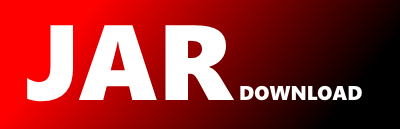
com.zuora.model.GetOrderLineItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.GetFulfillment;
import com.zuora.model.OrderLineItemBillingRule;
import com.zuora.model.OrderLineItemCategory;
import com.zuora.model.OrderLineItemInlineDiscountType;
import com.zuora.model.OrderLineItemState;
import com.zuora.model.OrderLineItemType;
import com.zuora.model.TaxMode;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* GetOrderLineItem
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class GetOrderLineItem {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_AMENDED_BY_ORDER_ON = "amendedByOrderOn";
@SerializedName(SERIALIZED_NAME_AMENDED_BY_ORDER_ON)
private String amendedByOrderOn;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_PAYMENT_TERM = "paymentTerm";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERM)
private String paymentTerm;
public static final String SERIALIZED_NAME_INVOICE_TEMPLATE_ID = "invoiceTemplateId";
@SerializedName(SERIALIZED_NAME_INVOICE_TEMPLATE_ID)
private String invoiceTemplateId;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_AMOUNT_WITHOUT_TAX = "amountWithoutTax";
@SerializedName(SERIALIZED_NAME_AMOUNT_WITHOUT_TAX)
private BigDecimal amountWithoutTax;
public static final String SERIALIZED_NAME_ITEM_NUMBER = "itemNumber";
@SerializedName(SERIALIZED_NAME_ITEM_NUMBER)
private String itemNumber;
public static final String SERIALIZED_NAME_U_O_M = "UOM";
@SerializedName(SERIALIZED_NAME_U_O_M)
private String UOM;
public static final String SERIALIZED_NAME_AMOUNT_PER_UNIT = "amountPerUnit";
@SerializedName(SERIALIZED_NAME_AMOUNT_PER_UNIT)
private BigDecimal amountPerUnit;
public static final String SERIALIZED_NAME_BILL_TARGET_DATE = "billTargetDate";
@SerializedName(SERIALIZED_NAME_BILL_TARGET_DATE)
private LocalDate billTargetDate;
public static final String SERIALIZED_NAME_BILL_TO = "billTo";
@SerializedName(SERIALIZED_NAME_BILL_TO)
private String billTo;
public static final String SERIALIZED_NAME_BILL_TO_SNAPSHOT_ID = "billToSnapshotId";
@SerializedName(SERIALIZED_NAME_BILL_TO_SNAPSHOT_ID)
private String billToSnapshotId;
public static final String SERIALIZED_NAME_BILLING_RULE = "billingRule";
@SerializedName(SERIALIZED_NAME_BILLING_RULE)
private OrderLineItemBillingRule billingRule = OrderLineItemBillingRule.TRIGGERWITHOUTFULFILLMENT;
public static final String SERIALIZED_NAME_ACCOUNTING_CODE = "accountingCode";
@SerializedName(SERIALIZED_NAME_ACCOUNTING_CODE)
private String accountingCode;
public static final String SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNTING_CODE = "adjustmentLiabilityAccountingCode";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNTING_CODE)
private String adjustmentLiabilityAccountingCode;
public static final String SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNTING_CODE = "adjustmentRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNTING_CODE)
private String adjustmentRevenueAccountingCode;
public static final String SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNTING_CODE = "contractAssetAccountingCode";
@SerializedName(SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNTING_CODE)
private String contractAssetAccountingCode;
public static final String SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNTING_CODE = "contractLiabilityAccountingCode";
@SerializedName(SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNTING_CODE)
private String contractLiabilityAccountingCode;
public static final String SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNTING_CODE = "contractRecognizedRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNTING_CODE)
private String contractRecognizedRevenueAccountingCode;
public static final String SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE = "deferredRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE)
private String deferredRevenueAccountingCode;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "customFields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
private Map customFields;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_DISCOUNT = "discount";
@SerializedName(SERIALIZED_NAME_DISCOUNT)
private BigDecimal discount;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING = "excludeItemBillingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBillingFromRevenueAccounting;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING = "excludeItemBookingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBookingFromRevenueAccounting;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_TIMING = "revenueRecognitionTiming";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_TIMING)
private String revenueRecognitionTiming;
public static final String SERIALIZED_NAME_REVENUE_AMORTIZATION_METHOD = "revenueAmortizationMethod";
@SerializedName(SERIALIZED_NAME_REVENUE_AMORTIZATION_METHOD)
private String revenueAmortizationMethod;
public static final String SERIALIZED_NAME_INLINE_DISCOUNT_PER_UNIT = "inlineDiscountPerUnit";
@SerializedName(SERIALIZED_NAME_INLINE_DISCOUNT_PER_UNIT)
private BigDecimal inlineDiscountPerUnit;
public static final String SERIALIZED_NAME_INLINE_DISCOUNT_TYPE = "inlineDiscountType";
@SerializedName(SERIALIZED_NAME_INLINE_DISCOUNT_TYPE)
private OrderLineItemInlineDiscountType inlineDiscountType;
public static final String SERIALIZED_NAME_INVOICE_GROUP_NUMBER = "invoiceGroupNumber";
@SerializedName(SERIALIZED_NAME_INVOICE_GROUP_NUMBER)
private String invoiceGroupNumber;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_ID = "invoiceOwnerAccountId";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_ID)
private String invoiceOwnerAccountId;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_NAME = "invoiceOwnerAccountName";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_NAME)
private String invoiceOwnerAccountName;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_NUMBER = "invoiceOwnerAccountNumber";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_NUMBER)
private String invoiceOwnerAccountNumber;
public static final String SERIALIZED_NAME_IS_ALLOCATION_ELIGIBLE = "isAllocationEligible";
@SerializedName(SERIALIZED_NAME_IS_ALLOCATION_ELIGIBLE)
private Boolean isAllocationEligible;
public static final String SERIALIZED_NAME_IS_UNBILLED = "isUnbilled";
@SerializedName(SERIALIZED_NAME_IS_UNBILLED)
private Boolean isUnbilled;
public static final String SERIALIZED_NAME_ITEM_CATEGORY = "itemCategory";
@SerializedName(SERIALIZED_NAME_ITEM_CATEGORY)
private OrderLineItemCategory itemCategory = OrderLineItemCategory.SALES;
public static final String SERIALIZED_NAME_ITEM_NAME = "itemName";
@SerializedName(SERIALIZED_NAME_ITEM_NAME)
private String itemName;
public static final String SERIALIZED_NAME_ITEM_STATE = "itemState";
@SerializedName(SERIALIZED_NAME_ITEM_STATE)
private OrderLineItemState itemState;
public static final String SERIALIZED_NAME_ITEM_TYPE = "itemType";
@SerializedName(SERIALIZED_NAME_ITEM_TYPE)
private OrderLineItemType itemType;
public static final String SERIALIZED_NAME_LIST_PRICE = "listPrice";
@SerializedName(SERIALIZED_NAME_LIST_PRICE)
private BigDecimal listPrice;
public static final String SERIALIZED_NAME_LIST_PRICE_PER_UNIT = "listPricePerUnit";
@SerializedName(SERIALIZED_NAME_LIST_PRICE_PER_UNIT)
private BigDecimal listPricePerUnit;
public static final String SERIALIZED_NAME_ORIGINAL_ORDER_ID = "originalOrderId";
@SerializedName(SERIALIZED_NAME_ORIGINAL_ORDER_ID)
private String originalOrderId;
public static final String SERIALIZED_NAME_ORIGINAL_ORDER_DATE = "originalOrderDate";
@SerializedName(SERIALIZED_NAME_ORIGINAL_ORDER_DATE)
private LocalDate originalOrderDate;
public static final String SERIALIZED_NAME_ORIGINAL_ORDER_LINE_ITEM_ID = "originalOrderLineItemId";
@SerializedName(SERIALIZED_NAME_ORIGINAL_ORDER_LINE_ITEM_ID)
private String originalOrderLineItemId;
public static final String SERIALIZED_NAME_ORIGINAL_ORDER_LINE_ITEM_NUMBER = "originalOrderLineItemNumber";
@SerializedName(SERIALIZED_NAME_ORIGINAL_ORDER_LINE_ITEM_NUMBER)
private String originalOrderLineItemNumber;
public static final String SERIALIZED_NAME_ORIGINAL_ORDER_NUMBER = "originalOrderNumber";
@SerializedName(SERIALIZED_NAME_ORIGINAL_ORDER_NUMBER)
private String originalOrderNumber;
public static final String SERIALIZED_NAME_OWNER_ACCOUNT_ID = "ownerAccountId";
@SerializedName(SERIALIZED_NAME_OWNER_ACCOUNT_ID)
private String ownerAccountId;
public static final String SERIALIZED_NAME_OWNER_ACCOUNT_NAME = "ownerAccountName";
@SerializedName(SERIALIZED_NAME_OWNER_ACCOUNT_NAME)
private String ownerAccountName;
public static final String SERIALIZED_NAME_OWNER_ACCOUNT_NUMBER = "ownerAccountNumber";
@SerializedName(SERIALIZED_NAME_OWNER_ACCOUNT_NUMBER)
private String ownerAccountNumber;
public static final String SERIALIZED_NAME_PRODUCT_CODE = "productCode";
@SerializedName(SERIALIZED_NAME_PRODUCT_CODE)
private String productCode;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_ID = "productRatePlanChargeId";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_ID)
private String productRatePlanChargeId;
public static final String SERIALIZED_NAME_PURCHASE_ORDER_NUMBER = "purchaseOrderNumber";
@SerializedName(SERIALIZED_NAME_PURCHASE_ORDER_NUMBER)
private String purchaseOrderNumber;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_QUANTITY_AVAILABLE_FOR_RETURN = "quantityAvailableForReturn";
@SerializedName(SERIALIZED_NAME_QUANTITY_AVAILABLE_FOR_RETURN)
private BigDecimal quantityAvailableForReturn;
public static final String SERIALIZED_NAME_QUANTITY_FULFILLED = "quantityFulfilled";
@SerializedName(SERIALIZED_NAME_QUANTITY_FULFILLED)
private BigDecimal quantityFulfilled;
public static final String SERIALIZED_NAME_QUANTITY_PENDING_FULFILLMENT = "quantityPendingFulfillment";
@SerializedName(SERIALIZED_NAME_QUANTITY_PENDING_FULFILLMENT)
private BigDecimal quantityPendingFulfillment;
public static final String SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE = "recognizedRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE)
private String recognizedRevenueAccountingCode;
public static final String SERIALIZED_NAME_RELATED_SUBSCRIPTION_NUMBER = "relatedSubscriptionNumber";
@SerializedName(SERIALIZED_NAME_RELATED_SUBSCRIPTION_NUMBER)
private String relatedSubscriptionNumber;
public static final String SERIALIZED_NAME_REQUIRES_FULFILLMENT = "requiresFulfillment";
@SerializedName(SERIALIZED_NAME_REQUIRES_FULFILLMENT)
private Boolean requiresFulfillment;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_RULE = "revenueRecognitionRule";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_RULE)
private String revenueRecognitionRule;
public static final String SERIALIZED_NAME_SEQUENCE_SET_ID = "sequenceSetId";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_ID)
private String sequenceSetId;
public static final String SERIALIZED_NAME_SOLD_TO = "soldTo";
@SerializedName(SERIALIZED_NAME_SOLD_TO)
private String soldTo;
public static final String SERIALIZED_NAME_SOLD_TO_SNAPSHOT_ID = "soldToSnapshotId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_SNAPSHOT_ID)
private String soldToSnapshotId;
public static final String SERIALIZED_NAME_SHIP_TO = "shipTo";
@SerializedName(SERIALIZED_NAME_SHIP_TO)
private String shipTo;
public static final String SERIALIZED_NAME_SHIP_TO_SNAPSHOT_ID = "shipToSnapshotId";
@SerializedName(SERIALIZED_NAME_SHIP_TO_SNAPSHOT_ID)
private String shipToSnapshotId;
public static final String SERIALIZED_NAME_TAX_CODE = "taxCode";
@SerializedName(SERIALIZED_NAME_TAX_CODE)
private String taxCode;
public static final String SERIALIZED_NAME_TAX_MODE = "taxMode";
@SerializedName(SERIALIZED_NAME_TAX_MODE)
private TaxMode taxMode;
public static final String SERIALIZED_NAME_TRANSACTION_END_DATE = "transactionEndDate";
@SerializedName(SERIALIZED_NAME_TRANSACTION_END_DATE)
private LocalDate transactionEndDate;
public static final String SERIALIZED_NAME_TRANSACTION_START_DATE = "transactionStartDate";
@SerializedName(SERIALIZED_NAME_TRANSACTION_START_DATE)
private LocalDate transactionStartDate;
public static final String SERIALIZED_NAME_UNBILLED_RECEIVABLES_ACCOUNTING_CODE = "unbilledReceivablesAccountingCode";
@SerializedName(SERIALIZED_NAME_UNBILLED_RECEIVABLES_ACCOUNTING_CODE)
private String unbilledReceivablesAccountingCode;
public static final String SERIALIZED_NAME_FULFILLMENTS = "fulfillments";
@SerializedName(SERIALIZED_NAME_FULFILLMENTS)
private List fulfillments;
public GetOrderLineItem() {
}
public GetOrderLineItem id(String id) {
this.id = id;
return this;
}
/**
* The sytem generated Id for the Order Line Item.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public GetOrderLineItem amendedByOrderOn(String amendedByOrderOn) {
this.amendedByOrderOn = amendedByOrderOn;
return this;
}
/**
* The date when the rate plan charge is amended through an order or amendment. This field is to standardize the booking date information to increase audit ability and traceability of data between Zuora Billing and Zuora Revenue. It is mapped as the booking date for a sale order line in Zuora Revenue.
* @return amendedByOrderOn
*/
@javax.annotation.Nullable
public String getAmendedByOrderOn() {
return amendedByOrderOn;
}
public void setAmendedByOrderOn(String amendedByOrderOn) {
this.amendedByOrderOn = amendedByOrderOn;
}
public GetOrderLineItem currency(String currency) {
this.currency = currency;
return this;
}
/**
* The currency for the Order Line Item.
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public GetOrderLineItem paymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
return this;
}
/**
* The payment term for the Order Line Item
* @return paymentTerm
*/
@javax.annotation.Nullable
public String getPaymentTerm() {
return paymentTerm;
}
public void setPaymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
}
public GetOrderLineItem invoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
return this;
}
/**
* The invoice template id for the Order Line Item
* @return invoiceTemplateId
*/
@javax.annotation.Nullable
public String getInvoiceTemplateId() {
return invoiceTemplateId;
}
public void setInvoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
}
public GetOrderLineItem amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The calculated gross amount for the Order Line Item.
* @return amount
*/
@javax.annotation.Nullable
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public GetOrderLineItem amountWithoutTax(BigDecimal amountWithoutTax) {
this.amountWithoutTax = amountWithoutTax;
return this;
}
/**
* The calculated gross amount for an order line item excluding tax. If the tax mode is tax exclusive, the value of this field equals that of the `amount` field. If the tax mode of an order line item is not set, the system treats it as tax exclusive by default. The value of the `amountWithoutTax` field equals that of the `amount` field. If you create an order line item from the product catalog, the tax mode and tax code of the product rate plan charge are used for the order line item by default. You can still overwrite this default set-up by setting the tax mode and tax code of the order line item.
* @return amountWithoutTax
*/
@javax.annotation.Nullable
public BigDecimal getAmountWithoutTax() {
return amountWithoutTax;
}
public void setAmountWithoutTax(BigDecimal amountWithoutTax) {
this.amountWithoutTax = amountWithoutTax;
}
public GetOrderLineItem itemNumber(String itemNumber) {
this.itemNumber = itemNumber;
return this;
}
/**
* The number for the Order Line Item.
* @return itemNumber
*/
@javax.annotation.Nullable
public String getItemNumber() {
return itemNumber;
}
public void setItemNumber(String itemNumber) {
this.itemNumber = itemNumber;
}
public GetOrderLineItem UOM(String UOM) {
this.UOM = UOM;
return this;
}
/**
* Specifies the units to measure usage.
* @return UOM
*/
@javax.annotation.Nullable
public String getUOM() {
return UOM;
}
public void setUOM(String UOM) {
this.UOM = UOM;
}
public GetOrderLineItem amountPerUnit(BigDecimal amountPerUnit) {
this.amountPerUnit = amountPerUnit;
return this;
}
/**
* The actual charged amount per unit for the Order Line Item.
* @return amountPerUnit
*/
@javax.annotation.Nullable
public BigDecimal getAmountPerUnit() {
return amountPerUnit;
}
public void setAmountPerUnit(BigDecimal amountPerUnit) {
this.amountPerUnit = amountPerUnit;
}
public GetOrderLineItem billTargetDate(LocalDate billTargetDate) {
this.billTargetDate = billTargetDate;
return this;
}
/**
* The target date for the Order Line Item to be picked up by bill run for billing.
* @return billTargetDate
*/
@javax.annotation.Nullable
public LocalDate getBillTargetDate() {
return billTargetDate;
}
public void setBillTargetDate(LocalDate billTargetDate) {
this.billTargetDate = billTargetDate;
}
public GetOrderLineItem billTo(String billTo) {
this.billTo = billTo;
return this;
}
/**
* The ID of a contact that belongs to the billing account of the order line item. Use this field to assign an existing account as the bill-to contact of an order line item.
* @return billTo
*/
@javax.annotation.Nullable
public String getBillTo() {
return billTo;
}
public void setBillTo(String billTo) {
this.billTo = billTo;
}
public GetOrderLineItem billToSnapshotId(String billToSnapshotId) {
this.billToSnapshotId = billToSnapshotId;
return this;
}
/**
* The snapshot of the ID for an account used as the sold-to contact of an order line item. This field is used to store the original information about the account, in case the information about the account is changed after the creation of the order line item. The `billToSnapshotId` field is exposed while retrieving the order line item details.
* @return billToSnapshotId
*/
@javax.annotation.Nullable
public String getBillToSnapshotId() {
return billToSnapshotId;
}
public void setBillToSnapshotId(String billToSnapshotId) {
this.billToSnapshotId = billToSnapshotId;
}
public GetOrderLineItem billingRule(OrderLineItemBillingRule billingRule) {
this.billingRule = billingRule;
return this;
}
/**
* Get billingRule
* @return billingRule
*/
@javax.annotation.Nullable
public OrderLineItemBillingRule getBillingRule() {
return billingRule;
}
public void setBillingRule(OrderLineItemBillingRule billingRule) {
this.billingRule = billingRule;
}
public GetOrderLineItem accountingCode(String accountingCode) {
this.accountingCode = accountingCode;
return this;
}
/**
* The accounting code for the Order Line Item.
* @return accountingCode
*/
@javax.annotation.Nullable
public String getAccountingCode() {
return accountingCode;
}
public void setAccountingCode(String accountingCode) {
this.accountingCode = accountingCode;
}
public GetOrderLineItem adjustmentLiabilityAccountingCode(String adjustmentLiabilityAccountingCode) {
this.adjustmentLiabilityAccountingCode = adjustmentLiabilityAccountingCode;
return this;
}
/**
* The accounting code on the Order Line Item object for customers using [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration).
* @return adjustmentLiabilityAccountingCode
*/
@javax.annotation.Nullable
public String getAdjustmentLiabilityAccountingCode() {
return adjustmentLiabilityAccountingCode;
}
public void setAdjustmentLiabilityAccountingCode(String adjustmentLiabilityAccountingCode) {
this.adjustmentLiabilityAccountingCode = adjustmentLiabilityAccountingCode;
}
public GetOrderLineItem adjustmentRevenueAccountingCode(String adjustmentRevenueAccountingCode) {
this.adjustmentRevenueAccountingCode = adjustmentRevenueAccountingCode;
return this;
}
/**
* The accounting code on the Order Line Item object for customers using [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration).
* @return adjustmentRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getAdjustmentRevenueAccountingCode() {
return adjustmentRevenueAccountingCode;
}
public void setAdjustmentRevenueAccountingCode(String adjustmentRevenueAccountingCode) {
this.adjustmentRevenueAccountingCode = adjustmentRevenueAccountingCode;
}
public GetOrderLineItem contractAssetAccountingCode(String contractAssetAccountingCode) {
this.contractAssetAccountingCode = contractAssetAccountingCode;
return this;
}
/**
* The accounting code on the Order Line Item object for customers using [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration).
* @return contractAssetAccountingCode
*/
@javax.annotation.Nullable
public String getContractAssetAccountingCode() {
return contractAssetAccountingCode;
}
public void setContractAssetAccountingCode(String contractAssetAccountingCode) {
this.contractAssetAccountingCode = contractAssetAccountingCode;
}
public GetOrderLineItem contractLiabilityAccountingCode(String contractLiabilityAccountingCode) {
this.contractLiabilityAccountingCode = contractLiabilityAccountingCode;
return this;
}
/**
* The accounting code on the Order Line Item object for customers using [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration).
* @return contractLiabilityAccountingCode
*/
@javax.annotation.Nullable
public String getContractLiabilityAccountingCode() {
return contractLiabilityAccountingCode;
}
public void setContractLiabilityAccountingCode(String contractLiabilityAccountingCode) {
this.contractLiabilityAccountingCode = contractLiabilityAccountingCode;
}
public GetOrderLineItem contractRecognizedRevenueAccountingCode(String contractRecognizedRevenueAccountingCode) {
this.contractRecognizedRevenueAccountingCode = contractRecognizedRevenueAccountingCode;
return this;
}
/**
* The accounting code on the Order Line Item object for customers using [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration).
* @return contractRecognizedRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getContractRecognizedRevenueAccountingCode() {
return contractRecognizedRevenueAccountingCode;
}
public void setContractRecognizedRevenueAccountingCode(String contractRecognizedRevenueAccountingCode) {
this.contractRecognizedRevenueAccountingCode = contractRecognizedRevenueAccountingCode;
}
public GetOrderLineItem deferredRevenueAccountingCode(String deferredRevenueAccountingCode) {
this.deferredRevenueAccountingCode = deferredRevenueAccountingCode;
return this;
}
/**
* The deferred revenue accounting code for the Order Line Item.
* @return deferredRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getDeferredRevenueAccountingCode() {
return deferredRevenueAccountingCode;
}
public void setDeferredRevenueAccountingCode(String deferredRevenueAccountingCode) {
this.deferredRevenueAccountingCode = deferredRevenueAccountingCode;
}
public GetOrderLineItem customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public GetOrderLineItem putCustomFieldsItem(String key, Object customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap<>();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Container for custom fields of an Order Line Item object.
* @return customFields
*/
@javax.annotation.Nullable
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public GetOrderLineItem description(String description) {
this.description = description;
return this;
}
/**
* The description of the Order Line Item.
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public GetOrderLineItem discount(BigDecimal discount) {
this.discount = discount;
return this;
}
/**
* This field shows the total discount amount that is applied to an order line item after the `inlineDiscountType`, `inlineDiscountPerUnit` and `quantity` fields are set. The inline discount is applied to the list price of an order line item (see the `listPrice` field).
* @return discount
*/
@javax.annotation.Nullable
public BigDecimal getDiscount() {
return discount;
}
public void setDiscount(BigDecimal discount) {
this.discount = discount;
}
public GetOrderLineItem excludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
return this;
}
/**
* The flag to exclude Order Line Item related invoice items, invoice item adjustments, credit memo items, and debit memo items from revenue accounting. **Note**: This field is only available if you have the [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration) feature enabled.
* @return excludeItemBillingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBillingFromRevenueAccounting() {
return excludeItemBillingFromRevenueAccounting;
}
public void setExcludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
}
public GetOrderLineItem excludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
return this;
}
/**
* The flag to exclude Order Line Item from revenue accounting. **Note**: This field is only available if you have the [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration) feature enabled.
* @return excludeItemBookingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBookingFromRevenueAccounting() {
return excludeItemBookingFromRevenueAccounting;
}
public void setExcludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
}
public GetOrderLineItem revenueRecognitionTiming(String revenueRecognitionTiming) {
this.revenueRecognitionTiming = revenueRecognitionTiming;
return this;
}
/**
* This field is used to dictate the type of revenue recognition timing.
* @return revenueRecognitionTiming
*/
@javax.annotation.Nullable
public String getRevenueRecognitionTiming() {
return revenueRecognitionTiming;
}
public void setRevenueRecognitionTiming(String revenueRecognitionTiming) {
this.revenueRecognitionTiming = revenueRecognitionTiming;
}
public GetOrderLineItem revenueAmortizationMethod(String revenueAmortizationMethod) {
this.revenueAmortizationMethod = revenueAmortizationMethod;
return this;
}
/**
* This field is used to dictate the type of revenue amortization method.
* @return revenueAmortizationMethod
*/
@javax.annotation.Nullable
public String getRevenueAmortizationMethod() {
return revenueAmortizationMethod;
}
public void setRevenueAmortizationMethod(String revenueAmortizationMethod) {
this.revenueAmortizationMethod = revenueAmortizationMethod;
}
public GetOrderLineItem inlineDiscountPerUnit(BigDecimal inlineDiscountPerUnit) {
this.inlineDiscountPerUnit = inlineDiscountPerUnit;
return this;
}
/**
* This field is used in accordance with the `inlineDiscountType` field, in the following manner: * If the `inlineDiscountType` field is set as `Percentage`, this field specifies the discount percentage for each unit of the order line item. For exmaple, if you specify `5` in this field, the discount percentage is 5%. * If the `inlineDiscountType` field is set as `FixedAmount`, this field specifies the discount amount on each unit of the order line item. For exmaple, if you specify `10` in this field, the discount amount on each unit of the order line item is 10. Once you set the `inlineDiscountType`, `inlineDiscountPerUnit`, and `listPricePerUnit` fields, the system will automatically generate the `amountPerUnit` field. You shall not set the `amountPerUnit` field by yourself.
* @return inlineDiscountPerUnit
*/
@javax.annotation.Nullable
public BigDecimal getInlineDiscountPerUnit() {
return inlineDiscountPerUnit;
}
public void setInlineDiscountPerUnit(BigDecimal inlineDiscountPerUnit) {
this.inlineDiscountPerUnit = inlineDiscountPerUnit;
}
public GetOrderLineItem inlineDiscountType(OrderLineItemInlineDiscountType inlineDiscountType) {
this.inlineDiscountType = inlineDiscountType;
return this;
}
/**
* Get inlineDiscountType
* @return inlineDiscountType
*/
@javax.annotation.Nullable
public OrderLineItemInlineDiscountType getInlineDiscountType() {
return inlineDiscountType;
}
public void setInlineDiscountType(OrderLineItemInlineDiscountType inlineDiscountType) {
this.inlineDiscountType = inlineDiscountType;
}
public GetOrderLineItem invoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
return this;
}
/**
* The invoice group number associated with the order line item.
* @return invoiceGroupNumber
*/
@javax.annotation.Nullable
public String getInvoiceGroupNumber() {
return invoiceGroupNumber;
}
public void setInvoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
}
public GetOrderLineItem invoiceOwnerAccountId(String invoiceOwnerAccountId) {
this.invoiceOwnerAccountId = invoiceOwnerAccountId;
return this;
}
/**
* The account ID of the invoice owner of the order line item.
* @return invoiceOwnerAccountId
*/
@javax.annotation.Nullable
public String getInvoiceOwnerAccountId() {
return invoiceOwnerAccountId;
}
public void setInvoiceOwnerAccountId(String invoiceOwnerAccountId) {
this.invoiceOwnerAccountId = invoiceOwnerAccountId;
}
public GetOrderLineItem invoiceOwnerAccountName(String invoiceOwnerAccountName) {
this.invoiceOwnerAccountName = invoiceOwnerAccountName;
return this;
}
/**
* The account name of the invoice owner of the order line item.
* @return invoiceOwnerAccountName
*/
@javax.annotation.Nullable
public String getInvoiceOwnerAccountName() {
return invoiceOwnerAccountName;
}
public void setInvoiceOwnerAccountName(String invoiceOwnerAccountName) {
this.invoiceOwnerAccountName = invoiceOwnerAccountName;
}
public GetOrderLineItem invoiceOwnerAccountNumber(String invoiceOwnerAccountNumber) {
this.invoiceOwnerAccountNumber = invoiceOwnerAccountNumber;
return this;
}
/**
* The account number of the invoice owner of the order line item.
* @return invoiceOwnerAccountNumber
*/
@javax.annotation.Nullable
public String getInvoiceOwnerAccountNumber() {
return invoiceOwnerAccountNumber;
}
public void setInvoiceOwnerAccountNumber(String invoiceOwnerAccountNumber) {
this.invoiceOwnerAccountNumber = invoiceOwnerAccountNumber;
}
public GetOrderLineItem isAllocationEligible(Boolean isAllocationEligible) {
this.isAllocationEligible = isAllocationEligible;
return this;
}
/**
* This field is used to identify if the charge segment is allocation eligible in revenue recognition. **Note**: This feature is in the **Early Adopter** phase. If you want to use the feature, submit a request at <a href=\"https://support.zuora.com/\" target=\"_blank\">Zuora Global Support</a>, and we will evaluate whether the feature is suitable for your use cases.
* @return isAllocationEligible
*/
@javax.annotation.Nullable
public Boolean getIsAllocationEligible() {
return isAllocationEligible;
}
public void setIsAllocationEligible(Boolean isAllocationEligible) {
this.isAllocationEligible = isAllocationEligible;
}
public GetOrderLineItem isUnbilled(Boolean isUnbilled) {
this.isUnbilled = isUnbilled;
return this;
}
/**
* This field is used to dictate how to perform the accounting during revenue recognition. **Note**: This feature is in the **Early Adopter** phase. If you want to use the feature, submit a request at <a href=\"https://support.zuora.com/\" target=\"_blank\">Zuora Global Support</a>, and we will evaluate whether the feature is suitable for your use cases.
* @return isUnbilled
*/
@javax.annotation.Nullable
public Boolean getIsUnbilled() {
return isUnbilled;
}
public void setIsUnbilled(Boolean isUnbilled) {
this.isUnbilled = isUnbilled;
}
public GetOrderLineItem itemCategory(OrderLineItemCategory itemCategory) {
this.itemCategory = itemCategory;
return this;
}
/**
* Get itemCategory
* @return itemCategory
*/
@javax.annotation.Nullable
public OrderLineItemCategory getItemCategory() {
return itemCategory;
}
public void setItemCategory(OrderLineItemCategory itemCategory) {
this.itemCategory = itemCategory;
}
public GetOrderLineItem itemName(String itemName) {
this.itemName = itemName;
return this;
}
/**
* The name of the Order Line Item.
* @return itemName
*/
@javax.annotation.Nullable
public String getItemName() {
return itemName;
}
public void setItemName(String itemName) {
this.itemName = itemName;
}
public GetOrderLineItem itemState(OrderLineItemState itemState) {
this.itemState = itemState;
return this;
}
/**
* Get itemState
* @return itemState
*/
@javax.annotation.Nullable
public OrderLineItemState getItemState() {
return itemState;
}
public void setItemState(OrderLineItemState itemState) {
this.itemState = itemState;
}
public GetOrderLineItem itemType(OrderLineItemType itemType) {
this.itemType = itemType;
return this;
}
/**
* Get itemType
* @return itemType
*/
@javax.annotation.Nullable
public OrderLineItemType getItemType() {
return itemType;
}
public void setItemType(OrderLineItemType itemType) {
this.itemType = itemType;
}
public GetOrderLineItem listPrice(BigDecimal listPrice) {
this.listPrice = listPrice;
return this;
}
/**
* The extended list price for an order line item, calculated by the formula: listPrice = listPricePerUnit * quantity
* @return listPrice
*/
@javax.annotation.Nullable
public BigDecimal getListPrice() {
return listPrice;
}
public void setListPrice(BigDecimal listPrice) {
this.listPrice = listPrice;
}
public GetOrderLineItem listPricePerUnit(BigDecimal listPricePerUnit) {
this.listPricePerUnit = listPricePerUnit;
return this;
}
/**
* The list price per unit for the Order Line Item.
* @return listPricePerUnit
*/
@javax.annotation.Nullable
public BigDecimal getListPricePerUnit() {
return listPricePerUnit;
}
public void setListPricePerUnit(BigDecimal listPricePerUnit) {
this.listPricePerUnit = listPricePerUnit;
}
public GetOrderLineItem originalOrderId(String originalOrderId) {
this.originalOrderId = originalOrderId;
return this;
}
/**
* The ID of the original sale order for a return order line item.
* @return originalOrderId
*/
@javax.annotation.Nullable
public String getOriginalOrderId() {
return originalOrderId;
}
public void setOriginalOrderId(String originalOrderId) {
this.originalOrderId = originalOrderId;
}
public GetOrderLineItem originalOrderDate(LocalDate originalOrderDate) {
this.originalOrderDate = originalOrderDate;
return this;
}
/**
* The date when the rate plan charge is created through an order or amendment. This field is to standardize the booking date information to increase audit ability and traceability of data between Zuora Billing and Zuora Revenue. It is mapped as the booking date for a sale order line in Zuora Revenue.
* @return originalOrderDate
*/
@javax.annotation.Nullable
public LocalDate getOriginalOrderDate() {
return originalOrderDate;
}
public void setOriginalOrderDate(LocalDate originalOrderDate) {
this.originalOrderDate = originalOrderDate;
}
public GetOrderLineItem originalOrderLineItemId(String originalOrderLineItemId) {
this.originalOrderLineItemId = originalOrderLineItemId;
return this;
}
/**
* The ID of the original sale order line item for a return order line item.
* @return originalOrderLineItemId
*/
@javax.annotation.Nullable
public String getOriginalOrderLineItemId() {
return originalOrderLineItemId;
}
public void setOriginalOrderLineItemId(String originalOrderLineItemId) {
this.originalOrderLineItemId = originalOrderLineItemId;
}
public GetOrderLineItem originalOrderLineItemNumber(String originalOrderLineItemNumber) {
this.originalOrderLineItemNumber = originalOrderLineItemNumber;
return this;
}
/**
* The number of the original sale order line item for a return order line item.
* @return originalOrderLineItemNumber
*/
@javax.annotation.Nullable
public String getOriginalOrderLineItemNumber() {
return originalOrderLineItemNumber;
}
public void setOriginalOrderLineItemNumber(String originalOrderLineItemNumber) {
this.originalOrderLineItemNumber = originalOrderLineItemNumber;
}
public GetOrderLineItem originalOrderNumber(String originalOrderNumber) {
this.originalOrderNumber = originalOrderNumber;
return this;
}
/**
* The number of the original sale order for a return order line item.
* @return originalOrderNumber
*/
@javax.annotation.Nullable
public String getOriginalOrderNumber() {
return originalOrderNumber;
}
public void setOriginalOrderNumber(String originalOrderNumber) {
this.originalOrderNumber = originalOrderNumber;
}
public GetOrderLineItem ownerAccountId(String ownerAccountId) {
this.ownerAccountId = ownerAccountId;
return this;
}
/**
* The account ID of the owner of the order line item.
* @return ownerAccountId
*/
@javax.annotation.Nullable
public String getOwnerAccountId() {
return ownerAccountId;
}
public void setOwnerAccountId(String ownerAccountId) {
this.ownerAccountId = ownerAccountId;
}
public GetOrderLineItem ownerAccountName(String ownerAccountName) {
this.ownerAccountName = ownerAccountName;
return this;
}
/**
* The account name of the owner of the order line item.
* @return ownerAccountName
*/
@javax.annotation.Nullable
public String getOwnerAccountName() {
return ownerAccountName;
}
public void setOwnerAccountName(String ownerAccountName) {
this.ownerAccountName = ownerAccountName;
}
public GetOrderLineItem ownerAccountNumber(String ownerAccountNumber) {
this.ownerAccountNumber = ownerAccountNumber;
return this;
}
/**
* The account number of the owner of the order line item.
* @return ownerAccountNumber
*/
@javax.annotation.Nullable
public String getOwnerAccountNumber() {
return ownerAccountNumber;
}
public void setOwnerAccountNumber(String ownerAccountNumber) {
this.ownerAccountNumber = ownerAccountNumber;
}
public GetOrderLineItem productCode(String productCode) {
this.productCode = productCode;
return this;
}
/**
* The product code for the Order Line Item.
* @return productCode
*/
@javax.annotation.Nullable
public String getProductCode() {
return productCode;
}
public void setProductCode(String productCode) {
this.productCode = productCode;
}
public GetOrderLineItem productRatePlanChargeId(String productRatePlanChargeId) {
this.productRatePlanChargeId = productRatePlanChargeId;
return this;
}
/**
* Id of a Product Rate Plan Charge. Only one-time charges are supported.
* @return productRatePlanChargeId
*/
@javax.annotation.Nullable
public String getProductRatePlanChargeId() {
return productRatePlanChargeId;
}
public void setProductRatePlanChargeId(String productRatePlanChargeId) {
this.productRatePlanChargeId = productRatePlanChargeId;
}
public GetOrderLineItem purchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
return this;
}
/**
* Used by customers to specify the Purchase Order Number provided by the buyer.
* @return purchaseOrderNumber
*/
@javax.annotation.Nullable
public String getPurchaseOrderNumber() {
return purchaseOrderNumber;
}
public void setPurchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
}
public GetOrderLineItem quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* The quantity of units, such as the number of authors in a hosted wiki service.
* @return quantity
*/
@javax.annotation.Nullable
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public GetOrderLineItem quantityAvailableForReturn(BigDecimal quantityAvailableForReturn) {
this.quantityAvailableForReturn = quantityAvailableForReturn;
return this;
}
/**
* The quantity that can be returned for an order line item.
* @return quantityAvailableForReturn
*/
@javax.annotation.Nullable
public BigDecimal getQuantityAvailableForReturn() {
return quantityAvailableForReturn;
}
public void setQuantityAvailableForReturn(BigDecimal quantityAvailableForReturn) {
this.quantityAvailableForReturn = quantityAvailableForReturn;
}
public GetOrderLineItem quantityFulfilled(BigDecimal quantityFulfilled) {
this.quantityFulfilled = quantityFulfilled;
return this;
}
/**
* The quantity that has been fulfilled by fulfillments for the order line item. This field will be updated automatically when related fulfillments become 'SentToBilling' or 'Complete' state.
* @return quantityFulfilled
*/
@javax.annotation.Nullable
public BigDecimal getQuantityFulfilled() {
return quantityFulfilled;
}
public void setQuantityFulfilled(BigDecimal quantityFulfilled) {
this.quantityFulfilled = quantityFulfilled;
}
public GetOrderLineItem quantityPendingFulfillment(BigDecimal quantityPendingFulfillment) {
this.quantityPendingFulfillment = quantityPendingFulfillment;
return this;
}
/**
* The quantity that's need to be fulfilled by fulfillments for the order line item. This field will be updated automatically when related fulfillments become 'SentToBilling' or 'Complete' state.
* @return quantityPendingFulfillment
*/
@javax.annotation.Nullable
public BigDecimal getQuantityPendingFulfillment() {
return quantityPendingFulfillment;
}
public void setQuantityPendingFulfillment(BigDecimal quantityPendingFulfillment) {
this.quantityPendingFulfillment = quantityPendingFulfillment;
}
public GetOrderLineItem recognizedRevenueAccountingCode(String recognizedRevenueAccountingCode) {
this.recognizedRevenueAccountingCode = recognizedRevenueAccountingCode;
return this;
}
/**
* The recognized revenue accounting code for the Order Line Item.
* @return recognizedRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getRecognizedRevenueAccountingCode() {
return recognizedRevenueAccountingCode;
}
public void setRecognizedRevenueAccountingCode(String recognizedRevenueAccountingCode) {
this.recognizedRevenueAccountingCode = recognizedRevenueAccountingCode;
}
public GetOrderLineItem relatedSubscriptionNumber(String relatedSubscriptionNumber) {
this.relatedSubscriptionNumber = relatedSubscriptionNumber;
return this;
}
/**
* Use this field to relate an order line item to a subscription when you create the order line item. * To relate an order line item to a new subscription which is yet to create in the same \"Create an order\" call, use this field in combination with the `subscriptions` > `subscriptionNumber` field in the \"Create order\" operation. Specify this field to the same value as that of the 'subscriptions' > `subscriptionNumber` field when you make the \"Create order\" call. * To relate an order line item to an existing subscription, specify this field to the subscription number of the existing subscription.
* @return relatedSubscriptionNumber
*/
@javax.annotation.Nullable
public String getRelatedSubscriptionNumber() {
return relatedSubscriptionNumber;
}
public void setRelatedSubscriptionNumber(String relatedSubscriptionNumber) {
this.relatedSubscriptionNumber = relatedSubscriptionNumber;
}
public GetOrderLineItem requiresFulfillment(Boolean requiresFulfillment) {
this.requiresFulfillment = requiresFulfillment;
return this;
}
/**
* The flag to show whether fulfillment is needed or not. It's derived from billing rule of the Order Line Item.
* @return requiresFulfillment
*/
@javax.annotation.Nullable
public Boolean getRequiresFulfillment() {
return requiresFulfillment;
}
public void setRequiresFulfillment(Boolean requiresFulfillment) {
this.requiresFulfillment = requiresFulfillment;
}
public GetOrderLineItem revenueRecognitionRule(String revenueRecognitionRule) {
this.revenueRecognitionRule = revenueRecognitionRule;
return this;
}
/**
* The Revenue Recognition rule for the Order Line Item.
* @return revenueRecognitionRule
*/
@javax.annotation.Nullable
public String getRevenueRecognitionRule() {
return revenueRecognitionRule;
}
public void setRevenueRecognitionRule(String revenueRecognitionRule) {
this.revenueRecognitionRule = revenueRecognitionRule;
}
public GetOrderLineItem sequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
return this;
}
/**
* The ID of the sequence set associated with the OrderLineItem.
* @return sequenceSetId
*/
@javax.annotation.Nullable
public String getSequenceSetId() {
return sequenceSetId;
}
public void setSequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
}
public GetOrderLineItem soldTo(String soldTo) {
this.soldTo = soldTo;
return this;
}
/**
* The ID of a contact that belongs to the owner acount or billing account of the order line item. Use this field to assign an existing account as the sold-to contact of an order line item.
* @return soldTo
*/
@javax.annotation.Nullable
public String getSoldTo() {
return soldTo;
}
public void setSoldTo(String soldTo) {
this.soldTo = soldTo;
}
public GetOrderLineItem soldToSnapshotId(String soldToSnapshotId) {
this.soldToSnapshotId = soldToSnapshotId;
return this;
}
/**
* The snapshot of the ID for an account used as the sold-to contact of an order line item. This field is used to store the original information about the account, in case the information about the account is changed after the creation of the order line item. The `soldToSnapshotId` field is exposed while retrieving the order line item details.
* @return soldToSnapshotId
*/
@javax.annotation.Nullable
public String getSoldToSnapshotId() {
return soldToSnapshotId;
}
public void setSoldToSnapshotId(String soldToSnapshotId) {
this.soldToSnapshotId = soldToSnapshotId;
}
public GetOrderLineItem shipTo(String shipTo) {
this.shipTo = shipTo;
return this;
}
/**
* The ID of a contact that belongs to the owner account or billing account of the order line item. Use this field to assign an existing account as the ship-to contact of an order line item.
* @return shipTo
*/
@javax.annotation.Nullable
public String getShipTo() {
return shipTo;
}
public void setShipTo(String shipTo) {
this.shipTo = shipTo;
}
public GetOrderLineItem shipToSnapshotId(String shipToSnapshotId) {
this.shipToSnapshotId = shipToSnapshotId;
return this;
}
/**
* The snapshot of the ID for an account used as the ship-to contact of an order line item. This field is used to store the original information about the account, in case the information about the account is changed after the creation of the order line item. The `shipToSnapshotId` field is exposed while retrieving the order line item details.
* @return shipToSnapshotId
*/
@javax.annotation.Nullable
public String getShipToSnapshotId() {
return shipToSnapshotId;
}
public void setShipToSnapshotId(String shipToSnapshotId) {
this.shipToSnapshotId = shipToSnapshotId;
}
public GetOrderLineItem taxCode(String taxCode) {
this.taxCode = taxCode;
return this;
}
/**
* The tax code for the Order Line Item.
* @return taxCode
*/
@javax.annotation.Nullable
public String getTaxCode() {
return taxCode;
}
public void setTaxCode(String taxCode) {
this.taxCode = taxCode;
}
public GetOrderLineItem taxMode(TaxMode taxMode) {
this.taxMode = taxMode;
return this;
}
/**
* Get taxMode
* @return taxMode
*/
@javax.annotation.Nullable
public TaxMode getTaxMode() {
return taxMode;
}
public void setTaxMode(TaxMode taxMode) {
this.taxMode = taxMode;
}
public GetOrderLineItem transactionEndDate(LocalDate transactionEndDate) {
this.transactionEndDate = transactionEndDate;
return this;
}
/**
* The date a transaction is completed. The default value of this field is the transaction start date. Also, the value of this field should always equal or be later than the value of the `transactionStartDate` field.
* @return transactionEndDate
*/
@javax.annotation.Nullable
public LocalDate getTransactionEndDate() {
return transactionEndDate;
}
public void setTransactionEndDate(LocalDate transactionEndDate) {
this.transactionEndDate = transactionEndDate;
}
public GetOrderLineItem transactionStartDate(LocalDate transactionStartDate) {
this.transactionStartDate = transactionStartDate;
return this;
}
/**
* The date a transaction starts. The default value of this field is the order date.
* @return transactionStartDate
*/
@javax.annotation.Nullable
public LocalDate getTransactionStartDate() {
return transactionStartDate;
}
public void setTransactionStartDate(LocalDate transactionStartDate) {
this.transactionStartDate = transactionStartDate;
}
public GetOrderLineItem unbilledReceivablesAccountingCode(String unbilledReceivablesAccountingCode) {
this.unbilledReceivablesAccountingCode = unbilledReceivablesAccountingCode;
return this;
}
/**
* The accounting code on the Order Line Item object for customers using [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration).
* @return unbilledReceivablesAccountingCode
*/
@javax.annotation.Nullable
public String getUnbilledReceivablesAccountingCode() {
return unbilledReceivablesAccountingCode;
}
public void setUnbilledReceivablesAccountingCode(String unbilledReceivablesAccountingCode) {
this.unbilledReceivablesAccountingCode = unbilledReceivablesAccountingCode;
}
public GetOrderLineItem fulfillments(List fulfillments) {
this.fulfillments = fulfillments;
return this;
}
public GetOrderLineItem addFulfillmentsItem(GetFulfillment fulfillmentsItem) {
if (this.fulfillments == null) {
this.fulfillments = new ArrayList<>();
}
this.fulfillments.add(fulfillmentsItem);
return this;
}
/**
* Container for the fulfillments attached to an order line item.
* @return fulfillments
*/
@javax.annotation.Nullable
public List getFulfillments() {
return fulfillments;
}
public void setFulfillments(List fulfillments) {
this.fulfillments = fulfillments;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the GetOrderLineItem instance itself
*/
public GetOrderLineItem putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GetOrderLineItem getOrderLineItem = (GetOrderLineItem) o;
return Objects.equals(this.id, getOrderLineItem.id) &&
Objects.equals(this.amendedByOrderOn, getOrderLineItem.amendedByOrderOn) &&
Objects.equals(this.currency, getOrderLineItem.currency) &&
Objects.equals(this.paymentTerm, getOrderLineItem.paymentTerm) &&
Objects.equals(this.invoiceTemplateId, getOrderLineItem.invoiceTemplateId) &&
Objects.equals(this.amount, getOrderLineItem.amount) &&
Objects.equals(this.amountWithoutTax, getOrderLineItem.amountWithoutTax) &&
Objects.equals(this.itemNumber, getOrderLineItem.itemNumber) &&
Objects.equals(this.UOM, getOrderLineItem.UOM) &&
Objects.equals(this.amountPerUnit, getOrderLineItem.amountPerUnit) &&
Objects.equals(this.billTargetDate, getOrderLineItem.billTargetDate) &&
Objects.equals(this.billTo, getOrderLineItem.billTo) &&
Objects.equals(this.billToSnapshotId, getOrderLineItem.billToSnapshotId) &&
Objects.equals(this.billingRule, getOrderLineItem.billingRule) &&
Objects.equals(this.accountingCode, getOrderLineItem.accountingCode) &&
Objects.equals(this.adjustmentLiabilityAccountingCode, getOrderLineItem.adjustmentLiabilityAccountingCode) &&
Objects.equals(this.adjustmentRevenueAccountingCode, getOrderLineItem.adjustmentRevenueAccountingCode) &&
Objects.equals(this.contractAssetAccountingCode, getOrderLineItem.contractAssetAccountingCode) &&
Objects.equals(this.contractLiabilityAccountingCode, getOrderLineItem.contractLiabilityAccountingCode) &&
Objects.equals(this.contractRecognizedRevenueAccountingCode, getOrderLineItem.contractRecognizedRevenueAccountingCode) &&
Objects.equals(this.deferredRevenueAccountingCode, getOrderLineItem.deferredRevenueAccountingCode) &&
Objects.equals(this.customFields, getOrderLineItem.customFields) &&
Objects.equals(this.description, getOrderLineItem.description) &&
Objects.equals(this.discount, getOrderLineItem.discount) &&
Objects.equals(this.excludeItemBillingFromRevenueAccounting, getOrderLineItem.excludeItemBillingFromRevenueAccounting) &&
Objects.equals(this.excludeItemBookingFromRevenueAccounting, getOrderLineItem.excludeItemBookingFromRevenueAccounting) &&
Objects.equals(this.revenueRecognitionTiming, getOrderLineItem.revenueRecognitionTiming) &&
Objects.equals(this.revenueAmortizationMethod, getOrderLineItem.revenueAmortizationMethod) &&
Objects.equals(this.inlineDiscountPerUnit, getOrderLineItem.inlineDiscountPerUnit) &&
Objects.equals(this.inlineDiscountType, getOrderLineItem.inlineDiscountType) &&
Objects.equals(this.invoiceGroupNumber, getOrderLineItem.invoiceGroupNumber) &&
Objects.equals(this.invoiceOwnerAccountId, getOrderLineItem.invoiceOwnerAccountId) &&
Objects.equals(this.invoiceOwnerAccountName, getOrderLineItem.invoiceOwnerAccountName) &&
Objects.equals(this.invoiceOwnerAccountNumber, getOrderLineItem.invoiceOwnerAccountNumber) &&
Objects.equals(this.isAllocationEligible, getOrderLineItem.isAllocationEligible) &&
Objects.equals(this.isUnbilled, getOrderLineItem.isUnbilled) &&
Objects.equals(this.itemCategory, getOrderLineItem.itemCategory) &&
Objects.equals(this.itemName, getOrderLineItem.itemName) &&
Objects.equals(this.itemState, getOrderLineItem.itemState) &&
Objects.equals(this.itemType, getOrderLineItem.itemType) &&
Objects.equals(this.listPrice, getOrderLineItem.listPrice) &&
Objects.equals(this.listPricePerUnit, getOrderLineItem.listPricePerUnit) &&
Objects.equals(this.originalOrderId, getOrderLineItem.originalOrderId) &&
Objects.equals(this.originalOrderDate, getOrderLineItem.originalOrderDate) &&
Objects.equals(this.originalOrderLineItemId, getOrderLineItem.originalOrderLineItemId) &&
Objects.equals(this.originalOrderLineItemNumber, getOrderLineItem.originalOrderLineItemNumber) &&
Objects.equals(this.originalOrderNumber, getOrderLineItem.originalOrderNumber) &&
Objects.equals(this.ownerAccountId, getOrderLineItem.ownerAccountId) &&
Objects.equals(this.ownerAccountName, getOrderLineItem.ownerAccountName) &&
Objects.equals(this.ownerAccountNumber, getOrderLineItem.ownerAccountNumber) &&
Objects.equals(this.productCode, getOrderLineItem.productCode) &&
Objects.equals(this.productRatePlanChargeId, getOrderLineItem.productRatePlanChargeId) &&
Objects.equals(this.purchaseOrderNumber, getOrderLineItem.purchaseOrderNumber) &&
Objects.equals(this.quantity, getOrderLineItem.quantity) &&
Objects.equals(this.quantityAvailableForReturn, getOrderLineItem.quantityAvailableForReturn) &&
Objects.equals(this.quantityFulfilled, getOrderLineItem.quantityFulfilled) &&
Objects.equals(this.quantityPendingFulfillment, getOrderLineItem.quantityPendingFulfillment) &&
Objects.equals(this.recognizedRevenueAccountingCode, getOrderLineItem.recognizedRevenueAccountingCode) &&
Objects.equals(this.relatedSubscriptionNumber, getOrderLineItem.relatedSubscriptionNumber) &&
Objects.equals(this.requiresFulfillment, getOrderLineItem.requiresFulfillment) &&
Objects.equals(this.revenueRecognitionRule, getOrderLineItem.revenueRecognitionRule) &&
Objects.equals(this.sequenceSetId, getOrderLineItem.sequenceSetId) &&
Objects.equals(this.soldTo, getOrderLineItem.soldTo) &&
Objects.equals(this.soldToSnapshotId, getOrderLineItem.soldToSnapshotId) &&
Objects.equals(this.shipTo, getOrderLineItem.shipTo) &&
Objects.equals(this.shipToSnapshotId, getOrderLineItem.shipToSnapshotId) &&
Objects.equals(this.taxCode, getOrderLineItem.taxCode) &&
Objects.equals(this.taxMode, getOrderLineItem.taxMode) &&
Objects.equals(this.transactionEndDate, getOrderLineItem.transactionEndDate) &&
Objects.equals(this.transactionStartDate, getOrderLineItem.transactionStartDate) &&
Objects.equals(this.unbilledReceivablesAccountingCode, getOrderLineItem.unbilledReceivablesAccountingCode) &&
Objects.equals(this.fulfillments, getOrderLineItem.fulfillments)&&
Objects.equals(this.additionalProperties, getOrderLineItem.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(id, amendedByOrderOn, currency, paymentTerm, invoiceTemplateId, amount, amountWithoutTax, itemNumber, UOM, amountPerUnit, billTargetDate, billTo, billToSnapshotId, billingRule, accountingCode, adjustmentLiabilityAccountingCode, adjustmentRevenueAccountingCode, contractAssetAccountingCode, contractLiabilityAccountingCode, contractRecognizedRevenueAccountingCode, deferredRevenueAccountingCode, customFields, description, discount, excludeItemBillingFromRevenueAccounting, excludeItemBookingFromRevenueAccounting, revenueRecognitionTiming, revenueAmortizationMethod, inlineDiscountPerUnit, inlineDiscountType, invoiceGroupNumber, invoiceOwnerAccountId, invoiceOwnerAccountName, invoiceOwnerAccountNumber, isAllocationEligible, isUnbilled, itemCategory, itemName, itemState, itemType, listPrice, listPricePerUnit, originalOrderId, originalOrderDate, originalOrderLineItemId, originalOrderLineItemNumber, originalOrderNumber, ownerAccountId, ownerAccountName, ownerAccountNumber, productCode, productRatePlanChargeId, purchaseOrderNumber, quantity, quantityAvailableForReturn, quantityFulfilled, quantityPendingFulfillment, recognizedRevenueAccountingCode, relatedSubscriptionNumber, requiresFulfillment, revenueRecognitionRule, sequenceSetId, soldTo, soldToSnapshotId, shipTo, shipToSnapshotId, taxCode, taxMode, transactionEndDate, transactionStartDate, unbilledReceivablesAccountingCode, fulfillments, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GetOrderLineItem {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" amendedByOrderOn: ").append(toIndentedString(amendedByOrderOn)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" paymentTerm: ").append(toIndentedString(paymentTerm)).append("\n");
sb.append(" invoiceTemplateId: ").append(toIndentedString(invoiceTemplateId)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" amountWithoutTax: ").append(toIndentedString(amountWithoutTax)).append("\n");
sb.append(" itemNumber: ").append(toIndentedString(itemNumber)).append("\n");
sb.append(" UOM: ").append(toIndentedString(UOM)).append("\n");
sb.append(" amountPerUnit: ").append(toIndentedString(amountPerUnit)).append("\n");
sb.append(" billTargetDate: ").append(toIndentedString(billTargetDate)).append("\n");
sb.append(" billTo: ").append(toIndentedString(billTo)).append("\n");
sb.append(" billToSnapshotId: ").append(toIndentedString(billToSnapshotId)).append("\n");
sb.append(" billingRule: ").append(toIndentedString(billingRule)).append("\n");
sb.append(" accountingCode: ").append(toIndentedString(accountingCode)).append("\n");
sb.append(" adjustmentLiabilityAccountingCode: ").append(toIndentedString(adjustmentLiabilityAccountingCode)).append("\n");
sb.append(" adjustmentRevenueAccountingCode: ").append(toIndentedString(adjustmentRevenueAccountingCode)).append("\n");
sb.append(" contractAssetAccountingCode: ").append(toIndentedString(contractAssetAccountingCode)).append("\n");
sb.append(" contractLiabilityAccountingCode: ").append(toIndentedString(contractLiabilityAccountingCode)).append("\n");
sb.append(" contractRecognizedRevenueAccountingCode: ").append(toIndentedString(contractRecognizedRevenueAccountingCode)).append("\n");
sb.append(" deferredRevenueAccountingCode: ").append(toIndentedString(deferredRevenueAccountingCode)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" discount: ").append(toIndentedString(discount)).append("\n");
sb.append(" excludeItemBillingFromRevenueAccounting: ").append(toIndentedString(excludeItemBillingFromRevenueAccounting)).append("\n");
sb.append(" excludeItemBookingFromRevenueAccounting: ").append(toIndentedString(excludeItemBookingFromRevenueAccounting)).append("\n");
sb.append(" revenueRecognitionTiming: ").append(toIndentedString(revenueRecognitionTiming)).append("\n");
sb.append(" revenueAmortizationMethod: ").append(toIndentedString(revenueAmortizationMethod)).append("\n");
sb.append(" inlineDiscountPerUnit: ").append(toIndentedString(inlineDiscountPerUnit)).append("\n");
sb.append(" inlineDiscountType: ").append(toIndentedString(inlineDiscountType)).append("\n");
sb.append(" invoiceGroupNumber: ").append(toIndentedString(invoiceGroupNumber)).append("\n");
sb.append(" invoiceOwnerAccountId: ").append(toIndentedString(invoiceOwnerAccountId)).append("\n");
sb.append(" invoiceOwnerAccountName: ").append(toIndentedString(invoiceOwnerAccountName)).append("\n");
sb.append(" invoiceOwnerAccountNumber: ").append(toIndentedString(invoiceOwnerAccountNumber)).append("\n");
sb.append(" isAllocationEligible: ").append(toIndentedString(isAllocationEligible)).append("\n");
sb.append(" isUnbilled: ").append(toIndentedString(isUnbilled)).append("\n");
sb.append(" itemCategory: ").append(toIndentedString(itemCategory)).append("\n");
sb.append(" itemName: ").append(toIndentedString(itemName)).append("\n");
sb.append(" itemState: ").append(toIndentedString(itemState)).append("\n");
sb.append(" itemType: ").append(toIndentedString(itemType)).append("\n");
sb.append(" listPrice: ").append(toIndentedString(listPrice)).append("\n");
sb.append(" listPricePerUnit: ").append(toIndentedString(listPricePerUnit)).append("\n");
sb.append(" originalOrderId: ").append(toIndentedString(originalOrderId)).append("\n");
sb.append(" originalOrderDate: ").append(toIndentedString(originalOrderDate)).append("\n");
sb.append(" originalOrderLineItemId: ").append(toIndentedString(originalOrderLineItemId)).append("\n");
sb.append(" originalOrderLineItemNumber: ").append(toIndentedString(originalOrderLineItemNumber)).append("\n");
sb.append(" originalOrderNumber: ").append(toIndentedString(originalOrderNumber)).append("\n");
sb.append(" ownerAccountId: ").append(toIndentedString(ownerAccountId)).append("\n");
sb.append(" ownerAccountName: ").append(toIndentedString(ownerAccountName)).append("\n");
sb.append(" ownerAccountNumber: ").append(toIndentedString(ownerAccountNumber)).append("\n");
sb.append(" productCode: ").append(toIndentedString(productCode)).append("\n");
sb.append(" productRatePlanChargeId: ").append(toIndentedString(productRatePlanChargeId)).append("\n");
sb.append(" purchaseOrderNumber: ").append(toIndentedString(purchaseOrderNumber)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" quantityAvailableForReturn: ").append(toIndentedString(quantityAvailableForReturn)).append("\n");
sb.append(" quantityFulfilled: ").append(toIndentedString(quantityFulfilled)).append("\n");
sb.append(" quantityPendingFulfillment: ").append(toIndentedString(quantityPendingFulfillment)).append("\n");
sb.append(" recognizedRevenueAccountingCode: ").append(toIndentedString(recognizedRevenueAccountingCode)).append("\n");
sb.append(" relatedSubscriptionNumber: ").append(toIndentedString(relatedSubscriptionNumber)).append("\n");
sb.append(" requiresFulfillment: ").append(toIndentedString(requiresFulfillment)).append("\n");
sb.append(" revenueRecognitionRule: ").append(toIndentedString(revenueRecognitionRule)).append("\n");
sb.append(" sequenceSetId: ").append(toIndentedString(sequenceSetId)).append("\n");
sb.append(" soldTo: ").append(toIndentedString(soldTo)).append("\n");
sb.append(" soldToSnapshotId: ").append(toIndentedString(soldToSnapshotId)).append("\n");
sb.append(" shipTo: ").append(toIndentedString(shipTo)).append("\n");
sb.append(" shipToSnapshotId: ").append(toIndentedString(shipToSnapshotId)).append("\n");
sb.append(" taxCode: ").append(toIndentedString(taxCode)).append("\n");
sb.append(" taxMode: ").append(toIndentedString(taxMode)).append("\n");
sb.append(" transactionEndDate: ").append(toIndentedString(transactionEndDate)).append("\n");
sb.append(" transactionStartDate: ").append(toIndentedString(transactionStartDate)).append("\n");
sb.append(" unbilledReceivablesAccountingCode: ").append(toIndentedString(unbilledReceivablesAccountingCode)).append("\n");
sb.append(" fulfillments: ").append(toIndentedString(fulfillments)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("id");
openapiFields.add("amendedByOrderOn");
openapiFields.add("currency");
openapiFields.add("paymentTerm");
openapiFields.add("invoiceTemplateId");
openapiFields.add("amount");
openapiFields.add("amountWithoutTax");
openapiFields.add("itemNumber");
openapiFields.add("UOM");
openapiFields.add("amountPerUnit");
openapiFields.add("billTargetDate");
openapiFields.add("billTo");
openapiFields.add("billToSnapshotId");
openapiFields.add("billingRule");
openapiFields.add("accountingCode");
openapiFields.add("adjustmentLiabilityAccountingCode");
openapiFields.add("adjustmentRevenueAccountingCode");
openapiFields.add("contractAssetAccountingCode");
openapiFields.add("contractLiabilityAccountingCode");
openapiFields.add("contractRecognizedRevenueAccountingCode");
openapiFields.add("deferredRevenueAccountingCode");
openapiFields.add("customFields");
openapiFields.add("description");
openapiFields.add("discount");
openapiFields.add("excludeItemBillingFromRevenueAccounting");
openapiFields.add("excludeItemBookingFromRevenueAccounting");
openapiFields.add("revenueRecognitionTiming");
openapiFields.add("revenueAmortizationMethod");
openapiFields.add("inlineDiscountPerUnit");
openapiFields.add("inlineDiscountType");
openapiFields.add("invoiceGroupNumber");
openapiFields.add("invoiceOwnerAccountId");
openapiFields.add("invoiceOwnerAccountName");
openapiFields.add("invoiceOwnerAccountNumber");
openapiFields.add("isAllocationEligible");
openapiFields.add("isUnbilled");
openapiFields.add("itemCategory");
openapiFields.add("itemName");
openapiFields.add("itemState");
openapiFields.add("itemType");
openapiFields.add("listPrice");
openapiFields.add("listPricePerUnit");
openapiFields.add("originalOrderId");
openapiFields.add("originalOrderDate");
openapiFields.add("originalOrderLineItemId");
openapiFields.add("originalOrderLineItemNumber");
openapiFields.add("originalOrderNumber");
openapiFields.add("ownerAccountId");
openapiFields.add("ownerAccountName");
openapiFields.add("ownerAccountNumber");
openapiFields.add("productCode");
openapiFields.add("productRatePlanChargeId");
openapiFields.add("purchaseOrderNumber");
openapiFields.add("quantity");
openapiFields.add("quantityAvailableForReturn");
openapiFields.add("quantityFulfilled");
openapiFields.add("quantityPendingFulfillment");
openapiFields.add("recognizedRevenueAccountingCode");
openapiFields.add("relatedSubscriptionNumber");
openapiFields.add("requiresFulfillment");
openapiFields.add("revenueRecognitionRule");
openapiFields.add("sequenceSetId");
openapiFields.add("soldTo");
openapiFields.add("soldToSnapshotId");
openapiFields.add("shipTo");
openapiFields.add("shipToSnapshotId");
openapiFields.add("taxCode");
openapiFields.add("taxMode");
openapiFields.add("transactionEndDate");
openapiFields.add("transactionStartDate");
openapiFields.add("unbilledReceivablesAccountingCode");
openapiFields.add("fulfillments");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to GetOrderLineItem
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!GetOrderLineItem.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in GetOrderLineItem is not found in the empty JSON string", GetOrderLineItem.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("amendedByOrderOn") != null && !jsonObj.get("amendedByOrderOn").isJsonNull()) && !jsonObj.get("amendedByOrderOn").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `amendedByOrderOn` to be a primitive type in the JSON string but got `%s`", jsonObj.get("amendedByOrderOn").toString()));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("paymentTerm") != null && !jsonObj.get("paymentTerm").isJsonNull()) && !jsonObj.get("paymentTerm").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentTerm` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentTerm").toString()));
}
if ((jsonObj.get("invoiceTemplateId") != null && !jsonObj.get("invoiceTemplateId").isJsonNull()) && !jsonObj.get("invoiceTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceTemplateId").toString()));
}
if ((jsonObj.get("itemNumber") != null && !jsonObj.get("itemNumber").isJsonNull()) && !jsonObj.get("itemNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `itemNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("itemNumber").toString()));
}
if ((jsonObj.get("UOM") != null && !jsonObj.get("UOM").isJsonNull()) && !jsonObj.get("UOM").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `UOM` to be a primitive type in the JSON string but got `%s`", jsonObj.get("UOM").toString()));
}
if ((jsonObj.get("billTo") != null && !jsonObj.get("billTo").isJsonNull()) && !jsonObj.get("billTo").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billTo` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billTo").toString()));
}
if ((jsonObj.get("billToSnapshotId") != null && !jsonObj.get("billToSnapshotId").isJsonNull()) && !jsonObj.get("billToSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billToSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billToSnapshotId").toString()));
}
// validate the optional field `billingRule`
if (jsonObj.get("billingRule") != null && !jsonObj.get("billingRule").isJsonNull()) {
OrderLineItemBillingRule.validateJsonElement(jsonObj.get("billingRule"));
}
if ((jsonObj.get("accountingCode") != null && !jsonObj.get("accountingCode").isJsonNull()) && !jsonObj.get("accountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountingCode").toString()));
}
if ((jsonObj.get("adjustmentLiabilityAccountingCode") != null && !jsonObj.get("adjustmentLiabilityAccountingCode").isJsonNull()) && !jsonObj.get("adjustmentLiabilityAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `adjustmentLiabilityAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("adjustmentLiabilityAccountingCode").toString()));
}
if ((jsonObj.get("adjustmentRevenueAccountingCode") != null && !jsonObj.get("adjustmentRevenueAccountingCode").isJsonNull()) && !jsonObj.get("adjustmentRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `adjustmentRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("adjustmentRevenueAccountingCode").toString()));
}
if ((jsonObj.get("contractAssetAccountingCode") != null && !jsonObj.get("contractAssetAccountingCode").isJsonNull()) && !jsonObj.get("contractAssetAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractAssetAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractAssetAccountingCode").toString()));
}
if ((jsonObj.get("contractLiabilityAccountingCode") != null && !jsonObj.get("contractLiabilityAccountingCode").isJsonNull()) && !jsonObj.get("contractLiabilityAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractLiabilityAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractLiabilityAccountingCode").toString()));
}
if ((jsonObj.get("contractRecognizedRevenueAccountingCode") != null && !jsonObj.get("contractRecognizedRevenueAccountingCode").isJsonNull()) && !jsonObj.get("contractRecognizedRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractRecognizedRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractRecognizedRevenueAccountingCode").toString()));
}
if ((jsonObj.get("deferredRevenueAccountingCode") != null && !jsonObj.get("deferredRevenueAccountingCode").isJsonNull()) && !jsonObj.get("deferredRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `deferredRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("deferredRevenueAccountingCode").toString()));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if ((jsonObj.get("revenueRecognitionTiming") != null && !jsonObj.get("revenueRecognitionTiming").isJsonNull()) && !jsonObj.get("revenueRecognitionTiming").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueRecognitionTiming` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueRecognitionTiming").toString()));
}
if ((jsonObj.get("revenueAmortizationMethod") != null && !jsonObj.get("revenueAmortizationMethod").isJsonNull()) && !jsonObj.get("revenueAmortizationMethod").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueAmortizationMethod` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueAmortizationMethod").toString()));
}
if ((jsonObj.get("inlineDiscountType") != null && !jsonObj.get("inlineDiscountType").isJsonNull()) && !jsonObj.get("inlineDiscountType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `inlineDiscountType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("inlineDiscountType").toString()));
}
// validate the optional field `inlineDiscountType`
if (jsonObj.get("inlineDiscountType") != null && !jsonObj.get("inlineDiscountType").isJsonNull()) {
OrderLineItemInlineDiscountType.validateJsonElement(jsonObj.get("inlineDiscountType"));
}
if ((jsonObj.get("invoiceGroupNumber") != null && !jsonObj.get("invoiceGroupNumber").isJsonNull()) && !jsonObj.get("invoiceGroupNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceGroupNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceGroupNumber").toString()));
}
if ((jsonObj.get("invoiceOwnerAccountId") != null && !jsonObj.get("invoiceOwnerAccountId").isJsonNull()) && !jsonObj.get("invoiceOwnerAccountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceOwnerAccountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceOwnerAccountId").toString()));
}
if ((jsonObj.get("invoiceOwnerAccountName") != null && !jsonObj.get("invoiceOwnerAccountName").isJsonNull()) && !jsonObj.get("invoiceOwnerAccountName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceOwnerAccountName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceOwnerAccountName").toString()));
}
if ((jsonObj.get("invoiceOwnerAccountNumber") != null && !jsonObj.get("invoiceOwnerAccountNumber").isJsonNull()) && !jsonObj.get("invoiceOwnerAccountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceOwnerAccountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceOwnerAccountNumber").toString()));
}
// validate the optional field `itemCategory`
if (jsonObj.get("itemCategory") != null && !jsonObj.get("itemCategory").isJsonNull()) {
OrderLineItemCategory.validateJsonElement(jsonObj.get("itemCategory"));
}
if ((jsonObj.get("itemName") != null && !jsonObj.get("itemName").isJsonNull()) && !jsonObj.get("itemName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `itemName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("itemName").toString()));
}
// validate the optional field `itemState`
if (jsonObj.get("itemState") != null && !jsonObj.get("itemState").isJsonNull()) {
OrderLineItemState.validateJsonElement(jsonObj.get("itemState"));
}
// validate the optional field `itemType`
if (jsonObj.get("itemType") != null && !jsonObj.get("itemType").isJsonNull()) {
OrderLineItemType.validateJsonElement(jsonObj.get("itemType"));
}
if ((jsonObj.get("originalOrderId") != null && !jsonObj.get("originalOrderId").isJsonNull()) && !jsonObj.get("originalOrderId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `originalOrderId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("originalOrderId").toString()));
}
if ((jsonObj.get("originalOrderLineItemId") != null && !jsonObj.get("originalOrderLineItemId").isJsonNull()) && !jsonObj.get("originalOrderLineItemId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `originalOrderLineItemId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("originalOrderLineItemId").toString()));
}
if ((jsonObj.get("originalOrderLineItemNumber") != null && !jsonObj.get("originalOrderLineItemNumber").isJsonNull()) && !jsonObj.get("originalOrderLineItemNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `originalOrderLineItemNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("originalOrderLineItemNumber").toString()));
}
if ((jsonObj.get("originalOrderNumber") != null && !jsonObj.get("originalOrderNumber").isJsonNull()) && !jsonObj.get("originalOrderNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `originalOrderNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("originalOrderNumber").toString()));
}
if ((jsonObj.get("ownerAccountId") != null && !jsonObj.get("ownerAccountId").isJsonNull()) && !jsonObj.get("ownerAccountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ownerAccountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ownerAccountId").toString()));
}
if ((jsonObj.get("ownerAccountName") != null && !jsonObj.get("ownerAccountName").isJsonNull()) && !jsonObj.get("ownerAccountName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ownerAccountName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ownerAccountName").toString()));
}
if ((jsonObj.get("ownerAccountNumber") != null && !jsonObj.get("ownerAccountNumber").isJsonNull()) && !jsonObj.get("ownerAccountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ownerAccountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ownerAccountNumber").toString()));
}
if ((jsonObj.get("productCode") != null && !jsonObj.get("productCode").isJsonNull()) && !jsonObj.get("productCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productCode").toString()));
}
if ((jsonObj.get("productRatePlanChargeId") != null && !jsonObj.get("productRatePlanChargeId").isJsonNull()) && !jsonObj.get("productRatePlanChargeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanChargeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productRatePlanChargeId").toString()));
}
if ((jsonObj.get("purchaseOrderNumber") != null && !jsonObj.get("purchaseOrderNumber").isJsonNull()) && !jsonObj.get("purchaseOrderNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `purchaseOrderNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("purchaseOrderNumber").toString()));
}
if ((jsonObj.get("recognizedRevenueAccountingCode") != null && !jsonObj.get("recognizedRevenueAccountingCode").isJsonNull()) && !jsonObj.get("recognizedRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `recognizedRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("recognizedRevenueAccountingCode").toString()));
}
if ((jsonObj.get("relatedSubscriptionNumber") != null && !jsonObj.get("relatedSubscriptionNumber").isJsonNull()) && !jsonObj.get("relatedSubscriptionNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `relatedSubscriptionNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("relatedSubscriptionNumber").toString()));
}
if ((jsonObj.get("revenueRecognitionRule") != null && !jsonObj.get("revenueRecognitionRule").isJsonNull()) && !jsonObj.get("revenueRecognitionRule").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueRecognitionRule` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueRecognitionRule").toString()));
}
if ((jsonObj.get("sequenceSetId") != null && !jsonObj.get("sequenceSetId").isJsonNull()) && !jsonObj.get("sequenceSetId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sequenceSetId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sequenceSetId").toString()));
}
if ((jsonObj.get("soldTo") != null && !jsonObj.get("soldTo").isJsonNull()) && !jsonObj.get("soldTo").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldTo` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldTo").toString()));
}
if ((jsonObj.get("soldToSnapshotId") != null && !jsonObj.get("soldToSnapshotId").isJsonNull()) && !jsonObj.get("soldToSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToSnapshotId").toString()));
}
if ((jsonObj.get("shipTo") != null && !jsonObj.get("shipTo").isJsonNull()) && !jsonObj.get("shipTo").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `shipTo` to be a primitive type in the JSON string but got `%s`", jsonObj.get("shipTo").toString()));
}
if ((jsonObj.get("shipToSnapshotId") != null && !jsonObj.get("shipToSnapshotId").isJsonNull()) && !jsonObj.get("shipToSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `shipToSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("shipToSnapshotId").toString()));
}
if ((jsonObj.get("taxCode") != null && !jsonObj.get("taxCode").isJsonNull()) && !jsonObj.get("taxCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxCode").toString()));
}
// validate the optional field `taxMode`
if (jsonObj.get("taxMode") != null && !jsonObj.get("taxMode").isJsonNull()) {
TaxMode.validateJsonElement(jsonObj.get("taxMode"));
}
if ((jsonObj.get("unbilledReceivablesAccountingCode") != null && !jsonObj.get("unbilledReceivablesAccountingCode").isJsonNull()) && !jsonObj.get("unbilledReceivablesAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `unbilledReceivablesAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("unbilledReceivablesAccountingCode").toString()));
}
if (jsonObj.get("fulfillments") != null && !jsonObj.get("fulfillments").isJsonNull()) {
JsonArray jsonArrayfulfillments = jsonObj.getAsJsonArray("fulfillments");
if (jsonArrayfulfillments != null) {
// ensure the json data is an array
if (!jsonObj.get("fulfillments").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `fulfillments` to be an array in the JSON string but got `%s`", jsonObj.get("fulfillments").toString()));
}
// validate the optional field `fulfillments` (array)
for (int i = 0; i < jsonArrayfulfillments.size(); i++) {
GetFulfillment.validateJsonElement(jsonArrayfulfillments.get(i));
};
}
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!GetOrderLineItem.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'GetOrderLineItem' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(GetOrderLineItem.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, GetOrderLineItem value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public GetOrderLineItem read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
GetOrderLineItem instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of GetOrderLineItem given an JSON string
*
* @param jsonString JSON string
* @return An instance of GetOrderLineItem
* @throws IOException if the JSON string is invalid with respect to GetOrderLineItem
*/
public static GetOrderLineItem fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, GetOrderLineItem.class);
}
/**
* Convert an instance of GetOrderLineItem to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy