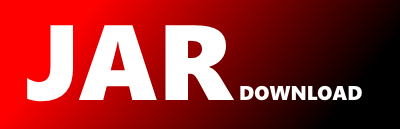
com.zuora.model.GetScheduledEventResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.GetScheduledEventResponseParametersValue;
import java.io.IOException;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* GetScheduledEventResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class GetScheduledEventResponse {
public static final String SERIALIZED_NAME_ACTIVE = "active";
@SerializedName(SERIALIZED_NAME_ACTIVE)
private Boolean active;
public static final String SERIALIZED_NAME_API_FIELD = "apiField";
@SerializedName(SERIALIZED_NAME_API_FIELD)
private String apiField;
public static final String SERIALIZED_NAME_API_OBJECT = "apiObject";
@SerializedName(SERIALIZED_NAME_API_OBJECT)
private String apiObject;
public static final String SERIALIZED_NAME_CONDITION = "condition";
@SerializedName(SERIALIZED_NAME_CONDITION)
private String condition;
public static final String SERIALIZED_NAME_CRON_EXPRESSION = "cronExpression";
@SerializedName(SERIALIZED_NAME_CRON_EXPRESSION)
private String cronExpression;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_DISPLAY_NAME = "displayName";
@SerializedName(SERIALIZED_NAME_DISPLAY_NAME)
private String displayName;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_NAMESPACE = "namespace";
@SerializedName(SERIALIZED_NAME_NAMESPACE)
private String namespace;
public static final String SERIALIZED_NAME_PARAMETERS = "parameters";
@SerializedName(SERIALIZED_NAME_PARAMETERS)
private Map parameters;
public GetScheduledEventResponse() {
}
public GetScheduledEventResponse active(Boolean active) {
this.active = active;
return this;
}
/**
* Indicate whether the scheduled event is active or inactive
* @return active
*/
@javax.annotation.Nullable
public Boolean getActive() {
return active;
}
public void setActive(Boolean active) {
this.active = active;
}
public GetScheduledEventResponse apiField(String apiField) {
this.apiField = apiField;
return this;
}
/**
* The base field of the base object in the `apiObject` field, should be in date or timestamp format. The scheduled event notifications are triggered based on this date and the event parameters (before or after a specified number of days) from notification definitions. Should be specified in the pattern: ^[A-Z][\\\\w\\\\-]*$
* @return apiField
*/
@javax.annotation.Nullable
public String getApiField() {
return apiField;
}
public void setApiField(String apiField) {
this.apiField = apiField;
}
public GetScheduledEventResponse apiObject(String apiObject) {
this.apiObject = apiObject;
return this;
}
/**
* The base object that the scheduled event is defined upon. The base object should contain a date or timestamp format field. Should be specified in the pattern: ^[A-Z][\\\\w\\\\-]*$
* @return apiObject
*/
@javax.annotation.Nullable
public String getApiObject() {
return apiObject;
}
public void setApiObject(String apiObject) {
this.apiObject = apiObject;
}
public GetScheduledEventResponse condition(String condition) {
this.condition = condition;
return this;
}
/**
* The filter rule conditions, written in [JEXL](http://commons.apache.org/proper/commons-jexl/). The scheduled event is triggered only if the condition is evaluated as true. The rule might contain event context merge fields and data source merge fields. Data source merge fields must be from [the base object of the event or from the joined objects of the base object](https://knowledgecenter.zuora.com/DC_Developers/M_Export_ZOQL#Data_Sources_and_Objects). Scheduled events with invalid merge fields will fail to evaluate, thus will not be triggered. For example, to trigger an invoice due date scheduled event to only invoices with an amount over 1000, you would define the following condition: ```Invoice.Amount > 1000``` `Invoice.Amount` refers to the `Amount` field of the Zuora object `Invoice`.
* @return condition
*/
@javax.annotation.Nullable
public String getCondition() {
return condition;
}
public void setCondition(String condition) {
this.condition = condition;
}
public GetScheduledEventResponse cronExpression(String cronExpression) {
this.cronExpression = cronExpression;
return this;
}
/**
* The cron expression defines the time when scheduled event notifications will be sent.
* @return cronExpression
*/
@javax.annotation.Nullable
public String getCronExpression() {
return cronExpression;
}
public void setCronExpression(String cronExpression) {
this.cronExpression = cronExpression;
}
public GetScheduledEventResponse description(String description) {
this.description = description;
return this;
}
/**
* The description of the scheduled event.
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public GetScheduledEventResponse displayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* The display name of the scheduled event.
* @return displayName
*/
@javax.annotation.Nullable
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public GetScheduledEventResponse id(String id) {
this.id = id;
return this;
}
/**
* Scheduled event ID.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public GetScheduledEventResponse name(String name) {
this.name = name;
return this;
}
/**
* The name of the scheduled event.
* @return name
*/
@javax.annotation.Nullable
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public GetScheduledEventResponse namespace(String namespace) {
this.namespace = namespace;
return this;
}
/**
* The namespace of the scheduled event name in the `name` field.
* @return namespace
*/
@javax.annotation.Nullable
public String getNamespace() {
return namespace;
}
public void setNamespace(String namespace) {
this.namespace = namespace;
}
public GetScheduledEventResponse parameters(Map parameters) {
this.parameters = parameters;
return this;
}
public GetScheduledEventResponse putParametersItem(String key, GetScheduledEventResponseParametersValue parametersItem) {
if (this.parameters == null) {
this.parameters = new HashMap<>();
}
this.parameters.put(key, parametersItem);
return this;
}
/**
* The parameter definitions of the filter rule. The names of the parameters must match with the filter rule and can't be duplicated. You should specify all the parameters when creating scheduled event notifications.
* @return parameters
*/
@javax.annotation.Nullable
public Map getParameters() {
return parameters;
}
public void setParameters(Map parameters) {
this.parameters = parameters;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the GetScheduledEventResponse instance itself
*/
public GetScheduledEventResponse putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GetScheduledEventResponse getScheduledEventResponse = (GetScheduledEventResponse) o;
return Objects.equals(this.active, getScheduledEventResponse.active) &&
Objects.equals(this.apiField, getScheduledEventResponse.apiField) &&
Objects.equals(this.apiObject, getScheduledEventResponse.apiObject) &&
Objects.equals(this.condition, getScheduledEventResponse.condition) &&
Objects.equals(this.cronExpression, getScheduledEventResponse.cronExpression) &&
Objects.equals(this.description, getScheduledEventResponse.description) &&
Objects.equals(this.displayName, getScheduledEventResponse.displayName) &&
Objects.equals(this.id, getScheduledEventResponse.id) &&
Objects.equals(this.name, getScheduledEventResponse.name) &&
Objects.equals(this.namespace, getScheduledEventResponse.namespace) &&
Objects.equals(this.parameters, getScheduledEventResponse.parameters)&&
Objects.equals(this.additionalProperties, getScheduledEventResponse.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(active, apiField, apiObject, condition, cronExpression, description, displayName, id, name, namespace, parameters, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GetScheduledEventResponse {\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append(" apiField: ").append(toIndentedString(apiField)).append("\n");
sb.append(" apiObject: ").append(toIndentedString(apiObject)).append("\n");
sb.append(" condition: ").append(toIndentedString(condition)).append("\n");
sb.append(" cronExpression: ").append(toIndentedString(cronExpression)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" displayName: ").append(toIndentedString(displayName)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" namespace: ").append(toIndentedString(namespace)).append("\n");
sb.append(" parameters: ").append(toIndentedString(parameters)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("active");
openapiFields.add("apiField");
openapiFields.add("apiObject");
openapiFields.add("condition");
openapiFields.add("cronExpression");
openapiFields.add("description");
openapiFields.add("displayName");
openapiFields.add("id");
openapiFields.add("name");
openapiFields.add("namespace");
openapiFields.add("parameters");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to GetScheduledEventResponse
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!GetScheduledEventResponse.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in GetScheduledEventResponse is not found in the empty JSON string", GetScheduledEventResponse.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("apiField") != null && !jsonObj.get("apiField").isJsonNull()) && !jsonObj.get("apiField").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `apiField` to be a primitive type in the JSON string but got `%s`", jsonObj.get("apiField").toString()));
}
if ((jsonObj.get("apiObject") != null && !jsonObj.get("apiObject").isJsonNull()) && !jsonObj.get("apiObject").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `apiObject` to be a primitive type in the JSON string but got `%s`", jsonObj.get("apiObject").toString()));
}
if ((jsonObj.get("condition") != null && !jsonObj.get("condition").isJsonNull()) && !jsonObj.get("condition").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `condition` to be a primitive type in the JSON string but got `%s`", jsonObj.get("condition").toString()));
}
if ((jsonObj.get("cronExpression") != null && !jsonObj.get("cronExpression").isJsonNull()) && !jsonObj.get("cronExpression").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cronExpression` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cronExpression").toString()));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if ((jsonObj.get("displayName") != null && !jsonObj.get("displayName").isJsonNull()) && !jsonObj.get("displayName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `displayName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("displayName").toString()));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("name") != null && !jsonObj.get("name").isJsonNull()) && !jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if ((jsonObj.get("namespace") != null && !jsonObj.get("namespace").isJsonNull()) && !jsonObj.get("namespace").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `namespace` to be a primitive type in the JSON string but got `%s`", jsonObj.get("namespace").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!GetScheduledEventResponse.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'GetScheduledEventResponse' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(GetScheduledEventResponse.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, GetScheduledEventResponse value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public GetScheduledEventResponse read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
GetScheduledEventResponse instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of GetScheduledEventResponse given an JSON string
*
* @param jsonString JSON string
* @return An instance of GetScheduledEventResponse
* @throws IOException if the JSON string is invalid with respect to GetScheduledEventResponse
*/
public static GetScheduledEventResponse fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, GetScheduledEventResponse.class);
}
/**
* Convert an instance of GetScheduledEventResponse to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy