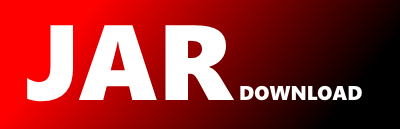
com.zuora.model.Job Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.DataBackfillJobStatus;
import com.zuora.model.JobType;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* Job
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class Job {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_IMPORT_TYPE = "importType";
@SerializedName(SERIALIZED_NAME_IMPORT_TYPE)
private JobType importType;
public static final String SERIALIZED_NAME_UPLOADED_FILE_ID = "uploadedFileId";
@SerializedName(SERIALIZED_NAME_UPLOADED_FILE_ID)
private String uploadedFileId;
public static final String SERIALIZED_NAME_UPLOADED_FILE_NAME = "uploadedFileName";
@SerializedName(SERIALIZED_NAME_UPLOADED_FILE_NAME)
private String uploadedFileName;
public static final String SERIALIZED_NAME_UPLOADED_FILE_URL = "uploadedFileUrl";
@SerializedName(SERIALIZED_NAME_UPLOADED_FILE_URL)
private String uploadedFileUrl;
public static final String SERIALIZED_NAME_UPLOADED_FILE_SIZE = "uploadedFileSize";
@SerializedName(SERIALIZED_NAME_UPLOADED_FILE_SIZE)
private String uploadedFileSize;
public static final String SERIALIZED_NAME_INPUT_FILE_SIZE = "inputFileSize";
@SerializedName(SERIALIZED_NAME_INPUT_FILE_SIZE)
private Long inputFileSize;
public static final String SERIALIZED_NAME_OUTPUT_SIZE = "outputSize";
@SerializedName(SERIALIZED_NAME_OUTPUT_SIZE)
private String outputSize;
public static final String SERIALIZED_NAME_OUTPUT_TYPE = "outputType";
@SerializedName(SERIALIZED_NAME_OUTPUT_TYPE)
private String outputType;
public static final String SERIALIZED_NAME_OUTPUT_FILE_SIZE = "outputFileSize";
@SerializedName(SERIALIZED_NAME_OUTPUT_FILE_SIZE)
private Long outputFileSize;
public static final String SERIALIZED_NAME_UPLOADED_BY = "uploadedBy";
@SerializedName(SERIALIZED_NAME_UPLOADED_BY)
private String uploadedBy;
public static final String SERIALIZED_NAME_UPLOADED_ON = "uploadedOn";
@SerializedName(SERIALIZED_NAME_UPLOADED_ON)
private OffsetDateTime uploadedOn;
public static final String SERIALIZED_NAME_COMPLETED_ON = "completedOn";
@SerializedName(SERIALIZED_NAME_COMPLETED_ON)
private OffsetDateTime completedOn;
public static final String SERIALIZED_NAME_STARTED_PROCESSING_ON = "startedProcessingOn";
@SerializedName(SERIALIZED_NAME_STARTED_PROCESSING_ON)
private OffsetDateTime startedProcessingOn;
public static final String SERIALIZED_NAME_RESULT_FILE_ID = "resultFileId";
@SerializedName(SERIALIZED_NAME_RESULT_FILE_ID)
private String resultFileId;
public static final String SERIALIZED_NAME_RESULT_FILE_NAME = "resultFileName";
@SerializedName(SERIALIZED_NAME_RESULT_FILE_NAME)
private String resultFileName;
public static final String SERIALIZED_NAME_RESULT_FILE_URL = "resultFileUrl";
@SerializedName(SERIALIZED_NAME_RESULT_FILE_URL)
private String resultFileUrl;
public static final String SERIALIZED_NAME_TOTAL_COUNT = "totalCount";
@SerializedName(SERIALIZED_NAME_TOTAL_COUNT)
private Integer totalCount;
public static final String SERIALIZED_NAME_FAILED_COUNT = "failedCount";
@SerializedName(SERIALIZED_NAME_FAILED_COUNT)
private Integer failedCount;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private DataBackfillJobStatus status;
public static final String SERIALIZED_NAME_FAILURE_MESSAGE = "failureMessage";
@SerializedName(SERIALIZED_NAME_FAILURE_MESSAGE)
private String failureMessage;
public static final String SERIALIZED_NAME_PROCESSED_COUNT = "processedCount";
@SerializedName(SERIALIZED_NAME_PROCESSED_COUNT)
private Long processedCount;
public static final String SERIALIZED_NAME_SUCCESS_COUNT = "successCount";
@SerializedName(SERIALIZED_NAME_SUCCESS_COUNT)
private Long successCount;
public static final String SERIALIZED_NAME_REMAINING_TIME = "remainingTime";
@SerializedName(SERIALIZED_NAME_REMAINING_TIME)
private Long remainingTime;
public static final String SERIALIZED_NAME_REMAINING_TIME_TEXT = "remainingTimeText";
@SerializedName(SERIALIZED_NAME_REMAINING_TIME_TEXT)
private String remainingTimeText;
public static final String SERIALIZED_NAME_COMPLETED_PERCENTAGE = "completedPercentage";
@SerializedName(SERIALIZED_NAME_COMPLETED_PERCENTAGE)
private Integer completedPercentage;
public Job() {
}
public Job id(String id) {
this.id = id;
return this;
}
/**
* Job id
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public Job importType(JobType importType) {
this.importType = importType;
return this;
}
/**
* Get importType
* @return importType
*/
@javax.annotation.Nullable
public JobType getImportType() {
return importType;
}
public void setImportType(JobType importType) {
this.importType = importType;
}
public Job uploadedFileId(String uploadedFileId) {
this.uploadedFileId = uploadedFileId;
return this;
}
/**
* Id of uploaded file
* @return uploadedFileId
*/
@javax.annotation.Nullable
public String getUploadedFileId() {
return uploadedFileId;
}
public void setUploadedFileId(String uploadedFileId) {
this.uploadedFileId = uploadedFileId;
}
public Job uploadedFileName(String uploadedFileName) {
this.uploadedFileName = uploadedFileName;
return this;
}
/**
* Name of uploaded file
* @return uploadedFileName
*/
@javax.annotation.Nullable
public String getUploadedFileName() {
return uploadedFileName;
}
public void setUploadedFileName(String uploadedFileName) {
this.uploadedFileName = uploadedFileName;
}
public Job uploadedFileUrl(String uploadedFileUrl) {
this.uploadedFileUrl = uploadedFileUrl;
return this;
}
/**
* Get uploadedFileUrl
* @return uploadedFileUrl
*/
@javax.annotation.Nullable
public String getUploadedFileUrl() {
return uploadedFileUrl;
}
public void setUploadedFileUrl(String uploadedFileUrl) {
this.uploadedFileUrl = uploadedFileUrl;
}
public Job uploadedFileSize(String uploadedFileSize) {
this.uploadedFileSize = uploadedFileSize;
return this;
}
/**
* Get uploadedFileSize
* @return uploadedFileSize
*/
@javax.annotation.Nullable
public String getUploadedFileSize() {
return uploadedFileSize;
}
public void setUploadedFileSize(String uploadedFileSize) {
this.uploadedFileSize = uploadedFileSize;
}
public Job inputFileSize(Long inputFileSize) {
this.inputFileSize = inputFileSize;
return this;
}
/**
* Get inputFileSize
* @return inputFileSize
*/
@javax.annotation.Nullable
public Long getInputFileSize() {
return inputFileSize;
}
public void setInputFileSize(Long inputFileSize) {
this.inputFileSize = inputFileSize;
}
public Job outputSize(String outputSize) {
this.outputSize = outputSize;
return this;
}
/**
* Get outputSize
* @return outputSize
*/
@javax.annotation.Nullable
public String getOutputSize() {
return outputSize;
}
public void setOutputSize(String outputSize) {
this.outputSize = outputSize;
}
public Job outputType(String outputType) {
this.outputType = outputType;
return this;
}
/**
* Get outputType
* @return outputType
*/
@javax.annotation.Nullable
public String getOutputType() {
return outputType;
}
public void setOutputType(String outputType) {
this.outputType = outputType;
}
public Job outputFileSize(Long outputFileSize) {
this.outputFileSize = outputFileSize;
return this;
}
/**
* Get outputFileSize
* @return outputFileSize
*/
@javax.annotation.Nullable
public Long getOutputFileSize() {
return outputFileSize;
}
public void setOutputFileSize(Long outputFileSize) {
this.outputFileSize = outputFileSize;
}
public Job uploadedBy(String uploadedBy) {
this.uploadedBy = uploadedBy;
return this;
}
/**
* Get uploadedBy
* @return uploadedBy
*/
@javax.annotation.Nullable
public String getUploadedBy() {
return uploadedBy;
}
public void setUploadedBy(String uploadedBy) {
this.uploadedBy = uploadedBy;
}
public Job uploadedOn(OffsetDateTime uploadedOn) {
this.uploadedOn = uploadedOn;
return this;
}
/**
* Get uploadedOn
* @return uploadedOn
*/
@javax.annotation.Nullable
public OffsetDateTime getUploadedOn() {
return uploadedOn;
}
public void setUploadedOn(OffsetDateTime uploadedOn) {
this.uploadedOn = uploadedOn;
}
public Job completedOn(OffsetDateTime completedOn) {
this.completedOn = completedOn;
return this;
}
/**
* Get completedOn
* @return completedOn
*/
@javax.annotation.Nullable
public OffsetDateTime getCompletedOn() {
return completedOn;
}
public void setCompletedOn(OffsetDateTime completedOn) {
this.completedOn = completedOn;
}
public Job startedProcessingOn(OffsetDateTime startedProcessingOn) {
this.startedProcessingOn = startedProcessingOn;
return this;
}
/**
* Get startedProcessingOn
* @return startedProcessingOn
*/
@javax.annotation.Nullable
public OffsetDateTime getStartedProcessingOn() {
return startedProcessingOn;
}
public void setStartedProcessingOn(OffsetDateTime startedProcessingOn) {
this.startedProcessingOn = startedProcessingOn;
}
public Job resultFileId(String resultFileId) {
this.resultFileId = resultFileId;
return this;
}
/**
* Get resultFileId
* @return resultFileId
*/
@javax.annotation.Nullable
public String getResultFileId() {
return resultFileId;
}
public void setResultFileId(String resultFileId) {
this.resultFileId = resultFileId;
}
public Job resultFileName(String resultFileName) {
this.resultFileName = resultFileName;
return this;
}
/**
* Get resultFileName
* @return resultFileName
*/
@javax.annotation.Nullable
public String getResultFileName() {
return resultFileName;
}
public void setResultFileName(String resultFileName) {
this.resultFileName = resultFileName;
}
public Job resultFileUrl(String resultFileUrl) {
this.resultFileUrl = resultFileUrl;
return this;
}
/**
* Get resultFileUrl
* @return resultFileUrl
*/
@javax.annotation.Nullable
public String getResultFileUrl() {
return resultFileUrl;
}
public void setResultFileUrl(String resultFileUrl) {
this.resultFileUrl = resultFileUrl;
}
public Job totalCount(Integer totalCount) {
this.totalCount = totalCount;
return this;
}
/**
* Get totalCount
* @return totalCount
*/
@javax.annotation.Nullable
public Integer getTotalCount() {
return totalCount;
}
public void setTotalCount(Integer totalCount) {
this.totalCount = totalCount;
}
public Job failedCount(Integer failedCount) {
this.failedCount = failedCount;
return this;
}
/**
* Get failedCount
* @return failedCount
*/
@javax.annotation.Nullable
public Integer getFailedCount() {
return failedCount;
}
public void setFailedCount(Integer failedCount) {
this.failedCount = failedCount;
}
public Job status(DataBackfillJobStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public DataBackfillJobStatus getStatus() {
return status;
}
public void setStatus(DataBackfillJobStatus status) {
this.status = status;
}
public Job failureMessage(String failureMessage) {
this.failureMessage = failureMessage;
return this;
}
/**
* Get failureMessage
* @return failureMessage
*/
@javax.annotation.Nullable
public String getFailureMessage() {
return failureMessage;
}
public void setFailureMessage(String failureMessage) {
this.failureMessage = failureMessage;
}
public Job processedCount(Long processedCount) {
this.processedCount = processedCount;
return this;
}
/**
* Get processedCount
* @return processedCount
*/
@javax.annotation.Nullable
public Long getProcessedCount() {
return processedCount;
}
public void setProcessedCount(Long processedCount) {
this.processedCount = processedCount;
}
public Job successCount(Long successCount) {
this.successCount = successCount;
return this;
}
/**
* Get successCount
* @return successCount
*/
@javax.annotation.Nullable
public Long getSuccessCount() {
return successCount;
}
public void setSuccessCount(Long successCount) {
this.successCount = successCount;
}
public Job remainingTime(Long remainingTime) {
this.remainingTime = remainingTime;
return this;
}
/**
* Get remainingTime
* @return remainingTime
*/
@javax.annotation.Nullable
public Long getRemainingTime() {
return remainingTime;
}
public void setRemainingTime(Long remainingTime) {
this.remainingTime = remainingTime;
}
public Job remainingTimeText(String remainingTimeText) {
this.remainingTimeText = remainingTimeText;
return this;
}
/**
* Get remainingTimeText
* @return remainingTimeText
*/
@javax.annotation.Nullable
public String getRemainingTimeText() {
return remainingTimeText;
}
public void setRemainingTimeText(String remainingTimeText) {
this.remainingTimeText = remainingTimeText;
}
public Job completedPercentage(Integer completedPercentage) {
this.completedPercentage = completedPercentage;
return this;
}
/**
* Get completedPercentage
* @return completedPercentage
*/
@javax.annotation.Nullable
public Integer getCompletedPercentage() {
return completedPercentage;
}
public void setCompletedPercentage(Integer completedPercentage) {
this.completedPercentage = completedPercentage;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the Job instance itself
*/
public Job putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Job job = (Job) o;
return Objects.equals(this.id, job.id) &&
Objects.equals(this.importType, job.importType) &&
Objects.equals(this.uploadedFileId, job.uploadedFileId) &&
Objects.equals(this.uploadedFileName, job.uploadedFileName) &&
Objects.equals(this.uploadedFileUrl, job.uploadedFileUrl) &&
Objects.equals(this.uploadedFileSize, job.uploadedFileSize) &&
Objects.equals(this.inputFileSize, job.inputFileSize) &&
Objects.equals(this.outputSize, job.outputSize) &&
Objects.equals(this.outputType, job.outputType) &&
Objects.equals(this.outputFileSize, job.outputFileSize) &&
Objects.equals(this.uploadedBy, job.uploadedBy) &&
Objects.equals(this.uploadedOn, job.uploadedOn) &&
Objects.equals(this.completedOn, job.completedOn) &&
Objects.equals(this.startedProcessingOn, job.startedProcessingOn) &&
Objects.equals(this.resultFileId, job.resultFileId) &&
Objects.equals(this.resultFileName, job.resultFileName) &&
Objects.equals(this.resultFileUrl, job.resultFileUrl) &&
Objects.equals(this.totalCount, job.totalCount) &&
Objects.equals(this.failedCount, job.failedCount) &&
Objects.equals(this.status, job.status) &&
Objects.equals(this.failureMessage, job.failureMessage) &&
Objects.equals(this.processedCount, job.processedCount) &&
Objects.equals(this.successCount, job.successCount) &&
Objects.equals(this.remainingTime, job.remainingTime) &&
Objects.equals(this.remainingTimeText, job.remainingTimeText) &&
Objects.equals(this.completedPercentage, job.completedPercentage)&&
Objects.equals(this.additionalProperties, job.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(id, importType, uploadedFileId, uploadedFileName, uploadedFileUrl, uploadedFileSize, inputFileSize, outputSize, outputType, outputFileSize, uploadedBy, uploadedOn, completedOn, startedProcessingOn, resultFileId, resultFileName, resultFileUrl, totalCount, failedCount, status, failureMessage, processedCount, successCount, remainingTime, remainingTimeText, completedPercentage, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Job {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" importType: ").append(toIndentedString(importType)).append("\n");
sb.append(" uploadedFileId: ").append(toIndentedString(uploadedFileId)).append("\n");
sb.append(" uploadedFileName: ").append(toIndentedString(uploadedFileName)).append("\n");
sb.append(" uploadedFileUrl: ").append(toIndentedString(uploadedFileUrl)).append("\n");
sb.append(" uploadedFileSize: ").append(toIndentedString(uploadedFileSize)).append("\n");
sb.append(" inputFileSize: ").append(toIndentedString(inputFileSize)).append("\n");
sb.append(" outputSize: ").append(toIndentedString(outputSize)).append("\n");
sb.append(" outputType: ").append(toIndentedString(outputType)).append("\n");
sb.append(" outputFileSize: ").append(toIndentedString(outputFileSize)).append("\n");
sb.append(" uploadedBy: ").append(toIndentedString(uploadedBy)).append("\n");
sb.append(" uploadedOn: ").append(toIndentedString(uploadedOn)).append("\n");
sb.append(" completedOn: ").append(toIndentedString(completedOn)).append("\n");
sb.append(" startedProcessingOn: ").append(toIndentedString(startedProcessingOn)).append("\n");
sb.append(" resultFileId: ").append(toIndentedString(resultFileId)).append("\n");
sb.append(" resultFileName: ").append(toIndentedString(resultFileName)).append("\n");
sb.append(" resultFileUrl: ").append(toIndentedString(resultFileUrl)).append("\n");
sb.append(" totalCount: ").append(toIndentedString(totalCount)).append("\n");
sb.append(" failedCount: ").append(toIndentedString(failedCount)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" failureMessage: ").append(toIndentedString(failureMessage)).append("\n");
sb.append(" processedCount: ").append(toIndentedString(processedCount)).append("\n");
sb.append(" successCount: ").append(toIndentedString(successCount)).append("\n");
sb.append(" remainingTime: ").append(toIndentedString(remainingTime)).append("\n");
sb.append(" remainingTimeText: ").append(toIndentedString(remainingTimeText)).append("\n");
sb.append(" completedPercentage: ").append(toIndentedString(completedPercentage)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("id");
openapiFields.add("importType");
openapiFields.add("uploadedFileId");
openapiFields.add("uploadedFileName");
openapiFields.add("uploadedFileUrl");
openapiFields.add("uploadedFileSize");
openapiFields.add("inputFileSize");
openapiFields.add("outputSize");
openapiFields.add("outputType");
openapiFields.add("outputFileSize");
openapiFields.add("uploadedBy");
openapiFields.add("uploadedOn");
openapiFields.add("completedOn");
openapiFields.add("startedProcessingOn");
openapiFields.add("resultFileId");
openapiFields.add("resultFileName");
openapiFields.add("resultFileUrl");
openapiFields.add("totalCount");
openapiFields.add("failedCount");
openapiFields.add("status");
openapiFields.add("failureMessage");
openapiFields.add("processedCount");
openapiFields.add("successCount");
openapiFields.add("remainingTime");
openapiFields.add("remainingTimeText");
openapiFields.add("completedPercentage");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to Job
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!Job.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in Job is not found in the empty JSON string", Job.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
// validate the optional field `importType`
if (jsonObj.get("importType") != null && !jsonObj.get("importType").isJsonNull()) {
JobType.validateJsonElement(jsonObj.get("importType"));
}
if ((jsonObj.get("uploadedFileId") != null && !jsonObj.get("uploadedFileId").isJsonNull()) && !jsonObj.get("uploadedFileId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `uploadedFileId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("uploadedFileId").toString()));
}
if ((jsonObj.get("uploadedFileName") != null && !jsonObj.get("uploadedFileName").isJsonNull()) && !jsonObj.get("uploadedFileName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `uploadedFileName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("uploadedFileName").toString()));
}
if ((jsonObj.get("uploadedFileUrl") != null && !jsonObj.get("uploadedFileUrl").isJsonNull()) && !jsonObj.get("uploadedFileUrl").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `uploadedFileUrl` to be a primitive type in the JSON string but got `%s`", jsonObj.get("uploadedFileUrl").toString()));
}
if ((jsonObj.get("uploadedFileSize") != null && !jsonObj.get("uploadedFileSize").isJsonNull()) && !jsonObj.get("uploadedFileSize").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `uploadedFileSize` to be a primitive type in the JSON string but got `%s`", jsonObj.get("uploadedFileSize").toString()));
}
if ((jsonObj.get("outputSize") != null && !jsonObj.get("outputSize").isJsonNull()) && !jsonObj.get("outputSize").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `outputSize` to be a primitive type in the JSON string but got `%s`", jsonObj.get("outputSize").toString()));
}
if ((jsonObj.get("outputType") != null && !jsonObj.get("outputType").isJsonNull()) && !jsonObj.get("outputType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `outputType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("outputType").toString()));
}
if ((jsonObj.get("uploadedBy") != null && !jsonObj.get("uploadedBy").isJsonNull()) && !jsonObj.get("uploadedBy").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `uploadedBy` to be a primitive type in the JSON string but got `%s`", jsonObj.get("uploadedBy").toString()));
}
if ((jsonObj.get("resultFileId") != null && !jsonObj.get("resultFileId").isJsonNull()) && !jsonObj.get("resultFileId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `resultFileId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("resultFileId").toString()));
}
if ((jsonObj.get("resultFileName") != null && !jsonObj.get("resultFileName").isJsonNull()) && !jsonObj.get("resultFileName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `resultFileName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("resultFileName").toString()));
}
if ((jsonObj.get("resultFileUrl") != null && !jsonObj.get("resultFileUrl").isJsonNull()) && !jsonObj.get("resultFileUrl").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `resultFileUrl` to be a primitive type in the JSON string but got `%s`", jsonObj.get("resultFileUrl").toString()));
}
// validate the optional field `status`
if (jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) {
DataBackfillJobStatus.validateJsonElement(jsonObj.get("status"));
}
if ((jsonObj.get("failureMessage") != null && !jsonObj.get("failureMessage").isJsonNull()) && !jsonObj.get("failureMessage").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `failureMessage` to be a primitive type in the JSON string but got `%s`", jsonObj.get("failureMessage").toString()));
}
if ((jsonObj.get("remainingTimeText") != null && !jsonObj.get("remainingTimeText").isJsonNull()) && !jsonObj.get("remainingTimeText").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `remainingTimeText` to be a primitive type in the JSON string but got `%s`", jsonObj.get("remainingTimeText").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!Job.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'Job' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(Job.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, Job value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public Job read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
Job instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of Job given an JSON string
*
* @param jsonString JSON string
* @return An instance of Job
* @throws IOException if the JSON string is invalid with respect to Job
*/
public static Job fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, Job.class);
}
/**
* Convert an instance of Job to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy