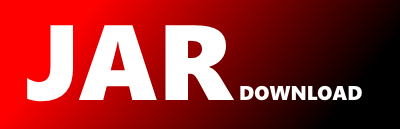
com.zuora.model.PostBusinessRegionsRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* PostBusinessRegionsRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class PostBusinessRegionsRequest {
public static final String SERIALIZED_NAME_COUNTRY = "country";
@SerializedName(SERIALIZED_NAME_COUNTRY)
private String country;
public static final String SERIALIZED_NAME_BUSINESS_NAME = "businessName";
@SerializedName(SERIALIZED_NAME_BUSINESS_NAME)
private String businessName;
public static final String SERIALIZED_NAME_BUSINESS_NUMBER = "businessNumber";
@SerializedName(SERIALIZED_NAME_BUSINESS_NUMBER)
private String businessNumber;
public static final String SERIALIZED_NAME_BUSINESS_NUMBER_SCHEMA_ID = "businessNumberSchemaId";
@SerializedName(SERIALIZED_NAME_BUSINESS_NUMBER_SCHEMA_ID)
private String businessNumberSchemaId;
public static final String SERIALIZED_NAME_TRADE_NAME = "tradeName";
@SerializedName(SERIALIZED_NAME_TRADE_NAME)
private String tradeName;
public static final String SERIALIZED_NAME_TAX_REGISTER_NUMBER = "taxRegisterNumber";
@SerializedName(SERIALIZED_NAME_TAX_REGISTER_NUMBER)
private String taxRegisterNumber;
public static final String SERIALIZED_NAME_ENDPOINT_ID = "endpointId";
@SerializedName(SERIALIZED_NAME_ENDPOINT_ID)
private String endpointId;
public static final String SERIALIZED_NAME_ENDPOINT_SCHEME_ID = "endpointSchemeId";
@SerializedName(SERIALIZED_NAME_ENDPOINT_SCHEME_ID)
private String endpointSchemeId;
public static final String SERIALIZED_NAME_ADDRESS_LINE1 = "addressLine1";
@SerializedName(SERIALIZED_NAME_ADDRESS_LINE1)
private String addressLine1;
public static final String SERIALIZED_NAME_ADDRESS_LINE2 = "addressLine2";
@SerializedName(SERIALIZED_NAME_ADDRESS_LINE2)
private String addressLine2;
public static final String SERIALIZED_NAME_POSTAL_CODE = "postalCode";
@SerializedName(SERIALIZED_NAME_POSTAL_CODE)
private String postalCode;
public static final String SERIALIZED_NAME_CITY = "city";
@SerializedName(SERIALIZED_NAME_CITY)
private String city;
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private String state;
public static final String SERIALIZED_NAME_CONTACT_NAME = "contactName";
@SerializedName(SERIALIZED_NAME_CONTACT_NAME)
private String contactName;
public static final String SERIALIZED_NAME_EMAIL = "email";
@SerializedName(SERIALIZED_NAME_EMAIL)
private String email;
public static final String SERIALIZED_NAME_PHONE_NUMBER = "phoneNumber";
@SerializedName(SERIALIZED_NAME_PHONE_NUMBER)
private String phoneNumber;
public static final String SERIALIZED_NAME_SERVICE_PROVIDER_ID = "serviceProviderId";
@SerializedName(SERIALIZED_NAME_SERVICE_PROVIDER_ID)
private String serviceProviderId;
public static final String SERIALIZED_NAME_DIGITAL_SIGNATURE_ENABLE = "digitalSignatureEnable";
@SerializedName(SERIALIZED_NAME_DIGITAL_SIGNATURE_ENABLE)
private Boolean digitalSignatureEnable;
public static final String SERIALIZED_NAME_DIGITAL_SIGNATURE_BOX_ENABLE = "digitalSignatureBoxEnable";
@SerializedName(SERIALIZED_NAME_DIGITAL_SIGNATURE_BOX_ENABLE)
private Boolean digitalSignatureBoxEnable;
public static final String SERIALIZED_NAME_DIGITAL_SIGNATURE_BOX_POS_X = "digitalSignatureBoxPosX";
@SerializedName(SERIALIZED_NAME_DIGITAL_SIGNATURE_BOX_POS_X)
private BigDecimal digitalSignatureBoxPosX;
public static final String SERIALIZED_NAME_DIGITAL_SIGNATURE_BOX_POS_Y = "digitalSignatureBoxPosY";
@SerializedName(SERIALIZED_NAME_DIGITAL_SIGNATURE_BOX_POS_Y)
private BigDecimal digitalSignatureBoxPosY;
public static final String SERIALIZED_NAME_RESPONSE_MAPPING = "responseMapping";
@SerializedName(SERIALIZED_NAME_RESPONSE_MAPPING)
private Map responseMapping;
/**
* The process type of the e-invoicing business region.
*/
@JsonAdapter(ProcessTypeEnum.Adapter.class)
public enum ProcessTypeEnum {
CLEARANCE("Clearance"),
CACELLATION("Cacellation"),
POSTAUDIT("PostAudit"),
PEPPOL("PEPPOL");
private String value;
ProcessTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ProcessTypeEnum fromValue(String value) {
for (ProcessTypeEnum b : ProcessTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ProcessTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ProcessTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ProcessTypeEnum.fromValue(value);
}
}
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
String value = jsonElement.getAsString();
ProcessTypeEnum.fromValue(value);
}
}
public static final String SERIALIZED_NAME_PROCESS_TYPE = "processType";
@SerializedName(SERIALIZED_NAME_PROCESS_TYPE)
private ProcessTypeEnum processType;
public static final String SERIALIZED_NAME_INVOICE_ENABLED = "invoiceEnabled";
@SerializedName(SERIALIZED_NAME_INVOICE_ENABLED)
private Boolean invoiceEnabled;
public static final String SERIALIZED_NAME_CREDIT_NOTE_ENABLED = "creditNoteEnabled";
@SerializedName(SERIALIZED_NAME_CREDIT_NOTE_ENABLED)
private Boolean creditNoteEnabled;
public static final String SERIALIZED_NAME_DEBIT_NOTE_ENABLED = "debitNoteEnabled";
@SerializedName(SERIALIZED_NAME_DEBIT_NOTE_ENABLED)
private Boolean debitNoteEnabled;
public PostBusinessRegionsRequest() {
}
public PostBusinessRegionsRequest country(String country) {
this.country = country;
return this;
}
/**
* The short name of a country or region where you must comply with e-invoicing requirements. For example, `IN` for India. For the full list of country names and codes, see <a href=\"https://knowledgecenter.zuora.com/Quick_References/Country%2C_State%2C_and_Province_Codes/A_Country_Names_and_Their_ISO_Codes\" target=\"_blank\">ISO Standard Country Codes</a>.
* @return country
*/
@javax.annotation.Nonnull
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
public PostBusinessRegionsRequest businessName(String businessName) {
this.businessName = businessName;
return this;
}
/**
* The full official name that the Seller is registered with the relevant legal authority.
* @return businessName
*/
@javax.annotation.Nonnull
public String getBusinessName() {
return businessName;
}
public void setBusinessName(String businessName) {
this.businessName = businessName;
}
public PostBusinessRegionsRequest businessNumber(String businessNumber) {
this.businessNumber = businessNumber;
return this;
}
/**
* The specify the unique identifier number of the legal entity or person that you do business with. For example, you must use a GSTIN for India and Tax Identification Number (TIN) for Saudi Arabia.
* @return businessNumber
*/
@javax.annotation.Nullable
public String getBusinessNumber() {
return businessNumber;
}
public void setBusinessNumber(String businessNumber) {
this.businessNumber = businessNumber;
}
public PostBusinessRegionsRequest businessNumberSchemaId(String businessNumberSchemaId) {
this.businessNumberSchemaId = businessNumberSchemaId;
return this;
}
/**
* The identification scheme identifier that an official registrar issues to identify the Seller as a legal entity or person.
* @return businessNumberSchemaId
*/
@javax.annotation.Nullable
public String getBusinessNumberSchemaId() {
return businessNumberSchemaId;
}
public void setBusinessNumberSchemaId(String businessNumberSchemaId) {
this.businessNumberSchemaId = businessNumberSchemaId;
}
public PostBusinessRegionsRequest tradeName(String tradeName) {
this.tradeName = tradeName;
return this;
}
/**
* The name that the Seller is known as, other than the legal business name.
* @return tradeName
*/
@javax.annotation.Nullable
public String getTradeName() {
return tradeName;
}
public void setTradeName(String tradeName) {
this.tradeName = tradeName;
}
public PostBusinessRegionsRequest taxRegisterNumber(String taxRegisterNumber) {
this.taxRegisterNumber = taxRegisterNumber;
return this;
}
/**
* The Seller's VAT identifier (also known as Seller VAT identification number) or the local identification (defined by the Seller’s address) of the Seller for tax purposes, or a reference that enables the Seller to state the registered tax status.
* @return taxRegisterNumber
*/
@javax.annotation.Nullable
public String getTaxRegisterNumber() {
return taxRegisterNumber;
}
public void setTaxRegisterNumber(String taxRegisterNumber) {
this.taxRegisterNumber = taxRegisterNumber;
}
public PostBusinessRegionsRequest endpointId(String endpointId) {
this.endpointId = endpointId;
return this;
}
/**
* The Seller's electronic address, to which the application-level response to the e-invoice file might be delivered.
* @return endpointId
*/
@javax.annotation.Nullable
public String getEndpointId() {
return endpointId;
}
public void setEndpointId(String endpointId) {
this.endpointId = endpointId;
}
public PostBusinessRegionsRequest endpointSchemeId(String endpointSchemeId) {
this.endpointSchemeId = endpointSchemeId;
return this;
}
/**
* The identification scheme identifier of the Seller’s electronic address.
* @return endpointSchemeId
*/
@javax.annotation.Nullable
public String getEndpointSchemeId() {
return endpointSchemeId;
}
public void setEndpointSchemeId(String endpointSchemeId) {
this.endpointSchemeId = endpointSchemeId;
}
public PostBusinessRegionsRequest addressLine1(String addressLine1) {
this.addressLine1 = addressLine1;
return this;
}
/**
* The first line of the Seller’s address, which is often a street address or business name.
* @return addressLine1
*/
@javax.annotation.Nullable
public String getAddressLine1() {
return addressLine1;
}
public void setAddressLine1(String addressLine1) {
this.addressLine1 = addressLine1;
}
public PostBusinessRegionsRequest addressLine2(String addressLine2) {
this.addressLine2 = addressLine2;
return this;
}
/**
* The second line of the Seller’s address, which is often the name of a building.
* @return addressLine2
*/
@javax.annotation.Nullable
public String getAddressLine2() {
return addressLine2;
}
public void setAddressLine2(String addressLine2) {
this.addressLine2 = addressLine2;
}
public PostBusinessRegionsRequest postalCode(String postalCode) {
this.postalCode = postalCode;
return this;
}
/**
* The short code that can identify the business address.
* @return postalCode
*/
@javax.annotation.Nullable
public String getPostalCode() {
return postalCode;
}
public void setPostalCode(String postalCode) {
this.postalCode = postalCode;
}
public PostBusinessRegionsRequest city(String city) {
this.city = city;
return this;
}
/**
* The the name of the city where the business is located.
* @return city
*/
@javax.annotation.Nullable
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public PostBusinessRegionsRequest state(String state) {
this.state = state;
return this;
}
/**
* The name of the state or province where the business is located.
* @return state
*/
@javax.annotation.Nullable
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public PostBusinessRegionsRequest contactName(String contactName) {
this.contactName = contactName;
return this;
}
/**
* The name of the Seller contact to receive e-invoicing data.
* @return contactName
*/
@javax.annotation.Nullable
public String getContactName() {
return contactName;
}
public void setContactName(String contactName) {
this.contactName = contactName;
}
public PostBusinessRegionsRequest email(String email) {
this.email = email;
return this;
}
/**
* The email address of the Seller contact to receive e-invoicing data.
* @return email
*/
@javax.annotation.Nullable
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public PostBusinessRegionsRequest phoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
return this;
}
/**
* The business phone number of the Seller contact to receive e-invoicing data.
* @return phoneNumber
*/
@javax.annotation.Nullable
public String getPhoneNumber() {
return phoneNumber;
}
public void setPhoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
}
public PostBusinessRegionsRequest serviceProviderId(String serviceProviderId) {
this.serviceProviderId = serviceProviderId;
return this;
}
/**
* The unique ID of the e-invoicing service provider that is associated to the business region.
* @return serviceProviderId
*/
@javax.annotation.Nullable
public String getServiceProviderId() {
return serviceProviderId;
}
public void setServiceProviderId(String serviceProviderId) {
this.serviceProviderId = serviceProviderId;
}
public PostBusinessRegionsRequest digitalSignatureEnable(Boolean digitalSignatureEnable) {
this.digitalSignatureEnable = digitalSignatureEnable;
return this;
}
/**
* It will control that whether the pdf should be signed by vendor.
* @return digitalSignatureEnable
*/
@javax.annotation.Nullable
public Boolean getDigitalSignatureEnable() {
return digitalSignatureEnable;
}
public void setDigitalSignatureEnable(Boolean digitalSignatureEnable) {
this.digitalSignatureEnable = digitalSignatureEnable;
}
public PostBusinessRegionsRequest digitalSignatureBoxEnable(Boolean digitalSignatureBoxEnable) {
this.digitalSignatureBoxEnable = digitalSignatureBoxEnable;
return this;
}
/**
* It will control whether the dignature box will be shown on the pdf.
* @return digitalSignatureBoxEnable
*/
@javax.annotation.Nullable
public Boolean getDigitalSignatureBoxEnable() {
return digitalSignatureBoxEnable;
}
public void setDigitalSignatureBoxEnable(Boolean digitalSignatureBoxEnable) {
this.digitalSignatureBoxEnable = digitalSignatureBoxEnable;
}
public PostBusinessRegionsRequest digitalSignatureBoxPosX(BigDecimal digitalSignatureBoxPosX) {
this.digitalSignatureBoxPosX = digitalSignatureBoxPosX;
return this;
}
/**
* It is the X-axis that the box will be shown.
* @return digitalSignatureBoxPosX
*/
@javax.annotation.Nullable
public BigDecimal getDigitalSignatureBoxPosX() {
return digitalSignatureBoxPosX;
}
public void setDigitalSignatureBoxPosX(BigDecimal digitalSignatureBoxPosX) {
this.digitalSignatureBoxPosX = digitalSignatureBoxPosX;
}
public PostBusinessRegionsRequest digitalSignatureBoxPosY(BigDecimal digitalSignatureBoxPosY) {
this.digitalSignatureBoxPosY = digitalSignatureBoxPosY;
return this;
}
/**
* It is the Y-axis that the box will be shown.
* @return digitalSignatureBoxPosY
*/
@javax.annotation.Nullable
public BigDecimal getDigitalSignatureBoxPosY() {
return digitalSignatureBoxPosY;
}
public void setDigitalSignatureBoxPosY(BigDecimal digitalSignatureBoxPosY) {
this.digitalSignatureBoxPosY = digitalSignatureBoxPosY;
}
public PostBusinessRegionsRequest responseMapping(Map responseMapping) {
this.responseMapping = responseMapping;
return this;
}
public PostBusinessRegionsRequest putResponseMappingItem(String key, Object responseMappingItem) {
if (this.responseMapping == null) {
this.responseMapping = new HashMap<>();
}
this.responseMapping.put(key, responseMappingItem);
return this;
}
/**
* The response mapping of the e-invoicing business region.
* @return responseMapping
*/
@javax.annotation.Nullable
public Map getResponseMapping() {
return responseMapping;
}
public void setResponseMapping(Map responseMapping) {
this.responseMapping = responseMapping;
}
public PostBusinessRegionsRequest processType(ProcessTypeEnum processType) {
this.processType = processType;
return this;
}
/**
* The process type of the e-invoicing business region.
* @return processType
*/
@javax.annotation.Nullable
public ProcessTypeEnum getProcessType() {
return processType;
}
public void setProcessType(ProcessTypeEnum processType) {
this.processType = processType;
}
public PostBusinessRegionsRequest invoiceEnabled(Boolean invoiceEnabled) {
this.invoiceEnabled = invoiceEnabled;
return this;
}
/**
* It will control that whether the invoice should be supported by the process type or not.
* @return invoiceEnabled
*/
@javax.annotation.Nullable
public Boolean getInvoiceEnabled() {
return invoiceEnabled;
}
public void setInvoiceEnabled(Boolean invoiceEnabled) {
this.invoiceEnabled = invoiceEnabled;
}
public PostBusinessRegionsRequest creditNoteEnabled(Boolean creditNoteEnabled) {
this.creditNoteEnabled = creditNoteEnabled;
return this;
}
/**
* It will control that whether the credit note should be supported by the process type or not.
* @return creditNoteEnabled
*/
@javax.annotation.Nullable
public Boolean getCreditNoteEnabled() {
return creditNoteEnabled;
}
public void setCreditNoteEnabled(Boolean creditNoteEnabled) {
this.creditNoteEnabled = creditNoteEnabled;
}
public PostBusinessRegionsRequest debitNoteEnabled(Boolean debitNoteEnabled) {
this.debitNoteEnabled = debitNoteEnabled;
return this;
}
/**
* It will control that whether the debit note should be supported by the process type or not.
* @return debitNoteEnabled
*/
@javax.annotation.Nullable
public Boolean getDebitNoteEnabled() {
return debitNoteEnabled;
}
public void setDebitNoteEnabled(Boolean debitNoteEnabled) {
this.debitNoteEnabled = debitNoteEnabled;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the PostBusinessRegionsRequest instance itself
*/
public PostBusinessRegionsRequest putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PostBusinessRegionsRequest postBusinessRegionsRequest = (PostBusinessRegionsRequest) o;
return Objects.equals(this.country, postBusinessRegionsRequest.country) &&
Objects.equals(this.businessName, postBusinessRegionsRequest.businessName) &&
Objects.equals(this.businessNumber, postBusinessRegionsRequest.businessNumber) &&
Objects.equals(this.businessNumberSchemaId, postBusinessRegionsRequest.businessNumberSchemaId) &&
Objects.equals(this.tradeName, postBusinessRegionsRequest.tradeName) &&
Objects.equals(this.taxRegisterNumber, postBusinessRegionsRequest.taxRegisterNumber) &&
Objects.equals(this.endpointId, postBusinessRegionsRequest.endpointId) &&
Objects.equals(this.endpointSchemeId, postBusinessRegionsRequest.endpointSchemeId) &&
Objects.equals(this.addressLine1, postBusinessRegionsRequest.addressLine1) &&
Objects.equals(this.addressLine2, postBusinessRegionsRequest.addressLine2) &&
Objects.equals(this.postalCode, postBusinessRegionsRequest.postalCode) &&
Objects.equals(this.city, postBusinessRegionsRequest.city) &&
Objects.equals(this.state, postBusinessRegionsRequest.state) &&
Objects.equals(this.contactName, postBusinessRegionsRequest.contactName) &&
Objects.equals(this.email, postBusinessRegionsRequest.email) &&
Objects.equals(this.phoneNumber, postBusinessRegionsRequest.phoneNumber) &&
Objects.equals(this.serviceProviderId, postBusinessRegionsRequest.serviceProviderId) &&
Objects.equals(this.digitalSignatureEnable, postBusinessRegionsRequest.digitalSignatureEnable) &&
Objects.equals(this.digitalSignatureBoxEnable, postBusinessRegionsRequest.digitalSignatureBoxEnable) &&
Objects.equals(this.digitalSignatureBoxPosX, postBusinessRegionsRequest.digitalSignatureBoxPosX) &&
Objects.equals(this.digitalSignatureBoxPosY, postBusinessRegionsRequest.digitalSignatureBoxPosY) &&
Objects.equals(this.responseMapping, postBusinessRegionsRequest.responseMapping) &&
Objects.equals(this.processType, postBusinessRegionsRequest.processType) &&
Objects.equals(this.invoiceEnabled, postBusinessRegionsRequest.invoiceEnabled) &&
Objects.equals(this.creditNoteEnabled, postBusinessRegionsRequest.creditNoteEnabled) &&
Objects.equals(this.debitNoteEnabled, postBusinessRegionsRequest.debitNoteEnabled)&&
Objects.equals(this.additionalProperties, postBusinessRegionsRequest.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(country, businessName, businessNumber, businessNumberSchemaId, tradeName, taxRegisterNumber, endpointId, endpointSchemeId, addressLine1, addressLine2, postalCode, city, state, contactName, email, phoneNumber, serviceProviderId, digitalSignatureEnable, digitalSignatureBoxEnable, digitalSignatureBoxPosX, digitalSignatureBoxPosY, responseMapping, processType, invoiceEnabled, creditNoteEnabled, debitNoteEnabled, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PostBusinessRegionsRequest {\n");
sb.append(" country: ").append(toIndentedString(country)).append("\n");
sb.append(" businessName: ").append(toIndentedString(businessName)).append("\n");
sb.append(" businessNumber: ").append(toIndentedString(businessNumber)).append("\n");
sb.append(" businessNumberSchemaId: ").append(toIndentedString(businessNumberSchemaId)).append("\n");
sb.append(" tradeName: ").append(toIndentedString(tradeName)).append("\n");
sb.append(" taxRegisterNumber: ").append(toIndentedString(taxRegisterNumber)).append("\n");
sb.append(" endpointId: ").append(toIndentedString(endpointId)).append("\n");
sb.append(" endpointSchemeId: ").append(toIndentedString(endpointSchemeId)).append("\n");
sb.append(" addressLine1: ").append(toIndentedString(addressLine1)).append("\n");
sb.append(" addressLine2: ").append(toIndentedString(addressLine2)).append("\n");
sb.append(" postalCode: ").append(toIndentedString(postalCode)).append("\n");
sb.append(" city: ").append(toIndentedString(city)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" contactName: ").append(toIndentedString(contactName)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" phoneNumber: ").append(toIndentedString(phoneNumber)).append("\n");
sb.append(" serviceProviderId: ").append(toIndentedString(serviceProviderId)).append("\n");
sb.append(" digitalSignatureEnable: ").append(toIndentedString(digitalSignatureEnable)).append("\n");
sb.append(" digitalSignatureBoxEnable: ").append(toIndentedString(digitalSignatureBoxEnable)).append("\n");
sb.append(" digitalSignatureBoxPosX: ").append(toIndentedString(digitalSignatureBoxPosX)).append("\n");
sb.append(" digitalSignatureBoxPosY: ").append(toIndentedString(digitalSignatureBoxPosY)).append("\n");
sb.append(" responseMapping: ").append(toIndentedString(responseMapping)).append("\n");
sb.append(" processType: ").append(toIndentedString(processType)).append("\n");
sb.append(" invoiceEnabled: ").append(toIndentedString(invoiceEnabled)).append("\n");
sb.append(" creditNoteEnabled: ").append(toIndentedString(creditNoteEnabled)).append("\n");
sb.append(" debitNoteEnabled: ").append(toIndentedString(debitNoteEnabled)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("country");
openapiFields.add("businessName");
openapiFields.add("businessNumber");
openapiFields.add("businessNumberSchemaId");
openapiFields.add("tradeName");
openapiFields.add("taxRegisterNumber");
openapiFields.add("endpointId");
openapiFields.add("endpointSchemeId");
openapiFields.add("addressLine1");
openapiFields.add("addressLine2");
openapiFields.add("postalCode");
openapiFields.add("city");
openapiFields.add("state");
openapiFields.add("contactName");
openapiFields.add("email");
openapiFields.add("phoneNumber");
openapiFields.add("serviceProviderId");
openapiFields.add("digitalSignatureEnable");
openapiFields.add("digitalSignatureBoxEnable");
openapiFields.add("digitalSignatureBoxPosX");
openapiFields.add("digitalSignatureBoxPosY");
openapiFields.add("responseMapping");
openapiFields.add("processType");
openapiFields.add("invoiceEnabled");
openapiFields.add("creditNoteEnabled");
openapiFields.add("debitNoteEnabled");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("country");
openapiRequiredFields.add("businessName");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to PostBusinessRegionsRequest
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!PostBusinessRegionsRequest.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in PostBusinessRegionsRequest is not found in the empty JSON string", PostBusinessRegionsRequest.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : PostBusinessRegionsRequest.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if (!jsonObj.get("country").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `country` to be a primitive type in the JSON string but got `%s`", jsonObj.get("country").toString()));
}
if (!jsonObj.get("businessName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `businessName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("businessName").toString()));
}
if ((jsonObj.get("businessNumber") != null && !jsonObj.get("businessNumber").isJsonNull()) && !jsonObj.get("businessNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `businessNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("businessNumber").toString()));
}
if ((jsonObj.get("businessNumberSchemaId") != null && !jsonObj.get("businessNumberSchemaId").isJsonNull()) && !jsonObj.get("businessNumberSchemaId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `businessNumberSchemaId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("businessNumberSchemaId").toString()));
}
if ((jsonObj.get("tradeName") != null && !jsonObj.get("tradeName").isJsonNull()) && !jsonObj.get("tradeName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `tradeName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("tradeName").toString()));
}
if ((jsonObj.get("taxRegisterNumber") != null && !jsonObj.get("taxRegisterNumber").isJsonNull()) && !jsonObj.get("taxRegisterNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxRegisterNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxRegisterNumber").toString()));
}
if ((jsonObj.get("endpointId") != null && !jsonObj.get("endpointId").isJsonNull()) && !jsonObj.get("endpointId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `endpointId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("endpointId").toString()));
}
if ((jsonObj.get("endpointSchemeId") != null && !jsonObj.get("endpointSchemeId").isJsonNull()) && !jsonObj.get("endpointSchemeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `endpointSchemeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("endpointSchemeId").toString()));
}
if ((jsonObj.get("addressLine1") != null && !jsonObj.get("addressLine1").isJsonNull()) && !jsonObj.get("addressLine1").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `addressLine1` to be a primitive type in the JSON string but got `%s`", jsonObj.get("addressLine1").toString()));
}
if ((jsonObj.get("addressLine2") != null && !jsonObj.get("addressLine2").isJsonNull()) && !jsonObj.get("addressLine2").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `addressLine2` to be a primitive type in the JSON string but got `%s`", jsonObj.get("addressLine2").toString()));
}
if ((jsonObj.get("postalCode") != null && !jsonObj.get("postalCode").isJsonNull()) && !jsonObj.get("postalCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `postalCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("postalCode").toString()));
}
if ((jsonObj.get("city") != null && !jsonObj.get("city").isJsonNull()) && !jsonObj.get("city").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `city` to be a primitive type in the JSON string but got `%s`", jsonObj.get("city").toString()));
}
if ((jsonObj.get("state") != null && !jsonObj.get("state").isJsonNull()) && !jsonObj.get("state").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `state` to be a primitive type in the JSON string but got `%s`", jsonObj.get("state").toString()));
}
if ((jsonObj.get("contactName") != null && !jsonObj.get("contactName").isJsonNull()) && !jsonObj.get("contactName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contactName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contactName").toString()));
}
if ((jsonObj.get("email") != null && !jsonObj.get("email").isJsonNull()) && !jsonObj.get("email").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `email` to be a primitive type in the JSON string but got `%s`", jsonObj.get("email").toString()));
}
if ((jsonObj.get("phoneNumber") != null && !jsonObj.get("phoneNumber").isJsonNull()) && !jsonObj.get("phoneNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `phoneNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("phoneNumber").toString()));
}
if ((jsonObj.get("serviceProviderId") != null && !jsonObj.get("serviceProviderId").isJsonNull()) && !jsonObj.get("serviceProviderId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `serviceProviderId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("serviceProviderId").toString()));
}
if ((jsonObj.get("processType") != null && !jsonObj.get("processType").isJsonNull()) && !jsonObj.get("processType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `processType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("processType").toString()));
}
// validate the optional field `processType`
if (jsonObj.get("processType") != null && !jsonObj.get("processType").isJsonNull()) {
ProcessTypeEnum.validateJsonElement(jsonObj.get("processType"));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!PostBusinessRegionsRequest.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'PostBusinessRegionsRequest' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(PostBusinessRegionsRequest.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, PostBusinessRegionsRequest value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public PostBusinessRegionsRequest read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
PostBusinessRegionsRequest instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of PostBusinessRegionsRequest given an JSON string
*
* @param jsonString JSON string
* @return An instance of PostBusinessRegionsRequest
* @throws IOException if the JSON string is invalid with respect to PostBusinessRegionsRequest
*/
public static PostBusinessRegionsRequest fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, PostBusinessRegionsRequest.class);
}
/**
* Convert an instance of PostBusinessRegionsRequest to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy