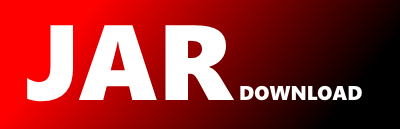
com.zuora.model.PaymentScheduleItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.PaymentScheduleBillingDocument;
import com.zuora.model.PaymentScheduleLinkedPaymentID;
import com.zuora.model.PaymentSchedulePaymentOptionFields;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* PaymentScheduleItem
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class PaymentScheduleItem {
public static final String SERIALIZED_NAME_ACCOUNT_ID = "accountId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_BALANCE = "balance";
@SerializedName(SERIALIZED_NAME_BALANCE)
private BigDecimal balance;
public static final String SERIALIZED_NAME_BILLING_DOCUMENT = "billingDocument";
@SerializedName(SERIALIZED_NAME_BILLING_DOCUMENT)
private PaymentScheduleBillingDocument billingDocument;
public static final String SERIALIZED_NAME_CANCELLATION_REASON = "cancellationReason";
@SerializedName(SERIALIZED_NAME_CANCELLATION_REASON)
private String cancellationReason;
public static final String SERIALIZED_NAME_CANCELLED_BY_ID = "cancelledById";
@SerializedName(SERIALIZED_NAME_CANCELLED_BY_ID)
private String cancelledById;
public static final String SERIALIZED_NAME_CANCELLED_ON = "cancelledOn";
@SerializedName(SERIALIZED_NAME_CANCELLED_ON)
private String cancelledOn;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_ERROR_MESSAGE = "errorMessage";
@SerializedName(SERIALIZED_NAME_ERROR_MESSAGE)
private String errorMessage;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_NUMBER = "number";
@SerializedName(SERIALIZED_NAME_NUMBER)
private String number;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY_ID = "paymentGatewayId";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY_ID)
private String paymentGatewayId;
public static final String SERIALIZED_NAME_PAYMENT_ID = "paymentId";
@Deprecated
@SerializedName(SERIALIZED_NAME_PAYMENT_ID)
private String paymentId;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "paymentMethodId";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_PAYMENT_OPTION = "paymentOption";
@SerializedName(SERIALIZED_NAME_PAYMENT_OPTION)
private List paymentOption;
public static final String SERIALIZED_NAME_PAYMENT_SCHEDULE_ID = "paymentScheduleId";
@SerializedName(SERIALIZED_NAME_PAYMENT_SCHEDULE_ID)
private String paymentScheduleId;
public static final String SERIALIZED_NAME_PAYMENT_SCHEDULE_NUMBER = "paymentScheduleNumber";
@SerializedName(SERIALIZED_NAME_PAYMENT_SCHEDULE_NUMBER)
private String paymentScheduleNumber;
public static final String SERIALIZED_NAME_PSI_PAYMENTS = "psiPayments";
@SerializedName(SERIALIZED_NAME_PSI_PAYMENTS)
private List psiPayments;
public static final String SERIALIZED_NAME_RUN_HOUR = "runHour";
@SerializedName(SERIALIZED_NAME_RUN_HOUR)
private Integer runHour;
public static final String SERIALIZED_NAME_SCHEDULED_DATE = "scheduledDate";
@SerializedName(SERIALIZED_NAME_SCHEDULED_DATE)
private String scheduledDate;
public static final String SERIALIZED_NAME_STANDALONE = "standalone";
@SerializedName(SERIALIZED_NAME_STANDALONE)
private Boolean standalone;
/**
* ID of the payment method of the payment schedule item. - `Pending`: Payment schedule item is waiting for processing. - `Processed`: The payment has been collected. - `Error`: Failed to collect the payment. - `Canceled`: After a pending payment schedule item is canceled by the user, the item is marked as `Canceled`.
*/
@JsonAdapter(StatusEnum.Adapter.class)
public enum StatusEnum {
PENDING("Pending"),
PROCESSED("Processed"),
ERROR("Error"),
CANCELED("Canceled");
private String value;
StatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StatusEnum fromValue(String value) {
for (StatusEnum b : StatusEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StatusEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StatusEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StatusEnum.fromValue(value);
}
}
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
String value = jsonElement.getAsString();
StatusEnum.fromValue(value);
}
}
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private StatusEnum status;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public PaymentScheduleItem() {
}
public PaymentScheduleItem accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* ID of the customer account that owns the payment schedule item, for example `402880e741112b310149b7343ef81234`.
* @return accountId
*/
@javax.annotation.Nullable
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public PaymentScheduleItem amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The total amount of the payment schedule.
* @return amount
*/
@javax.annotation.Nullable
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public PaymentScheduleItem balance(BigDecimal balance) {
this.balance = balance;
return this;
}
/**
* The remaining balance of payment schedule item.
* @return balance
*/
@javax.annotation.Nullable
public BigDecimal getBalance() {
return balance;
}
public void setBalance(BigDecimal balance) {
this.balance = balance;
}
public PaymentScheduleItem billingDocument(PaymentScheduleBillingDocument billingDocument) {
this.billingDocument = billingDocument;
return this;
}
/**
* Get billingDocument
* @return billingDocument
*/
@javax.annotation.Nullable
public PaymentScheduleBillingDocument getBillingDocument() {
return billingDocument;
}
public void setBillingDocument(PaymentScheduleBillingDocument billingDocument) {
this.billingDocument = billingDocument;
}
public PaymentScheduleItem cancellationReason(String cancellationReason) {
this.cancellationReason = cancellationReason;
return this;
}
/**
* The reason for the cancellation of payment schedule item.
* @return cancellationReason
*/
@javax.annotation.Nullable
public String getCancellationReason() {
return cancellationReason;
}
public void setCancellationReason(String cancellationReason) {
this.cancellationReason = cancellationReason;
}
public PaymentScheduleItem cancelledById(String cancelledById) {
this.cancelledById = cancelledById;
return this;
}
/**
* The ID of the user who cancel the payment schedule item.
* @return cancelledById
*/
@javax.annotation.Nullable
public String getCancelledById() {
return cancelledById;
}
public void setCancelledById(String cancelledById) {
this.cancelledById = cancelledById;
}
public PaymentScheduleItem cancelledOn(String cancelledOn) {
this.cancelledOn = cancelledOn;
return this;
}
/**
* The date and time when the payment schedule item was cancelled.
* @return cancelledOn
*/
@javax.annotation.Nullable
public String getCancelledOn() {
return cancelledOn;
}
public void setCancelledOn(String cancelledOn) {
this.cancelledOn = cancelledOn;
}
public PaymentScheduleItem createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* The ID of the user who created the payment schedule item.
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public PaymentScheduleItem createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* The date and time when the payment schedule item was created.
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public PaymentScheduleItem currency(String currency) {
this.currency = currency;
return this;
}
/**
* The currency of the payment.
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public PaymentScheduleItem description(String description) {
this.description = description;
return this;
}
/**
* The description of the payment schedule item.
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public PaymentScheduleItem errorMessage(String errorMessage) {
this.errorMessage = errorMessage;
return this;
}
/**
* The error message indicating if the error is related to the configuration or the payment collection.
* @return errorMessage
*/
@javax.annotation.Nullable
public String getErrorMessage() {
return errorMessage;
}
public void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
public PaymentScheduleItem id(String id) {
this.id = id;
return this;
}
/**
* ID of the payment schedule item. For example, `412880e749b72b310149b7343ef81346`.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public PaymentScheduleItem number(String number) {
this.number = number;
return this;
}
/**
* Number of the payment schedule item.
* @return number
*/
@javax.annotation.Nullable
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public PaymentScheduleItem paymentGatewayId(String paymentGatewayId) {
this.paymentGatewayId = paymentGatewayId;
return this;
}
/**
* ID of the payment gateway of the payment schedule item.
* @return paymentGatewayId
*/
@javax.annotation.Nullable
public String getPaymentGatewayId() {
return paymentGatewayId;
}
public void setPaymentGatewayId(String paymentGatewayId) {
this.paymentGatewayId = paymentGatewayId;
}
@Deprecated
public PaymentScheduleItem paymentId(String paymentId) {
this.paymentId = paymentId;
return this;
}
/**
* ID of the payment that is created by the payment schedule item, or linked to the payment schedule item. This field is only available if the request doesn’t specify `zuora-version`, or `zuora-version` is set to a value equal to or smaller than `336.0`.
* @return paymentId
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public String getPaymentId() {
return paymentId;
}
@Deprecated
public void setPaymentId(String paymentId) {
this.paymentId = paymentId;
}
public PaymentScheduleItem paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
return this;
}
/**
* ID of the payment method of the payment schedule item.
* @return paymentMethodId
*/
@javax.annotation.Nullable
public String getPaymentMethodId() {
return paymentMethodId;
}
public void setPaymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
}
public PaymentScheduleItem paymentOption(List paymentOption) {
this.paymentOption = paymentOption;
return this;
}
public PaymentScheduleItem addPaymentOptionItem(PaymentSchedulePaymentOptionFields paymentOptionItem) {
if (this.paymentOption == null) {
this.paymentOption = new ArrayList<>();
}
this.paymentOption.add(paymentOptionItem);
return this;
}
/**
* Container for the paymentOption items, which describe the transactional level rules for processing payments. Currently, only the Gateway Options type is supported. `paymentOption` of the payment schedule takes precedence over `paymentOption` of the payment schedule item. This field is only available if `zuora-version` is set to `337.0` or later.
* @return paymentOption
*/
@javax.annotation.Nullable
public List getPaymentOption() {
return paymentOption;
}
public void setPaymentOption(List paymentOption) {
this.paymentOption = paymentOption;
}
public PaymentScheduleItem paymentScheduleId(String paymentScheduleId) {
this.paymentScheduleId = paymentScheduleId;
return this;
}
/**
* ID of the payment schedule that contains the payment schedule item, for example, `ID402880e749b72b310149b7343ef80005`.
* @return paymentScheduleId
*/
@javax.annotation.Nullable
public String getPaymentScheduleId() {
return paymentScheduleId;
}
public void setPaymentScheduleId(String paymentScheduleId) {
this.paymentScheduleId = paymentScheduleId;
}
public PaymentScheduleItem paymentScheduleNumber(String paymentScheduleNumber) {
this.paymentScheduleNumber = paymentScheduleNumber;
return this;
}
/**
* Number of the payment schedule that contains the payment schedule item, for example, `ID402880e749b72b310149b7343ef80005`.
* @return paymentScheduleNumber
*/
@javax.annotation.Nullable
public String getPaymentScheduleNumber() {
return paymentScheduleNumber;
}
public void setPaymentScheduleNumber(String paymentScheduleNumber) {
this.paymentScheduleNumber = paymentScheduleNumber;
}
public PaymentScheduleItem psiPayments(List psiPayments) {
this.psiPayments = psiPayments;
return this;
}
public PaymentScheduleItem addPsiPaymentsItem(PaymentScheduleLinkedPaymentID psiPaymentsItem) {
if (this.psiPayments == null) {
this.psiPayments = new ArrayList<>();
}
this.psiPayments.add(psiPaymentsItem);
return this;
}
/**
* Container for payments linked to the payment schedule item.
* @return psiPayments
*/
@javax.annotation.Nullable
public List getPsiPayments() {
return psiPayments;
}
public void setPsiPayments(List psiPayments) {
this.psiPayments = psiPayments;
}
public PaymentScheduleItem runHour(Integer runHour) {
this.runHour = runHour;
return this;
}
/**
* At which hour in the day in the tenant’s timezone this payment will be collected. If the payment `runHour` and `scheduledDate` are backdated, the system will collect the payment when the next runHour occurs.
* @return runHour
*/
@javax.annotation.Nullable
public Integer getRunHour() {
return runHour;
}
public void setRunHour(Integer runHour) {
this.runHour = runHour;
}
public PaymentScheduleItem scheduledDate(String scheduledDate) {
this.scheduledDate = scheduledDate;
return this;
}
/**
* The scheduled date when the payment is processed.
* @return scheduledDate
*/
@javax.annotation.Nullable
public String getScheduledDate() {
return scheduledDate;
}
public void setScheduledDate(String scheduledDate) {
this.scheduledDate = scheduledDate;
}
public PaymentScheduleItem standalone(Boolean standalone) {
this.standalone = standalone;
return this;
}
/**
* Indicates if the payment created by the payment schedule item is a standalone payment or not.
* @return standalone
*/
@javax.annotation.Nullable
public Boolean getStandalone() {
return standalone;
}
public void setStandalone(Boolean standalone) {
this.standalone = standalone;
}
public PaymentScheduleItem status(StatusEnum status) {
this.status = status;
return this;
}
/**
* ID of the payment method of the payment schedule item. - `Pending`: Payment schedule item is waiting for processing. - `Processed`: The payment has been collected. - `Error`: Failed to collect the payment. - `Canceled`: After a pending payment schedule item is canceled by the user, the item is marked as `Canceled`.
* @return status
*/
@javax.annotation.Nullable
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public PaymentScheduleItem updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* The ID of the user who updated the payment schedule item.
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public PaymentScheduleItem updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* The date and time when the payment schedule item was last updated.
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the PaymentScheduleItem instance itself
*/
public PaymentScheduleItem putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentScheduleItem paymentScheduleItem = (PaymentScheduleItem) o;
return Objects.equals(this.accountId, paymentScheduleItem.accountId) &&
Objects.equals(this.amount, paymentScheduleItem.amount) &&
Objects.equals(this.balance, paymentScheduleItem.balance) &&
Objects.equals(this.billingDocument, paymentScheduleItem.billingDocument) &&
Objects.equals(this.cancellationReason, paymentScheduleItem.cancellationReason) &&
Objects.equals(this.cancelledById, paymentScheduleItem.cancelledById) &&
Objects.equals(this.cancelledOn, paymentScheduleItem.cancelledOn) &&
Objects.equals(this.createdById, paymentScheduleItem.createdById) &&
Objects.equals(this.createdDate, paymentScheduleItem.createdDate) &&
Objects.equals(this.currency, paymentScheduleItem.currency) &&
Objects.equals(this.description, paymentScheduleItem.description) &&
Objects.equals(this.errorMessage, paymentScheduleItem.errorMessage) &&
Objects.equals(this.id, paymentScheduleItem.id) &&
Objects.equals(this.number, paymentScheduleItem.number) &&
Objects.equals(this.paymentGatewayId, paymentScheduleItem.paymentGatewayId) &&
Objects.equals(this.paymentId, paymentScheduleItem.paymentId) &&
Objects.equals(this.paymentMethodId, paymentScheduleItem.paymentMethodId) &&
Objects.equals(this.paymentOption, paymentScheduleItem.paymentOption) &&
Objects.equals(this.paymentScheduleId, paymentScheduleItem.paymentScheduleId) &&
Objects.equals(this.paymentScheduleNumber, paymentScheduleItem.paymentScheduleNumber) &&
Objects.equals(this.psiPayments, paymentScheduleItem.psiPayments) &&
Objects.equals(this.runHour, paymentScheduleItem.runHour) &&
Objects.equals(this.scheduledDate, paymentScheduleItem.scheduledDate) &&
Objects.equals(this.standalone, paymentScheduleItem.standalone) &&
Objects.equals(this.status, paymentScheduleItem.status) &&
Objects.equals(this.updatedById, paymentScheduleItem.updatedById) &&
Objects.equals(this.updatedDate, paymentScheduleItem.updatedDate)&&
Objects.equals(this.additionalProperties, paymentScheduleItem.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(accountId, amount, balance, billingDocument, cancellationReason, cancelledById, cancelledOn, createdById, createdDate, currency, description, errorMessage, id, number, paymentGatewayId, paymentId, paymentMethodId, paymentOption, paymentScheduleId, paymentScheduleNumber, psiPayments, runHour, scheduledDate, standalone, status, updatedById, updatedDate, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentScheduleItem {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" balance: ").append(toIndentedString(balance)).append("\n");
sb.append(" billingDocument: ").append(toIndentedString(billingDocument)).append("\n");
sb.append(" cancellationReason: ").append(toIndentedString(cancellationReason)).append("\n");
sb.append(" cancelledById: ").append(toIndentedString(cancelledById)).append("\n");
sb.append(" cancelledOn: ").append(toIndentedString(cancelledOn)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" errorMessage: ").append(toIndentedString(errorMessage)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" number: ").append(toIndentedString(number)).append("\n");
sb.append(" paymentGatewayId: ").append(toIndentedString(paymentGatewayId)).append("\n");
sb.append(" paymentId: ").append(toIndentedString(paymentId)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" paymentOption: ").append(toIndentedString(paymentOption)).append("\n");
sb.append(" paymentScheduleId: ").append(toIndentedString(paymentScheduleId)).append("\n");
sb.append(" paymentScheduleNumber: ").append(toIndentedString(paymentScheduleNumber)).append("\n");
sb.append(" psiPayments: ").append(toIndentedString(psiPayments)).append("\n");
sb.append(" runHour: ").append(toIndentedString(runHour)).append("\n");
sb.append(" scheduledDate: ").append(toIndentedString(scheduledDate)).append("\n");
sb.append(" standalone: ").append(toIndentedString(standalone)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("accountId");
openapiFields.add("amount");
openapiFields.add("balance");
openapiFields.add("billingDocument");
openapiFields.add("cancellationReason");
openapiFields.add("cancelledById");
openapiFields.add("cancelledOn");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("currency");
openapiFields.add("description");
openapiFields.add("errorMessage");
openapiFields.add("id");
openapiFields.add("number");
openapiFields.add("paymentGatewayId");
openapiFields.add("paymentId");
openapiFields.add("paymentMethodId");
openapiFields.add("paymentOption");
openapiFields.add("paymentScheduleId");
openapiFields.add("paymentScheduleNumber");
openapiFields.add("psiPayments");
openapiFields.add("runHour");
openapiFields.add("scheduledDate");
openapiFields.add("standalone");
openapiFields.add("status");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to PaymentScheduleItem
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!PaymentScheduleItem.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in PaymentScheduleItem is not found in the empty JSON string", PaymentScheduleItem.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("accountId") != null && !jsonObj.get("accountId").isJsonNull()) && !jsonObj.get("accountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountId").toString()));
}
// validate the optional field `billingDocument`
if (jsonObj.get("billingDocument") != null && !jsonObj.get("billingDocument").isJsonNull()) {
PaymentScheduleBillingDocument.validateJsonElement(jsonObj.get("billingDocument"));
}
if ((jsonObj.get("cancellationReason") != null && !jsonObj.get("cancellationReason").isJsonNull()) && !jsonObj.get("cancellationReason").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cancellationReason` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cancellationReason").toString()));
}
if ((jsonObj.get("cancelledById") != null && !jsonObj.get("cancelledById").isJsonNull()) && !jsonObj.get("cancelledById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cancelledById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cancelledById").toString()));
}
if ((jsonObj.get("cancelledOn") != null && !jsonObj.get("cancelledOn").isJsonNull()) && !jsonObj.get("cancelledOn").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cancelledOn` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cancelledOn").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if ((jsonObj.get("errorMessage") != null && !jsonObj.get("errorMessage").isJsonNull()) && !jsonObj.get("errorMessage").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `errorMessage` to be a primitive type in the JSON string but got `%s`", jsonObj.get("errorMessage").toString()));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("number") != null && !jsonObj.get("number").isJsonNull()) && !jsonObj.get("number").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `number` to be a primitive type in the JSON string but got `%s`", jsonObj.get("number").toString()));
}
if ((jsonObj.get("paymentGatewayId") != null && !jsonObj.get("paymentGatewayId").isJsonNull()) && !jsonObj.get("paymentGatewayId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentGatewayId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentGatewayId").toString()));
}
if ((jsonObj.get("paymentId") != null && !jsonObj.get("paymentId").isJsonNull()) && !jsonObj.get("paymentId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentId").toString()));
}
if ((jsonObj.get("paymentMethodId") != null && !jsonObj.get("paymentMethodId").isJsonNull()) && !jsonObj.get("paymentMethodId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentMethodId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentMethodId").toString()));
}
if (jsonObj.get("paymentOption") != null && !jsonObj.get("paymentOption").isJsonNull()) {
JsonArray jsonArraypaymentOption = jsonObj.getAsJsonArray("paymentOption");
if (jsonArraypaymentOption != null) {
// ensure the json data is an array
if (!jsonObj.get("paymentOption").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentOption` to be an array in the JSON string but got `%s`", jsonObj.get("paymentOption").toString()));
}
// validate the optional field `paymentOption` (array)
for (int i = 0; i < jsonArraypaymentOption.size(); i++) {
PaymentSchedulePaymentOptionFields.validateJsonElement(jsonArraypaymentOption.get(i));
};
}
}
if ((jsonObj.get("paymentScheduleId") != null && !jsonObj.get("paymentScheduleId").isJsonNull()) && !jsonObj.get("paymentScheduleId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentScheduleId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentScheduleId").toString()));
}
if ((jsonObj.get("paymentScheduleNumber") != null && !jsonObj.get("paymentScheduleNumber").isJsonNull()) && !jsonObj.get("paymentScheduleNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentScheduleNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentScheduleNumber").toString()));
}
if (jsonObj.get("psiPayments") != null && !jsonObj.get("psiPayments").isJsonNull()) {
JsonArray jsonArraypsiPayments = jsonObj.getAsJsonArray("psiPayments");
if (jsonArraypsiPayments != null) {
// ensure the json data is an array
if (!jsonObj.get("psiPayments").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `psiPayments` to be an array in the JSON string but got `%s`", jsonObj.get("psiPayments").toString()));
}
// validate the optional field `psiPayments` (array)
for (int i = 0; i < jsonArraypsiPayments.size(); i++) {
PaymentScheduleLinkedPaymentID.validateJsonElement(jsonArraypsiPayments.get(i));
};
}
}
if ((jsonObj.get("scheduledDate") != null && !jsonObj.get("scheduledDate").isJsonNull()) && !jsonObj.get("scheduledDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `scheduledDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("scheduledDate").toString()));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
// validate the optional field `status`
if (jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) {
StatusEnum.validateJsonElement(jsonObj.get("status"));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!PaymentScheduleItem.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'PaymentScheduleItem' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(PaymentScheduleItem.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, PaymentScheduleItem value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public PaymentScheduleItem read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
PaymentScheduleItem instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of PaymentScheduleItem given an JSON string
*
* @param jsonString JSON string
* @return An instance of PaymentScheduleItem
* @throws IOException if the JSON string is invalid with respect to PaymentScheduleItem
*/
public static PaymentScheduleItem fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, PaymentScheduleItem.class);
}
/**
* Convert an instance of PaymentScheduleItem to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy