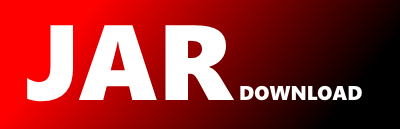
com.zuora.model.GetBillingPreviewRunResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.GetBillingPreviewRunResponseStorageOption;
import java.io.IOException;
import java.time.LocalDate;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* get billingPreviewRun response
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class GetBillingPreviewRunResponse {
public static final String SERIALIZED_NAME_RUN_NUMBER = "runNumber";
@SerializedName(SERIALIZED_NAME_RUN_NUMBER)
private String runNumber;
public static final String SERIALIZED_NAME_ASSUME_RENEWAL = "assumeRenewal";
@SerializedName(SERIALIZED_NAME_ASSUME_RENEWAL)
private String assumeRenewal;
public static final String SERIALIZED_NAME_BATCH = "batch";
@Deprecated
@SerializedName(SERIALIZED_NAME_BATCH)
private String batch;
public static final String SERIALIZED_NAME_BATCHES = "batches";
@SerializedName(SERIALIZED_NAME_BATCHES)
private String batches;
public static final String SERIALIZED_NAME_CHARGE_TYPE_TO_EXCLUDE = "chargeTypeToExclude";
@SerializedName(SERIALIZED_NAME_CHARGE_TYPE_TO_EXCLUDE)
private String chargeTypeToExclude;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_START_DATE = "startDate";
@SerializedName(SERIALIZED_NAME_START_DATE)
private String startDate;
public static final String SERIALIZED_NAME_END_DATE = "endDate";
@SerializedName(SERIALIZED_NAME_END_DATE)
private String endDate;
public static final String SERIALIZED_NAME_ERROR_MESSAGE = "errorMessage";
@SerializedName(SERIALIZED_NAME_ERROR_MESSAGE)
private String errorMessage;
public static final String SERIALIZED_NAME_INCLUDING_DRAFT_ITEMS = "includingDraftItems";
@SerializedName(SERIALIZED_NAME_INCLUDING_DRAFT_ITEMS)
private Boolean includingDraftItems;
public static final String SERIALIZED_NAME_INCLUDING_EVERGREEN_SUBSCRIPTION = "includingEvergreenSubscription";
@SerializedName(SERIALIZED_NAME_INCLUDING_EVERGREEN_SUBSCRIPTION)
private Boolean includingEvergreenSubscription;
public static final String SERIALIZED_NAME_RESULT_FILE_URL = "resultFileUrl";
@SerializedName(SERIALIZED_NAME_RESULT_FILE_URL)
private String resultFileUrl;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private String status;
public static final String SERIALIZED_NAME_STORAGE_OPTION = "storageOption";
@SerializedName(SERIALIZED_NAME_STORAGE_OPTION)
private GetBillingPreviewRunResponseStorageOption storageOption;
public static final String SERIALIZED_NAME_SUCCEEDED_ACCOUNTS = "succeededAccounts";
@SerializedName(SERIALIZED_NAME_SUCCEEDED_ACCOUNTS)
private Integer succeededAccounts;
public static final String SERIALIZED_NAME_SUCCESS = "success";
@SerializedName(SERIALIZED_NAME_SUCCESS)
private Boolean success;
public static final String SERIALIZED_NAME_TARGET_DATE = "targetDate";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public static final String SERIALIZED_NAME_TOTAL_ACCOUNTS = "totalAccounts";
@SerializedName(SERIALIZED_NAME_TOTAL_ACCOUNTS)
private Integer totalAccounts;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public GetBillingPreviewRunResponse() {
}
public GetBillingPreviewRunResponse runNumber(String runNumber) {
this.runNumber = runNumber;
return this;
}
/**
* The run number of the billing preview run.
* @return runNumber
*/
@javax.annotation.Nullable
public String getRunNumber() {
return runNumber;
}
public void setRunNumber(String runNumber) {
this.runNumber = runNumber;
}
public GetBillingPreviewRunResponse assumeRenewal(String assumeRenewal) {
this.assumeRenewal = assumeRenewal;
return this;
}
/**
*
* @return assumeRenewal
*/
@javax.annotation.Nullable
public String getAssumeRenewal() {
return assumeRenewal;
}
public void setAssumeRenewal(String assumeRenewal) {
this.assumeRenewal = assumeRenewal;
}
@Deprecated
public GetBillingPreviewRunResponse batch(String batch) {
this.batch = batch;
return this;
}
/**
* The customer batch included in the billing preview run. **Note**: This field is not available if you set the `zuora-version` request header to `314.0` or later.
* @return batch
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public String getBatch() {
return batch;
}
@Deprecated
public void setBatch(String batch) {
this.batch = batch;
}
public GetBillingPreviewRunResponse batches(String batches) {
this.batches = batches;
return this;
}
/**
* The customer batches included in the billing preview run. **Note**: This field is only available if you set the `zuora-version` request header to `314.0` or later.
* @return batches
*/
@javax.annotation.Nullable
public String getBatches() {
return batches;
}
public void setBatches(String batches) {
this.batches = batches;
}
public GetBillingPreviewRunResponse chargeTypeToExclude(String chargeTypeToExclude) {
this.chargeTypeToExclude = chargeTypeToExclude;
return this;
}
/**
* The charge types excluded from the forecast run.
* @return chargeTypeToExclude
*/
@javax.annotation.Nullable
public String getChargeTypeToExclude() {
return chargeTypeToExclude;
}
public void setChargeTypeToExclude(String chargeTypeToExclude) {
this.chargeTypeToExclude = chargeTypeToExclude;
}
public GetBillingPreviewRunResponse createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* The ID of the user who created the billing preview run.
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public GetBillingPreviewRunResponse createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* The date and time when the billing preview run was created.
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public GetBillingPreviewRunResponse startDate(String startDate) {
this.startDate = startDate;
return this;
}
/**
* The date and time when the billing preview run starts.
* @return startDate
*/
@javax.annotation.Nullable
public String getStartDate() {
return startDate;
}
public void setStartDate(String startDate) {
this.startDate = startDate;
}
public GetBillingPreviewRunResponse endDate(String endDate) {
this.endDate = endDate;
return this;
}
/**
* The date and time when the billing preview run completes.
* @return endDate
*/
@javax.annotation.Nullable
public String getEndDate() {
return endDate;
}
public void setEndDate(String endDate) {
this.endDate = endDate;
}
public GetBillingPreviewRunResponse errorMessage(String errorMessage) {
this.errorMessage = errorMessage;
return this;
}
/**
* The error message generated by a failed billing preview run.
* @return errorMessage
*/
@javax.annotation.Nullable
public String getErrorMessage() {
return errorMessage;
}
public void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
public GetBillingPreviewRunResponse includingDraftItems(Boolean includingDraftItems) {
this.includingDraftItems = includingDraftItems;
return this;
}
/**
* Whether draft document items are included in the billing preview run. By default, draft document items are not included.
* @return includingDraftItems
*/
@javax.annotation.Nullable
public Boolean getIncludingDraftItems() {
return includingDraftItems;
}
public void setIncludingDraftItems(Boolean includingDraftItems) {
this.includingDraftItems = includingDraftItems;
}
public GetBillingPreviewRunResponse includingEvergreenSubscription(Boolean includingEvergreenSubscription) {
this.includingEvergreenSubscription = includingEvergreenSubscription;
return this;
}
/**
* Indicates if evergreen subscriptions are included in the billing preview run.
* @return includingEvergreenSubscription
*/
@javax.annotation.Nullable
public Boolean getIncludingEvergreenSubscription() {
return includingEvergreenSubscription;
}
public void setIncludingEvergreenSubscription(Boolean includingEvergreenSubscription) {
this.includingEvergreenSubscription = includingEvergreenSubscription;
}
public GetBillingPreviewRunResponse resultFileUrl(String resultFileUrl) {
this.resultFileUrl = resultFileUrl;
return this;
}
/**
* The URL of the zipped CSV result file generated by the billing preview run. This file contains the preview invoice item data and credit memo item data for the specified customers. If the value of `storageOption` field is `Database`, the returned `resultFileUrl` field is null. **Note:** The credit memo item data is only available if you have Invoice Settlement feature enabled. The Invoice Settlement feature is generally available as of Zuora Billing Release 296 (March 2021). This feature includes Unapplied Payments, Credit and Debit Memo, and Invoice Item Settlement. If you want to enable Invoice Settlement, see [Invoice Settlement Enablement and Checklist Guide](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement/Invoice_Settlement_Migration_Checklist_and_Guide) for more information.
* @return resultFileUrl
*/
@javax.annotation.Nullable
public String getResultFileUrl() {
return resultFileUrl;
}
public void setResultFileUrl(String resultFileUrl) {
this.resultFileUrl = resultFileUrl;
}
public GetBillingPreviewRunResponse status(String status) {
this.status = status;
return this;
}
/**
* The status of the >billing preview run. **Possible values:** * 0: Pending * 1: Processing * 2: Completed * 3: Error * 4: Canceled
* @return status
*/
@javax.annotation.Nullable
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public GetBillingPreviewRunResponse storageOption(GetBillingPreviewRunResponseStorageOption storageOption) {
this.storageOption = storageOption;
return this;
}
/**
* Get storageOption
* @return storageOption
*/
@javax.annotation.Nullable
public GetBillingPreviewRunResponseStorageOption getStorageOption() {
return storageOption;
}
public void setStorageOption(GetBillingPreviewRunResponseStorageOption storageOption) {
this.storageOption = storageOption;
}
public GetBillingPreviewRunResponse succeededAccounts(Integer succeededAccounts) {
this.succeededAccounts = succeededAccounts;
return this;
}
/**
* The number of accounts for which preview invoice item data and credit memo item data was successfully generated during the billing preview run. **Note:** The credit memo item data is only available if you have Invoice Settlement feature enabled. The Invoice Settlement feature is generally available as of Zuora Billing Release 296 (March 2021). This feature includes Unapplied Payments, Credit and Debit Memo, and Invoice Item Settlement. If you want to enable Invoice Settlement, see [Invoice Settlement Enablement and Checklist Guide](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement/Invoice_Settlement_Migration_Checklist_and_Guide) for more information.
* @return succeededAccounts
*/
@javax.annotation.Nullable
public Integer getSucceededAccounts() {
return succeededAccounts;
}
public void setSucceededAccounts(Integer succeededAccounts) {
this.succeededAccounts = succeededAccounts;
}
public GetBillingPreviewRunResponse success(Boolean success) {
this.success = success;
return this;
}
/**
* Returns `true` if the request was processed successfully.
* @return success
*/
@javax.annotation.Nullable
public Boolean getSuccess() {
return success;
}
public void setSuccess(Boolean success) {
this.success = success;
}
public GetBillingPreviewRunResponse targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* The target date for the billing preview run.
* @return targetDate
*/
@javax.annotation.Nullable
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public GetBillingPreviewRunResponse totalAccounts(Integer totalAccounts) {
this.totalAccounts = totalAccounts;
return this;
}
/**
* The total number of accounts in the billing preview run.
* @return totalAccounts
*/
@javax.annotation.Nullable
public Integer getTotalAccounts() {
return totalAccounts;
}
public void setTotalAccounts(Integer totalAccounts) {
this.totalAccounts = totalAccounts;
}
public GetBillingPreviewRunResponse updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* The ID of the user who last updated the billing preview run.
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public GetBillingPreviewRunResponse updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* The date and time when the billing preview run was last updated.
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the GetBillingPreviewRunResponse instance itself
*/
public GetBillingPreviewRunResponse putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GetBillingPreviewRunResponse getBillingPreviewRunResponse = (GetBillingPreviewRunResponse) o;
return Objects.equals(this.runNumber, getBillingPreviewRunResponse.runNumber) &&
Objects.equals(this.assumeRenewal, getBillingPreviewRunResponse.assumeRenewal) &&
Objects.equals(this.batch, getBillingPreviewRunResponse.batch) &&
Objects.equals(this.batches, getBillingPreviewRunResponse.batches) &&
Objects.equals(this.chargeTypeToExclude, getBillingPreviewRunResponse.chargeTypeToExclude) &&
Objects.equals(this.createdById, getBillingPreviewRunResponse.createdById) &&
Objects.equals(this.createdDate, getBillingPreviewRunResponse.createdDate) &&
Objects.equals(this.startDate, getBillingPreviewRunResponse.startDate) &&
Objects.equals(this.endDate, getBillingPreviewRunResponse.endDate) &&
Objects.equals(this.errorMessage, getBillingPreviewRunResponse.errorMessage) &&
Objects.equals(this.includingDraftItems, getBillingPreviewRunResponse.includingDraftItems) &&
Objects.equals(this.includingEvergreenSubscription, getBillingPreviewRunResponse.includingEvergreenSubscription) &&
Objects.equals(this.resultFileUrl, getBillingPreviewRunResponse.resultFileUrl) &&
Objects.equals(this.status, getBillingPreviewRunResponse.status) &&
Objects.equals(this.storageOption, getBillingPreviewRunResponse.storageOption) &&
Objects.equals(this.succeededAccounts, getBillingPreviewRunResponse.succeededAccounts) &&
Objects.equals(this.success, getBillingPreviewRunResponse.success) &&
Objects.equals(this.targetDate, getBillingPreviewRunResponse.targetDate) &&
Objects.equals(this.totalAccounts, getBillingPreviewRunResponse.totalAccounts) &&
Objects.equals(this.updatedById, getBillingPreviewRunResponse.updatedById) &&
Objects.equals(this.updatedDate, getBillingPreviewRunResponse.updatedDate)&&
Objects.equals(this.additionalProperties, getBillingPreviewRunResponse.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(runNumber, assumeRenewal, batch, batches, chargeTypeToExclude, createdById, createdDate, startDate, endDate, errorMessage, includingDraftItems, includingEvergreenSubscription, resultFileUrl, status, storageOption, succeededAccounts, success, targetDate, totalAccounts, updatedById, updatedDate, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GetBillingPreviewRunResponse {\n");
sb.append(" runNumber: ").append(toIndentedString(runNumber)).append("\n");
sb.append(" assumeRenewal: ").append(toIndentedString(assumeRenewal)).append("\n");
sb.append(" batch: ").append(toIndentedString(batch)).append("\n");
sb.append(" batches: ").append(toIndentedString(batches)).append("\n");
sb.append(" chargeTypeToExclude: ").append(toIndentedString(chargeTypeToExclude)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" startDate: ").append(toIndentedString(startDate)).append("\n");
sb.append(" endDate: ").append(toIndentedString(endDate)).append("\n");
sb.append(" errorMessage: ").append(toIndentedString(errorMessage)).append("\n");
sb.append(" includingDraftItems: ").append(toIndentedString(includingDraftItems)).append("\n");
sb.append(" includingEvergreenSubscription: ").append(toIndentedString(includingEvergreenSubscription)).append("\n");
sb.append(" resultFileUrl: ").append(toIndentedString(resultFileUrl)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" storageOption: ").append(toIndentedString(storageOption)).append("\n");
sb.append(" succeededAccounts: ").append(toIndentedString(succeededAccounts)).append("\n");
sb.append(" success: ").append(toIndentedString(success)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" totalAccounts: ").append(toIndentedString(totalAccounts)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("runNumber");
openapiFields.add("assumeRenewal");
openapiFields.add("batch");
openapiFields.add("batches");
openapiFields.add("chargeTypeToExclude");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("startDate");
openapiFields.add("endDate");
openapiFields.add("errorMessage");
openapiFields.add("includingDraftItems");
openapiFields.add("includingEvergreenSubscription");
openapiFields.add("resultFileUrl");
openapiFields.add("status");
openapiFields.add("storageOption");
openapiFields.add("succeededAccounts");
openapiFields.add("success");
openapiFields.add("targetDate");
openapiFields.add("totalAccounts");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to GetBillingPreviewRunResponse
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!GetBillingPreviewRunResponse.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in GetBillingPreviewRunResponse is not found in the empty JSON string", GetBillingPreviewRunResponse.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("runNumber") != null && !jsonObj.get("runNumber").isJsonNull()) && !jsonObj.get("runNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `runNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("runNumber").toString()));
}
if ((jsonObj.get("assumeRenewal") != null && !jsonObj.get("assumeRenewal").isJsonNull()) && !jsonObj.get("assumeRenewal").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `assumeRenewal` to be a primitive type in the JSON string but got `%s`", jsonObj.get("assumeRenewal").toString()));
}
if ((jsonObj.get("batch") != null && !jsonObj.get("batch").isJsonNull()) && !jsonObj.get("batch").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `batch` to be a primitive type in the JSON string but got `%s`", jsonObj.get("batch").toString()));
}
if ((jsonObj.get("batches") != null && !jsonObj.get("batches").isJsonNull()) && !jsonObj.get("batches").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `batches` to be a primitive type in the JSON string but got `%s`", jsonObj.get("batches").toString()));
}
if ((jsonObj.get("chargeTypeToExclude") != null && !jsonObj.get("chargeTypeToExclude").isJsonNull()) && !jsonObj.get("chargeTypeToExclude").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeTypeToExclude` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeTypeToExclude").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("startDate") != null && !jsonObj.get("startDate").isJsonNull()) && !jsonObj.get("startDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `startDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("startDate").toString()));
}
if ((jsonObj.get("endDate") != null && !jsonObj.get("endDate").isJsonNull()) && !jsonObj.get("endDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `endDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("endDate").toString()));
}
if ((jsonObj.get("errorMessage") != null && !jsonObj.get("errorMessage").isJsonNull()) && !jsonObj.get("errorMessage").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `errorMessage` to be a primitive type in the JSON string but got `%s`", jsonObj.get("errorMessage").toString()));
}
if ((jsonObj.get("resultFileUrl") != null && !jsonObj.get("resultFileUrl").isJsonNull()) && !jsonObj.get("resultFileUrl").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `resultFileUrl` to be a primitive type in the JSON string but got `%s`", jsonObj.get("resultFileUrl").toString()));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
if ((jsonObj.get("storageOption") != null && !jsonObj.get("storageOption").isJsonNull()) && !jsonObj.get("storageOption").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `storageOption` to be a primitive type in the JSON string but got `%s`", jsonObj.get("storageOption").toString()));
}
// validate the optional field `storageOption`
if (jsonObj.get("storageOption") != null && !jsonObj.get("storageOption").isJsonNull()) {
GetBillingPreviewRunResponseStorageOption.validateJsonElement(jsonObj.get("storageOption"));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!GetBillingPreviewRunResponse.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'GetBillingPreviewRunResponse' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(GetBillingPreviewRunResponse.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, GetBillingPreviewRunResponse value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public GetBillingPreviewRunResponse read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
GetBillingPreviewRunResponse instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of GetBillingPreviewRunResponse given an JSON string
*
* @param jsonString JSON string
* @return An instance of GetBillingPreviewRunResponse
* @throws IOException if the JSON string is invalid with respect to GetBillingPreviewRunResponse
*/
public static GetBillingPreviewRunResponse fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, GetBillingPreviewRunResponse.class);
}
/**
* Convert an instance of GetBillingPreviewRunResponse to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy