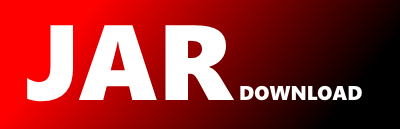
com.zuora.model.ExpandedPrepaidBalanceFund Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.ExpandedAccount;
import com.zuora.model.ExpandedPrepaidBalance;
import com.zuora.model.ExpandedRatePlanCharge;
import com.zuora.model.ExpandedValidityPeriodSummary;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* ExpandedPrepaidBalanceFund
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class ExpandedPrepaidBalanceFund {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "accountId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_BALANCE = "balance";
@SerializedName(SERIALIZED_NAME_BALANCE)
private BigDecimal balance;
public static final String SERIALIZED_NAME_CHARGE_SEGMENT_NUMBER = "chargeSegmentNumber";
@SerializedName(SERIALIZED_NAME_CHARGE_SEGMENT_NUMBER)
private Integer chargeSegmentNumber;
public static final String SERIALIZED_NAME_END_DATE = "endDate";
@SerializedName(SERIALIZED_NAME_END_DATE)
private LocalDate endDate;
public static final String SERIALIZED_NAME_FUNDED_BALANCE = "fundedBalance";
@SerializedName(SERIALIZED_NAME_FUNDED_BALANCE)
private BigDecimal fundedBalance;
public static final String SERIALIZED_NAME_FUND_SOURCE_TYPE = "fundSourceType";
@SerializedName(SERIALIZED_NAME_FUND_SOURCE_TYPE)
private String fundSourceType;
public static final String SERIALIZED_NAME_FUNDING_PRICE = "fundingPrice";
@SerializedName(SERIALIZED_NAME_FUNDING_PRICE)
private BigDecimal fundingPrice;
public static final String SERIALIZED_NAME_TOTAL_BILLED = "totalBilled";
@SerializedName(SERIALIZED_NAME_TOTAL_BILLED)
private BigDecimal totalBilled;
public static final String SERIALIZED_NAME_TOTAL_BALANCE = "totalBalance";
@SerializedName(SERIALIZED_NAME_TOTAL_BALANCE)
private BigDecimal totalBalance;
public static final String SERIALIZED_NAME_ORIGINAL_TOTAL_BALANCE = "originalTotalBalance";
@SerializedName(SERIALIZED_NAME_ORIGINAL_TOTAL_BALANCE)
private BigDecimal originalTotalBalance;
public static final String SERIALIZED_NAME_ORIGINAL_FUNDING_PRICE = "originalFundingPrice";
@SerializedName(SERIALIZED_NAME_ORIGINAL_FUNDING_PRICE)
private BigDecimal originalFundingPrice;
public static final String SERIALIZED_NAME_ORIGINAL_FUND_END_DATE = "originalFundEndDate";
@SerializedName(SERIALIZED_NAME_ORIGINAL_FUND_END_DATE)
private LocalDate originalFundEndDate;
public static final String SERIALIZED_NAME_ROLLOVER_VALIDITY_PERIOD_START_DATE = "rolloverValidityPeriodStartDate";
@SerializedName(SERIALIZED_NAME_ROLLOVER_VALIDITY_PERIOD_START_DATE)
private LocalDate rolloverValidityPeriodStartDate;
public static final String SERIALIZED_NAME_ROLLOVER_VALIDITY_PERIOD_END_DATE = "rolloverValidityPeriodEndDate";
@SerializedName(SERIALIZED_NAME_ROLLOVER_VALIDITY_PERIOD_END_DATE)
private LocalDate rolloverValidityPeriodEndDate;
public static final String SERIALIZED_NAME_PREPAID_BALANCE_ID = "prepaidBalanceId";
@SerializedName(SERIALIZED_NAME_PREPAID_BALANCE_ID)
private String prepaidBalanceId;
public static final String SERIALIZED_NAME_PRIORITY = "priority";
@SerializedName(SERIALIZED_NAME_PRIORITY)
private Integer priority;
public static final String SERIALIZED_NAME_SOURCE_ID = "sourceId";
@SerializedName(SERIALIZED_NAME_SOURCE_ID)
private String sourceId;
public static final String SERIALIZED_NAME_START_DATE = "startDate";
@SerializedName(SERIALIZED_NAME_START_DATE)
private LocalDate startDate;
public static final String SERIALIZED_NAME_VP_SUMMARY_ID = "vpSummaryId";
@SerializedName(SERIALIZED_NAME_VP_SUMMARY_ID)
private String vpSummaryId;
public static final String SERIALIZED_NAME_ROLLOVER_COUNT = "rolloverCount";
@SerializedName(SERIALIZED_NAME_ROLLOVER_COUNT)
private Integer rolloverCount;
public static final String SERIALIZED_NAME_ORIGIN_FUND_ID = "originFundId";
@SerializedName(SERIALIZED_NAME_ORIGIN_FUND_ID)
private String originFundId;
public static final String SERIALIZED_NAME_ROLLOVER_APPLY_OPTION = "rolloverApplyOption";
@SerializedName(SERIALIZED_NAME_ROLLOVER_APPLY_OPTION)
private String rolloverApplyOption;
public static final String SERIALIZED_NAME_DONE = "done";
@SerializedName(SERIALIZED_NAME_DONE)
private Integer done;
public static final String SERIALIZED_NAME_ACCOUNT = "account";
@SerializedName(SERIALIZED_NAME_ACCOUNT)
private ExpandedAccount account;
public static final String SERIALIZED_NAME_PREPAID_BALANCE = "prepaidBalance";
@SerializedName(SERIALIZED_NAME_PREPAID_BALANCE)
private ExpandedPrepaidBalance prepaidBalance;
public static final String SERIALIZED_NAME_SOURCE = "source";
@SerializedName(SERIALIZED_NAME_SOURCE)
private ExpandedRatePlanCharge source;
public static final String SERIALIZED_NAME_VP_SUMMARY = "vpSummary";
@SerializedName(SERIALIZED_NAME_VP_SUMMARY)
private ExpandedValidityPeriodSummary vpSummary;
public ExpandedPrepaidBalanceFund() {
}
public ExpandedPrepaidBalanceFund id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public ExpandedPrepaidBalanceFund createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* Get createdById
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public ExpandedPrepaidBalanceFund createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* Get createdDate
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public ExpandedPrepaidBalanceFund updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* Get updatedById
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public ExpandedPrepaidBalanceFund updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* Get updatedDate
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
public ExpandedPrepaidBalanceFund accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Get accountId
* @return accountId
*/
@javax.annotation.Nullable
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public ExpandedPrepaidBalanceFund balance(BigDecimal balance) {
this.balance = balance;
return this;
}
/**
* Get balance
* @return balance
*/
@javax.annotation.Nullable
public BigDecimal getBalance() {
return balance;
}
public void setBalance(BigDecimal balance) {
this.balance = balance;
}
public ExpandedPrepaidBalanceFund chargeSegmentNumber(Integer chargeSegmentNumber) {
this.chargeSegmentNumber = chargeSegmentNumber;
return this;
}
/**
* Get chargeSegmentNumber
* @return chargeSegmentNumber
*/
@javax.annotation.Nullable
public Integer getChargeSegmentNumber() {
return chargeSegmentNumber;
}
public void setChargeSegmentNumber(Integer chargeSegmentNumber) {
this.chargeSegmentNumber = chargeSegmentNumber;
}
public ExpandedPrepaidBalanceFund endDate(LocalDate endDate) {
this.endDate = endDate;
return this;
}
/**
* Get endDate
* @return endDate
*/
@javax.annotation.Nullable
public LocalDate getEndDate() {
return endDate;
}
public void setEndDate(LocalDate endDate) {
this.endDate = endDate;
}
public ExpandedPrepaidBalanceFund fundedBalance(BigDecimal fundedBalance) {
this.fundedBalance = fundedBalance;
return this;
}
/**
* Get fundedBalance
* @return fundedBalance
*/
@javax.annotation.Nullable
public BigDecimal getFundedBalance() {
return fundedBalance;
}
public void setFundedBalance(BigDecimal fundedBalance) {
this.fundedBalance = fundedBalance;
}
public ExpandedPrepaidBalanceFund fundSourceType(String fundSourceType) {
this.fundSourceType = fundSourceType;
return this;
}
/**
* Get fundSourceType
* @return fundSourceType
*/
@javax.annotation.Nullable
public String getFundSourceType() {
return fundSourceType;
}
public void setFundSourceType(String fundSourceType) {
this.fundSourceType = fundSourceType;
}
public ExpandedPrepaidBalanceFund fundingPrice(BigDecimal fundingPrice) {
this.fundingPrice = fundingPrice;
return this;
}
/**
* Get fundingPrice
* @return fundingPrice
*/
@javax.annotation.Nullable
public BigDecimal getFundingPrice() {
return fundingPrice;
}
public void setFundingPrice(BigDecimal fundingPrice) {
this.fundingPrice = fundingPrice;
}
public ExpandedPrepaidBalanceFund totalBilled(BigDecimal totalBilled) {
this.totalBilled = totalBilled;
return this;
}
/**
* Get totalBilled
* @return totalBilled
*/
@javax.annotation.Nullable
public BigDecimal getTotalBilled() {
return totalBilled;
}
public void setTotalBilled(BigDecimal totalBilled) {
this.totalBilled = totalBilled;
}
public ExpandedPrepaidBalanceFund totalBalance(BigDecimal totalBalance) {
this.totalBalance = totalBalance;
return this;
}
/**
* Get totalBalance
* @return totalBalance
*/
@javax.annotation.Nullable
public BigDecimal getTotalBalance() {
return totalBalance;
}
public void setTotalBalance(BigDecimal totalBalance) {
this.totalBalance = totalBalance;
}
public ExpandedPrepaidBalanceFund originalTotalBalance(BigDecimal originalTotalBalance) {
this.originalTotalBalance = originalTotalBalance;
return this;
}
/**
* Get originalTotalBalance
* @return originalTotalBalance
*/
@javax.annotation.Nullable
public BigDecimal getOriginalTotalBalance() {
return originalTotalBalance;
}
public void setOriginalTotalBalance(BigDecimal originalTotalBalance) {
this.originalTotalBalance = originalTotalBalance;
}
public ExpandedPrepaidBalanceFund originalFundingPrice(BigDecimal originalFundingPrice) {
this.originalFundingPrice = originalFundingPrice;
return this;
}
/**
* Get originalFundingPrice
* @return originalFundingPrice
*/
@javax.annotation.Nullable
public BigDecimal getOriginalFundingPrice() {
return originalFundingPrice;
}
public void setOriginalFundingPrice(BigDecimal originalFundingPrice) {
this.originalFundingPrice = originalFundingPrice;
}
public ExpandedPrepaidBalanceFund originalFundEndDate(LocalDate originalFundEndDate) {
this.originalFundEndDate = originalFundEndDate;
return this;
}
/**
* Get originalFundEndDate
* @return originalFundEndDate
*/
@javax.annotation.Nullable
public LocalDate getOriginalFundEndDate() {
return originalFundEndDate;
}
public void setOriginalFundEndDate(LocalDate originalFundEndDate) {
this.originalFundEndDate = originalFundEndDate;
}
public ExpandedPrepaidBalanceFund rolloverValidityPeriodStartDate(LocalDate rolloverValidityPeriodStartDate) {
this.rolloverValidityPeriodStartDate = rolloverValidityPeriodStartDate;
return this;
}
/**
* Get rolloverValidityPeriodStartDate
* @return rolloverValidityPeriodStartDate
*/
@javax.annotation.Nullable
public LocalDate getRolloverValidityPeriodStartDate() {
return rolloverValidityPeriodStartDate;
}
public void setRolloverValidityPeriodStartDate(LocalDate rolloverValidityPeriodStartDate) {
this.rolloverValidityPeriodStartDate = rolloverValidityPeriodStartDate;
}
public ExpandedPrepaidBalanceFund rolloverValidityPeriodEndDate(LocalDate rolloverValidityPeriodEndDate) {
this.rolloverValidityPeriodEndDate = rolloverValidityPeriodEndDate;
return this;
}
/**
* Get rolloverValidityPeriodEndDate
* @return rolloverValidityPeriodEndDate
*/
@javax.annotation.Nullable
public LocalDate getRolloverValidityPeriodEndDate() {
return rolloverValidityPeriodEndDate;
}
public void setRolloverValidityPeriodEndDate(LocalDate rolloverValidityPeriodEndDate) {
this.rolloverValidityPeriodEndDate = rolloverValidityPeriodEndDate;
}
public ExpandedPrepaidBalanceFund prepaidBalanceId(String prepaidBalanceId) {
this.prepaidBalanceId = prepaidBalanceId;
return this;
}
/**
* Get prepaidBalanceId
* @return prepaidBalanceId
*/
@javax.annotation.Nullable
public String getPrepaidBalanceId() {
return prepaidBalanceId;
}
public void setPrepaidBalanceId(String prepaidBalanceId) {
this.prepaidBalanceId = prepaidBalanceId;
}
public ExpandedPrepaidBalanceFund priority(Integer priority) {
this.priority = priority;
return this;
}
/**
* Get priority
* @return priority
*/
@javax.annotation.Nullable
public Integer getPriority() {
return priority;
}
public void setPriority(Integer priority) {
this.priority = priority;
}
public ExpandedPrepaidBalanceFund sourceId(String sourceId) {
this.sourceId = sourceId;
return this;
}
/**
* Get sourceId
* @return sourceId
*/
@javax.annotation.Nullable
public String getSourceId() {
return sourceId;
}
public void setSourceId(String sourceId) {
this.sourceId = sourceId;
}
public ExpandedPrepaidBalanceFund startDate(LocalDate startDate) {
this.startDate = startDate;
return this;
}
/**
* Get startDate
* @return startDate
*/
@javax.annotation.Nullable
public LocalDate getStartDate() {
return startDate;
}
public void setStartDate(LocalDate startDate) {
this.startDate = startDate;
}
public ExpandedPrepaidBalanceFund vpSummaryId(String vpSummaryId) {
this.vpSummaryId = vpSummaryId;
return this;
}
/**
* Get vpSummaryId
* @return vpSummaryId
*/
@javax.annotation.Nullable
public String getVpSummaryId() {
return vpSummaryId;
}
public void setVpSummaryId(String vpSummaryId) {
this.vpSummaryId = vpSummaryId;
}
public ExpandedPrepaidBalanceFund rolloverCount(Integer rolloverCount) {
this.rolloverCount = rolloverCount;
return this;
}
/**
* Get rolloverCount
* @return rolloverCount
*/
@javax.annotation.Nullable
public Integer getRolloverCount() {
return rolloverCount;
}
public void setRolloverCount(Integer rolloverCount) {
this.rolloverCount = rolloverCount;
}
public ExpandedPrepaidBalanceFund originFundId(String originFundId) {
this.originFundId = originFundId;
return this;
}
/**
* Get originFundId
* @return originFundId
*/
@javax.annotation.Nullable
public String getOriginFundId() {
return originFundId;
}
public void setOriginFundId(String originFundId) {
this.originFundId = originFundId;
}
public ExpandedPrepaidBalanceFund rolloverApplyOption(String rolloverApplyOption) {
this.rolloverApplyOption = rolloverApplyOption;
return this;
}
/**
* Get rolloverApplyOption
* @return rolloverApplyOption
*/
@javax.annotation.Nullable
public String getRolloverApplyOption() {
return rolloverApplyOption;
}
public void setRolloverApplyOption(String rolloverApplyOption) {
this.rolloverApplyOption = rolloverApplyOption;
}
public ExpandedPrepaidBalanceFund done(Integer done) {
this.done = done;
return this;
}
/**
* Get done
* @return done
*/
@javax.annotation.Nullable
public Integer getDone() {
return done;
}
public void setDone(Integer done) {
this.done = done;
}
public ExpandedPrepaidBalanceFund account(ExpandedAccount account) {
this.account = account;
return this;
}
/**
* Get account
* @return account
*/
@javax.annotation.Nullable
public ExpandedAccount getAccount() {
return account;
}
public void setAccount(ExpandedAccount account) {
this.account = account;
}
public ExpandedPrepaidBalanceFund prepaidBalance(ExpandedPrepaidBalance prepaidBalance) {
this.prepaidBalance = prepaidBalance;
return this;
}
/**
* Get prepaidBalance
* @return prepaidBalance
*/
@javax.annotation.Nullable
public ExpandedPrepaidBalance getPrepaidBalance() {
return prepaidBalance;
}
public void setPrepaidBalance(ExpandedPrepaidBalance prepaidBalance) {
this.prepaidBalance = prepaidBalance;
}
public ExpandedPrepaidBalanceFund source(ExpandedRatePlanCharge source) {
this.source = source;
return this;
}
/**
* Get source
* @return source
*/
@javax.annotation.Nullable
public ExpandedRatePlanCharge getSource() {
return source;
}
public void setSource(ExpandedRatePlanCharge source) {
this.source = source;
}
public ExpandedPrepaidBalanceFund vpSummary(ExpandedValidityPeriodSummary vpSummary) {
this.vpSummary = vpSummary;
return this;
}
/**
* Get vpSummary
* @return vpSummary
*/
@javax.annotation.Nullable
public ExpandedValidityPeriodSummary getVpSummary() {
return vpSummary;
}
public void setVpSummary(ExpandedValidityPeriodSummary vpSummary) {
this.vpSummary = vpSummary;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the ExpandedPrepaidBalanceFund instance itself
*/
public ExpandedPrepaidBalanceFund putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ExpandedPrepaidBalanceFund expandedPrepaidBalanceFund = (ExpandedPrepaidBalanceFund) o;
return Objects.equals(this.id, expandedPrepaidBalanceFund.id) &&
Objects.equals(this.createdById, expandedPrepaidBalanceFund.createdById) &&
Objects.equals(this.createdDate, expandedPrepaidBalanceFund.createdDate) &&
Objects.equals(this.updatedById, expandedPrepaidBalanceFund.updatedById) &&
Objects.equals(this.updatedDate, expandedPrepaidBalanceFund.updatedDate) &&
Objects.equals(this.accountId, expandedPrepaidBalanceFund.accountId) &&
Objects.equals(this.balance, expandedPrepaidBalanceFund.balance) &&
Objects.equals(this.chargeSegmentNumber, expandedPrepaidBalanceFund.chargeSegmentNumber) &&
Objects.equals(this.endDate, expandedPrepaidBalanceFund.endDate) &&
Objects.equals(this.fundedBalance, expandedPrepaidBalanceFund.fundedBalance) &&
Objects.equals(this.fundSourceType, expandedPrepaidBalanceFund.fundSourceType) &&
Objects.equals(this.fundingPrice, expandedPrepaidBalanceFund.fundingPrice) &&
Objects.equals(this.totalBilled, expandedPrepaidBalanceFund.totalBilled) &&
Objects.equals(this.totalBalance, expandedPrepaidBalanceFund.totalBalance) &&
Objects.equals(this.originalTotalBalance, expandedPrepaidBalanceFund.originalTotalBalance) &&
Objects.equals(this.originalFundingPrice, expandedPrepaidBalanceFund.originalFundingPrice) &&
Objects.equals(this.originalFundEndDate, expandedPrepaidBalanceFund.originalFundEndDate) &&
Objects.equals(this.rolloverValidityPeriodStartDate, expandedPrepaidBalanceFund.rolloverValidityPeriodStartDate) &&
Objects.equals(this.rolloverValidityPeriodEndDate, expandedPrepaidBalanceFund.rolloverValidityPeriodEndDate) &&
Objects.equals(this.prepaidBalanceId, expandedPrepaidBalanceFund.prepaidBalanceId) &&
Objects.equals(this.priority, expandedPrepaidBalanceFund.priority) &&
Objects.equals(this.sourceId, expandedPrepaidBalanceFund.sourceId) &&
Objects.equals(this.startDate, expandedPrepaidBalanceFund.startDate) &&
Objects.equals(this.vpSummaryId, expandedPrepaidBalanceFund.vpSummaryId) &&
Objects.equals(this.rolloverCount, expandedPrepaidBalanceFund.rolloverCount) &&
Objects.equals(this.originFundId, expandedPrepaidBalanceFund.originFundId) &&
Objects.equals(this.rolloverApplyOption, expandedPrepaidBalanceFund.rolloverApplyOption) &&
Objects.equals(this.done, expandedPrepaidBalanceFund.done) &&
Objects.equals(this.account, expandedPrepaidBalanceFund.account) &&
Objects.equals(this.prepaidBalance, expandedPrepaidBalanceFund.prepaidBalance) &&
Objects.equals(this.source, expandedPrepaidBalanceFund.source) &&
Objects.equals(this.vpSummary, expandedPrepaidBalanceFund.vpSummary)&&
Objects.equals(this.additionalProperties, expandedPrepaidBalanceFund.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(id, createdById, createdDate, updatedById, updatedDate, accountId, balance, chargeSegmentNumber, endDate, fundedBalance, fundSourceType, fundingPrice, totalBilled, totalBalance, originalTotalBalance, originalFundingPrice, originalFundEndDate, rolloverValidityPeriodStartDate, rolloverValidityPeriodEndDate, prepaidBalanceId, priority, sourceId, startDate, vpSummaryId, rolloverCount, originFundId, rolloverApplyOption, done, account, prepaidBalance, source, vpSummary, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ExpandedPrepaidBalanceFund {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" balance: ").append(toIndentedString(balance)).append("\n");
sb.append(" chargeSegmentNumber: ").append(toIndentedString(chargeSegmentNumber)).append("\n");
sb.append(" endDate: ").append(toIndentedString(endDate)).append("\n");
sb.append(" fundedBalance: ").append(toIndentedString(fundedBalance)).append("\n");
sb.append(" fundSourceType: ").append(toIndentedString(fundSourceType)).append("\n");
sb.append(" fundingPrice: ").append(toIndentedString(fundingPrice)).append("\n");
sb.append(" totalBilled: ").append(toIndentedString(totalBilled)).append("\n");
sb.append(" totalBalance: ").append(toIndentedString(totalBalance)).append("\n");
sb.append(" originalTotalBalance: ").append(toIndentedString(originalTotalBalance)).append("\n");
sb.append(" originalFundingPrice: ").append(toIndentedString(originalFundingPrice)).append("\n");
sb.append(" originalFundEndDate: ").append(toIndentedString(originalFundEndDate)).append("\n");
sb.append(" rolloverValidityPeriodStartDate: ").append(toIndentedString(rolloverValidityPeriodStartDate)).append("\n");
sb.append(" rolloverValidityPeriodEndDate: ").append(toIndentedString(rolloverValidityPeriodEndDate)).append("\n");
sb.append(" prepaidBalanceId: ").append(toIndentedString(prepaidBalanceId)).append("\n");
sb.append(" priority: ").append(toIndentedString(priority)).append("\n");
sb.append(" sourceId: ").append(toIndentedString(sourceId)).append("\n");
sb.append(" startDate: ").append(toIndentedString(startDate)).append("\n");
sb.append(" vpSummaryId: ").append(toIndentedString(vpSummaryId)).append("\n");
sb.append(" rolloverCount: ").append(toIndentedString(rolloverCount)).append("\n");
sb.append(" originFundId: ").append(toIndentedString(originFundId)).append("\n");
sb.append(" rolloverApplyOption: ").append(toIndentedString(rolloverApplyOption)).append("\n");
sb.append(" done: ").append(toIndentedString(done)).append("\n");
sb.append(" account: ").append(toIndentedString(account)).append("\n");
sb.append(" prepaidBalance: ").append(toIndentedString(prepaidBalance)).append("\n");
sb.append(" source: ").append(toIndentedString(source)).append("\n");
sb.append(" vpSummary: ").append(toIndentedString(vpSummary)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("id");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
openapiFields.add("accountId");
openapiFields.add("balance");
openapiFields.add("chargeSegmentNumber");
openapiFields.add("endDate");
openapiFields.add("fundedBalance");
openapiFields.add("fundSourceType");
openapiFields.add("fundingPrice");
openapiFields.add("totalBilled");
openapiFields.add("totalBalance");
openapiFields.add("originalTotalBalance");
openapiFields.add("originalFundingPrice");
openapiFields.add("originalFundEndDate");
openapiFields.add("rolloverValidityPeriodStartDate");
openapiFields.add("rolloverValidityPeriodEndDate");
openapiFields.add("prepaidBalanceId");
openapiFields.add("priority");
openapiFields.add("sourceId");
openapiFields.add("startDate");
openapiFields.add("vpSummaryId");
openapiFields.add("rolloverCount");
openapiFields.add("originFundId");
openapiFields.add("rolloverApplyOption");
openapiFields.add("done");
openapiFields.add("account");
openapiFields.add("prepaidBalance");
openapiFields.add("source");
openapiFields.add("vpSummary");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to ExpandedPrepaidBalanceFund
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!ExpandedPrepaidBalanceFund.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in ExpandedPrepaidBalanceFund is not found in the empty JSON string", ExpandedPrepaidBalanceFund.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
if ((jsonObj.get("accountId") != null && !jsonObj.get("accountId").isJsonNull()) && !jsonObj.get("accountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountId").toString()));
}
if ((jsonObj.get("fundSourceType") != null && !jsonObj.get("fundSourceType").isJsonNull()) && !jsonObj.get("fundSourceType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `fundSourceType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("fundSourceType").toString()));
}
if ((jsonObj.get("prepaidBalanceId") != null && !jsonObj.get("prepaidBalanceId").isJsonNull()) && !jsonObj.get("prepaidBalanceId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `prepaidBalanceId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("prepaidBalanceId").toString()));
}
if ((jsonObj.get("sourceId") != null && !jsonObj.get("sourceId").isJsonNull()) && !jsonObj.get("sourceId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sourceId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sourceId").toString()));
}
if ((jsonObj.get("vpSummaryId") != null && !jsonObj.get("vpSummaryId").isJsonNull()) && !jsonObj.get("vpSummaryId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `vpSummaryId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("vpSummaryId").toString()));
}
if ((jsonObj.get("originFundId") != null && !jsonObj.get("originFundId").isJsonNull()) && !jsonObj.get("originFundId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `originFundId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("originFundId").toString()));
}
if ((jsonObj.get("rolloverApplyOption") != null && !jsonObj.get("rolloverApplyOption").isJsonNull()) && !jsonObj.get("rolloverApplyOption").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `rolloverApplyOption` to be a primitive type in the JSON string but got `%s`", jsonObj.get("rolloverApplyOption").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!ExpandedPrepaidBalanceFund.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'ExpandedPrepaidBalanceFund' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(ExpandedPrepaidBalanceFund.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, ExpandedPrepaidBalanceFund value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public ExpandedPrepaidBalanceFund read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
ExpandedPrepaidBalanceFund instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of ExpandedPrepaidBalanceFund given an JSON string
*
* @param jsonString JSON string
* @return An instance of ExpandedPrepaidBalanceFund
* @throws IOException if the JSON string is invalid with respect to ExpandedPrepaidBalanceFund
*/
public static ExpandedPrepaidBalanceFund fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, ExpandedPrepaidBalanceFund.class);
}
/**
* Convert an instance of ExpandedPrepaidBalanceFund to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy