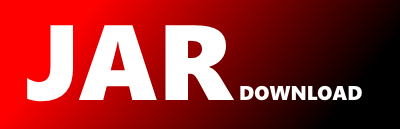
com.zuora.model.ExpandedProductRatePlanCharge Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.ExpandedProductRatePlan;
import com.zuora.model.ExpandedProductRatePlanChargeTier;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* ExpandedProductRatePlanCharge
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class ExpandedProductRatePlanCharge {
public static final String SERIALIZED_NAME_ACCOUNTING_CODE = "accountingCode";
@SerializedName(SERIALIZED_NAME_ACCOUNTING_CODE)
private String accountingCode;
public static final String SERIALIZED_NAME_APPLY_DISCOUNT_TO = "applyDiscountTo";
@SerializedName(SERIALIZED_NAME_APPLY_DISCOUNT_TO)
private String applyDiscountTo;
public static final String SERIALIZED_NAME_BILL_CYCLE_DAY = "billCycleDay";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_DAY)
private Long billCycleDay;
public static final String SERIALIZED_NAME_BILL_CYCLE_TYPE = "billCycleType";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_TYPE)
private String billCycleType;
public static final String SERIALIZED_NAME_BILLING_PERIOD = "billingPeriod";
@SerializedName(SERIALIZED_NAME_BILLING_PERIOD)
private String billingPeriod;
public static final String SERIALIZED_NAME_BILLING_PERIOD_ALIGNMENT = "billingPeriodAlignment";
@SerializedName(SERIALIZED_NAME_BILLING_PERIOD_ALIGNMENT)
private String billingPeriodAlignment;
public static final String SERIALIZED_NAME_BILLING_TIMING = "billingTiming";
@SerializedName(SERIALIZED_NAME_BILLING_TIMING)
private String billingTiming;
public static final String SERIALIZED_NAME_CHARGE_FUNCTION = "chargeFunction";
@SerializedName(SERIALIZED_NAME_CHARGE_FUNCTION)
private String chargeFunction;
public static final String SERIALIZED_NAME_CHARGE_MODEL = "chargeModel";
@SerializedName(SERIALIZED_NAME_CHARGE_MODEL)
private String chargeModel;
public static final String SERIALIZED_NAME_CHARGE_TYPE = "chargeType";
@SerializedName(SERIALIZED_NAME_CHARGE_TYPE)
private String chargeType;
public static final String SERIALIZED_NAME_CREDIT_OPTION = "creditOption";
@SerializedName(SERIALIZED_NAME_CREDIT_OPTION)
private String creditOption;
public static final String SERIALIZED_NAME_DEFAULT_QUANTITY = "defaultQuantity";
@SerializedName(SERIALIZED_NAME_DEFAULT_QUANTITY)
private BigDecimal defaultQuantity;
public static final String SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNT = "deferredRevenueAccount";
@SerializedName(SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNT)
private String deferredRevenueAccount;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_DISCOUNT_CLASS_ID = "discountClassId";
@SerializedName(SERIALIZED_NAME_DISCOUNT_CLASS_ID)
private String discountClassId;
public static final String SERIALIZED_NAME_DISCOUNT_LEVEL = "discountLevel";
@SerializedName(SERIALIZED_NAME_DISCOUNT_LEVEL)
private String discountLevel;
public static final String SERIALIZED_NAME_DRAWDOWN_RATE = "drawdownRate";
@SerializedName(SERIALIZED_NAME_DRAWDOWN_RATE)
private BigDecimal drawdownRate;
public static final String SERIALIZED_NAME_END_DATE_CONDITION = "endDateCondition";
@SerializedName(SERIALIZED_NAME_END_DATE_CONDITION)
private String endDateCondition;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING = "excludeItemBookingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBookingFromRevenueAccounting;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING = "excludeItemBillingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBillingFromRevenueAccounting;
public static final String SERIALIZED_NAME_INCLUDED_UNITS = "includedUnits";
@SerializedName(SERIALIZED_NAME_INCLUDED_UNITS)
private BigDecimal includedUnits;
public static final String SERIALIZED_NAME_IS_PREPAID = "isPrepaid";
@SerializedName(SERIALIZED_NAME_IS_PREPAID)
private Boolean isPrepaid;
public static final String SERIALIZED_NAME_IS_ROLLOVER = "isRollover";
@SerializedName(SERIALIZED_NAME_IS_ROLLOVER)
private Boolean isRollover;
public static final String SERIALIZED_NAME_IS_STACKED_DISCOUNT = "isStackedDiscount";
@SerializedName(SERIALIZED_NAME_IS_STACKED_DISCOUNT)
private Boolean isStackedDiscount;
public static final String SERIALIZED_NAME_LEGACY_REVENUE_REPORTING = "legacyRevenueReporting";
@SerializedName(SERIALIZED_NAME_LEGACY_REVENUE_REPORTING)
private Boolean legacyRevenueReporting;
public static final String SERIALIZED_NAME_LIST_PRICE_BASE = "listPriceBase";
@SerializedName(SERIALIZED_NAME_LIST_PRICE_BASE)
private String listPriceBase;
public static final String SERIALIZED_NAME_SPECIFIC_LIST_PRICE_BASE = "specificListPriceBase";
@SerializedName(SERIALIZED_NAME_SPECIFIC_LIST_PRICE_BASE)
private Integer specificListPriceBase;
public static final String SERIALIZED_NAME_MAX_QUANTITY = "maxQuantity";
@SerializedName(SERIALIZED_NAME_MAX_QUANTITY)
private BigDecimal maxQuantity;
public static final String SERIALIZED_NAME_MIN_QUANTITY = "minQuantity";
@SerializedName(SERIALIZED_NAME_MIN_QUANTITY)
private BigDecimal minQuantity;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_NUMBER_OF_PERIOD = "numberOfPeriod";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_PERIOD)
private Long numberOfPeriod;
public static final String SERIALIZED_NAME_OVERAGE_CALCULATION_OPTION = "overageCalculationOption";
@SerializedName(SERIALIZED_NAME_OVERAGE_CALCULATION_OPTION)
private String overageCalculationOption;
public static final String SERIALIZED_NAME_OVERAGE_UNUSED_UNITS_CREDIT_OPTION = "overageUnusedUnitsCreditOption";
@SerializedName(SERIALIZED_NAME_OVERAGE_UNUSED_UNITS_CREDIT_OPTION)
private String overageUnusedUnitsCreditOption;
public static final String SERIALIZED_NAME_PREPAID_OPERATION_TYPE = "prepaidOperationType";
@SerializedName(SERIALIZED_NAME_PREPAID_OPERATION_TYPE)
private String prepaidOperationType;
public static final String SERIALIZED_NAME_PRORATION_OPTION = "prorationOption";
@SerializedName(SERIALIZED_NAME_PRORATION_OPTION)
private String prorationOption;
public static final String SERIALIZED_NAME_PREPAID_QUANTITY = "prepaidQuantity";
@SerializedName(SERIALIZED_NAME_PREPAID_QUANTITY)
private BigDecimal prepaidQuantity;
public static final String SERIALIZED_NAME_PREPAID_TOTAL_QUANTITY = "prepaidTotalQuantity";
@SerializedName(SERIALIZED_NAME_PREPAID_TOTAL_QUANTITY)
private BigDecimal prepaidTotalQuantity;
public static final String SERIALIZED_NAME_PRICE_CHANGE_OPTION = "priceChangeOption";
@SerializedName(SERIALIZED_NAME_PRICE_CHANGE_OPTION)
private String priceChangeOption;
public static final String SERIALIZED_NAME_PRICE_INCREASE_PERCENTAGE = "priceIncreasePercentage";
@SerializedName(SERIALIZED_NAME_PRICE_INCREASE_PERCENTAGE)
private BigDecimal priceIncreasePercentage;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_NUMBER = "productRatePlanChargeNumber";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_NUMBER)
private String productRatePlanChargeNumber;
public static final String SERIALIZED_NAME_RATING_GROUP = "ratingGroup";
@SerializedName(SERIALIZED_NAME_RATING_GROUP)
private String ratingGroup;
public static final String SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNT = "recognizedRevenueAccount";
@SerializedName(SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNT)
private String recognizedRevenueAccount;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME = "revenueRecognitionRuleName";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME)
private String revenueRecognitionRuleName;
public static final String SERIALIZED_NAME_REV_REC_TRIGGER_CONDITION = "revRecTriggerCondition";
@SerializedName(SERIALIZED_NAME_REV_REC_TRIGGER_CONDITION)
private String revRecTriggerCondition;
public static final String SERIALIZED_NAME_ROLLOVER_APPLY = "rolloverApply";
@SerializedName(SERIALIZED_NAME_ROLLOVER_APPLY)
private String rolloverApply;
public static final String SERIALIZED_NAME_ROLLOVER_PERIODS = "rolloverPeriods";
@SerializedName(SERIALIZED_NAME_ROLLOVER_PERIODS)
private Long rolloverPeriods;
public static final String SERIALIZED_NAME_ROLLOVER_PERIOD_LENGTH = "rolloverPeriodLength";
@SerializedName(SERIALIZED_NAME_ROLLOVER_PERIOD_LENGTH)
private Integer rolloverPeriodLength;
public static final String SERIALIZED_NAME_SMOOTHING_MODEL = "smoothingModel";
@SerializedName(SERIALIZED_NAME_SMOOTHING_MODEL)
private String smoothingModel;
public static final String SERIALIZED_NAME_SPECIFIC_BILLING_PERIOD = "specificBillingPeriod";
@SerializedName(SERIALIZED_NAME_SPECIFIC_BILLING_PERIOD)
private Long specificBillingPeriod;
public static final String SERIALIZED_NAME_TAXABLE = "taxable";
@SerializedName(SERIALIZED_NAME_TAXABLE)
private Boolean taxable;
public static final String SERIALIZED_NAME_TAX_CODE = "taxCode";
@SerializedName(SERIALIZED_NAME_TAX_CODE)
private String taxCode;
public static final String SERIALIZED_NAME_TAX_MODE = "taxMode";
@SerializedName(SERIALIZED_NAME_TAX_MODE)
private String taxMode;
public static final String SERIALIZED_NAME_TRIGGER_EVENT = "triggerEvent";
@SerializedName(SERIALIZED_NAME_TRIGGER_EVENT)
private String triggerEvent;
public static final String SERIALIZED_NAME_UP_TO_PERIODS = "upToPeriods";
@SerializedName(SERIALIZED_NAME_UP_TO_PERIODS)
private Long upToPeriods;
public static final String SERIALIZED_NAME_UP_TO_PERIODS_TYPE = "upToPeriodsType";
@SerializedName(SERIALIZED_NAME_UP_TO_PERIODS_TYPE)
private String upToPeriodsType;
public static final String SERIALIZED_NAME_USAGE_RECORD_RATING_OPTION = "usageRecordRatingOption";
@SerializedName(SERIALIZED_NAME_USAGE_RECORD_RATING_OPTION)
private String usageRecordRatingOption;
public static final String SERIALIZED_NAME_USE_DISCOUNT_SPECIFIC_ACCOUNTING_CODE = "useDiscountSpecificAccountingCode";
@SerializedName(SERIALIZED_NAME_USE_DISCOUNT_SPECIFIC_ACCOUNTING_CODE)
private Boolean useDiscountSpecificAccountingCode;
public static final String SERIALIZED_NAME_USE_TENANT_DEFAULT_FOR_PRICE_CHANGE = "useTenantDefaultForPriceChange";
@SerializedName(SERIALIZED_NAME_USE_TENANT_DEFAULT_FOR_PRICE_CHANGE)
private Boolean useTenantDefaultForPriceChange;
public static final String SERIALIZED_NAME_VALIDITY_PERIOD_TYPE = "validityPeriodType";
@SerializedName(SERIALIZED_NAME_VALIDITY_PERIOD_TYPE)
private String validityPeriodType;
public static final String SERIALIZED_NAME_WEEKLY_BILL_CYCLE_DAY = "weeklyBillCycleDay";
@SerializedName(SERIALIZED_NAME_WEEKLY_BILL_CYCLE_DAY)
private String weeklyBillCycleDay;
public static final String SERIALIZED_NAME_PRICE_UPSELL_QUANTITY_STACKED = "priceUpsellQuantityStacked";
@SerializedName(SERIALIZED_NAME_PRICE_UPSELL_QUANTITY_STACKED)
private Boolean priceUpsellQuantityStacked;
public static final String SERIALIZED_NAME_DELIVERY_SCHEDULE_ID = "deliveryScheduleId";
@SerializedName(SERIALIZED_NAME_DELIVERY_SCHEDULE_ID)
private String deliveryScheduleId;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public static final String SERIALIZED_NAME_COMMITMENT_TYPE = "commitmentType";
@SerializedName(SERIALIZED_NAME_COMMITMENT_TYPE)
private String commitmentType;
public static final String SERIALIZED_NAME_IS_COMMITTED = "isCommitted";
@SerializedName(SERIALIZED_NAME_IS_COMMITTED)
private Boolean isCommitted;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_ID = "productRatePlanId";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_ID)
private String productRatePlanId;
public static final String SERIALIZED_NAME_IS_UNBILLED = "isUnbilled";
@SerializedName(SERIALIZED_NAME_IS_UNBILLED)
private Boolean isUnbilled;
public static final String SERIALIZED_NAME_IS_ALLOCATION_ELIGIBLE = "isAllocationEligible";
@SerializedName(SERIALIZED_NAME_IS_ALLOCATION_ELIGIBLE)
private Boolean isAllocationEligible;
public static final String SERIALIZED_NAME_PRODUCT_CATEGORY = "productCategory";
@SerializedName(SERIALIZED_NAME_PRODUCT_CATEGORY)
private String productCategory;
public static final String SERIALIZED_NAME_PRODUCT_CLASS = "productClass";
@SerializedName(SERIALIZED_NAME_PRODUCT_CLASS)
private String productClass;
public static final String SERIALIZED_NAME_PRODUCT_FAMILY = "productFamily";
@SerializedName(SERIALIZED_NAME_PRODUCT_FAMILY)
private String productFamily;
public static final String SERIALIZED_NAME_PRODUCT_LINE = "productLine";
@SerializedName(SERIALIZED_NAME_PRODUCT_LINE)
private String productLine;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_TIMING = "revenueRecognitionTiming";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_TIMING)
private String revenueRecognitionTiming;
public static final String SERIALIZED_NAME_REVENUE_AMORTIZATION_METHOD = "revenueAmortizationMethod";
@SerializedName(SERIALIZED_NAME_REVENUE_AMORTIZATION_METHOD)
private String revenueAmortizationMethod;
public static final String SERIALIZED_NAME_APPLY_TO_BILLING_PERIOD_PARTIALLY = "applyToBillingPeriodPartially";
@SerializedName(SERIALIZED_NAME_APPLY_TO_BILLING_PERIOD_PARTIALLY)
private Boolean applyToBillingPeriodPartially;
public static final String SERIALIZED_NAME_ACCOUNT_RECEIVABLE_ACCOUNTING_CODE_ID = "accountReceivableAccountingCodeId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_RECEIVABLE_ACCOUNTING_CODE_ID)
private String accountReceivableAccountingCodeId;
public static final String SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE_ID = "recognizedRevenueAccountingCodeId";
@SerializedName(SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE_ID)
private String recognizedRevenueAccountingCodeId;
public static final String SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE_ID = "deferredRevenueAccountingCodeId";
@SerializedName(SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE_ID)
private String deferredRevenueAccountingCodeId;
public static final String SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNTING_CODE_ID = "adjustmentLiabilityAccountingCodeId";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNTING_CODE_ID)
private String adjustmentLiabilityAccountingCodeId;
public static final String SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNTING_CODE_ID = "adjustmentRevenueAccountingCodeId";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNTING_CODE_ID)
private String adjustmentRevenueAccountingCodeId;
public static final String SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNTING_CODE_ID = "contractAssetAccountingCodeId";
@SerializedName(SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNTING_CODE_ID)
private String contractAssetAccountingCodeId;
public static final String SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNTING_CODE_ID = "contractLiabilityAccountingCodeId";
@SerializedName(SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNTING_CODE_ID)
private String contractLiabilityAccountingCodeId;
public static final String SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNTING_CODE_ID = "contractRecognizedRevenueAccountingCodeId";
@SerializedName(SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNTING_CODE_ID)
private String contractRecognizedRevenueAccountingCodeId;
public static final String SERIALIZED_NAME_UNBILLED_RECEIVABLES_ACCOUNTING_CODE_ID = "unbilledReceivablesAccountingCodeId";
@SerializedName(SERIALIZED_NAME_UNBILLED_RECEIVABLES_ACCOUNTING_CODE_ID)
private String unbilledReceivablesAccountingCodeId;
public static final String SERIALIZED_NAME_REV_REC_CODE = "revRecCode";
@SerializedName(SERIALIZED_NAME_REV_REC_CODE)
private String revRecCode;
public static final String SERIALIZED_NAME_U_O_M = "uOM";
@SerializedName(SERIALIZED_NAME_U_O_M)
private String uOM;
public static final String SERIALIZED_NAME_DRAWDOWN_UOM = "drawdownUom";
@SerializedName(SERIALIZED_NAME_DRAWDOWN_UOM)
private String drawdownUom;
public static final String SERIALIZED_NAME_PREPAID_UOM = "prepaidUom";
@SerializedName(SERIALIZED_NAME_PREPAID_UOM)
private String prepaidUom;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN = "productRatePlan";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN)
private ExpandedProductRatePlan productRatePlan;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_TIERS = "productRatePlanChargeTiers";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_TIERS)
private List productRatePlanChargeTiers;
public ExpandedProductRatePlanCharge() {
}
public ExpandedProductRatePlanCharge accountingCode(String accountingCode) {
this.accountingCode = accountingCode;
return this;
}
/**
* Get accountingCode
* @return accountingCode
*/
@javax.annotation.Nullable
public String getAccountingCode() {
return accountingCode;
}
public void setAccountingCode(String accountingCode) {
this.accountingCode = accountingCode;
}
public ExpandedProductRatePlanCharge applyDiscountTo(String applyDiscountTo) {
this.applyDiscountTo = applyDiscountTo;
return this;
}
/**
* Get applyDiscountTo
* @return applyDiscountTo
*/
@javax.annotation.Nullable
public String getApplyDiscountTo() {
return applyDiscountTo;
}
public void setApplyDiscountTo(String applyDiscountTo) {
this.applyDiscountTo = applyDiscountTo;
}
public ExpandedProductRatePlanCharge billCycleDay(Long billCycleDay) {
this.billCycleDay = billCycleDay;
return this;
}
/**
* Get billCycleDay
* @return billCycleDay
*/
@javax.annotation.Nullable
public Long getBillCycleDay() {
return billCycleDay;
}
public void setBillCycleDay(Long billCycleDay) {
this.billCycleDay = billCycleDay;
}
public ExpandedProductRatePlanCharge billCycleType(String billCycleType) {
this.billCycleType = billCycleType;
return this;
}
/**
* Get billCycleType
* @return billCycleType
*/
@javax.annotation.Nullable
public String getBillCycleType() {
return billCycleType;
}
public void setBillCycleType(String billCycleType) {
this.billCycleType = billCycleType;
}
public ExpandedProductRatePlanCharge billingPeriod(String billingPeriod) {
this.billingPeriod = billingPeriod;
return this;
}
/**
* Get billingPeriod
* @return billingPeriod
*/
@javax.annotation.Nullable
public String getBillingPeriod() {
return billingPeriod;
}
public void setBillingPeriod(String billingPeriod) {
this.billingPeriod = billingPeriod;
}
public ExpandedProductRatePlanCharge billingPeriodAlignment(String billingPeriodAlignment) {
this.billingPeriodAlignment = billingPeriodAlignment;
return this;
}
/**
* Get billingPeriodAlignment
* @return billingPeriodAlignment
*/
@javax.annotation.Nullable
public String getBillingPeriodAlignment() {
return billingPeriodAlignment;
}
public void setBillingPeriodAlignment(String billingPeriodAlignment) {
this.billingPeriodAlignment = billingPeriodAlignment;
}
public ExpandedProductRatePlanCharge billingTiming(String billingTiming) {
this.billingTiming = billingTiming;
return this;
}
/**
* Get billingTiming
* @return billingTiming
*/
@javax.annotation.Nullable
public String getBillingTiming() {
return billingTiming;
}
public void setBillingTiming(String billingTiming) {
this.billingTiming = billingTiming;
}
public ExpandedProductRatePlanCharge chargeFunction(String chargeFunction) {
this.chargeFunction = chargeFunction;
return this;
}
/**
* Get chargeFunction
* @return chargeFunction
*/
@javax.annotation.Nullable
public String getChargeFunction() {
return chargeFunction;
}
public void setChargeFunction(String chargeFunction) {
this.chargeFunction = chargeFunction;
}
public ExpandedProductRatePlanCharge chargeModel(String chargeModel) {
this.chargeModel = chargeModel;
return this;
}
/**
* Get chargeModel
* @return chargeModel
*/
@javax.annotation.Nullable
public String getChargeModel() {
return chargeModel;
}
public void setChargeModel(String chargeModel) {
this.chargeModel = chargeModel;
}
public ExpandedProductRatePlanCharge chargeType(String chargeType) {
this.chargeType = chargeType;
return this;
}
/**
* Get chargeType
* @return chargeType
*/
@javax.annotation.Nullable
public String getChargeType() {
return chargeType;
}
public void setChargeType(String chargeType) {
this.chargeType = chargeType;
}
public ExpandedProductRatePlanCharge creditOption(String creditOption) {
this.creditOption = creditOption;
return this;
}
/**
* Get creditOption
* @return creditOption
*/
@javax.annotation.Nullable
public String getCreditOption() {
return creditOption;
}
public void setCreditOption(String creditOption) {
this.creditOption = creditOption;
}
public ExpandedProductRatePlanCharge defaultQuantity(BigDecimal defaultQuantity) {
this.defaultQuantity = defaultQuantity;
return this;
}
/**
* Get defaultQuantity
* @return defaultQuantity
*/
@javax.annotation.Nullable
public BigDecimal getDefaultQuantity() {
return defaultQuantity;
}
public void setDefaultQuantity(BigDecimal defaultQuantity) {
this.defaultQuantity = defaultQuantity;
}
public ExpandedProductRatePlanCharge deferredRevenueAccount(String deferredRevenueAccount) {
this.deferredRevenueAccount = deferredRevenueAccount;
return this;
}
/**
* Get deferredRevenueAccount
* @return deferredRevenueAccount
*/
@javax.annotation.Nullable
public String getDeferredRevenueAccount() {
return deferredRevenueAccount;
}
public void setDeferredRevenueAccount(String deferredRevenueAccount) {
this.deferredRevenueAccount = deferredRevenueAccount;
}
public ExpandedProductRatePlanCharge description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public ExpandedProductRatePlanCharge discountClassId(String discountClassId) {
this.discountClassId = discountClassId;
return this;
}
/**
* Get discountClassId
* @return discountClassId
*/
@javax.annotation.Nullable
public String getDiscountClassId() {
return discountClassId;
}
public void setDiscountClassId(String discountClassId) {
this.discountClassId = discountClassId;
}
public ExpandedProductRatePlanCharge discountLevel(String discountLevel) {
this.discountLevel = discountLevel;
return this;
}
/**
* Get discountLevel
* @return discountLevel
*/
@javax.annotation.Nullable
public String getDiscountLevel() {
return discountLevel;
}
public void setDiscountLevel(String discountLevel) {
this.discountLevel = discountLevel;
}
public ExpandedProductRatePlanCharge drawdownRate(BigDecimal drawdownRate) {
this.drawdownRate = drawdownRate;
return this;
}
/**
* Get drawdownRate
* @return drawdownRate
*/
@javax.annotation.Nullable
public BigDecimal getDrawdownRate() {
return drawdownRate;
}
public void setDrawdownRate(BigDecimal drawdownRate) {
this.drawdownRate = drawdownRate;
}
public ExpandedProductRatePlanCharge endDateCondition(String endDateCondition) {
this.endDateCondition = endDateCondition;
return this;
}
/**
* Get endDateCondition
* @return endDateCondition
*/
@javax.annotation.Nullable
public String getEndDateCondition() {
return endDateCondition;
}
public void setEndDateCondition(String endDateCondition) {
this.endDateCondition = endDateCondition;
}
public ExpandedProductRatePlanCharge excludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
return this;
}
/**
* Get excludeItemBookingFromRevenueAccounting
* @return excludeItemBookingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBookingFromRevenueAccounting() {
return excludeItemBookingFromRevenueAccounting;
}
public void setExcludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
}
public ExpandedProductRatePlanCharge excludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
return this;
}
/**
* Get excludeItemBillingFromRevenueAccounting
* @return excludeItemBillingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBillingFromRevenueAccounting() {
return excludeItemBillingFromRevenueAccounting;
}
public void setExcludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
}
public ExpandedProductRatePlanCharge includedUnits(BigDecimal includedUnits) {
this.includedUnits = includedUnits;
return this;
}
/**
* Get includedUnits
* @return includedUnits
*/
@javax.annotation.Nullable
public BigDecimal getIncludedUnits() {
return includedUnits;
}
public void setIncludedUnits(BigDecimal includedUnits) {
this.includedUnits = includedUnits;
}
public ExpandedProductRatePlanCharge isPrepaid(Boolean isPrepaid) {
this.isPrepaid = isPrepaid;
return this;
}
/**
* Get isPrepaid
* @return isPrepaid
*/
@javax.annotation.Nullable
public Boolean getIsPrepaid() {
return isPrepaid;
}
public void setIsPrepaid(Boolean isPrepaid) {
this.isPrepaid = isPrepaid;
}
public ExpandedProductRatePlanCharge isRollover(Boolean isRollover) {
this.isRollover = isRollover;
return this;
}
/**
* Get isRollover
* @return isRollover
*/
@javax.annotation.Nullable
public Boolean getIsRollover() {
return isRollover;
}
public void setIsRollover(Boolean isRollover) {
this.isRollover = isRollover;
}
public ExpandedProductRatePlanCharge isStackedDiscount(Boolean isStackedDiscount) {
this.isStackedDiscount = isStackedDiscount;
return this;
}
/**
* Get isStackedDiscount
* @return isStackedDiscount
*/
@javax.annotation.Nullable
public Boolean getIsStackedDiscount() {
return isStackedDiscount;
}
public void setIsStackedDiscount(Boolean isStackedDiscount) {
this.isStackedDiscount = isStackedDiscount;
}
public ExpandedProductRatePlanCharge legacyRevenueReporting(Boolean legacyRevenueReporting) {
this.legacyRevenueReporting = legacyRevenueReporting;
return this;
}
/**
* Get legacyRevenueReporting
* @return legacyRevenueReporting
*/
@javax.annotation.Nullable
public Boolean getLegacyRevenueReporting() {
return legacyRevenueReporting;
}
public void setLegacyRevenueReporting(Boolean legacyRevenueReporting) {
this.legacyRevenueReporting = legacyRevenueReporting;
}
public ExpandedProductRatePlanCharge listPriceBase(String listPriceBase) {
this.listPriceBase = listPriceBase;
return this;
}
/**
* Get listPriceBase
* @return listPriceBase
*/
@javax.annotation.Nullable
public String getListPriceBase() {
return listPriceBase;
}
public void setListPriceBase(String listPriceBase) {
this.listPriceBase = listPriceBase;
}
public ExpandedProductRatePlanCharge specificListPriceBase(Integer specificListPriceBase) {
this.specificListPriceBase = specificListPriceBase;
return this;
}
/**
* Get specificListPriceBase
* @return specificListPriceBase
*/
@javax.annotation.Nullable
public Integer getSpecificListPriceBase() {
return specificListPriceBase;
}
public void setSpecificListPriceBase(Integer specificListPriceBase) {
this.specificListPriceBase = specificListPriceBase;
}
public ExpandedProductRatePlanCharge maxQuantity(BigDecimal maxQuantity) {
this.maxQuantity = maxQuantity;
return this;
}
/**
* Get maxQuantity
* @return maxQuantity
*/
@javax.annotation.Nullable
public BigDecimal getMaxQuantity() {
return maxQuantity;
}
public void setMaxQuantity(BigDecimal maxQuantity) {
this.maxQuantity = maxQuantity;
}
public ExpandedProductRatePlanCharge minQuantity(BigDecimal minQuantity) {
this.minQuantity = minQuantity;
return this;
}
/**
* Get minQuantity
* @return minQuantity
*/
@javax.annotation.Nullable
public BigDecimal getMinQuantity() {
return minQuantity;
}
public void setMinQuantity(BigDecimal minQuantity) {
this.minQuantity = minQuantity;
}
public ExpandedProductRatePlanCharge name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
*/
@javax.annotation.Nullable
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public ExpandedProductRatePlanCharge numberOfPeriod(Long numberOfPeriod) {
this.numberOfPeriod = numberOfPeriod;
return this;
}
/**
* Get numberOfPeriod
* @return numberOfPeriod
*/
@javax.annotation.Nullable
public Long getNumberOfPeriod() {
return numberOfPeriod;
}
public void setNumberOfPeriod(Long numberOfPeriod) {
this.numberOfPeriod = numberOfPeriod;
}
public ExpandedProductRatePlanCharge overageCalculationOption(String overageCalculationOption) {
this.overageCalculationOption = overageCalculationOption;
return this;
}
/**
* Get overageCalculationOption
* @return overageCalculationOption
*/
@javax.annotation.Nullable
public String getOverageCalculationOption() {
return overageCalculationOption;
}
public void setOverageCalculationOption(String overageCalculationOption) {
this.overageCalculationOption = overageCalculationOption;
}
public ExpandedProductRatePlanCharge overageUnusedUnitsCreditOption(String overageUnusedUnitsCreditOption) {
this.overageUnusedUnitsCreditOption = overageUnusedUnitsCreditOption;
return this;
}
/**
* Get overageUnusedUnitsCreditOption
* @return overageUnusedUnitsCreditOption
*/
@javax.annotation.Nullable
public String getOverageUnusedUnitsCreditOption() {
return overageUnusedUnitsCreditOption;
}
public void setOverageUnusedUnitsCreditOption(String overageUnusedUnitsCreditOption) {
this.overageUnusedUnitsCreditOption = overageUnusedUnitsCreditOption;
}
public ExpandedProductRatePlanCharge prepaidOperationType(String prepaidOperationType) {
this.prepaidOperationType = prepaidOperationType;
return this;
}
/**
* Get prepaidOperationType
* @return prepaidOperationType
*/
@javax.annotation.Nullable
public String getPrepaidOperationType() {
return prepaidOperationType;
}
public void setPrepaidOperationType(String prepaidOperationType) {
this.prepaidOperationType = prepaidOperationType;
}
public ExpandedProductRatePlanCharge prorationOption(String prorationOption) {
this.prorationOption = prorationOption;
return this;
}
/**
* Get prorationOption
* @return prorationOption
*/
@javax.annotation.Nullable
public String getProrationOption() {
return prorationOption;
}
public void setProrationOption(String prorationOption) {
this.prorationOption = prorationOption;
}
public ExpandedProductRatePlanCharge prepaidQuantity(BigDecimal prepaidQuantity) {
this.prepaidQuantity = prepaidQuantity;
return this;
}
/**
* Get prepaidQuantity
* @return prepaidQuantity
*/
@javax.annotation.Nullable
public BigDecimal getPrepaidQuantity() {
return prepaidQuantity;
}
public void setPrepaidQuantity(BigDecimal prepaidQuantity) {
this.prepaidQuantity = prepaidQuantity;
}
public ExpandedProductRatePlanCharge prepaidTotalQuantity(BigDecimal prepaidTotalQuantity) {
this.prepaidTotalQuantity = prepaidTotalQuantity;
return this;
}
/**
* Get prepaidTotalQuantity
* @return prepaidTotalQuantity
*/
@javax.annotation.Nullable
public BigDecimal getPrepaidTotalQuantity() {
return prepaidTotalQuantity;
}
public void setPrepaidTotalQuantity(BigDecimal prepaidTotalQuantity) {
this.prepaidTotalQuantity = prepaidTotalQuantity;
}
public ExpandedProductRatePlanCharge priceChangeOption(String priceChangeOption) {
this.priceChangeOption = priceChangeOption;
return this;
}
/**
* Get priceChangeOption
* @return priceChangeOption
*/
@javax.annotation.Nullable
public String getPriceChangeOption() {
return priceChangeOption;
}
public void setPriceChangeOption(String priceChangeOption) {
this.priceChangeOption = priceChangeOption;
}
public ExpandedProductRatePlanCharge priceIncreasePercentage(BigDecimal priceIncreasePercentage) {
this.priceIncreasePercentage = priceIncreasePercentage;
return this;
}
/**
* Get priceIncreasePercentage
* @return priceIncreasePercentage
*/
@javax.annotation.Nullable
public BigDecimal getPriceIncreasePercentage() {
return priceIncreasePercentage;
}
public void setPriceIncreasePercentage(BigDecimal priceIncreasePercentage) {
this.priceIncreasePercentage = priceIncreasePercentage;
}
public ExpandedProductRatePlanCharge productRatePlanChargeNumber(String productRatePlanChargeNumber) {
this.productRatePlanChargeNumber = productRatePlanChargeNumber;
return this;
}
/**
* Get productRatePlanChargeNumber
* @return productRatePlanChargeNumber
*/
@javax.annotation.Nullable
public String getProductRatePlanChargeNumber() {
return productRatePlanChargeNumber;
}
public void setProductRatePlanChargeNumber(String productRatePlanChargeNumber) {
this.productRatePlanChargeNumber = productRatePlanChargeNumber;
}
public ExpandedProductRatePlanCharge ratingGroup(String ratingGroup) {
this.ratingGroup = ratingGroup;
return this;
}
/**
* Get ratingGroup
* @return ratingGroup
*/
@javax.annotation.Nullable
public String getRatingGroup() {
return ratingGroup;
}
public void setRatingGroup(String ratingGroup) {
this.ratingGroup = ratingGroup;
}
public ExpandedProductRatePlanCharge recognizedRevenueAccount(String recognizedRevenueAccount) {
this.recognizedRevenueAccount = recognizedRevenueAccount;
return this;
}
/**
* Get recognizedRevenueAccount
* @return recognizedRevenueAccount
*/
@javax.annotation.Nullable
public String getRecognizedRevenueAccount() {
return recognizedRevenueAccount;
}
public void setRecognizedRevenueAccount(String recognizedRevenueAccount) {
this.recognizedRevenueAccount = recognizedRevenueAccount;
}
public ExpandedProductRatePlanCharge revenueRecognitionRuleName(String revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
return this;
}
/**
* Get revenueRecognitionRuleName
* @return revenueRecognitionRuleName
*/
@javax.annotation.Nullable
public String getRevenueRecognitionRuleName() {
return revenueRecognitionRuleName;
}
public void setRevenueRecognitionRuleName(String revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
}
public ExpandedProductRatePlanCharge revRecTriggerCondition(String revRecTriggerCondition) {
this.revRecTriggerCondition = revRecTriggerCondition;
return this;
}
/**
* Get revRecTriggerCondition
* @return revRecTriggerCondition
*/
@javax.annotation.Nullable
public String getRevRecTriggerCondition() {
return revRecTriggerCondition;
}
public void setRevRecTriggerCondition(String revRecTriggerCondition) {
this.revRecTriggerCondition = revRecTriggerCondition;
}
public ExpandedProductRatePlanCharge rolloverApply(String rolloverApply) {
this.rolloverApply = rolloverApply;
return this;
}
/**
* Get rolloverApply
* @return rolloverApply
*/
@javax.annotation.Nullable
public String getRolloverApply() {
return rolloverApply;
}
public void setRolloverApply(String rolloverApply) {
this.rolloverApply = rolloverApply;
}
public ExpandedProductRatePlanCharge rolloverPeriods(Long rolloverPeriods) {
this.rolloverPeriods = rolloverPeriods;
return this;
}
/**
* Get rolloverPeriods
* @return rolloverPeriods
*/
@javax.annotation.Nullable
public Long getRolloverPeriods() {
return rolloverPeriods;
}
public void setRolloverPeriods(Long rolloverPeriods) {
this.rolloverPeriods = rolloverPeriods;
}
public ExpandedProductRatePlanCharge rolloverPeriodLength(Integer rolloverPeriodLength) {
this.rolloverPeriodLength = rolloverPeriodLength;
return this;
}
/**
* Get rolloverPeriodLength
* @return rolloverPeriodLength
*/
@javax.annotation.Nullable
public Integer getRolloverPeriodLength() {
return rolloverPeriodLength;
}
public void setRolloverPeriodLength(Integer rolloverPeriodLength) {
this.rolloverPeriodLength = rolloverPeriodLength;
}
public ExpandedProductRatePlanCharge smoothingModel(String smoothingModel) {
this.smoothingModel = smoothingModel;
return this;
}
/**
* Get smoothingModel
* @return smoothingModel
*/
@javax.annotation.Nullable
public String getSmoothingModel() {
return smoothingModel;
}
public void setSmoothingModel(String smoothingModel) {
this.smoothingModel = smoothingModel;
}
public ExpandedProductRatePlanCharge specificBillingPeriod(Long specificBillingPeriod) {
this.specificBillingPeriod = specificBillingPeriod;
return this;
}
/**
* Get specificBillingPeriod
* @return specificBillingPeriod
*/
@javax.annotation.Nullable
public Long getSpecificBillingPeriod() {
return specificBillingPeriod;
}
public void setSpecificBillingPeriod(Long specificBillingPeriod) {
this.specificBillingPeriod = specificBillingPeriod;
}
public ExpandedProductRatePlanCharge taxable(Boolean taxable) {
this.taxable = taxable;
return this;
}
/**
* Get taxable
* @return taxable
*/
@javax.annotation.Nullable
public Boolean getTaxable() {
return taxable;
}
public void setTaxable(Boolean taxable) {
this.taxable = taxable;
}
public ExpandedProductRatePlanCharge taxCode(String taxCode) {
this.taxCode = taxCode;
return this;
}
/**
* Get taxCode
* @return taxCode
*/
@javax.annotation.Nullable
public String getTaxCode() {
return taxCode;
}
public void setTaxCode(String taxCode) {
this.taxCode = taxCode;
}
public ExpandedProductRatePlanCharge taxMode(String taxMode) {
this.taxMode = taxMode;
return this;
}
/**
* Get taxMode
* @return taxMode
*/
@javax.annotation.Nullable
public String getTaxMode() {
return taxMode;
}
public void setTaxMode(String taxMode) {
this.taxMode = taxMode;
}
public ExpandedProductRatePlanCharge triggerEvent(String triggerEvent) {
this.triggerEvent = triggerEvent;
return this;
}
/**
* Get triggerEvent
* @return triggerEvent
*/
@javax.annotation.Nullable
public String getTriggerEvent() {
return triggerEvent;
}
public void setTriggerEvent(String triggerEvent) {
this.triggerEvent = triggerEvent;
}
public ExpandedProductRatePlanCharge upToPeriods(Long upToPeriods) {
this.upToPeriods = upToPeriods;
return this;
}
/**
* Get upToPeriods
* @return upToPeriods
*/
@javax.annotation.Nullable
public Long getUpToPeriods() {
return upToPeriods;
}
public void setUpToPeriods(Long upToPeriods) {
this.upToPeriods = upToPeriods;
}
public ExpandedProductRatePlanCharge upToPeriodsType(String upToPeriodsType) {
this.upToPeriodsType = upToPeriodsType;
return this;
}
/**
* Get upToPeriodsType
* @return upToPeriodsType
*/
@javax.annotation.Nullable
public String getUpToPeriodsType() {
return upToPeriodsType;
}
public void setUpToPeriodsType(String upToPeriodsType) {
this.upToPeriodsType = upToPeriodsType;
}
public ExpandedProductRatePlanCharge usageRecordRatingOption(String usageRecordRatingOption) {
this.usageRecordRatingOption = usageRecordRatingOption;
return this;
}
/**
* Get usageRecordRatingOption
* @return usageRecordRatingOption
*/
@javax.annotation.Nullable
public String getUsageRecordRatingOption() {
return usageRecordRatingOption;
}
public void setUsageRecordRatingOption(String usageRecordRatingOption) {
this.usageRecordRatingOption = usageRecordRatingOption;
}
public ExpandedProductRatePlanCharge useDiscountSpecificAccountingCode(Boolean useDiscountSpecificAccountingCode) {
this.useDiscountSpecificAccountingCode = useDiscountSpecificAccountingCode;
return this;
}
/**
* Get useDiscountSpecificAccountingCode
* @return useDiscountSpecificAccountingCode
*/
@javax.annotation.Nullable
public Boolean getUseDiscountSpecificAccountingCode() {
return useDiscountSpecificAccountingCode;
}
public void setUseDiscountSpecificAccountingCode(Boolean useDiscountSpecificAccountingCode) {
this.useDiscountSpecificAccountingCode = useDiscountSpecificAccountingCode;
}
public ExpandedProductRatePlanCharge useTenantDefaultForPriceChange(Boolean useTenantDefaultForPriceChange) {
this.useTenantDefaultForPriceChange = useTenantDefaultForPriceChange;
return this;
}
/**
* Get useTenantDefaultForPriceChange
* @return useTenantDefaultForPriceChange
*/
@javax.annotation.Nullable
public Boolean getUseTenantDefaultForPriceChange() {
return useTenantDefaultForPriceChange;
}
public void setUseTenantDefaultForPriceChange(Boolean useTenantDefaultForPriceChange) {
this.useTenantDefaultForPriceChange = useTenantDefaultForPriceChange;
}
public ExpandedProductRatePlanCharge validityPeriodType(String validityPeriodType) {
this.validityPeriodType = validityPeriodType;
return this;
}
/**
* Get validityPeriodType
* @return validityPeriodType
*/
@javax.annotation.Nullable
public String getValidityPeriodType() {
return validityPeriodType;
}
public void setValidityPeriodType(String validityPeriodType) {
this.validityPeriodType = validityPeriodType;
}
public ExpandedProductRatePlanCharge weeklyBillCycleDay(String weeklyBillCycleDay) {
this.weeklyBillCycleDay = weeklyBillCycleDay;
return this;
}
/**
* Get weeklyBillCycleDay
* @return weeklyBillCycleDay
*/
@javax.annotation.Nullable
public String getWeeklyBillCycleDay() {
return weeklyBillCycleDay;
}
public void setWeeklyBillCycleDay(String weeklyBillCycleDay) {
this.weeklyBillCycleDay = weeklyBillCycleDay;
}
public ExpandedProductRatePlanCharge priceUpsellQuantityStacked(Boolean priceUpsellQuantityStacked) {
this.priceUpsellQuantityStacked = priceUpsellQuantityStacked;
return this;
}
/**
* Get priceUpsellQuantityStacked
* @return priceUpsellQuantityStacked
*/
@javax.annotation.Nullable
public Boolean getPriceUpsellQuantityStacked() {
return priceUpsellQuantityStacked;
}
public void setPriceUpsellQuantityStacked(Boolean priceUpsellQuantityStacked) {
this.priceUpsellQuantityStacked = priceUpsellQuantityStacked;
}
public ExpandedProductRatePlanCharge deliveryScheduleId(String deliveryScheduleId) {
this.deliveryScheduleId = deliveryScheduleId;
return this;
}
/**
* Get deliveryScheduleId
* @return deliveryScheduleId
*/
@javax.annotation.Nullable
public String getDeliveryScheduleId() {
return deliveryScheduleId;
}
public void setDeliveryScheduleId(String deliveryScheduleId) {
this.deliveryScheduleId = deliveryScheduleId;
}
public ExpandedProductRatePlanCharge id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public ExpandedProductRatePlanCharge createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* Get createdById
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public ExpandedProductRatePlanCharge createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* Get createdDate
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public ExpandedProductRatePlanCharge updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* Get updatedById
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public ExpandedProductRatePlanCharge updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* Get updatedDate
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
public ExpandedProductRatePlanCharge commitmentType(String commitmentType) {
this.commitmentType = commitmentType;
return this;
}
/**
* Get commitmentType
* @return commitmentType
*/
@javax.annotation.Nullable
public String getCommitmentType() {
return commitmentType;
}
public void setCommitmentType(String commitmentType) {
this.commitmentType = commitmentType;
}
public ExpandedProductRatePlanCharge isCommitted(Boolean isCommitted) {
this.isCommitted = isCommitted;
return this;
}
/**
* Get isCommitted
* @return isCommitted
*/
@javax.annotation.Nullable
public Boolean getIsCommitted() {
return isCommitted;
}
public void setIsCommitted(Boolean isCommitted) {
this.isCommitted = isCommitted;
}
public ExpandedProductRatePlanCharge productRatePlanId(String productRatePlanId) {
this.productRatePlanId = productRatePlanId;
return this;
}
/**
* Get productRatePlanId
* @return productRatePlanId
*/
@javax.annotation.Nullable
public String getProductRatePlanId() {
return productRatePlanId;
}
public void setProductRatePlanId(String productRatePlanId) {
this.productRatePlanId = productRatePlanId;
}
public ExpandedProductRatePlanCharge isUnbilled(Boolean isUnbilled) {
this.isUnbilled = isUnbilled;
return this;
}
/**
* Get isUnbilled
* @return isUnbilled
*/
@javax.annotation.Nullable
public Boolean getIsUnbilled() {
return isUnbilled;
}
public void setIsUnbilled(Boolean isUnbilled) {
this.isUnbilled = isUnbilled;
}
public ExpandedProductRatePlanCharge isAllocationEligible(Boolean isAllocationEligible) {
this.isAllocationEligible = isAllocationEligible;
return this;
}
/**
* Get isAllocationEligible
* @return isAllocationEligible
*/
@javax.annotation.Nullable
public Boolean getIsAllocationEligible() {
return isAllocationEligible;
}
public void setIsAllocationEligible(Boolean isAllocationEligible) {
this.isAllocationEligible = isAllocationEligible;
}
public ExpandedProductRatePlanCharge productCategory(String productCategory) {
this.productCategory = productCategory;
return this;
}
/**
* Get productCategory
* @return productCategory
*/
@javax.annotation.Nullable
public String getProductCategory() {
return productCategory;
}
public void setProductCategory(String productCategory) {
this.productCategory = productCategory;
}
public ExpandedProductRatePlanCharge productClass(String productClass) {
this.productClass = productClass;
return this;
}
/**
* Get productClass
* @return productClass
*/
@javax.annotation.Nullable
public String getProductClass() {
return productClass;
}
public void setProductClass(String productClass) {
this.productClass = productClass;
}
public ExpandedProductRatePlanCharge productFamily(String productFamily) {
this.productFamily = productFamily;
return this;
}
/**
* Get productFamily
* @return productFamily
*/
@javax.annotation.Nullable
public String getProductFamily() {
return productFamily;
}
public void setProductFamily(String productFamily) {
this.productFamily = productFamily;
}
public ExpandedProductRatePlanCharge productLine(String productLine) {
this.productLine = productLine;
return this;
}
/**
* Get productLine
* @return productLine
*/
@javax.annotation.Nullable
public String getProductLine() {
return productLine;
}
public void setProductLine(String productLine) {
this.productLine = productLine;
}
public ExpandedProductRatePlanCharge revenueRecognitionTiming(String revenueRecognitionTiming) {
this.revenueRecognitionTiming = revenueRecognitionTiming;
return this;
}
/**
* Get revenueRecognitionTiming
* @return revenueRecognitionTiming
*/
@javax.annotation.Nullable
public String getRevenueRecognitionTiming() {
return revenueRecognitionTiming;
}
public void setRevenueRecognitionTiming(String revenueRecognitionTiming) {
this.revenueRecognitionTiming = revenueRecognitionTiming;
}
public ExpandedProductRatePlanCharge revenueAmortizationMethod(String revenueAmortizationMethod) {
this.revenueAmortizationMethod = revenueAmortizationMethod;
return this;
}
/**
* Get revenueAmortizationMethod
* @return revenueAmortizationMethod
*/
@javax.annotation.Nullable
public String getRevenueAmortizationMethod() {
return revenueAmortizationMethod;
}
public void setRevenueAmortizationMethod(String revenueAmortizationMethod) {
this.revenueAmortizationMethod = revenueAmortizationMethod;
}
public ExpandedProductRatePlanCharge applyToBillingPeriodPartially(Boolean applyToBillingPeriodPartially) {
this.applyToBillingPeriodPartially = applyToBillingPeriodPartially;
return this;
}
/**
* Get applyToBillingPeriodPartially
* @return applyToBillingPeriodPartially
*/
@javax.annotation.Nullable
public Boolean getApplyToBillingPeriodPartially() {
return applyToBillingPeriodPartially;
}
public void setApplyToBillingPeriodPartially(Boolean applyToBillingPeriodPartially) {
this.applyToBillingPeriodPartially = applyToBillingPeriodPartially;
}
public ExpandedProductRatePlanCharge accountReceivableAccountingCodeId(String accountReceivableAccountingCodeId) {
this.accountReceivableAccountingCodeId = accountReceivableAccountingCodeId;
return this;
}
/**
* Get accountReceivableAccountingCodeId
* @return accountReceivableAccountingCodeId
*/
@javax.annotation.Nullable
public String getAccountReceivableAccountingCodeId() {
return accountReceivableAccountingCodeId;
}
public void setAccountReceivableAccountingCodeId(String accountReceivableAccountingCodeId) {
this.accountReceivableAccountingCodeId = accountReceivableAccountingCodeId;
}
public ExpandedProductRatePlanCharge recognizedRevenueAccountingCodeId(String recognizedRevenueAccountingCodeId) {
this.recognizedRevenueAccountingCodeId = recognizedRevenueAccountingCodeId;
return this;
}
/**
* Get recognizedRevenueAccountingCodeId
* @return recognizedRevenueAccountingCodeId
*/
@javax.annotation.Nullable
public String getRecognizedRevenueAccountingCodeId() {
return recognizedRevenueAccountingCodeId;
}
public void setRecognizedRevenueAccountingCodeId(String recognizedRevenueAccountingCodeId) {
this.recognizedRevenueAccountingCodeId = recognizedRevenueAccountingCodeId;
}
public ExpandedProductRatePlanCharge deferredRevenueAccountingCodeId(String deferredRevenueAccountingCodeId) {
this.deferredRevenueAccountingCodeId = deferredRevenueAccountingCodeId;
return this;
}
/**
* Get deferredRevenueAccountingCodeId
* @return deferredRevenueAccountingCodeId
*/
@javax.annotation.Nullable
public String getDeferredRevenueAccountingCodeId() {
return deferredRevenueAccountingCodeId;
}
public void setDeferredRevenueAccountingCodeId(String deferredRevenueAccountingCodeId) {
this.deferredRevenueAccountingCodeId = deferredRevenueAccountingCodeId;
}
public ExpandedProductRatePlanCharge adjustmentLiabilityAccountingCodeId(String adjustmentLiabilityAccountingCodeId) {
this.adjustmentLiabilityAccountingCodeId = adjustmentLiabilityAccountingCodeId;
return this;
}
/**
* Get adjustmentLiabilityAccountingCodeId
* @return adjustmentLiabilityAccountingCodeId
*/
@javax.annotation.Nullable
public String getAdjustmentLiabilityAccountingCodeId() {
return adjustmentLiabilityAccountingCodeId;
}
public void setAdjustmentLiabilityAccountingCodeId(String adjustmentLiabilityAccountingCodeId) {
this.adjustmentLiabilityAccountingCodeId = adjustmentLiabilityAccountingCodeId;
}
public ExpandedProductRatePlanCharge adjustmentRevenueAccountingCodeId(String adjustmentRevenueAccountingCodeId) {
this.adjustmentRevenueAccountingCodeId = adjustmentRevenueAccountingCodeId;
return this;
}
/**
* Get adjustmentRevenueAccountingCodeId
* @return adjustmentRevenueAccountingCodeId
*/
@javax.annotation.Nullable
public String getAdjustmentRevenueAccountingCodeId() {
return adjustmentRevenueAccountingCodeId;
}
public void setAdjustmentRevenueAccountingCodeId(String adjustmentRevenueAccountingCodeId) {
this.adjustmentRevenueAccountingCodeId = adjustmentRevenueAccountingCodeId;
}
public ExpandedProductRatePlanCharge contractAssetAccountingCodeId(String contractAssetAccountingCodeId) {
this.contractAssetAccountingCodeId = contractAssetAccountingCodeId;
return this;
}
/**
* Get contractAssetAccountingCodeId
* @return contractAssetAccountingCodeId
*/
@javax.annotation.Nullable
public String getContractAssetAccountingCodeId() {
return contractAssetAccountingCodeId;
}
public void setContractAssetAccountingCodeId(String contractAssetAccountingCodeId) {
this.contractAssetAccountingCodeId = contractAssetAccountingCodeId;
}
public ExpandedProductRatePlanCharge contractLiabilityAccountingCodeId(String contractLiabilityAccountingCodeId) {
this.contractLiabilityAccountingCodeId = contractLiabilityAccountingCodeId;
return this;
}
/**
* Get contractLiabilityAccountingCodeId
* @return contractLiabilityAccountingCodeId
*/
@javax.annotation.Nullable
public String getContractLiabilityAccountingCodeId() {
return contractLiabilityAccountingCodeId;
}
public void setContractLiabilityAccountingCodeId(String contractLiabilityAccountingCodeId) {
this.contractLiabilityAccountingCodeId = contractLiabilityAccountingCodeId;
}
public ExpandedProductRatePlanCharge contractRecognizedRevenueAccountingCodeId(String contractRecognizedRevenueAccountingCodeId) {
this.contractRecognizedRevenueAccountingCodeId = contractRecognizedRevenueAccountingCodeId;
return this;
}
/**
* Get contractRecognizedRevenueAccountingCodeId
* @return contractRecognizedRevenueAccountingCodeId
*/
@javax.annotation.Nullable
public String getContractRecognizedRevenueAccountingCodeId() {
return contractRecognizedRevenueAccountingCodeId;
}
public void setContractRecognizedRevenueAccountingCodeId(String contractRecognizedRevenueAccountingCodeId) {
this.contractRecognizedRevenueAccountingCodeId = contractRecognizedRevenueAccountingCodeId;
}
public ExpandedProductRatePlanCharge unbilledReceivablesAccountingCodeId(String unbilledReceivablesAccountingCodeId) {
this.unbilledReceivablesAccountingCodeId = unbilledReceivablesAccountingCodeId;
return this;
}
/**
* Get unbilledReceivablesAccountingCodeId
* @return unbilledReceivablesAccountingCodeId
*/
@javax.annotation.Nullable
public String getUnbilledReceivablesAccountingCodeId() {
return unbilledReceivablesAccountingCodeId;
}
public void setUnbilledReceivablesAccountingCodeId(String unbilledReceivablesAccountingCodeId) {
this.unbilledReceivablesAccountingCodeId = unbilledReceivablesAccountingCodeId;
}
public ExpandedProductRatePlanCharge revRecCode(String revRecCode) {
this.revRecCode = revRecCode;
return this;
}
/**
* Get revRecCode
* @return revRecCode
*/
@javax.annotation.Nullable
public String getRevRecCode() {
return revRecCode;
}
public void setRevRecCode(String revRecCode) {
this.revRecCode = revRecCode;
}
public ExpandedProductRatePlanCharge uOM(String uOM) {
this.uOM = uOM;
return this;
}
/**
* Get uOM
* @return uOM
*/
@javax.annotation.Nullable
public String getuOM() {
return uOM;
}
public void setuOM(String uOM) {
this.uOM = uOM;
}
public ExpandedProductRatePlanCharge drawdownUom(String drawdownUom) {
this.drawdownUom = drawdownUom;
return this;
}
/**
* Get drawdownUom
* @return drawdownUom
*/
@javax.annotation.Nullable
public String getDrawdownUom() {
return drawdownUom;
}
public void setDrawdownUom(String drawdownUom) {
this.drawdownUom = drawdownUom;
}
public ExpandedProductRatePlanCharge prepaidUom(String prepaidUom) {
this.prepaidUom = prepaidUom;
return this;
}
/**
* Get prepaidUom
* @return prepaidUom
*/
@javax.annotation.Nullable
public String getPrepaidUom() {
return prepaidUom;
}
public void setPrepaidUom(String prepaidUom) {
this.prepaidUom = prepaidUom;
}
public ExpandedProductRatePlanCharge productRatePlan(ExpandedProductRatePlan productRatePlan) {
this.productRatePlan = productRatePlan;
return this;
}
/**
* Get productRatePlan
* @return productRatePlan
*/
@javax.annotation.Nullable
public ExpandedProductRatePlan getProductRatePlan() {
return productRatePlan;
}
public void setProductRatePlan(ExpandedProductRatePlan productRatePlan) {
this.productRatePlan = productRatePlan;
}
public ExpandedProductRatePlanCharge productRatePlanChargeTiers(List productRatePlanChargeTiers) {
this.productRatePlanChargeTiers = productRatePlanChargeTiers;
return this;
}
public ExpandedProductRatePlanCharge addProductRatePlanChargeTiersItem(ExpandedProductRatePlanChargeTier productRatePlanChargeTiersItem) {
if (this.productRatePlanChargeTiers == null) {
this.productRatePlanChargeTiers = new ArrayList<>();
}
this.productRatePlanChargeTiers.add(productRatePlanChargeTiersItem);
return this;
}
/**
* Get productRatePlanChargeTiers
* @return productRatePlanChargeTiers
*/
@javax.annotation.Nullable
public List getProductRatePlanChargeTiers() {
return productRatePlanChargeTiers;
}
public void setProductRatePlanChargeTiers(List productRatePlanChargeTiers) {
this.productRatePlanChargeTiers = productRatePlanChargeTiers;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the ExpandedProductRatePlanCharge instance itself
*/
public ExpandedProductRatePlanCharge putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ExpandedProductRatePlanCharge expandedProductRatePlanCharge = (ExpandedProductRatePlanCharge) o;
return Objects.equals(this.accountingCode, expandedProductRatePlanCharge.accountingCode) &&
Objects.equals(this.applyDiscountTo, expandedProductRatePlanCharge.applyDiscountTo) &&
Objects.equals(this.billCycleDay, expandedProductRatePlanCharge.billCycleDay) &&
Objects.equals(this.billCycleType, expandedProductRatePlanCharge.billCycleType) &&
Objects.equals(this.billingPeriod, expandedProductRatePlanCharge.billingPeriod) &&
Objects.equals(this.billingPeriodAlignment, expandedProductRatePlanCharge.billingPeriodAlignment) &&
Objects.equals(this.billingTiming, expandedProductRatePlanCharge.billingTiming) &&
Objects.equals(this.chargeFunction, expandedProductRatePlanCharge.chargeFunction) &&
Objects.equals(this.chargeModel, expandedProductRatePlanCharge.chargeModel) &&
Objects.equals(this.chargeType, expandedProductRatePlanCharge.chargeType) &&
Objects.equals(this.creditOption, expandedProductRatePlanCharge.creditOption) &&
Objects.equals(this.defaultQuantity, expandedProductRatePlanCharge.defaultQuantity) &&
Objects.equals(this.deferredRevenueAccount, expandedProductRatePlanCharge.deferredRevenueAccount) &&
Objects.equals(this.description, expandedProductRatePlanCharge.description) &&
Objects.equals(this.discountClassId, expandedProductRatePlanCharge.discountClassId) &&
Objects.equals(this.discountLevel, expandedProductRatePlanCharge.discountLevel) &&
Objects.equals(this.drawdownRate, expandedProductRatePlanCharge.drawdownRate) &&
Objects.equals(this.endDateCondition, expandedProductRatePlanCharge.endDateCondition) &&
Objects.equals(this.excludeItemBookingFromRevenueAccounting, expandedProductRatePlanCharge.excludeItemBookingFromRevenueAccounting) &&
Objects.equals(this.excludeItemBillingFromRevenueAccounting, expandedProductRatePlanCharge.excludeItemBillingFromRevenueAccounting) &&
Objects.equals(this.includedUnits, expandedProductRatePlanCharge.includedUnits) &&
Objects.equals(this.isPrepaid, expandedProductRatePlanCharge.isPrepaid) &&
Objects.equals(this.isRollover, expandedProductRatePlanCharge.isRollover) &&
Objects.equals(this.isStackedDiscount, expandedProductRatePlanCharge.isStackedDiscount) &&
Objects.equals(this.legacyRevenueReporting, expandedProductRatePlanCharge.legacyRevenueReporting) &&
Objects.equals(this.listPriceBase, expandedProductRatePlanCharge.listPriceBase) &&
Objects.equals(this.specificListPriceBase, expandedProductRatePlanCharge.specificListPriceBase) &&
Objects.equals(this.maxQuantity, expandedProductRatePlanCharge.maxQuantity) &&
Objects.equals(this.minQuantity, expandedProductRatePlanCharge.minQuantity) &&
Objects.equals(this.name, expandedProductRatePlanCharge.name) &&
Objects.equals(this.numberOfPeriod, expandedProductRatePlanCharge.numberOfPeriod) &&
Objects.equals(this.overageCalculationOption, expandedProductRatePlanCharge.overageCalculationOption) &&
Objects.equals(this.overageUnusedUnitsCreditOption, expandedProductRatePlanCharge.overageUnusedUnitsCreditOption) &&
Objects.equals(this.prepaidOperationType, expandedProductRatePlanCharge.prepaidOperationType) &&
Objects.equals(this.prorationOption, expandedProductRatePlanCharge.prorationOption) &&
Objects.equals(this.prepaidQuantity, expandedProductRatePlanCharge.prepaidQuantity) &&
Objects.equals(this.prepaidTotalQuantity, expandedProductRatePlanCharge.prepaidTotalQuantity) &&
Objects.equals(this.priceChangeOption, expandedProductRatePlanCharge.priceChangeOption) &&
Objects.equals(this.priceIncreasePercentage, expandedProductRatePlanCharge.priceIncreasePercentage) &&
Objects.equals(this.productRatePlanChargeNumber, expandedProductRatePlanCharge.productRatePlanChargeNumber) &&
Objects.equals(this.ratingGroup, expandedProductRatePlanCharge.ratingGroup) &&
Objects.equals(this.recognizedRevenueAccount, expandedProductRatePlanCharge.recognizedRevenueAccount) &&
Objects.equals(this.revenueRecognitionRuleName, expandedProductRatePlanCharge.revenueRecognitionRuleName) &&
Objects.equals(this.revRecTriggerCondition, expandedProductRatePlanCharge.revRecTriggerCondition) &&
Objects.equals(this.rolloverApply, expandedProductRatePlanCharge.rolloverApply) &&
Objects.equals(this.rolloverPeriods, expandedProductRatePlanCharge.rolloverPeriods) &&
Objects.equals(this.rolloverPeriodLength, expandedProductRatePlanCharge.rolloverPeriodLength) &&
Objects.equals(this.smoothingModel, expandedProductRatePlanCharge.smoothingModel) &&
Objects.equals(this.specificBillingPeriod, expandedProductRatePlanCharge.specificBillingPeriod) &&
Objects.equals(this.taxable, expandedProductRatePlanCharge.taxable) &&
Objects.equals(this.taxCode, expandedProductRatePlanCharge.taxCode) &&
Objects.equals(this.taxMode, expandedProductRatePlanCharge.taxMode) &&
Objects.equals(this.triggerEvent, expandedProductRatePlanCharge.triggerEvent) &&
Objects.equals(this.upToPeriods, expandedProductRatePlanCharge.upToPeriods) &&
Objects.equals(this.upToPeriodsType, expandedProductRatePlanCharge.upToPeriodsType) &&
Objects.equals(this.usageRecordRatingOption, expandedProductRatePlanCharge.usageRecordRatingOption) &&
Objects.equals(this.useDiscountSpecificAccountingCode, expandedProductRatePlanCharge.useDiscountSpecificAccountingCode) &&
Objects.equals(this.useTenantDefaultForPriceChange, expandedProductRatePlanCharge.useTenantDefaultForPriceChange) &&
Objects.equals(this.validityPeriodType, expandedProductRatePlanCharge.validityPeriodType) &&
Objects.equals(this.weeklyBillCycleDay, expandedProductRatePlanCharge.weeklyBillCycleDay) &&
Objects.equals(this.priceUpsellQuantityStacked, expandedProductRatePlanCharge.priceUpsellQuantityStacked) &&
Objects.equals(this.deliveryScheduleId, expandedProductRatePlanCharge.deliveryScheduleId) &&
Objects.equals(this.id, expandedProductRatePlanCharge.id) &&
Objects.equals(this.createdById, expandedProductRatePlanCharge.createdById) &&
Objects.equals(this.createdDate, expandedProductRatePlanCharge.createdDate) &&
Objects.equals(this.updatedById, expandedProductRatePlanCharge.updatedById) &&
Objects.equals(this.updatedDate, expandedProductRatePlanCharge.updatedDate) &&
Objects.equals(this.commitmentType, expandedProductRatePlanCharge.commitmentType) &&
Objects.equals(this.isCommitted, expandedProductRatePlanCharge.isCommitted) &&
Objects.equals(this.productRatePlanId, expandedProductRatePlanCharge.productRatePlanId) &&
Objects.equals(this.isUnbilled, expandedProductRatePlanCharge.isUnbilled) &&
Objects.equals(this.isAllocationEligible, expandedProductRatePlanCharge.isAllocationEligible) &&
Objects.equals(this.productCategory, expandedProductRatePlanCharge.productCategory) &&
Objects.equals(this.productClass, expandedProductRatePlanCharge.productClass) &&
Objects.equals(this.productFamily, expandedProductRatePlanCharge.productFamily) &&
Objects.equals(this.productLine, expandedProductRatePlanCharge.productLine) &&
Objects.equals(this.revenueRecognitionTiming, expandedProductRatePlanCharge.revenueRecognitionTiming) &&
Objects.equals(this.revenueAmortizationMethod, expandedProductRatePlanCharge.revenueAmortizationMethod) &&
Objects.equals(this.applyToBillingPeriodPartially, expandedProductRatePlanCharge.applyToBillingPeriodPartially) &&
Objects.equals(this.accountReceivableAccountingCodeId, expandedProductRatePlanCharge.accountReceivableAccountingCodeId) &&
Objects.equals(this.recognizedRevenueAccountingCodeId, expandedProductRatePlanCharge.recognizedRevenueAccountingCodeId) &&
Objects.equals(this.deferredRevenueAccountingCodeId, expandedProductRatePlanCharge.deferredRevenueAccountingCodeId) &&
Objects.equals(this.adjustmentLiabilityAccountingCodeId, expandedProductRatePlanCharge.adjustmentLiabilityAccountingCodeId) &&
Objects.equals(this.adjustmentRevenueAccountingCodeId, expandedProductRatePlanCharge.adjustmentRevenueAccountingCodeId) &&
Objects.equals(this.contractAssetAccountingCodeId, expandedProductRatePlanCharge.contractAssetAccountingCodeId) &&
Objects.equals(this.contractLiabilityAccountingCodeId, expandedProductRatePlanCharge.contractLiabilityAccountingCodeId) &&
Objects.equals(this.contractRecognizedRevenueAccountingCodeId, expandedProductRatePlanCharge.contractRecognizedRevenueAccountingCodeId) &&
Objects.equals(this.unbilledReceivablesAccountingCodeId, expandedProductRatePlanCharge.unbilledReceivablesAccountingCodeId) &&
Objects.equals(this.revRecCode, expandedProductRatePlanCharge.revRecCode) &&
Objects.equals(this.uOM, expandedProductRatePlanCharge.uOM) &&
Objects.equals(this.drawdownUom, expandedProductRatePlanCharge.drawdownUom) &&
Objects.equals(this.prepaidUom, expandedProductRatePlanCharge.prepaidUom) &&
Objects.equals(this.productRatePlan, expandedProductRatePlanCharge.productRatePlan) &&
Objects.equals(this.productRatePlanChargeTiers, expandedProductRatePlanCharge.productRatePlanChargeTiers)&&
Objects.equals(this.additionalProperties, expandedProductRatePlanCharge.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(accountingCode, applyDiscountTo, billCycleDay, billCycleType, billingPeriod, billingPeriodAlignment, billingTiming, chargeFunction, chargeModel, chargeType, creditOption, defaultQuantity, deferredRevenueAccount, description, discountClassId, discountLevel, drawdownRate, endDateCondition, excludeItemBookingFromRevenueAccounting, excludeItemBillingFromRevenueAccounting, includedUnits, isPrepaid, isRollover, isStackedDiscount, legacyRevenueReporting, listPriceBase, specificListPriceBase, maxQuantity, minQuantity, name, numberOfPeriod, overageCalculationOption, overageUnusedUnitsCreditOption, prepaidOperationType, prorationOption, prepaidQuantity, prepaidTotalQuantity, priceChangeOption, priceIncreasePercentage, productRatePlanChargeNumber, ratingGroup, recognizedRevenueAccount, revenueRecognitionRuleName, revRecTriggerCondition, rolloverApply, rolloverPeriods, rolloverPeriodLength, smoothingModel, specificBillingPeriod, taxable, taxCode, taxMode, triggerEvent, upToPeriods, upToPeriodsType, usageRecordRatingOption, useDiscountSpecificAccountingCode, useTenantDefaultForPriceChange, validityPeriodType, weeklyBillCycleDay, priceUpsellQuantityStacked, deliveryScheduleId, id, createdById, createdDate, updatedById, updatedDate, commitmentType, isCommitted, productRatePlanId, isUnbilled, isAllocationEligible, productCategory, productClass, productFamily, productLine, revenueRecognitionTiming, revenueAmortizationMethod, applyToBillingPeriodPartially, accountReceivableAccountingCodeId, recognizedRevenueAccountingCodeId, deferredRevenueAccountingCodeId, adjustmentLiabilityAccountingCodeId, adjustmentRevenueAccountingCodeId, contractAssetAccountingCodeId, contractLiabilityAccountingCodeId, contractRecognizedRevenueAccountingCodeId, unbilledReceivablesAccountingCodeId, revRecCode, uOM, drawdownUom, prepaidUom, productRatePlan, productRatePlanChargeTiers, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ExpandedProductRatePlanCharge {\n");
sb.append(" accountingCode: ").append(toIndentedString(accountingCode)).append("\n");
sb.append(" applyDiscountTo: ").append(toIndentedString(applyDiscountTo)).append("\n");
sb.append(" billCycleDay: ").append(toIndentedString(billCycleDay)).append("\n");
sb.append(" billCycleType: ").append(toIndentedString(billCycleType)).append("\n");
sb.append(" billingPeriod: ").append(toIndentedString(billingPeriod)).append("\n");
sb.append(" billingPeriodAlignment: ").append(toIndentedString(billingPeriodAlignment)).append("\n");
sb.append(" billingTiming: ").append(toIndentedString(billingTiming)).append("\n");
sb.append(" chargeFunction: ").append(toIndentedString(chargeFunction)).append("\n");
sb.append(" chargeModel: ").append(toIndentedString(chargeModel)).append("\n");
sb.append(" chargeType: ").append(toIndentedString(chargeType)).append("\n");
sb.append(" creditOption: ").append(toIndentedString(creditOption)).append("\n");
sb.append(" defaultQuantity: ").append(toIndentedString(defaultQuantity)).append("\n");
sb.append(" deferredRevenueAccount: ").append(toIndentedString(deferredRevenueAccount)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" discountClassId: ").append(toIndentedString(discountClassId)).append("\n");
sb.append(" discountLevel: ").append(toIndentedString(discountLevel)).append("\n");
sb.append(" drawdownRate: ").append(toIndentedString(drawdownRate)).append("\n");
sb.append(" endDateCondition: ").append(toIndentedString(endDateCondition)).append("\n");
sb.append(" excludeItemBookingFromRevenueAccounting: ").append(toIndentedString(excludeItemBookingFromRevenueAccounting)).append("\n");
sb.append(" excludeItemBillingFromRevenueAccounting: ").append(toIndentedString(excludeItemBillingFromRevenueAccounting)).append("\n");
sb.append(" includedUnits: ").append(toIndentedString(includedUnits)).append("\n");
sb.append(" isPrepaid: ").append(toIndentedString(isPrepaid)).append("\n");
sb.append(" isRollover: ").append(toIndentedString(isRollover)).append("\n");
sb.append(" isStackedDiscount: ").append(toIndentedString(isStackedDiscount)).append("\n");
sb.append(" legacyRevenueReporting: ").append(toIndentedString(legacyRevenueReporting)).append("\n");
sb.append(" listPriceBase: ").append(toIndentedString(listPriceBase)).append("\n");
sb.append(" specificListPriceBase: ").append(toIndentedString(specificListPriceBase)).append("\n");
sb.append(" maxQuantity: ").append(toIndentedString(maxQuantity)).append("\n");
sb.append(" minQuantity: ").append(toIndentedString(minQuantity)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" numberOfPeriod: ").append(toIndentedString(numberOfPeriod)).append("\n");
sb.append(" overageCalculationOption: ").append(toIndentedString(overageCalculationOption)).append("\n");
sb.append(" overageUnusedUnitsCreditOption: ").append(toIndentedString(overageUnusedUnitsCreditOption)).append("\n");
sb.append(" prepaidOperationType: ").append(toIndentedString(prepaidOperationType)).append("\n");
sb.append(" prorationOption: ").append(toIndentedString(prorationOption)).append("\n");
sb.append(" prepaidQuantity: ").append(toIndentedString(prepaidQuantity)).append("\n");
sb.append(" prepaidTotalQuantity: ").append(toIndentedString(prepaidTotalQuantity)).append("\n");
sb.append(" priceChangeOption: ").append(toIndentedString(priceChangeOption)).append("\n");
sb.append(" priceIncreasePercentage: ").append(toIndentedString(priceIncreasePercentage)).append("\n");
sb.append(" productRatePlanChargeNumber: ").append(toIndentedString(productRatePlanChargeNumber)).append("\n");
sb.append(" ratingGroup: ").append(toIndentedString(ratingGroup)).append("\n");
sb.append(" recognizedRevenueAccount: ").append(toIndentedString(recognizedRevenueAccount)).append("\n");
sb.append(" revenueRecognitionRuleName: ").append(toIndentedString(revenueRecognitionRuleName)).append("\n");
sb.append(" revRecTriggerCondition: ").append(toIndentedString(revRecTriggerCondition)).append("\n");
sb.append(" rolloverApply: ").append(toIndentedString(rolloverApply)).append("\n");
sb.append(" rolloverPeriods: ").append(toIndentedString(rolloverPeriods)).append("\n");
sb.append(" rolloverPeriodLength: ").append(toIndentedString(rolloverPeriodLength)).append("\n");
sb.append(" smoothingModel: ").append(toIndentedString(smoothingModel)).append("\n");
sb.append(" specificBillingPeriod: ").append(toIndentedString(specificBillingPeriod)).append("\n");
sb.append(" taxable: ").append(toIndentedString(taxable)).append("\n");
sb.append(" taxCode: ").append(toIndentedString(taxCode)).append("\n");
sb.append(" taxMode: ").append(toIndentedString(taxMode)).append("\n");
sb.append(" triggerEvent: ").append(toIndentedString(triggerEvent)).append("\n");
sb.append(" upToPeriods: ").append(toIndentedString(upToPeriods)).append("\n");
sb.append(" upToPeriodsType: ").append(toIndentedString(upToPeriodsType)).append("\n");
sb.append(" usageRecordRatingOption: ").append(toIndentedString(usageRecordRatingOption)).append("\n");
sb.append(" useDiscountSpecificAccountingCode: ").append(toIndentedString(useDiscountSpecificAccountingCode)).append("\n");
sb.append(" useTenantDefaultForPriceChange: ").append(toIndentedString(useTenantDefaultForPriceChange)).append("\n");
sb.append(" validityPeriodType: ").append(toIndentedString(validityPeriodType)).append("\n");
sb.append(" weeklyBillCycleDay: ").append(toIndentedString(weeklyBillCycleDay)).append("\n");
sb.append(" priceUpsellQuantityStacked: ").append(toIndentedString(priceUpsellQuantityStacked)).append("\n");
sb.append(" deliveryScheduleId: ").append(toIndentedString(deliveryScheduleId)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" commitmentType: ").append(toIndentedString(commitmentType)).append("\n");
sb.append(" isCommitted: ").append(toIndentedString(isCommitted)).append("\n");
sb.append(" productRatePlanId: ").append(toIndentedString(productRatePlanId)).append("\n");
sb.append(" isUnbilled: ").append(toIndentedString(isUnbilled)).append("\n");
sb.append(" isAllocationEligible: ").append(toIndentedString(isAllocationEligible)).append("\n");
sb.append(" productCategory: ").append(toIndentedString(productCategory)).append("\n");
sb.append(" productClass: ").append(toIndentedString(productClass)).append("\n");
sb.append(" productFamily: ").append(toIndentedString(productFamily)).append("\n");
sb.append(" productLine: ").append(toIndentedString(productLine)).append("\n");
sb.append(" revenueRecognitionTiming: ").append(toIndentedString(revenueRecognitionTiming)).append("\n");
sb.append(" revenueAmortizationMethod: ").append(toIndentedString(revenueAmortizationMethod)).append("\n");
sb.append(" applyToBillingPeriodPartially: ").append(toIndentedString(applyToBillingPeriodPartially)).append("\n");
sb.append(" accountReceivableAccountingCodeId: ").append(toIndentedString(accountReceivableAccountingCodeId)).append("\n");
sb.append(" recognizedRevenueAccountingCodeId: ").append(toIndentedString(recognizedRevenueAccountingCodeId)).append("\n");
sb.append(" deferredRevenueAccountingCodeId: ").append(toIndentedString(deferredRevenueAccountingCodeId)).append("\n");
sb.append(" adjustmentLiabilityAccountingCodeId: ").append(toIndentedString(adjustmentLiabilityAccountingCodeId)).append("\n");
sb.append(" adjustmentRevenueAccountingCodeId: ").append(toIndentedString(adjustmentRevenueAccountingCodeId)).append("\n");
sb.append(" contractAssetAccountingCodeId: ").append(toIndentedString(contractAssetAccountingCodeId)).append("\n");
sb.append(" contractLiabilityAccountingCodeId: ").append(toIndentedString(contractLiabilityAccountingCodeId)).append("\n");
sb.append(" contractRecognizedRevenueAccountingCodeId: ").append(toIndentedString(contractRecognizedRevenueAccountingCodeId)).append("\n");
sb.append(" unbilledReceivablesAccountingCodeId: ").append(toIndentedString(unbilledReceivablesAccountingCodeId)).append("\n");
sb.append(" revRecCode: ").append(toIndentedString(revRecCode)).append("\n");
sb.append(" uOM: ").append(toIndentedString(uOM)).append("\n");
sb.append(" drawdownUom: ").append(toIndentedString(drawdownUom)).append("\n");
sb.append(" prepaidUom: ").append(toIndentedString(prepaidUom)).append("\n");
sb.append(" productRatePlan: ").append(toIndentedString(productRatePlan)).append("\n");
sb.append(" productRatePlanChargeTiers: ").append(toIndentedString(productRatePlanChargeTiers)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("accountingCode");
openapiFields.add("applyDiscountTo");
openapiFields.add("billCycleDay");
openapiFields.add("billCycleType");
openapiFields.add("billingPeriod");
openapiFields.add("billingPeriodAlignment");
openapiFields.add("billingTiming");
openapiFields.add("chargeFunction");
openapiFields.add("chargeModel");
openapiFields.add("chargeType");
openapiFields.add("creditOption");
openapiFields.add("defaultQuantity");
openapiFields.add("deferredRevenueAccount");
openapiFields.add("description");
openapiFields.add("discountClassId");
openapiFields.add("discountLevel");
openapiFields.add("drawdownRate");
openapiFields.add("endDateCondition");
openapiFields.add("excludeItemBookingFromRevenueAccounting");
openapiFields.add("excludeItemBillingFromRevenueAccounting");
openapiFields.add("includedUnits");
openapiFields.add("isPrepaid");
openapiFields.add("isRollover");
openapiFields.add("isStackedDiscount");
openapiFields.add("legacyRevenueReporting");
openapiFields.add("listPriceBase");
openapiFields.add("specificListPriceBase");
openapiFields.add("maxQuantity");
openapiFields.add("minQuantity");
openapiFields.add("name");
openapiFields.add("numberOfPeriod");
openapiFields.add("overageCalculationOption");
openapiFields.add("overageUnusedUnitsCreditOption");
openapiFields.add("prepaidOperationType");
openapiFields.add("prorationOption");
openapiFields.add("prepaidQuantity");
openapiFields.add("prepaidTotalQuantity");
openapiFields.add("priceChangeOption");
openapiFields.add("priceIncreasePercentage");
openapiFields.add("productRatePlanChargeNumber");
openapiFields.add("ratingGroup");
openapiFields.add("recognizedRevenueAccount");
openapiFields.add("revenueRecognitionRuleName");
openapiFields.add("revRecTriggerCondition");
openapiFields.add("rolloverApply");
openapiFields.add("rolloverPeriods");
openapiFields.add("rolloverPeriodLength");
openapiFields.add("smoothingModel");
openapiFields.add("specificBillingPeriod");
openapiFields.add("taxable");
openapiFields.add("taxCode");
openapiFields.add("taxMode");
openapiFields.add("triggerEvent");
openapiFields.add("upToPeriods");
openapiFields.add("upToPeriodsType");
openapiFields.add("usageRecordRatingOption");
openapiFields.add("useDiscountSpecificAccountingCode");
openapiFields.add("useTenantDefaultForPriceChange");
openapiFields.add("validityPeriodType");
openapiFields.add("weeklyBillCycleDay");
openapiFields.add("priceUpsellQuantityStacked");
openapiFields.add("deliveryScheduleId");
openapiFields.add("id");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
openapiFields.add("commitmentType");
openapiFields.add("isCommitted");
openapiFields.add("productRatePlanId");
openapiFields.add("isUnbilled");
openapiFields.add("isAllocationEligible");
openapiFields.add("productCategory");
openapiFields.add("productClass");
openapiFields.add("productFamily");
openapiFields.add("productLine");
openapiFields.add("revenueRecognitionTiming");
openapiFields.add("revenueAmortizationMethod");
openapiFields.add("applyToBillingPeriodPartially");
openapiFields.add("accountReceivableAccountingCodeId");
openapiFields.add("recognizedRevenueAccountingCodeId");
openapiFields.add("deferredRevenueAccountingCodeId");
openapiFields.add("adjustmentLiabilityAccountingCodeId");
openapiFields.add("adjustmentRevenueAccountingCodeId");
openapiFields.add("contractAssetAccountingCodeId");
openapiFields.add("contractLiabilityAccountingCodeId");
openapiFields.add("contractRecognizedRevenueAccountingCodeId");
openapiFields.add("unbilledReceivablesAccountingCodeId");
openapiFields.add("revRecCode");
openapiFields.add("uOM");
openapiFields.add("drawdownUom");
openapiFields.add("prepaidUom");
openapiFields.add("productRatePlan");
openapiFields.add("productRatePlanChargeTiers");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to ExpandedProductRatePlanCharge
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!ExpandedProductRatePlanCharge.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in ExpandedProductRatePlanCharge is not found in the empty JSON string", ExpandedProductRatePlanCharge.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("accountingCode") != null && !jsonObj.get("accountingCode").isJsonNull()) && !jsonObj.get("accountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountingCode").toString()));
}
if ((jsonObj.get("applyDiscountTo") != null && !jsonObj.get("applyDiscountTo").isJsonNull()) && !jsonObj.get("applyDiscountTo").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `applyDiscountTo` to be a primitive type in the JSON string but got `%s`", jsonObj.get("applyDiscountTo").toString()));
}
if ((jsonObj.get("billCycleType") != null && !jsonObj.get("billCycleType").isJsonNull()) && !jsonObj.get("billCycleType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billCycleType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billCycleType").toString()));
}
if ((jsonObj.get("billingPeriod") != null && !jsonObj.get("billingPeriod").isJsonNull()) && !jsonObj.get("billingPeriod").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billingPeriod` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billingPeriod").toString()));
}
if ((jsonObj.get("billingPeriodAlignment") != null && !jsonObj.get("billingPeriodAlignment").isJsonNull()) && !jsonObj.get("billingPeriodAlignment").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billingPeriodAlignment` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billingPeriodAlignment").toString()));
}
if ((jsonObj.get("billingTiming") != null && !jsonObj.get("billingTiming").isJsonNull()) && !jsonObj.get("billingTiming").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billingTiming` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billingTiming").toString()));
}
if ((jsonObj.get("chargeFunction") != null && !jsonObj.get("chargeFunction").isJsonNull()) && !jsonObj.get("chargeFunction").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeFunction` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeFunction").toString()));
}
if ((jsonObj.get("chargeModel") != null && !jsonObj.get("chargeModel").isJsonNull()) && !jsonObj.get("chargeModel").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeModel` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeModel").toString()));
}
if ((jsonObj.get("chargeType") != null && !jsonObj.get("chargeType").isJsonNull()) && !jsonObj.get("chargeType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeType").toString()));
}
if ((jsonObj.get("creditOption") != null && !jsonObj.get("creditOption").isJsonNull()) && !jsonObj.get("creditOption").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creditOption` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creditOption").toString()));
}
if ((jsonObj.get("deferredRevenueAccount") != null && !jsonObj.get("deferredRevenueAccount").isJsonNull()) && !jsonObj.get("deferredRevenueAccount").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `deferredRevenueAccount` to be a primitive type in the JSON string but got `%s`", jsonObj.get("deferredRevenueAccount").toString()));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if ((jsonObj.get("discountClassId") != null && !jsonObj.get("discountClassId").isJsonNull()) && !jsonObj.get("discountClassId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `discountClassId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("discountClassId").toString()));
}
if ((jsonObj.get("discountLevel") != null && !jsonObj.get("discountLevel").isJsonNull()) && !jsonObj.get("discountLevel").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `discountLevel` to be a primitive type in the JSON string but got `%s`", jsonObj.get("discountLevel").toString()));
}
if ((jsonObj.get("endDateCondition") != null && !jsonObj.get("endDateCondition").isJsonNull()) && !jsonObj.get("endDateCondition").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `endDateCondition` to be a primitive type in the JSON string but got `%s`", jsonObj.get("endDateCondition").toString()));
}
if ((jsonObj.get("listPriceBase") != null && !jsonObj.get("listPriceBase").isJsonNull()) && !jsonObj.get("listPriceBase").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `listPriceBase` to be a primitive type in the JSON string but got `%s`", jsonObj.get("listPriceBase").toString()));
}
if ((jsonObj.get("name") != null && !jsonObj.get("name").isJsonNull()) && !jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if ((jsonObj.get("overageCalculationOption") != null && !jsonObj.get("overageCalculationOption").isJsonNull()) && !jsonObj.get("overageCalculationOption").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `overageCalculationOption` to be a primitive type in the JSON string but got `%s`", jsonObj.get("overageCalculationOption").toString()));
}
if ((jsonObj.get("overageUnusedUnitsCreditOption") != null && !jsonObj.get("overageUnusedUnitsCreditOption").isJsonNull()) && !jsonObj.get("overageUnusedUnitsCreditOption").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `overageUnusedUnitsCreditOption` to be a primitive type in the JSON string but got `%s`", jsonObj.get("overageUnusedUnitsCreditOption").toString()));
}
if ((jsonObj.get("prepaidOperationType") != null && !jsonObj.get("prepaidOperationType").isJsonNull()) && !jsonObj.get("prepaidOperationType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `prepaidOperationType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("prepaidOperationType").toString()));
}
if ((jsonObj.get("prorationOption") != null && !jsonObj.get("prorationOption").isJsonNull()) && !jsonObj.get("prorationOption").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `prorationOption` to be a primitive type in the JSON string but got `%s`", jsonObj.get("prorationOption").toString()));
}
if ((jsonObj.get("priceChangeOption") != null && !jsonObj.get("priceChangeOption").isJsonNull()) && !jsonObj.get("priceChangeOption").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `priceChangeOption` to be a primitive type in the JSON string but got `%s`", jsonObj.get("priceChangeOption").toString()));
}
if ((jsonObj.get("productRatePlanChargeNumber") != null && !jsonObj.get("productRatePlanChargeNumber").isJsonNull()) && !jsonObj.get("productRatePlanChargeNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanChargeNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productRatePlanChargeNumber").toString()));
}
if ((jsonObj.get("ratingGroup") != null && !jsonObj.get("ratingGroup").isJsonNull()) && !jsonObj.get("ratingGroup").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ratingGroup` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ratingGroup").toString()));
}
if ((jsonObj.get("recognizedRevenueAccount") != null && !jsonObj.get("recognizedRevenueAccount").isJsonNull()) && !jsonObj.get("recognizedRevenueAccount").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `recognizedRevenueAccount` to be a primitive type in the JSON string but got `%s`", jsonObj.get("recognizedRevenueAccount").toString()));
}
if ((jsonObj.get("revenueRecognitionRuleName") != null && !jsonObj.get("revenueRecognitionRuleName").isJsonNull()) && !jsonObj.get("revenueRecognitionRuleName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueRecognitionRuleName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueRecognitionRuleName").toString()));
}
if ((jsonObj.get("revRecTriggerCondition") != null && !jsonObj.get("revRecTriggerCondition").isJsonNull()) && !jsonObj.get("revRecTriggerCondition").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revRecTriggerCondition` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revRecTriggerCondition").toString()));
}
if ((jsonObj.get("rolloverApply") != null && !jsonObj.get("rolloverApply").isJsonNull()) && !jsonObj.get("rolloverApply").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `rolloverApply` to be a primitive type in the JSON string but got `%s`", jsonObj.get("rolloverApply").toString()));
}
if ((jsonObj.get("smoothingModel") != null && !jsonObj.get("smoothingModel").isJsonNull()) && !jsonObj.get("smoothingModel").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `smoothingModel` to be a primitive type in the JSON string but got `%s`", jsonObj.get("smoothingModel").toString()));
}
if ((jsonObj.get("taxCode") != null && !jsonObj.get("taxCode").isJsonNull()) && !jsonObj.get("taxCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxCode").toString()));
}
if ((jsonObj.get("taxMode") != null && !jsonObj.get("taxMode").isJsonNull()) && !jsonObj.get("taxMode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxMode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxMode").toString()));
}
if ((jsonObj.get("triggerEvent") != null && !jsonObj.get("triggerEvent").isJsonNull()) && !jsonObj.get("triggerEvent").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `triggerEvent` to be a primitive type in the JSON string but got `%s`", jsonObj.get("triggerEvent").toString()));
}
if ((jsonObj.get("upToPeriodsType") != null && !jsonObj.get("upToPeriodsType").isJsonNull()) && !jsonObj.get("upToPeriodsType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `upToPeriodsType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("upToPeriodsType").toString()));
}
if ((jsonObj.get("usageRecordRatingOption") != null && !jsonObj.get("usageRecordRatingOption").isJsonNull()) && !jsonObj.get("usageRecordRatingOption").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `usageRecordRatingOption` to be a primitive type in the JSON string but got `%s`", jsonObj.get("usageRecordRatingOption").toString()));
}
if ((jsonObj.get("validityPeriodType") != null && !jsonObj.get("validityPeriodType").isJsonNull()) && !jsonObj.get("validityPeriodType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `validityPeriodType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("validityPeriodType").toString()));
}
if ((jsonObj.get("weeklyBillCycleDay") != null && !jsonObj.get("weeklyBillCycleDay").isJsonNull()) && !jsonObj.get("weeklyBillCycleDay").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `weeklyBillCycleDay` to be a primitive type in the JSON string but got `%s`", jsonObj.get("weeklyBillCycleDay").toString()));
}
if ((jsonObj.get("deliveryScheduleId") != null && !jsonObj.get("deliveryScheduleId").isJsonNull()) && !jsonObj.get("deliveryScheduleId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `deliveryScheduleId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("deliveryScheduleId").toString()));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
if ((jsonObj.get("commitmentType") != null && !jsonObj.get("commitmentType").isJsonNull()) && !jsonObj.get("commitmentType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `commitmentType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("commitmentType").toString()));
}
if ((jsonObj.get("productRatePlanId") != null && !jsonObj.get("productRatePlanId").isJsonNull()) && !jsonObj.get("productRatePlanId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productRatePlanId").toString()));
}
if ((jsonObj.get("productCategory") != null && !jsonObj.get("productCategory").isJsonNull()) && !jsonObj.get("productCategory").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productCategory` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productCategory").toString()));
}
if ((jsonObj.get("productClass") != null && !jsonObj.get("productClass").isJsonNull()) && !jsonObj.get("productClass").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productClass` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productClass").toString()));
}
if ((jsonObj.get("productFamily") != null && !jsonObj.get("productFamily").isJsonNull()) && !jsonObj.get("productFamily").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productFamily` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productFamily").toString()));
}
if ((jsonObj.get("productLine") != null && !jsonObj.get("productLine").isJsonNull()) && !jsonObj.get("productLine").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productLine` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productLine").toString()));
}
if ((jsonObj.get("revenueRecognitionTiming") != null && !jsonObj.get("revenueRecognitionTiming").isJsonNull()) && !jsonObj.get("revenueRecognitionTiming").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueRecognitionTiming` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueRecognitionTiming").toString()));
}
if ((jsonObj.get("revenueAmortizationMethod") != null && !jsonObj.get("revenueAmortizationMethod").isJsonNull()) && !jsonObj.get("revenueAmortizationMethod").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueAmortizationMethod` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueAmortizationMethod").toString()));
}
if ((jsonObj.get("accountReceivableAccountingCodeId") != null && !jsonObj.get("accountReceivableAccountingCodeId").isJsonNull()) && !jsonObj.get("accountReceivableAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountReceivableAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountReceivableAccountingCodeId").toString()));
}
if ((jsonObj.get("recognizedRevenueAccountingCodeId") != null && !jsonObj.get("recognizedRevenueAccountingCodeId").isJsonNull()) && !jsonObj.get("recognizedRevenueAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `recognizedRevenueAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("recognizedRevenueAccountingCodeId").toString()));
}
if ((jsonObj.get("deferredRevenueAccountingCodeId") != null && !jsonObj.get("deferredRevenueAccountingCodeId").isJsonNull()) && !jsonObj.get("deferredRevenueAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `deferredRevenueAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("deferredRevenueAccountingCodeId").toString()));
}
if ((jsonObj.get("adjustmentLiabilityAccountingCodeId") != null && !jsonObj.get("adjustmentLiabilityAccountingCodeId").isJsonNull()) && !jsonObj.get("adjustmentLiabilityAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `adjustmentLiabilityAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("adjustmentLiabilityAccountingCodeId").toString()));
}
if ((jsonObj.get("adjustmentRevenueAccountingCodeId") != null && !jsonObj.get("adjustmentRevenueAccountingCodeId").isJsonNull()) && !jsonObj.get("adjustmentRevenueAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `adjustmentRevenueAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("adjustmentRevenueAccountingCodeId").toString()));
}
if ((jsonObj.get("contractAssetAccountingCodeId") != null && !jsonObj.get("contractAssetAccountingCodeId").isJsonNull()) && !jsonObj.get("contractAssetAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractAssetAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractAssetAccountingCodeId").toString()));
}
if ((jsonObj.get("contractLiabilityAccountingCodeId") != null && !jsonObj.get("contractLiabilityAccountingCodeId").isJsonNull()) && !jsonObj.get("contractLiabilityAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractLiabilityAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractLiabilityAccountingCodeId").toString()));
}
if ((jsonObj.get("contractRecognizedRevenueAccountingCodeId") != null && !jsonObj.get("contractRecognizedRevenueAccountingCodeId").isJsonNull()) && !jsonObj.get("contractRecognizedRevenueAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractRecognizedRevenueAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractRecognizedRevenueAccountingCodeId").toString()));
}
if ((jsonObj.get("unbilledReceivablesAccountingCodeId") != null && !jsonObj.get("unbilledReceivablesAccountingCodeId").isJsonNull()) && !jsonObj.get("unbilledReceivablesAccountingCodeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `unbilledReceivablesAccountingCodeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("unbilledReceivablesAccountingCodeId").toString()));
}
if ((jsonObj.get("revRecCode") != null && !jsonObj.get("revRecCode").isJsonNull()) && !jsonObj.get("revRecCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revRecCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revRecCode").toString()));
}
if ((jsonObj.get("uOM") != null && !jsonObj.get("uOM").isJsonNull()) && !jsonObj.get("uOM").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `uOM` to be a primitive type in the JSON string but got `%s`", jsonObj.get("uOM").toString()));
}
if ((jsonObj.get("drawdownUom") != null && !jsonObj.get("drawdownUom").isJsonNull()) && !jsonObj.get("drawdownUom").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `drawdownUom` to be a primitive type in the JSON string but got `%s`", jsonObj.get("drawdownUom").toString()));
}
if ((jsonObj.get("prepaidUom") != null && !jsonObj.get("prepaidUom").isJsonNull()) && !jsonObj.get("prepaidUom").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `prepaidUom` to be a primitive type in the JSON string but got `%s`", jsonObj.get("prepaidUom").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("productRatePlanChargeTiers") != null && !jsonObj.get("productRatePlanChargeTiers").isJsonNull() && !jsonObj.get("productRatePlanChargeTiers").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanChargeTiers` to be an array in the JSON string but got `%s`", jsonObj.get("productRatePlanChargeTiers").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!ExpandedProductRatePlanCharge.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'ExpandedProductRatePlanCharge' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(ExpandedProductRatePlanCharge.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, ExpandedProductRatePlanCharge value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public ExpandedProductRatePlanCharge read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
ExpandedProductRatePlanCharge instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of ExpandedProductRatePlanCharge given an JSON string
*
* @param jsonString JSON string
* @return An instance of ExpandedProductRatePlanCharge
* @throws IOException if the JSON string is invalid with respect to ExpandedProductRatePlanCharge
*/
public static ExpandedProductRatePlanCharge fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, ExpandedProductRatePlanCharge.class);
}
/**
* Convert an instance of ExpandedProductRatePlanCharge to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy