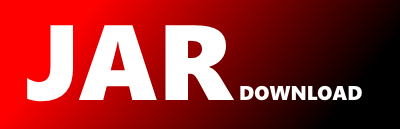
com.zuora.model.PostPublicEmailTemplateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.PostPublicEmailTemplateRequestCcEmailType;
import com.zuora.model.PostPublicEmailTemplateRequestEncodingType;
import com.zuora.model.PostPublicEmailTemplateRequestFromEmailType;
import com.zuora.model.PostPublicEmailTemplateRequestReplyToEmailType;
import com.zuora.model.PostPublicEmailTemplateRequestToEmailType;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* PostPublicEmailTemplateRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class PostPublicEmailTemplateRequest {
public static final String SERIALIZED_NAME_ACTIVE = "active";
@SerializedName(SERIALIZED_NAME_ACTIVE)
private Boolean active = true;
public static final String SERIALIZED_NAME_BCC_EMAIL_ADDRESS = "bccEmailAddress";
@SerializedName(SERIALIZED_NAME_BCC_EMAIL_ADDRESS)
private String bccEmailAddress;
public static final String SERIALIZED_NAME_CC_EMAIL_ADDRESS = "ccEmailAddress";
@SerializedName(SERIALIZED_NAME_CC_EMAIL_ADDRESS)
private String ccEmailAddress;
public static final String SERIALIZED_NAME_CC_EMAIL_TYPE = "ccEmailType";
@SerializedName(SERIALIZED_NAME_CC_EMAIL_TYPE)
private PostPublicEmailTemplateRequestCcEmailType ccEmailType = PostPublicEmailTemplateRequestCcEmailType.SPECIFICEMAILS;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_EMAIL_BODY = "emailBody";
@SerializedName(SERIALIZED_NAME_EMAIL_BODY)
private String emailBody;
public static final String SERIALIZED_NAME_EMAIL_SUBJECT = "emailSubject";
@SerializedName(SERIALIZED_NAME_EMAIL_SUBJECT)
private String emailSubject;
public static final String SERIALIZED_NAME_ENCODING_TYPE = "encodingType";
@SerializedName(SERIALIZED_NAME_ENCODING_TYPE)
private PostPublicEmailTemplateRequestEncodingType encodingType = PostPublicEmailTemplateRequestEncodingType.UTF8;
public static final String SERIALIZED_NAME_EVENT_CATEGORY = "eventCategory";
@SerializedName(SERIALIZED_NAME_EVENT_CATEGORY)
private BigDecimal eventCategory;
public static final String SERIALIZED_NAME_EVENT_TYPE_NAME = "eventTypeName";
@SerializedName(SERIALIZED_NAME_EVENT_TYPE_NAME)
private String eventTypeName;
public static final String SERIALIZED_NAME_EVENT_TYPE_NAMESPACE = "eventTypeNamespace";
@SerializedName(SERIALIZED_NAME_EVENT_TYPE_NAMESPACE)
private String eventTypeNamespace;
public static final String SERIALIZED_NAME_FROM_EMAIL_ADDRESS = "fromEmailAddress";
@SerializedName(SERIALIZED_NAME_FROM_EMAIL_ADDRESS)
private String fromEmailAddress;
public static final String SERIALIZED_NAME_FROM_EMAIL_TYPE = "fromEmailType";
@SerializedName(SERIALIZED_NAME_FROM_EMAIL_TYPE)
private PostPublicEmailTemplateRequestFromEmailType fromEmailType;
public static final String SERIALIZED_NAME_FROM_NAME = "fromName";
@SerializedName(SERIALIZED_NAME_FROM_NAME)
private String fromName;
public static final String SERIALIZED_NAME_IS_HTML = "isHtml";
@SerializedName(SERIALIZED_NAME_IS_HTML)
private Boolean isHtml = false;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_REPLY_TO_EMAIL_ADDRESS = "replyToEmailAddress";
@SerializedName(SERIALIZED_NAME_REPLY_TO_EMAIL_ADDRESS)
private String replyToEmailAddress;
public static final String SERIALIZED_NAME_REPLY_TO_EMAIL_TYPE = "replyToEmailType";
@SerializedName(SERIALIZED_NAME_REPLY_TO_EMAIL_TYPE)
private PostPublicEmailTemplateRequestReplyToEmailType replyToEmailType;
public static final String SERIALIZED_NAME_TO_EMAIL_ADDRESS = "toEmailAddress";
@SerializedName(SERIALIZED_NAME_TO_EMAIL_ADDRESS)
private String toEmailAddress;
public static final String SERIALIZED_NAME_TO_EMAIL_TYPE = "toEmailType";
@SerializedName(SERIALIZED_NAME_TO_EMAIL_TYPE)
private PostPublicEmailTemplateRequestToEmailType toEmailType;
public PostPublicEmailTemplateRequest() {
}
public PostPublicEmailTemplateRequest active(Boolean active) {
this.active = active;
return this;
}
/**
* The status of the email template. The default value is `true`.
* @return active
*/
@javax.annotation.Nullable
public Boolean getActive() {
return active;
}
public void setActive(Boolean active) {
this.active = active;
}
public PostPublicEmailTemplateRequest bccEmailAddress(String bccEmailAddress) {
this.bccEmailAddress = bccEmailAddress;
return this;
}
/**
* The email bcc address.
* @return bccEmailAddress
*/
@javax.annotation.Nullable
public String getBccEmailAddress() {
return bccEmailAddress;
}
public void setBccEmailAddress(String bccEmailAddress) {
this.bccEmailAddress = bccEmailAddress;
}
public PostPublicEmailTemplateRequest ccEmailAddress(String ccEmailAddress) {
this.ccEmailAddress = ccEmailAddress;
return this;
}
/**
* The email CC address.
* @return ccEmailAddress
*/
@javax.annotation.Nullable
public String getCcEmailAddress() {
return ccEmailAddress;
}
public void setCcEmailAddress(String ccEmailAddress) {
this.ccEmailAddress = ccEmailAddress;
}
public PostPublicEmailTemplateRequest ccEmailType(PostPublicEmailTemplateRequestCcEmailType ccEmailType) {
this.ccEmailType = ccEmailType;
return this;
}
/**
* Get ccEmailType
* @return ccEmailType
*/
@javax.annotation.Nullable
public PostPublicEmailTemplateRequestCcEmailType getCcEmailType() {
return ccEmailType;
}
public void setCcEmailType(PostPublicEmailTemplateRequestCcEmailType ccEmailType) {
this.ccEmailType = ccEmailType;
}
public PostPublicEmailTemplateRequest description(String description) {
this.description = description;
return this;
}
/**
* The description of the email template.
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public PostPublicEmailTemplateRequest emailBody(String emailBody) {
this.emailBody = emailBody;
return this;
}
/**
* The email body. You can add merge fields in the email object using angle brackets. You can also embed HTML tags if `isHtml` is `true`.
* @return emailBody
*/
@javax.annotation.Nonnull
public String getEmailBody() {
return emailBody;
}
public void setEmailBody(String emailBody) {
this.emailBody = emailBody;
}
public PostPublicEmailTemplateRequest emailSubject(String emailSubject) {
this.emailSubject = emailSubject;
return this;
}
/**
* The email subject. Users can add merge fields in the email subject using angle brackets.
* @return emailSubject
*/
@javax.annotation.Nonnull
public String getEmailSubject() {
return emailSubject;
}
public void setEmailSubject(String emailSubject) {
this.emailSubject = emailSubject;
}
public PostPublicEmailTemplateRequest encodingType(PostPublicEmailTemplateRequestEncodingType encodingType) {
this.encodingType = encodingType;
return this;
}
/**
* Get encodingType
* @return encodingType
*/
@javax.annotation.Nullable
public PostPublicEmailTemplateRequestEncodingType getEncodingType() {
return encodingType;
}
public void setEncodingType(PostPublicEmailTemplateRequestEncodingType encodingType) {
this.encodingType = encodingType;
}
public PostPublicEmailTemplateRequest eventCategory(BigDecimal eventCategory) {
this.eventCategory = eventCategory;
return this;
}
/**
* If you specify this field, the email template is created based on a standard event. See [Standard Event Categories](https://knowledgecenter.zuora.com/Central_Platform/Notifications/A_Standard_Events/Standard_Event_Category_Code_for_Notification_Histories_API) for all standard event category codes.
* @return eventCategory
*/
@javax.annotation.Nullable
public BigDecimal getEventCategory() {
return eventCategory;
}
public void setEventCategory(BigDecimal eventCategory) {
this.eventCategory = eventCategory;
}
public PostPublicEmailTemplateRequest eventTypeName(String eventTypeName) {
this.eventTypeName = eventTypeName;
return this;
}
/**
* The name of the custom event or custom scheduled event. If you specify this field, the email template is created based on the corresponding custom event or custom scheduled event.
* @return eventTypeName
*/
@javax.annotation.Nullable
public String getEventTypeName() {
return eventTypeName;
}
public void setEventTypeName(String eventTypeName) {
this.eventTypeName = eventTypeName;
}
public PostPublicEmailTemplateRequest eventTypeNamespace(String eventTypeNamespace) {
this.eventTypeNamespace = eventTypeNamespace;
return this;
}
/**
* The namespace of the `eventTypeName` field. The `eventTypeName` has the `user.notification` namespace by default. Note that if the `eventTypeName` is a standard event type, you must specify the `com.zuora.notification` namespace; otherwise, you will get an error. For example, if you want to create an email template on the `OrderActionProcessed` event, you must specify `com.zuora.notification` for this field.
* @return eventTypeNamespace
*/
@javax.annotation.Nullable
public String getEventTypeNamespace() {
return eventTypeNamespace;
}
public void setEventTypeNamespace(String eventTypeNamespace) {
this.eventTypeNamespace = eventTypeNamespace;
}
public PostPublicEmailTemplateRequest fromEmailAddress(String fromEmailAddress) {
this.fromEmailAddress = fromEmailAddress;
return this;
}
/**
* If fromEmailType is SpecificEmail, this field is required.
* @return fromEmailAddress
*/
@javax.annotation.Nullable
public String getFromEmailAddress() {
return fromEmailAddress;
}
public void setFromEmailAddress(String fromEmailAddress) {
this.fromEmailAddress = fromEmailAddress;
}
public PostPublicEmailTemplateRequest fromEmailType(PostPublicEmailTemplateRequestFromEmailType fromEmailType) {
this.fromEmailType = fromEmailType;
return this;
}
/**
* Get fromEmailType
* @return fromEmailType
*/
@javax.annotation.Nonnull
public PostPublicEmailTemplateRequestFromEmailType getFromEmailType() {
return fromEmailType;
}
public void setFromEmailType(PostPublicEmailTemplateRequestFromEmailType fromEmailType) {
this.fromEmailType = fromEmailType;
}
public PostPublicEmailTemplateRequest fromName(String fromName) {
this.fromName = fromName;
return this;
}
/**
* The name of the email sender.
* @return fromName
*/
@javax.annotation.Nullable
public String getFromName() {
return fromName;
}
public void setFromName(String fromName) {
this.fromName = fromName;
}
public PostPublicEmailTemplateRequest isHtml(Boolean isHtml) {
this.isHtml = isHtml;
return this;
}
/**
* Indicates whether the style of email body is HTML. The default value is `false`.
* @return isHtml
*/
@javax.annotation.Nullable
public Boolean getIsHtml() {
return isHtml;
}
public void setIsHtml(Boolean isHtml) {
this.isHtml = isHtml;
}
public PostPublicEmailTemplateRequest name(String name) {
this.name = name;
return this;
}
/**
* The name of the email template, a unique name in a tenant.
* @return name
*/
@javax.annotation.Nonnull
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public PostPublicEmailTemplateRequest replyToEmailAddress(String replyToEmailAddress) {
this.replyToEmailAddress = replyToEmailAddress;
return this;
}
/**
* If replyToEmailType is SpecificEmail, this field is required.
* @return replyToEmailAddress
*/
@javax.annotation.Nullable
public String getReplyToEmailAddress() {
return replyToEmailAddress;
}
public void setReplyToEmailAddress(String replyToEmailAddress) {
this.replyToEmailAddress = replyToEmailAddress;
}
public PostPublicEmailTemplateRequest replyToEmailType(PostPublicEmailTemplateRequestReplyToEmailType replyToEmailType) {
this.replyToEmailType = replyToEmailType;
return this;
}
/**
* Get replyToEmailType
* @return replyToEmailType
*/
@javax.annotation.Nullable
public PostPublicEmailTemplateRequestReplyToEmailType getReplyToEmailType() {
return replyToEmailType;
}
public void setReplyToEmailType(PostPublicEmailTemplateRequestReplyToEmailType replyToEmailType) {
this.replyToEmailType = replyToEmailType;
}
public PostPublicEmailTemplateRequest toEmailAddress(String toEmailAddress) {
this.toEmailAddress = toEmailAddress;
return this;
}
/**
* If toEmailType is SpecificEmail, this field is required.
* @return toEmailAddress
*/
@javax.annotation.Nullable
public String getToEmailAddress() {
return toEmailAddress;
}
public void setToEmailAddress(String toEmailAddress) {
this.toEmailAddress = toEmailAddress;
}
public PostPublicEmailTemplateRequest toEmailType(PostPublicEmailTemplateRequestToEmailType toEmailType) {
this.toEmailType = toEmailType;
return this;
}
/**
* Get toEmailType
* @return toEmailType
*/
@javax.annotation.Nonnull
public PostPublicEmailTemplateRequestToEmailType getToEmailType() {
return toEmailType;
}
public void setToEmailType(PostPublicEmailTemplateRequestToEmailType toEmailType) {
this.toEmailType = toEmailType;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the PostPublicEmailTemplateRequest instance itself
*/
public PostPublicEmailTemplateRequest putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PostPublicEmailTemplateRequest postPublicEmailTemplateRequest = (PostPublicEmailTemplateRequest) o;
return Objects.equals(this.active, postPublicEmailTemplateRequest.active) &&
Objects.equals(this.bccEmailAddress, postPublicEmailTemplateRequest.bccEmailAddress) &&
Objects.equals(this.ccEmailAddress, postPublicEmailTemplateRequest.ccEmailAddress) &&
Objects.equals(this.ccEmailType, postPublicEmailTemplateRequest.ccEmailType) &&
Objects.equals(this.description, postPublicEmailTemplateRequest.description) &&
Objects.equals(this.emailBody, postPublicEmailTemplateRequest.emailBody) &&
Objects.equals(this.emailSubject, postPublicEmailTemplateRequest.emailSubject) &&
Objects.equals(this.encodingType, postPublicEmailTemplateRequest.encodingType) &&
Objects.equals(this.eventCategory, postPublicEmailTemplateRequest.eventCategory) &&
Objects.equals(this.eventTypeName, postPublicEmailTemplateRequest.eventTypeName) &&
Objects.equals(this.eventTypeNamespace, postPublicEmailTemplateRequest.eventTypeNamespace) &&
Objects.equals(this.fromEmailAddress, postPublicEmailTemplateRequest.fromEmailAddress) &&
Objects.equals(this.fromEmailType, postPublicEmailTemplateRequest.fromEmailType) &&
Objects.equals(this.fromName, postPublicEmailTemplateRequest.fromName) &&
Objects.equals(this.isHtml, postPublicEmailTemplateRequest.isHtml) &&
Objects.equals(this.name, postPublicEmailTemplateRequest.name) &&
Objects.equals(this.replyToEmailAddress, postPublicEmailTemplateRequest.replyToEmailAddress) &&
Objects.equals(this.replyToEmailType, postPublicEmailTemplateRequest.replyToEmailType) &&
Objects.equals(this.toEmailAddress, postPublicEmailTemplateRequest.toEmailAddress) &&
Objects.equals(this.toEmailType, postPublicEmailTemplateRequest.toEmailType)&&
Objects.equals(this.additionalProperties, postPublicEmailTemplateRequest.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(active, bccEmailAddress, ccEmailAddress, ccEmailType, description, emailBody, emailSubject, encodingType, eventCategory, eventTypeName, eventTypeNamespace, fromEmailAddress, fromEmailType, fromName, isHtml, name, replyToEmailAddress, replyToEmailType, toEmailAddress, toEmailType, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PostPublicEmailTemplateRequest {\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append(" bccEmailAddress: ").append(toIndentedString(bccEmailAddress)).append("\n");
sb.append(" ccEmailAddress: ").append(toIndentedString(ccEmailAddress)).append("\n");
sb.append(" ccEmailType: ").append(toIndentedString(ccEmailType)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" emailBody: ").append(toIndentedString(emailBody)).append("\n");
sb.append(" emailSubject: ").append(toIndentedString(emailSubject)).append("\n");
sb.append(" encodingType: ").append(toIndentedString(encodingType)).append("\n");
sb.append(" eventCategory: ").append(toIndentedString(eventCategory)).append("\n");
sb.append(" eventTypeName: ").append(toIndentedString(eventTypeName)).append("\n");
sb.append(" eventTypeNamespace: ").append(toIndentedString(eventTypeNamespace)).append("\n");
sb.append(" fromEmailAddress: ").append(toIndentedString(fromEmailAddress)).append("\n");
sb.append(" fromEmailType: ").append(toIndentedString(fromEmailType)).append("\n");
sb.append(" fromName: ").append(toIndentedString(fromName)).append("\n");
sb.append(" isHtml: ").append(toIndentedString(isHtml)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" replyToEmailAddress: ").append(toIndentedString(replyToEmailAddress)).append("\n");
sb.append(" replyToEmailType: ").append(toIndentedString(replyToEmailType)).append("\n");
sb.append(" toEmailAddress: ").append(toIndentedString(toEmailAddress)).append("\n");
sb.append(" toEmailType: ").append(toIndentedString(toEmailType)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("active");
openapiFields.add("bccEmailAddress");
openapiFields.add("ccEmailAddress");
openapiFields.add("ccEmailType");
openapiFields.add("description");
openapiFields.add("emailBody");
openapiFields.add("emailSubject");
openapiFields.add("encodingType");
openapiFields.add("eventCategory");
openapiFields.add("eventTypeName");
openapiFields.add("eventTypeNamespace");
openapiFields.add("fromEmailAddress");
openapiFields.add("fromEmailType");
openapiFields.add("fromName");
openapiFields.add("isHtml");
openapiFields.add("name");
openapiFields.add("replyToEmailAddress");
openapiFields.add("replyToEmailType");
openapiFields.add("toEmailAddress");
openapiFields.add("toEmailType");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("emailBody");
openapiRequiredFields.add("emailSubject");
openapiRequiredFields.add("fromEmailType");
openapiRequiredFields.add("name");
openapiRequiredFields.add("toEmailType");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to PostPublicEmailTemplateRequest
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!PostPublicEmailTemplateRequest.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in PostPublicEmailTemplateRequest is not found in the empty JSON string", PostPublicEmailTemplateRequest.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : PostPublicEmailTemplateRequest.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("bccEmailAddress") != null && !jsonObj.get("bccEmailAddress").isJsonNull()) && !jsonObj.get("bccEmailAddress").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bccEmailAddress` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bccEmailAddress").toString()));
}
if ((jsonObj.get("ccEmailAddress") != null && !jsonObj.get("ccEmailAddress").isJsonNull()) && !jsonObj.get("ccEmailAddress").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ccEmailAddress` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ccEmailAddress").toString()));
}
// validate the optional field `ccEmailType`
if (jsonObj.get("ccEmailType") != null && !jsonObj.get("ccEmailType").isJsonNull()) {
PostPublicEmailTemplateRequestCcEmailType.validateJsonElement(jsonObj.get("ccEmailType"));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if (!jsonObj.get("emailBody").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `emailBody` to be a primitive type in the JSON string but got `%s`", jsonObj.get("emailBody").toString()));
}
if (!jsonObj.get("emailSubject").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `emailSubject` to be a primitive type in the JSON string but got `%s`", jsonObj.get("emailSubject").toString()));
}
// validate the optional field `encodingType`
if (jsonObj.get("encodingType") != null && !jsonObj.get("encodingType").isJsonNull()) {
PostPublicEmailTemplateRequestEncodingType.validateJsonElement(jsonObj.get("encodingType"));
}
if ((jsonObj.get("eventTypeName") != null && !jsonObj.get("eventTypeName").isJsonNull()) && !jsonObj.get("eventTypeName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `eventTypeName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("eventTypeName").toString()));
}
if ((jsonObj.get("eventTypeNamespace") != null && !jsonObj.get("eventTypeNamespace").isJsonNull()) && !jsonObj.get("eventTypeNamespace").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `eventTypeNamespace` to be a primitive type in the JSON string but got `%s`", jsonObj.get("eventTypeNamespace").toString()));
}
if ((jsonObj.get("fromEmailAddress") != null && !jsonObj.get("fromEmailAddress").isJsonNull()) && !jsonObj.get("fromEmailAddress").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `fromEmailAddress` to be a primitive type in the JSON string but got `%s`", jsonObj.get("fromEmailAddress").toString()));
}
if (!jsonObj.get("fromEmailType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `fromEmailType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("fromEmailType").toString()));
}
// validate the required field `fromEmailType`
PostPublicEmailTemplateRequestFromEmailType.validateJsonElement(jsonObj.get("fromEmailType"));
if ((jsonObj.get("fromName") != null && !jsonObj.get("fromName").isJsonNull()) && !jsonObj.get("fromName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `fromName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("fromName").toString()));
}
if (!jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if ((jsonObj.get("replyToEmailAddress") != null && !jsonObj.get("replyToEmailAddress").isJsonNull()) && !jsonObj.get("replyToEmailAddress").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `replyToEmailAddress` to be a primitive type in the JSON string but got `%s`", jsonObj.get("replyToEmailAddress").toString()));
}
if ((jsonObj.get("replyToEmailType") != null && !jsonObj.get("replyToEmailType").isJsonNull()) && !jsonObj.get("replyToEmailType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `replyToEmailType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("replyToEmailType").toString()));
}
// validate the optional field `replyToEmailType`
if (jsonObj.get("replyToEmailType") != null && !jsonObj.get("replyToEmailType").isJsonNull()) {
PostPublicEmailTemplateRequestReplyToEmailType.validateJsonElement(jsonObj.get("replyToEmailType"));
}
if ((jsonObj.get("toEmailAddress") != null && !jsonObj.get("toEmailAddress").isJsonNull()) && !jsonObj.get("toEmailAddress").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `toEmailAddress` to be a primitive type in the JSON string but got `%s`", jsonObj.get("toEmailAddress").toString()));
}
if (!jsonObj.get("toEmailType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `toEmailType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("toEmailType").toString()));
}
// validate the required field `toEmailType`
PostPublicEmailTemplateRequestToEmailType.validateJsonElement(jsonObj.get("toEmailType"));
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!PostPublicEmailTemplateRequest.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'PostPublicEmailTemplateRequest' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(PostPublicEmailTemplateRequest.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, PostPublicEmailTemplateRequest value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public PostPublicEmailTemplateRequest read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
PostPublicEmailTemplateRequest instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of PostPublicEmailTemplateRequest given an JSON string
*
* @param jsonString JSON string
* @return An instance of PostPublicEmailTemplateRequest
* @throws IOException if the JSON string is invalid with respect to PostPublicEmailTemplateRequest
*/
public static PostPublicEmailTemplateRequest fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, PostPublicEmailTemplateRequest.class);
}
/**
* Convert an instance of PostPublicEmailTemplateRequest to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy