializedName(SERIALIZED_NAME_SOURCE)
private String source;
public static final String SERIALIZED_NAME_SOURCE_NAME = "sourceName";
@SerializedName(SERIALIZED_NAME_SOURCE_NAME)
private String sourceName;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private String status;
public static final String SERIALIZED_NAME_SUBMITTED_ON = "submittedOn";
@SerializedName(SERIALIZED_NAME_SUBMITTED_ON)
private String submittedOn;
public static final String SERIALIZED_NAME_TRANSFERRED_TO_ACCOUNTING = "transferredToAccounting";
@SerializedName(SERIALIZED_NAME_TRANSFERRED_TO_ACCOUNTING)
private String transferredToAccounting;
public static final String SERIALIZED_NAME_TRANSACTION_SOURCE = "transactionSource";
@SerializedName(SERIALIZED_NAME_TRANSACTION_SOURCE)
private String transactionSource;
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private String type;
public static final String SERIALIZED_NAME_UNAPPLIED_AMOUNT = "unappliedAmount";
@SerializedName(SERIALIZED_NAME_UNAPPLIED_AMOUNT)
private BigDecimal unappliedAmount;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public static final String SERIALIZED_NAME_LAST_EMAIL_DATE_TIME = "lastEmailDateTime";
@SerializedName(SERIALIZED_NAME_LAST_EMAIL_DATE_TIME)
private String lastEmailDateTime;
public static final String SERIALIZED_NAME_GATEWAY_ROUTING_EXECUTION_ID = "gatewayRoutingExecutionId";
@SerializedName(SERIALIZED_NAME_GATEWAY_ROUTING_EXECUTION_ID)
private String gatewayRoutingExecutionId;
public static final String SERIALIZED_NAME_GATEWAY = "gateway";
@SerializedName(SERIALIZED_NAME_GATEWAY)
private String gateway;
public static final String SERIALIZED_NAME_ACCOUNT = "account";
@SerializedName(SERIALIZED_NAME_ACCOUNT)
private ExpandedAccount account;
public static final String SERIALIZED_NAME_PAYMENT_METHOD = "paymentMethod";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD)
private ExpandedPaymentMethod paymentMethod;
public static final String SERIALIZED_NAME_PAYMENT_APPLICATIONS = "paymentApplications";
@SerializedName(SERIALIZED_NAME_PAYMENT_APPLICATIONS)
private List paymentApplications;
public ExpandedPayment() {
}
public ExpandedPayment accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Get accountId
* @return accountId
*/
@javax.annotation.Nullable
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public ExpandedPayment accountingCode(String accountingCode) {
this.accountingCode = accountingCode;
return this;
}
/**
* Get accountingCode
* @return accountingCode
*/
@javax.annotation.Nullable
public String getAccountingCode() {
return accountingCode;
}
public void setAccountingCode(String accountingCode) {
this.accountingCode = accountingCode;
}
public ExpandedPayment amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* Get amount
* @return amount
*/
@javax.annotation.Nullable
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public ExpandedPayment appliedAmount(BigDecimal appliedAmount) {
this.appliedAmount = appliedAmount;
return this;
}
/**
* Get appliedAmount
* @return appliedAmount
*/
@javax.annotation.Nullable
public BigDecimal getAppliedAmount() {
return appliedAmount;
}
public void setAppliedAmount(BigDecimal appliedAmount) {
this.appliedAmount = appliedAmount;
}
public ExpandedPayment appliedCreditBalanceAmount(BigDecimal appliedCreditBalanceAmount) {
this.appliedCreditBalanceAmount = appliedCreditBalanceAmount;
return this;
}
/**
* Get appliedCreditBalanceAmount
* @return appliedCreditBalanceAmount
*/
@javax.annotation.Nullable
public BigDecimal getAppliedCreditBalanceAmount() {
return appliedCreditBalanceAmount;
}
public void setAppliedCreditBalanceAmount(BigDecimal appliedCreditBalanceAmount) {
this.appliedCreditBalanceAmount = appliedCreditBalanceAmount;
}
public ExpandedPayment authTransactionId(String authTransactionId) {
this.authTransactionId = authTransactionId;
return this;
}
/**
* Get authTransactionId
* @return authTransactionId
*/
@javax.annotation.Nullable
public String getAuthTransactionId() {
return authTransactionId;
}
public void setAuthTransactionId(String authTransactionId) {
this.authTransactionId = authTransactionId;
}
public ExpandedPayment bankIdentificationNumber(String bankIdentificationNumber) {
this.bankIdentificationNumber = bankIdentificationNumber;
return this;
}
/**
* Get bankIdentificationNumber
* @return bankIdentificationNumber
*/
@javax.annotation.Nullable
public String getBankIdentificationNumber() {
return bankIdentificationNumber;
}
public void setBankIdentificationNumber(String bankIdentificationNumber) {
this.bankIdentificationNumber = bankIdentificationNumber;
}
public ExpandedPayment cancelledOn(String cancelledOn) {
this.cancelledOn = cancelledOn;
return this;
}
/**
* Get cancelledOn
* @return cancelledOn
*/
@javax.annotation.Nullable
public String getCancelledOn() {
return cancelledOn;
}
public void setCancelledOn(String cancelledOn) {
this.cancelledOn = cancelledOn;
}
public ExpandedPayment comment(String comment) {
this.comment = comment;
return this;
}
/**
* Get comment
* @return comment
*/
@javax.annotation.Nullable
public String getComment() {
return comment;
}
public void setComment(String comment) {
this.comment = comment;
}
public ExpandedPayment currency(String currency) {
this.currency = currency;
return this;
}
/**
* Get currency
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public ExpandedPayment effectiveDate(LocalDate effectiveDate) {
this.effectiveDate = effectiveDate;
return this;
}
/**
* Get effectiveDate
* @return effectiveDate
*/
@javax.annotation.Nullable
public LocalDate getEffectiveDate() {
return effectiveDate;
}
public void setEffectiveDate(LocalDate effectiveDate) {
this.effectiveDate = effectiveDate;
}
public ExpandedPayment gatewayOrderId(String gatewayOrderId) {
this.gatewayOrderId = gatewayOrderId;
return this;
}
/**
* Get gatewayOrderId
* @return gatewayOrderId
*/
@javax.annotation.Nullable
public String getGatewayOrderId() {
return gatewayOrderId;
}
public void setGatewayOrderId(String gatewayOrderId) {
this.gatewayOrderId = gatewayOrderId;
}
public ExpandedPayment gatewayReconciliationReason(String gatewayReconciliationReason) {
this.gatewayReconciliationReason = gatewayReconciliationReason;
return this;
}
/**
* Get gatewayReconciliationReason
* @return gatewayReconciliationReason
*/
@javax.annotation.Nullable
public String getGatewayReconciliationReason() {
return gatewayReconciliationReason;
}
public void setGatewayReconciliationReason(String gatewayReconciliationReason) {
this.gatewayReconciliationReason = gatewayReconciliationReason;
}
public ExpandedPayment gatewayReconciliationStatus(String gatewayReconciliationStatus) {
this.gatewayReconciliationStatus = gatewayReconciliationStatus;
return this;
}
/**
* Get gatewayReconciliationStatus
* @return gatewayReconciliationStatus
*/
@javax.annotation.Nullable
public String getGatewayReconciliationStatus() {
return gatewayReconciliationStatus;
}
public void setGatewayReconciliationStatus(String gatewayReconciliationStatus) {
this.gatewayReconciliationStatus = gatewayReconciliationStatus;
}
public ExpandedPayment gatewayResponse(String gatewayResponse) {
this.gatewayResponse = gatewayResponse;
return this;
}
/**
* Get gatewayResponse
* @return gatewayResponse
*/
@javax.annotation.Nullable
public String getGatewayResponse() {
return gatewayResponse;
}
public void setGatewayResponse(String gatewayResponse) {
this.gatewayResponse = gatewayResponse;
}
public ExpandedPayment gatewayResponseCode(String gatewayResponseCode) {
this.gatewayResponseCode = gatewayResponseCode;
return this;
}
/**
* Get gatewayResponseCode
* @return gatewayResponseCode
*/
@javax.annotation.Nullable
public String getGatewayResponseCode() {
return gatewayResponseCode;
}
public void setGatewayResponseCode(String gatewayResponseCode) {
this.gatewayResponseCode = gatewayResponseCode;
}
public ExpandedPayment gatewayState(String gatewayState) {
this.gatewayState = gatewayState;
return this;
}
/**
* Get gatewayState
* @return gatewayState
*/
@javax.annotation.Nullable
public String getGatewayState() {
return gatewayState;
}
public void setGatewayState(String gatewayState) {
this.gatewayState = gatewayState;
}
public ExpandedPayment gatewayTransactionState(String gatewayTransactionState) {
this.gatewayTransactionState = gatewayTransactionState;
return this;
}
/**
* Get gatewayTransactionState
* @return gatewayTransactionState
*/
@javax.annotation.Nullable
public String getGatewayTransactionState() {
return gatewayTransactionState;
}
public void setGatewayTransactionState(String gatewayTransactionState) {
this.gatewayTransactionState = gatewayTransactionState;
}
public ExpandedPayment isStandalone(Boolean isStandalone) {
this.isStandalone = isStandalone;
return this;
}
/**
* Get isStandalone
* @return isStandalone
*/
@javax.annotation.Nullable
public Boolean getIsStandalone() {
return isStandalone;
}
public void setIsStandalone(Boolean isStandalone) {
this.isStandalone = isStandalone;
}
public ExpandedPayment markedForSubmissionOn(String markedForSubmissionOn) {
this.markedForSubmissionOn = markedForSubmissionOn;
return this;
}
/**
* Get markedForSubmissionOn
* @return markedForSubmissionOn
*/
@javax.annotation.Nullable
public String getMarkedForSubmissionOn() {
return markedForSubmissionOn;
}
public void setMarkedForSubmissionOn(String markedForSubmissionOn) {
this.markedForSubmissionOn = markedForSubmissionOn;
}
public ExpandedPayment paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
return this;
}
/**
* Get paymentMethodId
* @return paymentMethodId
*/
@javax.annotation.Nullable
public String getPaymentMethodId() {
return paymentMethodId;
}
public void setPaymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
}
public ExpandedPayment paymentMethodSnapshotId(String paymentMethodSnapshotId) {
this.paymentMethodSnapshotId = paymentMethodSnapshotId;
return this;
}
/**
* Get paymentMethodSnapshotId
* @return paymentMethodSnapshotId
*/
@javax.annotation.Nullable
public String getPaymentMethodSnapshotId() {
return paymentMethodSnapshotId;
}
public void setPaymentMethodSnapshotId(String paymentMethodSnapshotId) {
this.paymentMethodSnapshotId = paymentMethodSnapshotId;
}
public ExpandedPayment paymentOptionId(String paymentOptionId) {
this.paymentOptionId = paymentOptionId;
return this;
}
/**
* Get paymentOptionId
* @return paymentOptionId
*/
@javax.annotation.Nullable
public String getPaymentOptionId() {
return paymentOptionId;
}
public void setPaymentOptionId(String paymentOptionId) {
this.paymentOptionId = paymentOptionId;
}
public ExpandedPayment paymentNumber(String paymentNumber) {
this.paymentNumber = paymentNumber;
return this;
}
/**
* Get paymentNumber
* @return paymentNumber
*/
@javax.annotation.Nullable
public String getPaymentNumber() {
return paymentNumber;
}
public void setPaymentNumber(String paymentNumber) {
this.paymentNumber = paymentNumber;
}
public ExpandedPayment payoutId(String payoutId) {
this.payoutId = payoutId;
return this;
}
/**
* Get payoutId
* @return payoutId
*/
@javax.annotation.Nullable
public String getPayoutId() {
return payoutId;
}
public void setPayoutId(String payoutId) {
this.payoutId = payoutId;
}
public ExpandedPayment prepayment(Boolean prepayment) {
this.prepayment = prepayment;
return this;
}
/**
* Get prepayment
* @return prepayment
*/
@javax.annotation.Nullable
public Boolean getPrepayment() {
return prepayment;
}
public void setPrepayment(Boolean prepayment) {
this.prepayment = prepayment;
}
public ExpandedPayment referencedPaymentID(String referencedPaymentID) {
this.referencedPaymentID = referencedPaymentID;
return this;
}
/**
* Get referencedPaymentID
* @return referencedPaymentID
*/
@javax.annotation.Nullable
public String getReferencedPaymentID() {
return referencedPaymentID;
}
public void setReferencedPaymentID(String referencedPaymentID) {
this.referencedPaymentID = referencedPaymentID;
}
public ExpandedPayment referenceId(String referenceId) {
this.referenceId = referenceId;
return this;
}
/**
* Get referenceId
* @return referenceId
*/
@javax.annotation.Nullable
public String getReferenceId() {
return referenceId;
}
public void setReferenceId(String referenceId) {
this.referenceId = referenceId;
}
public ExpandedPayment refundAmount(BigDecimal refundAmount) {
this.refundAmount = refundAmount;
return this;
}
/**
* Get refundAmount
* @return refundAmount
*/
@javax.annotation.Nullable
public BigDecimal getRefundAmount() {
return refundAmount;
}
public void setRefundAmount(BigDecimal refundAmount) {
this.refundAmount = refundAmount;
}
public ExpandedPayment secondPaymentReferenceId(String secondPaymentReferenceId) {
this.secondPaymentReferenceId = secondPaymentReferenceId;
return this;
}
/**
* Get secondPaymentReferenceId
* @return secondPaymentReferenceId
*/
@javax.annotation.Nullable
public String getSecondPaymentReferenceId() {
return secondPaymentReferenceId;
}
public void setSecondPaymentReferenceId(String secondPaymentReferenceId) {
this.secondPaymentReferenceId = secondPaymentReferenceId;
}
public ExpandedPayment settledOn(String settledOn) {
this.settledOn = settledOn;
return this;
}
/**
* Get settledOn
* @return settledOn
*/
@javax.annotation.Nullable
public String getSettledOn() {
return settledOn;
}
public void setSettledOn(String settledOn) {
this.settledOn = settledOn;
}
public ExpandedPayment softDescriptor(String softDescriptor) {
this.softDescriptor = softDescriptor;
return this;
}
/**
* Get softDescriptor
* @return softDescriptor
*/
@javax.annotation.Nullable
public String getSoftDescriptor() {
return softDescriptor;
}
public void setSoftDescriptor(String softDescriptor) {
this.softDescriptor = softDescriptor;
}
public ExpandedPayment softDescriptorPhone(String softDescriptorPhone) {
this.softDescriptorPhone = softDescriptorPhone;
return this;
}
/**
* Get softDescriptorPhone
* @return softDescriptorPhone
*/
@javax.annotation.Nullable
public String getSoftDescriptorPhone() {
return softDescriptorPhone;
}
public void setSoftDescriptorPhone(String softDescriptorPhone) {
this.softDescriptorPhone = softDescriptorPhone;
}
public ExpandedPayment source(String source) {
this.source = source;
return this;
}
/**
* Get source
* @return source
*/
@javax.annotation.Nullable
public String getSource() {
return source;
}
public void setSource(String source) {
this.source = source;
}
public ExpandedPayment sourceName(String sourceName) {
this.sourceName = sourceName;
return this;
}
/**
* Get sourceName
* @return sourceName
*/
@javax.annotation.Nullable
public String getSourceName() {
return sourceName;
}
public void setSourceName(String sourceName) {
this.sourceName = sourceName;
}
public ExpandedPayment status(String status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public ExpandedPayment submittedOn(String submittedOn) {
this.submittedOn = submittedOn;
return this;
}
/**
* Get submittedOn
* @return submittedOn
*/
@javax.annotation.Nullable
public String getSubmittedOn() {
return submittedOn;
}
public void setSubmittedOn(String submittedOn) {
this.submittedOn = submittedOn;
}
public ExpandedPayment transferredToAccounting(String transferredToAccounting) {
this.transferredToAccounting = transferredToAccounting;
return this;
}
/**
* Get transferredToAccounting
* @return transferredToAccounting
*/
@javax.annotation.Nullable
public String getTransferredToAccounting() {
return transferredToAccounting;
}
public void setTransferredToAccounting(String transferredToAccounting) {
this.transferredToAccounting = transferredToAccounting;
}
public ExpandedPayment transactionSource(String transactionSource) {
this.transactionSource = transactionSource;
return this;
}
/**
* Get transactionSource
* @return transactionSource
*/
@javax.annotation.Nullable
public String getTransactionSource() {
return transactionSource;
}
public void setTransactionSource(String transactionSource) {
this.transactionSource = transactionSource;
}
public ExpandedPayment type(String type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
*/
@javax.annotation.Nullable
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public ExpandedPayment unappliedAmount(BigDecimal unappliedAmount) {
this.unappliedAmount = unappliedAmount;
return this;
}
/**
* Get unappliedAmount
* @return unappliedAmount
*/
@javax.annotation.Nullable
public BigDecimal getUnappliedAmount() {
return unappliedAmount;
}
public void setUnappliedAmount(BigDecimal unappliedAmount) {
this.unappliedAmount = unappliedAmount;
}
public ExpandedPayment id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public ExpandedPayment createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* Get createdById
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public ExpandedPayment createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* Get createdDate
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public ExpandedPayment updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* Get updatedById
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public ExpandedPayment updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* Get updatedDate
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
public ExpandedPayment lastEmailDateTime(String lastEmailDateTime) {
this.lastEmailDateTime = lastEmailDateTime;
return this;
}
/**
* Get lastEmailDateTime
* @return lastEmailDateTime
*/
@javax.annotation.Nullable
public String getLastEmailDateTime() {
return lastEmailDateTime;
}
public void setLastEmailDateTime(String lastEmailDateTime) {
this.lastEmailDateTime = lastEmailDateTime;
}
public ExpandedPayment gatewayRoutingExecutionId(String gatewayRoutingExecutionId) {
this.gatewayRoutingExecutionId = gatewayRoutingExecutionId;
return this;
}
/**
* Get gatewayRoutingExecutionId
* @return gatewayRoutingExecutionId
*/
@javax.annotation.Nullable
public String getGatewayRoutingExecutionId() {
return gatewayRoutingExecutionId;
}
public void setGatewayRoutingExecutionId(String gatewayRoutingExecutionId) {
this.gatewayRoutingExecutionId = gatewayRoutingExecutionId;
}
public ExpandedPayment gateway(String gateway) {
this.gateway = gateway;
return this;
}
/**
* Get gateway
* @return gateway
*/
@javax.annotation.Nullable
public String getGateway() {
return gateway;
}
public void setGateway(String gateway) {
this.gateway = gateway;
}
public ExpandedPayment account(ExpandedAccount account) {
this.account = account;
return this;
}
/**
* Get account
* @return account
*/
@javax.annotation.Nullable
public ExpandedAccount getAccount() {
return account;
}
public void setAccount(ExpandedAccount account) {
this.account = account;
}
public ExpandedPayment paymentMethod(ExpandedPaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
return this;
}
/**
* Get paymentMethod
* @return paymentMethod
*/
@javax.annotation.Nullable
public ExpandedPaymentMethod getPaymentMethod() {
return paymentMethod;
}
public void setPaymentMethod(ExpandedPaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
}
public ExpandedPayment paymentApplications(List paymentApplications) {
this.paymentApplications = paymentApplications;
return this;
}
public ExpandedPayment addPaymentApplicationsItem(ExpandedPaymentApplication paymentApplicationsItem) {
if (this.paymentApplications == null) {
this.paymentApplications = new ArrayList<>();
}
this.paymentApplications.add(paymentApplicationsItem);
return this;
}
/**
* Get paymentApplications
* @return paymentApplications
*/
@javax.annotation.Nullable
public List getPaymentApplications() {
return paymentApplications;
}
public void setPaymentApplications(List paymentApplications) {
this.paymentApplications = paymentApplications;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the ExpandedPayment instance itself
*/
public ExpandedPayment putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ExpandedPayment expandedPayment = (ExpandedPayment) o;
return Objects.equals(this.accountId, expandedPayment.accountId) &&
Objects.equals(this.accountingCode, expandedPayment.accountingCode) &&
Objects.equals(this.amount, expandedPayment.amount) &&
Objects.equals(this.appliedAmount, expandedPayment.appliedAmount) &&
Objects.equals(this.appliedCreditBalanceAmount, expandedPayment.appliedCreditBalanceAmount) &&
Objects.equals(this.authTransactionId, expandedPayment.authTransactionId) &&
Objects.equals(this.bankIdentificationNumber, expandedPayment.bankIdentificationNumber) &&
Objects.equals(this.cancelledOn, expandedPayment.cancelledOn) &&
Objects.equals(this.comment, expandedPayment.comment) &&
Objects.equals(this.currency, expandedPayment.currency) &&
Objects.equals(this.effectiveDate, expandedPayment.effectiveDate) &&
Objects.equals(this.gatewayOrderId, expandedPayment.gatewayOrderId) &&
Objects.equals(this.gatewayReconciliationReason, expandedPayment.gatewayReconciliationReason) &&
Objects.equals(this.gatewayReconciliationStatus, expandedPayment.gatewayReconciliationStatus) &&
Objects.equals(this.gatewayResponse, expandedPayment.gatewayResponse) &&
Objects.equals(this.gatewayResponseCode, expandedPayment.gatewayResponseCode) &&
Objects.equals(this.gatewayState, expandedPayment.gatewayState) &&
Objects.equals(this.gatewayTransactionState, expandedPayment.gatewayTransactionState) &&
Objects.equals(this.isStandalone, expandedPayment.isStandalone) &&
Objects.equals(this.markedForSubmissionOn, expandedPayment.markedForSubmissionOn) &&
Objects.equals(this.paymentMethodId, expandedPayment.paymentMethodId) &&
Objects.equals(this.paymentMethodSnapshotId, expandedPayment.paymentMethodSnapshotId) &&
Objects.equals(this.paymentOptionId, expandedPayment.paymentOptionId) &&
Objects.equals(this.paymentNumber, expandedPayment.paymentNumber) &&
Objects.equals(this.payoutId, expandedPayment.payoutId) &&
Objects.equals(this.prepayment, expandedPayment.prepayment) &&
Objects.equals(this.referencedPaymentID, expandedPayment.referencedPaymentID) &&
Objects.equals(this.referenceId, expandedPayment.referenceId) &&
Objects.equals(this.refundAmount, expandedPayment.refundAmount) &&
Objects.equals(this.secondPaymentReferenceId, expandedPayment.secondPaymentReferenceId) &&
Objects.equals(this.settledOn, expandedPayment.settledOn) &&
Objects.equals(this.softDescriptor, expandedPayment.softDescriptor) &&
Objects.equals(this.softDescriptorPhone, expandedPayment.softDescriptorPhone) &&
Objects.equals(this.source, expandedPayment.source) &&
Objects.equals(this.sourceName, expandedPayment.sourceName) &&
Objects.equals(this.status, expandedPayment.status) &&
Objects.equals(this.submittedOn, expandedPayment.submittedOn) &&
Objects.equals(this.transferredToAccounting, expandedPayment.transferredToAccounting) &&
Objects.equals(this.transactionSource, expandedPayment.transactionSource) &&
Objects.equals(this.type, expandedPayment.type) &&
Objects.equals(this.unappliedAmount, expandedPayment.unappliedAmount) &&
Objects.equals(this.id, expandedPayment.id) &&
Objects.equals(this.createdById, expandedPayment.createdById) &&
Objects.equals(this.createdDate, expandedPayment.createdDate) &&
Objects.equals(this.updatedById, expandedPayment.updatedById) &&
Objects.equals(this.updatedDate, expandedPayment.updatedDate) &&
Objects.equals(this.lastEmailDateTime, expandedPayment.lastEmailDateTime) &&
Objects.equals(this.gatewayRoutingExecutionId, expandedPayment.gatewayRoutingExecutionId) &&
Objects.equals(this.gateway, expandedPayment.gateway) &&
Objects.equals(this.account, expandedPayment.account) &&
Objects.equals(this.paymentMethod, expandedPayment.paymentMethod) &&
Objects.equals(this.paymentApplications, expandedPayment.paymentApplications)&&
Objects.equals(this.additionalProperties, expandedPayment.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(accountId, accountingCode, amount, appliedAmount, appliedCreditBalanceAmount, authTransactionId, bankIdentificationNumber, cancelledOn, comment, currency, effectiveDate, gatewayOrderId, gatewayReconciliationReason, gatewayReconciliationStatus, gatewayResponse, gatewayResponseCode, gatewayState, gatewayTransactionState, isStandalone, markedForSubmissionOn, paymentMethodId, paymentMethodSnapshotId, paymentOptionId, paymentNumber, payoutId, prepayment, referencedPaymentID, referenceId, refundAmount, secondPaymentReferenceId, settledOn, softDescriptor, softDescriptorPhone, source, sourceName, status, submittedOn, transferredToAccounting, transactionSource, type, unappliedAmount, id, createdById, createdDate, updatedById, updatedDate, lastEmailDateTime, gatewayRoutingExecutionId, gateway, account, paymentMethod, paymentApplications, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ExpandedPayment {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountingCode: ").append(toIndentedString(accountingCode)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" appliedAmount: ").append(toIndentedString(appliedAmount)).append("\n");
sb.append(" appliedCreditBalanceAmount: ").append(toIndentedString(appliedCreditBalanceAmount)).append("\n");
sb.append(" authTransactionId: ").append(toIndentedString(authTransactionId)).append("\n");
sb.append(" bankIdentificationNumber: ").append(toIndentedString(bankIdentificationNumber)).append("\n");
sb.append(" cancelledOn: ").append(toIndentedString(cancelledOn)).append("\n");
sb.append(" comment: ").append(toIndentedString(comment)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" effectiveDate: ").append(toIndentedString(effectiveDate)).append("\n");
sb.append(" gatewayOrderId: ").append(toIndentedString(gatewayOrderId)).append("\n");
sb.append(" gatewayReconciliationReason: ").append(toIndentedString(gatewayReconciliationReason)).append("\n");
sb.append(" gatewayReconciliationStatus: ").append(toIndentedString(gatewayReconciliationStatus)).append("\n");
sb.append(" gatewayResponse: ").append(toIndentedString(gatewayResponse)).append("\n");
sb.append(" gatewayResponseCode: ").append(toIndentedString(gatewayResponseCode)).append("\n");
sb.append(" gatewayState: ").append(toIndentedString(gatewayState)).append("\n");
sb.append(" gatewayTransactionState: ").append(toIndentedString(gatewayTransactionState)).append("\n");
sb.append(" isStandalone: ").append(toIndentedString(isStandalone)).append("\n");
sb.append(" markedForSubmissionOn: ").append(toIndentedString(markedForSubmissionOn)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" paymentMethodSnapshotId: ").append(toIndentedString(paymentMethodSnapshotId)).append("\n");
sb.append(" paymentOptionId: ").append(toIndentedString(paymentOptionId)).append("\n");
sb.append(" paymentNumber: ").append(toIndentedString(paymentNumber)).append("\n");
sb.append(" payoutId: ").append(toIndentedString(payoutId)).append("\n");
sb.append(" prepayment: ").append(toIndentedString(prepayment)).append("\n");
sb.append(" referencedPaymentID: ").append(toIndentedString(referencedPaymentID)).append("\n");
sb.append(" referenceId: ").append(toIndentedString(referenceId)).append("\n");
sb.append(" refundAmount: ").append(toIndentedString(refundAmount)).append("\n");
sb.append(" secondPaymentReferenceId: ").append(toIndentedString(secondPaymentReferenceId)).append("\n");
sb.append(" settledOn: ").append(toIndentedString(settledOn)).append("\n");
sb.append(" softDescriptor: ").append(toIndentedString(softDescriptor)).append("\n");
sb.append(" softDescriptorPhone: ").append(toIndentedString(softDescriptorPhone)).append("\n");
sb.append(" source: ").append(toIndentedString(source)).append("\n");
sb.append(" sourceName: ").append(toIndentedString(sourceName)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" submittedOn: ").append(toIndentedString(submittedOn)).append("\n");
sb.append(" transferredToAccounting: ").append(toIndentedString(transferredToAccounting)).append("\n");
sb.append(" transactionSource: ").append(toIndentedString(transactionSource)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" unappliedAmount: ").append(toIndentedString(unappliedAmount)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" lastEmailDateTime: ").append(toIndentedString(lastEmailDateTime)).append("\n");
sb.append(" gatewayRoutingExecutionId: ").append(toIndentedString(gatewayRoutingExecutionId)).append("\n");
sb.append(" gateway: ").append(toIndentedString(gateway)).append("\n");
sb.append(" account: ").append(toIndentedString(account)).append("\n");
sb.append(" paymentMethod: ").append(toIndentedString(paymentMethod)).append("\n");
sb.append(" paymentApplications: ").append(toIndentedString(paymentApplications)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("accountId");
openapiFields.add("accountingCode");
openapiFields.add("amount");
openapiFields.add("appliedAmount");
openapiFields.add("appliedCreditBalanceAmount");
openapiFields.add("authTransactionId");
openapiFields.add("bankIdentificationNumber");
openapiFields.add("cancelledOn");
openapiFields.add("comment");
openapiFields.add("currency");
openapiFields.add("effectiveDate");
openapiFields.add("gatewayOrderId");
openapiFields.add("gatewayReconciliationReason");
openapiFields.add("gatewayReconciliationStatus");
openapiFields.add("gatewayResponse");
openapiFields.add("gatewayResponseCode");
openapiFields.add("gatewayState");
openapiFields.add("gatewayTransactionState");
openapiFields.add("isStandalone");
openapiFields.add("markedForSubmissionOn");
openapiFields.add("paymentMethodId");
openapiFields.add("paymentMethodSnapshotId");
openapiFields.add("paymentOptionId");
openapiFields.add("paymentNumber");
openapiFields.add("payoutId");
openapiFields.add("prepayment");
openapiFields.add("referencedPaymentID");
openapiFields.add("referenceId");
openapiFields.add("refundAmount");
openapiFields.add("secondPaymentReferenceId");
openapiFields.add("settledOn");
openapiFields.add("softDescriptor");
openapiFields.add("softDescriptorPhone");
openapiFields.add("source");
openapiFields.add("sourceName");
openapiFields.add("status");
openapiFields.add("submittedOn");
openapiFields.add("transferredToAccounting");
openapiFields.add("transactionSource");
openapiFields.add("type");
openapiFields.add("unappliedAmount");
openapiFields.add("id");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
openapiFields.add("lastEmailDateTime");
openapiFields.add("gatewayRoutingExecutionId");
openapiFields.add("gateway");
openapiFields.add("account");
openapiFields.add("paymentMethod");
openapiFields.add("paymentApplications");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to ExpandedPayment
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!ExpandedPayment.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in ExpandedPayment is not found in the empty JSON string", ExpandedPayment.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("accountId") != null && !jsonObj.get("accountId").isJsonNull()) && !jsonObj.get("accountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountId").toString()));
}
if ((jsonObj.get("accountingCode") != null && !jsonObj.get("accountingCode").isJsonNull()) && !jsonObj.get("accountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountingCode").toString()));
}
if ((jsonObj.get("authTransactionId") != null && !jsonObj.get("authTransactionId").isJsonNull()) && !jsonObj.get("authTransactionId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `authTransactionId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("authTransactionId").toString()));
}
if ((jsonObj.get("bankIdentificationNumber") != null && !jsonObj.get("bankIdentificationNumber").isJsonNull()) && !jsonObj.get("bankIdentificationNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankIdentificationNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankIdentificationNumber").toString()));
}
if ((jsonObj.get("cancelledOn") != null && !jsonObj.get("cancelledOn").isJsonNull()) && !jsonObj.get("cancelledOn").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cancelledOn` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cancelledOn").toString()));
}
if ((jsonObj.get("comment") != null && !jsonObj.get("comment").isJsonNull()) && !jsonObj.get("comment").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `comment` to be a primitive type in the JSON string but got `%s`", jsonObj.get("comment").toString()));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("gatewayOrderId") != null && !jsonObj.get("gatewayOrderId").isJsonNull()) && !jsonObj.get("gatewayOrderId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayOrderId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayOrderId").toString()));
}
if ((jsonObj.get("gatewayReconciliationReason") != null && !jsonObj.get("gatewayReconciliationReason").isJsonNull()) && !jsonObj.get("gatewayReconciliationReason").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayReconciliationReason` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayReconciliationReason").toString()));
}
if ((jsonObj.get("gatewayReconciliationStatus") != null && !jsonObj.get("gatewayReconciliationStatus").isJsonNull()) && !jsonObj.get("gatewayReconciliationStatus").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayReconciliationStatus` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayReconciliationStatus").toString()));
}
if ((jsonObj.get("gatewayResponse") != null && !jsonObj.get("gatewayResponse").isJsonNull()) && !jsonObj.get("gatewayResponse").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayResponse` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayResponse").toString()));
}
if ((jsonObj.get("gatewayResponseCode") != null && !jsonObj.get("gatewayResponseCode").isJsonNull()) && !jsonObj.get("gatewayResponseCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayResponseCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayResponseCode").toString()));
}
if ((jsonObj.get("gatewayState") != null && !jsonObj.get("gatewayState").isJsonNull()) && !jsonObj.get("gatewayState").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayState` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayState").toString()));
}
if ((jsonObj.get("gatewayTransactionState") != null && !jsonObj.get("gatewayTransactionState").isJsonNull()) && !jsonObj.get("gatewayTransactionState").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayTransactionState` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayTransactionState").toString()));
}
if ((jsonObj.get("markedForSubmissionOn") != null && !jsonObj.get("markedForSubmissionOn").isJsonNull()) && !jsonObj.get("markedForSubmissionOn").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `markedForSubmissionOn` to be a primitive type in the JSON string but got `%s`", jsonObj.get("markedForSubmissionOn").toString()));
}
if ((jsonObj.get("paymentMethodId") != null && !jsonObj.get("paymentMethodId").isJsonNull()) && !jsonObj.get("paymentMethodId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentMethodId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentMethodId").toString()));
}
if ((jsonObj.get("paymentMethodSnapshotId") != null && !jsonObj.get("paymentMethodSnapshotId").isJsonNull()) && !jsonObj.get("paymentMethodSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentMethodSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentMethodSnapshotId").toString()));
}
if ((jsonObj.get("paymentOptionId") != null && !jsonObj.get("paymentOptionId").isJsonNull()) && !jsonObj.get("paymentOptionId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentOptionId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentOptionId").toString()));
}
if ((jsonObj.get("paymentNumber") != null && !jsonObj.get("paymentNumber").isJsonNull()) && !jsonObj.get("paymentNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentNumber").toString()));
}
if ((jsonObj.get("payoutId") != null && !jsonObj.get("payoutId").isJsonNull()) && !jsonObj.get("payoutId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `payoutId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("payoutId").toString()));
}
if ((jsonObj.get("referencedPaymentID") != null && !jsonObj.get("referencedPaymentID").isJsonNull()) && !jsonObj.get("referencedPaymentID").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `referencedPaymentID` to be a primitive type in the JSON string but got `%s`", jsonObj.get("referencedPaymentID").toString()));
}
if ((jsonObj.get("referenceId") != null && !jsonObj.get("referenceId").isJsonNull()) && !jsonObj.get("referenceId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `referenceId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("referenceId").toString()));
}
if ((jsonObj.get("secondPaymentReferenceId") != null && !jsonObj.get("secondPaymentReferenceId").isJsonNull()) && !jsonObj.get("secondPaymentReferenceId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `secondPaymentReferenceId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("secondPaymentReferenceId").toString()));
}
if ((jsonObj.get("settledOn") != null && !jsonObj.get("settledOn").isJsonNull()) && !jsonObj.get("settledOn").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `settledOn` to be a primitive type in the JSON string but got `%s`", jsonObj.get("settledOn").toString()));
}
if ((jsonObj.get("softDescriptor") != null && !jsonObj.get("softDescriptor").isJsonNull()) && !jsonObj.get("softDescriptor").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `softDescriptor` to be a primitive type in the JSON string but got `%s`", jsonObj.get("softDescriptor").toString()));
}
if ((jsonObj.get("softDescriptorPhone") != null && !jsonObj.get("softDescriptorPhone").isJsonNull()) && !jsonObj.get("softDescriptorPhone").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `softDescriptorPhone` to be a primitive type in the JSON string but got `%s`", jsonObj.get("softDescriptorPhone").toString()));
}
if ((jsonObj.get("source") != null && !jsonObj.get("source").isJsonNull()) && !jsonObj.get("source").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `source` to be a primitive type in the JSON string but got `%s`", jsonObj.get("source").toString()));
}
if ((jsonObj.get("sourceName") != null && !jsonObj.get("sourceName").isJsonNull()) && !jsonObj.get("sourceName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sourceName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sourceName").toString()));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
if ((jsonObj.get("submittedOn") != null && !jsonObj.get("submittedOn").isJsonNull()) && !jsonObj.get("submittedOn").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `submittedOn` to be a primitive type in the JSON string but got `%s`", jsonObj.get("submittedOn").toString()));
}
if ((jsonObj.get("transferredToAccounting") != null && !jsonObj.get("transferredToAccounting").isJsonNull()) && !jsonObj.get("transferredToAccounting").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `transferredToAccounting` to be a primitive type in the JSON string but got `%s`", jsonObj.get("transferredToAccounting").toString()));
}
if ((jsonObj.get("transactionSource") != null && !jsonObj.get("transactionSource").isJsonNull()) && !jsonObj.get("transactionSource").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `transactionSource` to be a primitive type in the JSON string but got `%s`", jsonObj.get("transactionSource").toString()));
}
if ((jsonObj.get("type") != null && !jsonObj.get("type").isJsonNull()) && !jsonObj.get("type").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `type` to be a primitive type in the JSON string but got `%s`", jsonObj.get("type").toString()));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
if ((jsonObj.get("lastEmailDateTime") != null && !jsonObj.get("lastEmailDateTime").isJsonNull()) && !jsonObj.get("lastEmailDateTime").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `lastEmailDateTime` to be a primitive type in the JSON string but got `%s`", jsonObj.get("lastEmailDateTime").toString()));
}
if ((jsonObj.get("gatewayRoutingExecutionId") != null && !jsonObj.get("gatewayRoutingExecutionId").isJsonNull()) && !jsonObj.get("gatewayRoutingExecutionId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayRoutingExecutionId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayRoutingExecutionId").toString()));
}
if ((jsonObj.get("gateway") != null && !jsonObj.get("gateway").isJsonNull()) && !jsonObj.get("gateway").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gateway` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gateway").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("paymentApplications") != null && !jsonObj.get("paymentApplications").isJsonNull() && !jsonObj.get("paymentApplications").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentApplications` to be an array in the JSON string but got `%s`", jsonObj.get("paymentApplications").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!ExpandedPayment.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'ExpandedPayment' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(ExpandedPayment.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, ExpandedPayment value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public ExpandedPayment read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
ExpandedPayment instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of ExpandedPayment given an JSON string
*
* @param jsonString JSON string
* @return An instance of ExpandedPayment
* @throws IOException if the JSON string is invalid with respect to ExpandedPayment
*/
public static ExpandedPayment fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, ExpandedPayment.class);
}
/**
* Convert an instance of ExpandedPayment to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy