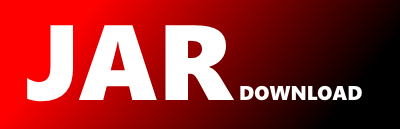
com.zuora.model.OrderAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.OrderActionAddProduct;
import com.zuora.model.OrderActionCancelSubscription;
import com.zuora.model.OrderActionChangePlan;
import com.zuora.model.OrderActionCreateSubscription;
import com.zuora.model.OrderActionOwnerTransfer;
import com.zuora.model.OrderActionRemoveProduct;
import com.zuora.model.OrderActionRenewSubscription;
import com.zuora.model.OrderActionResume;
import com.zuora.model.OrderActionSuspend;
import com.zuora.model.OrderActionTermsAndConditions;
import com.zuora.model.OrderActionType;
import com.zuora.model.OrderActionUpdateProduct;
import com.zuora.model.OrderItem;
import com.zuora.model.OrderMetric;
import com.zuora.model.TriggerDate;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* Represents the processed order action.
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class OrderAction {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_CREATE_SUBSCRIPTION = "createSubscription";
@SerializedName(SERIALIZED_NAME_CREATE_SUBSCRIPTION)
private OrderActionCreateSubscription createSubscription;
public static final String SERIALIZED_NAME_ADD_PRODUCT = "addProduct";
@SerializedName(SERIALIZED_NAME_ADD_PRODUCT)
private OrderActionAddProduct addProduct;
public static final String SERIALIZED_NAME_UPDATE_PRODUCT = "updateProduct";
@SerializedName(SERIALIZED_NAME_UPDATE_PRODUCT)
private OrderActionUpdateProduct updateProduct;
public static final String SERIALIZED_NAME_CANCEL_SUBSCRIPTION = "cancelSubscription";
@SerializedName(SERIALIZED_NAME_CANCEL_SUBSCRIPTION)
private OrderActionCancelSubscription cancelSubscription;
public static final String SERIALIZED_NAME_CHANGE_PLAN = "changePlan";
@SerializedName(SERIALIZED_NAME_CHANGE_PLAN)
private OrderActionChangePlan changePlan;
public static final String SERIALIZED_NAME_OWNER_TRANSFER = "ownerTransfer";
@SerializedName(SERIALIZED_NAME_OWNER_TRANSFER)
private OrderActionOwnerTransfer ownerTransfer;
public static final String SERIALIZED_NAME_REMOVE_PRODUCT = "removeProduct";
@SerializedName(SERIALIZED_NAME_REMOVE_PRODUCT)
private OrderActionRemoveProduct removeProduct;
public static final String SERIALIZED_NAME_RENEW_SUBSCRIPTION = "renewSubscription";
@SerializedName(SERIALIZED_NAME_RENEW_SUBSCRIPTION)
private OrderActionRenewSubscription renewSubscription;
public static final String SERIALIZED_NAME_SUSPEND = "suspend";
@SerializedName(SERIALIZED_NAME_SUSPEND)
private OrderActionSuspend suspend;
public static final String SERIALIZED_NAME_RESUME = "resume";
@SerializedName(SERIALIZED_NAME_RESUME)
private OrderActionResume resume;
public static final String SERIALIZED_NAME_TERMS_AND_CONDITIONS = "termsAndConditions";
@SerializedName(SERIALIZED_NAME_TERMS_AND_CONDITIONS)
private OrderActionTermsAndConditions termsAndConditions;
public static final String SERIALIZED_NAME_CHANGE_REASON = "changeReason";
@SerializedName(SERIALIZED_NAME_CHANGE_REASON)
private String changeReason;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "customFields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
private Map customFields;
public static final String SERIALIZED_NAME_SEQUENCE = "sequence";
@SerializedName(SERIALIZED_NAME_SEQUENCE)
private Integer sequence;
public static final String SERIALIZED_NAME_ORDER_ITEMS = "orderItems";
@SerializedName(SERIALIZED_NAME_ORDER_ITEMS)
private List orderItems;
public static final String SERIALIZED_NAME_ORDER_METRICS = "orderMetrics";
@SerializedName(SERIALIZED_NAME_ORDER_METRICS)
private List orderMetrics;
public static final String SERIALIZED_NAME_TRIGGER_DATES = "triggerDates";
@SerializedName(SERIALIZED_NAME_TRIGGER_DATES)
private List triggerDates;
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private OrderActionType type;
public OrderAction() {
}
public OrderAction id(String id) {
this.id = id;
return this;
}
/**
* The Id of the order action processed in the order.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public OrderAction createSubscription(OrderActionCreateSubscription createSubscription) {
this.createSubscription = createSubscription;
return this;
}
/**
* Get createSubscription
* @return createSubscription
*/
@javax.annotation.Nullable
public OrderActionCreateSubscription getCreateSubscription() {
return createSubscription;
}
public void setCreateSubscription(OrderActionCreateSubscription createSubscription) {
this.createSubscription = createSubscription;
}
public OrderAction addProduct(OrderActionAddProduct addProduct) {
this.addProduct = addProduct;
return this;
}
/**
* Get addProduct
* @return addProduct
*/
@javax.annotation.Nullable
public OrderActionAddProduct getAddProduct() {
return addProduct;
}
public void setAddProduct(OrderActionAddProduct addProduct) {
this.addProduct = addProduct;
}
public OrderAction updateProduct(OrderActionUpdateProduct updateProduct) {
this.updateProduct = updateProduct;
return this;
}
/**
* Get updateProduct
* @return updateProduct
*/
@javax.annotation.Nullable
public OrderActionUpdateProduct getUpdateProduct() {
return updateProduct;
}
public void setUpdateProduct(OrderActionUpdateProduct updateProduct) {
this.updateProduct = updateProduct;
}
public OrderAction cancelSubscription(OrderActionCancelSubscription cancelSubscription) {
this.cancelSubscription = cancelSubscription;
return this;
}
/**
* Get cancelSubscription
* @return cancelSubscription
*/
@javax.annotation.Nullable
public OrderActionCancelSubscription getCancelSubscription() {
return cancelSubscription;
}
public void setCancelSubscription(OrderActionCancelSubscription cancelSubscription) {
this.cancelSubscription = cancelSubscription;
}
public OrderAction changePlan(OrderActionChangePlan changePlan) {
this.changePlan = changePlan;
return this;
}
/**
* Get changePlan
* @return changePlan
*/
@javax.annotation.Nullable
public OrderActionChangePlan getChangePlan() {
return changePlan;
}
public void setChangePlan(OrderActionChangePlan changePlan) {
this.changePlan = changePlan;
}
public OrderAction ownerTransfer(OrderActionOwnerTransfer ownerTransfer) {
this.ownerTransfer = ownerTransfer;
return this;
}
/**
* Get ownerTransfer
* @return ownerTransfer
*/
@javax.annotation.Nullable
public OrderActionOwnerTransfer getOwnerTransfer() {
return ownerTransfer;
}
public void setOwnerTransfer(OrderActionOwnerTransfer ownerTransfer) {
this.ownerTransfer = ownerTransfer;
}
public OrderAction removeProduct(OrderActionRemoveProduct removeProduct) {
this.removeProduct = removeProduct;
return this;
}
/**
* Get removeProduct
* @return removeProduct
*/
@javax.annotation.Nullable
public OrderActionRemoveProduct getRemoveProduct() {
return removeProduct;
}
public void setRemoveProduct(OrderActionRemoveProduct removeProduct) {
this.removeProduct = removeProduct;
}
public OrderAction renewSubscription(OrderActionRenewSubscription renewSubscription) {
this.renewSubscription = renewSubscription;
return this;
}
/**
* Get renewSubscription
* @return renewSubscription
*/
@javax.annotation.Nullable
public OrderActionRenewSubscription getRenewSubscription() {
return renewSubscription;
}
public void setRenewSubscription(OrderActionRenewSubscription renewSubscription) {
this.renewSubscription = renewSubscription;
}
public OrderAction suspend(OrderActionSuspend suspend) {
this.suspend = suspend;
return this;
}
/**
* Get suspend
* @return suspend
*/
@javax.annotation.Nullable
public OrderActionSuspend getSuspend() {
return suspend;
}
public void setSuspend(OrderActionSuspend suspend) {
this.suspend = suspend;
}
public OrderAction resume(OrderActionResume resume) {
this.resume = resume;
return this;
}
/**
* Get resume
* @return resume
*/
@javax.annotation.Nullable
public OrderActionResume getResume() {
return resume;
}
public void setResume(OrderActionResume resume) {
this.resume = resume;
}
public OrderAction termsAndConditions(OrderActionTermsAndConditions termsAndConditions) {
this.termsAndConditions = termsAndConditions;
return this;
}
/**
* Get termsAndConditions
* @return termsAndConditions
*/
@javax.annotation.Nullable
public OrderActionTermsAndConditions getTermsAndConditions() {
return termsAndConditions;
}
public void setTermsAndConditions(OrderActionTermsAndConditions termsAndConditions) {
this.termsAndConditions = termsAndConditions;
}
public OrderAction changeReason(String changeReason) {
this.changeReason = changeReason;
return this;
}
/**
* The change reason set for an order action when an order is created.
* @return changeReason
*/
@javax.annotation.Nullable
public String getChangeReason() {
return changeReason;
}
public void setChangeReason(String changeReason) {
this.changeReason = changeReason;
}
public OrderAction customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public OrderAction putCustomFieldsItem(String key, Object customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap<>();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Container for custom fields of an Order Action object.
* @return customFields
*/
@javax.annotation.Nullable
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public OrderAction sequence(Integer sequence) {
this.sequence = sequence;
return this;
}
/**
* The sequence of the order actions processed in the order.
* @return sequence
*/
@javax.annotation.Nullable
public Integer getSequence() {
return sequence;
}
public void setSequence(Integer sequence) {
this.sequence = sequence;
}
public OrderAction orderItems(List orderItems) {
this.orderItems = orderItems;
return this;
}
public OrderAction addOrderItemsItem(OrderItem orderItemsItem) {
if (this.orderItems == null) {
this.orderItems = new ArrayList<>();
}
this.orderItems.add(orderItemsItem);
return this;
}
/**
* The `orderItems` nested field is only available to existing Orders customers who already have access to the field. **Note:** The following Order Metrics have been deprecated. Any new customers who onboard on [Orders](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/AA_Overview_of_Orders) or [Orders Harmonization](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/Orders_Harmonization/Orders_Harmonization) will not get these metrics. * The Order ELP and Order Item objects * The \"Generated Reason\" and \"Order Item ID\" fields in the Order MRR, Order TCB, Order TCV, and Order Quantity objects Existing Orders customers who have these metrics will continue to be supported.
* @return orderItems
*/
@javax.annotation.Nullable
public List getOrderItems() {
return orderItems;
}
public void setOrderItems(List orderItems) {
this.orderItems = orderItems;
}
public OrderAction orderMetrics(List orderMetrics) {
this.orderMetrics = orderMetrics;
return this;
}
public OrderAction addOrderMetricsItem(OrderMetric orderMetricsItem) {
if (this.orderMetrics == null) {
this.orderMetrics = new ArrayList<>();
}
this.orderMetrics.add(orderMetricsItem);
return this;
}
/**
* The container for order metrics. **Note:** The following Order Metrics have been deprecated. Any new customers who onboard on [Orders](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/AA_Overview_of_Orders) or [Orders Harmonization](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/Orders_Harmonization/Orders_Harmonization) will not get these metrics. * The Order ELP and Order Item objects * The \"Generated Reason\" and \"Order Item ID\" fields in the Order MRR, Order TCB, Order TCV, and Order Quantity objects Existing Orders customers who have these metrics will continue to be supported. **Note:** As of Zuora Billing Release 306, Zuora has upgraded the methodologies for calculating metrics in [Orders](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders). The new methodologies are reflected in the following Order Delta Metrics objects. * [Order Delta Mrr](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/Order_Delta_Metrics/Order_Delta_Mrr) * [Order Delta Tcv](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/Order_Delta_Metrics/Order_Delta_Tcv) * [Order Delta Tcb](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/Order_Delta_Metrics/Order_Delta_Tcb) It is recommended that all customers use the new [Order Delta Metrics](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/Order_Delta_Metrics/AA_Overview_of_Order_Delta_Metrics). If you are an existing [Order Metrics](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/AA_Overview_of_Orders/Key_Metrics_for_Orders) customer and want to migrate to Order Delta Metrics, submit a request at [Zuora Global Support](https://support.zuora.com/). Whereas new customers, and existing customers not currently on [Order Metrics](https://knowledgecenter.zuora.com/Billing/Subscriptions/Orders/AA_Overview_of_Orders/Key_Metrics_for_Orders), will no longer have access to Order Metrics, existing customers currently using Order Metrics will continue to be supported.
* @return orderMetrics
*/
@javax.annotation.Nullable
public List getOrderMetrics() {
return orderMetrics;
}
public void setOrderMetrics(List orderMetrics) {
this.orderMetrics = orderMetrics;
}
public OrderAction triggerDates(List triggerDates) {
this.triggerDates = triggerDates;
return this;
}
public OrderAction addTriggerDatesItem(TriggerDate triggerDatesItem) {
if (this.triggerDates == null) {
this.triggerDates = new ArrayList<>();
}
this.triggerDates.add(triggerDatesItem);
return this;
}
/**
* Container for the contract effective, service activation, and customer acceptance dates of the order action. If [Zuora is configured to require service activation](https://knowledgecenter.zuora.com/CB_Billing/Billing_Settings/Define_Default_Subscription_Settings#Require_Service_Activation_of_Orders.3F) and the `ServiceActivation` field is not set for a `CreateSubscription` order action, a `Pending` order and a `Pending Activation` subscription are created. If [Zuora is configured to require customer acceptance](https://knowledgecenter.zuora.com/CB_Billing/Billing_Settings/Define_Default_Subscription_Settings#Require_Customer_Acceptance_of_Orders.3F) and the `CustomerAcceptance` field is not set for a `CreateSubscription` order action, a `Pending` order and a `Pending Acceptance` subscription are created. At the same time, if the service activation date field is also required and not set, a `Pending` order and a `Pending Activation` subscription are created instead. If [Zuora is configured to require service activation](https://knowledgecenter.zuora.com/CB_Billing/Billing_Settings/Define_Default_Subscription_Settings#Require_Service_Activation_of_Orders.3F) and the `ServiceActivation` field is not set for either of the following order actions, a `Pending` order is created. The subscription status is not impacted. **Note:** This feature is in **Limited Availability**. If you want to have access to the feature, submit a request at [Zuora Global Support](http://support.zuora.com/). * AddProduct * UpdateProduct * RemoveProduct * RenewSubscription * TermsAndConditions If [Zuora is configured to require customer acceptance](https://knowledgecenter.zuora.com/CB_Billing/Billing_Settings/Define_Default_Subscription_Settings#Require_Customer_Acceptance_of_Orders.3F) and the `CustomerAcceptance` field is not set for either of the following order actions, a `Pending` order is created. The subscription status is not impacted. **Note:** This feature is in **Limited Availability**. If you want to have access to the feature, submit a request at [Zuora Global Support](http://support.zuora.com/). * AddProduct * UpdateProduct * RemoveProduct * RenewSubscription * TermsAndConditions
* @return triggerDates
*/
@javax.annotation.Nullable
public List getTriggerDates() {
return triggerDates;
}
public void setTriggerDates(List triggerDates) {
this.triggerDates = triggerDates;
}
public OrderAction type(OrderActionType type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
*/
@javax.annotation.Nullable
public OrderActionType getType() {
return type;
}
public void setType(OrderActionType type) {
this.type = type;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the OrderAction instance itself
*/
public OrderAction putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
OrderAction orderAction = (OrderAction) o;
return Objects.equals(this.id, orderAction.id) &&
Objects.equals(this.createSubscription, orderAction.createSubscription) &&
Objects.equals(this.addProduct, orderAction.addProduct) &&
Objects.equals(this.updateProduct, orderAction.updateProduct) &&
Objects.equals(this.cancelSubscription, orderAction.cancelSubscription) &&
Objects.equals(this.changePlan, orderAction.changePlan) &&
Objects.equals(this.ownerTransfer, orderAction.ownerTransfer) &&
Objects.equals(this.removeProduct, orderAction.removeProduct) &&
Objects.equals(this.renewSubscription, orderAction.renewSubscription) &&
Objects.equals(this.suspend, orderAction.suspend) &&
Objects.equals(this.resume, orderAction.resume) &&
Objects.equals(this.termsAndConditions, orderAction.termsAndConditions) &&
Objects.equals(this.changeReason, orderAction.changeReason) &&
Objects.equals(this.customFields, orderAction.customFields) &&
Objects.equals(this.sequence, orderAction.sequence) &&
Objects.equals(this.orderItems, orderAction.orderItems) &&
Objects.equals(this.orderMetrics, orderAction.orderMetrics) &&
Objects.equals(this.triggerDates, orderAction.triggerDates) &&
Objects.equals(this.type, orderAction.type)&&
Objects.equals(this.additionalProperties, orderAction.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(id, createSubscription, addProduct, updateProduct, cancelSubscription, changePlan, ownerTransfer, removeProduct, renewSubscription, suspend, resume, termsAndConditions, changeReason, customFields, sequence, orderItems, orderMetrics, triggerDates, type, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class OrderAction {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" createSubscription: ").append(toIndentedString(createSubscription)).append("\n");
sb.append(" addProduct: ").append(toIndentedString(addProduct)).append("\n");
sb.append(" updateProduct: ").append(toIndentedString(updateProduct)).append("\n");
sb.append(" cancelSubscription: ").append(toIndentedString(cancelSubscription)).append("\n");
sb.append(" changePlan: ").append(toIndentedString(changePlan)).append("\n");
sb.append(" ownerTransfer: ").append(toIndentedString(ownerTransfer)).append("\n");
sb.append(" removeProduct: ").append(toIndentedString(removeProduct)).append("\n");
sb.append(" renewSubscription: ").append(toIndentedString(renewSubscription)).append("\n");
sb.append(" suspend: ").append(toIndentedString(suspend)).append("\n");
sb.append(" resume: ").append(toIndentedString(resume)).append("\n");
sb.append(" termsAndConditions: ").append(toIndentedString(termsAndConditions)).append("\n");
sb.append(" changeReason: ").append(toIndentedString(changeReason)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" sequence: ").append(toIndentedString(sequence)).append("\n");
sb.append(" orderItems: ").append(toIndentedString(orderItems)).append("\n");
sb.append(" orderMetrics: ").append(toIndentedString(orderMetrics)).append("\n");
sb.append(" triggerDates: ").append(toIndentedString(triggerDates)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("id");
openapiFields.add("createSubscription");
openapiFields.add("addProduct");
openapiFields.add("updateProduct");
openapiFields.add("cancelSubscription");
openapiFields.add("changePlan");
openapiFields.add("ownerTransfer");
openapiFields.add("removeProduct");
openapiFields.add("renewSubscription");
openapiFields.add("suspend");
openapiFields.add("resume");
openapiFields.add("termsAndConditions");
openapiFields.add("changeReason");
openapiFields.add("customFields");
openapiFields.add("sequence");
openapiFields.add("orderItems");
openapiFields.add("orderMetrics");
openapiFields.add("triggerDates");
openapiFields.add("type");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to OrderAction
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!OrderAction.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in OrderAction is not found in the empty JSON string", OrderAction.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
// validate the optional field `createSubscription`
if (jsonObj.get("createSubscription") != null && !jsonObj.get("createSubscription").isJsonNull()) {
OrderActionCreateSubscription.validateJsonElement(jsonObj.get("createSubscription"));
}
// validate the optional field `addProduct`
if (jsonObj.get("addProduct") != null && !jsonObj.get("addProduct").isJsonNull()) {
OrderActionAddProduct.validateJsonElement(jsonObj.get("addProduct"));
}
// validate the optional field `updateProduct`
if (jsonObj.get("updateProduct") != null && !jsonObj.get("updateProduct").isJsonNull()) {
OrderActionUpdateProduct.validateJsonElement(jsonObj.get("updateProduct"));
}
// validate the optional field `cancelSubscription`
if (jsonObj.get("cancelSubscription") != null && !jsonObj.get("cancelSubscription").isJsonNull()) {
OrderActionCancelSubscription.validateJsonElement(jsonObj.get("cancelSubscription"));
}
// validate the optional field `changePlan`
if (jsonObj.get("changePlan") != null && !jsonObj.get("changePlan").isJsonNull()) {
OrderActionChangePlan.validateJsonElement(jsonObj.get("changePlan"));
}
// validate the optional field `ownerTransfer`
if (jsonObj.get("ownerTransfer") != null && !jsonObj.get("ownerTransfer").isJsonNull()) {
OrderActionOwnerTransfer.validateJsonElement(jsonObj.get("ownerTransfer"));
}
// validate the optional field `removeProduct`
if (jsonObj.get("removeProduct") != null && !jsonObj.get("removeProduct").isJsonNull()) {
OrderActionRemoveProduct.validateJsonElement(jsonObj.get("removeProduct"));
}
// validate the optional field `renewSubscription`
if (jsonObj.get("renewSubscription") != null && !jsonObj.get("renewSubscription").isJsonNull()) {
OrderActionRenewSubscription.validateJsonElement(jsonObj.get("renewSubscription"));
}
// validate the optional field `suspend`
if (jsonObj.get("suspend") != null && !jsonObj.get("suspend").isJsonNull()) {
OrderActionSuspend.validateJsonElement(jsonObj.get("suspend"));
}
// validate the optional field `resume`
if (jsonObj.get("resume") != null && !jsonObj.get("resume").isJsonNull()) {
OrderActionResume.validateJsonElement(jsonObj.get("resume"));
}
// validate the optional field `termsAndConditions`
if (jsonObj.get("termsAndConditions") != null && !jsonObj.get("termsAndConditions").isJsonNull()) {
OrderActionTermsAndConditions.validateJsonElement(jsonObj.get("termsAndConditions"));
}
if ((jsonObj.get("changeReason") != null && !jsonObj.get("changeReason").isJsonNull()) && !jsonObj.get("changeReason").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `changeReason` to be a primitive type in the JSON string but got `%s`", jsonObj.get("changeReason").toString()));
}
if (jsonObj.get("orderItems") != null && !jsonObj.get("orderItems").isJsonNull()) {
JsonArray jsonArrayorderItems = jsonObj.getAsJsonArray("orderItems");
if (jsonArrayorderItems != null) {
// ensure the json data is an array
if (!jsonObj.get("orderItems").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `orderItems` to be an array in the JSON string but got `%s`", jsonObj.get("orderItems").toString()));
}
// validate the optional field `orderItems` (array)
for (int i = 0; i < jsonArrayorderItems.size(); i++) {
OrderItem.validateJsonElement(jsonArrayorderItems.get(i));
};
}
}
if (jsonObj.get("orderMetrics") != null && !jsonObj.get("orderMetrics").isJsonNull()) {
JsonArray jsonArrayorderMetrics = jsonObj.getAsJsonArray("orderMetrics");
if (jsonArrayorderMetrics != null) {
// ensure the json data is an array
if (!jsonObj.get("orderMetrics").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `orderMetrics` to be an array in the JSON string but got `%s`", jsonObj.get("orderMetrics").toString()));
}
// validate the optional field `orderMetrics` (array)
for (int i = 0; i < jsonArrayorderMetrics.size(); i++) {
OrderMetric.validateJsonElement(jsonArrayorderMetrics.get(i));
};
}
}
if (jsonObj.get("triggerDates") != null && !jsonObj.get("triggerDates").isJsonNull()) {
JsonArray jsonArraytriggerDates = jsonObj.getAsJsonArray("triggerDates");
if (jsonArraytriggerDates != null) {
// ensure the json data is an array
if (!jsonObj.get("triggerDates").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `triggerDates` to be an array in the JSON string but got `%s`", jsonObj.get("triggerDates").toString()));
}
// validate the optional field `triggerDates` (array)
for (int i = 0; i < jsonArraytriggerDates.size(); i++) {
TriggerDate.validateJsonElement(jsonArraytriggerDates.get(i));
};
}
}
if ((jsonObj.get("type") != null && !jsonObj.get("type").isJsonNull()) && !jsonObj.get("type").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `type` to be a primitive type in the JSON string but got `%s`", jsonObj.get("type").toString()));
}
// validate the optional field `type`
if (jsonObj.get("type") != null && !jsonObj.get("type").isJsonNull()) {
OrderActionType.validateJsonElement(jsonObj.get("type"));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!OrderAction.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'OrderAction' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(OrderAction.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, OrderAction value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public OrderAction read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
OrderAction instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of OrderAction given an JSON string
*
* @param jsonString JSON string
* @return An instance of OrderAction
* @throws IOException if the JSON string is invalid with respect to OrderAction
*/
public static OrderAction fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, OrderAction.class);
}
/**
* Convert an instance of OrderAction to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy