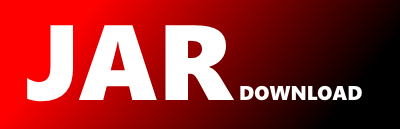
com.zuora.model.GetPaymentMethodForAccountResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.AccountPMMandateInfo;
import com.zuora.model.GetAccountPMAccountHolderInfo;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* GetPaymentMethodForAccountResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class GetPaymentMethodForAccountResponse {
public static final String SERIALIZED_NAME_I_B_A_N = "IBAN";
@SerializedName(SERIALIZED_NAME_I_B_A_N)
private String IBAN;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "accountNumber";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_BANK_CODE = "bankCode";
@SerializedName(SERIALIZED_NAME_BANK_CODE)
private String bankCode;
public static final String SERIALIZED_NAME_BANK_TRANSFER_TYPE = "bankTransferType";
@SerializedName(SERIALIZED_NAME_BANK_TRANSFER_TYPE)
private String bankTransferType;
public static final String SERIALIZED_NAME_BRANCH_CODE = "branchCode";
@SerializedName(SERIALIZED_NAME_BRANCH_CODE)
private String branchCode;
public static final String SERIALIZED_NAME_BUSINESS_IDENTIFICATION_CODE = "businessIdentificationCode";
@SerializedName(SERIALIZED_NAME_BUSINESS_IDENTIFICATION_CODE)
private String businessIdentificationCode;
public static final String SERIALIZED_NAME_IDENTITY_NUMBER = "identityNumber";
@SerializedName(SERIALIZED_NAME_IDENTITY_NUMBER)
private String identityNumber;
public static final String SERIALIZED_NAME_BANK_A_B_A_CODE = "bankABACode";
@SerializedName(SERIALIZED_NAME_BANK_A_B_A_CODE)
private String bankABACode;
public static final String SERIALIZED_NAME_BANK_ACCOUNT_NAME = "bankAccountName";
@SerializedName(SERIALIZED_NAME_BANK_ACCOUNT_NAME)
private String bankAccountName;
public static final String SERIALIZED_NAME_CARD_NUMBER = "cardNumber";
@SerializedName(SERIALIZED_NAME_CARD_NUMBER)
private String cardNumber;
public static final String SERIALIZED_NAME_EXPIRATION_MONTH = "expirationMonth";
@SerializedName(SERIALIZED_NAME_EXPIRATION_MONTH)
private Integer expirationMonth;
public static final String SERIALIZED_NAME_EXPIRATION_YEAR = "expirationYear";
@SerializedName(SERIALIZED_NAME_EXPIRATION_YEAR)
private Integer expirationYear;
public static final String SERIALIZED_NAME_SECURITY_CODE = "securityCode";
@SerializedName(SERIALIZED_NAME_SECURITY_CODE)
private String securityCode;
public static final String SERIALIZED_NAME_B_A_I_D = "BAID";
@SerializedName(SERIALIZED_NAME_B_A_I_D)
private String BAID;
public static final String SERIALIZED_NAME_EMAIL = "email";
@SerializedName(SERIALIZED_NAME_EMAIL)
private String email;
public static final String SERIALIZED_NAME_PREAPPROVAL_KEY = "preapprovalKey";
@SerializedName(SERIALIZED_NAME_PREAPPROVAL_KEY)
private String preapprovalKey;
public static final String SERIALIZED_NAME_GOOGLE_B_I_N = "googleBIN";
@SerializedName(SERIALIZED_NAME_GOOGLE_B_I_N)
private String googleBIN;
public static final String SERIALIZED_NAME_GOOGLE_CARD_NUMBER = "googleCardNumber";
@SerializedName(SERIALIZED_NAME_GOOGLE_CARD_NUMBER)
private String googleCardNumber;
public static final String SERIALIZED_NAME_GOOGLE_CARD_TYPE = "googleCardType";
@SerializedName(SERIALIZED_NAME_GOOGLE_CARD_TYPE)
private String googleCardType;
public static final String SERIALIZED_NAME_GOOGLE_EXPIRY_DATE = "googleExpiryDate";
@SerializedName(SERIALIZED_NAME_GOOGLE_EXPIRY_DATE)
private String googleExpiryDate;
public static final String SERIALIZED_NAME_GOOGLE_GATEWAY_TOKEN = "googleGatewayToken";
@SerializedName(SERIALIZED_NAME_GOOGLE_GATEWAY_TOKEN)
private String googleGatewayToken;
public static final String SERIALIZED_NAME_APPLE_B_I_N = "appleBIN";
@SerializedName(SERIALIZED_NAME_APPLE_B_I_N)
private String appleBIN;
public static final String SERIALIZED_NAME_APPLE_CARD_NUMBER = "appleCardNumber";
@SerializedName(SERIALIZED_NAME_APPLE_CARD_NUMBER)
private String appleCardNumber;
public static final String SERIALIZED_NAME_APPLE_CARD_TYPE = "appleCardType";
@SerializedName(SERIALIZED_NAME_APPLE_CARD_TYPE)
private String appleCardType;
public static final String SERIALIZED_NAME_APPLE_EXPIRY_DATE = "appleExpiryDate";
@SerializedName(SERIALIZED_NAME_APPLE_EXPIRY_DATE)
private String appleExpiryDate;
public static final String SERIALIZED_NAME_APPLE_GATEWAY_TOKEN = "appleGatewayToken";
@SerializedName(SERIALIZED_NAME_APPLE_GATEWAY_TOKEN)
private String appleGatewayToken;
public static final String SERIALIZED_NAME_ACCOUNT_HOLDER_INFO = "accountHolderInfo";
@SerializedName(SERIALIZED_NAME_ACCOUNT_HOLDER_INFO)
private GetAccountPMAccountHolderInfo accountHolderInfo;
public static final String SERIALIZED_NAME_BANK_IDENTIFICATION_NUMBER = "bankIdentificationNumber";
@SerializedName(SERIALIZED_NAME_BANK_IDENTIFICATION_NUMBER)
private String bankIdentificationNumber;
public static final String SERIALIZED_NAME_CREATED_BY = "createdBy";
@SerializedName(SERIALIZED_NAME_CREATED_BY)
private String createdBy;
public static final String SERIALIZED_NAME_CREATED_ON = "createdOn";
@SerializedName(SERIALIZED_NAME_CREATED_ON)
private OffsetDateTime createdOn;
public static final String SERIALIZED_NAME_CREDIT_CARD_MASK_NUMBER = "creditCardMaskNumber";
@SerializedName(SERIALIZED_NAME_CREDIT_CARD_MASK_NUMBER)
private String creditCardMaskNumber;
public static final String SERIALIZED_NAME_CREDIT_CARD_TYPE = "creditCardType";
@SerializedName(SERIALIZED_NAME_CREDIT_CARD_TYPE)
private String creditCardType;
public static final String SERIALIZED_NAME_DEVICE_SESSION_ID = "deviceSessionId";
@SerializedName(SERIALIZED_NAME_DEVICE_SESSION_ID)
private String deviceSessionId;
/**
* Indicates whether the mandate is an existing mandate.
*/
@JsonAdapter(ExistingMandateEnum.Adapter.class)
public enum ExistingMandateEnum {
YES("Yes"),
NO("No");
private String value;
ExistingMandateEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ExistingMandateEnum fromValue(String value) {
for (ExistingMandateEnum b : ExistingMandateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ExistingMandateEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ExistingMandateEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ExistingMandateEnum.fromValue(value);
}
}
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
String value = jsonElement.getAsString();
ExistingMandateEnum.fromValue(value);
}
}
public static final String SERIALIZED_NAME_EXISTING_MANDATE = "existingMandate";
@SerializedName(SERIALIZED_NAME_EXISTING_MANDATE)
private ExistingMandateEnum existingMandate;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_IP_ADDRESS = "ipAddress";
@SerializedName(SERIALIZED_NAME_IP_ADDRESS)
private String ipAddress;
public static final String SERIALIZED_NAME_IS_DEFAULT = "isDefault";
@SerializedName(SERIALIZED_NAME_IS_DEFAULT)
private Boolean isDefault;
public static final String SERIALIZED_NAME_LAST_FAILED_SALE_TRANSACTION_DATE = "lastFailedSaleTransactionDate";
@SerializedName(SERIALIZED_NAME_LAST_FAILED_SALE_TRANSACTION_DATE)
private OffsetDateTime lastFailedSaleTransactionDate;
public static final String SERIALIZED_NAME_LAST_TRANSACTION = "lastTransaction";
@SerializedName(SERIALIZED_NAME_LAST_TRANSACTION)
private String lastTransaction;
public static final String SERIALIZED_NAME_LAST_TRANSACTION_TIME = "lastTransactionTime";
@SerializedName(SERIALIZED_NAME_LAST_TRANSACTION_TIME)
private OffsetDateTime lastTransactionTime;
public static final String SERIALIZED_NAME_MANDATE_INFO = "mandateInfo";
@SerializedName(SERIALIZED_NAME_MANDATE_INFO)
private AccountPMMandateInfo mandateInfo;
public static final String SERIALIZED_NAME_MAX_CONSECUTIVE_PAYMENT_FAILURES = "maxConsecutivePaymentFailures";
@SerializedName(SERIALIZED_NAME_MAX_CONSECUTIVE_PAYMENT_FAILURES)
private Integer maxConsecutivePaymentFailures;
public static final String SERIALIZED_NAME_NUM_CONSECUTIVE_FAILURES = "numConsecutiveFailures";
@SerializedName(SERIALIZED_NAME_NUM_CONSECUTIVE_FAILURES)
private Integer numConsecutiveFailures;
public static final String SERIALIZED_NAME_PAYMENT_RETRY_WINDOW = "paymentRetryWindow";
@SerializedName(SERIALIZED_NAME_PAYMENT_RETRY_WINDOW)
private Integer paymentRetryWindow;
public static final String SERIALIZED_NAME_SECOND_TOKEN_ID = "secondTokenId";
@SerializedName(SERIALIZED_NAME_SECOND_TOKEN_ID)
private String secondTokenId;
/**
* The status of the payment method.
*/
@JsonAdapter(StatusEnum.Adapter.class)
public enum StatusEnum {
ACTIVE("Active"),
CLOSED("Closed"),
SCRUBBED("Scrubbed");
private String value;
StatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StatusEnum fromValue(String value) {
for (StatusEnum b : StatusEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StatusEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StatusEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StatusEnum.fromValue(value);
}
}
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
String value = jsonElement.getAsString();
StatusEnum.fromValue(value);
}
}
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private StatusEnum status;
public static final String SERIALIZED_NAME_TOKEN_ID = "tokenId";
@SerializedName(SERIALIZED_NAME_TOKEN_ID)
private String tokenId;
public static final String SERIALIZED_NAME_TOTAL_NUMBER_OF_ERROR_PAYMENTS = "totalNumberOfErrorPayments";
@SerializedName(SERIALIZED_NAME_TOTAL_NUMBER_OF_ERROR_PAYMENTS)
private Integer totalNumberOfErrorPayments;
public static final String SERIALIZED_NAME_TOTAL_NUMBER_OF_PROCESSED_PAYMENTS = "totalNumberOfProcessedPayments";
@SerializedName(SERIALIZED_NAME_TOTAL_NUMBER_OF_PROCESSED_PAYMENTS)
private Integer totalNumberOfProcessedPayments;
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private String type;
public static final String SERIALIZED_NAME_UPDATED_BY = "updatedBy";
@SerializedName(SERIALIZED_NAME_UPDATED_BY)
private String updatedBy;
public static final String SERIALIZED_NAME_UPDATED_ON = "updatedOn";
@SerializedName(SERIALIZED_NAME_UPDATED_ON)
private OffsetDateTime updatedOn;
public static final String SERIALIZED_NAME_USE_DEFAULT_RETRY_RULE = "useDefaultRetryRule";
@SerializedName(SERIALIZED_NAME_USE_DEFAULT_RETRY_RULE)
private Boolean useDefaultRetryRule;
public GetPaymentMethodForAccountResponse() {
}
public GetPaymentMethodForAccountResponse IBAN(String IBAN) {
this.IBAN = IBAN;
return this;
}
/**
* The International Bank Account Number used to create the SEPA payment method. The value is masked.
* @return IBAN
*/
@javax.annotation.Nullable
public String getIBAN() {
return IBAN;
}
public void setIBAN(String IBAN) {
this.IBAN = IBAN;
}
public GetPaymentMethodForAccountResponse accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* The number of the customer's bank account and it is masked.
* @return accountNumber
*/
@javax.annotation.Nullable
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public GetPaymentMethodForAccountResponse bankCode(String bankCode) {
this.bankCode = bankCode;
return this;
}
/**
* The sort code or number that identifies the bank. This is also known as the sort code.
* @return bankCode
*/
@javax.annotation.Nullable
public String getBankCode() {
return bankCode;
}
public void setBankCode(String bankCode) {
this.bankCode = bankCode;
}
public GetPaymentMethodForAccountResponse bankTransferType(String bankTransferType) {
this.bankTransferType = bankTransferType;
return this;
}
/**
* The type of the Bank Transfer payment method. For example, `SEPA`.
* @return bankTransferType
*/
@javax.annotation.Nullable
public String getBankTransferType() {
return bankTransferType;
}
public void setBankTransferType(String bankTransferType) {
this.bankTransferType = bankTransferType;
}
public GetPaymentMethodForAccountResponse branchCode(String branchCode) {
this.branchCode = branchCode;
return this;
}
/**
* The branch code of the bank used for Direct Debit.
* @return branchCode
*/
@javax.annotation.Nullable
public String getBranchCode() {
return branchCode;
}
public void setBranchCode(String branchCode) {
this.branchCode = branchCode;
}
public GetPaymentMethodForAccountResponse businessIdentificationCode(String businessIdentificationCode) {
this.businessIdentificationCode = businessIdentificationCode;
return this;
}
/**
* The BIC code used for SEPA. The value is masked.
* @return businessIdentificationCode
*/
@javax.annotation.Nullable
public String getBusinessIdentificationCode() {
return businessIdentificationCode;
}
public void setBusinessIdentificationCode(String businessIdentificationCode) {
this.businessIdentificationCode = businessIdentificationCode;
}
public GetPaymentMethodForAccountResponse identityNumber(String identityNumber) {
this.identityNumber = identityNumber;
return this;
}
/**
* The identity number used for Bank Transfer.
* @return identityNumber
*/
@javax.annotation.Nullable
public String getIdentityNumber() {
return identityNumber;
}
public void setIdentityNumber(String identityNumber) {
this.identityNumber = identityNumber;
}
public GetPaymentMethodForAccountResponse bankABACode(String bankABACode) {
this.bankABACode = bankABACode;
return this;
}
/**
* The nine-digit routing number or ABA number used by banks. This field is only required if the `type` field is set to `ACH`.
* @return bankABACode
*/
@javax.annotation.Nullable
public String getBankABACode() {
return bankABACode;
}
public void setBankABACode(String bankABACode) {
this.bankABACode = bankABACode;
}
public GetPaymentMethodForAccountResponse bankAccountName(String bankAccountName) {
this.bankAccountName = bankAccountName;
return this;
}
/**
* The name of the account holder, which can be either a person or a company. This field is only required if the `type` field is set to `ACH`.
* @return bankAccountName
*/
@javax.annotation.Nullable
public String getBankAccountName() {
return bankAccountName;
}
public void setBankAccountName(String bankAccountName) {
this.bankAccountName = bankAccountName;
}
public GetPaymentMethodForAccountResponse cardNumber(String cardNumber) {
this.cardNumber = cardNumber;
return this;
}
/**
* The masked credit card number. When `cardNumber` is `null`, the following fields will not be returned: - `expirationMonth` - `expirationYear` - `accountHolderInfo`
* @return cardNumber
*/
@javax.annotation.Nullable
public String getCardNumber() {
return cardNumber;
}
public void setCardNumber(String cardNumber) {
this.cardNumber = cardNumber;
}
public GetPaymentMethodForAccountResponse expirationMonth(Integer expirationMonth) {
this.expirationMonth = expirationMonth;
return this;
}
/**
* One or two digits expiration month (1-12).
* @return expirationMonth
*/
@javax.annotation.Nullable
public Integer getExpirationMonth() {
return expirationMonth;
}
public void setExpirationMonth(Integer expirationMonth) {
this.expirationMonth = expirationMonth;
}
public GetPaymentMethodForAccountResponse expirationYear(Integer expirationYear) {
this.expirationYear = expirationYear;
return this;
}
/**
* Four-digit expiration year.
* @return expirationYear
*/
@javax.annotation.Nullable
public Integer getExpirationYear() {
return expirationYear;
}
public void setExpirationYear(Integer expirationYear) {
this.expirationYear = expirationYear;
}
public GetPaymentMethodForAccountResponse securityCode(String securityCode) {
this.securityCode = securityCode;
return this;
}
/**
* The CVV or CVV2 security code for the credit card or debit card. Only required if changing expirationMonth, expirationYear, or cardHolderName. To ensure PCI compliance, this value isn''t stored and can''t be queried.
* @return securityCode
*/
@javax.annotation.Nullable
public String getSecurityCode() {
return securityCode;
}
public void setSecurityCode(String securityCode) {
this.securityCode = securityCode;
}
public GetPaymentMethodForAccountResponse BAID(String BAID) {
this.BAID = BAID;
return this;
}
/**
* ID of a PayPal billing agreement. For example, I-1TJ3GAGG82Y9.
* @return BAID
*/
@javax.annotation.Nullable
public String getBAID() {
return BAID;
}
public void setBAID(String BAID) {
this.BAID = BAID;
}
public GetPaymentMethodForAccountResponse email(String email) {
this.email = email;
return this;
}
/**
* Email address associated with the PayPal payment method.
* @return email
*/
@javax.annotation.Nullable
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public GetPaymentMethodForAccountResponse preapprovalKey(String preapprovalKey) {
this.preapprovalKey = preapprovalKey;
return this;
}
/**
* The PayPal preapproval key.
* @return preapprovalKey
*/
@javax.annotation.Nullable
public String getPreapprovalKey() {
return preapprovalKey;
}
public void setPreapprovalKey(String preapprovalKey) {
this.preapprovalKey = preapprovalKey;
}
public GetPaymentMethodForAccountResponse googleBIN(String googleBIN) {
this.googleBIN = googleBIN;
return this;
}
/**
* This field is only available for Google Pay payment methods.
* @return googleBIN
*/
@javax.annotation.Nullable
public String getGoogleBIN() {
return googleBIN;
}
public void setGoogleBIN(String googleBIN) {
this.googleBIN = googleBIN;
}
public GetPaymentMethodForAccountResponse googleCardNumber(String googleCardNumber) {
this.googleCardNumber = googleCardNumber;
return this;
}
/**
* This field is only available for Google Pay payment methods.
* @return googleCardNumber
*/
@javax.annotation.Nullable
public String getGoogleCardNumber() {
return googleCardNumber;
}
public void setGoogleCardNumber(String googleCardNumber) {
this.googleCardNumber = googleCardNumber;
}
public GetPaymentMethodForAccountResponse googleCardType(String googleCardType) {
this.googleCardType = googleCardType;
return this;
}
/**
* This field is only available for Google Pay payment methods. For Google Pay payment methods on Adyen, the first 100 characters of [paymentMethodVariant](https://docs.adyen.com/development-resources/paymentmethodvariant) returned from Adyen are stored in this field.
* @return googleCardType
*/
@javax.annotation.Nullable
public String getGoogleCardType() {
return googleCardType;
}
public void setGoogleCardType(String googleCardType) {
this.googleCardType = googleCardType;
}
public GetPaymentMethodForAccountResponse googleExpiryDate(String googleExpiryDate) {
this.googleExpiryDate = googleExpiryDate;
return this;
}
/**
* This field is only available for Google Pay payment methods.
* @return googleExpiryDate
*/
@javax.annotation.Nullable
public String getGoogleExpiryDate() {
return googleExpiryDate;
}
public void setGoogleExpiryDate(String googleExpiryDate) {
this.googleExpiryDate = googleExpiryDate;
}
public GetPaymentMethodForAccountResponse googleGatewayToken(String googleGatewayToken) {
this.googleGatewayToken = googleGatewayToken;
return this;
}
/**
* This field is only available for Google Pay payment methods.
* @return googleGatewayToken
*/
@javax.annotation.Nullable
public String getGoogleGatewayToken() {
return googleGatewayToken;
}
public void setGoogleGatewayToken(String googleGatewayToken) {
this.googleGatewayToken = googleGatewayToken;
}
public GetPaymentMethodForAccountResponse appleBIN(String appleBIN) {
this.appleBIN = appleBIN;
return this;
}
/**
* This field is only available for Apple Pay payment methods.
* @return appleBIN
*/
@javax.annotation.Nullable
public String getAppleBIN() {
return appleBIN;
}
public void setAppleBIN(String appleBIN) {
this.appleBIN = appleBIN;
}
public GetPaymentMethodForAccountResponse appleCardNumber(String appleCardNumber) {
this.appleCardNumber = appleCardNumber;
return this;
}
/**
* This field is only available for Apple Pay payment methods.
* @return appleCardNumber
*/
@javax.annotation.Nullable
public String getAppleCardNumber() {
return appleCardNumber;
}
public void setAppleCardNumber(String appleCardNumber) {
this.appleCardNumber = appleCardNumber;
}
public GetPaymentMethodForAccountResponse appleCardType(String appleCardType) {
this.appleCardType = appleCardType;
return this;
}
/**
* This field is only available for Apple Pay payment methods. For Apple Pay payment methods on Adyen, the first 100 characters of [paymentMethodVariant](https://docs.adyen.com/development-resources/paymentmethodvariant) returned from Adyen are stored in this field.
* @return appleCardType
*/
@javax.annotation.Nullable
public String getAppleCardType() {
return appleCardType;
}
public void setAppleCardType(String appleCardType) {
this.appleCardType = appleCardType;
}
public GetPaymentMethodForAccountResponse appleExpiryDate(String appleExpiryDate) {
this.appleExpiryDate = appleExpiryDate;
return this;
}
/**
* This field is only available for Apple Pay payment methods.
* @return appleExpiryDate
*/
@javax.annotation.Nullable
public String getAppleExpiryDate() {
return appleExpiryDate;
}
public void setAppleExpiryDate(String appleExpiryDate) {
this.appleExpiryDate = appleExpiryDate;
}
public GetPaymentMethodForAccountResponse appleGatewayToken(String appleGatewayToken) {
this.appleGatewayToken = appleGatewayToken;
return this;
}
/**
* This field is only available for Apple Pay payment methods.
* @return appleGatewayToken
*/
@javax.annotation.Nullable
public String getAppleGatewayToken() {
return appleGatewayToken;
}
public void setAppleGatewayToken(String appleGatewayToken) {
this.appleGatewayToken = appleGatewayToken;
}
public GetPaymentMethodForAccountResponse accountHolderInfo(GetAccountPMAccountHolderInfo accountHolderInfo) {
this.accountHolderInfo = accountHolderInfo;
return this;
}
/**
* Get accountHolderInfo
* @return accountHolderInfo
*/
@javax.annotation.Nullable
public GetAccountPMAccountHolderInfo getAccountHolderInfo() {
return accountHolderInfo;
}
public void setAccountHolderInfo(GetAccountPMAccountHolderInfo accountHolderInfo) {
this.accountHolderInfo = accountHolderInfo;
}
public GetPaymentMethodForAccountResponse bankIdentificationNumber(String bankIdentificationNumber) {
this.bankIdentificationNumber = bankIdentificationNumber;
return this;
}
/**
* The first six or eight digits of the payment method's number, such as the credit card number or account number. Banks use this number to identify a payment method.
* @return bankIdentificationNumber
*/
@javax.annotation.Nullable
public String getBankIdentificationNumber() {
return bankIdentificationNumber;
}
public void setBankIdentificationNumber(String bankIdentificationNumber) {
this.bankIdentificationNumber = bankIdentificationNumber;
}
public GetPaymentMethodForAccountResponse createdBy(String createdBy) {
this.createdBy = createdBy;
return this;
}
/**
* ID of the user who created this payment method.
* @return createdBy
*/
@javax.annotation.Nullable
public String getCreatedBy() {
return createdBy;
}
public void setCreatedBy(String createdBy) {
this.createdBy = createdBy;
}
public GetPaymentMethodForAccountResponse createdOn(OffsetDateTime createdOn) {
this.createdOn = createdOn;
return this;
}
/**
* The date and time when the payment method was created, in `yyyy-mm-dd hh:mm:ss` format.
* @return createdOn
*/
@javax.annotation.Nullable
public OffsetDateTime getCreatedOn() {
return createdOn;
}
public void setCreatedOn(OffsetDateTime createdOn) {
this.createdOn = createdOn;
}
public GetPaymentMethodForAccountResponse creditCardMaskNumber(String creditCardMaskNumber) {
this.creditCardMaskNumber = creditCardMaskNumber;
return this;
}
/**
* The masked credit card number, such as: ``` *********1112 ``` **Note:** This field is only returned for Credit Card Reference Transaction payment type.
* @return creditCardMaskNumber
*/
@javax.annotation.Nullable
public String getCreditCardMaskNumber() {
return creditCardMaskNumber;
}
public void setCreditCardMaskNumber(String creditCardMaskNumber) {
this.creditCardMaskNumber = creditCardMaskNumber;
}
public GetPaymentMethodForAccountResponse creditCardType(String creditCardType) {
this.creditCardType = creditCardType;
return this;
}
/**
* The type of the credit card or debit card. Possible values include `Visa`, `MasterCard`, `AmericanExpress`, `Discover`, `JCB`, and `Diners`. For more information about credit card types supported by different payment gateways, see [Supported Payment Gateways](https://knowledgecenter.zuora.com/CB_Billing/M_Payment_Gateways/Supported_Payment_Gateways). **Note:** This field is only returned for the Credit Card and Debit Card payment types.
* @return creditCardType
*/
@javax.annotation.Nullable
public String getCreditCardType() {
return creditCardType;
}
public void setCreditCardType(String creditCardType) {
this.creditCardType = creditCardType;
}
public GetPaymentMethodForAccountResponse deviceSessionId(String deviceSessionId) {
this.deviceSessionId = deviceSessionId;
return this;
}
/**
* The session ID of the user when the `PaymentMethod` was created or updated.
* @return deviceSessionId
*/
@javax.annotation.Nullable
public String getDeviceSessionId() {
return deviceSessionId;
}
public void setDeviceSessionId(String deviceSessionId) {
this.deviceSessionId = deviceSessionId;
}
public GetPaymentMethodForAccountResponse existingMandate(ExistingMandateEnum existingMandate) {
this.existingMandate = existingMandate;
return this;
}
/**
* Indicates whether the mandate is an existing mandate.
* @return existingMandate
*/
@javax.annotation.Nullable
public ExistingMandateEnum getExistingMandate() {
return existingMandate;
}
public void setExistingMandate(ExistingMandateEnum existingMandate) {
this.existingMandate = existingMandate;
}
public GetPaymentMethodForAccountResponse id(String id) {
this.id = id;
return this;
}
/**
* The payment method ID.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public GetPaymentMethodForAccountResponse ipAddress(String ipAddress) {
this.ipAddress = ipAddress;
return this;
}
/**
* The IP address of the user when the payment method was created or updated.
* @return ipAddress
*/
@javax.annotation.Nullable
public String getIpAddress() {
return ipAddress;
}
public void setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
}
public GetPaymentMethodForAccountResponse isDefault(Boolean isDefault) {
this.isDefault = isDefault;
return this;
}
/**
* Indicates whether this payment method is the default payment method for the account.
* @return isDefault
*/
@javax.annotation.Nullable
public Boolean getIsDefault() {
return isDefault;
}
public void setIsDefault(Boolean isDefault) {
this.isDefault = isDefault;
}
public GetPaymentMethodForAccountResponse lastFailedSaleTransactionDate(OffsetDateTime lastFailedSaleTransactionDate) {
this.lastFailedSaleTransactionDate = lastFailedSaleTransactionDate;
return this;
}
/**
* The date of the last failed attempt to collect payment with this payment method.
* @return lastFailedSaleTransactionDate
*/
@javax.annotation.Nullable
public OffsetDateTime getLastFailedSaleTransactionDate() {
return lastFailedSaleTransactionDate;
}
public void setLastFailedSaleTransactionDate(OffsetDateTime lastFailedSaleTransactionDate) {
this.lastFailedSaleTransactionDate = lastFailedSaleTransactionDate;
}
public GetPaymentMethodForAccountResponse lastTransaction(String lastTransaction) {
this.lastTransaction = lastTransaction;
return this;
}
/**
* ID of the last transaction of this payment method.
* @return lastTransaction
*/
@javax.annotation.Nullable
public String getLastTransaction() {
return lastTransaction;
}
public void setLastTransaction(String lastTransaction) {
this.lastTransaction = lastTransaction;
}
public GetPaymentMethodForAccountResponse lastTransactionTime(OffsetDateTime lastTransactionTime) {
this.lastTransactionTime = lastTransactionTime;
return this;
}
/**
* The time when the last transaction of this payment method happened.
* @return lastTransactionTime
*/
@javax.annotation.Nullable
public OffsetDateTime getLastTransactionTime() {
return lastTransactionTime;
}
public void setLastTransactionTime(OffsetDateTime lastTransactionTime) {
this.lastTransactionTime = lastTransactionTime;
}
public GetPaymentMethodForAccountResponse mandateInfo(AccountPMMandateInfo mandateInfo) {
this.mandateInfo = mandateInfo;
return this;
}
/**
* Get mandateInfo
* @return mandateInfo
*/
@javax.annotation.Nullable
public AccountPMMandateInfo getMandateInfo() {
return mandateInfo;
}
public void setMandateInfo(AccountPMMandateInfo mandateInfo) {
this.mandateInfo = mandateInfo;
}
public GetPaymentMethodForAccountResponse maxConsecutivePaymentFailures(Integer maxConsecutivePaymentFailures) {
this.maxConsecutivePaymentFailures = maxConsecutivePaymentFailures;
return this;
}
/**
* The number of allowable consecutive failures Zuora attempts with the payment method before stopping.
* @return maxConsecutivePaymentFailures
*/
@javax.annotation.Nullable
public Integer getMaxConsecutivePaymentFailures() {
return maxConsecutivePaymentFailures;
}
public void setMaxConsecutivePaymentFailures(Integer maxConsecutivePaymentFailures) {
this.maxConsecutivePaymentFailures = maxConsecutivePaymentFailures;
}
public GetPaymentMethodForAccountResponse numConsecutiveFailures(Integer numConsecutiveFailures) {
this.numConsecutiveFailures = numConsecutiveFailures;
return this;
}
/**
* The number of consecutive failed payments for this payment method. It is reset to `0` upon successful payment.
* @return numConsecutiveFailures
*/
@javax.annotation.Nullable
public Integer getNumConsecutiveFailures() {
return numConsecutiveFailures;
}
public void setNumConsecutiveFailures(Integer numConsecutiveFailures) {
this.numConsecutiveFailures = numConsecutiveFailures;
}
public GetPaymentMethodForAccountResponse paymentRetryWindow(Integer paymentRetryWindow) {
this.paymentRetryWindow = paymentRetryWindow;
return this;
}
/**
* The retry interval setting, which prevents making a payment attempt if the last failed attempt was within the last specified number of hours.
* @return paymentRetryWindow
*/
@javax.annotation.Nullable
public Integer getPaymentRetryWindow() {
return paymentRetryWindow;
}
public void setPaymentRetryWindow(Integer paymentRetryWindow) {
this.paymentRetryWindow = paymentRetryWindow;
}
public GetPaymentMethodForAccountResponse secondTokenId(String secondTokenId) {
this.secondTokenId = secondTokenId;
return this;
}
/**
* A gateway unique identifier that replaces sensitive payment method data. **Note:** This field is only returned for the Credit Card Reference Transaction payment type.
* @return secondTokenId
*/
@javax.annotation.Nullable
public String getSecondTokenId() {
return secondTokenId;
}
public void setSecondTokenId(String secondTokenId) {
this.secondTokenId = secondTokenId;
}
public GetPaymentMethodForAccountResponse status(StatusEnum status) {
this.status = status;
return this;
}
/**
* The status of the payment method.
* @return status
*/
@javax.annotation.Nullable
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public GetPaymentMethodForAccountResponse tokenId(String tokenId) {
this.tokenId = tokenId;
return this;
}
/**
* A gateway unique identifier that replaces sensitive payment method data or represents a gateway's unique customer profile. **Note:** This field is only returned for the Credit Card Reference Transaction payment type.
* @return tokenId
*/
@javax.annotation.Nullable
public String getTokenId() {
return tokenId;
}
public void setTokenId(String tokenId) {
this.tokenId = tokenId;
}
public GetPaymentMethodForAccountResponse totalNumberOfErrorPayments(Integer totalNumberOfErrorPayments) {
this.totalNumberOfErrorPayments = totalNumberOfErrorPayments;
return this;
}
/**
* The number of error payments that used this payment method.
* @return totalNumberOfErrorPayments
*/
@javax.annotation.Nullable
public Integer getTotalNumberOfErrorPayments() {
return totalNumberOfErrorPayments;
}
public void setTotalNumberOfErrorPayments(Integer totalNumberOfErrorPayments) {
this.totalNumberOfErrorPayments = totalNumberOfErrorPayments;
}
public GetPaymentMethodForAccountResponse totalNumberOfProcessedPayments(Integer totalNumberOfProcessedPayments) {
this.totalNumberOfProcessedPayments = totalNumberOfProcessedPayments;
return this;
}
/**
* The number of successful payments that used this payment method.
* @return totalNumberOfProcessedPayments
*/
@javax.annotation.Nullable
public Integer getTotalNumberOfProcessedPayments() {
return totalNumberOfProcessedPayments;
}
public void setTotalNumberOfProcessedPayments(Integer totalNumberOfProcessedPayments) {
this.totalNumberOfProcessedPayments = totalNumberOfProcessedPayments;
}
public GetPaymentMethodForAccountResponse type(String type) {
this.type = type;
return this;
}
/**
* The type of the payment method. For example, `CreditCard`.
* @return type
*/
@javax.annotation.Nullable
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public GetPaymentMethodForAccountResponse updatedBy(String updatedBy) {
this.updatedBy = updatedBy;
return this;
}
/**
* ID of the user who made the last update to this payment method.
* @return updatedBy
*/
@javax.annotation.Nullable
public String getUpdatedBy() {
return updatedBy;
}
public void setUpdatedBy(String updatedBy) {
this.updatedBy = updatedBy;
}
public GetPaymentMethodForAccountResponse updatedOn(OffsetDateTime updatedOn) {
this.updatedOn = updatedOn;
return this;
}
/**
* The last date and time when the payment method was updated, in `yyyy-mm-dd hh:mm:ss` format.
* @return updatedOn
*/
@javax.annotation.Nullable
public OffsetDateTime getUpdatedOn() {
return updatedOn;
}
public void setUpdatedOn(OffsetDateTime updatedOn) {
this.updatedOn = updatedOn;
}
public GetPaymentMethodForAccountResponse useDefaultRetryRule(Boolean useDefaultRetryRule) {
this.useDefaultRetryRule = useDefaultRetryRule;
return this;
}
/**
* Indicates whether this payment method uses the default retry rules configured in the Zuora Payments settings.
* @return useDefaultRetryRule
*/
@javax.annotation.Nullable
public Boolean getUseDefaultRetryRule() {
return useDefaultRetryRule;
}
public void setUseDefaultRetryRule(Boolean useDefaultRetryRule) {
this.useDefaultRetryRule = useDefaultRetryRule;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the GetPaymentMethodForAccountResponse instance itself
*/
public GetPaymentMethodForAccountResponse putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GetPaymentMethodForAccountResponse getPaymentMethodForAccountResponse = (GetPaymentMethodForAccountResponse) o;
return Objects.equals(this.IBAN, getPaymentMethodForAccountResponse.IBAN) &&
Objects.equals(this.accountNumber, getPaymentMethodForAccountResponse.accountNumber) &&
Objects.equals(this.bankCode, getPaymentMethodForAccountResponse.bankCode) &&
Objects.equals(this.bankTransferType, getPaymentMethodForAccountResponse.bankTransferType) &&
Objects.equals(this.branchCode, getPaymentMethodForAccountResponse.branchCode) &&
Objects.equals(this.businessIdentificationCode, getPaymentMethodForAccountResponse.businessIdentificationCode) &&
Objects.equals(this.identityNumber, getPaymentMethodForAccountResponse.identityNumber) &&
Objects.equals(this.bankABACode, getPaymentMethodForAccountResponse.bankABACode) &&
Objects.equals(this.bankAccountName, getPaymentMethodForAccountResponse.bankAccountName) &&
Objects.equals(this.cardNumber, getPaymentMethodForAccountResponse.cardNumber) &&
Objects.equals(this.expirationMonth, getPaymentMethodForAccountResponse.expirationMonth) &&
Objects.equals(this.expirationYear, getPaymentMethodForAccountResponse.expirationYear) &&
Objects.equals(this.securityCode, getPaymentMethodForAccountResponse.securityCode) &&
Objects.equals(this.BAID, getPaymentMethodForAccountResponse.BAID) &&
Objects.equals(this.email, getPaymentMethodForAccountResponse.email) &&
Objects.equals(this.preapprovalKey, getPaymentMethodForAccountResponse.preapprovalKey) &&
Objects.equals(this.googleBIN, getPaymentMethodForAccountResponse.googleBIN) &&
Objects.equals(this.googleCardNumber, getPaymentMethodForAccountResponse.googleCardNumber) &&
Objects.equals(this.googleCardType, getPaymentMethodForAccountResponse.googleCardType) &&
Objects.equals(this.googleExpiryDate, getPaymentMethodForAccountResponse.googleExpiryDate) &&
Objects.equals(this.googleGatewayToken, getPaymentMethodForAccountResponse.googleGatewayToken) &&
Objects.equals(this.appleBIN, getPaymentMethodForAccountResponse.appleBIN) &&
Objects.equals(this.appleCardNumber, getPaymentMethodForAccountResponse.appleCardNumber) &&
Objects.equals(this.appleCardType, getPaymentMethodForAccountResponse.appleCardType) &&
Objects.equals(this.appleExpiryDate, getPaymentMethodForAccountResponse.appleExpiryDate) &&
Objects.equals(this.appleGatewayToken, getPaymentMethodForAccountResponse.appleGatewayToken) &&
Objects.equals(this.accountHolderInfo, getPaymentMethodForAccountResponse.accountHolderInfo) &&
Objects.equals(this.bankIdentificationNumber, getPaymentMethodForAccountResponse.bankIdentificationNumber) &&
Objects.equals(this.createdBy, getPaymentMethodForAccountResponse.createdBy) &&
Objects.equals(this.createdOn, getPaymentMethodForAccountResponse.createdOn) &&
Objects.equals(this.creditCardMaskNumber, getPaymentMethodForAccountResponse.creditCardMaskNumber) &&
Objects.equals(this.creditCardType, getPaymentMethodForAccountResponse.creditCardType) &&
Objects.equals(this.deviceSessionId, getPaymentMethodForAccountResponse.deviceSessionId) &&
Objects.equals(this.existingMandate, getPaymentMethodForAccountResponse.existingMandate) &&
Objects.equals(this.id, getPaymentMethodForAccountResponse.id) &&
Objects.equals(this.ipAddress, getPaymentMethodForAccountResponse.ipAddress) &&
Objects.equals(this.isDefault, getPaymentMethodForAccountResponse.isDefault) &&
Objects.equals(this.lastFailedSaleTransactionDate, getPaymentMethodForAccountResponse.lastFailedSaleTransactionDate) &&
Objects.equals(this.lastTransaction, getPaymentMethodForAccountResponse.lastTransaction) &&
Objects.equals(this.lastTransactionTime, getPaymentMethodForAccountResponse.lastTransactionTime) &&
Objects.equals(this.mandateInfo, getPaymentMethodForAccountResponse.mandateInfo) &&
Objects.equals(this.maxConsecutivePaymentFailures, getPaymentMethodForAccountResponse.maxConsecutivePaymentFailures) &&
Objects.equals(this.numConsecutiveFailures, getPaymentMethodForAccountResponse.numConsecutiveFailures) &&
Objects.equals(this.paymentRetryWindow, getPaymentMethodForAccountResponse.paymentRetryWindow) &&
Objects.equals(this.secondTokenId, getPaymentMethodForAccountResponse.secondTokenId) &&
Objects.equals(this.status, getPaymentMethodForAccountResponse.status) &&
Objects.equals(this.tokenId, getPaymentMethodForAccountResponse.tokenId) &&
Objects.equals(this.totalNumberOfErrorPayments, getPaymentMethodForAccountResponse.totalNumberOfErrorPayments) &&
Objects.equals(this.totalNumberOfProcessedPayments, getPaymentMethodForAccountResponse.totalNumberOfProcessedPayments) &&
Objects.equals(this.type, getPaymentMethodForAccountResponse.type) &&
Objects.equals(this.updatedBy, getPaymentMethodForAccountResponse.updatedBy) &&
Objects.equals(this.updatedOn, getPaymentMethodForAccountResponse.updatedOn) &&
Objects.equals(this.useDefaultRetryRule, getPaymentMethodForAccountResponse.useDefaultRetryRule)&&
Objects.equals(this.additionalProperties, getPaymentMethodForAccountResponse.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(IBAN, accountNumber, bankCode, bankTransferType, branchCode, businessIdentificationCode, identityNumber, bankABACode, bankAccountName, cardNumber, expirationMonth, expirationYear, securityCode, BAID, email, preapprovalKey, googleBIN, googleCardNumber, googleCardType, googleExpiryDate, googleGatewayToken, appleBIN, appleCardNumber, appleCardType, appleExpiryDate, appleGatewayToken, accountHolderInfo, bankIdentificationNumber, createdBy, createdOn, creditCardMaskNumber, creditCardType, deviceSessionId, existingMandate, id, ipAddress, isDefault, lastFailedSaleTransactionDate, lastTransaction, lastTransactionTime, mandateInfo, maxConsecutivePaymentFailures, numConsecutiveFailures, paymentRetryWindow, secondTokenId, status, tokenId, totalNumberOfErrorPayments, totalNumberOfProcessedPayments, type, updatedBy, updatedOn, useDefaultRetryRule, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GetPaymentMethodForAccountResponse {\n");
sb.append(" IBAN: ").append(toIndentedString(IBAN)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" bankCode: ").append(toIndentedString(bankCode)).append("\n");
sb.append(" bankTransferType: ").append(toIndentedString(bankTransferType)).append("\n");
sb.append(" branchCode: ").append(toIndentedString(branchCode)).append("\n");
sb.append(" businessIdentificationCode: ").append(toIndentedString(businessIdentificationCode)).append("\n");
sb.append(" identityNumber: ").append(toIndentedString(identityNumber)).append("\n");
sb.append(" bankABACode: ").append(toIndentedString(bankABACode)).append("\n");
sb.append(" bankAccountName: ").append(toIndentedString(bankAccountName)).append("\n");
sb.append(" cardNumber: ").append(toIndentedString(cardNumber)).append("\n");
sb.append(" expirationMonth: ").append(toIndentedString(expirationMonth)).append("\n");
sb.append(" expirationYear: ").append(toIndentedString(expirationYear)).append("\n");
sb.append(" securityCode: ").append(toIndentedString(securityCode)).append("\n");
sb.append(" BAID: ").append(toIndentedString(BAID)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" preapprovalKey: ").append(toIndentedString(preapprovalKey)).append("\n");
sb.append(" googleBIN: ").append(toIndentedString(googleBIN)).append("\n");
sb.append(" googleCardNumber: ").append(toIndentedString(googleCardNumber)).append("\n");
sb.append(" googleCardType: ").append(toIndentedString(googleCardType)).append("\n");
sb.append(" googleExpiryDate: ").append(toIndentedString(googleExpiryDate)).append("\n");
sb.append(" googleGatewayToken: ").append(toIndentedString(googleGatewayToken)).append("\n");
sb.append(" appleBIN: ").append(toIndentedString(appleBIN)).append("\n");
sb.append(" appleCardNumber: ").append(toIndentedString(appleCardNumber)).append("\n");
sb.append(" appleCardType: ").append(toIndentedString(appleCardType)).append("\n");
sb.append(" appleExpiryDate: ").append(toIndentedString(appleExpiryDate)).append("\n");
sb.append(" appleGatewayToken: ").append(toIndentedString(appleGatewayToken)).append("\n");
sb.append(" accountHolderInfo: ").append(toIndentedString(accountHolderInfo)).append("\n");
sb.append(" bankIdentificationNumber: ").append(toIndentedString(bankIdentificationNumber)).append("\n");
sb.append(" createdBy: ").append(toIndentedString(createdBy)).append("\n");
sb.append(" createdOn: ").append(toIndentedString(createdOn)).append("\n");
sb.append(" creditCardMaskNumber: ").append(toIndentedString(creditCardMaskNumber)).append("\n");
sb.append(" creditCardType: ").append(toIndentedString(creditCardType)).append("\n");
sb.append(" deviceSessionId: ").append(toIndentedString(deviceSessionId)).append("\n");
sb.append(" existingMandate: ").append(toIndentedString(existingMandate)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" ipAddress: ").append(toIndentedString(ipAddress)).append("\n");
sb.append(" isDefault: ").append(toIndentedString(isDefault)).append("\n");
sb.append(" lastFailedSaleTransactionDate: ").append(toIndentedString(lastFailedSaleTransactionDate)).append("\n");
sb.append(" lastTransaction: ").append(toIndentedString(lastTransaction)).append("\n");
sb.append(" lastTransactionTime: ").append(toIndentedString(lastTransactionTime)).append("\n");
sb.append(" mandateInfo: ").append(toIndentedString(mandateInfo)).append("\n");
sb.append(" maxConsecutivePaymentFailures: ").append(toIndentedString(maxConsecutivePaymentFailures)).append("\n");
sb.append(" numConsecutiveFailures: ").append(toIndentedString(numConsecutiveFailures)).append("\n");
sb.append(" paymentRetryWindow: ").append(toIndentedString(paymentRetryWindow)).append("\n");
sb.append(" secondTokenId: ").append(toIndentedString(secondTokenId)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" tokenId: ").append(toIndentedString(tokenId)).append("\n");
sb.append(" totalNumberOfErrorPayments: ").append(toIndentedString(totalNumberOfErrorPayments)).append("\n");
sb.append(" totalNumberOfProcessedPayments: ").append(toIndentedString(totalNumberOfProcessedPayments)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" updatedBy: ").append(toIndentedString(updatedBy)).append("\n");
sb.append(" updatedOn: ").append(toIndentedString(updatedOn)).append("\n");
sb.append(" useDefaultRetryRule: ").append(toIndentedString(useDefaultRetryRule)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("IBAN");
openapiFields.add("accountNumber");
openapiFields.add("bankCode");
openapiFields.add("bankTransferType");
openapiFields.add("branchCode");
openapiFields.add("businessIdentificationCode");
openapiFields.add("identityNumber");
openapiFields.add("bankABACode");
openapiFields.add("bankAccountName");
openapiFields.add("cardNumber");
openapiFields.add("expirationMonth");
openapiFields.add("expirationYear");
openapiFields.add("securityCode");
openapiFields.add("BAID");
openapiFields.add("email");
openapiFields.add("preapprovalKey");
openapiFields.add("googleBIN");
openapiFields.add("googleCardNumber");
openapiFields.add("googleCardType");
openapiFields.add("googleExpiryDate");
openapiFields.add("googleGatewayToken");
openapiFields.add("appleBIN");
openapiFields.add("appleCardNumber");
openapiFields.add("appleCardType");
openapiFields.add("appleExpiryDate");
openapiFields.add("appleGatewayToken");
openapiFields.add("accountHolderInfo");
openapiFields.add("bankIdentificationNumber");
openapiFields.add("createdBy");
openapiFields.add("createdOn");
openapiFields.add("creditCardMaskNumber");
openapiFields.add("creditCardType");
openapiFields.add("deviceSessionId");
openapiFields.add("existingMandate");
openapiFields.add("id");
openapiFields.add("ipAddress");
openapiFields.add("isDefault");
openapiFields.add("lastFailedSaleTransactionDate");
openapiFields.add("lastTransaction");
openapiFields.add("lastTransactionTime");
openapiFields.add("mandateInfo");
openapiFields.add("maxConsecutivePaymentFailures");
openapiFields.add("numConsecutiveFailures");
openapiFields.add("paymentRetryWindow");
openapiFields.add("secondTokenId");
openapiFields.add("status");
openapiFields.add("tokenId");
openapiFields.add("totalNumberOfErrorPayments");
openapiFields.add("totalNumberOfProcessedPayments");
openapiFields.add("type");
openapiFields.add("updatedBy");
openapiFields.add("updatedOn");
openapiFields.add("useDefaultRetryRule");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to GetPaymentMethodForAccountResponse
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!GetPaymentMethodForAccountResponse.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in GetPaymentMethodForAccountResponse is not found in the empty JSON string", GetPaymentMethodForAccountResponse.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("IBAN") != null && !jsonObj.get("IBAN").isJsonNull()) && !jsonObj.get("IBAN").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IBAN` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IBAN").toString()));
}
if ((jsonObj.get("accountNumber") != null && !jsonObj.get("accountNumber").isJsonNull()) && !jsonObj.get("accountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountNumber").toString()));
}
if ((jsonObj.get("bankCode") != null && !jsonObj.get("bankCode").isJsonNull()) && !jsonObj.get("bankCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankCode").toString()));
}
if ((jsonObj.get("bankTransferType") != null && !jsonObj.get("bankTransferType").isJsonNull()) && !jsonObj.get("bankTransferType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankTransferType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankTransferType").toString()));
}
if ((jsonObj.get("branchCode") != null && !jsonObj.get("branchCode").isJsonNull()) && !jsonObj.get("branchCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `branchCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("branchCode").toString()));
}
if ((jsonObj.get("businessIdentificationCode") != null && !jsonObj.get("businessIdentificationCode").isJsonNull()) && !jsonObj.get("businessIdentificationCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `businessIdentificationCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("businessIdentificationCode").toString()));
}
if ((jsonObj.get("identityNumber") != null && !jsonObj.get("identityNumber").isJsonNull()) && !jsonObj.get("identityNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `identityNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("identityNumber").toString()));
}
if ((jsonObj.get("bankABACode") != null && !jsonObj.get("bankABACode").isJsonNull()) && !jsonObj.get("bankABACode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankABACode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankABACode").toString()));
}
if ((jsonObj.get("bankAccountName") != null && !jsonObj.get("bankAccountName").isJsonNull()) && !jsonObj.get("bankAccountName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankAccountName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankAccountName").toString()));
}
if ((jsonObj.get("cardNumber") != null && !jsonObj.get("cardNumber").isJsonNull()) && !jsonObj.get("cardNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cardNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cardNumber").toString()));
}
if ((jsonObj.get("securityCode") != null && !jsonObj.get("securityCode").isJsonNull()) && !jsonObj.get("securityCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `securityCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("securityCode").toString()));
}
if ((jsonObj.get("BAID") != null && !jsonObj.get("BAID").isJsonNull()) && !jsonObj.get("BAID").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `BAID` to be a primitive type in the JSON string but got `%s`", jsonObj.get("BAID").toString()));
}
if ((jsonObj.get("email") != null && !jsonObj.get("email").isJsonNull()) && !jsonObj.get("email").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `email` to be a primitive type in the JSON string but got `%s`", jsonObj.get("email").toString()));
}
if ((jsonObj.get("preapprovalKey") != null && !jsonObj.get("preapprovalKey").isJsonNull()) && !jsonObj.get("preapprovalKey").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `preapprovalKey` to be a primitive type in the JSON string but got `%s`", jsonObj.get("preapprovalKey").toString()));
}
if ((jsonObj.get("googleBIN") != null && !jsonObj.get("googleBIN").isJsonNull()) && !jsonObj.get("googleBIN").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `googleBIN` to be a primitive type in the JSON string but got `%s`", jsonObj.get("googleBIN").toString()));
}
if ((jsonObj.get("googleCardNumber") != null && !jsonObj.get("googleCardNumber").isJsonNull()) && !jsonObj.get("googleCardNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `googleCardNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("googleCardNumber").toString()));
}
if ((jsonObj.get("googleCardType") != null && !jsonObj.get("googleCardType").isJsonNull()) && !jsonObj.get("googleCardType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `googleCardType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("googleCardType").toString()));
}
if ((jsonObj.get("googleExpiryDate") != null && !jsonObj.get("googleExpiryDate").isJsonNull()) && !jsonObj.get("googleExpiryDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `googleExpiryDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("googleExpiryDate").toString()));
}
if ((jsonObj.get("googleGatewayToken") != null && !jsonObj.get("googleGatewayToken").isJsonNull()) && !jsonObj.get("googleGatewayToken").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `googleGatewayToken` to be a primitive type in the JSON string but got `%s`", jsonObj.get("googleGatewayToken").toString()));
}
if ((jsonObj.get("appleBIN") != null && !jsonObj.get("appleBIN").isJsonNull()) && !jsonObj.get("appleBIN").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `appleBIN` to be a primitive type in the JSON string but got `%s`", jsonObj.get("appleBIN").toString()));
}
if ((jsonObj.get("appleCardNumber") != null && !jsonObj.get("appleCardNumber").isJsonNull()) && !jsonObj.get("appleCardNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `appleCardNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("appleCardNumber").toString()));
}
if ((jsonObj.get("appleCardType") != null && !jsonObj.get("appleCardType").isJsonNull()) && !jsonObj.get("appleCardType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `appleCardType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("appleCardType").toString()));
}
if ((jsonObj.get("appleExpiryDate") != null && !jsonObj.get("appleExpiryDate").isJsonNull()) && !jsonObj.get("appleExpiryDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `appleExpiryDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("appleExpiryDate").toString()));
}
if ((jsonObj.get("appleGatewayToken") != null && !jsonObj.get("appleGatewayToken").isJsonNull()) && !jsonObj.get("appleGatewayToken").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `appleGatewayToken` to be a primitive type in the JSON string but got `%s`", jsonObj.get("appleGatewayToken").toString()));
}
// validate the optional field `accountHolderInfo`
if (jsonObj.get("accountHolderInfo") != null && !jsonObj.get("accountHolderInfo").isJsonNull()) {
GetAccountPMAccountHolderInfo.validateJsonElement(jsonObj.get("accountHolderInfo"));
}
if ((jsonObj.get("bankIdentificationNumber") != null && !jsonObj.get("bankIdentificationNumber").isJsonNull()) && !jsonObj.get("bankIdentificationNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankIdentificationNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankIdentificationNumber").toString()));
}
if ((jsonObj.get("createdBy") != null && !jsonObj.get("createdBy").isJsonNull()) && !jsonObj.get("createdBy").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdBy` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdBy").toString()));
}
if ((jsonObj.get("creditCardMaskNumber") != null && !jsonObj.get("creditCardMaskNumber").isJsonNull()) && !jsonObj.get("creditCardMaskNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creditCardMaskNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creditCardMaskNumber").toString()));
}
if ((jsonObj.get("creditCardType") != null && !jsonObj.get("creditCardType").isJsonNull()) && !jsonObj.get("creditCardType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creditCardType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creditCardType").toString()));
}
if ((jsonObj.get("deviceSessionId") != null && !jsonObj.get("deviceSessionId").isJsonNull()) && !jsonObj.get("deviceSessionId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `deviceSessionId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("deviceSessionId").toString()));
}
if ((jsonObj.get("existingMandate") != null && !jsonObj.get("existingMandate").isJsonNull()) && !jsonObj.get("existingMandate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `existingMandate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("existingMandate").toString()));
}
// validate the optional field `existingMandate`
if (jsonObj.get("existingMandate") != null && !jsonObj.get("existingMandate").isJsonNull()) {
ExistingMandateEnum.validateJsonElement(jsonObj.get("existingMandate"));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("ipAddress") != null && !jsonObj.get("ipAddress").isJsonNull()) && !jsonObj.get("ipAddress").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ipAddress` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ipAddress").toString()));
}
if ((jsonObj.get("lastTransaction") != null && !jsonObj.get("lastTransaction").isJsonNull()) && !jsonObj.get("lastTransaction").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `lastTransaction` to be a primitive type in the JSON string but got `%s`", jsonObj.get("lastTransaction").toString()));
}
// validate the optional field `mandateInfo`
if (jsonObj.get("mandateInfo") != null && !jsonObj.get("mandateInfo").isJsonNull()) {
AccountPMMandateInfo.validateJsonElement(jsonObj.get("mandateInfo"));
}
if ((jsonObj.get("secondTokenId") != null && !jsonObj.get("secondTokenId").isJsonNull()) && !jsonObj.get("secondTokenId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `secondTokenId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("secondTokenId").toString()));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
// validate the optional field `status`
if (jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) {
StatusEnum.validateJsonElement(jsonObj.get("status"));
}
if ((jsonObj.get("tokenId") != null && !jsonObj.get("tokenId").isJsonNull()) && !jsonObj.get("tokenId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `tokenId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("tokenId").toString()));
}
if ((jsonObj.get("type") != null && !jsonObj.get("type").isJsonNull()) && !jsonObj.get("type").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `type` to be a primitive type in the JSON string but got `%s`", jsonObj.get("type").toString()));
}
if ((jsonObj.get("updatedBy") != null && !jsonObj.get("updatedBy").isJsonNull()) && !jsonObj.get("updatedBy").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedBy` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedBy").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!GetPaymentMethodForAccountResponse.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'GetPaymentMethodForAccountResponse' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(GetPaymentMethodForAccountResponse.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, GetPaymentMethodForAccountResponse value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public GetPaymentMethodForAccountResponse read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
GetPaymentMethodForAccountResponse instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of GetPaymentMethodForAccountResponse given an JSON string
*
* @param jsonString JSON string
* @return An instance of GetPaymentMethodForAccountResponse
* @throws IOException if the JSON string is invalid with respect to GetPaymentMethodForAccountResponse
*/
public static GetPaymentMethodForAccountResponse fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, GetPaymentMethodForAccountResponse.class);
}
/**
* Convert an instance of GetPaymentMethodForAccountResponse to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy