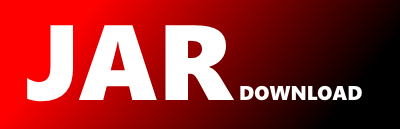
com.zuora.model.GetSubscriptionResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.AccountBasicInfo;
import com.zuora.model.Contact;
import com.zuora.model.ExternallyManagedBy;
import com.zuora.model.FailedReason;
import com.zuora.model.SubscriptionRatePlan;
import com.zuora.model.SubscriptionStatus;
import com.zuora.model.SubscriptionStatusHistory;
import com.zuora.model.TermPeriodType;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* GetSubscriptionResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class GetSubscriptionResponse {
public static final String SERIALIZED_NAME_PROCESS_ID = "processId";
@SerializedName(SERIALIZED_NAME_PROCESS_ID)
private String processId;
public static final String SERIALIZED_NAME_REQUEST_ID = "requestId";
@SerializedName(SERIALIZED_NAME_REQUEST_ID)
private String requestId;
public static final String SERIALIZED_NAME_REASONS = "reasons";
@SerializedName(SERIALIZED_NAME_REASONS)
private List reasons;
public static final String SERIALIZED_NAME_SUCCESS = "success";
@SerializedName(SERIALIZED_NAME_SUCCESS)
private Boolean success;
public static final String SERIALIZED_NAME_CPQ_BUNDLE_JSON_ID_Q_T = "CpqBundleJsonId__QT";
@SerializedName(SERIALIZED_NAME_CPQ_BUNDLE_JSON_ID_Q_T)
private String cpqBundleJsonIdQT;
public static final String SERIALIZED_NAME_OPPORTUNITY_CLOSE_DATE_Q_T = "OpportunityCloseDate__QT";
@SerializedName(SERIALIZED_NAME_OPPORTUNITY_CLOSE_DATE_Q_T)
private LocalDate opportunityCloseDateQT;
public static final String SERIALIZED_NAME_OPPORTUNITY_NAME_Q_T = "OpportunityName__QT";
@SerializedName(SERIALIZED_NAME_OPPORTUNITY_NAME_Q_T)
private String opportunityNameQT;
public static final String SERIALIZED_NAME_QUOTE_BUSINESS_TYPE_Q_T = "QuoteBusinessType__QT";
@SerializedName(SERIALIZED_NAME_QUOTE_BUSINESS_TYPE_Q_T)
private String quoteBusinessTypeQT;
public static final String SERIALIZED_NAME_QUOTE_NUMBER_Q_T = "QuoteNumber__QT";
@SerializedName(SERIALIZED_NAME_QUOTE_NUMBER_Q_T)
private String quoteNumberQT;
public static final String SERIALIZED_NAME_QUOTE_TYPE_Q_T = "QuoteType__QT";
@SerializedName(SERIALIZED_NAME_QUOTE_TYPE_Q_T)
private String quoteTypeQT;
public static final String SERIALIZED_NAME_INTEGRATION_ID_N_S = "IntegrationId__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_ID_N_S)
private String integrationIdNS;
public static final String SERIALIZED_NAME_INTEGRATION_STATUS_N_S = "IntegrationStatus__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_STATUS_N_S)
private String integrationStatusNS;
public static final String SERIALIZED_NAME_PROJECT_N_S = "Project__NS";
@SerializedName(SERIALIZED_NAME_PROJECT_N_S)
private String projectNS;
public static final String SERIALIZED_NAME_SALES_ORDER_N_S = "SalesOrder__NS";
@SerializedName(SERIALIZED_NAME_SALES_ORDER_N_S)
private String salesOrderNS;
public static final String SERIALIZED_NAME_SYNC_DATE_N_S = "SyncDate__NS";
@SerializedName(SERIALIZED_NAME_SYNC_DATE_N_S)
private String syncDateNS;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_SUBSCRIPTION_NUMBER = "subscriptionNumber";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_NUMBER)
private String subscriptionNumber;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "accountId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NAME = "accountName";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NAME)
private String accountName;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "accountNumber";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_AUTO_RENEW = "autoRenew";
@SerializedName(SERIALIZED_NAME_AUTO_RENEW)
private Boolean autoRenew;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT = "billToContact";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT)
private Contact billToContact;
public static final String SERIALIZED_NAME_CANCEL_REASON = "cancelReason";
@SerializedName(SERIALIZED_NAME_CANCEL_REASON)
private String cancelReason;
public static final String SERIALIZED_NAME_CONTRACT_EFFECTIVE_DATE = "contractEffectiveDate";
@SerializedName(SERIALIZED_NAME_CONTRACT_EFFECTIVE_DATE)
private LocalDate contractEffectiveDate;
public static final String SERIALIZED_NAME_CONTRACTED_MRR = "contractedMrr";
@SerializedName(SERIALIZED_NAME_CONTRACTED_MRR)
private BigDecimal contractedMrr;
public static final String SERIALIZED_NAME_CURRENT_TERM = "currentTerm";
@SerializedName(SERIALIZED_NAME_CURRENT_TERM)
private Long currentTerm;
public static final String SERIALIZED_NAME_CURRENT_TERM_PERIOD_TYPE = "currentTermPeriodType";
@SerializedName(SERIALIZED_NAME_CURRENT_TERM_PERIOD_TYPE)
private TermPeriodType currentTermPeriodType;
public static final String SERIALIZED_NAME_CUSTOMER_ACCEPTANCE_DATE = "customerAcceptanceDate";
@SerializedName(SERIALIZED_NAME_CUSTOMER_ACCEPTANCE_DATE)
private LocalDate customerAcceptanceDate;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_CREATE_TIME = "createTime";
@SerializedName(SERIALIZED_NAME_CREATE_TIME)
private String createTime;
public static final String SERIALIZED_NAME_UPDATE_TIME = "updateTime";
@SerializedName(SERIALIZED_NAME_UPDATE_TIME)
private String updateTime;
public static final String SERIALIZED_NAME_EXTERNALLY_MANAGED_BY = "externallyManagedBy";
@SerializedName(SERIALIZED_NAME_EXTERNALLY_MANAGED_BY)
private ExternallyManagedBy externallyManagedBy;
public static final String SERIALIZED_NAME_INITIAL_TERM = "initialTerm";
@SerializedName(SERIALIZED_NAME_INITIAL_TERM)
private Long initialTerm;
public static final String SERIALIZED_NAME_INITIAL_TERM_PERIOD_TYPE = "initialTermPeriodType";
@SerializedName(SERIALIZED_NAME_INITIAL_TERM_PERIOD_TYPE)
private TermPeriodType initialTermPeriodType;
public static final String SERIALIZED_NAME_INVOICE_GROUP_NUMBER = "invoiceGroupNumber";
@SerializedName(SERIALIZED_NAME_INVOICE_GROUP_NUMBER)
private String invoiceGroupNumber;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_ID = "invoiceOwnerAccountId";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_ID)
private String invoiceOwnerAccountId;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_NAME = "invoiceOwnerAccountName";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_NAME)
private String invoiceOwnerAccountName;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_NUMBER = "invoiceOwnerAccountNumber";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_NUMBER)
private String invoiceOwnerAccountNumber;
public static final String SERIALIZED_NAME_INVOICE_SCHEDULE_ID = "invoiceScheduleId";
@SerializedName(SERIALIZED_NAME_INVOICE_SCHEDULE_ID)
private String invoiceScheduleId;
public static final String SERIALIZED_NAME_INVOICE_SEPARATELY = "invoiceSeparately";
@SerializedName(SERIALIZED_NAME_INVOICE_SEPARATELY)
private String invoiceSeparately;
public static final String SERIALIZED_NAME_INVOICE_TEMPLATE_ID = "invoiceTemplateId";
@SerializedName(SERIALIZED_NAME_INVOICE_TEMPLATE_ID)
private String invoiceTemplateId;
public static final String SERIALIZED_NAME_INVOICE_TEMPLATE_NAME = "invoiceTemplateName";
@SerializedName(SERIALIZED_NAME_INVOICE_TEMPLATE_NAME)
private String invoiceTemplateName;
public static final String SERIALIZED_NAME_IS_LATEST_VERSION = "isLatestVersion";
@SerializedName(SERIALIZED_NAME_IS_LATEST_VERSION)
private Boolean isLatestVersion;
public static final String SERIALIZED_NAME_LAST_BOOKING_DATE = "lastBookingDate";
@SerializedName(SERIALIZED_NAME_LAST_BOOKING_DATE)
private LocalDate lastBookingDate;
public static final String SERIALIZED_NAME_NOTES = "notes";
@SerializedName(SERIALIZED_NAME_NOTES)
private String notes;
public static final String SERIALIZED_NAME_ORDER_NUMBER = "orderNumber";
@SerializedName(SERIALIZED_NAME_ORDER_NUMBER)
private String orderNumber;
public static final String SERIALIZED_NAME_PAYMENT_TERM = "paymentTerm";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERM)
private String paymentTerm;
public static final String SERIALIZED_NAME_RATE_PLANS = "ratePlans";
@SerializedName(SERIALIZED_NAME_RATE_PLANS)
private List ratePlans;
public static final String SERIALIZED_NAME_RENEWAL_SETTING = "renewalSetting";
@SerializedName(SERIALIZED_NAME_RENEWAL_SETTING)
private String renewalSetting;
public static final String SERIALIZED_NAME_RENEWAL_TERM = "renewalTerm";
@SerializedName(SERIALIZED_NAME_RENEWAL_TERM)
private Long renewalTerm;
public static final String SERIALIZED_NAME_RENEWAL_TERM_PERIOD_TYPE = "renewalTermPeriodType";
@SerializedName(SERIALIZED_NAME_RENEWAL_TERM_PERIOD_TYPE)
private TermPeriodType renewalTermPeriodType;
public static final String SERIALIZED_NAME_REVISION = "revision";
@SerializedName(SERIALIZED_NAME_REVISION)
private String revision;
public static final String SERIALIZED_NAME_SEQUENCE_SET_ID = "sequenceSetId";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_ID)
private String sequenceSetId;
public static final String SERIALIZED_NAME_SEQUENCE_SET_NAME = "sequenceSetName";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_NAME)
private String sequenceSetName;
public static final String SERIALIZED_NAME_SERVICE_ACTIVATION_DATE = "serviceActivationDate";
@SerializedName(SERIALIZED_NAME_SERVICE_ACTIVATION_DATE)
private LocalDate serviceActivationDate;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT = "soldToContact";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT)
private Contact soldToContact;
public static final String SERIALIZED_NAME_SHIP_TO_CONTACT = "shipToContact";
@SerializedName(SERIALIZED_NAME_SHIP_TO_CONTACT)
private Contact shipToContact;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private SubscriptionStatus status;
public static final String SERIALIZED_NAME_STATUS_HISTORY = "statusHistory";
@SerializedName(SERIALIZED_NAME_STATUS_HISTORY)
private List statusHistory;
public static final String SERIALIZED_NAME_SUBSCRIPTION_START_DATE = "subscriptionStartDate";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_START_DATE)
private LocalDate subscriptionStartDate;
public static final String SERIALIZED_NAME_SUBSCRIPTION_END_DATE = "subscriptionEndDate";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_END_DATE)
private LocalDate subscriptionEndDate;
public static final String SERIALIZED_NAME_TERM_END_DATE = "termEndDate";
@SerializedName(SERIALIZED_NAME_TERM_END_DATE)
private LocalDate termEndDate;
public static final String SERIALIZED_NAME_TERM_START_DATE = "termStartDate";
@SerializedName(SERIALIZED_NAME_TERM_START_DATE)
private LocalDate termStartDate;
public static final String SERIALIZED_NAME_TERM_TYPE = "termType";
@SerializedName(SERIALIZED_NAME_TERM_TYPE)
private String termType;
public static final String SERIALIZED_NAME_SCHEDULED_CANCEL_DATE = "scheduledCancelDate";
@SerializedName(SERIALIZED_NAME_SCHEDULED_CANCEL_DATE)
private LocalDate scheduledCancelDate;
public static final String SERIALIZED_NAME_SCHEDULED_SUSPEND_DATE = "scheduledSuspendDate";
@SerializedName(SERIALIZED_NAME_SCHEDULED_SUSPEND_DATE)
private LocalDate scheduledSuspendDate;
public static final String SERIALIZED_NAME_SCHEDULED_RESUME_DATE = "scheduledResumeDate";
@SerializedName(SERIALIZED_NAME_SCHEDULED_RESUME_DATE)
private LocalDate scheduledResumeDate;
public static final String SERIALIZED_NAME_TOTAL_CONTRACTED_VALUE = "totalContractedValue";
@SerializedName(SERIALIZED_NAME_TOTAL_CONTRACTED_VALUE)
private BigDecimal totalContractedValue;
public static final String SERIALIZED_NAME_VERSION = "version";
@SerializedName(SERIALIZED_NAME_VERSION)
private Long version;
public static final String SERIALIZED_NAME_CONTRACTED_NET_MRR = "contractedNetMrr";
@SerializedName(SERIALIZED_NAME_CONTRACTED_NET_MRR)
private BigDecimal contractedNetMrr;
public static final String SERIALIZED_NAME_AS_OF_DAY_GROSS_MRR = "asOfDayGrossMrr";
@SerializedName(SERIALIZED_NAME_AS_OF_DAY_GROSS_MRR)
private BigDecimal asOfDayGrossMrr;
public static final String SERIALIZED_NAME_AS_OF_DAY_NET_MRR = "asOfDayNetMrr";
@SerializedName(SERIALIZED_NAME_AS_OF_DAY_NET_MRR)
private BigDecimal asOfDayNetMrr;
public static final String SERIALIZED_NAME_NET_TOTAL_CONTRACTED_VALUE = "netTotalContractedValue";
@SerializedName(SERIALIZED_NAME_NET_TOTAL_CONTRACTED_VALUE)
private BigDecimal netTotalContractedValue;
public static final String SERIALIZED_NAME_ACCOUNT_OWNER_DETAILS = "accountOwnerDetails";
@SerializedName(SERIALIZED_NAME_ACCOUNT_OWNER_DETAILS)
private AccountBasicInfo accountOwnerDetails;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_DETAILS = "invoiceOwnerAccountDetails";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_DETAILS)
private AccountBasicInfo invoiceOwnerAccountDetails;
public GetSubscriptionResponse() {
}
public GetSubscriptionResponse processId(String processId) {
this.processId = processId;
return this;
}
/**
* The Id of the process that handle the operation.
* @return processId
*/
@javax.annotation.Nullable
public String getProcessId() {
return processId;
}
public void setProcessId(String processId) {
this.processId = processId;
}
public GetSubscriptionResponse requestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* Unique request identifier. If you need to contact us about a specific request, providing the request identifier will ensure the fastest possible resolution.
* @return requestId
*/
@javax.annotation.Nullable
public String getRequestId() {
return requestId;
}
public void setRequestId(String requestId) {
this.requestId = requestId;
}
public GetSubscriptionResponse reasons(List reasons) {
this.reasons = reasons;
return this;
}
public GetSubscriptionResponse addReasonsItem(FailedReason reasonsItem) {
if (this.reasons == null) {
this.reasons = new ArrayList<>();
}
this.reasons.add(reasonsItem);
return this;
}
/**
* Get reasons
* @return reasons
*/
@javax.annotation.Nullable
public List getReasons() {
return reasons;
}
public void setReasons(List reasons) {
this.reasons = reasons;
}
public GetSubscriptionResponse success(Boolean success) {
this.success = success;
return this;
}
/**
* Indicates whether the call succeeded.
* @return success
*/
@javax.annotation.Nullable
public Boolean getSuccess() {
return success;
}
public void setSuccess(Boolean success) {
this.success = success;
}
public GetSubscriptionResponse cpqBundleJsonIdQT(String cpqBundleJsonIdQT) {
this.cpqBundleJsonIdQT = cpqBundleJsonIdQT;
return this;
}
/**
* The Bundle product structures from Zuora Quotes if you utilize Bundling in Salesforce. Do not change the value in this field.
* @return cpqBundleJsonIdQT
*/
@javax.annotation.Nullable
public String getCpqBundleJsonIdQT() {
return cpqBundleJsonIdQT;
}
public void setCpqBundleJsonIdQT(String cpqBundleJsonIdQT) {
this.cpqBundleJsonIdQT = cpqBundleJsonIdQT;
}
public GetSubscriptionResponse opportunityCloseDateQT(LocalDate opportunityCloseDateQT) {
this.opportunityCloseDateQT = opportunityCloseDateQT;
return this;
}
/**
* The closing date of the Opportunity. This field is used in Zuora data sources to report on Subscription metrics. If the subscription originated from Zuora Quotes, the value is populated with the value from Zuora Quotes.
* @return opportunityCloseDateQT
*/
@javax.annotation.Nullable
public LocalDate getOpportunityCloseDateQT() {
return opportunityCloseDateQT;
}
public void setOpportunityCloseDateQT(LocalDate opportunityCloseDateQT) {
this.opportunityCloseDateQT = opportunityCloseDateQT;
}
public GetSubscriptionResponse opportunityNameQT(String opportunityNameQT) {
this.opportunityNameQT = opportunityNameQT;
return this;
}
/**
* The unique identifier of the Opportunity. This field is used in Zuora data sources to report on Subscription metrics. If the subscription originated from Zuora Quotes, the value is populated with the value from Zuora Quotes.
* @return opportunityNameQT
*/
@javax.annotation.Nullable
public String getOpportunityNameQT() {
return opportunityNameQT;
}
public void setOpportunityNameQT(String opportunityNameQT) {
this.opportunityNameQT = opportunityNameQT;
}
public GetSubscriptionResponse quoteBusinessTypeQT(String quoteBusinessTypeQT) {
this.quoteBusinessTypeQT = quoteBusinessTypeQT;
return this;
}
/**
* The specific identifier for the type of business transaction the Quote represents such as New, Upsell, Downsell, Renewal or Churn. This field is used in Zuora data sources to report on Subscription metrics. If the subscription originated from Zuora Quotes, the value is populated with the value from Zuora Quotes.
* @return quoteBusinessTypeQT
*/
@javax.annotation.Nullable
public String getQuoteBusinessTypeQT() {
return quoteBusinessTypeQT;
}
public void setQuoteBusinessTypeQT(String quoteBusinessTypeQT) {
this.quoteBusinessTypeQT = quoteBusinessTypeQT;
}
public GetSubscriptionResponse quoteNumberQT(String quoteNumberQT) {
this.quoteNumberQT = quoteNumberQT;
return this;
}
/**
* The unique identifier of the Quote. This field is used in Zuora data sources to report on Subscription metrics. If the subscription originated from Zuora Quotes, the value is populated with the value from Zuora Quotes.
* @return quoteNumberQT
*/
@javax.annotation.Nullable
public String getQuoteNumberQT() {
return quoteNumberQT;
}
public void setQuoteNumberQT(String quoteNumberQT) {
this.quoteNumberQT = quoteNumberQT;
}
public GetSubscriptionResponse quoteTypeQT(String quoteTypeQT) {
this.quoteTypeQT = quoteTypeQT;
return this;
}
/**
* The Quote type that represents the subscription lifecycle stage such as New, Amendment, Renew or Cancel. This field is used in Zuora data sources to report on Subscription metrics. If the subscription originated from Zuora Quotes, the value is populated with the value from Zuora Quotes.
* @return quoteTypeQT
*/
@javax.annotation.Nullable
public String getQuoteTypeQT() {
return quoteTypeQT;
}
public void setQuoteTypeQT(String quoteTypeQT) {
this.quoteTypeQT = quoteTypeQT;
}
public GetSubscriptionResponse integrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
return this;
}
/**
* ID of the corresponding object in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationIdNS
*/
@javax.annotation.Nullable
public String getIntegrationIdNS() {
return integrationIdNS;
}
public void setIntegrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
}
public GetSubscriptionResponse integrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
return this;
}
/**
* Status of the subscription's synchronization with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationStatusNS
*/
@javax.annotation.Nullable
public String getIntegrationStatusNS() {
return integrationStatusNS;
}
public void setIntegrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
}
public GetSubscriptionResponse projectNS(String projectNS) {
this.projectNS = projectNS;
return this;
}
/**
* The NetSuite project that the subscription was created from. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return projectNS
*/
@javax.annotation.Nullable
public String getProjectNS() {
return projectNS;
}
public void setProjectNS(String projectNS) {
this.projectNS = projectNS;
}
public GetSubscriptionResponse salesOrderNS(String salesOrderNS) {
this.salesOrderNS = salesOrderNS;
return this;
}
/**
* The NetSuite sales order than the subscription was created from. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return salesOrderNS
*/
@javax.annotation.Nullable
public String getSalesOrderNS() {
return salesOrderNS;
}
public void setSalesOrderNS(String salesOrderNS) {
this.salesOrderNS = salesOrderNS;
}
public GetSubscriptionResponse syncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
return this;
}
/**
* Date when the subscription was synchronized with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return syncDateNS
*/
@javax.annotation.Nullable
public String getSyncDateNS() {
return syncDateNS;
}
public void setSyncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
}
public GetSubscriptionResponse id(String id) {
this.id = id;
return this;
}
/**
* Subscription ID.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public GetSubscriptionResponse subscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
return this;
}
/**
* Subscription number.
* @return subscriptionNumber
*/
@javax.annotation.Nullable
public String getSubscriptionNumber() {
return subscriptionNumber;
}
public void setSubscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
}
public GetSubscriptionResponse accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* The ID of the account associated with this subscription.
* @return accountId
*/
@javax.annotation.Nullable
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public GetSubscriptionResponse accountName(String accountName) {
this.accountName = accountName;
return this;
}
/**
* The name of the account associated with this subscription.
* @return accountName
*/
@javax.annotation.Nullable
public String getAccountName() {
return accountName;
}
public void setAccountName(String accountName) {
this.accountName = accountName;
}
public GetSubscriptionResponse accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* The number of the account associated with this subscription.
* @return accountNumber
*/
@javax.annotation.Nullable
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public GetSubscriptionResponse autoRenew(Boolean autoRenew) {
this.autoRenew = autoRenew;
return this;
}
/**
* If `true`, the subscription automatically renews at the end of the term. Default is `false`.
* @return autoRenew
*/
@javax.annotation.Nullable
public Boolean getAutoRenew() {
return autoRenew;
}
public void setAutoRenew(Boolean autoRenew) {
this.autoRenew = autoRenew;
}
public GetSubscriptionResponse billToContact(Contact billToContact) {
this.billToContact = billToContact;
return this;
}
/**
* Get billToContact
* @return billToContact
*/
@javax.annotation.Nullable
public Contact getBillToContact() {
return billToContact;
}
public void setBillToContact(Contact billToContact) {
this.billToContact = billToContact;
}
public GetSubscriptionResponse cancelReason(String cancelReason) {
this.cancelReason = cancelReason;
return this;
}
/**
* The reason for a subscription cancellation copied from the `changeReason` field of a Cancel Subscription order action. This field contains valid value only if a subscription is cancelled through the Orders UI or API. Otherwise, the value for this field will always be `null`.
* @return cancelReason
*/
@javax.annotation.Nullable
public String getCancelReason() {
return cancelReason;
}
public void setCancelReason(String cancelReason) {
this.cancelReason = cancelReason;
}
public GetSubscriptionResponse contractEffectiveDate(LocalDate contractEffectiveDate) {
this.contractEffectiveDate = contractEffectiveDate;
return this;
}
/**
* Effective contract date for this subscription, as yyyy-mm-dd.
* @return contractEffectiveDate
*/
@javax.annotation.Nullable
public LocalDate getContractEffectiveDate() {
return contractEffectiveDate;
}
public void setContractEffectiveDate(LocalDate contractEffectiveDate) {
this.contractEffectiveDate = contractEffectiveDate;
}
public GetSubscriptionResponse contractedMrr(BigDecimal contractedMrr) {
this.contractedMrr = contractedMrr;
return this;
}
/**
* Monthly recurring revenue of the subscription.
* @return contractedMrr
*/
@javax.annotation.Nullable
public BigDecimal getContractedMrr() {
return contractedMrr;
}
public void setContractedMrr(BigDecimal contractedMrr) {
this.contractedMrr = contractedMrr;
}
public GetSubscriptionResponse currentTerm(Long currentTerm) {
this.currentTerm = currentTerm;
return this;
}
/**
* The length of the period for the current subscription term.
* @return currentTerm
*/
@javax.annotation.Nullable
public Long getCurrentTerm() {
return currentTerm;
}
public void setCurrentTerm(Long currentTerm) {
this.currentTerm = currentTerm;
}
public GetSubscriptionResponse currentTermPeriodType(TermPeriodType currentTermPeriodType) {
this.currentTermPeriodType = currentTermPeriodType;
return this;
}
/**
* Get currentTermPeriodType
* @return currentTermPeriodType
*/
@javax.annotation.Nullable
public TermPeriodType getCurrentTermPeriodType() {
return currentTermPeriodType;
}
public void setCurrentTermPeriodType(TermPeriodType currentTermPeriodType) {
this.currentTermPeriodType = currentTermPeriodType;
}
public GetSubscriptionResponse customerAcceptanceDate(LocalDate customerAcceptanceDate) {
this.customerAcceptanceDate = customerAcceptanceDate;
return this;
}
/**
* The date on which the services or products within a subscription have been accepted by the customer, as yyyy-mm-dd.
* @return customerAcceptanceDate
*/
@javax.annotation.Nullable
public LocalDate getCustomerAcceptanceDate() {
return customerAcceptanceDate;
}
public void setCustomerAcceptanceDate(LocalDate customerAcceptanceDate) {
this.customerAcceptanceDate = customerAcceptanceDate;
}
public GetSubscriptionResponse currency(String currency) {
this.currency = currency;
return this;
}
/**
* The currency of the subscription.
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public GetSubscriptionResponse createTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* The date when the subscription was created, as yyyy-mm-dd HH:MM:SS.
* @return createTime
*/
@javax.annotation.Nullable
public String getCreateTime() {
return createTime;
}
public void setCreateTime(String createTime) {
this.createTime = createTime;
}
public GetSubscriptionResponse updateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
/**
* The date when the subscription was last updated, as yyyy-mm-dd HH:MM:SS.
* @return updateTime
*/
@javax.annotation.Nullable
public String getUpdateTime() {
return updateTime;
}
public void setUpdateTime(String updateTime) {
this.updateTime = updateTime;
}
public GetSubscriptionResponse externallyManagedBy(ExternallyManagedBy externallyManagedBy) {
this.externallyManagedBy = externallyManagedBy;
return this;
}
/**
* Get externallyManagedBy
* @return externallyManagedBy
*/
@javax.annotation.Nullable
public ExternallyManagedBy getExternallyManagedBy() {
return externallyManagedBy;
}
public void setExternallyManagedBy(ExternallyManagedBy externallyManagedBy) {
this.externallyManagedBy = externallyManagedBy;
}
public GetSubscriptionResponse initialTerm(Long initialTerm) {
this.initialTerm = initialTerm;
return this;
}
/**
* The length of the period for the first subscription term.
* @return initialTerm
*/
@javax.annotation.Nullable
public Long getInitialTerm() {
return initialTerm;
}
public void setInitialTerm(Long initialTerm) {
this.initialTerm = initialTerm;
}
public GetSubscriptionResponse initialTermPeriodType(TermPeriodType initialTermPeriodType) {
this.initialTermPeriodType = initialTermPeriodType;
return this;
}
/**
* Get initialTermPeriodType
* @return initialTermPeriodType
*/
@javax.annotation.Nullable
public TermPeriodType getInitialTermPeriodType() {
return initialTermPeriodType;
}
public void setInitialTermPeriodType(TermPeriodType initialTermPeriodType) {
this.initialTermPeriodType = initialTermPeriodType;
}
public GetSubscriptionResponse invoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
return this;
}
/**
* The number of invoice group associated with the subscription. **Note**: This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_customers_at_subscription_level/Flexible_Billing_Attributes\" target=\"_blank\">Flexible Billing Attributes</a> feature enabled.
* @return invoiceGroupNumber
*/
@javax.annotation.Nullable
public String getInvoiceGroupNumber() {
return invoiceGroupNumber;
}
public void setInvoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
}
public GetSubscriptionResponse invoiceOwnerAccountId(String invoiceOwnerAccountId) {
this.invoiceOwnerAccountId = invoiceOwnerAccountId;
return this;
}
/**
*
* @return invoiceOwnerAccountId
*/
@javax.annotation.Nullable
public String getInvoiceOwnerAccountId() {
return invoiceOwnerAccountId;
}
public void setInvoiceOwnerAccountId(String invoiceOwnerAccountId) {
this.invoiceOwnerAccountId = invoiceOwnerAccountId;
}
public GetSubscriptionResponse invoiceOwnerAccountName(String invoiceOwnerAccountName) {
this.invoiceOwnerAccountName = invoiceOwnerAccountName;
return this;
}
/**
*
* @return invoiceOwnerAccountName
*/
@javax.annotation.Nullable
public String getInvoiceOwnerAccountName() {
return invoiceOwnerAccountName;
}
public void setInvoiceOwnerAccountName(String invoiceOwnerAccountName) {
this.invoiceOwnerAccountName = invoiceOwnerAccountName;
}
public GetSubscriptionResponse invoiceOwnerAccountNumber(String invoiceOwnerAccountNumber) {
this.invoiceOwnerAccountNumber = invoiceOwnerAccountNumber;
return this;
}
/**
*
* @return invoiceOwnerAccountNumber
*/
@javax.annotation.Nullable
public String getInvoiceOwnerAccountNumber() {
return invoiceOwnerAccountNumber;
}
public void setInvoiceOwnerAccountNumber(String invoiceOwnerAccountNumber) {
this.invoiceOwnerAccountNumber = invoiceOwnerAccountNumber;
}
public GetSubscriptionResponse invoiceScheduleId(String invoiceScheduleId) {
this.invoiceScheduleId = invoiceScheduleId;
return this;
}
/**
* The ID of the invoice schedule associated with the subscription. If multiple invoice schedules are created for different terms of a subscription, this field stores the latest invoice schedule. **Note**: This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Billing_Schedule\" target=\"_blank\">Billing Schedule</a> feature in the **Early Adopter** phase enabled.
* @return invoiceScheduleId
*/
@javax.annotation.Nullable
public String getInvoiceScheduleId() {
return invoiceScheduleId;
}
public void setInvoiceScheduleId(String invoiceScheduleId) {
this.invoiceScheduleId = invoiceScheduleId;
}
public GetSubscriptionResponse invoiceSeparately(String invoiceSeparately) {
this.invoiceSeparately = invoiceSeparately;
return this;
}
/**
* Separates a single subscription from other subscriptions and creates an invoice for the subscription. If the value is `true`, the subscription is billed separately from other subscriptions. If the value is `false`, the subscription is included with other subscriptions in the account invoice.
* @return invoiceSeparately
*/
@javax.annotation.Nullable
public String getInvoiceSeparately() {
return invoiceSeparately;
}
public void setInvoiceSeparately(String invoiceSeparately) {
this.invoiceSeparately = invoiceSeparately;
}
public GetSubscriptionResponse invoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
return this;
}
/**
* The ID of the invoice template associated with the subscription. **Note**: - If you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_customers_at_subscription_level/Flexible_Billing_Attributes\" target=\"_blank\">Flexible Billing Attributes</a> feature disabled, this field is unavailable in the request body and the value of this field is `null` in the response body. - If you have the Flexible Billing Attributes feature enabled, and you do not specify this field in the request or you select **Default Template from Account** for this field during subscription creation, the value of this field is automatically set to `null` in the response body.
* @return invoiceTemplateId
*/
@javax.annotation.Nullable
public String getInvoiceTemplateId() {
return invoiceTemplateId;
}
public void setInvoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
}
public GetSubscriptionResponse invoiceTemplateName(String invoiceTemplateName) {
this.invoiceTemplateName = invoiceTemplateName;
return this;
}
/**
* The name of the invoice template associated with the subscription. **Note**: - If you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_customers_at_subscription_level/Flexible_Billing_Attributes\" target=\"_blank\">Flexible Billing Attributes</a> feature disabled, the value of this field is `null` in the response body. - If you have the Flexible Billing Attributes feature enabled, and you do not specify the `invoiceTemplateId` field in the request or you select **Default Template from Account** for the `invoiceTemplateId` field during subscription creation, the value of the `invoiceTemplateName` field is automatically set to `null` in the response body.
* @return invoiceTemplateName
*/
@javax.annotation.Nullable
public String getInvoiceTemplateName() {
return invoiceTemplateName;
}
public void setInvoiceTemplateName(String invoiceTemplateName) {
this.invoiceTemplateName = invoiceTemplateName;
}
public GetSubscriptionResponse isLatestVersion(Boolean isLatestVersion) {
this.isLatestVersion = isLatestVersion;
return this;
}
/**
* If `true`, the current subscription object is the latest version.
* @return isLatestVersion
*/
@javax.annotation.Nullable
public Boolean getIsLatestVersion() {
return isLatestVersion;
}
public void setIsLatestVersion(Boolean isLatestVersion) {
this.isLatestVersion = isLatestVersion;
}
public GetSubscriptionResponse lastBookingDate(LocalDate lastBookingDate) {
this.lastBookingDate = lastBookingDate;
return this;
}
/**
* The last booking date of the subscription object. This field is writable only when the subscription is newly created as a first version subscription. You can override the date value when creating a subscription through the Subscribe and Amend API or the subscription creation UI (non-Orders). Otherwise, the default value `today` is set per the user's timezone. The value of this field is as follows: * For a new subscription created by the [Subscribe and Amend APIs](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/Orders_Harmonization/Orders_Migration_Guidance#Subscribe_and_Amend_APIs_to_Migrate), this field has the value of the subscription creation date. * For a subscription changed by an amendment, this field has the value of the amendment booking date. * For a subscription created or changed by an order, this field has the value of the order date.
* @return lastBookingDate
*/
@javax.annotation.Nullable
public LocalDate getLastBookingDate() {
return lastBookingDate;
}
public void setLastBookingDate(LocalDate lastBookingDate) {
this.lastBookingDate = lastBookingDate;
}
public GetSubscriptionResponse notes(String notes) {
this.notes = notes;
return this;
}
/**
* A string of up to 65,535 characters.
* @return notes
*/
@javax.annotation.Nullable
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
this.notes = notes;
}
public GetSubscriptionResponse orderNumber(String orderNumber) {
this.orderNumber = orderNumber;
return this;
}
/**
* The order number of the order in which the changes on the subscription are made. **Note:** This field is only available if you have the [Order Metrics](https://knowledgecenter.zuora.com/BC_Subscription_Management/Orders/AA_Overview_of_Orders#Order_Metrics) feature enabled. If you wish to have access to the feature, submit a request at [Zuora Global Support](http://support.zuora.com/). We will investigate your use cases and data before enabling this feature for you.
* @return orderNumber
*/
@javax.annotation.Nullable
public String getOrderNumber() {
return orderNumber;
}
public void setOrderNumber(String orderNumber) {
this.orderNumber = orderNumber;
}
public GetSubscriptionResponse paymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
return this;
}
/**
* The name of the payment term associated with the subscription. For example, `Net 30`. The payment term determines the due dates of invoices. **Note**: - If you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_customers_at_subscription_level/Flexible_Billing_Attributes\" target=\"_blank\">Flexible Billing Attributes</a> feature disabled, this field is unavailable in the request body and the value of this field is `null` in the response body. - If you have the Flexible Billing Attributes feature enabled, and you do not specify this field in the request or you select **Default Term from Account** for this field during subscription creation, the value of this field is automatically set to `null` in the response body.
* @return paymentTerm
*/
@javax.annotation.Nullable
public String getPaymentTerm() {
return paymentTerm;
}
public void setPaymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
}
public GetSubscriptionResponse ratePlans(List ratePlans) {
this.ratePlans = ratePlans;
return this;
}
public GetSubscriptionResponse addRatePlansItem(SubscriptionRatePlan ratePlansItem) {
if (this.ratePlans == null) {
this.ratePlans = new ArrayList<>();
}
this.ratePlans.add(ratePlansItem);
return this;
}
/**
* Container for rate plans.
* @return ratePlans
*/
@javax.annotation.Nullable
public List getRatePlans() {
return ratePlans;
}
public void setRatePlans(List ratePlans) {
this.ratePlans = ratePlans;
}
public GetSubscriptionResponse renewalSetting(String renewalSetting) {
this.renewalSetting = renewalSetting;
return this;
}
/**
* Specifies whether a termed subscription will remain `TERMED` or change to `EVERGREEN` when it is renewed. Values are: * `RENEW_WITH_SPECIFIC_TERM` (default) * `RENEW_TO_EVERGREEN`
* @return renewalSetting
*/
@javax.annotation.Nullable
public String getRenewalSetting() {
return renewalSetting;
}
public void setRenewalSetting(String renewalSetting) {
this.renewalSetting = renewalSetting;
}
public GetSubscriptionResponse renewalTerm(Long renewalTerm) {
this.renewalTerm = renewalTerm;
return this;
}
/**
* The length of the period for the subscription renewal term.
* @return renewalTerm
*/
@javax.annotation.Nullable
public Long getRenewalTerm() {
return renewalTerm;
}
public void setRenewalTerm(Long renewalTerm) {
this.renewalTerm = renewalTerm;
}
public GetSubscriptionResponse renewalTermPeriodType(TermPeriodType renewalTermPeriodType) {
this.renewalTermPeriodType = renewalTermPeriodType;
return this;
}
/**
* Get renewalTermPeriodType
* @return renewalTermPeriodType
*/
@javax.annotation.Nullable
public TermPeriodType getRenewalTermPeriodType() {
return renewalTermPeriodType;
}
public void setRenewalTermPeriodType(TermPeriodType renewalTermPeriodType) {
this.renewalTermPeriodType = renewalTermPeriodType;
}
public GetSubscriptionResponse revision(String revision) {
this.revision = revision;
return this;
}
/**
* An auto-generated decimal value uniquely tagged with a subscription. The value always contains one decimal place, for example, the revision of a new subscription is 1.0. If a further version of the subscription is created, the revision value will be increased by 1. Also, the revision value is always incremental regardless of deletion of subscription versions.
* @return revision
*/
@javax.annotation.Nullable
public String getRevision() {
return revision;
}
public void setRevision(String revision) {
this.revision = revision;
}
public GetSubscriptionResponse sequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
return this;
}
/**
* The ID of the sequence set associated with the subscription. **Note**: - If you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_customers_at_subscription_level/Flexible_Billing_Attributes\" target=\"_blank\">Flexible Billing Attributes</a> feature disabled, this field is unavailable in the request body and the value of this field is `null` in the response body. - If you have the Flexible Billing Attributes feature enabled, and you do not specify this field in the request or you select **Default Set from Account** for this field during subscription creation, the value of this field is automatically set to `null` in the response body.
* @return sequenceSetId
*/
@javax.annotation.Nullable
public String getSequenceSetId() {
return sequenceSetId;
}
public void setSequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
}
public GetSubscriptionResponse sequenceSetName(String sequenceSetName) {
this.sequenceSetName = sequenceSetName;
return this;
}
/**
* The name of the sequence set associated with the subscription. **Note**: - If you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Bill_customers_at_subscription_level/Flexible_Billing_Attributes\" target=\"_blank\">Flexible Billing Attributes</a> feature disabled, the value of this field is `null` in the response body. - If you have the Flexible Billing Attributes feature enabled, and you do not specify the `sequenceSetId` field in the request or you select **Default Template from Account** for the `sequenceSetId` field during subscription creation, the value of the `sequenceSetName` field is automatically set to `null` in the response body.
* @return sequenceSetName
*/
@javax.annotation.Nullable
public String getSequenceSetName() {
return sequenceSetName;
}
public void setSequenceSetName(String sequenceSetName) {
this.sequenceSetName = sequenceSetName;
}
public GetSubscriptionResponse serviceActivationDate(LocalDate serviceActivationDate) {
this.serviceActivationDate = serviceActivationDate;
return this;
}
/**
* The date on which the services or products within a subscription have been activated and access has been provided to the customer, as yyyy-mm-dd
* @return serviceActivationDate
*/
@javax.annotation.Nullable
public LocalDate getServiceActivationDate() {
return serviceActivationDate;
}
public void setServiceActivationDate(LocalDate serviceActivationDate) {
this.serviceActivationDate = serviceActivationDate;
}
public GetSubscriptionResponse soldToContact(Contact soldToContact) {
this.soldToContact = soldToContact;
return this;
}
/**
* Get soldToContact
* @return soldToContact
*/
@javax.annotation.Nullable
public Contact getSoldToContact() {
return soldToContact;
}
public void setSoldToContact(Contact soldToContact) {
this.soldToContact = soldToContact;
}
public GetSubscriptionResponse shipToContact(Contact shipToContact) {
this.shipToContact = shipToContact;
return this;
}
/**
* Get shipToContact
* @return shipToContact
*/
@javax.annotation.Nullable
public Contact getShipToContact() {
return shipToContact;
}
public void setShipToContact(Contact shipToContact) {
this.shipToContact = shipToContact;
}
public GetSubscriptionResponse status(SubscriptionStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public SubscriptionStatus getStatus() {
return status;
}
public void setStatus(SubscriptionStatus status) {
this.status = status;
}
public GetSubscriptionResponse statusHistory(List statusHistory) {
this.statusHistory = statusHistory;
return this;
}
public GetSubscriptionResponse addStatusHistoryItem(SubscriptionStatusHistory statusHistoryItem) {
if (this.statusHistory == null) {
this.statusHistory = new ArrayList<>();
}
this.statusHistory.add(statusHistoryItem);
return this;
}
/**
* Container for status history.
* @return statusHistory
*/
@javax.annotation.Nullable
public List getStatusHistory() {
return statusHistory;
}
public void setStatusHistory(List statusHistory) {
this.statusHistory = statusHistory;
}
public GetSubscriptionResponse subscriptionStartDate(LocalDate subscriptionStartDate) {
this.subscriptionStartDate = subscriptionStartDate;
return this;
}
/**
* Date the subscription becomes effective.
* @return subscriptionStartDate
*/
@javax.annotation.Nullable
public LocalDate getSubscriptionStartDate() {
return subscriptionStartDate;
}
public void setSubscriptionStartDate(LocalDate subscriptionStartDate) {
this.subscriptionStartDate = subscriptionStartDate;
}
public GetSubscriptionResponse subscriptionEndDate(LocalDate subscriptionEndDate) {
this.subscriptionEndDate = subscriptionEndDate;
return this;
}
/**
* The date when the subscription term ends, where the subscription ends at midnight the day before. For example, if the `subscriptionEndDate` is 12/31/2016, the subscriptions ends at midnight (00:00:00 hours) on 12/30/2016. This date is the same as the term end date or the cancelation date, as appropriate.
* @return subscriptionEndDate
*/
@javax.annotation.Nullable
public LocalDate getSubscriptionEndDate() {
return subscriptionEndDate;
}
public void setSubscriptionEndDate(LocalDate subscriptionEndDate) {
this.subscriptionEndDate = subscriptionEndDate;
}
public GetSubscriptionResponse termEndDate(LocalDate termEndDate) {
this.termEndDate = termEndDate;
return this;
}
/**
* Date the subscription term ends. If the subscription is evergreen, this is null or is the cancellation date (if one has been set).
* @return termEndDate
*/
@javax.annotation.Nullable
public LocalDate getTermEndDate() {
return termEndDate;
}
public void setTermEndDate(LocalDate termEndDate) {
this.termEndDate = termEndDate;
}
public GetSubscriptionResponse termStartDate(LocalDate termStartDate) {
this.termStartDate = termStartDate;
return this;
}
/**
* Date the subscription term begins. If this is a renewal subscription, this date is different from the subscription start date.
* @return termStartDate
*/
@javax.annotation.Nullable
public LocalDate getTermStartDate() {
return termStartDate;
}
public void setTermStartDate(LocalDate termStartDate) {
this.termStartDate = termStartDate;
}
public GetSubscriptionResponse termType(String termType) {
this.termType = termType;
return this;
}
/**
* Possible values are: `TERMED`, `EVERGREEN`.
* @return termType
*/
@javax.annotation.Nullable
public String getTermType() {
return termType;
}
public void setTermType(String termType) {
this.termType = termType;
}
public GetSubscriptionResponse scheduledCancelDate(LocalDate scheduledCancelDate) {
this.scheduledCancelDate = scheduledCancelDate;
return this;
}
/**
* Get scheduledCancelDate
* @return scheduledCancelDate
*/
@javax.annotation.Nullable
public LocalDate getScheduledCancelDate() {
return scheduledCancelDate;
}
public void setScheduledCancelDate(LocalDate scheduledCancelDate) {
this.scheduledCancelDate = scheduledCancelDate;
}
public GetSubscriptionResponse scheduledSuspendDate(LocalDate scheduledSuspendDate) {
this.scheduledSuspendDate = scheduledSuspendDate;
return this;
}
/**
* Get scheduledSuspendDate
* @return scheduledSuspendDate
*/
@javax.annotation.Nullable
public LocalDate getScheduledSuspendDate() {
return scheduledSuspendDate;
}
public void setScheduledSuspendDate(LocalDate scheduledSuspendDate) {
this.scheduledSuspendDate = scheduledSuspendDate;
}
public GetSubscriptionResponse scheduledResumeDate(LocalDate scheduledResumeDate) {
this.scheduledResumeDate = scheduledResumeDate;
return this;
}
/**
* Get scheduledResumeDate
* @return scheduledResumeDate
*/
@javax.annotation.Nullable
public LocalDate getScheduledResumeDate() {
return scheduledResumeDate;
}
public void setScheduledResumeDate(LocalDate scheduledResumeDate) {
this.scheduledResumeDate = scheduledResumeDate;
}
public GetSubscriptionResponse totalContractedValue(BigDecimal totalContractedValue) {
this.totalContractedValue = totalContractedValue;
return this;
}
/**
* Total contracted value of the subscription.
* @return totalContractedValue
*/
@javax.annotation.Nullable
public BigDecimal getTotalContractedValue() {
return totalContractedValue;
}
public void setTotalContractedValue(BigDecimal totalContractedValue) {
this.totalContractedValue = totalContractedValue;
}
public GetSubscriptionResponse version(Long version) {
this.version = version;
return this;
}
/**
* This is the subscription version automatically generated by Zuora Billing. Each order or amendment creates a new version of the subscription, which incorporates the changes made in the order or amendment.
* @return version
*/
@javax.annotation.Nullable
public Long getVersion() {
return version;
}
public void setVersion(Long version) {
this.version = version;
}
public GetSubscriptionResponse contractedNetMrr(BigDecimal contractedNetMrr) {
this.contractedNetMrr = contractedNetMrr;
return this;
}
/**
* Monthly recurring revenue of the subscription inclusive of all the discounts applicable, including the fixed-amount discounts and percentage discounts.
* @return contractedNetMrr
*/
@javax.annotation.Nullable
public BigDecimal getContractedNetMrr() {
return contractedNetMrr;
}
public void setContractedNetMrr(BigDecimal contractedNetMrr) {
this.contractedNetMrr = contractedNetMrr;
}
public GetSubscriptionResponse asOfDayGrossMrr(BigDecimal asOfDayGrossMrr) {
this.asOfDayGrossMrr = asOfDayGrossMrr;
return this;
}
/**
* Monthly recurring revenue of the subscription exclusive of any discounts applicable as of specified day.
* @return asOfDayGrossMrr
*/
@javax.annotation.Nullable
public BigDecimal getAsOfDayGrossMrr() {
return asOfDayGrossMrr;
}
public void setAsOfDayGrossMrr(BigDecimal asOfDayGrossMrr) {
this.asOfDayGrossMrr = asOfDayGrossMrr;
}
public GetSubscriptionResponse asOfDayNetMrr(BigDecimal asOfDayNetMrr) {
this.asOfDayNetMrr = asOfDayNetMrr;
return this;
}
/**
* Monthly recurring revenue of the subscription inclusive of all the discounts applicable, including the fixed-amount discounts and percentage discounts as of specified day.
* @return asOfDayNetMrr
*/
@javax.annotation.Nullable
public BigDecimal getAsOfDayNetMrr() {
return asOfDayNetMrr;
}
public void setAsOfDayNetMrr(BigDecimal asOfDayNetMrr) {
this.asOfDayNetMrr = asOfDayNetMrr;
}
public GetSubscriptionResponse netTotalContractedValue(BigDecimal netTotalContractedValue) {
this.netTotalContractedValue = netTotalContractedValue;
return this;
}
/**
* Total contracted value of the subscription inclusive of all the discounts applicable, including the fixed-amount discounts and percentage discounts.
* @return netTotalContractedValue
*/
@javax.annotation.Nullable
public BigDecimal getNetTotalContractedValue() {
return netTotalContractedValue;
}
public void setNetTotalContractedValue(BigDecimal netTotalContractedValue) {
this.netTotalContractedValue = netTotalContractedValue;
}
public GetSubscriptionResponse accountOwnerDetails(AccountBasicInfo accountOwnerDetails) {
this.accountOwnerDetails = accountOwnerDetails;
return this;
}
/**
* Get accountOwnerDetails
* @return accountOwnerDetails
*/
@javax.annotation.Nullable
public AccountBasicInfo getAccountOwnerDetails() {
return accountOwnerDetails;
}
public void setAccountOwnerDetails(AccountBasicInfo accountOwnerDetails) {
this.accountOwnerDetails = accountOwnerDetails;
}
public GetSubscriptionResponse invoiceOwnerAccountDetails(AccountBasicInfo invoiceOwnerAccountDetails) {
this.invoiceOwnerAccountDetails = invoiceOwnerAccountDetails;
return this;
}
/**
* Get invoiceOwnerAccountDetails
* @return invoiceOwnerAccountDetails
*/
@javax.annotation.Nullable
public AccountBasicInfo getInvoiceOwnerAccountDetails() {
return invoiceOwnerAccountDetails;
}
public void setInvoiceOwnerAccountDetails(AccountBasicInfo invoiceOwnerAccountDetails) {
this.invoiceOwnerAccountDetails = invoiceOwnerAccountDetails;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the GetSubscriptionResponse instance itself
*/
public GetSubscriptionResponse putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GetSubscriptionResponse getSubscriptionResponse = (GetSubscriptionResponse) o;
return Objects.equals(this.processId, getSubscriptionResponse.processId) &&
Objects.equals(this.requestId, getSubscriptionResponse.requestId) &&
Objects.equals(this.reasons, getSubscriptionResponse.reasons) &&
Objects.equals(this.success, getSubscriptionResponse.success) &&
Objects.equals(this.cpqBundleJsonIdQT, getSubscriptionResponse.cpqBundleJsonIdQT) &&
Objects.equals(this.opportunityCloseDateQT, getSubscriptionResponse.opportunityCloseDateQT) &&
Objects.equals(this.opportunityNameQT, getSubscriptionResponse.opportunityNameQT) &&
Objects.equals(this.quoteBusinessTypeQT, getSubscriptionResponse.quoteBusinessTypeQT) &&
Objects.equals(this.quoteNumberQT, getSubscriptionResponse.quoteNumberQT) &&
Objects.equals(this.quoteTypeQT, getSubscriptionResponse.quoteTypeQT) &&
Objects.equals(this.integrationIdNS, getSubscriptionResponse.integrationIdNS) &&
Objects.equals(this.integrationStatusNS, getSubscriptionResponse.integrationStatusNS) &&
Objects.equals(this.projectNS, getSubscriptionResponse.projectNS) &&
Objects.equals(this.salesOrderNS, getSubscriptionResponse.salesOrderNS) &&
Objects.equals(this.syncDateNS, getSubscriptionResponse.syncDateNS) &&
Objects.equals(this.id, getSubscriptionResponse.id) &&
Objects.equals(this.subscriptionNumber, getSubscriptionResponse.subscriptionNumber) &&
Objects.equals(this.accountId, getSubscriptionResponse.accountId) &&
Objects.equals(this.accountName, getSubscriptionResponse.accountName) &&
Objects.equals(this.accountNumber, getSubscriptionResponse.accountNumber) &&
Objects.equals(this.autoRenew, getSubscriptionResponse.autoRenew) &&
Objects.equals(this.billToContact, getSubscriptionResponse.billToContact) &&
Objects.equals(this.cancelReason, getSubscriptionResponse.cancelReason) &&
Objects.equals(this.contractEffectiveDate, getSubscriptionResponse.contractEffectiveDate) &&
Objects.equals(this.contractedMrr, getSubscriptionResponse.contractedMrr) &&
Objects.equals(this.currentTerm, getSubscriptionResponse.currentTerm) &&
Objects.equals(this.currentTermPeriodType, getSubscriptionResponse.currentTermPeriodType) &&
Objects.equals(this.customerAcceptanceDate, getSubscriptionResponse.customerAcceptanceDate) &&
Objects.equals(this.currency, getSubscriptionResponse.currency) &&
Objects.equals(this.createTime, getSubscriptionResponse.createTime) &&
Objects.equals(this.updateTime, getSubscriptionResponse.updateTime) &&
Objects.equals(this.externallyManagedBy, getSubscriptionResponse.externallyManagedBy) &&
Objects.equals(this.initialTerm, getSubscriptionResponse.initialTerm) &&
Objects.equals(this.initialTermPeriodType, getSubscriptionResponse.initialTermPeriodType) &&
Objects.equals(this.invoiceGroupNumber, getSubscriptionResponse.invoiceGroupNumber) &&
Objects.equals(this.invoiceOwnerAccountId, getSubscriptionResponse.invoiceOwnerAccountId) &&
Objects.equals(this.invoiceOwnerAccountName, getSubscriptionResponse.invoiceOwnerAccountName) &&
Objects.equals(this.invoiceOwnerAccountNumber, getSubscriptionResponse.invoiceOwnerAccountNumber) &&
Objects.equals(this.invoiceScheduleId, getSubscriptionResponse.invoiceScheduleId) &&
Objects.equals(this.invoiceSeparately, getSubscriptionResponse.invoiceSeparately) &&
Objects.equals(this.invoiceTemplateId, getSubscriptionResponse.invoiceTemplateId) &&
Objects.equals(this.invoiceTemplateName, getSubscriptionResponse.invoiceTemplateName) &&
Objects.equals(this.isLatestVersion, getSubscriptionResponse.isLatestVersion) &&
Objects.equals(this.lastBookingDate, getSubscriptionResponse.lastBookingDate) &&
Objects.equals(this.notes, getSubscriptionResponse.notes) &&
Objects.equals(this.orderNumber, getSubscriptionResponse.orderNumber) &&
Objects.equals(this.paymentTerm, getSubscriptionResponse.paymentTerm) &&
Objects.equals(this.ratePlans, getSubscriptionResponse.ratePlans) &&
Objects.equals(this.renewalSetting, getSubscriptionResponse.renewalSetting) &&
Objects.equals(this.renewalTerm, getSubscriptionResponse.renewalTerm) &&
Objects.equals(this.renewalTermPeriodType, getSubscriptionResponse.renewalTermPeriodType) &&
Objects.equals(this.revision, getSubscriptionResponse.revision) &&
Objects.equals(this.sequenceSetId, getSubscriptionResponse.sequenceSetId) &&
Objects.equals(this.sequenceSetName, getSubscriptionResponse.sequenceSetName) &&
Objects.equals(this.serviceActivationDate, getSubscriptionResponse.serviceActivationDate) &&
Objects.equals(this.soldToContact, getSubscriptionResponse.soldToContact) &&
Objects.equals(this.shipToContact, getSubscriptionResponse.shipToContact) &&
Objects.equals(this.status, getSubscriptionResponse.status) &&
Objects.equals(this.statusHistory, getSubscriptionResponse.statusHistory) &&
Objects.equals(this.subscriptionStartDate, getSubscriptionResponse.subscriptionStartDate) &&
Objects.equals(this.subscriptionEndDate, getSubscriptionResponse.subscriptionEndDate) &&
Objects.equals(this.termEndDate, getSubscriptionResponse.termEndDate) &&
Objects.equals(this.termStartDate, getSubscriptionResponse.termStartDate) &&
Objects.equals(this.termType, getSubscriptionResponse.termType) &&
Objects.equals(this.scheduledCancelDate, getSubscriptionResponse.scheduledCancelDate) &&
Objects.equals(this.scheduledSuspendDate, getSubscriptionResponse.scheduledSuspendDate) &&
Objects.equals(this.scheduledResumeDate, getSubscriptionResponse.scheduledResumeDate) &&
Objects.equals(this.totalContractedValue, getSubscriptionResponse.totalContractedValue) &&
Objects.equals(this.version, getSubscriptionResponse.version) &&
Objects.equals(this.contractedNetMrr, getSubscriptionResponse.contractedNetMrr) &&
Objects.equals(this.asOfDayGrossMrr, getSubscriptionResponse.asOfDayGrossMrr) &&
Objects.equals(this.asOfDayNetMrr, getSubscriptionResponse.asOfDayNetMrr) &&
Objects.equals(this.netTotalContractedValue, getSubscriptionResponse.netTotalContractedValue) &&
Objects.equals(this.accountOwnerDetails, getSubscriptionResponse.accountOwnerDetails) &&
Objects.equals(this.invoiceOwnerAccountDetails, getSubscriptionResponse.invoiceOwnerAccountDetails)&&
Objects.equals(this.additionalProperties, getSubscriptionResponse.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(processId, requestId, reasons, success, cpqBundleJsonIdQT, opportunityCloseDateQT, opportunityNameQT, quoteBusinessTypeQT, quoteNumberQT, quoteTypeQT, integrationIdNS, integrationStatusNS, projectNS, salesOrderNS, syncDateNS, id, subscriptionNumber, accountId, accountName, accountNumber, autoRenew, billToContact, cancelReason, contractEffectiveDate, contractedMrr, currentTerm, currentTermPeriodType, customerAcceptanceDate, currency, createTime, updateTime, externallyManagedBy, initialTerm, initialTermPeriodType, invoiceGroupNumber, invoiceOwnerAccountId, invoiceOwnerAccountName, invoiceOwnerAccountNumber, invoiceScheduleId, invoiceSeparately, invoiceTemplateId, invoiceTemplateName, isLatestVersion, lastBookingDate, notes, orderNumber, paymentTerm, ratePlans, renewalSetting, renewalTerm, renewalTermPeriodType, revision, sequenceSetId, sequenceSetName, serviceActivationDate, soldToContact, shipToContact, status, statusHistory, subscriptionStartDate, subscriptionEndDate, termEndDate, termStartDate, termType, scheduledCancelDate, scheduledSuspendDate, scheduledResumeDate, totalContractedValue, version, contractedNetMrr, asOfDayGrossMrr, asOfDayNetMrr, netTotalContractedValue, accountOwnerDetails, invoiceOwnerAccountDetails, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GetSubscriptionResponse {\n");
sb.append(" processId: ").append(toIndentedString(processId)).append("\n");
sb.append(" requestId: ").append(toIndentedString(requestId)).append("\n");
sb.append(" reasons: ").append(toIndentedString(reasons)).append("\n");
sb.append(" success: ").append(toIndentedString(success)).append("\n");
sb.append(" cpqBundleJsonIdQT: ").append(toIndentedString(cpqBundleJsonIdQT)).append("\n");
sb.append(" opportunityCloseDateQT: ").append(toIndentedString(opportunityCloseDateQT)).append("\n");
sb.append(" opportunityNameQT: ").append(toIndentedString(opportunityNameQT)).append("\n");
sb.append(" quoteBusinessTypeQT: ").append(toIndentedString(quoteBusinessTypeQT)).append("\n");
sb.append(" quoteNumberQT: ").append(toIndentedString(quoteNumberQT)).append("\n");
sb.append(" quoteTypeQT: ").append(toIndentedString(quoteTypeQT)).append("\n");
sb.append(" integrationIdNS: ").append(toIndentedString(integrationIdNS)).append("\n");
sb.append(" integrationStatusNS: ").append(toIndentedString(integrationStatusNS)).append("\n");
sb.append(" projectNS: ").append(toIndentedString(projectNS)).append("\n");
sb.append(" salesOrderNS: ").append(toIndentedString(salesOrderNS)).append("\n");
sb.append(" syncDateNS: ").append(toIndentedString(syncDateNS)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" subscriptionNumber: ").append(toIndentedString(subscriptionNumber)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountName: ").append(toIndentedString(accountName)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" autoRenew: ").append(toIndentedString(autoRenew)).append("\n");
sb.append(" billToContact: ").append(toIndentedString(billToContact)).append("\n");
sb.append(" cancelReason: ").append(toIndentedString(cancelReason)).append("\n");
sb.append(" contractEffectiveDate: ").append(toIndentedString(contractEffectiveDate)).append("\n");
sb.append(" contractedMrr: ").append(toIndentedString(contractedMrr)).append("\n");
sb.append(" currentTerm: ").append(toIndentedString(currentTerm)).append("\n");
sb.append(" currentTermPeriodType: ").append(toIndentedString(currentTermPeriodType)).append("\n");
sb.append(" customerAcceptanceDate: ").append(toIndentedString(customerAcceptanceDate)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" createTime: ").append(toIndentedString(createTime)).append("\n");
sb.append(" updateTime: ").append(toIndentedString(updateTime)).append("\n");
sb.append(" externallyManagedBy: ").append(toIndentedString(externallyManagedBy)).append("\n");
sb.append(" initialTerm: ").append(toIndentedString(initialTerm)).append("\n");
sb.append(" initialTermPeriodType: ").append(toIndentedString(initialTermPeriodType)).append("\n");
sb.append(" invoiceGroupNumber: ").append(toIndentedString(invoiceGroupNumber)).append("\n");
sb.append(" invoiceOwnerAccountId: ").append(toIndentedString(invoiceOwnerAccountId)).append("\n");
sb.append(" invoiceOwnerAccountName: ").append(toIndentedString(invoiceOwnerAccountName)).append("\n");
sb.append(" invoiceOwnerAccountNumber: ").append(toIndentedString(invoiceOwnerAccountNumber)).append("\n");
sb.append(" invoiceScheduleId: ").append(toIndentedString(invoiceScheduleId)).append("\n");
sb.append(" invoiceSeparately: ").append(toIndentedString(invoiceSeparately)).append("\n");
sb.append(" invoiceTemplateId: ").append(toIndentedString(invoiceTemplateId)).append("\n");
sb.append(" invoiceTemplateName: ").append(toIndentedString(invoiceTemplateName)).append("\n");
sb.append(" isLatestVersion: ").append(toIndentedString(isLatestVersion)).append("\n");
sb.append(" lastBookingDate: ").append(toIndentedString(lastBookingDate)).append("\n");
sb.append(" notes: ").append(toIndentedString(notes)).append("\n");
sb.append(" orderNumber: ").append(toIndentedString(orderNumber)).append("\n");
sb.append(" paymentTerm: ").append(toIndentedString(paymentTerm)).append("\n");
sb.append(" ratePlans: ").append(toIndentedString(ratePlans)).append("\n");
sb.append(" renewalSetting: ").append(toIndentedString(renewalSetting)).append("\n");
sb.append(" renewalTerm: ").append(toIndentedString(renewalTerm)).append("\n");
sb.append(" renewalTermPeriodType: ").append(toIndentedString(renewalTermPeriodType)).append("\n");
sb.append(" revision: ").append(toIndentedString(revision)).append("\n");
sb.append(" sequenceSetId: ").append(toIndentedString(sequenceSetId)).append("\n");
sb.append(" sequenceSetName: ").append(toIndentedString(sequenceSetName)).append("\n");
sb.append(" serviceActivationDate: ").append(toIndentedString(serviceActivationDate)).append("\n");
sb.append(" soldToContact: ").append(toIndentedString(soldToContact)).append("\n");
sb.append(" shipToContact: ").append(toIndentedString(shipToContact)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" statusHistory: ").append(toIndentedString(statusHistory)).append("\n");
sb.append(" subscriptionStartDate: ").append(toIndentedString(subscriptionStartDate)).append("\n");
sb.append(" subscriptionEndDate: ").append(toIndentedString(subscriptionEndDate)).append("\n");
sb.append(" termEndDate: ").append(toIndentedString(termEndDate)).append("\n");
sb.append(" termStartDate: ").append(toIndentedString(termStartDate)).append("\n");
sb.append(" termType: ").append(toIndentedString(termType)).append("\n");
sb.append(" scheduledCancelDate: ").append(toIndentedString(scheduledCancelDate)).append("\n");
sb.append(" scheduledSuspendDate: ").append(toIndentedString(scheduledSuspendDate)).append("\n");
sb.append(" scheduledResumeDate: ").append(toIndentedString(scheduledResumeDate)).append("\n");
sb.append(" totalContractedValue: ").append(toIndentedString(totalContractedValue)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" contractedNetMrr: ").append(toIndentedString(contractedNetMrr)).append("\n");
sb.append(" asOfDayGrossMrr: ").append(toIndentedString(asOfDayGrossMrr)).append("\n");
sb.append(" asOfDayNetMrr: ").append(toIndentedString(asOfDayNetMrr)).append("\n");
sb.append(" netTotalContractedValue: ").append(toIndentedString(netTotalContractedValue)).append("\n");
sb.append(" accountOwnerDetails: ").append(toIndentedString(accountOwnerDetails)).append("\n");
sb.append(" invoiceOwnerAccountDetails: ").append(toIndentedString(invoiceOwnerAccountDetails)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("processId");
openapiFields.add("requestId");
openapiFields.add("reasons");
openapiFields.add("success");
openapiFields.add("CpqBundleJsonId__QT");
openapiFields.add("OpportunityCloseDate__QT");
openapiFields.add("OpportunityName__QT");
openapiFields.add("QuoteBusinessType__QT");
openapiFields.add("QuoteNumber__QT");
openapiFields.add("QuoteType__QT");
openapiFields.add("IntegrationId__NS");
openapiFields.add("IntegrationStatus__NS");
openapiFields.add("Project__NS");
openapiFields.add("SalesOrder__NS");
openapiFields.add("SyncDate__NS");
openapiFields.add("id");
openapiFields.add("subscriptionNumber");
openapiFields.add("accountId");
openapiFields.add("accountName");
openapiFields.add("accountNumber");
openapiFields.add("autoRenew");
openapiFields.add("billToContact");
openapiFields.add("cancelReason");
openapiFields.add("contractEffectiveDate");
openapiFields.add("contractedMrr");
openapiFields.add("currentTerm");
openapiFields.add("currentTermPeriodType");
openapiFields.add("customerAcceptanceDate");
openapiFields.add("currency");
openapiFields.add("createTime");
openapiFields.add("updateTime");
openapiFields.add("externallyManagedBy");
openapiFields.add("initialTerm");
openapiFields.add("initialTermPeriodType");
openapiFields.add("invoiceGroupNumber");
openapiFields.add("invoiceOwnerAccountId");
openapiFields.add("invoiceOwnerAccountName");
openapiFields.add("invoiceOwnerAccountNumber");
openapiFields.add("invoiceScheduleId");
openapiFields.add("invoiceSeparately");
openapiFields.add("invoiceTemplateId");
openapiFields.add("invoiceTemplateName");
openapiFields.add("isLatestVersion");
openapiFields.add("lastBookingDate");
openapiFields.add("notes");
openapiFields.add("orderNumber");
openapiFields.add("paymentTerm");
openapiFields.add("ratePlans");
openapiFields.add("renewalSetting");
openapiFields.add("renewalTerm");
openapiFields.add("renewalTermPeriodType");
openapiFields.add("revision");
openapiFields.add("sequenceSetId");
openapiFields.add("sequenceSetName");
openapiFields.add("serviceActivationDate");
openapiFields.add("soldToContact");
openapiFields.add("shipToContact");
openapiFields.add("status");
openapiFields.add("statusHistory");
openapiFields.add("subscriptionStartDate");
openapiFields.add("subscriptionEndDate");
openapiFields.add("termEndDate");
openapiFields.add("termStartDate");
openapiFields.add("termType");
openapiFields.add("scheduledCancelDate");
openapiFields.add("scheduledSuspendDate");
openapiFields.add("scheduledResumeDate");
openapiFields.add("totalContractedValue");
openapiFields.add("version");
openapiFields.add("contractedNetMrr");
openapiFields.add("asOfDayGrossMrr");
openapiFields.add("asOfDayNetMrr");
openapiFields.add("netTotalContractedValue");
openapiFields.add("accountOwnerDetails");
openapiFields.add("invoiceOwnerAccountDetails");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to GetSubscriptionResponse
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!GetSubscriptionResponse.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in GetSubscriptionResponse is not found in the empty JSON string", GetSubscriptionResponse.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("processId") != null && !jsonObj.get("processId").isJsonNull()) && !jsonObj.get("processId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `processId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("processId").toString()));
}
if ((jsonObj.get("requestId") != null && !jsonObj.get("requestId").isJsonNull()) && !jsonObj.get("requestId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `requestId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("requestId").toString()));
}
if (jsonObj.get("reasons") != null && !jsonObj.get("reasons").isJsonNull()) {
JsonArray jsonArrayreasons = jsonObj.getAsJsonArray("reasons");
if (jsonArrayreasons != null) {
// ensure the json data is an array
if (!jsonObj.get("reasons").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `reasons` to be an array in the JSON string but got `%s`", jsonObj.get("reasons").toString()));
}
// validate the optional field `reasons` (array)
for (int i = 0; i < jsonArrayreasons.size(); i++) {
FailedReason.validateJsonElement(jsonArrayreasons.get(i));
};
}
}
if ((jsonObj.get("CpqBundleJsonId__QT") != null && !jsonObj.get("CpqBundleJsonId__QT").isJsonNull()) && !jsonObj.get("CpqBundleJsonId__QT").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `CpqBundleJsonId__QT` to be a primitive type in the JSON string but got `%s`", jsonObj.get("CpqBundleJsonId__QT").toString()));
}
if ((jsonObj.get("OpportunityName__QT") != null && !jsonObj.get("OpportunityName__QT").isJsonNull()) && !jsonObj.get("OpportunityName__QT").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `OpportunityName__QT` to be a primitive type in the JSON string but got `%s`", jsonObj.get("OpportunityName__QT").toString()));
}
if ((jsonObj.get("QuoteBusinessType__QT") != null && !jsonObj.get("QuoteBusinessType__QT").isJsonNull()) && !jsonObj.get("QuoteBusinessType__QT").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `QuoteBusinessType__QT` to be a primitive type in the JSON string but got `%s`", jsonObj.get("QuoteBusinessType__QT").toString()));
}
if ((jsonObj.get("QuoteNumber__QT") != null && !jsonObj.get("QuoteNumber__QT").isJsonNull()) && !jsonObj.get("QuoteNumber__QT").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `QuoteNumber__QT` to be a primitive type in the JSON string but got `%s`", jsonObj.get("QuoteNumber__QT").toString()));
}
if ((jsonObj.get("QuoteType__QT") != null && !jsonObj.get("QuoteType__QT").isJsonNull()) && !jsonObj.get("QuoteType__QT").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `QuoteType__QT` to be a primitive type in the JSON string but got `%s`", jsonObj.get("QuoteType__QT").toString()));
}
if ((jsonObj.get("IntegrationId__NS") != null && !jsonObj.get("IntegrationId__NS").isJsonNull()) && !jsonObj.get("IntegrationId__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationId__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationId__NS").toString()));
}
if ((jsonObj.get("IntegrationStatus__NS") != null && !jsonObj.get("IntegrationStatus__NS").isJsonNull()) && !jsonObj.get("IntegrationStatus__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationStatus__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationStatus__NS").toString()));
}
if ((jsonObj.get("Project__NS") != null && !jsonObj.get("Project__NS").isJsonNull()) && !jsonObj.get("Project__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Project__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Project__NS").toString()));
}
if ((jsonObj.get("SalesOrder__NS") != null && !jsonObj.get("SalesOrder__NS").isJsonNull()) && !jsonObj.get("SalesOrder__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SalesOrder__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SalesOrder__NS").toString()));
}
if ((jsonObj.get("SyncDate__NS") != null && !jsonObj.get("SyncDate__NS").isJsonNull()) && !jsonObj.get("SyncDate__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SyncDate__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SyncDate__NS").toString()));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("subscriptionNumber") != null && !jsonObj.get("subscriptionNumber").isJsonNull()) && !jsonObj.get("subscriptionNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("subscriptionNumber").toString()));
}
if ((jsonObj.get("accountId") != null && !jsonObj.get("accountId").isJsonNull()) && !jsonObj.get("accountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountId").toString()));
}
if ((jsonObj.get("accountName") != null && !jsonObj.get("accountName").isJsonNull()) && !jsonObj.get("accountName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountName").toString()));
}
if ((jsonObj.get("accountNumber") != null && !jsonObj.get("accountNumber").isJsonNull()) && !jsonObj.get("accountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountNumber").toString()));
}
// validate the optional field `billToContact`
if (jsonObj.get("billToContact") != null && !jsonObj.get("billToContact").isJsonNull()) {
Contact.validateJsonElement(jsonObj.get("billToContact"));
}
if ((jsonObj.get("cancelReason") != null && !jsonObj.get("cancelReason").isJsonNull()) && !jsonObj.get("cancelReason").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cancelReason` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cancelReason").toString()));
}
// validate the optional field `currentTermPeriodType`
if (jsonObj.get("currentTermPeriodType") != null && !jsonObj.get("currentTermPeriodType").isJsonNull()) {
TermPeriodType.validateJsonElement(jsonObj.get("currentTermPeriodType"));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("createTime") != null && !jsonObj.get("createTime").isJsonNull()) && !jsonObj.get("createTime").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createTime` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createTime").toString()));
}
if ((jsonObj.get("updateTime") != null && !jsonObj.get("updateTime").isJsonNull()) && !jsonObj.get("updateTime").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updateTime` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updateTime").toString()));
}
// validate the optional field `externallyManagedBy`
if (jsonObj.get("externallyManagedBy") != null && !jsonObj.get("externallyManagedBy").isJsonNull()) {
ExternallyManagedBy.validateJsonElement(jsonObj.get("externallyManagedBy"));
}
// validate the optional field `initialTermPeriodType`
if (jsonObj.get("initialTermPeriodType") != null && !jsonObj.get("initialTermPeriodType").isJsonNull()) {
TermPeriodType.validateJsonElement(jsonObj.get("initialTermPeriodType"));
}
if ((jsonObj.get("invoiceGroupNumber") != null && !jsonObj.get("invoiceGroupNumber").isJsonNull()) && !jsonObj.get("invoiceGroupNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceGroupNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceGroupNumber").toString()));
}
if ((jsonObj.get("invoiceOwnerAccountId") != null && !jsonObj.get("invoiceOwnerAccountId").isJsonNull()) && !jsonObj.get("invoiceOwnerAccountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceOwnerAccountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceOwnerAccountId").toString()));
}
if ((jsonObj.get("invoiceOwnerAccountName") != null && !jsonObj.get("invoiceOwnerAccountName").isJsonNull()) && !jsonObj.get("invoiceOwnerAccountName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceOwnerAccountName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceOwnerAccountName").toString()));
}
if ((jsonObj.get("invoiceOwnerAccountNumber") != null && !jsonObj.get("invoiceOwnerAccountNumber").isJsonNull()) && !jsonObj.get("invoiceOwnerAccountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceOwnerAccountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceOwnerAccountNumber").toString()));
}
if ((jsonObj.get("invoiceScheduleId") != null && !jsonObj.get("invoiceScheduleId").isJsonNull()) && !jsonObj.get("invoiceScheduleId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceScheduleId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceScheduleId").toString()));
}
if ((jsonObj.get("invoiceSeparately") != null && !jsonObj.get("invoiceSeparately").isJsonNull()) && !jsonObj.get("invoiceSeparately").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceSeparately` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceSeparately").toString()));
}
if ((jsonObj.get("invoiceTemplateId") != null && !jsonObj.get("invoiceTemplateId").isJsonNull()) && !jsonObj.get("invoiceTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceTemplateId").toString()));
}
if ((jsonObj.get("invoiceTemplateName") != null && !jsonObj.get("invoiceTemplateName").isJsonNull()) && !jsonObj.get("invoiceTemplateName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceTemplateName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceTemplateName").toString()));
}
if ((jsonObj.get("notes") != null && !jsonObj.get("notes").isJsonNull()) && !jsonObj.get("notes").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `notes` to be a primitive type in the JSON string but got `%s`", jsonObj.get("notes").toString()));
}
if ((jsonObj.get("orderNumber") != null && !jsonObj.get("orderNumber").isJsonNull()) && !jsonObj.get("orderNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `orderNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("orderNumber").toString()));
}
if ((jsonObj.get("paymentTerm") != null && !jsonObj.get("paymentTerm").isJsonNull()) && !jsonObj.get("paymentTerm").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentTerm` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentTerm").toString()));
}
if (jsonObj.get("ratePlans") != null && !jsonObj.get("ratePlans").isJsonNull()) {
JsonArray jsonArrayratePlans = jsonObj.getAsJsonArray("ratePlans");
if (jsonArrayratePlans != null) {
// ensure the json data is an array
if (!jsonObj.get("ratePlans").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `ratePlans` to be an array in the JSON string but got `%s`", jsonObj.get("ratePlans").toString()));
}
// validate the optional field `ratePlans` (array)
for (int i = 0; i < jsonArrayratePlans.size(); i++) {
SubscriptionRatePlan.validateJsonElement(jsonArrayratePlans.get(i));
};
}
}
if ((jsonObj.get("renewalSetting") != null && !jsonObj.get("renewalSetting").isJsonNull()) && !jsonObj.get("renewalSetting").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `renewalSetting` to be a primitive type in the JSON string but got `%s`", jsonObj.get("renewalSetting").toString()));
}
// validate the optional field `renewalTermPeriodType`
if (jsonObj.get("renewalTermPeriodType") != null && !jsonObj.get("renewalTermPeriodType").isJsonNull()) {
TermPeriodType.validateJsonElement(jsonObj.get("renewalTermPeriodType"));
}
if ((jsonObj.get("revision") != null && !jsonObj.get("revision").isJsonNull()) && !jsonObj.get("revision").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revision` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revision").toString()));
}
if ((jsonObj.get("sequenceSetId") != null && !jsonObj.get("sequenceSetId").isJsonNull()) && !jsonObj.get("sequenceSetId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sequenceSetId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sequenceSetId").toString()));
}
if ((jsonObj.get("sequenceSetName") != null && !jsonObj.get("sequenceSetName").isJsonNull()) && !jsonObj.get("sequenceSetName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sequenceSetName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sequenceSetName").toString()));
}
// validate the optional field `soldToContact`
if (jsonObj.get("soldToContact") != null && !jsonObj.get("soldToContact").isJsonNull()) {
Contact.validateJsonElement(jsonObj.get("soldToContact"));
}
// validate the optional field `shipToContact`
if (jsonObj.get("shipToContact") != null && !jsonObj.get("shipToContact").isJsonNull()) {
Contact.validateJsonElement(jsonObj.get("shipToContact"));
}
// validate the optional field `status`
if (jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) {
SubscriptionStatus.validateJsonElement(jsonObj.get("status"));
}
if (jsonObj.get("statusHistory") != null && !jsonObj.get("statusHistory").isJsonNull()) {
JsonArray jsonArraystatusHistory = jsonObj.getAsJsonArray("statusHistory");
if (jsonArraystatusHistory != null) {
// ensure the json data is an array
if (!jsonObj.get("statusHistory").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `statusHistory` to be an array in the JSON string but got `%s`", jsonObj.get("statusHistory").toString()));
}
// validate the optional field `statusHistory` (array)
for (int i = 0; i < jsonArraystatusHistory.size(); i++) {
SubscriptionStatusHistory.validateJsonElement(jsonArraystatusHistory.get(i));
};
}
}
if ((jsonObj.get("termType") != null && !jsonObj.get("termType").isJsonNull()) && !jsonObj.get("termType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `termType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("termType").toString()));
}
// validate the optional field `accountOwnerDetails`
if (jsonObj.get("accountOwnerDetails") != null && !jsonObj.get("accountOwnerDetails").isJsonNull()) {
AccountBasicInfo.validateJsonElement(jsonObj.get("accountOwnerDetails"));
}
// validate the optional field `invoiceOwnerAccountDetails`
if (jsonObj.get("invoiceOwnerAccountDetails") != null && !jsonObj.get("invoiceOwnerAccountDetails").isJsonNull()) {
AccountBasicInfo.validateJsonElement(jsonObj.get("invoiceOwnerAccountDetails"));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!GetSubscriptionResponse.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'GetSubscriptionResponse' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(GetSubscriptionResponse.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, GetSubscriptionResponse value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public GetSubscriptionResponse read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
GetSubscriptionResponse instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of GetSubscriptionResponse given an JSON string
*
* @param jsonString JSON string
* @return An instance of GetSubscriptionResponse
* @throws IOException if the JSON string is invalid with respect to GetSubscriptionResponse
*/
public static GetSubscriptionResponse fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, GetSubscriptionResponse.class);
}
/**
* Convert an instance of GetSubscriptionResponse to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy