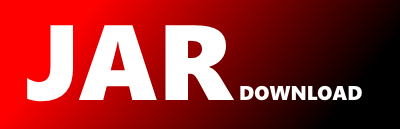
com.zuora.model.InvoiceItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.BillingDocumentItemProcessingType;
import com.zuora.model.BillingDocumentItemSourceType;
import com.zuora.model.ChargeType;
import com.zuora.model.RevRecTrigger;
import com.zuora.model.TaxMode;
import com.zuora.model.TaxationItemsData;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* InvoiceItem
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class InvoiceItem {
public static final String SERIALIZED_NAME_INTEGRATION_ID_N_S = "IntegrationId__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_ID_N_S)
private String integrationIdNS;
public static final String SERIALIZED_NAME_INTEGRATION_STATUS_N_S = "IntegrationStatus__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_STATUS_N_S)
private String integrationStatusNS;
public static final String SERIALIZED_NAME_SYNC_DATE_N_S = "SyncDate__NS";
@SerializedName(SERIALIZED_NAME_SYNC_DATE_N_S)
private String syncDateNS;
public static final String SERIALIZED_NAME_ACCOUNTING_CODE = "accountingCode";
@SerializedName(SERIALIZED_NAME_ACCOUNTING_CODE)
private String accountingCode;
public static final String SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNTING_CODE = "adjustmentLiabilityAccountingCode";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNTING_CODE)
private String adjustmentLiabilityAccountingCode;
public static final String SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNTING_CODE = "adjustmentRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNTING_CODE)
private String adjustmentRevenueAccountingCode;
public static final String SERIALIZED_NAME_APPLIED_TO_ITEM_ID = "appliedToItemId";
@SerializedName(SERIALIZED_NAME_APPLIED_TO_ITEM_ID)
private String appliedToItemId;
public static final String SERIALIZED_NAME_AVAILABLE_TO_CREDIT_AMOUNT = "availableToCreditAmount";
@SerializedName(SERIALIZED_NAME_AVAILABLE_TO_CREDIT_AMOUNT)
private BigDecimal availableToCreditAmount;
public static final String SERIALIZED_NAME_BALANCE = "balance";
@SerializedName(SERIALIZED_NAME_BALANCE)
private BigDecimal balance;
public static final String SERIALIZED_NAME_BOOKING_REFERENCE = "bookingReference";
@SerializedName(SERIALIZED_NAME_BOOKING_REFERENCE)
private String bookingReference;
public static final String SERIALIZED_NAME_CHARGE_AMOUNT = "chargeAmount";
@SerializedName(SERIALIZED_NAME_CHARGE_AMOUNT)
private BigDecimal chargeAmount;
public static final String SERIALIZED_NAME_CHARGE_DATE = "chargeDate";
@SerializedName(SERIALIZED_NAME_CHARGE_DATE)
private String chargeDate;
public static final String SERIALIZED_NAME_CHARGE_DESCRIPTION = "chargeDescription";
@SerializedName(SERIALIZED_NAME_CHARGE_DESCRIPTION)
private String chargeDescription;
public static final String SERIALIZED_NAME_CHARGE_ID = "chargeId";
@SerializedName(SERIALIZED_NAME_CHARGE_ID)
private String chargeId;
public static final String SERIALIZED_NAME_CHARGE_NAME = "chargeName";
@SerializedName(SERIALIZED_NAME_CHARGE_NAME)
private String chargeName;
public static final String SERIALIZED_NAME_CHARGE_TYPE = "chargeType";
@SerializedName(SERIALIZED_NAME_CHARGE_TYPE)
private ChargeType chargeType;
public static final String SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNTING_CODE = "contractAssetAccountingCode";
@SerializedName(SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNTING_CODE)
private String contractAssetAccountingCode;
public static final String SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNTING_CODE = "contractLiabilityAccountingCode";
@SerializedName(SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNTING_CODE)
private String contractLiabilityAccountingCode;
public static final String SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNTING_CODE = "contractRecognizedRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNTING_CODE)
private String contractRecognizedRevenueAccountingCode;
public static final String SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE = "deferredRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE)
private String deferredRevenueAccountingCode;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING = "excludeItemBillingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBillingFromRevenueAccounting;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_INVOICE_SCHEDULE_ID = "invoiceScheduleId";
@SerializedName(SERIALIZED_NAME_INVOICE_SCHEDULE_ID)
private String invoiceScheduleId;
public static final String SERIALIZED_NAME_INVOICE_SCHEDULE_ITEM_ID = "invoiceScheduleItemId";
@SerializedName(SERIALIZED_NAME_INVOICE_SCHEDULE_ITEM_ID)
private String invoiceScheduleItemId;
public static final String SERIALIZED_NAME_ITEM_TYPE = "itemType";
@SerializedName(SERIALIZED_NAME_ITEM_TYPE)
private String itemType;
public static final String SERIALIZED_NAME_PROCESSING_TYPE = "processingType";
@SerializedName(SERIALIZED_NAME_PROCESSING_TYPE)
private BillingDocumentItemProcessingType processingType;
public static final String SERIALIZED_NAME_PRODUCT_NAME = "productName";
@SerializedName(SERIALIZED_NAME_PRODUCT_NAME)
private String productName;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_ID = "productRatePlanChargeId";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_ID)
private String productRatePlanChargeId;
public static final String SERIALIZED_NAME_PURCHASE_ORDER_NUMBER = "purchaseOrderNumber";
@SerializedName(SERIALIZED_NAME_PURCHASE_ORDER_NUMBER)
private String purchaseOrderNumber;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE = "recognizedRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE)
private String recognizedRevenueAccountingCode;
public static final String SERIALIZED_NAME_REV_REC_CODE = "revRecCode";
@SerializedName(SERIALIZED_NAME_REV_REC_CODE)
private String revRecCode;
public static final String SERIALIZED_NAME_REV_REC_TRIGGER_CONDITION = "revRecTriggerCondition";
@SerializedName(SERIALIZED_NAME_REV_REC_TRIGGER_CONDITION)
private RevRecTrigger revRecTriggerCondition;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME = "revenueRecognitionRuleName";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME)
private String revenueRecognitionRuleName;
public static final String SERIALIZED_NAME_SERVICE_END_DATE = "serviceEndDate";
@SerializedName(SERIALIZED_NAME_SERVICE_END_DATE)
private LocalDate serviceEndDate;
public static final String SERIALIZED_NAME_SERVICE_START_DATE = "serviceStartDate";
@SerializedName(SERIALIZED_NAME_SERVICE_START_DATE)
private LocalDate serviceStartDate;
public static final String SERIALIZED_NAME_SHIP_TO_CONTACT_ID = "shipToContactId";
@SerializedName(SERIALIZED_NAME_SHIP_TO_CONTACT_ID)
private String shipToContactId;
public static final String SERIALIZED_NAME_SKU = "sku";
@SerializedName(SERIALIZED_NAME_SKU)
private String sku;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT_ID = "soldToContactId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT_ID)
private String soldToContactId;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT_SNAPSHOT_ID = "soldToContactSnapshotId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT_SNAPSHOT_ID)
private String soldToContactSnapshotId;
public static final String SERIALIZED_NAME_SOURCE_ITEM_TYPE = "sourceItemType";
@SerializedName(SERIALIZED_NAME_SOURCE_ITEM_TYPE)
private BillingDocumentItemSourceType sourceItemType;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ID = "subscriptionId";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ID)
private String subscriptionId;
public static final String SERIALIZED_NAME_SUBSCRIPTION_NAME = "subscriptionName";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_NAME)
private String subscriptionName;
public static final String SERIALIZED_NAME_TAX_AMOUNT = "taxAmount";
@SerializedName(SERIALIZED_NAME_TAX_AMOUNT)
private BigDecimal taxAmount;
public static final String SERIALIZED_NAME_TAX_CODE = "taxCode";
@SerializedName(SERIALIZED_NAME_TAX_CODE)
private String taxCode;
public static final String SERIALIZED_NAME_TAX_MODE = "taxMode";
@SerializedName(SERIALIZED_NAME_TAX_MODE)
private TaxMode taxMode;
public static final String SERIALIZED_NAME_TAXATION_ITEMS = "taxationItems";
@SerializedName(SERIALIZED_NAME_TAXATION_ITEMS)
private TaxationItemsData taxationItems;
public static final String SERIALIZED_NAME_UNBILLED_RECEIVABLES_ACCOUNTING_CODE = "unbilledReceivablesAccountingCode";
@SerializedName(SERIALIZED_NAME_UNBILLED_RECEIVABLES_ACCOUNTING_CODE)
private String unbilledReceivablesAccountingCode;
public static final String SERIALIZED_NAME_UNIT_OF_MEASURE = "unitOfMeasure";
@SerializedName(SERIALIZED_NAME_UNIT_OF_MEASURE)
private String unitOfMeasure;
public static final String SERIALIZED_NAME_UNIT_PRICE = "unitPrice";
@SerializedName(SERIALIZED_NAME_UNIT_PRICE)
private BigDecimal unitPrice;
public static final String SERIALIZED_NAME_NUMBER_OF_DELIVERIES = "numberOfDeliveries";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_DELIVERIES)
private BigDecimal numberOfDeliveries;
public static final String SERIALIZED_NAME_REFLECT_DISCOUNT_IN_NET_AMOUNT = "reflectDiscountInNetAmount";
@SerializedName(SERIALIZED_NAME_REFLECT_DISCOUNT_IN_NET_AMOUNT)
private Boolean reflectDiscountInNetAmount;
public InvoiceItem() {
}
public InvoiceItem integrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
return this;
}
/**
* ID of the corresponding object in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationIdNS
*/
@javax.annotation.Nullable
public String getIntegrationIdNS() {
return integrationIdNS;
}
public void setIntegrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
}
public InvoiceItem integrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
return this;
}
/**
* Status of the invoice item's synchronization with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationStatusNS
*/
@javax.annotation.Nullable
public String getIntegrationStatusNS() {
return integrationStatusNS;
}
public void setIntegrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
}
public InvoiceItem syncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
return this;
}
/**
* Date when the invoice item was synchronized with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return syncDateNS
*/
@javax.annotation.Nullable
public String getSyncDateNS() {
return syncDateNS;
}
public void setSyncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
}
public InvoiceItem accountingCode(String accountingCode) {
this.accountingCode = accountingCode;
return this;
}
/**
* The accounting code associated with the invoice item.
* @return accountingCode
*/
@javax.annotation.Nullable
public String getAccountingCode() {
return accountingCode;
}
public void setAccountingCode(String accountingCode) {
this.accountingCode = accountingCode;
}
public InvoiceItem adjustmentLiabilityAccountingCode(String adjustmentLiabilityAccountingCode) {
this.adjustmentLiabilityAccountingCode = adjustmentLiabilityAccountingCode;
return this;
}
/**
* The accounting code for adjustment liability. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return adjustmentLiabilityAccountingCode
*/
@javax.annotation.Nullable
public String getAdjustmentLiabilityAccountingCode() {
return adjustmentLiabilityAccountingCode;
}
public void setAdjustmentLiabilityAccountingCode(String adjustmentLiabilityAccountingCode) {
this.adjustmentLiabilityAccountingCode = adjustmentLiabilityAccountingCode;
}
public InvoiceItem adjustmentRevenueAccountingCode(String adjustmentRevenueAccountingCode) {
this.adjustmentRevenueAccountingCode = adjustmentRevenueAccountingCode;
return this;
}
/**
* The accounting code for adjustment revenue. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return adjustmentRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getAdjustmentRevenueAccountingCode() {
return adjustmentRevenueAccountingCode;
}
public void setAdjustmentRevenueAccountingCode(String adjustmentRevenueAccountingCode) {
this.adjustmentRevenueAccountingCode = adjustmentRevenueAccountingCode;
}
public InvoiceItem appliedToItemId(String appliedToItemId) {
this.appliedToItemId = appliedToItemId;
return this;
}
/**
* The unique ID of the invoice item that the discount charge is applied to.
* @return appliedToItemId
*/
@javax.annotation.Nullable
public String getAppliedToItemId() {
return appliedToItemId;
}
public void setAppliedToItemId(String appliedToItemId) {
this.appliedToItemId = appliedToItemId;
}
public InvoiceItem availableToCreditAmount(BigDecimal availableToCreditAmount) {
this.availableToCreditAmount = availableToCreditAmount;
return this;
}
/**
* The amount of the invoice item that is available to credit.
* @return availableToCreditAmount
*/
@javax.annotation.Nullable
public BigDecimal getAvailableToCreditAmount() {
return availableToCreditAmount;
}
public void setAvailableToCreditAmount(BigDecimal availableToCreditAmount) {
this.availableToCreditAmount = availableToCreditAmount;
}
public InvoiceItem balance(BigDecimal balance) {
this.balance = balance;
return this;
}
/**
* The balance of the invoice item.
* @return balance
*/
@javax.annotation.Nullable
public BigDecimal getBalance() {
return balance;
}
public void setBalance(BigDecimal balance) {
this.balance = balance;
}
public InvoiceItem bookingReference(String bookingReference) {
this.bookingReference = bookingReference;
return this;
}
/**
* The booking reference of the invoice item.
* @return bookingReference
*/
@javax.annotation.Nullable
public String getBookingReference() {
return bookingReference;
}
public void setBookingReference(String bookingReference) {
this.bookingReference = bookingReference;
}
public InvoiceItem chargeAmount(BigDecimal chargeAmount) {
this.chargeAmount = chargeAmount;
return this;
}
/**
* The amount of the charge. This amount does not include taxes regardless if the charge's tax mode is inclusive or exclusive.
* @return chargeAmount
*/
@javax.annotation.Nullable
public BigDecimal getChargeAmount() {
return chargeAmount;
}
public void setChargeAmount(BigDecimal chargeAmount) {
this.chargeAmount = chargeAmount;
}
public InvoiceItem chargeDate(String chargeDate) {
this.chargeDate = chargeDate;
return this;
}
/**
* The date when the invoice item is charged, in `yyyy-mm-dd hh:mm:ss` format.
* @return chargeDate
*/
@javax.annotation.Nullable
public String getChargeDate() {
return chargeDate;
}
public void setChargeDate(String chargeDate) {
this.chargeDate = chargeDate;
}
public InvoiceItem chargeDescription(String chargeDescription) {
this.chargeDescription = chargeDescription;
return this;
}
/**
* The description of the charge.
* @return chargeDescription
*/
@javax.annotation.Nullable
public String getChargeDescription() {
return chargeDescription;
}
public void setChargeDescription(String chargeDescription) {
this.chargeDescription = chargeDescription;
}
public InvoiceItem chargeId(String chargeId) {
this.chargeId = chargeId;
return this;
}
/**
* The unique ID of the charge.
* @return chargeId
*/
@javax.annotation.Nullable
public String getChargeId() {
return chargeId;
}
public void setChargeId(String chargeId) {
this.chargeId = chargeId;
}
public InvoiceItem chargeName(String chargeName) {
this.chargeName = chargeName;
return this;
}
/**
* The name of the charge.
* @return chargeName
*/
@javax.annotation.Nullable
public String getChargeName() {
return chargeName;
}
public void setChargeName(String chargeName) {
this.chargeName = chargeName;
}
public InvoiceItem chargeType(ChargeType chargeType) {
this.chargeType = chargeType;
return this;
}
/**
* Get chargeType
* @return chargeType
*/
@javax.annotation.Nullable
public ChargeType getChargeType() {
return chargeType;
}
public void setChargeType(ChargeType chargeType) {
this.chargeType = chargeType;
}
public InvoiceItem contractAssetAccountingCode(String contractAssetAccountingCode) {
this.contractAssetAccountingCode = contractAssetAccountingCode;
return this;
}
/**
* The accounting code for contract asset. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return contractAssetAccountingCode
*/
@javax.annotation.Nullable
public String getContractAssetAccountingCode() {
return contractAssetAccountingCode;
}
public void setContractAssetAccountingCode(String contractAssetAccountingCode) {
this.contractAssetAccountingCode = contractAssetAccountingCode;
}
public InvoiceItem contractLiabilityAccountingCode(String contractLiabilityAccountingCode) {
this.contractLiabilityAccountingCode = contractLiabilityAccountingCode;
return this;
}
/**
* The accounting code for contract liability. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return contractLiabilityAccountingCode
*/
@javax.annotation.Nullable
public String getContractLiabilityAccountingCode() {
return contractLiabilityAccountingCode;
}
public void setContractLiabilityAccountingCode(String contractLiabilityAccountingCode) {
this.contractLiabilityAccountingCode = contractLiabilityAccountingCode;
}
public InvoiceItem contractRecognizedRevenueAccountingCode(String contractRecognizedRevenueAccountingCode) {
this.contractRecognizedRevenueAccountingCode = contractRecognizedRevenueAccountingCode;
return this;
}
/**
* The accounting code for contract recognized revenue. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return contractRecognizedRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getContractRecognizedRevenueAccountingCode() {
return contractRecognizedRevenueAccountingCode;
}
public void setContractRecognizedRevenueAccountingCode(String contractRecognizedRevenueAccountingCode) {
this.contractRecognizedRevenueAccountingCode = contractRecognizedRevenueAccountingCode;
}
public InvoiceItem deferredRevenueAccountingCode(String deferredRevenueAccountingCode) {
this.deferredRevenueAccountingCode = deferredRevenueAccountingCode;
return this;
}
/**
* The deferred revenue accounting code associated with the invoice item. **Note:** This field is only available if you have Zuora Finance enabled.
* @return deferredRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getDeferredRevenueAccountingCode() {
return deferredRevenueAccountingCode;
}
public void setDeferredRevenueAccountingCode(String deferredRevenueAccountingCode) {
this.deferredRevenueAccountingCode = deferredRevenueAccountingCode;
}
public InvoiceItem description(String description) {
this.description = description;
return this;
}
/**
* The description of the invoice item.
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public InvoiceItem excludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
return this;
}
/**
* The flag to exclude the invoice item from revenue accounting. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return excludeItemBillingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBillingFromRevenueAccounting() {
return excludeItemBillingFromRevenueAccounting;
}
public void setExcludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
}
public InvoiceItem id(String id) {
this.id = id;
return this;
}
/**
* Item ID.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public InvoiceItem invoiceScheduleId(String invoiceScheduleId) {
this.invoiceScheduleId = invoiceScheduleId;
return this;
}
/**
* The ID of the invoice schedule item by which Invoice Schedule Item the invoice item is generated by when the Invoice Schedule Item is executed. **Note**: This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Billing_Schedule\" target=\"_blank\">Billing Schedule</a> feature in the **Early Adopter** phase enabled.
* @return invoiceScheduleId
*/
@javax.annotation.Nullable
public String getInvoiceScheduleId() {
return invoiceScheduleId;
}
public void setInvoiceScheduleId(String invoiceScheduleId) {
this.invoiceScheduleId = invoiceScheduleId;
}
public InvoiceItem invoiceScheduleItemId(String invoiceScheduleItemId) {
this.invoiceScheduleItemId = invoiceScheduleItemId;
return this;
}
/**
* The ID of the invoice schedule item associated with the invoice item. **Note**: This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Billing_Schedule\" target=\"_blank\">Billing Schedule</a> feature in the **Early Adopter** phase enabled.
* @return invoiceScheduleItemId
*/
@javax.annotation.Nullable
public String getInvoiceScheduleItemId() {
return invoiceScheduleItemId;
}
public void setInvoiceScheduleItemId(String invoiceScheduleItemId) {
this.invoiceScheduleItemId = invoiceScheduleItemId;
}
public InvoiceItem itemType(String itemType) {
this.itemType = itemType;
return this;
}
/**
* The type of the invoice item.
* @return itemType
*/
@javax.annotation.Nullable
public String getItemType() {
return itemType;
}
public void setItemType(String itemType) {
this.itemType = itemType;
}
public InvoiceItem processingType(BillingDocumentItemProcessingType processingType) {
this.processingType = processingType;
return this;
}
/**
* Get processingType
* @return processingType
*/
@javax.annotation.Nullable
public BillingDocumentItemProcessingType getProcessingType() {
return processingType;
}
public void setProcessingType(BillingDocumentItemProcessingType processingType) {
this.processingType = processingType;
}
public InvoiceItem productName(String productName) {
this.productName = productName;
return this;
}
/**
* Name of the product associated with this item.
* @return productName
*/
@javax.annotation.Nullable
public String getProductName() {
return productName;
}
public void setProductName(String productName) {
this.productName = productName;
}
public InvoiceItem productRatePlanChargeId(String productRatePlanChargeId) {
this.productRatePlanChargeId = productRatePlanChargeId;
return this;
}
/**
* The ID of the product rate plan charge that the invoice item is created from.
* @return productRatePlanChargeId
*/
@javax.annotation.Nullable
public String getProductRatePlanChargeId() {
return productRatePlanChargeId;
}
public void setProductRatePlanChargeId(String productRatePlanChargeId) {
this.productRatePlanChargeId = productRatePlanChargeId;
}
public InvoiceItem purchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
return this;
}
/**
* The purchase order number associated with the invoice item.
* @return purchaseOrderNumber
*/
@javax.annotation.Nullable
public String getPurchaseOrderNumber() {
return purchaseOrderNumber;
}
public void setPurchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
}
public InvoiceItem quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* The quantity of this item, in the configured unit of measure for the charge.
* @return quantity
*/
@javax.annotation.Nullable
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public InvoiceItem recognizedRevenueAccountingCode(String recognizedRevenueAccountingCode) {
this.recognizedRevenueAccountingCode = recognizedRevenueAccountingCode;
return this;
}
/**
* The recognized revenue accounting code associated with the invoice item. **Note:** This field is only available if you have Zuora Finance enabled.
* @return recognizedRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getRecognizedRevenueAccountingCode() {
return recognizedRevenueAccountingCode;
}
public void setRecognizedRevenueAccountingCode(String recognizedRevenueAccountingCode) {
this.recognizedRevenueAccountingCode = recognizedRevenueAccountingCode;
}
public InvoiceItem revRecCode(String revRecCode) {
this.revRecCode = revRecCode;
return this;
}
/**
* The revenue recognition code.
* @return revRecCode
*/
@javax.annotation.Nullable
public String getRevRecCode() {
return revRecCode;
}
public void setRevRecCode(String revRecCode) {
this.revRecCode = revRecCode;
}
public InvoiceItem revRecTriggerCondition(RevRecTrigger revRecTriggerCondition) {
this.revRecTriggerCondition = revRecTriggerCondition;
return this;
}
/**
* Get revRecTriggerCondition
* @return revRecTriggerCondition
*/
@javax.annotation.Nullable
public RevRecTrigger getRevRecTriggerCondition() {
return revRecTriggerCondition;
}
public void setRevRecTriggerCondition(RevRecTrigger revRecTriggerCondition) {
this.revRecTriggerCondition = revRecTriggerCondition;
}
public InvoiceItem revenueRecognitionRuleName(String revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
return this;
}
/**
* The revenue recognition rule of the invoice item. **Note:** This field is only available if you have Zuora Finance enabled.
* @return revenueRecognitionRuleName
*/
@javax.annotation.Nullable
public String getRevenueRecognitionRuleName() {
return revenueRecognitionRuleName;
}
public void setRevenueRecognitionRuleName(String revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
}
public InvoiceItem serviceEndDate(LocalDate serviceEndDate) {
this.serviceEndDate = serviceEndDate;
return this;
}
/**
* The end date of the service period for this item, i.e., the last day of the service period, as _yyyy-mm-dd_.
* @return serviceEndDate
*/
@javax.annotation.Nullable
public LocalDate getServiceEndDate() {
return serviceEndDate;
}
public void setServiceEndDate(LocalDate serviceEndDate) {
this.serviceEndDate = serviceEndDate;
}
public InvoiceItem serviceStartDate(LocalDate serviceStartDate) {
this.serviceStartDate = serviceStartDate;
return this;
}
/**
* The start date of the service period for this item, as _yyyy-mm-dd_. For a one-time fee item, the date of the charge.
* @return serviceStartDate
*/
@javax.annotation.Nullable
public LocalDate getServiceStartDate() {
return serviceStartDate;
}
public void setServiceStartDate(LocalDate serviceStartDate) {
this.serviceStartDate = serviceStartDate;
}
public InvoiceItem shipToContactId(String shipToContactId) {
this.shipToContactId = shipToContactId;
return this;
}
/**
* The ID of the ship-to contact associated with the invoice item.
* @return shipToContactId
*/
@javax.annotation.Nullable
public String getShipToContactId() {
return shipToContactId;
}
public void setShipToContactId(String shipToContactId) {
this.shipToContactId = shipToContactId;
}
public InvoiceItem sku(String sku) {
this.sku = sku;
return this;
}
/**
* The SKU of the invoice item.
* @return sku
*/
@javax.annotation.Nullable
public String getSku() {
return sku;
}
public void setSku(String sku) {
this.sku = sku;
}
public InvoiceItem soldToContactId(String soldToContactId) {
this.soldToContactId = soldToContactId;
return this;
}
/**
* The ID of the sold-to contact associated with the invoice item. **Note**: If you have the Flexible Billing Attributes feature disabled, the value of this field is `null`.
* @return soldToContactId
*/
@javax.annotation.Nullable
public String getSoldToContactId() {
return soldToContactId;
}
public void setSoldToContactId(String soldToContactId) {
this.soldToContactId = soldToContactId;
}
public InvoiceItem soldToContactSnapshotId(String soldToContactSnapshotId) {
this.soldToContactSnapshotId = soldToContactSnapshotId;
return this;
}
/**
* The ID of the sold-to contact snapshot associated with the invoice item. **Note**: If you have the Flexible Billing Attributes feature disabled, the value of this field is `null`.
* @return soldToContactSnapshotId
*/
@javax.annotation.Nullable
public String getSoldToContactSnapshotId() {
return soldToContactSnapshotId;
}
public void setSoldToContactSnapshotId(String soldToContactSnapshotId) {
this.soldToContactSnapshotId = soldToContactSnapshotId;
}
public InvoiceItem sourceItemType(BillingDocumentItemSourceType sourceItemType) {
this.sourceItemType = sourceItemType;
return this;
}
/**
* Get sourceItemType
* @return sourceItemType
*/
@javax.annotation.Nullable
public BillingDocumentItemSourceType getSourceItemType() {
return sourceItemType;
}
public void setSourceItemType(BillingDocumentItemSourceType sourceItemType) {
this.sourceItemType = sourceItemType;
}
public InvoiceItem subscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/**
* The ID of the subscription for this item.
* @return subscriptionId
*/
@javax.annotation.Nullable
public String getSubscriptionId() {
return subscriptionId;
}
public void setSubscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
}
public InvoiceItem subscriptionName(String subscriptionName) {
this.subscriptionName = subscriptionName;
return this;
}
/**
* The name of the subscription for this item.
* @return subscriptionName
*/
@javax.annotation.Nullable
public String getSubscriptionName() {
return subscriptionName;
}
public void setSubscriptionName(String subscriptionName) {
this.subscriptionName = subscriptionName;
}
public InvoiceItem taxAmount(BigDecimal taxAmount) {
this.taxAmount = taxAmount;
return this;
}
/**
* Tax applied to the charge.
* @return taxAmount
*/
@javax.annotation.Nullable
public BigDecimal getTaxAmount() {
return taxAmount;
}
public void setTaxAmount(BigDecimal taxAmount) {
this.taxAmount = taxAmount;
}
public InvoiceItem taxCode(String taxCode) {
this.taxCode = taxCode;
return this;
}
/**
* The tax code of the invoice item. **Note** Only when taxation feature is enabled, this field can be presented.
* @return taxCode
*/
@javax.annotation.Nullable
public String getTaxCode() {
return taxCode;
}
public void setTaxCode(String taxCode) {
this.taxCode = taxCode;
}
public InvoiceItem taxMode(TaxMode taxMode) {
this.taxMode = taxMode;
return this;
}
/**
* Get taxMode
* @return taxMode
*/
@javax.annotation.Nullable
public TaxMode getTaxMode() {
return taxMode;
}
public void setTaxMode(TaxMode taxMode) {
this.taxMode = taxMode;
}
public InvoiceItem taxationItems(TaxationItemsData taxationItems) {
this.taxationItems = taxationItems;
return this;
}
/**
* Get taxationItems
* @return taxationItems
*/
@javax.annotation.Nullable
public TaxationItemsData getTaxationItems() {
return taxationItems;
}
public void setTaxationItems(TaxationItemsData taxationItems) {
this.taxationItems = taxationItems;
}
public InvoiceItem unbilledReceivablesAccountingCode(String unbilledReceivablesAccountingCode) {
this.unbilledReceivablesAccountingCode = unbilledReceivablesAccountingCode;
return this;
}
/**
* The accounting code for unbilled receivables. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return unbilledReceivablesAccountingCode
*/
@javax.annotation.Nullable
public String getUnbilledReceivablesAccountingCode() {
return unbilledReceivablesAccountingCode;
}
public void setUnbilledReceivablesAccountingCode(String unbilledReceivablesAccountingCode) {
this.unbilledReceivablesAccountingCode = unbilledReceivablesAccountingCode;
}
public InvoiceItem unitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
return this;
}
/**
* Unit used to measure consumption.
* @return unitOfMeasure
*/
@javax.annotation.Nullable
public String getUnitOfMeasure() {
return unitOfMeasure;
}
public void setUnitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
}
public InvoiceItem unitPrice(BigDecimal unitPrice) {
this.unitPrice = unitPrice;
return this;
}
/**
* The per-unit price of the invoice item.
* @return unitPrice
*/
@javax.annotation.Nullable
public BigDecimal getUnitPrice() {
return unitPrice;
}
public void setUnitPrice(BigDecimal unitPrice) {
this.unitPrice = unitPrice;
}
public InvoiceItem numberOfDeliveries(BigDecimal numberOfDeliveries) {
this.numberOfDeliveries = numberOfDeliveries;
return this;
}
/**
* The number of delivery for charge. **Note**: This field is available only if you have the Delivery Pricing feature enabled.
* @return numberOfDeliveries
*/
@javax.annotation.Nullable
public BigDecimal getNumberOfDeliveries() {
return numberOfDeliveries;
}
public void setNumberOfDeliveries(BigDecimal numberOfDeliveries) {
this.numberOfDeliveries = numberOfDeliveries;
}
public InvoiceItem reflectDiscountInNetAmount(Boolean reflectDiscountInNetAmount) {
this.reflectDiscountInNetAmount = reflectDiscountInNetAmount;
return this;
}
/**
* The flag to reflect Discount in Apply To Charge Net Amount.
* @return reflectDiscountInNetAmount
*/
@javax.annotation.Nullable
public Boolean getReflectDiscountInNetAmount() {
return reflectDiscountInNetAmount;
}
public void setReflectDiscountInNetAmount(Boolean reflectDiscountInNetAmount) {
this.reflectDiscountInNetAmount = reflectDiscountInNetAmount;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the InvoiceItem instance itself
*/
public InvoiceItem putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
InvoiceItem invoiceItem = (InvoiceItem) o;
return Objects.equals(this.integrationIdNS, invoiceItem.integrationIdNS) &&
Objects.equals(this.integrationStatusNS, invoiceItem.integrationStatusNS) &&
Objects.equals(this.syncDateNS, invoiceItem.syncDateNS) &&
Objects.equals(this.accountingCode, invoiceItem.accountingCode) &&
Objects.equals(this.adjustmentLiabilityAccountingCode, invoiceItem.adjustmentLiabilityAccountingCode) &&
Objects.equals(this.adjustmentRevenueAccountingCode, invoiceItem.adjustmentRevenueAccountingCode) &&
Objects.equals(this.appliedToItemId, invoiceItem.appliedToItemId) &&
Objects.equals(this.availableToCreditAmount, invoiceItem.availableToCreditAmount) &&
Objects.equals(this.balance, invoiceItem.balance) &&
Objects.equals(this.bookingReference, invoiceItem.bookingReference) &&
Objects.equals(this.chargeAmount, invoiceItem.chargeAmount) &&
Objects.equals(this.chargeDate, invoiceItem.chargeDate) &&
Objects.equals(this.chargeDescription, invoiceItem.chargeDescription) &&
Objects.equals(this.chargeId, invoiceItem.chargeId) &&
Objects.equals(this.chargeName, invoiceItem.chargeName) &&
Objects.equals(this.chargeType, invoiceItem.chargeType) &&
Objects.equals(this.contractAssetAccountingCode, invoiceItem.contractAssetAccountingCode) &&
Objects.equals(this.contractLiabilityAccountingCode, invoiceItem.contractLiabilityAccountingCode) &&
Objects.equals(this.contractRecognizedRevenueAccountingCode, invoiceItem.contractRecognizedRevenueAccountingCode) &&
Objects.equals(this.deferredRevenueAccountingCode, invoiceItem.deferredRevenueAccountingCode) &&
Objects.equals(this.description, invoiceItem.description) &&
Objects.equals(this.excludeItemBillingFromRevenueAccounting, invoiceItem.excludeItemBillingFromRevenueAccounting) &&
Objects.equals(this.id, invoiceItem.id) &&
Objects.equals(this.invoiceScheduleId, invoiceItem.invoiceScheduleId) &&
Objects.equals(this.invoiceScheduleItemId, invoiceItem.invoiceScheduleItemId) &&
Objects.equals(this.itemType, invoiceItem.itemType) &&
Objects.equals(this.processingType, invoiceItem.processingType) &&
Objects.equals(this.productName, invoiceItem.productName) &&
Objects.equals(this.productRatePlanChargeId, invoiceItem.productRatePlanChargeId) &&
Objects.equals(this.purchaseOrderNumber, invoiceItem.purchaseOrderNumber) &&
Objects.equals(this.quantity, invoiceItem.quantity) &&
Objects.equals(this.recognizedRevenueAccountingCode, invoiceItem.recognizedRevenueAccountingCode) &&
Objects.equals(this.revRecCode, invoiceItem.revRecCode) &&
Objects.equals(this.revRecTriggerCondition, invoiceItem.revRecTriggerCondition) &&
Objects.equals(this.revenueRecognitionRuleName, invoiceItem.revenueRecognitionRuleName) &&
Objects.equals(this.serviceEndDate, invoiceItem.serviceEndDate) &&
Objects.equals(this.serviceStartDate, invoiceItem.serviceStartDate) &&
Objects.equals(this.shipToContactId, invoiceItem.shipToContactId) &&
Objects.equals(this.sku, invoiceItem.sku) &&
Objects.equals(this.soldToContactId, invoiceItem.soldToContactId) &&
Objects.equals(this.soldToContactSnapshotId, invoiceItem.soldToContactSnapshotId) &&
Objects.equals(this.sourceItemType, invoiceItem.sourceItemType) &&
Objects.equals(this.subscriptionId, invoiceItem.subscriptionId) &&
Objects.equals(this.subscriptionName, invoiceItem.subscriptionName) &&
Objects.equals(this.taxAmount, invoiceItem.taxAmount) &&
Objects.equals(this.taxCode, invoiceItem.taxCode) &&
Objects.equals(this.taxMode, invoiceItem.taxMode) &&
Objects.equals(this.taxationItems, invoiceItem.taxationItems) &&
Objects.equals(this.unbilledReceivablesAccountingCode, invoiceItem.unbilledReceivablesAccountingCode) &&
Objects.equals(this.unitOfMeasure, invoiceItem.unitOfMeasure) &&
Objects.equals(this.unitPrice, invoiceItem.unitPrice) &&
Objects.equals(this.numberOfDeliveries, invoiceItem.numberOfDeliveries) &&
Objects.equals(this.reflectDiscountInNetAmount, invoiceItem.reflectDiscountInNetAmount)&&
Objects.equals(this.additionalProperties, invoiceItem.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(integrationIdNS, integrationStatusNS, syncDateNS, accountingCode, adjustmentLiabilityAccountingCode, adjustmentRevenueAccountingCode, appliedToItemId, availableToCreditAmount, balance, bookingReference, chargeAmount, chargeDate, chargeDescription, chargeId, chargeName, chargeType, contractAssetAccountingCode, contractLiabilityAccountingCode, contractRecognizedRevenueAccountingCode, deferredRevenueAccountingCode, description, excludeItemBillingFromRevenueAccounting, id, invoiceScheduleId, invoiceScheduleItemId, itemType, processingType, productName, productRatePlanChargeId, purchaseOrderNumber, quantity, recognizedRevenueAccountingCode, revRecCode, revRecTriggerCondition, revenueRecognitionRuleName, serviceEndDate, serviceStartDate, shipToContactId, sku, soldToContactId, soldToContactSnapshotId, sourceItemType, subscriptionId, subscriptionName, taxAmount, taxCode, taxMode, taxationItems, unbilledReceivablesAccountingCode, unitOfMeasure, unitPrice, numberOfDeliveries, reflectDiscountInNetAmount, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class InvoiceItem {\n");
sb.append(" integrationIdNS: ").append(toIndentedString(integrationIdNS)).append("\n");
sb.append(" integrationStatusNS: ").append(toIndentedString(integrationStatusNS)).append("\n");
sb.append(" syncDateNS: ").append(toIndentedString(syncDateNS)).append("\n");
sb.append(" accountingCode: ").append(toIndentedString(accountingCode)).append("\n");
sb.append(" adjustmentLiabilityAccountingCode: ").append(toIndentedString(adjustmentLiabilityAccountingCode)).append("\n");
sb.append(" adjustmentRevenueAccountingCode: ").append(toIndentedString(adjustmentRevenueAccountingCode)).append("\n");
sb.append(" appliedToItemId: ").append(toIndentedString(appliedToItemId)).append("\n");
sb.append(" availableToCreditAmount: ").append(toIndentedString(availableToCreditAmount)).append("\n");
sb.append(" balance: ").append(toIndentedString(balance)).append("\n");
sb.append(" bookingReference: ").append(toIndentedString(bookingReference)).append("\n");
sb.append(" chargeAmount: ").append(toIndentedString(chargeAmount)).append("\n");
sb.append(" chargeDate: ").append(toIndentedString(chargeDate)).append("\n");
sb.append(" chargeDescription: ").append(toIndentedString(chargeDescription)).append("\n");
sb.append(" chargeId: ").append(toIndentedString(chargeId)).append("\n");
sb.append(" chargeName: ").append(toIndentedString(chargeName)).append("\n");
sb.append(" chargeType: ").append(toIndentedString(chargeType)).append("\n");
sb.append(" contractAssetAccountingCode: ").append(toIndentedString(contractAssetAccountingCode)).append("\n");
sb.append(" contractLiabilityAccountingCode: ").append(toIndentedString(contractLiabilityAccountingCode)).append("\n");
sb.append(" contractRecognizedRevenueAccountingCode: ").append(toIndentedString(contractRecognizedRevenueAccountingCode)).append("\n");
sb.append(" deferredRevenueAccountingCode: ").append(toIndentedString(deferredRevenueAccountingCode)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" excludeItemBillingFromRevenueAccounting: ").append(toIndentedString(excludeItemBillingFromRevenueAccounting)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" invoiceScheduleId: ").append(toIndentedString(invoiceScheduleId)).append("\n");
sb.append(" invoiceScheduleItemId: ").append(toIndentedString(invoiceScheduleItemId)).append("\n");
sb.append(" itemType: ").append(toIndentedString(itemType)).append("\n");
sb.append(" processingType: ").append(toIndentedString(processingType)).append("\n");
sb.append(" productName: ").append(toIndentedString(productName)).append("\n");
sb.append(" productRatePlanChargeId: ").append(toIndentedString(productRatePlanChargeId)).append("\n");
sb.append(" purchaseOrderNumber: ").append(toIndentedString(purchaseOrderNumber)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" recognizedRevenueAccountingCode: ").append(toIndentedString(recognizedRevenueAccountingCode)).append("\n");
sb.append(" revRecCode: ").append(toIndentedString(revRecCode)).append("\n");
sb.append(" revRecTriggerCondition: ").append(toIndentedString(revRecTriggerCondition)).append("\n");
sb.append(" revenueRecognitionRuleName: ").append(toIndentedString(revenueRecognitionRuleName)).append("\n");
sb.append(" serviceEndDate: ").append(toIndentedString(serviceEndDate)).append("\n");
sb.append(" serviceStartDate: ").append(toIndentedString(serviceStartDate)).append("\n");
sb.append(" shipToContactId: ").append(toIndentedString(shipToContactId)).append("\n");
sb.append(" sku: ").append(toIndentedString(sku)).append("\n");
sb.append(" soldToContactId: ").append(toIndentedString(soldToContactId)).append("\n");
sb.append(" soldToContactSnapshotId: ").append(toIndentedString(soldToContactSnapshotId)).append("\n");
sb.append(" sourceItemType: ").append(toIndentedString(sourceItemType)).append("\n");
sb.append(" subscriptionId: ").append(toIndentedString(subscriptionId)).append("\n");
sb.append(" subscriptionName: ").append(toIndentedString(subscriptionName)).append("\n");
sb.append(" taxAmount: ").append(toIndentedString(taxAmount)).append("\n");
sb.append(" taxCode: ").append(toIndentedString(taxCode)).append("\n");
sb.append(" taxMode: ").append(toIndentedString(taxMode)).append("\n");
sb.append(" taxationItems: ").append(toIndentedString(taxationItems)).append("\n");
sb.append(" unbilledReceivablesAccountingCode: ").append(toIndentedString(unbilledReceivablesAccountingCode)).append("\n");
sb.append(" unitOfMeasure: ").append(toIndentedString(unitOfMeasure)).append("\n");
sb.append(" unitPrice: ").append(toIndentedString(unitPrice)).append("\n");
sb.append(" numberOfDeliveries: ").append(toIndentedString(numberOfDeliveries)).append("\n");
sb.append(" reflectDiscountInNetAmount: ").append(toIndentedString(reflectDiscountInNetAmount)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("IntegrationId__NS");
openapiFields.add("IntegrationStatus__NS");
openapiFields.add("SyncDate__NS");
openapiFields.add("accountingCode");
openapiFields.add("adjustmentLiabilityAccountingCode");
openapiFields.add("adjustmentRevenueAccountingCode");
openapiFields.add("appliedToItemId");
openapiFields.add("availableToCreditAmount");
openapiFields.add("balance");
openapiFields.add("bookingReference");
openapiFields.add("chargeAmount");
openapiFields.add("chargeDate");
openapiFields.add("chargeDescription");
openapiFields.add("chargeId");
openapiFields.add("chargeName");
openapiFields.add("chargeType");
openapiFields.add("contractAssetAccountingCode");
openapiFields.add("contractLiabilityAccountingCode");
openapiFields.add("contractRecognizedRevenueAccountingCode");
openapiFields.add("deferredRevenueAccountingCode");
openapiFields.add("description");
openapiFields.add("excludeItemBillingFromRevenueAccounting");
openapiFields.add("id");
openapiFields.add("invoiceScheduleId");
openapiFields.add("invoiceScheduleItemId");
openapiFields.add("itemType");
openapiFields.add("processingType");
openapiFields.add("productName");
openapiFields.add("productRatePlanChargeId");
openapiFields.add("purchaseOrderNumber");
openapiFields.add("quantity");
openapiFields.add("recognizedRevenueAccountingCode");
openapiFields.add("revRecCode");
openapiFields.add("revRecTriggerCondition");
openapiFields.add("revenueRecognitionRuleName");
openapiFields.add("serviceEndDate");
openapiFields.add("serviceStartDate");
openapiFields.add("shipToContactId");
openapiFields.add("sku");
openapiFields.add("soldToContactId");
openapiFields.add("soldToContactSnapshotId");
openapiFields.add("sourceItemType");
openapiFields.add("subscriptionId");
openapiFields.add("subscriptionName");
openapiFields.add("taxAmount");
openapiFields.add("taxCode");
openapiFields.add("taxMode");
openapiFields.add("taxationItems");
openapiFields.add("unbilledReceivablesAccountingCode");
openapiFields.add("unitOfMeasure");
openapiFields.add("unitPrice");
openapiFields.add("numberOfDeliveries");
openapiFields.add("reflectDiscountInNetAmount");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to InvoiceItem
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!InvoiceItem.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in InvoiceItem is not found in the empty JSON string", InvoiceItem.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("IntegrationId__NS") != null && !jsonObj.get("IntegrationId__NS").isJsonNull()) && !jsonObj.get("IntegrationId__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationId__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationId__NS").toString()));
}
if ((jsonObj.get("IntegrationStatus__NS") != null && !jsonObj.get("IntegrationStatus__NS").isJsonNull()) && !jsonObj.get("IntegrationStatus__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationStatus__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationStatus__NS").toString()));
}
if ((jsonObj.get("SyncDate__NS") != null && !jsonObj.get("SyncDate__NS").isJsonNull()) && !jsonObj.get("SyncDate__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SyncDate__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SyncDate__NS").toString()));
}
if ((jsonObj.get("accountingCode") != null && !jsonObj.get("accountingCode").isJsonNull()) && !jsonObj.get("accountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountingCode").toString()));
}
if ((jsonObj.get("adjustmentLiabilityAccountingCode") != null && !jsonObj.get("adjustmentLiabilityAccountingCode").isJsonNull()) && !jsonObj.get("adjustmentLiabilityAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `adjustmentLiabilityAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("adjustmentLiabilityAccountingCode").toString()));
}
if ((jsonObj.get("adjustmentRevenueAccountingCode") != null && !jsonObj.get("adjustmentRevenueAccountingCode").isJsonNull()) && !jsonObj.get("adjustmentRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `adjustmentRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("adjustmentRevenueAccountingCode").toString()));
}
if ((jsonObj.get("appliedToItemId") != null && !jsonObj.get("appliedToItemId").isJsonNull()) && !jsonObj.get("appliedToItemId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `appliedToItemId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("appliedToItemId").toString()));
}
if ((jsonObj.get("balance") != null && !jsonObj.get("balance").isJsonNull()) && !jsonObj.get("balance").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `balance` to be a primitive type in the JSON string but got `%s`", jsonObj.get("balance").toString()));
}
if ((jsonObj.get("bookingReference") != null && !jsonObj.get("bookingReference").isJsonNull()) && !jsonObj.get("bookingReference").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bookingReference` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bookingReference").toString()));
}
if ((jsonObj.get("chargeAmount") != null && !jsonObj.get("chargeAmount").isJsonNull()) && !jsonObj.get("chargeAmount").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeAmount` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeAmount").toString()));
}
if ((jsonObj.get("chargeDate") != null && !jsonObj.get("chargeDate").isJsonNull()) && !jsonObj.get("chargeDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeDate").toString()));
}
if ((jsonObj.get("chargeDescription") != null && !jsonObj.get("chargeDescription").isJsonNull()) && !jsonObj.get("chargeDescription").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeDescription` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeDescription").toString()));
}
if ((jsonObj.get("chargeId") != null && !jsonObj.get("chargeId").isJsonNull()) && !jsonObj.get("chargeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeId").toString()));
}
if ((jsonObj.get("chargeName") != null && !jsonObj.get("chargeName").isJsonNull()) && !jsonObj.get("chargeName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeName").toString()));
}
if ((jsonObj.get("chargeType") != null && !jsonObj.get("chargeType").isJsonNull()) && !jsonObj.get("chargeType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeType").toString()));
}
// validate the optional field `chargeType`
if (jsonObj.get("chargeType") != null && !jsonObj.get("chargeType").isJsonNull()) {
ChargeType.validateJsonElement(jsonObj.get("chargeType"));
}
if ((jsonObj.get("contractAssetAccountingCode") != null && !jsonObj.get("contractAssetAccountingCode").isJsonNull()) && !jsonObj.get("contractAssetAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractAssetAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractAssetAccountingCode").toString()));
}
if ((jsonObj.get("contractLiabilityAccountingCode") != null && !jsonObj.get("contractLiabilityAccountingCode").isJsonNull()) && !jsonObj.get("contractLiabilityAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractLiabilityAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractLiabilityAccountingCode").toString()));
}
if ((jsonObj.get("contractRecognizedRevenueAccountingCode") != null && !jsonObj.get("contractRecognizedRevenueAccountingCode").isJsonNull()) && !jsonObj.get("contractRecognizedRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractRecognizedRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractRecognizedRevenueAccountingCode").toString()));
}
if ((jsonObj.get("deferredRevenueAccountingCode") != null && !jsonObj.get("deferredRevenueAccountingCode").isJsonNull()) && !jsonObj.get("deferredRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `deferredRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("deferredRevenueAccountingCode").toString()));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("invoiceScheduleId") != null && !jsonObj.get("invoiceScheduleId").isJsonNull()) && !jsonObj.get("invoiceScheduleId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceScheduleId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceScheduleId").toString()));
}
if ((jsonObj.get("invoiceScheduleItemId") != null && !jsonObj.get("invoiceScheduleItemId").isJsonNull()) && !jsonObj.get("invoiceScheduleItemId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceScheduleItemId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceScheduleItemId").toString()));
}
if ((jsonObj.get("itemType") != null && !jsonObj.get("itemType").isJsonNull()) && !jsonObj.get("itemType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `itemType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("itemType").toString()));
}
if ((jsonObj.get("processingType") != null && !jsonObj.get("processingType").isJsonNull()) && !jsonObj.get("processingType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `processingType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("processingType").toString()));
}
// validate the optional field `processingType`
if (jsonObj.get("processingType") != null && !jsonObj.get("processingType").isJsonNull()) {
BillingDocumentItemProcessingType.validateJsonElement(jsonObj.get("processingType"));
}
if ((jsonObj.get("productName") != null && !jsonObj.get("productName").isJsonNull()) && !jsonObj.get("productName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productName").toString()));
}
if ((jsonObj.get("productRatePlanChargeId") != null && !jsonObj.get("productRatePlanChargeId").isJsonNull()) && !jsonObj.get("productRatePlanChargeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanChargeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productRatePlanChargeId").toString()));
}
if ((jsonObj.get("purchaseOrderNumber") != null && !jsonObj.get("purchaseOrderNumber").isJsonNull()) && !jsonObj.get("purchaseOrderNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `purchaseOrderNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("purchaseOrderNumber").toString()));
}
if ((jsonObj.get("recognizedRevenueAccountingCode") != null && !jsonObj.get("recognizedRevenueAccountingCode").isJsonNull()) && !jsonObj.get("recognizedRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `recognizedRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("recognizedRevenueAccountingCode").toString()));
}
if ((jsonObj.get("revRecCode") != null && !jsonObj.get("revRecCode").isJsonNull()) && !jsonObj.get("revRecCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revRecCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revRecCode").toString()));
}
if ((jsonObj.get("revRecTriggerCondition") != null && !jsonObj.get("revRecTriggerCondition").isJsonNull()) && !jsonObj.get("revRecTriggerCondition").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revRecTriggerCondition` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revRecTriggerCondition").toString()));
}
// validate the optional field `revRecTriggerCondition`
if (jsonObj.get("revRecTriggerCondition") != null && !jsonObj.get("revRecTriggerCondition").isJsonNull()) {
RevRecTrigger.validateJsonElement(jsonObj.get("revRecTriggerCondition"));
}
if ((jsonObj.get("revenueRecognitionRuleName") != null && !jsonObj.get("revenueRecognitionRuleName").isJsonNull()) && !jsonObj.get("revenueRecognitionRuleName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueRecognitionRuleName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueRecognitionRuleName").toString()));
}
if ((jsonObj.get("shipToContactId") != null && !jsonObj.get("shipToContactId").isJsonNull()) && !jsonObj.get("shipToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `shipToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("shipToContactId").toString()));
}
if ((jsonObj.get("sku") != null && !jsonObj.get("sku").isJsonNull()) && !jsonObj.get("sku").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sku` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sku").toString()));
}
if ((jsonObj.get("soldToContactId") != null && !jsonObj.get("soldToContactId").isJsonNull()) && !jsonObj.get("soldToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToContactId").toString()));
}
if ((jsonObj.get("soldToContactSnapshotId") != null && !jsonObj.get("soldToContactSnapshotId").isJsonNull()) && !jsonObj.get("soldToContactSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToContactSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToContactSnapshotId").toString()));
}
if ((jsonObj.get("sourceItemType") != null && !jsonObj.get("sourceItemType").isJsonNull()) && !jsonObj.get("sourceItemType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sourceItemType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sourceItemType").toString()));
}
// validate the optional field `sourceItemType`
if (jsonObj.get("sourceItemType") != null && !jsonObj.get("sourceItemType").isJsonNull()) {
BillingDocumentItemSourceType.validateJsonElement(jsonObj.get("sourceItemType"));
}
if ((jsonObj.get("subscriptionId") != null && !jsonObj.get("subscriptionId").isJsonNull()) && !jsonObj.get("subscriptionId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("subscriptionId").toString()));
}
if ((jsonObj.get("subscriptionName") != null && !jsonObj.get("subscriptionName").isJsonNull()) && !jsonObj.get("subscriptionName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("subscriptionName").toString()));
}
if ((jsonObj.get("taxAmount") != null && !jsonObj.get("taxAmount").isJsonNull()) && !jsonObj.get("taxAmount").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxAmount` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxAmount").toString()));
}
if ((jsonObj.get("taxCode") != null && !jsonObj.get("taxCode").isJsonNull()) && !jsonObj.get("taxCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxCode").toString()));
}
if ((jsonObj.get("taxMode") != null && !jsonObj.get("taxMode").isJsonNull()) && !jsonObj.get("taxMode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxMode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxMode").toString()));
}
// validate the optional field `taxMode`
if (jsonObj.get("taxMode") != null && !jsonObj.get("taxMode").isJsonNull()) {
TaxMode.validateJsonElement(jsonObj.get("taxMode"));
}
// validate the optional field `taxationItems`
if (jsonObj.get("taxationItems") != null && !jsonObj.get("taxationItems").isJsonNull()) {
TaxationItemsData.validateJsonElement(jsonObj.get("taxationItems"));
}
if ((jsonObj.get("unbilledReceivablesAccountingCode") != null && !jsonObj.get("unbilledReceivablesAccountingCode").isJsonNull()) && !jsonObj.get("unbilledReceivablesAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `unbilledReceivablesAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("unbilledReceivablesAccountingCode").toString()));
}
if ((jsonObj.get("unitOfMeasure") != null && !jsonObj.get("unitOfMeasure").isJsonNull()) && !jsonObj.get("unitOfMeasure").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `unitOfMeasure` to be a primitive type in the JSON string but got `%s`", jsonObj.get("unitOfMeasure").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!InvoiceItem.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'InvoiceItem' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(InvoiceItem.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, InvoiceItem value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public InvoiceItem read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
InvoiceItem instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of InvoiceItem given an JSON string
*
* @param jsonString JSON string
* @return An instance of InvoiceItem
* @throws IOException if the JSON string is invalid with respect to InvoiceItem
*/
public static InvoiceItem fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, InvoiceItem.class);
}
/**
* Convert an instance of InvoiceItem to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy