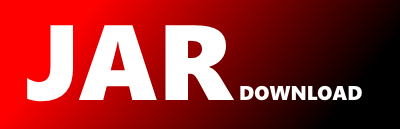
com.zuora.model.ExpandedSubscription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.ExpandedAccount;
import com.zuora.model.ExpandedContact;
import com.zuora.model.ExpandedInvoiceItem;
import com.zuora.model.ExpandedOrders;
import com.zuora.model.ExpandedRatePlan;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* ExpandedSubscription
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class ExpandedSubscription {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "accountId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_AUTO_RENEW = "autoRenew";
@SerializedName(SERIALIZED_NAME_AUTO_RENEW)
private Boolean autoRenew;
public static final String SERIALIZED_NAME_CANCELLED_DATE = "cancelledDate";
@SerializedName(SERIALIZED_NAME_CANCELLED_DATE)
private LocalDate cancelledDate;
public static final String SERIALIZED_NAME_CONTRACT_ACCEPTANCE_DATE = "contractAcceptanceDate";
@SerializedName(SERIALIZED_NAME_CONTRACT_ACCEPTANCE_DATE)
private LocalDate contractAcceptanceDate;
public static final String SERIALIZED_NAME_CONTRACT_EFFECTIVE_DATE = "contractEffectiveDate";
@SerializedName(SERIALIZED_NAME_CONTRACT_EFFECTIVE_DATE)
private LocalDate contractEffectiveDate;
public static final String SERIALIZED_NAME_CREATOR_ACCOUNT_ID = "creatorAccountId";
@SerializedName(SERIALIZED_NAME_CREATOR_ACCOUNT_ID)
private String creatorAccountId;
public static final String SERIALIZED_NAME_CREATOR_INVOICE_OWNER_ID = "creatorInvoiceOwnerId";
@SerializedName(SERIALIZED_NAME_CREATOR_INVOICE_OWNER_ID)
private String creatorInvoiceOwnerId;
public static final String SERIALIZED_NAME_CURRENT_TERM = "currentTerm";
@SerializedName(SERIALIZED_NAME_CURRENT_TERM)
private Long currentTerm;
public static final String SERIALIZED_NAME_CURRENT_TERM_PERIOD_TYPE = "currentTermPeriodType";
@SerializedName(SERIALIZED_NAME_CURRENT_TERM_PERIOD_TYPE)
private String currentTermPeriodType;
public static final String SERIALIZED_NAME_INITIAL_TERM = "initialTerm";
@SerializedName(SERIALIZED_NAME_INITIAL_TERM)
private Long initialTerm;
public static final String SERIALIZED_NAME_INITIAL_TERM_PERIOD_TYPE = "initialTermPeriodType";
@SerializedName(SERIALIZED_NAME_INITIAL_TERM_PERIOD_TYPE)
private String initialTermPeriodType;
public static final String SERIALIZED_NAME_INVOICE_GROUP_NUMBER = "invoiceGroupNumber";
@SerializedName(SERIALIZED_NAME_INVOICE_GROUP_NUMBER)
private String invoiceGroupNumber;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ID = "invoiceOwnerId";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ID)
private String invoiceOwnerId;
public static final String SERIALIZED_NAME_IS_INVOICE_SEPARATE = "isInvoiceSeparate";
@SerializedName(SERIALIZED_NAME_IS_INVOICE_SEPARATE)
private Boolean isInvoiceSeparate;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_NOTES = "notes";
@SerializedName(SERIALIZED_NAME_NOTES)
private String notes;
public static final String SERIALIZED_NAME_ORIGINAL_CREATED_DATE = "originalCreatedDate";
@SerializedName(SERIALIZED_NAME_ORIGINAL_CREATED_DATE)
private String originalCreatedDate;
public static final String SERIALIZED_NAME_ORIGINAL_ID = "originalId";
@SerializedName(SERIALIZED_NAME_ORIGINAL_ID)
private String originalId;
public static final String SERIALIZED_NAME_PREVIOUS_SUBSCRIPTION_ID = "previousSubscriptionId";
@SerializedName(SERIALIZED_NAME_PREVIOUS_SUBSCRIPTION_ID)
private String previousSubscriptionId;
public static final String SERIALIZED_NAME_RENEWAL_SETTING = "renewalSetting";
@SerializedName(SERIALIZED_NAME_RENEWAL_SETTING)
private String renewalSetting;
public static final String SERIALIZED_NAME_RENEWAL_TERM = "renewalTerm";
@SerializedName(SERIALIZED_NAME_RENEWAL_TERM)
private Long renewalTerm;
public static final String SERIALIZED_NAME_RENEWAL_TERM_PERIOD_TYPE = "renewalTermPeriodType";
@SerializedName(SERIALIZED_NAME_RENEWAL_TERM_PERIOD_TYPE)
private String renewalTermPeriodType;
public static final String SERIALIZED_NAME_REVISION = "revision";
@SerializedName(SERIALIZED_NAME_REVISION)
private String revision;
public static final String SERIALIZED_NAME_SERVICE_ACTIVATION_DATE = "serviceActivationDate";
@SerializedName(SERIALIZED_NAME_SERVICE_ACTIVATION_DATE)
private LocalDate serviceActivationDate;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private String status;
public static final String SERIALIZED_NAME_IS_LATEST_VERSION = "isLatestVersion";
@SerializedName(SERIALIZED_NAME_IS_LATEST_VERSION)
private Boolean isLatestVersion;
public static final String SERIALIZED_NAME_SUBSCRIPTION_END_DATE = "subscriptionEndDate";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_END_DATE)
private LocalDate subscriptionEndDate;
public static final String SERIALIZED_NAME_SUBSCRIPTION_START_DATE = "subscriptionStartDate";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_START_DATE)
private LocalDate subscriptionStartDate;
public static final String SERIALIZED_NAME_SUBSCRIPTION_VERSION_AMENDMENT_ID = "subscriptionVersionAmendmentId";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_VERSION_AMENDMENT_ID)
private String subscriptionVersionAmendmentId;
public static final String SERIALIZED_NAME_TERM_END_DATE = "termEndDate";
@SerializedName(SERIALIZED_NAME_TERM_END_DATE)
private LocalDate termEndDate;
public static final String SERIALIZED_NAME_TERM_START_DATE = "termStartDate";
@SerializedName(SERIALIZED_NAME_TERM_START_DATE)
private LocalDate termStartDate;
public static final String SERIALIZED_NAME_TERM_TYPE = "termType";
@SerializedName(SERIALIZED_NAME_TERM_TYPE)
private String termType;
public static final String SERIALIZED_NAME_VERSION = "version";
@SerializedName(SERIALIZED_NAME_VERSION)
private Long version;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public static final String SERIALIZED_NAME_C_M_R_R = "cMRR";
@SerializedName(SERIALIZED_NAME_C_M_R_R)
private BigDecimal cMRR;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT_SNAPSHOT_ID = "billToContactSnapshotId";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT_SNAPSHOT_ID)
private String billToContactSnapshotId;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT_ID = "billToContactId";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT_ID)
private String billToContactId;
public static final String SERIALIZED_NAME_INVOICE_TEMPLATE_ID = "invoiceTemplateId";
@SerializedName(SERIALIZED_NAME_INVOICE_TEMPLATE_ID)
private String invoiceTemplateId;
public static final String SERIALIZED_NAME_SEQUENCE_SET_ID = "sequenceSetId";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_ID)
private String sequenceSetId;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT_ID = "soldToContactId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT_ID)
private String soldToContactId;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT_SNAPSHOT_ID = "soldToContactSnapshotId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT_SNAPSHOT_ID)
private String soldToContactSnapshotId;
public static final String SERIALIZED_NAME_EXTERNALLY_MANAGED_BY = "externallyManagedBy";
@SerializedName(SERIALIZED_NAME_EXTERNALLY_MANAGED_BY)
private String externallyManagedBy;
public static final String SERIALIZED_NAME_LAST_BOOKING_DATE = "lastBookingDate";
@SerializedName(SERIALIZED_NAME_LAST_BOOKING_DATE)
private LocalDate lastBookingDate;
public static final String SERIALIZED_NAME_INVOICE_SCHEDULE_ID = "invoiceScheduleId";
@SerializedName(SERIALIZED_NAME_INVOICE_SCHEDULE_ID)
private String invoiceScheduleId;
public static final String SERIALIZED_NAME_CANCEL_REASON = "cancelReason";
@SerializedName(SERIALIZED_NAME_CANCEL_REASON)
private String cancelReason;
public static final String SERIALIZED_NAME_PREPAYMENT = "prepayment";
@SerializedName(SERIALIZED_NAME_PREPAYMENT)
private Boolean prepayment;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_IS_SINGLE_VERSIONED = "isSingleVersioned";
@SerializedName(SERIALIZED_NAME_IS_SINGLE_VERSIONED)
private Boolean isSingleVersioned;
public static final String SERIALIZED_NAME_ORDER_ID = "orderId";
@SerializedName(SERIALIZED_NAME_ORDER_ID)
private String orderId;
public static final String SERIALIZED_NAME_RAMP_ID = "rampId";
@SerializedName(SERIALIZED_NAME_RAMP_ID)
private String rampId;
public static final String SERIALIZED_NAME_PAYMENT_TERM = "paymentTerm";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERM)
private String paymentTerm;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "paymentMethodId";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY_ID = "paymentGatewayId";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY_ID)
private String paymentGatewayId;
public static final String SERIALIZED_NAME_QUOTE_NUMBER_Q_T = "quoteNumber__QT";
@SerializedName(SERIALIZED_NAME_QUOTE_NUMBER_Q_T)
private String quoteNumberQT;
public static final String SERIALIZED_NAME_QUOTE_TYPE_Q_T = "quoteType__QT";
@SerializedName(SERIALIZED_NAME_QUOTE_TYPE_Q_T)
private String quoteTypeQT;
public static final String SERIALIZED_NAME_QUOTE_BUSINESS_TYPE_Q_T = "quoteBusinessType__QT";
@SerializedName(SERIALIZED_NAME_QUOTE_BUSINESS_TYPE_Q_T)
private String quoteBusinessTypeQT;
public static final String SERIALIZED_NAME_OPPORTUNITY_NAME_Q_T = "opportunityName__QT";
@SerializedName(SERIALIZED_NAME_OPPORTUNITY_NAME_Q_T)
private String opportunityNameQT;
public static final String SERIALIZED_NAME_OPPORTUNITY_CLOSE_DATE_Q_T = "opportunityCloseDate__QT";
@SerializedName(SERIALIZED_NAME_OPPORTUNITY_CLOSE_DATE_Q_T)
private LocalDate opportunityCloseDateQT;
public static final String SERIALIZED_NAME_CPQ_BUNDLE_JSON_ID_Q_T = "cpqBundleJsonId__QT";
@SerializedName(SERIALIZED_NAME_CPQ_BUNDLE_JSON_ID_Q_T)
private String cpqBundleJsonIdQT;
public static final String SERIALIZED_NAME_ACCOUNT = "account";
@SerializedName(SERIALIZED_NAME_ACCOUNT)
private ExpandedAccount account;
public static final String SERIALIZED_NAME_INVOICE_OWNER = "invoiceOwner";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER)
private ExpandedAccount invoiceOwner;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT = "billToContact";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT)
private ExpandedContact billToContact;
public static final String SERIALIZED_NAME_INVOICE_ITEMS = "invoiceItems";
@SerializedName(SERIALIZED_NAME_INVOICE_ITEMS)
private List invoiceItems;
public static final String SERIALIZED_NAME_RATE_PLANS = "ratePlans";
@SerializedName(SERIALIZED_NAME_RATE_PLANS)
private List ratePlans;
public static final String SERIALIZED_NAME_ORDER = "order";
@SerializedName(SERIALIZED_NAME_ORDER)
private ExpandedOrders order;
public ExpandedSubscription() {
}
public ExpandedSubscription id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public ExpandedSubscription accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Get accountId
* @return accountId
*/
@javax.annotation.Nullable
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public ExpandedSubscription autoRenew(Boolean autoRenew) {
this.autoRenew = autoRenew;
return this;
}
/**
* Get autoRenew
* @return autoRenew
*/
@javax.annotation.Nullable
public Boolean getAutoRenew() {
return autoRenew;
}
public void setAutoRenew(Boolean autoRenew) {
this.autoRenew = autoRenew;
}
public ExpandedSubscription cancelledDate(LocalDate cancelledDate) {
this.cancelledDate = cancelledDate;
return this;
}
/**
* Get cancelledDate
* @return cancelledDate
*/
@javax.annotation.Nullable
public LocalDate getCancelledDate() {
return cancelledDate;
}
public void setCancelledDate(LocalDate cancelledDate) {
this.cancelledDate = cancelledDate;
}
public ExpandedSubscription contractAcceptanceDate(LocalDate contractAcceptanceDate) {
this.contractAcceptanceDate = contractAcceptanceDate;
return this;
}
/**
* Get contractAcceptanceDate
* @return contractAcceptanceDate
*/
@javax.annotation.Nullable
public LocalDate getContractAcceptanceDate() {
return contractAcceptanceDate;
}
public void setContractAcceptanceDate(LocalDate contractAcceptanceDate) {
this.contractAcceptanceDate = contractAcceptanceDate;
}
public ExpandedSubscription contractEffectiveDate(LocalDate contractEffectiveDate) {
this.contractEffectiveDate = contractEffectiveDate;
return this;
}
/**
* Get contractEffectiveDate
* @return contractEffectiveDate
*/
@javax.annotation.Nullable
public LocalDate getContractEffectiveDate() {
return contractEffectiveDate;
}
public void setContractEffectiveDate(LocalDate contractEffectiveDate) {
this.contractEffectiveDate = contractEffectiveDate;
}
public ExpandedSubscription creatorAccountId(String creatorAccountId) {
this.creatorAccountId = creatorAccountId;
return this;
}
/**
* Get creatorAccountId
* @return creatorAccountId
*/
@javax.annotation.Nullable
public String getCreatorAccountId() {
return creatorAccountId;
}
public void setCreatorAccountId(String creatorAccountId) {
this.creatorAccountId = creatorAccountId;
}
public ExpandedSubscription creatorInvoiceOwnerId(String creatorInvoiceOwnerId) {
this.creatorInvoiceOwnerId = creatorInvoiceOwnerId;
return this;
}
/**
* Get creatorInvoiceOwnerId
* @return creatorInvoiceOwnerId
*/
@javax.annotation.Nullable
public String getCreatorInvoiceOwnerId() {
return creatorInvoiceOwnerId;
}
public void setCreatorInvoiceOwnerId(String creatorInvoiceOwnerId) {
this.creatorInvoiceOwnerId = creatorInvoiceOwnerId;
}
public ExpandedSubscription currentTerm(Long currentTerm) {
this.currentTerm = currentTerm;
return this;
}
/**
* Get currentTerm
* @return currentTerm
*/
@javax.annotation.Nullable
public Long getCurrentTerm() {
return currentTerm;
}
public void setCurrentTerm(Long currentTerm) {
this.currentTerm = currentTerm;
}
public ExpandedSubscription currentTermPeriodType(String currentTermPeriodType) {
this.currentTermPeriodType = currentTermPeriodType;
return this;
}
/**
* Get currentTermPeriodType
* @return currentTermPeriodType
*/
@javax.annotation.Nullable
public String getCurrentTermPeriodType() {
return currentTermPeriodType;
}
public void setCurrentTermPeriodType(String currentTermPeriodType) {
this.currentTermPeriodType = currentTermPeriodType;
}
public ExpandedSubscription initialTerm(Long initialTerm) {
this.initialTerm = initialTerm;
return this;
}
/**
* Get initialTerm
* @return initialTerm
*/
@javax.annotation.Nullable
public Long getInitialTerm() {
return initialTerm;
}
public void setInitialTerm(Long initialTerm) {
this.initialTerm = initialTerm;
}
public ExpandedSubscription initialTermPeriodType(String initialTermPeriodType) {
this.initialTermPeriodType = initialTermPeriodType;
return this;
}
/**
* Get initialTermPeriodType
* @return initialTermPeriodType
*/
@javax.annotation.Nullable
public String getInitialTermPeriodType() {
return initialTermPeriodType;
}
public void setInitialTermPeriodType(String initialTermPeriodType) {
this.initialTermPeriodType = initialTermPeriodType;
}
public ExpandedSubscription invoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
return this;
}
/**
* Get invoiceGroupNumber
* @return invoiceGroupNumber
*/
@javax.annotation.Nullable
public String getInvoiceGroupNumber() {
return invoiceGroupNumber;
}
public void setInvoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
}
public ExpandedSubscription invoiceOwnerId(String invoiceOwnerId) {
this.invoiceOwnerId = invoiceOwnerId;
return this;
}
/**
* Get invoiceOwnerId
* @return invoiceOwnerId
*/
@javax.annotation.Nullable
public String getInvoiceOwnerId() {
return invoiceOwnerId;
}
public void setInvoiceOwnerId(String invoiceOwnerId) {
this.invoiceOwnerId = invoiceOwnerId;
}
public ExpandedSubscription isInvoiceSeparate(Boolean isInvoiceSeparate) {
this.isInvoiceSeparate = isInvoiceSeparate;
return this;
}
/**
* Get isInvoiceSeparate
* @return isInvoiceSeparate
*/
@javax.annotation.Nullable
public Boolean getIsInvoiceSeparate() {
return isInvoiceSeparate;
}
public void setIsInvoiceSeparate(Boolean isInvoiceSeparate) {
this.isInvoiceSeparate = isInvoiceSeparate;
}
public ExpandedSubscription name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
*/
@javax.annotation.Nullable
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public ExpandedSubscription notes(String notes) {
this.notes = notes;
return this;
}
/**
* Get notes
* @return notes
*/
@javax.annotation.Nullable
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
this.notes = notes;
}
public ExpandedSubscription originalCreatedDate(String originalCreatedDate) {
this.originalCreatedDate = originalCreatedDate;
return this;
}
/**
* Get originalCreatedDate
* @return originalCreatedDate
*/
@javax.annotation.Nullable
public String getOriginalCreatedDate() {
return originalCreatedDate;
}
public void setOriginalCreatedDate(String originalCreatedDate) {
this.originalCreatedDate = originalCreatedDate;
}
public ExpandedSubscription originalId(String originalId) {
this.originalId = originalId;
return this;
}
/**
* Get originalId
* @return originalId
*/
@javax.annotation.Nullable
public String getOriginalId() {
return originalId;
}
public void setOriginalId(String originalId) {
this.originalId = originalId;
}
public ExpandedSubscription previousSubscriptionId(String previousSubscriptionId) {
this.previousSubscriptionId = previousSubscriptionId;
return this;
}
/**
* Get previousSubscriptionId
* @return previousSubscriptionId
*/
@javax.annotation.Nullable
public String getPreviousSubscriptionId() {
return previousSubscriptionId;
}
public void setPreviousSubscriptionId(String previousSubscriptionId) {
this.previousSubscriptionId = previousSubscriptionId;
}
public ExpandedSubscription renewalSetting(String renewalSetting) {
this.renewalSetting = renewalSetting;
return this;
}
/**
* Get renewalSetting
* @return renewalSetting
*/
@javax.annotation.Nullable
public String getRenewalSetting() {
return renewalSetting;
}
public void setRenewalSetting(String renewalSetting) {
this.renewalSetting = renewalSetting;
}
public ExpandedSubscription renewalTerm(Long renewalTerm) {
this.renewalTerm = renewalTerm;
return this;
}
/**
* Get renewalTerm
* @return renewalTerm
*/
@javax.annotation.Nullable
public Long getRenewalTerm() {
return renewalTerm;
}
public void setRenewalTerm(Long renewalTerm) {
this.renewalTerm = renewalTerm;
}
public ExpandedSubscription renewalTermPeriodType(String renewalTermPeriodType) {
this.renewalTermPeriodType = renewalTermPeriodType;
return this;
}
/**
* Get renewalTermPeriodType
* @return renewalTermPeriodType
*/
@javax.annotation.Nullable
public String getRenewalTermPeriodType() {
return renewalTermPeriodType;
}
public void setRenewalTermPeriodType(String renewalTermPeriodType) {
this.renewalTermPeriodType = renewalTermPeriodType;
}
public ExpandedSubscription revision(String revision) {
this.revision = revision;
return this;
}
/**
* Get revision
* @return revision
*/
@javax.annotation.Nullable
public String getRevision() {
return revision;
}
public void setRevision(String revision) {
this.revision = revision;
}
public ExpandedSubscription serviceActivationDate(LocalDate serviceActivationDate) {
this.serviceActivationDate = serviceActivationDate;
return this;
}
/**
* Get serviceActivationDate
* @return serviceActivationDate
*/
@javax.annotation.Nullable
public LocalDate getServiceActivationDate() {
return serviceActivationDate;
}
public void setServiceActivationDate(LocalDate serviceActivationDate) {
this.serviceActivationDate = serviceActivationDate;
}
public ExpandedSubscription status(String status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public ExpandedSubscription isLatestVersion(Boolean isLatestVersion) {
this.isLatestVersion = isLatestVersion;
return this;
}
/**
* Get isLatestVersion
* @return isLatestVersion
*/
@javax.annotation.Nullable
public Boolean getIsLatestVersion() {
return isLatestVersion;
}
public void setIsLatestVersion(Boolean isLatestVersion) {
this.isLatestVersion = isLatestVersion;
}
public ExpandedSubscription subscriptionEndDate(LocalDate subscriptionEndDate) {
this.subscriptionEndDate = subscriptionEndDate;
return this;
}
/**
* Get subscriptionEndDate
* @return subscriptionEndDate
*/
@javax.annotation.Nullable
public LocalDate getSubscriptionEndDate() {
return subscriptionEndDate;
}
public void setSubscriptionEndDate(LocalDate subscriptionEndDate) {
this.subscriptionEndDate = subscriptionEndDate;
}
public ExpandedSubscription subscriptionStartDate(LocalDate subscriptionStartDate) {
this.subscriptionStartDate = subscriptionStartDate;
return this;
}
/**
* Get subscriptionStartDate
* @return subscriptionStartDate
*/
@javax.annotation.Nullable
public LocalDate getSubscriptionStartDate() {
return subscriptionStartDate;
}
public void setSubscriptionStartDate(LocalDate subscriptionStartDate) {
this.subscriptionStartDate = subscriptionStartDate;
}
public ExpandedSubscription subscriptionVersionAmendmentId(String subscriptionVersionAmendmentId) {
this.subscriptionVersionAmendmentId = subscriptionVersionAmendmentId;
return this;
}
/**
* Get subscriptionVersionAmendmentId
* @return subscriptionVersionAmendmentId
*/
@javax.annotation.Nullable
public String getSubscriptionVersionAmendmentId() {
return subscriptionVersionAmendmentId;
}
public void setSubscriptionVersionAmendmentId(String subscriptionVersionAmendmentId) {
this.subscriptionVersionAmendmentId = subscriptionVersionAmendmentId;
}
public ExpandedSubscription termEndDate(LocalDate termEndDate) {
this.termEndDate = termEndDate;
return this;
}
/**
* Get termEndDate
* @return termEndDate
*/
@javax.annotation.Nullable
public LocalDate getTermEndDate() {
return termEndDate;
}
public void setTermEndDate(LocalDate termEndDate) {
this.termEndDate = termEndDate;
}
public ExpandedSubscription termStartDate(LocalDate termStartDate) {
this.termStartDate = termStartDate;
return this;
}
/**
* Get termStartDate
* @return termStartDate
*/
@javax.annotation.Nullable
public LocalDate getTermStartDate() {
return termStartDate;
}
public void setTermStartDate(LocalDate termStartDate) {
this.termStartDate = termStartDate;
}
public ExpandedSubscription termType(String termType) {
this.termType = termType;
return this;
}
/**
* Get termType
* @return termType
*/
@javax.annotation.Nullable
public String getTermType() {
return termType;
}
public void setTermType(String termType) {
this.termType = termType;
}
public ExpandedSubscription version(Long version) {
this.version = version;
return this;
}
/**
* Get version
* @return version
*/
@javax.annotation.Nullable
public Long getVersion() {
return version;
}
public void setVersion(Long version) {
this.version = version;
}
public ExpandedSubscription createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* Get createdById
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public ExpandedSubscription createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* Get createdDate
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public ExpandedSubscription updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* Get updatedById
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public ExpandedSubscription updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* Get updatedDate
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
public ExpandedSubscription cMRR(BigDecimal cMRR) {
this.cMRR = cMRR;
return this;
}
/**
* Get cMRR
* @return cMRR
*/
@javax.annotation.Nullable
public BigDecimal getcMRR() {
return cMRR;
}
public void setcMRR(BigDecimal cMRR) {
this.cMRR = cMRR;
}
public ExpandedSubscription billToContactSnapshotId(String billToContactSnapshotId) {
this.billToContactSnapshotId = billToContactSnapshotId;
return this;
}
/**
* Get billToContactSnapshotId
* @return billToContactSnapshotId
*/
@javax.annotation.Nullable
public String getBillToContactSnapshotId() {
return billToContactSnapshotId;
}
public void setBillToContactSnapshotId(String billToContactSnapshotId) {
this.billToContactSnapshotId = billToContactSnapshotId;
}
public ExpandedSubscription billToContactId(String billToContactId) {
this.billToContactId = billToContactId;
return this;
}
/**
* Get billToContactId
* @return billToContactId
*/
@javax.annotation.Nullable
public String getBillToContactId() {
return billToContactId;
}
public void setBillToContactId(String billToContactId) {
this.billToContactId = billToContactId;
}
public ExpandedSubscription invoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
return this;
}
/**
* Get invoiceTemplateId
* @return invoiceTemplateId
*/
@javax.annotation.Nullable
public String getInvoiceTemplateId() {
return invoiceTemplateId;
}
public void setInvoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
}
public ExpandedSubscription sequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
return this;
}
/**
* Get sequenceSetId
* @return sequenceSetId
*/
@javax.annotation.Nullable
public String getSequenceSetId() {
return sequenceSetId;
}
public void setSequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
}
public ExpandedSubscription soldToContactId(String soldToContactId) {
this.soldToContactId = soldToContactId;
return this;
}
/**
* Get soldToContactId
* @return soldToContactId
*/
@javax.annotation.Nullable
public String getSoldToContactId() {
return soldToContactId;
}
public void setSoldToContactId(String soldToContactId) {
this.soldToContactId = soldToContactId;
}
public ExpandedSubscription soldToContactSnapshotId(String soldToContactSnapshotId) {
this.soldToContactSnapshotId = soldToContactSnapshotId;
return this;
}
/**
* Get soldToContactSnapshotId
* @return soldToContactSnapshotId
*/
@javax.annotation.Nullable
public String getSoldToContactSnapshotId() {
return soldToContactSnapshotId;
}
public void setSoldToContactSnapshotId(String soldToContactSnapshotId) {
this.soldToContactSnapshotId = soldToContactSnapshotId;
}
public ExpandedSubscription externallyManagedBy(String externallyManagedBy) {
this.externallyManagedBy = externallyManagedBy;
return this;
}
/**
* Get externallyManagedBy
* @return externallyManagedBy
*/
@javax.annotation.Nullable
public String getExternallyManagedBy() {
return externallyManagedBy;
}
public void setExternallyManagedBy(String externallyManagedBy) {
this.externallyManagedBy = externallyManagedBy;
}
public ExpandedSubscription lastBookingDate(LocalDate lastBookingDate) {
this.lastBookingDate = lastBookingDate;
return this;
}
/**
* Get lastBookingDate
* @return lastBookingDate
*/
@javax.annotation.Nullable
public LocalDate getLastBookingDate() {
return lastBookingDate;
}
public void setLastBookingDate(LocalDate lastBookingDate) {
this.lastBookingDate = lastBookingDate;
}
public ExpandedSubscription invoiceScheduleId(String invoiceScheduleId) {
this.invoiceScheduleId = invoiceScheduleId;
return this;
}
/**
* Get invoiceScheduleId
* @return invoiceScheduleId
*/
@javax.annotation.Nullable
public String getInvoiceScheduleId() {
return invoiceScheduleId;
}
public void setInvoiceScheduleId(String invoiceScheduleId) {
this.invoiceScheduleId = invoiceScheduleId;
}
public ExpandedSubscription cancelReason(String cancelReason) {
this.cancelReason = cancelReason;
return this;
}
/**
* Get cancelReason
* @return cancelReason
*/
@javax.annotation.Nullable
public String getCancelReason() {
return cancelReason;
}
public void setCancelReason(String cancelReason) {
this.cancelReason = cancelReason;
}
public ExpandedSubscription prepayment(Boolean prepayment) {
this.prepayment = prepayment;
return this;
}
/**
* Get prepayment
* @return prepayment
*/
@javax.annotation.Nullable
public Boolean getPrepayment() {
return prepayment;
}
public void setPrepayment(Boolean prepayment) {
this.prepayment = prepayment;
}
public ExpandedSubscription currency(String currency) {
this.currency = currency;
return this;
}
/**
* Get currency
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public ExpandedSubscription isSingleVersioned(Boolean isSingleVersioned) {
this.isSingleVersioned = isSingleVersioned;
return this;
}
/**
* Get isSingleVersioned
* @return isSingleVersioned
*/
@javax.annotation.Nullable
public Boolean getIsSingleVersioned() {
return isSingleVersioned;
}
public void setIsSingleVersioned(Boolean isSingleVersioned) {
this.isSingleVersioned = isSingleVersioned;
}
public ExpandedSubscription orderId(String orderId) {
this.orderId = orderId;
return this;
}
/**
* Get orderId
* @return orderId
*/
@javax.annotation.Nullable
public String getOrderId() {
return orderId;
}
public void setOrderId(String orderId) {
this.orderId = orderId;
}
public ExpandedSubscription rampId(String rampId) {
this.rampId = rampId;
return this;
}
/**
* Get rampId
* @return rampId
*/
@javax.annotation.Nullable
public String getRampId() {
return rampId;
}
public void setRampId(String rampId) {
this.rampId = rampId;
}
public ExpandedSubscription paymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
return this;
}
/**
* Get paymentTerm
* @return paymentTerm
*/
@javax.annotation.Nullable
public String getPaymentTerm() {
return paymentTerm;
}
public void setPaymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
}
public ExpandedSubscription paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
return this;
}
/**
* Get paymentMethodId
* @return paymentMethodId
*/
@javax.annotation.Nullable
public String getPaymentMethodId() {
return paymentMethodId;
}
public void setPaymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
}
public ExpandedSubscription paymentGatewayId(String paymentGatewayId) {
this.paymentGatewayId = paymentGatewayId;
return this;
}
/**
* Get paymentGatewayId
* @return paymentGatewayId
*/
@javax.annotation.Nullable
public String getPaymentGatewayId() {
return paymentGatewayId;
}
public void setPaymentGatewayId(String paymentGatewayId) {
this.paymentGatewayId = paymentGatewayId;
}
public ExpandedSubscription quoteNumberQT(String quoteNumberQT) {
this.quoteNumberQT = quoteNumberQT;
return this;
}
/**
* Get quoteNumberQT
* @return quoteNumberQT
*/
@javax.annotation.Nullable
public String getQuoteNumberQT() {
return quoteNumberQT;
}
public void setQuoteNumberQT(String quoteNumberQT) {
this.quoteNumberQT = quoteNumberQT;
}
public ExpandedSubscription quoteTypeQT(String quoteTypeQT) {
this.quoteTypeQT = quoteTypeQT;
return this;
}
/**
* Get quoteTypeQT
* @return quoteTypeQT
*/
@javax.annotation.Nullable
public String getQuoteTypeQT() {
return quoteTypeQT;
}
public void setQuoteTypeQT(String quoteTypeQT) {
this.quoteTypeQT = quoteTypeQT;
}
public ExpandedSubscription quoteBusinessTypeQT(String quoteBusinessTypeQT) {
this.quoteBusinessTypeQT = quoteBusinessTypeQT;
return this;
}
/**
* Get quoteBusinessTypeQT
* @return quoteBusinessTypeQT
*/
@javax.annotation.Nullable
public String getQuoteBusinessTypeQT() {
return quoteBusinessTypeQT;
}
public void setQuoteBusinessTypeQT(String quoteBusinessTypeQT) {
this.quoteBusinessTypeQT = quoteBusinessTypeQT;
}
public ExpandedSubscription opportunityNameQT(String opportunityNameQT) {
this.opportunityNameQT = opportunityNameQT;
return this;
}
/**
* Get opportunityNameQT
* @return opportunityNameQT
*/
@javax.annotation.Nullable
public String getOpportunityNameQT() {
return opportunityNameQT;
}
public void setOpportunityNameQT(String opportunityNameQT) {
this.opportunityNameQT = opportunityNameQT;
}
public ExpandedSubscription opportunityCloseDateQT(LocalDate opportunityCloseDateQT) {
this.opportunityCloseDateQT = opportunityCloseDateQT;
return this;
}
/**
* Get opportunityCloseDateQT
* @return opportunityCloseDateQT
*/
@javax.annotation.Nullable
public LocalDate getOpportunityCloseDateQT() {
return opportunityCloseDateQT;
}
public void setOpportunityCloseDateQT(LocalDate opportunityCloseDateQT) {
this.opportunityCloseDateQT = opportunityCloseDateQT;
}
public ExpandedSubscription cpqBundleJsonIdQT(String cpqBundleJsonIdQT) {
this.cpqBundleJsonIdQT = cpqBundleJsonIdQT;
return this;
}
/**
* Get cpqBundleJsonIdQT
* @return cpqBundleJsonIdQT
*/
@javax.annotation.Nullable
public String getCpqBundleJsonIdQT() {
return cpqBundleJsonIdQT;
}
public void setCpqBundleJsonIdQT(String cpqBundleJsonIdQT) {
this.cpqBundleJsonIdQT = cpqBundleJsonIdQT;
}
public ExpandedSubscription account(ExpandedAccount account) {
this.account = account;
return this;
}
/**
* Get account
* @return account
*/
@javax.annotation.Nullable
public ExpandedAccount getAccount() {
return account;
}
public void setAccount(ExpandedAccount account) {
this.account = account;
}
public ExpandedSubscription invoiceOwner(ExpandedAccount invoiceOwner) {
this.invoiceOwner = invoiceOwner;
return this;
}
/**
* Get invoiceOwner
* @return invoiceOwner
*/
@javax.annotation.Nullable
public ExpandedAccount getInvoiceOwner() {
return invoiceOwner;
}
public void setInvoiceOwner(ExpandedAccount invoiceOwner) {
this.invoiceOwner = invoiceOwner;
}
public ExpandedSubscription billToContact(ExpandedContact billToContact) {
this.billToContact = billToContact;
return this;
}
/**
* Get billToContact
* @return billToContact
*/
@javax.annotation.Nullable
public ExpandedContact getBillToContact() {
return billToContact;
}
public void setBillToContact(ExpandedContact billToContact) {
this.billToContact = billToContact;
}
public ExpandedSubscription invoiceItems(List invoiceItems) {
this.invoiceItems = invoiceItems;
return this;
}
public ExpandedSubscription addInvoiceItemsItem(ExpandedInvoiceItem invoiceItemsItem) {
if (this.invoiceItems == null) {
this.invoiceItems = new ArrayList<>();
}
this.invoiceItems.add(invoiceItemsItem);
return this;
}
/**
* Get invoiceItems
* @return invoiceItems
*/
@javax.annotation.Nullable
public List getInvoiceItems() {
return invoiceItems;
}
public void setInvoiceItems(List invoiceItems) {
this.invoiceItems = invoiceItems;
}
public ExpandedSubscription ratePlans(List ratePlans) {
this.ratePlans = ratePlans;
return this;
}
public ExpandedSubscription addRatePlansItem(ExpandedRatePlan ratePlansItem) {
if (this.ratePlans == null) {
this.ratePlans = new ArrayList<>();
}
this.ratePlans.add(ratePlansItem);
return this;
}
/**
* Get ratePlans
* @return ratePlans
*/
@javax.annotation.Nullable
public List getRatePlans() {
return ratePlans;
}
public void setRatePlans(List ratePlans) {
this.ratePlans = ratePlans;
}
public ExpandedSubscription order(ExpandedOrders order) {
this.order = order;
return this;
}
/**
* Get order
* @return order
*/
@javax.annotation.Nullable
public ExpandedOrders getOrder() {
return order;
}
public void setOrder(ExpandedOrders order) {
this.order = order;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the ExpandedSubscription instance itself
*/
public ExpandedSubscription putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ExpandedSubscription expandedSubscription = (ExpandedSubscription) o;
return Objects.equals(this.id, expandedSubscription.id) &&
Objects.equals(this.accountId, expandedSubscription.accountId) &&
Objects.equals(this.autoRenew, expandedSubscription.autoRenew) &&
Objects.equals(this.cancelledDate, expandedSubscription.cancelledDate) &&
Objects.equals(this.contractAcceptanceDate, expandedSubscription.contractAcceptanceDate) &&
Objects.equals(this.contractEffectiveDate, expandedSubscription.contractEffectiveDate) &&
Objects.equals(this.creatorAccountId, expandedSubscription.creatorAccountId) &&
Objects.equals(this.creatorInvoiceOwnerId, expandedSubscription.creatorInvoiceOwnerId) &&
Objects.equals(this.currentTerm, expandedSubscription.currentTerm) &&
Objects.equals(this.currentTermPeriodType, expandedSubscription.currentTermPeriodType) &&
Objects.equals(this.initialTerm, expandedSubscription.initialTerm) &&
Objects.equals(this.initialTermPeriodType, expandedSubscription.initialTermPeriodType) &&
Objects.equals(this.invoiceGroupNumber, expandedSubscription.invoiceGroupNumber) &&
Objects.equals(this.invoiceOwnerId, expandedSubscription.invoiceOwnerId) &&
Objects.equals(this.isInvoiceSeparate, expandedSubscription.isInvoiceSeparate) &&
Objects.equals(this.name, expandedSubscription.name) &&
Objects.equals(this.notes, expandedSubscription.notes) &&
Objects.equals(this.originalCreatedDate, expandedSubscription.originalCreatedDate) &&
Objects.equals(this.originalId, expandedSubscription.originalId) &&
Objects.equals(this.previousSubscriptionId, expandedSubscription.previousSubscriptionId) &&
Objects.equals(this.renewalSetting, expandedSubscription.renewalSetting) &&
Objects.equals(this.renewalTerm, expandedSubscription.renewalTerm) &&
Objects.equals(this.renewalTermPeriodType, expandedSubscription.renewalTermPeriodType) &&
Objects.equals(this.revision, expandedSubscription.revision) &&
Objects.equals(this.serviceActivationDate, expandedSubscription.serviceActivationDate) &&
Objects.equals(this.status, expandedSubscription.status) &&
Objects.equals(this.isLatestVersion, expandedSubscription.isLatestVersion) &&
Objects.equals(this.subscriptionEndDate, expandedSubscription.subscriptionEndDate) &&
Objects.equals(this.subscriptionStartDate, expandedSubscription.subscriptionStartDate) &&
Objects.equals(this.subscriptionVersionAmendmentId, expandedSubscription.subscriptionVersionAmendmentId) &&
Objects.equals(this.termEndDate, expandedSubscription.termEndDate) &&
Objects.equals(this.termStartDate, expandedSubscription.termStartDate) &&
Objects.equals(this.termType, expandedSubscription.termType) &&
Objects.equals(this.version, expandedSubscription.version) &&
Objects.equals(this.createdById, expandedSubscription.createdById) &&
Objects.equals(this.createdDate, expandedSubscription.createdDate) &&
Objects.equals(this.updatedById, expandedSubscription.updatedById) &&
Objects.equals(this.updatedDate, expandedSubscription.updatedDate) &&
Objects.equals(this.cMRR, expandedSubscription.cMRR) &&
Objects.equals(this.billToContactSnapshotId, expandedSubscription.billToContactSnapshotId) &&
Objects.equals(this.billToContactId, expandedSubscription.billToContactId) &&
Objects.equals(this.invoiceTemplateId, expandedSubscription.invoiceTemplateId) &&
Objects.equals(this.sequenceSetId, expandedSubscription.sequenceSetId) &&
Objects.equals(this.soldToContactId, expandedSubscription.soldToContactId) &&
Objects.equals(this.soldToContactSnapshotId, expandedSubscription.soldToContactSnapshotId) &&
Objects.equals(this.externallyManagedBy, expandedSubscription.externallyManagedBy) &&
Objects.equals(this.lastBookingDate, expandedSubscription.lastBookingDate) &&
Objects.equals(this.invoiceScheduleId, expandedSubscription.invoiceScheduleId) &&
Objects.equals(this.cancelReason, expandedSubscription.cancelReason) &&
Objects.equals(this.prepayment, expandedSubscription.prepayment) &&
Objects.equals(this.currency, expandedSubscription.currency) &&
Objects.equals(this.isSingleVersioned, expandedSubscription.isSingleVersioned) &&
Objects.equals(this.orderId, expandedSubscription.orderId) &&
Objects.equals(this.rampId, expandedSubscription.rampId) &&
Objects.equals(this.paymentTerm, expandedSubscription.paymentTerm) &&
Objects.equals(this.paymentMethodId, expandedSubscription.paymentMethodId) &&
Objects.equals(this.paymentGatewayId, expandedSubscription.paymentGatewayId) &&
Objects.equals(this.quoteNumberQT, expandedSubscription.quoteNumberQT) &&
Objects.equals(this.quoteTypeQT, expandedSubscription.quoteTypeQT) &&
Objects.equals(this.quoteBusinessTypeQT, expandedSubscription.quoteBusinessTypeQT) &&
Objects.equals(this.opportunityNameQT, expandedSubscription.opportunityNameQT) &&
Objects.equals(this.opportunityCloseDateQT, expandedSubscription.opportunityCloseDateQT) &&
Objects.equals(this.cpqBundleJsonIdQT, expandedSubscription.cpqBundleJsonIdQT) &&
Objects.equals(this.account, expandedSubscription.account) &&
Objects.equals(this.invoiceOwner, expandedSubscription.invoiceOwner) &&
Objects.equals(this.billToContact, expandedSubscription.billToContact) &&
Objects.equals(this.invoiceItems, expandedSubscription.invoiceItems) &&
Objects.equals(this.ratePlans, expandedSubscription.ratePlans) &&
Objects.equals(this.order, expandedSubscription.order)&&
Objects.equals(this.additionalProperties, expandedSubscription.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(id, accountId, autoRenew, cancelledDate, contractAcceptanceDate, contractEffectiveDate, creatorAccountId, creatorInvoiceOwnerId, currentTerm, currentTermPeriodType, initialTerm, initialTermPeriodType, invoiceGroupNumber, invoiceOwnerId, isInvoiceSeparate, name, notes, originalCreatedDate, originalId, previousSubscriptionId, renewalSetting, renewalTerm, renewalTermPeriodType, revision, serviceActivationDate, status, isLatestVersion, subscriptionEndDate, subscriptionStartDate, subscriptionVersionAmendmentId, termEndDate, termStartDate, termType, version, createdById, createdDate, updatedById, updatedDate, cMRR, billToContactSnapshotId, billToContactId, invoiceTemplateId, sequenceSetId, soldToContactId, soldToContactSnapshotId, externallyManagedBy, lastBookingDate, invoiceScheduleId, cancelReason, prepayment, currency, isSingleVersioned, orderId, rampId, paymentTerm, paymentMethodId, paymentGatewayId, quoteNumberQT, quoteTypeQT, quoteBusinessTypeQT, opportunityNameQT, opportunityCloseDateQT, cpqBundleJsonIdQT, account, invoiceOwner, billToContact, invoiceItems, ratePlans, order, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ExpandedSubscription {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" autoRenew: ").append(toIndentedString(autoRenew)).append("\n");
sb.append(" cancelledDate: ").append(toIndentedString(cancelledDate)).append("\n");
sb.append(" contractAcceptanceDate: ").append(toIndentedString(contractAcceptanceDate)).append("\n");
sb.append(" contractEffectiveDate: ").append(toIndentedString(contractEffectiveDate)).append("\n");
sb.append(" creatorAccountId: ").append(toIndentedString(creatorAccountId)).append("\n");
sb.append(" creatorInvoiceOwnerId: ").append(toIndentedString(creatorInvoiceOwnerId)).append("\n");
sb.append(" currentTerm: ").append(toIndentedString(currentTerm)).append("\n");
sb.append(" currentTermPeriodType: ").append(toIndentedString(currentTermPeriodType)).append("\n");
sb.append(" initialTerm: ").append(toIndentedString(initialTerm)).append("\n");
sb.append(" initialTermPeriodType: ").append(toIndentedString(initialTermPeriodType)).append("\n");
sb.append(" invoiceGroupNumber: ").append(toIndentedString(invoiceGroupNumber)).append("\n");
sb.append(" invoiceOwnerId: ").append(toIndentedString(invoiceOwnerId)).append("\n");
sb.append(" isInvoiceSeparate: ").append(toIndentedString(isInvoiceSeparate)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" notes: ").append(toIndentedString(notes)).append("\n");
sb.append(" originalCreatedDate: ").append(toIndentedString(originalCreatedDate)).append("\n");
sb.append(" originalId: ").append(toIndentedString(originalId)).append("\n");
sb.append(" previousSubscriptionId: ").append(toIndentedString(previousSubscriptionId)).append("\n");
sb.append(" renewalSetting: ").append(toIndentedString(renewalSetting)).append("\n");
sb.append(" renewalTerm: ").append(toIndentedString(renewalTerm)).append("\n");
sb.append(" renewalTermPeriodType: ").append(toIndentedString(renewalTermPeriodType)).append("\n");
sb.append(" revision: ").append(toIndentedString(revision)).append("\n");
sb.append(" serviceActivationDate: ").append(toIndentedString(serviceActivationDate)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" isLatestVersion: ").append(toIndentedString(isLatestVersion)).append("\n");
sb.append(" subscriptionEndDate: ").append(toIndentedString(subscriptionEndDate)).append("\n");
sb.append(" subscriptionStartDate: ").append(toIndentedString(subscriptionStartDate)).append("\n");
sb.append(" subscriptionVersionAmendmentId: ").append(toIndentedString(subscriptionVersionAmendmentId)).append("\n");
sb.append(" termEndDate: ").append(toIndentedString(termEndDate)).append("\n");
sb.append(" termStartDate: ").append(toIndentedString(termStartDate)).append("\n");
sb.append(" termType: ").append(toIndentedString(termType)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" cMRR: ").append(toIndentedString(cMRR)).append("\n");
sb.append(" billToContactSnapshotId: ").append(toIndentedString(billToContactSnapshotId)).append("\n");
sb.append(" billToContactId: ").append(toIndentedString(billToContactId)).append("\n");
sb.append(" invoiceTemplateId: ").append(toIndentedString(invoiceTemplateId)).append("\n");
sb.append(" sequenceSetId: ").append(toIndentedString(sequenceSetId)).append("\n");
sb.append(" soldToContactId: ").append(toIndentedString(soldToContactId)).append("\n");
sb.append(" soldToContactSnapshotId: ").append(toIndentedString(soldToContactSnapshotId)).append("\n");
sb.append(" externallyManagedBy: ").append(toIndentedString(externallyManagedBy)).append("\n");
sb.append(" lastBookingDate: ").append(toIndentedString(lastBookingDate)).append("\n");
sb.append(" invoiceScheduleId: ").append(toIndentedString(invoiceScheduleId)).append("\n");
sb.append(" cancelReason: ").append(toIndentedString(cancelReason)).append("\n");
sb.append(" prepayment: ").append(toIndentedString(prepayment)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" isSingleVersioned: ").append(toIndentedString(isSingleVersioned)).append("\n");
sb.append(" orderId: ").append(toIndentedString(orderId)).append("\n");
sb.append(" rampId: ").append(toIndentedString(rampId)).append("\n");
sb.append(" paymentTerm: ").append(toIndentedString(paymentTerm)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" paymentGatewayId: ").append(toIndentedString(paymentGatewayId)).append("\n");
sb.append(" quoteNumberQT: ").append(toIndentedString(quoteNumberQT)).append("\n");
sb.append(" quoteTypeQT: ").append(toIndentedString(quoteTypeQT)).append("\n");
sb.append(" quoteBusinessTypeQT: ").append(toIndentedString(quoteBusinessTypeQT)).append("\n");
sb.append(" opportunityNameQT: ").append(toIndentedString(opportunityNameQT)).append("\n");
sb.append(" opportunityCloseDateQT: ").append(toIndentedString(opportunityCloseDateQT)).append("\n");
sb.append(" cpqBundleJsonIdQT: ").append(toIndentedString(cpqBundleJsonIdQT)).append("\n");
sb.append(" account: ").append(toIndentedString(account)).append("\n");
sb.append(" invoiceOwner: ").append(toIndentedString(invoiceOwner)).append("\n");
sb.append(" billToContact: ").append(toIndentedString(billToContact)).append("\n");
sb.append(" invoiceItems: ").append(toIndentedString(invoiceItems)).append("\n");
sb.append(" ratePlans: ").append(toIndentedString(ratePlans)).append("\n");
sb.append(" order: ").append(toIndentedString(order)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("id");
openapiFields.add("accountId");
openapiFields.add("autoRenew");
openapiFields.add("cancelledDate");
openapiFields.add("contractAcceptanceDate");
openapiFields.add("contractEffectiveDate");
openapiFields.add("creatorAccountId");
openapiFields.add("creatorInvoiceOwnerId");
openapiFields.add("currentTerm");
openapiFields.add("currentTermPeriodType");
openapiFields.add("initialTerm");
openapiFields.add("initialTermPeriodType");
openapiFields.add("invoiceGroupNumber");
openapiFields.add("invoiceOwnerId");
openapiFields.add("isInvoiceSeparate");
openapiFields.add("name");
openapiFields.add("notes");
openapiFields.add("originalCreatedDate");
openapiFields.add("originalId");
openapiFields.add("previousSubscriptionId");
openapiFields.add("renewalSetting");
openapiFields.add("renewalTerm");
openapiFields.add("renewalTermPeriodType");
openapiFields.add("revision");
openapiFields.add("serviceActivationDate");
openapiFields.add("status");
openapiFields.add("isLatestVersion");
openapiFields.add("subscriptionEndDate");
openapiFields.add("subscriptionStartDate");
openapiFields.add("subscriptionVersionAmendmentId");
openapiFields.add("termEndDate");
openapiFields.add("termStartDate");
openapiFields.add("termType");
openapiFields.add("version");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
openapiFields.add("cMRR");
openapiFields.add("billToContactSnapshotId");
openapiFields.add("billToContactId");
openapiFields.add("invoiceTemplateId");
openapiFields.add("sequenceSetId");
openapiFields.add("soldToContactId");
openapiFields.add("soldToContactSnapshotId");
openapiFields.add("externallyManagedBy");
openapiFields.add("lastBookingDate");
openapiFields.add("invoiceScheduleId");
openapiFields.add("cancelReason");
openapiFields.add("prepayment");
openapiFields.add("currency");
openapiFields.add("isSingleVersioned");
openapiFields.add("orderId");
openapiFields.add("rampId");
openapiFields.add("paymentTerm");
openapiFields.add("paymentMethodId");
openapiFields.add("paymentGatewayId");
openapiFields.add("quoteNumber__QT");
openapiFields.add("quoteType__QT");
openapiFields.add("quoteBusinessType__QT");
openapiFields.add("opportunityName__QT");
openapiFields.add("opportunityCloseDate__QT");
openapiFields.add("cpqBundleJsonId__QT");
openapiFields.add("account");
openapiFields.add("invoiceOwner");
openapiFields.add("billToContact");
openapiFields.add("invoiceItems");
openapiFields.add("ratePlans");
openapiFields.add("order");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to ExpandedSubscription
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!ExpandedSubscription.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in ExpandedSubscription is not found in the empty JSON string", ExpandedSubscription.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("accountId") != null && !jsonObj.get("accountId").isJsonNull()) && !jsonObj.get("accountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountId").toString()));
}
if ((jsonObj.get("creatorAccountId") != null && !jsonObj.get("creatorAccountId").isJsonNull()) && !jsonObj.get("creatorAccountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creatorAccountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creatorAccountId").toString()));
}
if ((jsonObj.get("creatorInvoiceOwnerId") != null && !jsonObj.get("creatorInvoiceOwnerId").isJsonNull()) && !jsonObj.get("creatorInvoiceOwnerId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creatorInvoiceOwnerId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creatorInvoiceOwnerId").toString()));
}
if ((jsonObj.get("currentTermPeriodType") != null && !jsonObj.get("currentTermPeriodType").isJsonNull()) && !jsonObj.get("currentTermPeriodType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currentTermPeriodType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currentTermPeriodType").toString()));
}
if ((jsonObj.get("initialTermPeriodType") != null && !jsonObj.get("initialTermPeriodType").isJsonNull()) && !jsonObj.get("initialTermPeriodType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `initialTermPeriodType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("initialTermPeriodType").toString()));
}
if ((jsonObj.get("invoiceGroupNumber") != null && !jsonObj.get("invoiceGroupNumber").isJsonNull()) && !jsonObj.get("invoiceGroupNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceGroupNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceGroupNumber").toString()));
}
if ((jsonObj.get("invoiceOwnerId") != null && !jsonObj.get("invoiceOwnerId").isJsonNull()) && !jsonObj.get("invoiceOwnerId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceOwnerId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceOwnerId").toString()));
}
if ((jsonObj.get("name") != null && !jsonObj.get("name").isJsonNull()) && !jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if ((jsonObj.get("notes") != null && !jsonObj.get("notes").isJsonNull()) && !jsonObj.get("notes").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `notes` to be a primitive type in the JSON string but got `%s`", jsonObj.get("notes").toString()));
}
if ((jsonObj.get("originalCreatedDate") != null && !jsonObj.get("originalCreatedDate").isJsonNull()) && !jsonObj.get("originalCreatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `originalCreatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("originalCreatedDate").toString()));
}
if ((jsonObj.get("originalId") != null && !jsonObj.get("originalId").isJsonNull()) && !jsonObj.get("originalId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `originalId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("originalId").toString()));
}
if ((jsonObj.get("previousSubscriptionId") != null && !jsonObj.get("previousSubscriptionId").isJsonNull()) && !jsonObj.get("previousSubscriptionId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `previousSubscriptionId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("previousSubscriptionId").toString()));
}
if ((jsonObj.get("renewalSetting") != null && !jsonObj.get("renewalSetting").isJsonNull()) && !jsonObj.get("renewalSetting").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `renewalSetting` to be a primitive type in the JSON string but got `%s`", jsonObj.get("renewalSetting").toString()));
}
if ((jsonObj.get("renewalTermPeriodType") != null && !jsonObj.get("renewalTermPeriodType").isJsonNull()) && !jsonObj.get("renewalTermPeriodType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `renewalTermPeriodType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("renewalTermPeriodType").toString()));
}
if ((jsonObj.get("revision") != null && !jsonObj.get("revision").isJsonNull()) && !jsonObj.get("revision").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revision` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revision").toString()));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
if ((jsonObj.get("subscriptionVersionAmendmentId") != null && !jsonObj.get("subscriptionVersionAmendmentId").isJsonNull()) && !jsonObj.get("subscriptionVersionAmendmentId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionVersionAmendmentId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("subscriptionVersionAmendmentId").toString()));
}
if ((jsonObj.get("termType") != null && !jsonObj.get("termType").isJsonNull()) && !jsonObj.get("termType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `termType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("termType").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
if ((jsonObj.get("billToContactSnapshotId") != null && !jsonObj.get("billToContactSnapshotId").isJsonNull()) && !jsonObj.get("billToContactSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billToContactSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billToContactSnapshotId").toString()));
}
if ((jsonObj.get("billToContactId") != null && !jsonObj.get("billToContactId").isJsonNull()) && !jsonObj.get("billToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billToContactId").toString()));
}
if ((jsonObj.get("invoiceTemplateId") != null && !jsonObj.get("invoiceTemplateId").isJsonNull()) && !jsonObj.get("invoiceTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceTemplateId").toString()));
}
if ((jsonObj.get("sequenceSetId") != null && !jsonObj.get("sequenceSetId").isJsonNull()) && !jsonObj.get("sequenceSetId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sequenceSetId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sequenceSetId").toString()));
}
if ((jsonObj.get("soldToContactId") != null && !jsonObj.get("soldToContactId").isJsonNull()) && !jsonObj.get("soldToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToContactId").toString()));
}
if ((jsonObj.get("soldToContactSnapshotId") != null && !jsonObj.get("soldToContactSnapshotId").isJsonNull()) && !jsonObj.get("soldToContactSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToContactSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToContactSnapshotId").toString()));
}
if ((jsonObj.get("externallyManagedBy") != null && !jsonObj.get("externallyManagedBy").isJsonNull()) && !jsonObj.get("externallyManagedBy").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `externallyManagedBy` to be a primitive type in the JSON string but got `%s`", jsonObj.get("externallyManagedBy").toString()));
}
if ((jsonObj.get("invoiceScheduleId") != null && !jsonObj.get("invoiceScheduleId").isJsonNull()) && !jsonObj.get("invoiceScheduleId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceScheduleId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceScheduleId").toString()));
}
if ((jsonObj.get("cancelReason") != null && !jsonObj.get("cancelReason").isJsonNull()) && !jsonObj.get("cancelReason").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cancelReason` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cancelReason").toString()));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("orderId") != null && !jsonObj.get("orderId").isJsonNull()) && !jsonObj.get("orderId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `orderId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("orderId").toString()));
}
if ((jsonObj.get("rampId") != null && !jsonObj.get("rampId").isJsonNull()) && !jsonObj.get("rampId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `rampId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("rampId").toString()));
}
if ((jsonObj.get("paymentTerm") != null && !jsonObj.get("paymentTerm").isJsonNull()) && !jsonObj.get("paymentTerm").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentTerm` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentTerm").toString()));
}
if ((jsonObj.get("paymentMethodId") != null && !jsonObj.get("paymentMethodId").isJsonNull()) && !jsonObj.get("paymentMethodId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentMethodId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentMethodId").toString()));
}
if ((jsonObj.get("paymentGatewayId") != null && !jsonObj.get("paymentGatewayId").isJsonNull()) && !jsonObj.get("paymentGatewayId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentGatewayId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentGatewayId").toString()));
}
if ((jsonObj.get("quoteNumber__QT") != null && !jsonObj.get("quoteNumber__QT").isJsonNull()) && !jsonObj.get("quoteNumber__QT").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `quoteNumber__QT` to be a primitive type in the JSON string but got `%s`", jsonObj.get("quoteNumber__QT").toString()));
}
if ((jsonObj.get("quoteType__QT") != null && !jsonObj.get("quoteType__QT").isJsonNull()) && !jsonObj.get("quoteType__QT").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `quoteType__QT` to be a primitive type in the JSON string but got `%s`", jsonObj.get("quoteType__QT").toString()));
}
if ((jsonObj.get("quoteBusinessType__QT") != null && !jsonObj.get("quoteBusinessType__QT").isJsonNull()) && !jsonObj.get("quoteBusinessType__QT").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `quoteBusinessType__QT` to be a primitive type in the JSON string but got `%s`", jsonObj.get("quoteBusinessType__QT").toString()));
}
if ((jsonObj.get("opportunityName__QT") != null && !jsonObj.get("opportunityName__QT").isJsonNull()) && !jsonObj.get("opportunityName__QT").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `opportunityName__QT` to be a primitive type in the JSON string but got `%s`", jsonObj.get("opportunityName__QT").toString()));
}
if ((jsonObj.get("cpqBundleJsonId__QT") != null && !jsonObj.get("cpqBundleJsonId__QT").isJsonNull()) && !jsonObj.get("cpqBundleJsonId__QT").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cpqBundleJsonId__QT` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cpqBundleJsonId__QT").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("invoiceItems") != null && !jsonObj.get("invoiceItems").isJsonNull() && !jsonObj.get("invoiceItems").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceItems` to be an array in the JSON string but got `%s`", jsonObj.get("invoiceItems").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("ratePlans") != null && !jsonObj.get("ratePlans").isJsonNull() && !jsonObj.get("ratePlans").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `ratePlans` to be an array in the JSON string but got `%s`", jsonObj.get("ratePlans").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!ExpandedSubscription.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'ExpandedSubscription' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(ExpandedSubscription.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, ExpandedSubscription value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public ExpandedSubscription read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
ExpandedSubscription instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of ExpandedSubscription given an JSON string
*
* @param jsonString JSON string
* @return An instance of ExpandedSubscription
* @throws IOException if the JSON string is invalid with respect to ExpandedSubscription
*/
public static ExpandedSubscription fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, ExpandedSubscription.class);
}
/**
* Convert an instance of ExpandedSubscription to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy