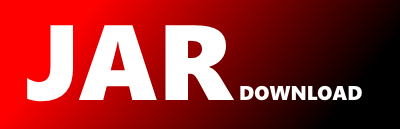
com.zuora.model.GetProductRatePlanChargeResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.ApplyDiscountTo;
import com.zuora.model.BillCycleType;
import com.zuora.model.BillingPeriodAlignmentProductRatePlanChargeRest;
import com.zuora.model.BillingPeriodProductRatePlanChargeRest;
import com.zuora.model.BillingTimingProductRatePlanChargeRest;
import com.zuora.model.ChargeFunction;
import com.zuora.model.ChargeModelConfiguration;
import com.zuora.model.ChargeModelProductRatePlanChargeRest;
import com.zuora.model.ChargeType;
import com.zuora.model.CommitmentType;
import com.zuora.model.DeliveryScheduleProductRatePlanCharge;
import com.zuora.model.DiscountLevel;
import com.zuora.model.EndDateConditionProductRatePlanChargeRest;
import com.zuora.model.ListPriceBase;
import com.zuora.model.OverageCalculationOption;
import com.zuora.model.OverageUnusedUnitsCreditOption;
import com.zuora.model.PrepaidOperationType;
import com.zuora.model.PriceChangeOption;
import com.zuora.model.PriceIncreaseOption;
import com.zuora.model.ProductRatePlanChargeObjectNSFieldsIncludeChildrenNS;
import com.zuora.model.ProductRatePlanChargeObjectNSFieldsItemTypeNS;
import com.zuora.model.ProductRatePlanChargeObjectNSFieldsRevRecEndNS;
import com.zuora.model.ProductRatePlanChargeObjectNSFieldsRevRecStartNS;
import com.zuora.model.RatingGroup;
import com.zuora.model.RatingGroupsOperatorType;
import com.zuora.model.RevRecTriggerConditionProductRatePlanChargeRest;
import com.zuora.model.RevenueRecognitionRuleName;
import com.zuora.model.RolloverApply;
import com.zuora.model.SmoothingModel;
import com.zuora.model.TaxMode;
import com.zuora.model.TriggerEventProductRatePlanChargeRest;
import com.zuora.model.UpToPeriodsTypeProductRatePlanChargeRest;
import com.zuora.model.UsageRecordRatingOption;
import com.zuora.model.ValidityPeriodType;
import com.zuora.model.WeeklyBillCycleDay;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.OffsetDateTime;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* GetProductRatePlanChargeResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class GetProductRatePlanChargeResponse {
public static final String SERIALIZED_NAME_ID = "Id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_ID = "ProductRatePlanId";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_ID)
private String productRatePlanId;
public static final String SERIALIZED_NAME_ACCOUNTING_CODE = "AccountingCode";
@SerializedName(SERIALIZED_NAME_ACCOUNTING_CODE)
private String accountingCode;
public static final String SERIALIZED_NAME_APPLY_DISCOUNT_TO = "ApplyDiscountTo";
@SerializedName(SERIALIZED_NAME_APPLY_DISCOUNT_TO)
private ApplyDiscountTo applyDiscountTo;
public static final String SERIALIZED_NAME_BILL_CYCLE_DAY = "BillCycleDay";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_DAY)
private Integer billCycleDay;
public static final String SERIALIZED_NAME_BILL_CYCLE_TYPE = "BillCycleType";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_TYPE)
private BillCycleType billCycleType;
public static final String SERIALIZED_NAME_BILLING_PERIOD = "BillingPeriod";
@SerializedName(SERIALIZED_NAME_BILLING_PERIOD)
private BillingPeriodProductRatePlanChargeRest billingPeriod;
public static final String SERIALIZED_NAME_BILLING_PERIOD_ALIGNMENT = "BillingPeriodAlignment";
@SerializedName(SERIALIZED_NAME_BILLING_PERIOD_ALIGNMENT)
private BillingPeriodAlignmentProductRatePlanChargeRest billingPeriodAlignment;
public static final String SERIALIZED_NAME_BILLING_TIMING = "BillingTiming";
@SerializedName(SERIALIZED_NAME_BILLING_TIMING)
private BillingTimingProductRatePlanChargeRest billingTiming;
public static final String SERIALIZED_NAME_CHARGE_FUNCTION = "ChargeFunction";
@SerializedName(SERIALIZED_NAME_CHARGE_FUNCTION)
private ChargeFunction chargeFunction;
public static final String SERIALIZED_NAME_CHARGE_MODEL = "ChargeModel";
@SerializedName(SERIALIZED_NAME_CHARGE_MODEL)
private ChargeModelProductRatePlanChargeRest chargeModel;
public static final String SERIALIZED_NAME_CHARGE_MODEL_CONFIGURATION = "ChargeModelConfiguration";
@SerializedName(SERIALIZED_NAME_CHARGE_MODEL_CONFIGURATION)
private ChargeModelConfiguration chargeModelConfiguration;
public static final String SERIALIZED_NAME_CHARGE_TYPE = "ChargeType";
@SerializedName(SERIALIZED_NAME_CHARGE_TYPE)
private ChargeType chargeType;
public static final String SERIALIZED_NAME_COMMITMENT_TYPE = "CommitmentType";
@SerializedName(SERIALIZED_NAME_COMMITMENT_TYPE)
private CommitmentType commitmentType;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "CreatedById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "CreatedDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private OffsetDateTime createdDate;
public static final String SERIALIZED_NAME_DEFAULT_QUANTITY = "DefaultQuantity";
@SerializedName(SERIALIZED_NAME_DEFAULT_QUANTITY)
private Double defaultQuantity;
public static final String SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNT = "DeferredRevenueAccount";
@SerializedName(SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNT)
private String deferredRevenueAccount;
public static final String SERIALIZED_NAME_DELIVERY_SCHEDULE = "DeliverySchedule";
@SerializedName(SERIALIZED_NAME_DELIVERY_SCHEDULE)
private DeliveryScheduleProductRatePlanCharge deliverySchedule;
public static final String SERIALIZED_NAME_DESCRIPTION = "Description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_DISCOUNT_LEVEL = "DiscountLevel";
@SerializedName(SERIALIZED_NAME_DISCOUNT_LEVEL)
private DiscountLevel discountLevel;
public static final String SERIALIZED_NAME_DRAWDOWN_RATE = "DrawdownRate";
@SerializedName(SERIALIZED_NAME_DRAWDOWN_RATE)
private BigDecimal drawdownRate;
public static final String SERIALIZED_NAME_DRAWDOWN_UOM = "DrawdownUom";
@SerializedName(SERIALIZED_NAME_DRAWDOWN_UOM)
private String drawdownUom;
public static final String SERIALIZED_NAME_END_DATE_CONDITION = "EndDateCondition";
@SerializedName(SERIALIZED_NAME_END_DATE_CONDITION)
private EndDateConditionProductRatePlanChargeRest endDateCondition = EndDateConditionProductRatePlanChargeRest.SUBSCRIPTIONEND;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING = "ExcludeItemBillingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBillingFromRevenueAccounting;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING = "ExcludeItemBookingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBookingFromRevenueAccounting;
public static final String SERIALIZED_NAME_INCLUDED_UNITS = "IncludedUnits";
@SerializedName(SERIALIZED_NAME_INCLUDED_UNITS)
private Double includedUnits;
public static final String SERIALIZED_NAME_IS_PREPAID = "IsPrepaid";
@SerializedName(SERIALIZED_NAME_IS_PREPAID)
private Boolean isPrepaid;
public static final String SERIALIZED_NAME_IS_ROLLOVER = "IsRollover";
@SerializedName(SERIALIZED_NAME_IS_ROLLOVER)
private Boolean isRollover;
public static final String SERIALIZED_NAME_IS_STACKED_DISCOUNT = "IsStackedDiscount";
@SerializedName(SERIALIZED_NAME_IS_STACKED_DISCOUNT)
private Boolean isStackedDiscount;
public static final String SERIALIZED_NAME_IS_UNBILLED = "IsUnbilled";
@SerializedName(SERIALIZED_NAME_IS_UNBILLED)
private Boolean isUnbilled;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_TIMING = "RevenueRecognitionTiming";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_TIMING)
private String revenueRecognitionTiming;
public static final String SERIALIZED_NAME_REVENUE_AMORTIZATION_METHOD = "RevenueAmortizationMethod";
@SerializedName(SERIALIZED_NAME_REVENUE_AMORTIZATION_METHOD)
private String revenueAmortizationMethod;
public static final String SERIALIZED_NAME_LEGACY_REVENUE_REPORTING = "LegacyRevenueReporting";
@SerializedName(SERIALIZED_NAME_LEGACY_REVENUE_REPORTING)
private Boolean legacyRevenueReporting;
public static final String SERIALIZED_NAME_LIST_PRICE_BASE = "ListPriceBase";
@SerializedName(SERIALIZED_NAME_LIST_PRICE_BASE)
private ListPriceBase listPriceBase;
public static final String SERIALIZED_NAME_MIN_QUANTITY = "MinQuantity";
@SerializedName(SERIALIZED_NAME_MIN_QUANTITY)
private Double minQuantity;
public static final String SERIALIZED_NAME_MAX_QUANTITY = "MaxQuantity";
@SerializedName(SERIALIZED_NAME_MAX_QUANTITY)
private Double maxQuantity;
public static final String SERIALIZED_NAME_NAME = "Name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_NUMBER_OF_PERIOD = "NumberOfPeriod";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_PERIOD)
private Long numberOfPeriod;
public static final String SERIALIZED_NAME_OVERAGE_CALCULATION_OPTION = "OverageCalculationOption";
@SerializedName(SERIALIZED_NAME_OVERAGE_CALCULATION_OPTION)
private OverageCalculationOption overageCalculationOption;
public static final String SERIALIZED_NAME_OVERAGE_UNUSED_UNITS_CREDIT_OPTION = "OverageUnusedUnitsCreditOption";
@SerializedName(SERIALIZED_NAME_OVERAGE_UNUSED_UNITS_CREDIT_OPTION)
private OverageUnusedUnitsCreditOption overageUnusedUnitsCreditOption;
public static final String SERIALIZED_NAME_PREPAID_OPERATION_TYPE = "PrepaidOperationType";
@SerializedName(SERIALIZED_NAME_PREPAID_OPERATION_TYPE)
private PrepaidOperationType prepaidOperationType;
public static final String SERIALIZED_NAME_PREPAID_QUANTITY = "prepaidQuantity";
@SerializedName(SERIALIZED_NAME_PREPAID_QUANTITY)
private BigDecimal prepaidQuantity;
public static final String SERIALIZED_NAME_PREPAID_TOTAL_QUANTITY = "prepaidTotalQuantity";
@SerializedName(SERIALIZED_NAME_PREPAID_TOTAL_QUANTITY)
private BigDecimal prepaidTotalQuantity;
public static final String SERIALIZED_NAME_PREPAID_UOM = "prepaidUom";
@SerializedName(SERIALIZED_NAME_PREPAID_UOM)
private String prepaidUom;
public static final String SERIALIZED_NAME_PREPAY_PERIODS = "prepayPeriods";
@SerializedName(SERIALIZED_NAME_PREPAY_PERIODS)
private Long prepayPeriods;
public static final String SERIALIZED_NAME_PRICE_CHANGE_OPTION = "PriceChangeOption";
@SerializedName(SERIALIZED_NAME_PRICE_CHANGE_OPTION)
private PriceChangeOption priceChangeOption = PriceChangeOption.NOCHANGE;
public static final String SERIALIZED_NAME_PRICE_INCREASE_PERCENTAGE = "PriceIncreasePercentage";
@SerializedName(SERIALIZED_NAME_PRICE_INCREASE_PERCENTAGE)
private Double priceIncreasePercentage;
public static final String SERIALIZED_NAME_PRICE_INCREASE_OPTION = "PriceIncreaseOption";
@SerializedName(SERIALIZED_NAME_PRICE_INCREASE_OPTION)
private PriceIncreaseOption priceIncreaseOption;
public static final String SERIALIZED_NAME_RATING_GROUP = "RatingGroup";
@SerializedName(SERIALIZED_NAME_RATING_GROUP)
private RatingGroup ratingGroup;
public static final String SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNT = "RecognizedRevenueAccount";
@SerializedName(SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNT)
private String recognizedRevenueAccount;
public static final String SERIALIZED_NAME_REV_REC_CODE = "RevRecCode";
@SerializedName(SERIALIZED_NAME_REV_REC_CODE)
private String revRecCode;
public static final String SERIALIZED_NAME_REV_REC_TRIGGER_CONDITION = "RevRecTriggerCondition";
@SerializedName(SERIALIZED_NAME_REV_REC_TRIGGER_CONDITION)
private RevRecTriggerConditionProductRatePlanChargeRest revRecTriggerCondition;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME = "RevenueRecognitionRuleName";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME)
private RevenueRecognitionRuleName revenueRecognitionRuleName;
public static final String SERIALIZED_NAME_ROLLOVER_APPLY = "RolloverApply";
@SerializedName(SERIALIZED_NAME_ROLLOVER_APPLY)
private RolloverApply rolloverApply;
public static final String SERIALIZED_NAME_ROLLOVER_PERIODS = "RolloverPeriods";
@SerializedName(SERIALIZED_NAME_ROLLOVER_PERIODS)
private BigDecimal rolloverPeriods;
public static final String SERIALIZED_NAME_SMOOTHING_MODEL = "SmoothingModel";
@SerializedName(SERIALIZED_NAME_SMOOTHING_MODEL)
private SmoothingModel smoothingModel;
public static final String SERIALIZED_NAME_SPECIFIC_BILLING_PERIOD = "SpecificBillingPeriod";
@SerializedName(SERIALIZED_NAME_SPECIFIC_BILLING_PERIOD)
private Long specificBillingPeriod;
public static final String SERIALIZED_NAME_SPECIFIC_LIST_PRICE_BASE = "SpecificListPriceBase";
@SerializedName(SERIALIZED_NAME_SPECIFIC_LIST_PRICE_BASE)
private Object specificListPriceBase;
public static final String SERIALIZED_NAME_TAX_CODE = "TaxCode";
@SerializedName(SERIALIZED_NAME_TAX_CODE)
private String taxCode;
public static final String SERIALIZED_NAME_TAX_MODE = "TaxMode";
@SerializedName(SERIALIZED_NAME_TAX_MODE)
private TaxMode taxMode;
public static final String SERIALIZED_NAME_TAXABLE = "Taxable";
@SerializedName(SERIALIZED_NAME_TAXABLE)
private Boolean taxable;
public static final String SERIALIZED_NAME_TRIGGER_EVENT = "TriggerEvent";
@SerializedName(SERIALIZED_NAME_TRIGGER_EVENT)
private TriggerEventProductRatePlanChargeRest triggerEvent;
public static final String SERIALIZED_NAME_U_O_M = "UOM";
@SerializedName(SERIALIZED_NAME_U_O_M)
private String UOM;
public static final String SERIALIZED_NAME_UP_TO_PERIODS = "UpToPeriods";
@SerializedName(SERIALIZED_NAME_UP_TO_PERIODS)
private Long upToPeriods;
public static final String SERIALIZED_NAME_UP_TO_PERIODS_TYPE = "UpToPeriodsType";
@SerializedName(SERIALIZED_NAME_UP_TO_PERIODS_TYPE)
private UpToPeriodsTypeProductRatePlanChargeRest upToPeriodsType = UpToPeriodsTypeProductRatePlanChargeRest.BILLING_PERIODS;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "UpdatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "UpdatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private OffsetDateTime updatedDate;
public static final String SERIALIZED_NAME_USAGE_RECORD_RATING_OPTION = "UsageRecordRatingOption";
@SerializedName(SERIALIZED_NAME_USAGE_RECORD_RATING_OPTION)
private UsageRecordRatingOption usageRecordRatingOption;
public static final String SERIALIZED_NAME_USE_DISCOUNT_SPECIFIC_ACCOUNTING_CODE = "UseDiscountSpecificAccountingCode";
@SerializedName(SERIALIZED_NAME_USE_DISCOUNT_SPECIFIC_ACCOUNTING_CODE)
private Boolean useDiscountSpecificAccountingCode;
public static final String SERIALIZED_NAME_USE_TENANT_DEFAULT_FOR_PRICE_CHANGE = "UseTenantDefaultForPriceChange";
@SerializedName(SERIALIZED_NAME_USE_TENANT_DEFAULT_FOR_PRICE_CHANGE)
private Boolean useTenantDefaultForPriceChange;
public static final String SERIALIZED_NAME_VALIDITY_PERIOD_TYPE = "ValidityPeriodType";
@SerializedName(SERIALIZED_NAME_VALIDITY_PERIOD_TYPE)
private ValidityPeriodType validityPeriodType;
public static final String SERIALIZED_NAME_WEEKLY_BILL_CYCLE_DAY = "WeeklyBillCycleDay";
@SerializedName(SERIALIZED_NAME_WEEKLY_BILL_CYCLE_DAY)
private WeeklyBillCycleDay weeklyBillCycleDay;
public static final String SERIALIZED_NAME_RATING_GROUPS_OPERATOR_TYPE = "RatingGroupsOperatorType";
@SerializedName(SERIALIZED_NAME_RATING_GROUPS_OPERATOR_TYPE)
private RatingGroupsOperatorType ratingGroupsOperatorType;
public static final String SERIALIZED_NAME_CLASS_N_S = "Class__NS";
@SerializedName(SERIALIZED_NAME_CLASS_N_S)
private String classNS;
public static final String SERIALIZED_NAME_DEFERRED_REV_ACCOUNT_N_S = "DeferredRevAccount__NS";
@SerializedName(SERIALIZED_NAME_DEFERRED_REV_ACCOUNT_N_S)
private String deferredRevAccountNS;
public static final String SERIALIZED_NAME_DEPARTMENT_N_S = "Department__NS";
@SerializedName(SERIALIZED_NAME_DEPARTMENT_N_S)
private String departmentNS;
public static final String SERIALIZED_NAME_INCLUDE_CHILDREN_N_S = "IncludeChildren__NS";
@SerializedName(SERIALIZED_NAME_INCLUDE_CHILDREN_N_S)
private ProductRatePlanChargeObjectNSFieldsIncludeChildrenNS includeChildrenNS;
public static final String SERIALIZED_NAME_INTEGRATION_ID_N_S = "IntegrationId__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_ID_N_S)
private String integrationIdNS;
public static final String SERIALIZED_NAME_INTEGRATION_STATUS_N_S = "IntegrationStatus__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_STATUS_N_S)
private String integrationStatusNS;
public static final String SERIALIZED_NAME_ITEM_TYPE_N_S = "ItemType__NS";
@SerializedName(SERIALIZED_NAME_ITEM_TYPE_N_S)
private ProductRatePlanChargeObjectNSFieldsItemTypeNS itemTypeNS;
public static final String SERIALIZED_NAME_LOCATION_N_S = "Location__NS";
@SerializedName(SERIALIZED_NAME_LOCATION_N_S)
private String locationNS;
public static final String SERIALIZED_NAME_RECOGNIZED_REV_ACCOUNT_N_S = "RecognizedRevAccount__NS";
@SerializedName(SERIALIZED_NAME_RECOGNIZED_REV_ACCOUNT_N_S)
private String recognizedRevAccountNS;
public static final String SERIALIZED_NAME_REV_REC_END_N_S = "RevRecEnd__NS";
@SerializedName(SERIALIZED_NAME_REV_REC_END_N_S)
private ProductRatePlanChargeObjectNSFieldsRevRecEndNS revRecEndNS;
public static final String SERIALIZED_NAME_REV_REC_START_N_S = "RevRecStart__NS";
@SerializedName(SERIALIZED_NAME_REV_REC_START_N_S)
private ProductRatePlanChargeObjectNSFieldsRevRecStartNS revRecStartNS;
public static final String SERIALIZED_NAME_REV_REC_TEMPLATE_TYPE_N_S = "RevRecTemplateType__NS";
@SerializedName(SERIALIZED_NAME_REV_REC_TEMPLATE_TYPE_N_S)
private String revRecTemplateTypeNS;
public static final String SERIALIZED_NAME_SUBSIDIARY_N_S = "Subsidiary__NS";
@SerializedName(SERIALIZED_NAME_SUBSIDIARY_N_S)
private String subsidiaryNS;
public static final String SERIALIZED_NAME_SYNC_DATE_N_S = "SyncDate__NS";
@SerializedName(SERIALIZED_NAME_SYNC_DATE_N_S)
private String syncDateNS;
public GetProductRatePlanChargeResponse() {
}
public GetProductRatePlanChargeResponse id(String id) {
this.id = id;
return this;
}
/**
* Object identifier.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public GetProductRatePlanChargeResponse productRatePlanId(String productRatePlanId) {
this.productRatePlanId = productRatePlanId;
return this;
}
/**
* The ID of the product rate plan associated with this product rate plan charge.
* @return productRatePlanId
*/
@javax.annotation.Nullable
public String getProductRatePlanId() {
return productRatePlanId;
}
public void setProductRatePlanId(String productRatePlanId) {
this.productRatePlanId = productRatePlanId;
}
public GetProductRatePlanChargeResponse accountingCode(String accountingCode) {
this.accountingCode = accountingCode;
return this;
}
/**
* The accounting code for the charge. Accounting codes group transactions that contain similar accounting attributes.
* @return accountingCode
*/
@javax.annotation.Nullable
public String getAccountingCode() {
return accountingCode;
}
public void setAccountingCode(String accountingCode) {
this.accountingCode = accountingCode;
}
public GetProductRatePlanChargeResponse applyDiscountTo(ApplyDiscountTo applyDiscountTo) {
this.applyDiscountTo = applyDiscountTo;
return this;
}
/**
* Get applyDiscountTo
* @return applyDiscountTo
*/
@javax.annotation.Nullable
public ApplyDiscountTo getApplyDiscountTo() {
return applyDiscountTo;
}
public void setApplyDiscountTo(ApplyDiscountTo applyDiscountTo) {
this.applyDiscountTo = applyDiscountTo;
}
public GetProductRatePlanChargeResponse billCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
return this;
}
/**
* Sets the bill cycle day (BCD) for the charge. The BCD determines which day of the month customer is billed. The BCD value in the account can override the BCD in this object. StackedDiscount **Character limit**: 2 **Values**: a valid BCD integer, 1 - 31
* @return billCycleDay
*/
@javax.annotation.Nullable
public Integer getBillCycleDay() {
return billCycleDay;
}
public void setBillCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
}
public GetProductRatePlanChargeResponse billCycleType(BillCycleType billCycleType) {
this.billCycleType = billCycleType;
return this;
}
/**
* Get billCycleType
* @return billCycleType
*/
@javax.annotation.Nullable
public BillCycleType getBillCycleType() {
return billCycleType;
}
public void setBillCycleType(BillCycleType billCycleType) {
this.billCycleType = billCycleType;
}
public GetProductRatePlanChargeResponse billingPeriod(BillingPeriodProductRatePlanChargeRest billingPeriod) {
this.billingPeriod = billingPeriod;
return this;
}
/**
* Get billingPeriod
* @return billingPeriod
*/
@javax.annotation.Nullable
public BillingPeriodProductRatePlanChargeRest getBillingPeriod() {
return billingPeriod;
}
public void setBillingPeriod(BillingPeriodProductRatePlanChargeRest billingPeriod) {
this.billingPeriod = billingPeriod;
}
public GetProductRatePlanChargeResponse billingPeriodAlignment(BillingPeriodAlignmentProductRatePlanChargeRest billingPeriodAlignment) {
this.billingPeriodAlignment = billingPeriodAlignment;
return this;
}
/**
* Get billingPeriodAlignment
* @return billingPeriodAlignment
*/
@javax.annotation.Nullable
public BillingPeriodAlignmentProductRatePlanChargeRest getBillingPeriodAlignment() {
return billingPeriodAlignment;
}
public void setBillingPeriodAlignment(BillingPeriodAlignmentProductRatePlanChargeRest billingPeriodAlignment) {
this.billingPeriodAlignment = billingPeriodAlignment;
}
public GetProductRatePlanChargeResponse billingTiming(BillingTimingProductRatePlanChargeRest billingTiming) {
this.billingTiming = billingTiming;
return this;
}
/**
* Get billingTiming
* @return billingTiming
*/
@javax.annotation.Nullable
public BillingTimingProductRatePlanChargeRest getBillingTiming() {
return billingTiming;
}
public void setBillingTiming(BillingTimingProductRatePlanChargeRest billingTiming) {
this.billingTiming = billingTiming;
}
public GetProductRatePlanChargeResponse chargeFunction(ChargeFunction chargeFunction) {
this.chargeFunction = chargeFunction;
return this;
}
/**
* Get chargeFunction
* @return chargeFunction
*/
@javax.annotation.Nullable
public ChargeFunction getChargeFunction() {
return chargeFunction;
}
public void setChargeFunction(ChargeFunction chargeFunction) {
this.chargeFunction = chargeFunction;
}
public GetProductRatePlanChargeResponse chargeModel(ChargeModelProductRatePlanChargeRest chargeModel) {
this.chargeModel = chargeModel;
return this;
}
/**
* Get chargeModel
* @return chargeModel
*/
@javax.annotation.Nullable
public ChargeModelProductRatePlanChargeRest getChargeModel() {
return chargeModel;
}
public void setChargeModel(ChargeModelProductRatePlanChargeRest chargeModel) {
this.chargeModel = chargeModel;
}
public GetProductRatePlanChargeResponse chargeModelConfiguration(ChargeModelConfiguration chargeModelConfiguration) {
this.chargeModelConfiguration = chargeModelConfiguration;
return this;
}
/**
* Get chargeModelConfiguration
* @return chargeModelConfiguration
*/
@javax.annotation.Nullable
public ChargeModelConfiguration getChargeModelConfiguration() {
return chargeModelConfiguration;
}
public void setChargeModelConfiguration(ChargeModelConfiguration chargeModelConfiguration) {
this.chargeModelConfiguration = chargeModelConfiguration;
}
public GetProductRatePlanChargeResponse chargeType(ChargeType chargeType) {
this.chargeType = chargeType;
return this;
}
/**
* Get chargeType
* @return chargeType
*/
@javax.annotation.Nullable
public ChargeType getChargeType() {
return chargeType;
}
public void setChargeType(ChargeType chargeType) {
this.chargeType = chargeType;
}
public GetProductRatePlanChargeResponse commitmentType(CommitmentType commitmentType) {
this.commitmentType = commitmentType;
return this;
}
/**
* Get commitmentType
* @return commitmentType
*/
@javax.annotation.Nullable
public CommitmentType getCommitmentType() {
return commitmentType;
}
public void setCommitmentType(CommitmentType commitmentType) {
this.commitmentType = commitmentType;
}
public GetProductRatePlanChargeResponse createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* The automatically generated ID of the Zuora user who created the `ProductRatePlanCharge` object.
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public GetProductRatePlanChargeResponse createdDate(OffsetDateTime createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* The date when the `ProductRatePlanCharge` object was created.
* @return createdDate
*/
@javax.annotation.Nullable
public OffsetDateTime getCreatedDate() {
return createdDate;
}
public void setCreatedDate(OffsetDateTime createdDate) {
this.createdDate = createdDate;
}
public GetProductRatePlanChargeResponse defaultQuantity(Double defaultQuantity) {
this.defaultQuantity = defaultQuantity;
return this;
}
/**
* The default quantity of units, such as the number of authors in a hosted wiki service. This field is required if you use a per-unit pricing model. **Character limit**: 16 **Values**: a valid quantity value. **Note**: When `ChargeModel` is `Tiered Pricing` or `Volume Pricing`, if this field is not specified, the value will default to `0`.
* @return defaultQuantity
*/
@javax.annotation.Nullable
public Double getDefaultQuantity() {
return defaultQuantity;
}
public void setDefaultQuantity(Double defaultQuantity) {
this.defaultQuantity = defaultQuantity;
}
public GetProductRatePlanChargeResponse deferredRevenueAccount(String deferredRevenueAccount) {
this.deferredRevenueAccount = deferredRevenueAccount;
return this;
}
/**
* The name of the deferred revenue account for this charge. This feature is in **Limited Availability**. If you wish to have access to the feature, submit a request at [Zuora Global Support](http://support.zuora.com/).
* @return deferredRevenueAccount
*/
@javax.annotation.Nullable
public String getDeferredRevenueAccount() {
return deferredRevenueAccount;
}
public void setDeferredRevenueAccount(String deferredRevenueAccount) {
this.deferredRevenueAccount = deferredRevenueAccount;
}
public GetProductRatePlanChargeResponse deliverySchedule(DeliveryScheduleProductRatePlanCharge deliverySchedule) {
this.deliverySchedule = deliverySchedule;
return this;
}
/**
* Get deliverySchedule
* @return deliverySchedule
*/
@javax.annotation.Nullable
public DeliveryScheduleProductRatePlanCharge getDeliverySchedule() {
return deliverySchedule;
}
public void setDeliverySchedule(DeliveryScheduleProductRatePlanCharge deliverySchedule) {
this.deliverySchedule = deliverySchedule;
}
public GetProductRatePlanChargeResponse description(String description) {
this.description = description;
return this;
}
/**
* A description of the charge.
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public GetProductRatePlanChargeResponse discountLevel(DiscountLevel discountLevel) {
this.discountLevel = discountLevel;
return this;
}
/**
* Get discountLevel
* @return discountLevel
*/
@javax.annotation.Nullable
public DiscountLevel getDiscountLevel() {
return discountLevel;
}
public void setDiscountLevel(DiscountLevel discountLevel) {
this.discountLevel = discountLevel;
}
public GetProductRatePlanChargeResponse drawdownRate(BigDecimal drawdownRate) {
this.drawdownRate = drawdownRate;
return this;
}
/**
* **Note**: This field is only available if you have the [Prepaid with Drawdown](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown) feature enabled. The [conversion rate](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown/Create_drawdown_charge#UOM_Conversion) between Usage UOM and Drawdown UOM for a [drawdown charge](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown/Create_drawdown_charge). Must be a positive number (>0).
* @return drawdownRate
*/
@javax.annotation.Nullable
public BigDecimal getDrawdownRate() {
return drawdownRate;
}
public void setDrawdownRate(BigDecimal drawdownRate) {
this.drawdownRate = drawdownRate;
}
public GetProductRatePlanChargeResponse drawdownUom(String drawdownUom) {
this.drawdownUom = drawdownUom;
return this;
}
/**
* **Note**: This field is only available if you have the [Prepaid with Drawdown](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown) feature enabled. Unit of measurement for a [drawdown charge](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown/Create_drawdown_charge).
* @return drawdownUom
*/
@javax.annotation.Nullable
public String getDrawdownUom() {
return drawdownUom;
}
public void setDrawdownUom(String drawdownUom) {
this.drawdownUom = drawdownUom;
}
public GetProductRatePlanChargeResponse endDateCondition(EndDateConditionProductRatePlanChargeRest endDateCondition) {
this.endDateCondition = endDateCondition;
return this;
}
/**
* Get endDateCondition
* @return endDateCondition
*/
@javax.annotation.Nullable
public EndDateConditionProductRatePlanChargeRest getEndDateCondition() {
return endDateCondition;
}
public void setEndDateCondition(EndDateConditionProductRatePlanChargeRest endDateCondition) {
this.endDateCondition = endDateCondition;
}
public GetProductRatePlanChargeResponse excludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
return this;
}
/**
* The flag to exclude the related invoice items, invoice item adjustments, credit memo items, and debit memo items from revenue accounting. **Notes**: - To use this field, you must set the `X-Zuora-WSDL-Version` request header to `115` or later. Otherwise, an error occurs. - This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return excludeItemBillingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBillingFromRevenueAccounting() {
return excludeItemBillingFromRevenueAccounting;
}
public void setExcludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
}
public GetProductRatePlanChargeResponse excludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
return this;
}
/**
* The flag to exclude the related rate plan charges and order line items from revenue accounting. **Notes**: - To use this field, you must set the `X-Zuora-WSDL-Version` request header to `115` or later. Otherwise, an error occurs. - This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return excludeItemBookingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBookingFromRevenueAccounting() {
return excludeItemBookingFromRevenueAccounting;
}
public void setExcludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
}
public GetProductRatePlanChargeResponse includedUnits(Double includedUnits) {
this.includedUnits = includedUnits;
return this;
}
/**
* Specifies the number of units in the base set of units. **Character limit**: 16 **Values**: a positive decimal value
* @return includedUnits
*/
@javax.annotation.Nullable
public Double getIncludedUnits() {
return includedUnits;
}
public void setIncludedUnits(Double includedUnits) {
this.includedUnits = includedUnits;
}
public GetProductRatePlanChargeResponse isPrepaid(Boolean isPrepaid) {
this.isPrepaid = isPrepaid;
return this;
}
/**
* **Note**: This field is only available if you have the [Prepaid with Drawdown](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown) feature enabled. Indicates whether this charge is a prepayment (topup) charge or a drawdown charge. Values: `true` or `false`.
* @return isPrepaid
*/
@javax.annotation.Nullable
public Boolean getIsPrepaid() {
return isPrepaid;
}
public void setIsPrepaid(Boolean isPrepaid) {
this.isPrepaid = isPrepaid;
}
public GetProductRatePlanChargeResponse isRollover(Boolean isRollover) {
this.isRollover = isRollover;
return this;
}
/**
* **Note**: This field is only available if you have the [Prepaid with Drawdown](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown) feature enabled. To use this field, you must set the `X-Zuora-WSDL-Version` request header to 114 or higher. Otherwise, an error occurs. The value is either \"True\" or \"False\". It determines whether the rollover fields are needed.
* @return isRollover
*/
@javax.annotation.Nullable
public Boolean getIsRollover() {
return isRollover;
}
public void setIsRollover(Boolean isRollover) {
this.isRollover = isRollover;
}
public GetProductRatePlanChargeResponse isStackedDiscount(Boolean isStackedDiscount) {
this.isStackedDiscount = isStackedDiscount;
return this;
}
/**
* **Note**: This field is only applicable to the Discount - Percentage charge model. To use this field, you must set the `X-Zuora-WSDL-Version` request header to 130 or higher. Otherwise, an error occurs. This field indicates whether the discount is to be calculated as stacked discount. Possible values are as follows: - `true`: This is a stacked discount, which should be calculated by stacking with other discounts. - `false`: This is not a stacked discount, which should be calculated in sequence with other discounts. For more information, see [Stacked discounts](https://knowledgecenter.zuora.com/Zuora_Billing/Products/Product_Catalog/B_Charge_Models/B_Discount_Charge_Models).
* @return isStackedDiscount
*/
@javax.annotation.Nullable
public Boolean getIsStackedDiscount() {
return isStackedDiscount;
}
public void setIsStackedDiscount(Boolean isStackedDiscount) {
this.isStackedDiscount = isStackedDiscount;
}
public GetProductRatePlanChargeResponse isUnbilled(Boolean isUnbilled) {
this.isUnbilled = isUnbilled;
return this;
}
/**
* This field is used to dictate how to perform the accounting during revenue recognition. **Note**: This feature is in the **Early Adopter** phase. If you want to use the feature, submit a request at <a href=\"https://support.zuora.com/\" target=\"_blank\">Zuora Global Support</a>, and we will evaluate whether the feature is suitable for your use cases.
* @return isUnbilled
*/
@javax.annotation.Nullable
public Boolean getIsUnbilled() {
return isUnbilled;
}
public void setIsUnbilled(Boolean isUnbilled) {
this.isUnbilled = isUnbilled;
}
public GetProductRatePlanChargeResponse revenueRecognitionTiming(String revenueRecognitionTiming) {
this.revenueRecognitionTiming = revenueRecognitionTiming;
return this;
}
/**
* This field is used to dictate the type of revenue recognition timing.
* @return revenueRecognitionTiming
*/
@javax.annotation.Nullable
public String getRevenueRecognitionTiming() {
return revenueRecognitionTiming;
}
public void setRevenueRecognitionTiming(String revenueRecognitionTiming) {
this.revenueRecognitionTiming = revenueRecognitionTiming;
}
public GetProductRatePlanChargeResponse revenueAmortizationMethod(String revenueAmortizationMethod) {
this.revenueAmortizationMethod = revenueAmortizationMethod;
return this;
}
/**
* This field is used to dictate the type of revenue amortization method.
* @return revenueAmortizationMethod
*/
@javax.annotation.Nullable
public String getRevenueAmortizationMethod() {
return revenueAmortizationMethod;
}
public void setRevenueAmortizationMethod(String revenueAmortizationMethod) {
this.revenueAmortizationMethod = revenueAmortizationMethod;
}
public GetProductRatePlanChargeResponse legacyRevenueReporting(Boolean legacyRevenueReporting) {
this.legacyRevenueReporting = legacyRevenueReporting;
return this;
}
/**
*
* @return legacyRevenueReporting
*/
@javax.annotation.Nullable
public Boolean getLegacyRevenueReporting() {
return legacyRevenueReporting;
}
public void setLegacyRevenueReporting(Boolean legacyRevenueReporting) {
this.legacyRevenueReporting = legacyRevenueReporting;
}
public GetProductRatePlanChargeResponse listPriceBase(ListPriceBase listPriceBase) {
this.listPriceBase = listPriceBase;
return this;
}
/**
* Get listPriceBase
* @return listPriceBase
*/
@javax.annotation.Nullable
public ListPriceBase getListPriceBase() {
return listPriceBase;
}
public void setListPriceBase(ListPriceBase listPriceBase) {
this.listPriceBase = listPriceBase;
}
public GetProductRatePlanChargeResponse minQuantity(Double minQuantity) {
this.minQuantity = minQuantity;
return this;
}
/**
* Specifies the minimum number of units for this charge. Use this field and the `MaxQuantity` field to create a range of units allowed in a product rate plan charge. **Character limit**: 16 **Values**: a positive decimal value
* @return minQuantity
*/
@javax.annotation.Nullable
public Double getMinQuantity() {
return minQuantity;
}
public void setMinQuantity(Double minQuantity) {
this.minQuantity = minQuantity;
}
public GetProductRatePlanChargeResponse maxQuantity(Double maxQuantity) {
this.maxQuantity = maxQuantity;
return this;
}
/**
* Specifies the maximum number of units for this charge. Use this field and the `MinQuantity` field to create a range of units allowed in a product rate plan charge. **Character limit**: 16 **Values**: a positive decimal value
* @return maxQuantity
*/
@javax.annotation.Nullable
public Double getMaxQuantity() {
return maxQuantity;
}
public void setMaxQuantity(Double maxQuantity) {
this.maxQuantity = maxQuantity;
}
public GetProductRatePlanChargeResponse name(String name) {
this.name = name;
return this;
}
/**
* The name of the product rate plan charge.
* @return name
*/
@javax.annotation.Nullable
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public GetProductRatePlanChargeResponse numberOfPeriod(Long numberOfPeriod) {
this.numberOfPeriod = numberOfPeriod;
return this;
}
/**
* Specifies the number of periods to use when calculating charges in an overage smoothing charge model. The valid value is a positive whole number.
* @return numberOfPeriod
*/
@javax.annotation.Nullable
public Long getNumberOfPeriod() {
return numberOfPeriod;
}
public void setNumberOfPeriod(Long numberOfPeriod) {
this.numberOfPeriod = numberOfPeriod;
}
public GetProductRatePlanChargeResponse overageCalculationOption(OverageCalculationOption overageCalculationOption) {
this.overageCalculationOption = overageCalculationOption;
return this;
}
/**
* Get overageCalculationOption
* @return overageCalculationOption
*/
@javax.annotation.Nullable
public OverageCalculationOption getOverageCalculationOption() {
return overageCalculationOption;
}
public void setOverageCalculationOption(OverageCalculationOption overageCalculationOption) {
this.overageCalculationOption = overageCalculationOption;
}
public GetProductRatePlanChargeResponse overageUnusedUnitsCreditOption(OverageUnusedUnitsCreditOption overageUnusedUnitsCreditOption) {
this.overageUnusedUnitsCreditOption = overageUnusedUnitsCreditOption;
return this;
}
/**
* Get overageUnusedUnitsCreditOption
* @return overageUnusedUnitsCreditOption
*/
@javax.annotation.Nullable
public OverageUnusedUnitsCreditOption getOverageUnusedUnitsCreditOption() {
return overageUnusedUnitsCreditOption;
}
public void setOverageUnusedUnitsCreditOption(OverageUnusedUnitsCreditOption overageUnusedUnitsCreditOption) {
this.overageUnusedUnitsCreditOption = overageUnusedUnitsCreditOption;
}
public GetProductRatePlanChargeResponse prepaidOperationType(PrepaidOperationType prepaidOperationType) {
this.prepaidOperationType = prepaidOperationType;
return this;
}
/**
* Get prepaidOperationType
* @return prepaidOperationType
*/
@javax.annotation.Nullable
public PrepaidOperationType getPrepaidOperationType() {
return prepaidOperationType;
}
public void setPrepaidOperationType(PrepaidOperationType prepaidOperationType) {
this.prepaidOperationType = prepaidOperationType;
}
public GetProductRatePlanChargeResponse prepaidQuantity(BigDecimal prepaidQuantity) {
this.prepaidQuantity = prepaidQuantity;
return this;
}
/**
* **Note**: This field is only available if you have the [Prepaid with Drawdown](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown) feature enabled. The number of units included in a [prepayment charge](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown/Create_prepayment_charge). Must be a positive number (>0).
* @return prepaidQuantity
*/
@javax.annotation.Nullable
public BigDecimal getPrepaidQuantity() {
return prepaidQuantity;
}
public void setPrepaidQuantity(BigDecimal prepaidQuantity) {
this.prepaidQuantity = prepaidQuantity;
}
public GetProductRatePlanChargeResponse prepaidTotalQuantity(BigDecimal prepaidTotalQuantity) {
this.prepaidTotalQuantity = prepaidTotalQuantity;
return this;
}
/**
* **Note**: This field is only available if you have the [Prepaid with Drawdown](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown) feature enabled. The total amount of units that end customers can use during a validity period when they subscribe to a [prepayment charge](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown/Create_prepayment_charge).
* @return prepaidTotalQuantity
*/
@javax.annotation.Nullable
public BigDecimal getPrepaidTotalQuantity() {
return prepaidTotalQuantity;
}
public void setPrepaidTotalQuantity(BigDecimal prepaidTotalQuantity) {
this.prepaidTotalQuantity = prepaidTotalQuantity;
}
public GetProductRatePlanChargeResponse prepaidUom(String prepaidUom) {
this.prepaidUom = prepaidUom;
return this;
}
/**
* **Note**: This field is only available if you have the [Prepaid with Drawdown](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown) feature enabled. Unit of measurement for a [prepayment charge](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown/Create_prepayment_charge).
* @return prepaidUom
*/
@javax.annotation.Nullable
public String getPrepaidUom() {
return prepaidUom;
}
public void setPrepaidUom(String prepaidUom) {
this.prepaidUom = prepaidUom;
}
public GetProductRatePlanChargeResponse prepayPeriods(Long prepayPeriods) {
this.prepayPeriods = prepayPeriods;
return this;
}
/**
* The number of periods to which prepayment is set. **Note:** This field is only available if you already have the prepayment feature enabled. The prepayment feature is deprecated and available only for backward compatibility. Zuora does not support enabling this feature anymore.
* @return prepayPeriods
*/
@javax.annotation.Nullable
public Long getPrepayPeriods() {
return prepayPeriods;
}
public void setPrepayPeriods(Long prepayPeriods) {
this.prepayPeriods = prepayPeriods;
}
public GetProductRatePlanChargeResponse priceChangeOption(PriceChangeOption priceChangeOption) {
this.priceChangeOption = priceChangeOption;
return this;
}
/**
* Get priceChangeOption
* @return priceChangeOption
*/
@javax.annotation.Nullable
public PriceChangeOption getPriceChangeOption() {
return priceChangeOption;
}
public void setPriceChangeOption(PriceChangeOption priceChangeOption) {
this.priceChangeOption = priceChangeOption;
}
public GetProductRatePlanChargeResponse priceIncreasePercentage(Double priceIncreasePercentage) {
this.priceIncreasePercentage = priceIncreasePercentage;
return this;
}
/**
* Specifies the percentage to increase or decrease the price of a termed subscription's renewal. Use this field if you set the value to `SpecificPercentageValue`. **Character limit**: 16 **Values**: a decimal value between -100 and 100
* @return priceIncreasePercentage
*/
@javax.annotation.Nullable
public Double getPriceIncreasePercentage() {
return priceIncreasePercentage;
}
public void setPriceIncreasePercentage(Double priceIncreasePercentage) {
this.priceIncreasePercentage = priceIncreasePercentage;
}
public GetProductRatePlanChargeResponse priceIncreaseOption(PriceIncreaseOption priceIncreaseOption) {
this.priceIncreaseOption = priceIncreaseOption;
return this;
}
/**
* Get priceIncreaseOption
* @return priceIncreaseOption
*/
@javax.annotation.Nullable
public PriceIncreaseOption getPriceIncreaseOption() {
return priceIncreaseOption;
}
public void setPriceIncreaseOption(PriceIncreaseOption priceIncreaseOption) {
this.priceIncreaseOption = priceIncreaseOption;
}
public GetProductRatePlanChargeResponse ratingGroup(RatingGroup ratingGroup) {
this.ratingGroup = ratingGroup;
return this;
}
/**
* Get ratingGroup
* @return ratingGroup
*/
@javax.annotation.Nullable
public RatingGroup getRatingGroup() {
return ratingGroup;
}
public void setRatingGroup(RatingGroup ratingGroup) {
this.ratingGroup = ratingGroup;
}
public GetProductRatePlanChargeResponse recognizedRevenueAccount(String recognizedRevenueAccount) {
this.recognizedRevenueAccount = recognizedRevenueAccount;
return this;
}
/**
* The name of the recognized revenue account for this charge. - Required when the Allow Blank Accounting Code setting is No. - Optional when the Allow Blank Accounting Code setting is Yes. This feature is in **Limited Availability**. If you wish to have access to the feature, submit a request at [Zuora Global Support](http://support.zuora.com/).
* @return recognizedRevenueAccount
*/
@javax.annotation.Nullable
public String getRecognizedRevenueAccount() {
return recognizedRevenueAccount;
}
public void setRecognizedRevenueAccount(String recognizedRevenueAccount) {
this.recognizedRevenueAccount = recognizedRevenueAccount;
}
public GetProductRatePlanChargeResponse revRecCode(String revRecCode) {
this.revRecCode = revRecCode;
return this;
}
/**
* Associates this product rate plan charge with a specific revenue recognition code.
* @return revRecCode
*/
@javax.annotation.Nullable
public String getRevRecCode() {
return revRecCode;
}
public void setRevRecCode(String revRecCode) {
this.revRecCode = revRecCode;
}
public GetProductRatePlanChargeResponse revRecTriggerCondition(RevRecTriggerConditionProductRatePlanChargeRest revRecTriggerCondition) {
this.revRecTriggerCondition = revRecTriggerCondition;
return this;
}
/**
* Get revRecTriggerCondition
* @return revRecTriggerCondition
*/
@javax.annotation.Nullable
public RevRecTriggerConditionProductRatePlanChargeRest getRevRecTriggerCondition() {
return revRecTriggerCondition;
}
public void setRevRecTriggerCondition(RevRecTriggerConditionProductRatePlanChargeRest revRecTriggerCondition) {
this.revRecTriggerCondition = revRecTriggerCondition;
}
public GetProductRatePlanChargeResponse revenueRecognitionRuleName(RevenueRecognitionRuleName revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
return this;
}
/**
* Get revenueRecognitionRuleName
* @return revenueRecognitionRuleName
*/
@javax.annotation.Nullable
public RevenueRecognitionRuleName getRevenueRecognitionRuleName() {
return revenueRecognitionRuleName;
}
public void setRevenueRecognitionRuleName(RevenueRecognitionRuleName revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
}
public GetProductRatePlanChargeResponse rolloverApply(RolloverApply rolloverApply) {
this.rolloverApply = rolloverApply;
return this;
}
/**
* Get rolloverApply
* @return rolloverApply
*/
@javax.annotation.Nullable
public RolloverApply getRolloverApply() {
return rolloverApply;
}
public void setRolloverApply(RolloverApply rolloverApply) {
this.rolloverApply = rolloverApply;
}
public GetProductRatePlanChargeResponse rolloverPeriods(BigDecimal rolloverPeriods) {
this.rolloverPeriods = rolloverPeriods;
return this;
}
/**
* **Note**: This field is only available if you have the [Prepaid with Drawdown](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown) feature enabled. To use this field, you must set the `X-Zuora-WSDL-Version` request header to 114 or higher. Otherwise, an error occurs. This field defines the number of rollover periods, it is restricted to 3.
* @return rolloverPeriods
*/
@javax.annotation.Nullable
public BigDecimal getRolloverPeriods() {
return rolloverPeriods;
}
public void setRolloverPeriods(BigDecimal rolloverPeriods) {
this.rolloverPeriods = rolloverPeriods;
}
public GetProductRatePlanChargeResponse smoothingModel(SmoothingModel smoothingModel) {
this.smoothingModel = smoothingModel;
return this;
}
/**
* Get smoothingModel
* @return smoothingModel
*/
@javax.annotation.Nullable
public SmoothingModel getSmoothingModel() {
return smoothingModel;
}
public void setSmoothingModel(SmoothingModel smoothingModel) {
this.smoothingModel = smoothingModel;
}
public GetProductRatePlanChargeResponse specificBillingPeriod(Long specificBillingPeriod) {
this.specificBillingPeriod = specificBillingPeriod;
return this;
}
/**
* Customizes the number of months or weeks for the charges billing period. This field is required if you set the value of the BillingPeriod field to `Specific Months` or `Specific Weeks`. The valid value is a positive integer.
* @return specificBillingPeriod
*/
@javax.annotation.Nullable
public Long getSpecificBillingPeriod() {
return specificBillingPeriod;
}
public void setSpecificBillingPeriod(Long specificBillingPeriod) {
this.specificBillingPeriod = specificBillingPeriod;
}
public GetProductRatePlanChargeResponse specificListPriceBase(Object specificListPriceBase) {
this.specificListPriceBase = specificListPriceBase;
return this;
}
/**
* The number of months for the list price base of the charge. The value of this field is `null` if you do not set the value of the `ListPriceBase` field to `Per Specific Months`. **Notes**: - This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Billing/Subscriptions/Product_Catalog/I_Annual_List_Price\" target=\"_blank\">Annual List Price</a> feature enabled. - To use this field, you must set the `X-Zuora-WSDL-Version` request header to `129` or later. Otherwise, an error occurs. - The value of this field is `null` if you do not set the value of the `ListPriceBase` field to `Per Specific Months`.
* @return specificListPriceBase
*/
@javax.annotation.Nullable
public Object getSpecificListPriceBase() {
return specificListPriceBase;
}
public void setSpecificListPriceBase(Object specificListPriceBase) {
this.specificListPriceBase = specificListPriceBase;
}
public GetProductRatePlanChargeResponse taxCode(String taxCode) {
this.taxCode = taxCode;
return this;
}
/**
* Specifies the tax code for taxation rules. Required when the Taxable field is set to `True`. **Note**: This value affects the tax calculation of rate plan charges that come from the `ProductRatePlanCharge`.
* @return taxCode
*/
@javax.annotation.Nullable
public String getTaxCode() {
return taxCode;
}
public void setTaxCode(String taxCode) {
this.taxCode = taxCode;
}
public GetProductRatePlanChargeResponse taxMode(TaxMode taxMode) {
this.taxMode = taxMode;
return this;
}
/**
* Get taxMode
* @return taxMode
*/
@javax.annotation.Nullable
public TaxMode getTaxMode() {
return taxMode;
}
public void setTaxMode(TaxMode taxMode) {
this.taxMode = taxMode;
}
public GetProductRatePlanChargeResponse taxable(Boolean taxable) {
this.taxable = taxable;
return this;
}
/**
* Determines whether the charge is taxable. When set to `True`, the TaxMode and TaxCode fields are required when creating or updating th ProductRatePlanCharge object. **Character limit**: 5 **Values**: `True`, `False` **Note**: This value affects the tax calculation of rate plan charges that come from the `ProductRatePlanCharge`.
* @return taxable
*/
@javax.annotation.Nullable
public Boolean getTaxable() {
return taxable;
}
public void setTaxable(Boolean taxable) {
this.taxable = taxable;
}
public GetProductRatePlanChargeResponse triggerEvent(TriggerEventProductRatePlanChargeRest triggerEvent) {
this.triggerEvent = triggerEvent;
return this;
}
/**
* Get triggerEvent
* @return triggerEvent
*/
@javax.annotation.Nullable
public TriggerEventProductRatePlanChargeRest getTriggerEvent() {
return triggerEvent;
}
public void setTriggerEvent(TriggerEventProductRatePlanChargeRest triggerEvent) {
this.triggerEvent = triggerEvent;
}
public GetProductRatePlanChargeResponse UOM(String UOM) {
this.UOM = UOM;
return this;
}
/**
* Specifies the units to measure usage. **Note**: You must specify this field when creating the following charge models: - Per Unit Pricing - Volume Pricin - Overage Pricing - Tiered Pricing - Tiered with Overage Pricing
* @return UOM
*/
@javax.annotation.Nullable
public String getUOM() {
return UOM;
}
public void setUOM(String UOM) {
this.UOM = UOM;
}
public GetProductRatePlanChargeResponse upToPeriods(Long upToPeriods) {
this.upToPeriods = upToPeriods;
return this;
}
/**
* Specifies the length of the period during which the charge is active. If this period ends before the subscription ends, the charge ends when this period ends. **Character limit**: 5 **Values**: a whole number between 0 and 65535, exclusive **Notes**: - You must use this field together with the `UpToPeriodsType` field to specify the time period. This field is applicable only when the `EndDateCondition` field is set to `FixedPeriod`. - If the subscription end date is subsequently changed through a Renewal, or Terms and Conditions amendment, the charge end date will change accordingly up to the original period end.
* @return upToPeriods
*/
@javax.annotation.Nullable
public Long getUpToPeriods() {
return upToPeriods;
}
public void setUpToPeriods(Long upToPeriods) {
this.upToPeriods = upToPeriods;
}
public GetProductRatePlanChargeResponse upToPeriodsType(UpToPeriodsTypeProductRatePlanChargeRest upToPeriodsType) {
this.upToPeriodsType = upToPeriodsType;
return this;
}
/**
* Get upToPeriodsType
* @return upToPeriodsType
*/
@javax.annotation.Nullable
public UpToPeriodsTypeProductRatePlanChargeRest getUpToPeriodsType() {
return upToPeriodsType;
}
public void setUpToPeriodsType(UpToPeriodsTypeProductRatePlanChargeRest upToPeriodsType) {
this.upToPeriodsType = upToPeriodsType;
}
public GetProductRatePlanChargeResponse updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* The ID of the last user to update the object.
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public GetProductRatePlanChargeResponse updatedDate(OffsetDateTime updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* The date when the object was last updated.
* @return updatedDate
*/
@javax.annotation.Nullable
public OffsetDateTime getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(OffsetDateTime updatedDate) {
this.updatedDate = updatedDate;
}
public GetProductRatePlanChargeResponse usageRecordRatingOption(UsageRecordRatingOption usageRecordRatingOption) {
this.usageRecordRatingOption = usageRecordRatingOption;
return this;
}
/**
* Get usageRecordRatingOption
* @return usageRecordRatingOption
*/
@javax.annotation.Nullable
public UsageRecordRatingOption getUsageRecordRatingOption() {
return usageRecordRatingOption;
}
public void setUsageRecordRatingOption(UsageRecordRatingOption usageRecordRatingOption) {
this.usageRecordRatingOption = usageRecordRatingOption;
}
public GetProductRatePlanChargeResponse useDiscountSpecificAccountingCode(Boolean useDiscountSpecificAccountingCode) {
this.useDiscountSpecificAccountingCode = useDiscountSpecificAccountingCode;
return this;
}
/**
* Determines whether to define a new accounting code for the new discount charge. **Character limit**: 5 **Values**: `True`, `False`
* @return useDiscountSpecificAccountingCode
*/
@javax.annotation.Nullable
public Boolean getUseDiscountSpecificAccountingCode() {
return useDiscountSpecificAccountingCode;
}
public void setUseDiscountSpecificAccountingCode(Boolean useDiscountSpecificAccountingCode) {
this.useDiscountSpecificAccountingCode = useDiscountSpecificAccountingCode;
}
public GetProductRatePlanChargeResponse useTenantDefaultForPriceChange(Boolean useTenantDefaultForPriceChange) {
this.useTenantDefaultForPriceChange = useTenantDefaultForPriceChange;
return this;
}
/**
* Applies the tenant-level percentage uplift value for an automatic price change to a termed subscription's renewal. **Character limit**: 5 **Values**: `true`, `false`
* @return useTenantDefaultForPriceChange
*/
@javax.annotation.Nullable
public Boolean getUseTenantDefaultForPriceChange() {
return useTenantDefaultForPriceChange;
}
public void setUseTenantDefaultForPriceChange(Boolean useTenantDefaultForPriceChange) {
this.useTenantDefaultForPriceChange = useTenantDefaultForPriceChange;
}
public GetProductRatePlanChargeResponse validityPeriodType(ValidityPeriodType validityPeriodType) {
this.validityPeriodType = validityPeriodType;
return this;
}
/**
* Get validityPeriodType
* @return validityPeriodType
*/
@javax.annotation.Nullable
public ValidityPeriodType getValidityPeriodType() {
return validityPeriodType;
}
public void setValidityPeriodType(ValidityPeriodType validityPeriodType) {
this.validityPeriodType = validityPeriodType;
}
public GetProductRatePlanChargeResponse weeklyBillCycleDay(WeeklyBillCycleDay weeklyBillCycleDay) {
this.weeklyBillCycleDay = weeklyBillCycleDay;
return this;
}
/**
* Get weeklyBillCycleDay
* @return weeklyBillCycleDay
*/
@javax.annotation.Nullable
public WeeklyBillCycleDay getWeeklyBillCycleDay() {
return weeklyBillCycleDay;
}
public void setWeeklyBillCycleDay(WeeklyBillCycleDay weeklyBillCycleDay) {
this.weeklyBillCycleDay = weeklyBillCycleDay;
}
public GetProductRatePlanChargeResponse ratingGroupsOperatorType(RatingGroupsOperatorType ratingGroupsOperatorType) {
this.ratingGroupsOperatorType = ratingGroupsOperatorType;
return this;
}
/**
* Get ratingGroupsOperatorType
* @return ratingGroupsOperatorType
*/
@javax.annotation.Nullable
public RatingGroupsOperatorType getRatingGroupsOperatorType() {
return ratingGroupsOperatorType;
}
public void setRatingGroupsOperatorType(RatingGroupsOperatorType ratingGroupsOperatorType) {
this.ratingGroupsOperatorType = ratingGroupsOperatorType;
}
public GetProductRatePlanChargeResponse classNS(String classNS) {
this.classNS = classNS;
return this;
}
/**
* Class associated with the corresponding item in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return classNS
*/
@javax.annotation.Nullable
public String getClassNS() {
return classNS;
}
public void setClassNS(String classNS) {
this.classNS = classNS;
}
public GetProductRatePlanChargeResponse deferredRevAccountNS(String deferredRevAccountNS) {
this.deferredRevAccountNS = deferredRevAccountNS;
return this;
}
/**
* Deferrred revenue account associated with the corresponding item in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return deferredRevAccountNS
*/
@javax.annotation.Nullable
public String getDeferredRevAccountNS() {
return deferredRevAccountNS;
}
public void setDeferredRevAccountNS(String deferredRevAccountNS) {
this.deferredRevAccountNS = deferredRevAccountNS;
}
public GetProductRatePlanChargeResponse departmentNS(String departmentNS) {
this.departmentNS = departmentNS;
return this;
}
/**
* Department associated with the corresponding item in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return departmentNS
*/
@javax.annotation.Nullable
public String getDepartmentNS() {
return departmentNS;
}
public void setDepartmentNS(String departmentNS) {
this.departmentNS = departmentNS;
}
public GetProductRatePlanChargeResponse includeChildrenNS(ProductRatePlanChargeObjectNSFieldsIncludeChildrenNS includeChildrenNS) {
this.includeChildrenNS = includeChildrenNS;
return this;
}
/**
* Get includeChildrenNS
* @return includeChildrenNS
*/
@javax.annotation.Nullable
public ProductRatePlanChargeObjectNSFieldsIncludeChildrenNS getIncludeChildrenNS() {
return includeChildrenNS;
}
public void setIncludeChildrenNS(ProductRatePlanChargeObjectNSFieldsIncludeChildrenNS includeChildrenNS) {
this.includeChildrenNS = includeChildrenNS;
}
public GetProductRatePlanChargeResponse integrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
return this;
}
/**
* ID of the corresponding object in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationIdNS
*/
@javax.annotation.Nullable
public String getIntegrationIdNS() {
return integrationIdNS;
}
public void setIntegrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
}
public GetProductRatePlanChargeResponse integrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
return this;
}
/**
* Status of the product rate plan charge's synchronization with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationStatusNS
*/
@javax.annotation.Nullable
public String getIntegrationStatusNS() {
return integrationStatusNS;
}
public void setIntegrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
}
public GetProductRatePlanChargeResponse itemTypeNS(ProductRatePlanChargeObjectNSFieldsItemTypeNS itemTypeNS) {
this.itemTypeNS = itemTypeNS;
return this;
}
/**
* Get itemTypeNS
* @return itemTypeNS
*/
@javax.annotation.Nullable
public ProductRatePlanChargeObjectNSFieldsItemTypeNS getItemTypeNS() {
return itemTypeNS;
}
public void setItemTypeNS(ProductRatePlanChargeObjectNSFieldsItemTypeNS itemTypeNS) {
this.itemTypeNS = itemTypeNS;
}
public GetProductRatePlanChargeResponse locationNS(String locationNS) {
this.locationNS = locationNS;
return this;
}
/**
* Location associated with the corresponding item in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return locationNS
*/
@javax.annotation.Nullable
public String getLocationNS() {
return locationNS;
}
public void setLocationNS(String locationNS) {
this.locationNS = locationNS;
}
public GetProductRatePlanChargeResponse recognizedRevAccountNS(String recognizedRevAccountNS) {
this.recognizedRevAccountNS = recognizedRevAccountNS;
return this;
}
/**
* Recognized revenue account associated with the corresponding item in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return recognizedRevAccountNS
*/
@javax.annotation.Nullable
public String getRecognizedRevAccountNS() {
return recognizedRevAccountNS;
}
public void setRecognizedRevAccountNS(String recognizedRevAccountNS) {
this.recognizedRevAccountNS = recognizedRevAccountNS;
}
public GetProductRatePlanChargeResponse revRecEndNS(ProductRatePlanChargeObjectNSFieldsRevRecEndNS revRecEndNS) {
this.revRecEndNS = revRecEndNS;
return this;
}
/**
* Get revRecEndNS
* @return revRecEndNS
*/
@javax.annotation.Nullable
public ProductRatePlanChargeObjectNSFieldsRevRecEndNS getRevRecEndNS() {
return revRecEndNS;
}
public void setRevRecEndNS(ProductRatePlanChargeObjectNSFieldsRevRecEndNS revRecEndNS) {
this.revRecEndNS = revRecEndNS;
}
public GetProductRatePlanChargeResponse revRecStartNS(ProductRatePlanChargeObjectNSFieldsRevRecStartNS revRecStartNS) {
this.revRecStartNS = revRecStartNS;
return this;
}
/**
* Get revRecStartNS
* @return revRecStartNS
*/
@javax.annotation.Nullable
public ProductRatePlanChargeObjectNSFieldsRevRecStartNS getRevRecStartNS() {
return revRecStartNS;
}
public void setRevRecStartNS(ProductRatePlanChargeObjectNSFieldsRevRecStartNS revRecStartNS) {
this.revRecStartNS = revRecStartNS;
}
public GetProductRatePlanChargeResponse revRecTemplateTypeNS(String revRecTemplateTypeNS) {
this.revRecTemplateTypeNS = revRecTemplateTypeNS;
return this;
}
/**
* Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return revRecTemplateTypeNS
*/
@javax.annotation.Nullable
public String getRevRecTemplateTypeNS() {
return revRecTemplateTypeNS;
}
public void setRevRecTemplateTypeNS(String revRecTemplateTypeNS) {
this.revRecTemplateTypeNS = revRecTemplateTypeNS;
}
public GetProductRatePlanChargeResponse subsidiaryNS(String subsidiaryNS) {
this.subsidiaryNS = subsidiaryNS;
return this;
}
/**
* Subsidiary associated with the corresponding item in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return subsidiaryNS
*/
@javax.annotation.Nullable
public String getSubsidiaryNS() {
return subsidiaryNS;
}
public void setSubsidiaryNS(String subsidiaryNS) {
this.subsidiaryNS = subsidiaryNS;
}
public GetProductRatePlanChargeResponse syncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
return this;
}
/**
* Date when the product rate plan charge was synchronized with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return syncDateNS
*/
@javax.annotation.Nullable
public String getSyncDateNS() {
return syncDateNS;
}
public void setSyncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the GetProductRatePlanChargeResponse instance itself
*/
public GetProductRatePlanChargeResponse putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GetProductRatePlanChargeResponse getProductRatePlanChargeResponse = (GetProductRatePlanChargeResponse) o;
return Objects.equals(this.id, getProductRatePlanChargeResponse.id) &&
Objects.equals(this.productRatePlanId, getProductRatePlanChargeResponse.productRatePlanId) &&
Objects.equals(this.accountingCode, getProductRatePlanChargeResponse.accountingCode) &&
Objects.equals(this.applyDiscountTo, getProductRatePlanChargeResponse.applyDiscountTo) &&
Objects.equals(this.billCycleDay, getProductRatePlanChargeResponse.billCycleDay) &&
Objects.equals(this.billCycleType, getProductRatePlanChargeResponse.billCycleType) &&
Objects.equals(this.billingPeriod, getProductRatePlanChargeResponse.billingPeriod) &&
Objects.equals(this.billingPeriodAlignment, getProductRatePlanChargeResponse.billingPeriodAlignment) &&
Objects.equals(this.billingTiming, getProductRatePlanChargeResponse.billingTiming) &&
Objects.equals(this.chargeFunction, getProductRatePlanChargeResponse.chargeFunction) &&
Objects.equals(this.chargeModel, getProductRatePlanChargeResponse.chargeModel) &&
Objects.equals(this.chargeModelConfiguration, getProductRatePlanChargeResponse.chargeModelConfiguration) &&
Objects.equals(this.chargeType, getProductRatePlanChargeResponse.chargeType) &&
Objects.equals(this.commitmentType, getProductRatePlanChargeResponse.commitmentType) &&
Objects.equals(this.createdById, getProductRatePlanChargeResponse.createdById) &&
Objects.equals(this.createdDate, getProductRatePlanChargeResponse.createdDate) &&
Objects.equals(this.defaultQuantity, getProductRatePlanChargeResponse.defaultQuantity) &&
Objects.equals(this.deferredRevenueAccount, getProductRatePlanChargeResponse.deferredRevenueAccount) &&
Objects.equals(this.deliverySchedule, getProductRatePlanChargeResponse.deliverySchedule) &&
Objects.equals(this.description, getProductRatePlanChargeResponse.description) &&
Objects.equals(this.discountLevel, getProductRatePlanChargeResponse.discountLevel) &&
Objects.equals(this.drawdownRate, getProductRatePlanChargeResponse.drawdownRate) &&
Objects.equals(this.drawdownUom, getProductRatePlanChargeResponse.drawdownUom) &&
Objects.equals(this.endDateCondition, getProductRatePlanChargeResponse.endDateCondition) &&
Objects.equals(this.excludeItemBillingFromRevenueAccounting, getProductRatePlanChargeResponse.excludeItemBillingFromRevenueAccounting) &&
Objects.equals(this.excludeItemBookingFromRevenueAccounting, getProductRatePlanChargeResponse.excludeItemBookingFromRevenueAccounting) &&
Objects.equals(this.includedUnits, getProductRatePlanChargeResponse.includedUnits) &&
Objects.equals(this.isPrepaid, getProductRatePlanChargeResponse.isPrepaid) &&
Objects.equals(this.isRollover, getProductRatePlanChargeResponse.isRollover) &&
Objects.equals(this.isStackedDiscount, getProductRatePlanChargeResponse.isStackedDiscount) &&
Objects.equals(this.isUnbilled, getProductRatePlanChargeResponse.isUnbilled) &&
Objects.equals(this.revenueRecognitionTiming, getProductRatePlanChargeResponse.revenueRecognitionTiming) &&
Objects.equals(this.revenueAmortizationMethod, getProductRatePlanChargeResponse.revenueAmortizationMethod) &&
Objects.equals(this.legacyRevenueReporting, getProductRatePlanChargeResponse.legacyRevenueReporting) &&
Objects.equals(this.listPriceBase, getProductRatePlanChargeResponse.listPriceBase) &&
Objects.equals(this.minQuantity, getProductRatePlanChargeResponse.minQuantity) &&
Objects.equals(this.maxQuantity, getProductRatePlanChargeResponse.maxQuantity) &&
Objects.equals(this.name, getProductRatePlanChargeResponse.name) &&
Objects.equals(this.numberOfPeriod, getProductRatePlanChargeResponse.numberOfPeriod) &&
Objects.equals(this.overageCalculationOption, getProductRatePlanChargeResponse.overageCalculationOption) &&
Objects.equals(this.overageUnusedUnitsCreditOption, getProductRatePlanChargeResponse.overageUnusedUnitsCreditOption) &&
Objects.equals(this.prepaidOperationType, getProductRatePlanChargeResponse.prepaidOperationType) &&
Objects.equals(this.prepaidQuantity, getProductRatePlanChargeResponse.prepaidQuantity) &&
Objects.equals(this.prepaidTotalQuantity, getProductRatePlanChargeResponse.prepaidTotalQuantity) &&
Objects.equals(this.prepaidUom, getProductRatePlanChargeResponse.prepaidUom) &&
Objects.equals(this.prepayPeriods, getProductRatePlanChargeResponse.prepayPeriods) &&
Objects.equals(this.priceChangeOption, getProductRatePlanChargeResponse.priceChangeOption) &&
Objects.equals(this.priceIncreasePercentage, getProductRatePlanChargeResponse.priceIncreasePercentage) &&
Objects.equals(this.priceIncreaseOption, getProductRatePlanChargeResponse.priceIncreaseOption) &&
Objects.equals(this.ratingGroup, getProductRatePlanChargeResponse.ratingGroup) &&
Objects.equals(this.recognizedRevenueAccount, getProductRatePlanChargeResponse.recognizedRevenueAccount) &&
Objects.equals(this.revRecCode, getProductRatePlanChargeResponse.revRecCode) &&
Objects.equals(this.revRecTriggerCondition, getProductRatePlanChargeResponse.revRecTriggerCondition) &&
Objects.equals(this.revenueRecognitionRuleName, getProductRatePlanChargeResponse.revenueRecognitionRuleName) &&
Objects.equals(this.rolloverApply, getProductRatePlanChargeResponse.rolloverApply) &&
Objects.equals(this.rolloverPeriods, getProductRatePlanChargeResponse.rolloverPeriods) &&
Objects.equals(this.smoothingModel, getProductRatePlanChargeResponse.smoothingModel) &&
Objects.equals(this.specificBillingPeriod, getProductRatePlanChargeResponse.specificBillingPeriod) &&
Objects.equals(this.specificListPriceBase, getProductRatePlanChargeResponse.specificListPriceBase) &&
Objects.equals(this.taxCode, getProductRatePlanChargeResponse.taxCode) &&
Objects.equals(this.taxMode, getProductRatePlanChargeResponse.taxMode) &&
Objects.equals(this.taxable, getProductRatePlanChargeResponse.taxable) &&
Objects.equals(this.triggerEvent, getProductRatePlanChargeResponse.triggerEvent) &&
Objects.equals(this.UOM, getProductRatePlanChargeResponse.UOM) &&
Objects.equals(this.upToPeriods, getProductRatePlanChargeResponse.upToPeriods) &&
Objects.equals(this.upToPeriodsType, getProductRatePlanChargeResponse.upToPeriodsType) &&
Objects.equals(this.updatedById, getProductRatePlanChargeResponse.updatedById) &&
Objects.equals(this.updatedDate, getProductRatePlanChargeResponse.updatedDate) &&
Objects.equals(this.usageRecordRatingOption, getProductRatePlanChargeResponse.usageRecordRatingOption) &&
Objects.equals(this.useDiscountSpecificAccountingCode, getProductRatePlanChargeResponse.useDiscountSpecificAccountingCode) &&
Objects.equals(this.useTenantDefaultForPriceChange, getProductRatePlanChargeResponse.useTenantDefaultForPriceChange) &&
Objects.equals(this.validityPeriodType, getProductRatePlanChargeResponse.validityPeriodType) &&
Objects.equals(this.weeklyBillCycleDay, getProductRatePlanChargeResponse.weeklyBillCycleDay) &&
Objects.equals(this.ratingGroupsOperatorType, getProductRatePlanChargeResponse.ratingGroupsOperatorType) &&
Objects.equals(this.classNS, getProductRatePlanChargeResponse.classNS) &&
Objects.equals(this.deferredRevAccountNS, getProductRatePlanChargeResponse.deferredRevAccountNS) &&
Objects.equals(this.departmentNS, getProductRatePlanChargeResponse.departmentNS) &&
Objects.equals(this.includeChildrenNS, getProductRatePlanChargeResponse.includeChildrenNS) &&
Objects.equals(this.integrationIdNS, getProductRatePlanChargeResponse.integrationIdNS) &&
Objects.equals(this.integrationStatusNS, getProductRatePlanChargeResponse.integrationStatusNS) &&
Objects.equals(this.itemTypeNS, getProductRatePlanChargeResponse.itemTypeNS) &&
Objects.equals(this.locationNS, getProductRatePlanChargeResponse.locationNS) &&
Objects.equals(this.recognizedRevAccountNS, getProductRatePlanChargeResponse.recognizedRevAccountNS) &&
Objects.equals(this.revRecEndNS, getProductRatePlanChargeResponse.revRecEndNS) &&
Objects.equals(this.revRecStartNS, getProductRatePlanChargeResponse.revRecStartNS) &&
Objects.equals(this.revRecTemplateTypeNS, getProductRatePlanChargeResponse.revRecTemplateTypeNS) &&
Objects.equals(this.subsidiaryNS, getProductRatePlanChargeResponse.subsidiaryNS) &&
Objects.equals(this.syncDateNS, getProductRatePlanChargeResponse.syncDateNS)&&
Objects.equals(this.additionalProperties, getProductRatePlanChargeResponse.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(id, productRatePlanId, accountingCode, applyDiscountTo, billCycleDay, billCycleType, billingPeriod, billingPeriodAlignment, billingTiming, chargeFunction, chargeModel, chargeModelConfiguration, chargeType, commitmentType, createdById, createdDate, defaultQuantity, deferredRevenueAccount, deliverySchedule, description, discountLevel, drawdownRate, drawdownUom, endDateCondition, excludeItemBillingFromRevenueAccounting, excludeItemBookingFromRevenueAccounting, includedUnits, isPrepaid, isRollover, isStackedDiscount, isUnbilled, revenueRecognitionTiming, revenueAmortizationMethod, legacyRevenueReporting, listPriceBase, minQuantity, maxQuantity, name, numberOfPeriod, overageCalculationOption, overageUnusedUnitsCreditOption, prepaidOperationType, prepaidQuantity, prepaidTotalQuantity, prepaidUom, prepayPeriods, priceChangeOption, priceIncreasePercentage, priceIncreaseOption, ratingGroup, recognizedRevenueAccount, revRecCode, revRecTriggerCondition, revenueRecognitionRuleName, rolloverApply, rolloverPeriods, smoothingModel, specificBillingPeriod, specificListPriceBase, taxCode, taxMode, taxable, triggerEvent, UOM, upToPeriods, upToPeriodsType, updatedById, updatedDate, usageRecordRatingOption, useDiscountSpecificAccountingCode, useTenantDefaultForPriceChange, validityPeriodType, weeklyBillCycleDay, ratingGroupsOperatorType, classNS, deferredRevAccountNS, departmentNS, includeChildrenNS, integrationIdNS, integrationStatusNS, itemTypeNS, locationNS, recognizedRevAccountNS, revRecEndNS, revRecStartNS, revRecTemplateTypeNS, subsidiaryNS, syncDateNS, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GetProductRatePlanChargeResponse {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" productRatePlanId: ").append(toIndentedString(productRatePlanId)).append("\n");
sb.append(" accountingCode: ").append(toIndentedString(accountingCode)).append("\n");
sb.append(" applyDiscountTo: ").append(toIndentedString(applyDiscountTo)).append("\n");
sb.append(" billCycleDay: ").append(toIndentedString(billCycleDay)).append("\n");
sb.append(" billCycleType: ").append(toIndentedString(billCycleType)).append("\n");
sb.append(" billingPeriod: ").append(toIndentedString(billingPeriod)).append("\n");
sb.append(" billingPeriodAlignment: ").append(toIndentedString(billingPeriodAlignment)).append("\n");
sb.append(" billingTiming: ").append(toIndentedString(billingTiming)).append("\n");
sb.append(" chargeFunction: ").append(toIndentedString(chargeFunction)).append("\n");
sb.append(" chargeModel: ").append(toIndentedString(chargeModel)).append("\n");
sb.append(" chargeModelConfiguration: ").append(toIndentedString(chargeModelConfiguration)).append("\n");
sb.append(" chargeType: ").append(toIndentedString(chargeType)).append("\n");
sb.append(" commitmentType: ").append(toIndentedString(commitmentType)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" defaultQuantity: ").append(toIndentedString(defaultQuantity)).append("\n");
sb.append(" deferredRevenueAccount: ").append(toIndentedString(deferredRevenueAccount)).append("\n");
sb.append(" deliverySchedule: ").append(toIndentedString(deliverySchedule)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" discountLevel: ").append(toIndentedString(discountLevel)).append("\n");
sb.append(" drawdownRate: ").append(toIndentedString(drawdownRate)).append("\n");
sb.append(" drawdownUom: ").append(toIndentedString(drawdownUom)).append("\n");
sb.append(" endDateCondition: ").append(toIndentedString(endDateCondition)).append("\n");
sb.append(" excludeItemBillingFromRevenueAccounting: ").append(toIndentedString(excludeItemBillingFromRevenueAccounting)).append("\n");
sb.append(" excludeItemBookingFromRevenueAccounting: ").append(toIndentedString(excludeItemBookingFromRevenueAccounting)).append("\n");
sb.append(" includedUnits: ").append(toIndentedString(includedUnits)).append("\n");
sb.append(" isPrepaid: ").append(toIndentedString(isPrepaid)).append("\n");
sb.append(" isRollover: ").append(toIndentedString(isRollover)).append("\n");
sb.append(" isStackedDiscount: ").append(toIndentedString(isStackedDiscount)).append("\n");
sb.append(" isUnbilled: ").append(toIndentedString(isUnbilled)).append("\n");
sb.append(" revenueRecognitionTiming: ").append(toIndentedString(revenueRecognitionTiming)).append("\n");
sb.append(" revenueAmortizationMethod: ").append(toIndentedString(revenueAmortizationMethod)).append("\n");
sb.append(" legacyRevenueReporting: ").append(toIndentedString(legacyRevenueReporting)).append("\n");
sb.append(" listPriceBase: ").append(toIndentedString(listPriceBase)).append("\n");
sb.append(" minQuantity: ").append(toIndentedString(minQuantity)).append("\n");
sb.append(" maxQuantity: ").append(toIndentedString(maxQuantity)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" numberOfPeriod: ").append(toIndentedString(numberOfPeriod)).append("\n");
sb.append(" overageCalculationOption: ").append(toIndentedString(overageCalculationOption)).append("\n");
sb.append(" overageUnusedUnitsCreditOption: ").append(toIndentedString(overageUnusedUnitsCreditOption)).append("\n");
sb.append(" prepaidOperationType: ").append(toIndentedString(prepaidOperationType)).append("\n");
sb.append(" prepaidQuantity: ").append(toIndentedString(prepaidQuantity)).append("\n");
sb.append(" prepaidTotalQuantity: ").append(toIndentedString(prepaidTotalQuantity)).append("\n");
sb.append(" prepaidUom: ").append(toIndentedString(prepaidUom)).append("\n");
sb.append(" prepayPeriods: ").append(toIndentedString(prepayPeriods)).append("\n");
sb.append(" priceChangeOption: ").append(toIndentedString(priceChangeOption)).append("\n");
sb.append(" priceIncreasePercentage: ").append(toIndentedString(priceIncreasePercentage)).append("\n");
sb.append(" priceIncreaseOption: ").append(toIndentedString(priceIncreaseOption)).append("\n");
sb.append(" ratingGroup: ").append(toIndentedString(ratingGroup)).append("\n");
sb.append(" recognizedRevenueAccount: ").append(toIndentedString(recognizedRevenueAccount)).append("\n");
sb.append(" revRecCode: ").append(toIndentedString(revRecCode)).append("\n");
sb.append(" revRecTriggerCondition: ").append(toIndentedString(revRecTriggerCondition)).append("\n");
sb.append(" revenueRecognitionRuleName: ").append(toIndentedString(revenueRecognitionRuleName)).append("\n");
sb.append(" rolloverApply: ").append(toIndentedString(rolloverApply)).append("\n");
sb.append(" rolloverPeriods: ").append(toIndentedString(rolloverPeriods)).append("\n");
sb.append(" smoothingModel: ").append(toIndentedString(smoothingModel)).append("\n");
sb.append(" specificBillingPeriod: ").append(toIndentedString(specificBillingPeriod)).append("\n");
sb.append(" specificListPriceBase: ").append(toIndentedString(specificListPriceBase)).append("\n");
sb.append(" taxCode: ").append(toIndentedString(taxCode)).append("\n");
sb.append(" taxMode: ").append(toIndentedString(taxMode)).append("\n");
sb.append(" taxable: ").append(toIndentedString(taxable)).append("\n");
sb.append(" triggerEvent: ").append(toIndentedString(triggerEvent)).append("\n");
sb.append(" UOM: ").append(toIndentedString(UOM)).append("\n");
sb.append(" upToPeriods: ").append(toIndentedString(upToPeriods)).append("\n");
sb.append(" upToPeriodsType: ").append(toIndentedString(upToPeriodsType)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" usageRecordRatingOption: ").append(toIndentedString(usageRecordRatingOption)).append("\n");
sb.append(" useDiscountSpecificAccountingCode: ").append(toIndentedString(useDiscountSpecificAccountingCode)).append("\n");
sb.append(" useTenantDefaultForPriceChange: ").append(toIndentedString(useTenantDefaultForPriceChange)).append("\n");
sb.append(" validityPeriodType: ").append(toIndentedString(validityPeriodType)).append("\n");
sb.append(" weeklyBillCycleDay: ").append(toIndentedString(weeklyBillCycleDay)).append("\n");
sb.append(" ratingGroupsOperatorType: ").append(toIndentedString(ratingGroupsOperatorType)).append("\n");
sb.append(" classNS: ").append(toIndentedString(classNS)).append("\n");
sb.append(" deferredRevAccountNS: ").append(toIndentedString(deferredRevAccountNS)).append("\n");
sb.append(" departmentNS: ").append(toIndentedString(departmentNS)).append("\n");
sb.append(" includeChildrenNS: ").append(toIndentedString(includeChildrenNS)).append("\n");
sb.append(" integrationIdNS: ").append(toIndentedString(integrationIdNS)).append("\n");
sb.append(" integrationStatusNS: ").append(toIndentedString(integrationStatusNS)).append("\n");
sb.append(" itemTypeNS: ").append(toIndentedString(itemTypeNS)).append("\n");
sb.append(" locationNS: ").append(toIndentedString(locationNS)).append("\n");
sb.append(" recognizedRevAccountNS: ").append(toIndentedString(recognizedRevAccountNS)).append("\n");
sb.append(" revRecEndNS: ").append(toIndentedString(revRecEndNS)).append("\n");
sb.append(" revRecStartNS: ").append(toIndentedString(revRecStartNS)).append("\n");
sb.append(" revRecTemplateTypeNS: ").append(toIndentedString(revRecTemplateTypeNS)).append("\n");
sb.append(" subsidiaryNS: ").append(toIndentedString(subsidiaryNS)).append("\n");
sb.append(" syncDateNS: ").append(toIndentedString(syncDateNS)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("Id");
openapiFields.add("ProductRatePlanId");
openapiFields.add("AccountingCode");
openapiFields.add("ApplyDiscountTo");
openapiFields.add("BillCycleDay");
openapiFields.add("BillCycleType");
openapiFields.add("BillingPeriod");
openapiFields.add("BillingPeriodAlignment");
openapiFields.add("BillingTiming");
openapiFields.add("ChargeFunction");
openapiFields.add("ChargeModel");
openapiFields.add("ChargeModelConfiguration");
openapiFields.add("ChargeType");
openapiFields.add("CommitmentType");
openapiFields.add("CreatedById");
openapiFields.add("CreatedDate");
openapiFields.add("DefaultQuantity");
openapiFields.add("DeferredRevenueAccount");
openapiFields.add("DeliverySchedule");
openapiFields.add("Description");
openapiFields.add("DiscountLevel");
openapiFields.add("DrawdownRate");
openapiFields.add("DrawdownUom");
openapiFields.add("EndDateCondition");
openapiFields.add("ExcludeItemBillingFromRevenueAccounting");
openapiFields.add("ExcludeItemBookingFromRevenueAccounting");
openapiFields.add("IncludedUnits");
openapiFields.add("IsPrepaid");
openapiFields.add("IsRollover");
openapiFields.add("IsStackedDiscount");
openapiFields.add("IsUnbilled");
openapiFields.add("RevenueRecognitionTiming");
openapiFields.add("RevenueAmortizationMethod");
openapiFields.add("LegacyRevenueReporting");
openapiFields.add("ListPriceBase");
openapiFields.add("MinQuantity");
openapiFields.add("MaxQuantity");
openapiFields.add("Name");
openapiFields.add("NumberOfPeriod");
openapiFields.add("OverageCalculationOption");
openapiFields.add("OverageUnusedUnitsCreditOption");
openapiFields.add("PrepaidOperationType");
openapiFields.add("prepaidQuantity");
openapiFields.add("prepaidTotalQuantity");
openapiFields.add("prepaidUom");
openapiFields.add("prepayPeriods");
openapiFields.add("PriceChangeOption");
openapiFields.add("PriceIncreasePercentage");
openapiFields.add("PriceIncreaseOption");
openapiFields.add("RatingGroup");
openapiFields.add("RecognizedRevenueAccount");
openapiFields.add("RevRecCode");
openapiFields.add("RevRecTriggerCondition");
openapiFields.add("RevenueRecognitionRuleName");
openapiFields.add("RolloverApply");
openapiFields.add("RolloverPeriods");
openapiFields.add("SmoothingModel");
openapiFields.add("SpecificBillingPeriod");
openapiFields.add("SpecificListPriceBase");
openapiFields.add("TaxCode");
openapiFields.add("TaxMode");
openapiFields.add("Taxable");
openapiFields.add("TriggerEvent");
openapiFields.add("UOM");
openapiFields.add("UpToPeriods");
openapiFields.add("UpToPeriodsType");
openapiFields.add("UpdatedById");
openapiFields.add("UpdatedDate");
openapiFields.add("UsageRecordRatingOption");
openapiFields.add("UseDiscountSpecificAccountingCode");
openapiFields.add("UseTenantDefaultForPriceChange");
openapiFields.add("ValidityPeriodType");
openapiFields.add("WeeklyBillCycleDay");
openapiFields.add("RatingGroupsOperatorType");
openapiFields.add("Class__NS");
openapiFields.add("DeferredRevAccount__NS");
openapiFields.add("Department__NS");
openapiFields.add("IncludeChildren__NS");
openapiFields.add("IntegrationId__NS");
openapiFields.add("IntegrationStatus__NS");
openapiFields.add("ItemType__NS");
openapiFields.add("Location__NS");
openapiFields.add("RecognizedRevAccount__NS");
openapiFields.add("RevRecEnd__NS");
openapiFields.add("RevRecStart__NS");
openapiFields.add("RevRecTemplateType__NS");
openapiFields.add("Subsidiary__NS");
openapiFields.add("SyncDate__NS");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to GetProductRatePlanChargeResponse
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!GetProductRatePlanChargeResponse.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in GetProductRatePlanChargeResponse is not found in the empty JSON string", GetProductRatePlanChargeResponse.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("Id") != null && !jsonObj.get("Id").isJsonNull()) && !jsonObj.get("Id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Id").toString()));
}
if ((jsonObj.get("ProductRatePlanId") != null && !jsonObj.get("ProductRatePlanId").isJsonNull()) && !jsonObj.get("ProductRatePlanId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ProductRatePlanId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ProductRatePlanId").toString()));
}
if ((jsonObj.get("AccountingCode") != null && !jsonObj.get("AccountingCode").isJsonNull()) && !jsonObj.get("AccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `AccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("AccountingCode").toString()));
}
// validate the optional field `ApplyDiscountTo`
if (jsonObj.get("ApplyDiscountTo") != null && !jsonObj.get("ApplyDiscountTo").isJsonNull()) {
ApplyDiscountTo.validateJsonElement(jsonObj.get("ApplyDiscountTo"));
}
// validate the optional field `BillCycleType`
if (jsonObj.get("BillCycleType") != null && !jsonObj.get("BillCycleType").isJsonNull()) {
BillCycleType.validateJsonElement(jsonObj.get("BillCycleType"));
}
// validate the optional field `BillingPeriod`
if (jsonObj.get("BillingPeriod") != null && !jsonObj.get("BillingPeriod").isJsonNull()) {
BillingPeriodProductRatePlanChargeRest.validateJsonElement(jsonObj.get("BillingPeriod"));
}
// validate the optional field `BillingPeriodAlignment`
if (jsonObj.get("BillingPeriodAlignment") != null && !jsonObj.get("BillingPeriodAlignment").isJsonNull()) {
BillingPeriodAlignmentProductRatePlanChargeRest.validateJsonElement(jsonObj.get("BillingPeriodAlignment"));
}
// validate the optional field `BillingTiming`
if (jsonObj.get("BillingTiming") != null && !jsonObj.get("BillingTiming").isJsonNull()) {
BillingTimingProductRatePlanChargeRest.validateJsonElement(jsonObj.get("BillingTiming"));
}
// validate the optional field `ChargeFunction`
if (jsonObj.get("ChargeFunction") != null && !jsonObj.get("ChargeFunction").isJsonNull()) {
ChargeFunction.validateJsonElement(jsonObj.get("ChargeFunction"));
}
// validate the optional field `ChargeModel`
if (jsonObj.get("ChargeModel") != null && !jsonObj.get("ChargeModel").isJsonNull()) {
ChargeModelProductRatePlanChargeRest.validateJsonElement(jsonObj.get("ChargeModel"));
}
// validate the optional field `ChargeModelConfiguration`
if (jsonObj.get("ChargeModelConfiguration") != null && !jsonObj.get("ChargeModelConfiguration").isJsonNull()) {
ChargeModelConfiguration.validateJsonElement(jsonObj.get("ChargeModelConfiguration"));
}
// validate the optional field `ChargeType`
if (jsonObj.get("ChargeType") != null && !jsonObj.get("ChargeType").isJsonNull()) {
ChargeType.validateJsonElement(jsonObj.get("ChargeType"));
}
// validate the optional field `CommitmentType`
if (jsonObj.get("CommitmentType") != null && !jsonObj.get("CommitmentType").isJsonNull()) {
CommitmentType.validateJsonElement(jsonObj.get("CommitmentType"));
}
if ((jsonObj.get("CreatedById") != null && !jsonObj.get("CreatedById").isJsonNull()) && !jsonObj.get("CreatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `CreatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("CreatedById").toString()));
}
if ((jsonObj.get("DeferredRevenueAccount") != null && !jsonObj.get("DeferredRevenueAccount").isJsonNull()) && !jsonObj.get("DeferredRevenueAccount").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `DeferredRevenueAccount` to be a primitive type in the JSON string but got `%s`", jsonObj.get("DeferredRevenueAccount").toString()));
}
// validate the optional field `DeliverySchedule`
if (jsonObj.get("DeliverySchedule") != null && !jsonObj.get("DeliverySchedule").isJsonNull()) {
DeliveryScheduleProductRatePlanCharge.validateJsonElement(jsonObj.get("DeliverySchedule"));
}
if ((jsonObj.get("Description") != null && !jsonObj.get("Description").isJsonNull()) && !jsonObj.get("Description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Description").toString()));
}
// validate the optional field `DiscountLevel`
if (jsonObj.get("DiscountLevel") != null && !jsonObj.get("DiscountLevel").isJsonNull()) {
DiscountLevel.validateJsonElement(jsonObj.get("DiscountLevel"));
}
if ((jsonObj.get("DrawdownUom") != null && !jsonObj.get("DrawdownUom").isJsonNull()) && !jsonObj.get("DrawdownUom").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `DrawdownUom` to be a primitive type in the JSON string but got `%s`", jsonObj.get("DrawdownUom").toString()));
}
// validate the optional field `EndDateCondition`
if (jsonObj.get("EndDateCondition") != null && !jsonObj.get("EndDateCondition").isJsonNull()) {
EndDateConditionProductRatePlanChargeRest.validateJsonElement(jsonObj.get("EndDateCondition"));
}
if ((jsonObj.get("RevenueRecognitionTiming") != null && !jsonObj.get("RevenueRecognitionTiming").isJsonNull()) && !jsonObj.get("RevenueRecognitionTiming").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `RevenueRecognitionTiming` to be a primitive type in the JSON string but got `%s`", jsonObj.get("RevenueRecognitionTiming").toString()));
}
if ((jsonObj.get("RevenueAmortizationMethod") != null && !jsonObj.get("RevenueAmortizationMethod").isJsonNull()) && !jsonObj.get("RevenueAmortizationMethod").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `RevenueAmortizationMethod` to be a primitive type in the JSON string but got `%s`", jsonObj.get("RevenueAmortizationMethod").toString()));
}
// validate the optional field `ListPriceBase`
if (jsonObj.get("ListPriceBase") != null && !jsonObj.get("ListPriceBase").isJsonNull()) {
ListPriceBase.validateJsonElement(jsonObj.get("ListPriceBase"));
}
if ((jsonObj.get("Name") != null && !jsonObj.get("Name").isJsonNull()) && !jsonObj.get("Name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Name").toString()));
}
// validate the optional field `OverageCalculationOption`
if (jsonObj.get("OverageCalculationOption") != null && !jsonObj.get("OverageCalculationOption").isJsonNull()) {
OverageCalculationOption.validateJsonElement(jsonObj.get("OverageCalculationOption"));
}
// validate the optional field `OverageUnusedUnitsCreditOption`
if (jsonObj.get("OverageUnusedUnitsCreditOption") != null && !jsonObj.get("OverageUnusedUnitsCreditOption").isJsonNull()) {
OverageUnusedUnitsCreditOption.validateJsonElement(jsonObj.get("OverageUnusedUnitsCreditOption"));
}
// validate the optional field `PrepaidOperationType`
if (jsonObj.get("PrepaidOperationType") != null && !jsonObj.get("PrepaidOperationType").isJsonNull()) {
PrepaidOperationType.validateJsonElement(jsonObj.get("PrepaidOperationType"));
}
if ((jsonObj.get("prepaidUom") != null && !jsonObj.get("prepaidUom").isJsonNull()) && !jsonObj.get("prepaidUom").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `prepaidUom` to be a primitive type in the JSON string but got `%s`", jsonObj.get("prepaidUom").toString()));
}
// validate the optional field `PriceChangeOption`
if (jsonObj.get("PriceChangeOption") != null && !jsonObj.get("PriceChangeOption").isJsonNull()) {
PriceChangeOption.validateJsonElement(jsonObj.get("PriceChangeOption"));
}
// validate the optional field `PriceIncreaseOption`
if (jsonObj.get("PriceIncreaseOption") != null && !jsonObj.get("PriceIncreaseOption").isJsonNull()) {
PriceIncreaseOption.validateJsonElement(jsonObj.get("PriceIncreaseOption"));
}
// validate the optional field `RatingGroup`
if (jsonObj.get("RatingGroup") != null && !jsonObj.get("RatingGroup").isJsonNull()) {
RatingGroup.validateJsonElement(jsonObj.get("RatingGroup"));
}
if ((jsonObj.get("RecognizedRevenueAccount") != null && !jsonObj.get("RecognizedRevenueAccount").isJsonNull()) && !jsonObj.get("RecognizedRevenueAccount").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `RecognizedRevenueAccount` to be a primitive type in the JSON string but got `%s`", jsonObj.get("RecognizedRevenueAccount").toString()));
}
if ((jsonObj.get("RevRecCode") != null && !jsonObj.get("RevRecCode").isJsonNull()) && !jsonObj.get("RevRecCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `RevRecCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("RevRecCode").toString()));
}
// validate the optional field `RevRecTriggerCondition`
if (jsonObj.get("RevRecTriggerCondition") != null && !jsonObj.get("RevRecTriggerCondition").isJsonNull()) {
RevRecTriggerConditionProductRatePlanChargeRest.validateJsonElement(jsonObj.get("RevRecTriggerCondition"));
}
// validate the optional field `RevenueRecognitionRuleName`
if (jsonObj.get("RevenueRecognitionRuleName") != null && !jsonObj.get("RevenueRecognitionRuleName").isJsonNull()) {
RevenueRecognitionRuleName.validateJsonElement(jsonObj.get("RevenueRecognitionRuleName"));
}
// validate the optional field `RolloverApply`
if (jsonObj.get("RolloverApply") != null && !jsonObj.get("RolloverApply").isJsonNull()) {
RolloverApply.validateJsonElement(jsonObj.get("RolloverApply"));
}
// validate the optional field `SmoothingModel`
if (jsonObj.get("SmoothingModel") != null && !jsonObj.get("SmoothingModel").isJsonNull()) {
SmoothingModel.validateJsonElement(jsonObj.get("SmoothingModel"));
}
if ((jsonObj.get("TaxCode") != null && !jsonObj.get("TaxCode").isJsonNull()) && !jsonObj.get("TaxCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `TaxCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("TaxCode").toString()));
}
// validate the optional field `TaxMode`
if (jsonObj.get("TaxMode") != null && !jsonObj.get("TaxMode").isJsonNull()) {
TaxMode.validateJsonElement(jsonObj.get("TaxMode"));
}
// validate the optional field `TriggerEvent`
if (jsonObj.get("TriggerEvent") != null && !jsonObj.get("TriggerEvent").isJsonNull()) {
TriggerEventProductRatePlanChargeRest.validateJsonElement(jsonObj.get("TriggerEvent"));
}
if ((jsonObj.get("UOM") != null && !jsonObj.get("UOM").isJsonNull()) && !jsonObj.get("UOM").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `UOM` to be a primitive type in the JSON string but got `%s`", jsonObj.get("UOM").toString()));
}
// validate the optional field `UpToPeriodsType`
if (jsonObj.get("UpToPeriodsType") != null && !jsonObj.get("UpToPeriodsType").isJsonNull()) {
UpToPeriodsTypeProductRatePlanChargeRest.validateJsonElement(jsonObj.get("UpToPeriodsType"));
}
if ((jsonObj.get("UpdatedById") != null && !jsonObj.get("UpdatedById").isJsonNull()) && !jsonObj.get("UpdatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `UpdatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("UpdatedById").toString()));
}
// validate the optional field `UsageRecordRatingOption`
if (jsonObj.get("UsageRecordRatingOption") != null && !jsonObj.get("UsageRecordRatingOption").isJsonNull()) {
UsageRecordRatingOption.validateJsonElement(jsonObj.get("UsageRecordRatingOption"));
}
// validate the optional field `ValidityPeriodType`
if (jsonObj.get("ValidityPeriodType") != null && !jsonObj.get("ValidityPeriodType").isJsonNull()) {
ValidityPeriodType.validateJsonElement(jsonObj.get("ValidityPeriodType"));
}
// validate the optional field `WeeklyBillCycleDay`
if (jsonObj.get("WeeklyBillCycleDay") != null && !jsonObj.get("WeeklyBillCycleDay").isJsonNull()) {
WeeklyBillCycleDay.validateJsonElement(jsonObj.get("WeeklyBillCycleDay"));
}
// validate the optional field `RatingGroupsOperatorType`
if (jsonObj.get("RatingGroupsOperatorType") != null && !jsonObj.get("RatingGroupsOperatorType").isJsonNull()) {
RatingGroupsOperatorType.validateJsonElement(jsonObj.get("RatingGroupsOperatorType"));
}
if ((jsonObj.get("Class__NS") != null && !jsonObj.get("Class__NS").isJsonNull()) && !jsonObj.get("Class__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Class__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Class__NS").toString()));
}
if ((jsonObj.get("DeferredRevAccount__NS") != null && !jsonObj.get("DeferredRevAccount__NS").isJsonNull()) && !jsonObj.get("DeferredRevAccount__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `DeferredRevAccount__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("DeferredRevAccount__NS").toString()));
}
if ((jsonObj.get("Department__NS") != null && !jsonObj.get("Department__NS").isJsonNull()) && !jsonObj.get("Department__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Department__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Department__NS").toString()));
}
if ((jsonObj.get("IncludeChildren__NS") != null && !jsonObj.get("IncludeChildren__NS").isJsonNull()) && !jsonObj.get("IncludeChildren__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IncludeChildren__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IncludeChildren__NS").toString()));
}
// validate the optional field `IncludeChildren__NS`
if (jsonObj.get("IncludeChildren__NS") != null && !jsonObj.get("IncludeChildren__NS").isJsonNull()) {
ProductRatePlanChargeObjectNSFieldsIncludeChildrenNS.validateJsonElement(jsonObj.get("IncludeChildren__NS"));
}
if ((jsonObj.get("IntegrationId__NS") != null && !jsonObj.get("IntegrationId__NS").isJsonNull()) && !jsonObj.get("IntegrationId__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationId__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationId__NS").toString()));
}
if ((jsonObj.get("IntegrationStatus__NS") != null && !jsonObj.get("IntegrationStatus__NS").isJsonNull()) && !jsonObj.get("IntegrationStatus__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationStatus__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationStatus__NS").toString()));
}
if ((jsonObj.get("ItemType__NS") != null && !jsonObj.get("ItemType__NS").isJsonNull()) && !jsonObj.get("ItemType__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ItemType__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ItemType__NS").toString()));
}
// validate the optional field `ItemType__NS`
if (jsonObj.get("ItemType__NS") != null && !jsonObj.get("ItemType__NS").isJsonNull()) {
ProductRatePlanChargeObjectNSFieldsItemTypeNS.validateJsonElement(jsonObj.get("ItemType__NS"));
}
if ((jsonObj.get("Location__NS") != null && !jsonObj.get("Location__NS").isJsonNull()) && !jsonObj.get("Location__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Location__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Location__NS").toString()));
}
if ((jsonObj.get("RecognizedRevAccount__NS") != null && !jsonObj.get("RecognizedRevAccount__NS").isJsonNull()) && !jsonObj.get("RecognizedRevAccount__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `RecognizedRevAccount__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("RecognizedRevAccount__NS").toString()));
}
if ((jsonObj.get("RevRecEnd__NS") != null && !jsonObj.get("RevRecEnd__NS").isJsonNull()) && !jsonObj.get("RevRecEnd__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `RevRecEnd__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("RevRecEnd__NS").toString()));
}
// validate the optional field `RevRecEnd__NS`
if (jsonObj.get("RevRecEnd__NS") != null && !jsonObj.get("RevRecEnd__NS").isJsonNull()) {
ProductRatePlanChargeObjectNSFieldsRevRecEndNS.validateJsonElement(jsonObj.get("RevRecEnd__NS"));
}
if ((jsonObj.get("RevRecStart__NS") != null && !jsonObj.get("RevRecStart__NS").isJsonNull()) && !jsonObj.get("RevRecStart__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `RevRecStart__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("RevRecStart__NS").toString()));
}
// validate the optional field `RevRecStart__NS`
if (jsonObj.get("RevRecStart__NS") != null && !jsonObj.get("RevRecStart__NS").isJsonNull()) {
ProductRatePlanChargeObjectNSFieldsRevRecStartNS.validateJsonElement(jsonObj.get("RevRecStart__NS"));
}
if ((jsonObj.get("RevRecTemplateType__NS") != null && !jsonObj.get("RevRecTemplateType__NS").isJsonNull()) && !jsonObj.get("RevRecTemplateType__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `RevRecTemplateType__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("RevRecTemplateType__NS").toString()));
}
if ((jsonObj.get("Subsidiary__NS") != null && !jsonObj.get("Subsidiary__NS").isJsonNull()) && !jsonObj.get("Subsidiary__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Subsidiary__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Subsidiary__NS").toString()));
}
if ((jsonObj.get("SyncDate__NS") != null && !jsonObj.get("SyncDate__NS").isJsonNull()) && !jsonObj.get("SyncDate__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SyncDate__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SyncDate__NS").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!GetProductRatePlanChargeResponse.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'GetProductRatePlanChargeResponse' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(GetProductRatePlanChargeResponse.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, GetProductRatePlanChargeResponse value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public GetProductRatePlanChargeResponse read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
GetProductRatePlanChargeResponse instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of GetProductRatePlanChargeResponse given an JSON string
*
* @param jsonString JSON string
* @return An instance of GetProductRatePlanChargeResponse
* @throws IOException if the JSON string is invalid with respect to GetProductRatePlanChargeResponse
*/
public static GetProductRatePlanChargeResponse fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, GetProductRatePlanChargeResponse.class);
}
/**
* Convert an instance of GetProductRatePlanChargeResponse to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy