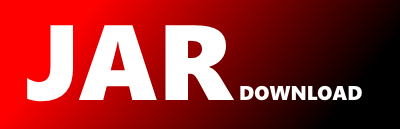
com.zuora.model.Order Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.CreateOrderResponse;
import com.zuora.model.OrderCategory;
import com.zuora.model.OrderExistingAccountDetails;
import com.zuora.model.OrderLineItem;
import com.zuora.model.OrderSchedulingOptions;
import com.zuora.model.OrderStatus;
import com.zuora.model.OrderSubscriptions;
import java.io.IOException;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* Represents the order information that will be returned in the GET call.
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class Order {
public static final String SERIALIZED_NAME_CATEGORY = "category";
@SerializedName(SERIALIZED_NAME_CATEGORY)
private OrderCategory category = OrderCategory.NEWSALES;
public static final String SERIALIZED_NAME_CREATED_BY = "createdBy";
@SerializedName(SERIALIZED_NAME_CREATED_BY)
private String createdBy;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "customFields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
private Map customFields;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_EXISTING_ACCOUNT_NUMBER = "existingAccountNumber";
@SerializedName(SERIALIZED_NAME_EXISTING_ACCOUNT_NUMBER)
private String existingAccountNumber;
public static final String SERIALIZED_NAME_EXISTING_ACCOUNT_DETAILS = "existingAccountDetails";
@SerializedName(SERIALIZED_NAME_EXISTING_ACCOUNT_DETAILS)
private OrderExistingAccountDetails existingAccountDetails;
public static final String SERIALIZED_NAME_INVOICE_SCHEDULE_ID = "invoiceScheduleId";
@SerializedName(SERIALIZED_NAME_INVOICE_SCHEDULE_ID)
private String invoiceScheduleId;
public static final String SERIALIZED_NAME_ORDER_DATE = "orderDate";
@SerializedName(SERIALIZED_NAME_ORDER_DATE)
private LocalDate orderDate;
public static final String SERIALIZED_NAME_ORDER_LINE_ITEMS = "orderLineItems";
@SerializedName(SERIALIZED_NAME_ORDER_LINE_ITEMS)
private List orderLineItems;
public static final String SERIALIZED_NAME_ORDER_NUMBER = "orderNumber";
@SerializedName(SERIALIZED_NAME_ORDER_NUMBER)
private String orderNumber;
public static final String SERIALIZED_NAME_REASON_CODE = "reasonCode";
@SerializedName(SERIALIZED_NAME_REASON_CODE)
private String reasonCode;
public static final String SERIALIZED_NAME_SCHEDULING_OPTIONS = "schedulingOptions";
@SerializedName(SERIALIZED_NAME_SCHEDULING_OPTIONS)
private OrderSchedulingOptions schedulingOptions;
public static final String SERIALIZED_NAME_SCHEDULED_ORDER_ACTIVATION_RESPONSE = "scheduledOrderActivationResponse";
@SerializedName(SERIALIZED_NAME_SCHEDULED_ORDER_ACTIVATION_RESPONSE)
private CreateOrderResponse scheduledOrderActivationResponse;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private OrderStatus status;
public static final String SERIALIZED_NAME_SUBSCRIPTIONS = "subscriptions";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTIONS)
private List subscriptions;
public static final String SERIALIZED_NAME_UPDATED_BY = "updatedBy";
@SerializedName(SERIALIZED_NAME_UPDATED_BY)
private String updatedBy;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public Order() {
}
public Order category(OrderCategory category) {
this.category = category;
return this;
}
/**
* Get category
* @return category
*/
@javax.annotation.Nullable
public OrderCategory getCategory() {
return category;
}
public void setCategory(OrderCategory category) {
this.category = category;
}
public Order createdBy(String createdBy) {
this.createdBy = createdBy;
return this;
}
/**
* The ID of the user who created this order.
* @return createdBy
*/
@javax.annotation.Nullable
public String getCreatedBy() {
return createdBy;
}
public void setCreatedBy(String createdBy) {
this.createdBy = createdBy;
}
public Order createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* The time that the order gets created in the system, in the `YYYY-MM-DD HH:MM:SS` format.
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public Order currency(String currency) {
this.currency = currency;
return this;
}
/**
* Currency code.
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public Order customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public Order putCustomFieldsItem(String key, Object customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap<>();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Container for custom fields of an Order object.
* @return customFields
*/
@javax.annotation.Nullable
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public Order description(String description) {
this.description = description;
return this;
}
/**
* A description of the order.
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Order existingAccountNumber(String existingAccountNumber) {
this.existingAccountNumber = existingAccountNumber;
return this;
}
/**
* The account number that this order has been created under. This is also the invoice owner of the subscriptions included in this order.
* @return existingAccountNumber
*/
@javax.annotation.Nullable
public String getExistingAccountNumber() {
return existingAccountNumber;
}
public void setExistingAccountNumber(String existingAccountNumber) {
this.existingAccountNumber = existingAccountNumber;
}
public Order existingAccountDetails(OrderExistingAccountDetails existingAccountDetails) {
this.existingAccountDetails = existingAccountDetails;
return this;
}
/**
* Get existingAccountDetails
* @return existingAccountDetails
*/
@javax.annotation.Nullable
public OrderExistingAccountDetails getExistingAccountDetails() {
return existingAccountDetails;
}
public void setExistingAccountDetails(OrderExistingAccountDetails existingAccountDetails) {
this.existingAccountDetails = existingAccountDetails;
}
public Order invoiceScheduleId(String invoiceScheduleId) {
this.invoiceScheduleId = invoiceScheduleId;
return this;
}
/**
* The ID of the invoice schedule associated with the order. **Note**: This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Billing_Schedule\" target=\"_blank\">Billing Schedule</a> feature in the **Early Adopter** phase enabled.
* @return invoiceScheduleId
*/
@javax.annotation.Nullable
public String getInvoiceScheduleId() {
return invoiceScheduleId;
}
public void setInvoiceScheduleId(String invoiceScheduleId) {
this.invoiceScheduleId = invoiceScheduleId;
}
public Order orderDate(LocalDate orderDate) {
this.orderDate = orderDate;
return this;
}
/**
* The date when the order is signed. All the order actions under this order will use this order date as the contract effective date if no additinal contractEffectiveDate is provided.
* @return orderDate
*/
@javax.annotation.Nullable
public LocalDate getOrderDate() {
return orderDate;
}
public void setOrderDate(LocalDate orderDate) {
this.orderDate = orderDate;
}
public Order orderLineItems(List orderLineItems) {
this.orderLineItems = orderLineItems;
return this;
}
public Order addOrderLineItemsItem(OrderLineItem orderLineItemsItem) {
if (this.orderLineItems == null) {
this.orderLineItems = new ArrayList<>();
}
this.orderLineItems.add(orderLineItemsItem);
return this;
}
/**
* [Order Line Items](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/Order_Line_Items/AA_Overview_of_Order_Line_Items) are non subscription based items created by an Order, representing transactional charges such as one-time fees, physical goods, or professional service charges that are not sold as subscription services. With the Order Line Items feature enabled, you can now launch non-subscription and unified monetization business models in Zuora, in addition to subscription business models. **Note:** The [Order Line Items](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/Order_Line_Items/AA_Overview_of_Order_Line_Items) feature is now generally available to all Zuora customers. You need to enable the [Orders](https://knowledgecenter.zuora.com/BC_Subscription_Management/Orders/AA_Overview_of_Orders#Orders) feature to access the [Order Line Items](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/Order_Line_Items/AA_Overview_of_Order_Line_Items) feature. As of Zuora Billing Release 313 (November 2021), new customers who onboard on [Orders](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/AA_Overview_of_Orders) will have the [Order Line Items](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/Order_Line_Items) feature enabled by default.
* @return orderLineItems
*/
@javax.annotation.Nullable
public List getOrderLineItems() {
return orderLineItems;
}
public void setOrderLineItems(List orderLineItems) {
this.orderLineItems = orderLineItems;
}
public Order orderNumber(String orderNumber) {
this.orderNumber = orderNumber;
return this;
}
/**
* The order number of the order.
* @return orderNumber
*/
@javax.annotation.Nullable
public String getOrderNumber() {
return orderNumber;
}
public void setOrderNumber(String orderNumber) {
this.orderNumber = orderNumber;
}
public Order reasonCode(String reasonCode) {
this.reasonCode = reasonCode;
return this;
}
/**
* Values of reason code configured in **Billing Settings** > **Configure Reason Codes** through Zuora UI. Indicates the reason when a return order line item occurs.
* @return reasonCode
*/
@javax.annotation.Nullable
public String getReasonCode() {
return reasonCode;
}
public void setReasonCode(String reasonCode) {
this.reasonCode = reasonCode;
}
public Order schedulingOptions(OrderSchedulingOptions schedulingOptions) {
this.schedulingOptions = schedulingOptions;
return this;
}
/**
* Get schedulingOptions
* @return schedulingOptions
*/
@javax.annotation.Nullable
public OrderSchedulingOptions getSchedulingOptions() {
return schedulingOptions;
}
public void setSchedulingOptions(OrderSchedulingOptions schedulingOptions) {
this.schedulingOptions = schedulingOptions;
}
public Order scheduledOrderActivationResponse(CreateOrderResponse scheduledOrderActivationResponse) {
this.scheduledOrderActivationResponse = scheduledOrderActivationResponse;
return this;
}
/**
* Get scheduledOrderActivationResponse
* @return scheduledOrderActivationResponse
*/
@javax.annotation.Nullable
public CreateOrderResponse getScheduledOrderActivationResponse() {
return scheduledOrderActivationResponse;
}
public void setScheduledOrderActivationResponse(CreateOrderResponse scheduledOrderActivationResponse) {
this.scheduledOrderActivationResponse = scheduledOrderActivationResponse;
}
public Order status(OrderStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public OrderStatus getStatus() {
return status;
}
public void setStatus(OrderStatus status) {
this.status = status;
}
public Order subscriptions(List subscriptions) {
this.subscriptions = subscriptions;
return this;
}
public Order addSubscriptionsItem(OrderSubscriptions subscriptionsItem) {
if (this.subscriptions == null) {
this.subscriptions = new ArrayList<>();
}
this.subscriptions.add(subscriptionsItem);
return this;
}
/**
* Represents a processed subscription, including the origin request (order actions) that create this version of subscription and the processing result (order metrics). The reference part in the request will be overridden with the info in the new subscription version.
* @return subscriptions
*/
@javax.annotation.Nullable
public List getSubscriptions() {
return subscriptions;
}
public void setSubscriptions(List subscriptions) {
this.subscriptions = subscriptions;
}
public Order updatedBy(String updatedBy) {
this.updatedBy = updatedBy;
return this;
}
/**
* The ID of the user who updated this order.
* @return updatedBy
*/
@javax.annotation.Nullable
public String getUpdatedBy() {
return updatedBy;
}
public void setUpdatedBy(String updatedBy) {
this.updatedBy = updatedBy;
}
public Order updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* The time that the order gets updated in the system(for example, an order description update), in the `YYYY-MM-DD HH:MM:SS` format.
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the Order instance itself
*/
public Order putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Order order = (Order) o;
return Objects.equals(this.category, order.category) &&
Objects.equals(this.createdBy, order.createdBy) &&
Objects.equals(this.createdDate, order.createdDate) &&
Objects.equals(this.currency, order.currency) &&
Objects.equals(this.customFields, order.customFields) &&
Objects.equals(this.description, order.description) &&
Objects.equals(this.existingAccountNumber, order.existingAccountNumber) &&
Objects.equals(this.existingAccountDetails, order.existingAccountDetails) &&
Objects.equals(this.invoiceScheduleId, order.invoiceScheduleId) &&
Objects.equals(this.orderDate, order.orderDate) &&
Objects.equals(this.orderLineItems, order.orderLineItems) &&
Objects.equals(this.orderNumber, order.orderNumber) &&
Objects.equals(this.reasonCode, order.reasonCode) &&
Objects.equals(this.schedulingOptions, order.schedulingOptions) &&
Objects.equals(this.scheduledOrderActivationResponse, order.scheduledOrderActivationResponse) &&
Objects.equals(this.status, order.status) &&
Objects.equals(this.subscriptions, order.subscriptions) &&
Objects.equals(this.updatedBy, order.updatedBy) &&
Objects.equals(this.updatedDate, order.updatedDate)&&
Objects.equals(this.additionalProperties, order.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(category, createdBy, createdDate, currency, customFields, description, existingAccountNumber, existingAccountDetails, invoiceScheduleId, orderDate, orderLineItems, orderNumber, reasonCode, schedulingOptions, scheduledOrderActivationResponse, status, subscriptions, updatedBy, updatedDate, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Order {\n");
sb.append(" category: ").append(toIndentedString(category)).append("\n");
sb.append(" createdBy: ").append(toIndentedString(createdBy)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" existingAccountNumber: ").append(toIndentedString(existingAccountNumber)).append("\n");
sb.append(" existingAccountDetails: ").append(toIndentedString(existingAccountDetails)).append("\n");
sb.append(" invoiceScheduleId: ").append(toIndentedString(invoiceScheduleId)).append("\n");
sb.append(" orderDate: ").append(toIndentedString(orderDate)).append("\n");
sb.append(" orderLineItems: ").append(toIndentedString(orderLineItems)).append("\n");
sb.append(" orderNumber: ").append(toIndentedString(orderNumber)).append("\n");
sb.append(" reasonCode: ").append(toIndentedString(reasonCode)).append("\n");
sb.append(" schedulingOptions: ").append(toIndentedString(schedulingOptions)).append("\n");
sb.append(" scheduledOrderActivationResponse: ").append(toIndentedString(scheduledOrderActivationResponse)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" subscriptions: ").append(toIndentedString(subscriptions)).append("\n");
sb.append(" updatedBy: ").append(toIndentedString(updatedBy)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("category");
openapiFields.add("createdBy");
openapiFields.add("createdDate");
openapiFields.add("currency");
openapiFields.add("customFields");
openapiFields.add("description");
openapiFields.add("existingAccountNumber");
openapiFields.add("existingAccountDetails");
openapiFields.add("invoiceScheduleId");
openapiFields.add("orderDate");
openapiFields.add("orderLineItems");
openapiFields.add("orderNumber");
openapiFields.add("reasonCode");
openapiFields.add("schedulingOptions");
openapiFields.add("scheduledOrderActivationResponse");
openapiFields.add("status");
openapiFields.add("subscriptions");
openapiFields.add("updatedBy");
openapiFields.add("updatedDate");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to Order
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!Order.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in Order is not found in the empty JSON string", Order.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
// validate the optional field `category`
if (jsonObj.get("category") != null && !jsonObj.get("category").isJsonNull()) {
OrderCategory.validateJsonElement(jsonObj.get("category"));
}
if ((jsonObj.get("createdBy") != null && !jsonObj.get("createdBy").isJsonNull()) && !jsonObj.get("createdBy").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdBy` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdBy").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if ((jsonObj.get("existingAccountNumber") != null && !jsonObj.get("existingAccountNumber").isJsonNull()) && !jsonObj.get("existingAccountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `existingAccountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("existingAccountNumber").toString()));
}
// validate the optional field `existingAccountDetails`
if (jsonObj.get("existingAccountDetails") != null && !jsonObj.get("existingAccountDetails").isJsonNull()) {
OrderExistingAccountDetails.validateJsonElement(jsonObj.get("existingAccountDetails"));
}
if ((jsonObj.get("invoiceScheduleId") != null && !jsonObj.get("invoiceScheduleId").isJsonNull()) && !jsonObj.get("invoiceScheduleId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceScheduleId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceScheduleId").toString()));
}
if (jsonObj.get("orderLineItems") != null && !jsonObj.get("orderLineItems").isJsonNull()) {
JsonArray jsonArrayorderLineItems = jsonObj.getAsJsonArray("orderLineItems");
if (jsonArrayorderLineItems != null) {
// ensure the json data is an array
if (!jsonObj.get("orderLineItems").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `orderLineItems` to be an array in the JSON string but got `%s`", jsonObj.get("orderLineItems").toString()));
}
// validate the optional field `orderLineItems` (array)
for (int i = 0; i < jsonArrayorderLineItems.size(); i++) {
OrderLineItem.validateJsonElement(jsonArrayorderLineItems.get(i));
};
}
}
if ((jsonObj.get("orderNumber") != null && !jsonObj.get("orderNumber").isJsonNull()) && !jsonObj.get("orderNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `orderNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("orderNumber").toString()));
}
if ((jsonObj.get("reasonCode") != null && !jsonObj.get("reasonCode").isJsonNull()) && !jsonObj.get("reasonCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `reasonCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("reasonCode").toString()));
}
// validate the optional field `schedulingOptions`
if (jsonObj.get("schedulingOptions") != null && !jsonObj.get("schedulingOptions").isJsonNull()) {
OrderSchedulingOptions.validateJsonElement(jsonObj.get("schedulingOptions"));
}
// validate the optional field `scheduledOrderActivationResponse`
if (jsonObj.get("scheduledOrderActivationResponse") != null && !jsonObj.get("scheduledOrderActivationResponse").isJsonNull()) {
CreateOrderResponse.validateJsonElement(jsonObj.get("scheduledOrderActivationResponse"));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
// validate the optional field `status`
if (jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) {
OrderStatus.validateJsonElement(jsonObj.get("status"));
}
if (jsonObj.get("subscriptions") != null && !jsonObj.get("subscriptions").isJsonNull()) {
JsonArray jsonArraysubscriptions = jsonObj.getAsJsonArray("subscriptions");
if (jsonArraysubscriptions != null) {
// ensure the json data is an array
if (!jsonObj.get("subscriptions").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptions` to be an array in the JSON string but got `%s`", jsonObj.get("subscriptions").toString()));
}
// validate the optional field `subscriptions` (array)
for (int i = 0; i < jsonArraysubscriptions.size(); i++) {
OrderSubscriptions.validateJsonElement(jsonArraysubscriptions.get(i));
};
}
}
if ((jsonObj.get("updatedBy") != null && !jsonObj.get("updatedBy").isJsonNull()) && !jsonObj.get("updatedBy").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedBy` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedBy").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!Order.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'Order' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(Order.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, Order value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public Order read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
Order instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of Order given an JSON string
*
* @param jsonString JSON string
* @return An instance of Order
* @throws IOException if the JSON string is invalid with respect to Order
*/
public static Order fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, Order.class);
}
/**
* Convert an instance of Order to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy