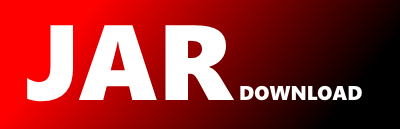
com.zuora.model.PreviewSubscriptionRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.CreateSubscriptionRatePlan;
import com.zuora.model.PreviewSubscriptionAccountInfo;
import java.io.IOException;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* PreviewSubscriptionRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class PreviewSubscriptionRequest {
public static final String SERIALIZED_NAME_ACCOUNT_KEY = "accountKey";
@SerializedName(SERIALIZED_NAME_ACCOUNT_KEY)
private String accountKey;
public static final String SERIALIZED_NAME_CONTRACT_EFFECTIVE_DATE = "contractEffectiveDate";
@SerializedName(SERIALIZED_NAME_CONTRACT_EFFECTIVE_DATE)
private LocalDate contractEffectiveDate;
public static final String SERIALIZED_NAME_CUSTOMER_ACCEPTANCE_DATE = "customerAcceptanceDate";
@SerializedName(SERIALIZED_NAME_CUSTOMER_ACCEPTANCE_DATE)
private LocalDate customerAcceptanceDate;
public static final String SERIALIZED_NAME_DOCUMENT_DATE = "documentDate";
@SerializedName(SERIALIZED_NAME_DOCUMENT_DATE)
private LocalDate documentDate;
public static final String SERIALIZED_NAME_INCLUDE_EXISTING_DRAFT_DOC_ITEMS = "includeExistingDraftDocItems";
@SerializedName(SERIALIZED_NAME_INCLUDE_EXISTING_DRAFT_DOC_ITEMS)
private Boolean includeExistingDraftDocItems;
public static final String SERIALIZED_NAME_INCLUDE_EXISTING_DRAFT_INVOICE_ITEMS = "includeExistingDraftInvoiceItems";
@Deprecated
@SerializedName(SERIALIZED_NAME_INCLUDE_EXISTING_DRAFT_INVOICE_ITEMS)
private Boolean includeExistingDraftInvoiceItems;
public static final String SERIALIZED_NAME_INITIAL_TERM = "initialTerm";
@SerializedName(SERIALIZED_NAME_INITIAL_TERM)
private Long initialTerm;
public static final String SERIALIZED_NAME_INITIAL_TERM_PERIOD_TYPE = "initialTermPeriodType";
@SerializedName(SERIALIZED_NAME_INITIAL_TERM_PERIOD_TYPE)
private String initialTermPeriodType;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_KEY = "invoiceOwnerAccountKey";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_KEY)
private String invoiceOwnerAccountKey;
public static final String SERIALIZED_NAME_INVOICE_TARGET_DATE = "invoiceTargetDate";
@Deprecated
@SerializedName(SERIALIZED_NAME_INVOICE_TARGET_DATE)
private LocalDate invoiceTargetDate;
public static final String SERIALIZED_NAME_NOTES = "notes";
@SerializedName(SERIALIZED_NAME_NOTES)
private String notes;
public static final String SERIALIZED_NAME_PREVIEW_ACCOUNT_INFO = "previewAccountInfo";
@SerializedName(SERIALIZED_NAME_PREVIEW_ACCOUNT_INFO)
private PreviewSubscriptionAccountInfo previewAccountInfo;
public static final String SERIALIZED_NAME_PREVIEW_TYPE = "previewType";
@SerializedName(SERIALIZED_NAME_PREVIEW_TYPE)
private String previewType;
public static final String SERIALIZED_NAME_SERVICE_ACTIVATION_DATE = "serviceActivationDate";
@SerializedName(SERIALIZED_NAME_SERVICE_ACTIVATION_DATE)
private LocalDate serviceActivationDate;
public static final String SERIALIZED_NAME_SUBSCRIBE_TO_RATE_PLANS = "subscribeToRatePlans";
@SerializedName(SERIALIZED_NAME_SUBSCRIBE_TO_RATE_PLANS)
private List subscribeToRatePlans;
public static final String SERIALIZED_NAME_TARGET_DATE = "targetDate";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public static final String SERIALIZED_NAME_TERM_START_DATE = "termStartDate";
@SerializedName(SERIALIZED_NAME_TERM_START_DATE)
private LocalDate termStartDate;
public static final String SERIALIZED_NAME_TERM_TYPE = "termType";
@SerializedName(SERIALIZED_NAME_TERM_TYPE)
private String termType;
public PreviewSubscriptionRequest() {
}
public PreviewSubscriptionRequest accountKey(String accountKey) {
this.accountKey = accountKey;
return this;
}
/**
* Customer account number or ID. You must specify the account information either in this field or in the `previewAccountInfo` field with the following conditions: * If you already have a customer account, specify the account number or ID in this field. * If you do not have a customer account, provide account information in the `previewAccountInfo` field.
* @return accountKey
*/
@javax.annotation.Nullable
public String getAccountKey() {
return accountKey;
}
public void setAccountKey(String accountKey) {
this.accountKey = accountKey;
}
public PreviewSubscriptionRequest contractEffectiveDate(LocalDate contractEffectiveDate) {
this.contractEffectiveDate = contractEffectiveDate;
return this;
}
/**
* Effective contract date for this subscription, as yyyy-mm-dd.
* @return contractEffectiveDate
*/
@javax.annotation.Nonnull
public LocalDate getContractEffectiveDate() {
return contractEffectiveDate;
}
public void setContractEffectiveDate(LocalDate contractEffectiveDate) {
this.contractEffectiveDate = contractEffectiveDate;
}
public PreviewSubscriptionRequest customerAcceptanceDate(LocalDate customerAcceptanceDate) {
this.customerAcceptanceDate = customerAcceptanceDate;
return this;
}
/**
* The date on which the services or products within a subscription have been accepted by the customer, as yyyy-mm-dd. Default value is dependent on the value of other fields. See **Notes** section for more details.
* @return customerAcceptanceDate
*/
@javax.annotation.Nullable
public LocalDate getCustomerAcceptanceDate() {
return customerAcceptanceDate;
}
public void setCustomerAcceptanceDate(LocalDate customerAcceptanceDate) {
this.customerAcceptanceDate = customerAcceptanceDate;
}
public PreviewSubscriptionRequest documentDate(LocalDate documentDate) {
this.documentDate = documentDate;
return this;
}
/**
* The date of the billing document, in `yyyy-mm-dd` format. It represents the invoice date for invoices, credit memo date for credit memos, and debit memo date for debit memos. - If this field is specified, the specified date is used as the billing document date. - If this field is not specified, the date specified in the `targetDate` is used as the billing document date.
* @return documentDate
*/
@javax.annotation.Nullable
public LocalDate getDocumentDate() {
return documentDate;
}
public void setDocumentDate(LocalDate documentDate) {
this.documentDate = documentDate;
}
public PreviewSubscriptionRequest includeExistingDraftDocItems(Boolean includeExistingDraftDocItems) {
this.includeExistingDraftDocItems = includeExistingDraftDocItems;
return this;
}
/**
* Specifies whether to include draft invoice items in subscription previews. Values are: * `true` (default). Includes draft invoice items in the preview result. * `false`. Excludes draft invoice items in the preview result. **Note:** This field is in Zuora REST API version control. Supported minor versions are 207.0 or later. To use this field in the method, you must set the **zuora-version** parameter to the minor version number in the request header. See [Zuora REST API Versions](https://www.zuora.com/developer/api-references/api/overview/#section/API-Versions) for more information.
* @return includeExistingDraftDocItems
*/
@javax.annotation.Nullable
public Boolean getIncludeExistingDraftDocItems() {
return includeExistingDraftDocItems;
}
public void setIncludeExistingDraftDocItems(Boolean includeExistingDraftDocItems) {
this.includeExistingDraftDocItems = includeExistingDraftDocItems;
}
@Deprecated
public PreviewSubscriptionRequest includeExistingDraftInvoiceItems(Boolean includeExistingDraftInvoiceItems) {
this.includeExistingDraftInvoiceItems = includeExistingDraftInvoiceItems;
return this;
}
/**
* Specifies whether to include draft invoice items in previews. Values are: * `true` (default). Includes draft invoice items in the preview result. * `false`. Excludes draft invoice items in the preview result. **Note:** This field is in Zuora REST API version control. Supported minor versions are 186.0, 187.0, 188.0, 189.0, 196.0, and 206.0. See [Zuora REST API Versions](https://www.zuora.com/developer/api-references/api/overview/#section/API-Versions) for more information.
* @return includeExistingDraftInvoiceItems
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public Boolean getIncludeExistingDraftInvoiceItems() {
return includeExistingDraftInvoiceItems;
}
@Deprecated
public void setIncludeExistingDraftInvoiceItems(Boolean includeExistingDraftInvoiceItems) {
this.includeExistingDraftInvoiceItems = includeExistingDraftInvoiceItems;
}
public PreviewSubscriptionRequest initialTerm(Long initialTerm) {
this.initialTerm = initialTerm;
return this;
}
/**
* Duration of the first term of the subscription, in whole months. If `termType` is `TERMED`, then this field is required, and the value must be greater than `0`. If `termType` is `EVERGREEN`, this field is ignored.
* @return initialTerm
*/
@javax.annotation.Nullable
public Long getInitialTerm() {
return initialTerm;
}
public void setInitialTerm(Long initialTerm) {
this.initialTerm = initialTerm;
}
public PreviewSubscriptionRequest initialTermPeriodType(String initialTermPeriodType) {
this.initialTermPeriodType = initialTermPeriodType;
return this;
}
/**
* The period type of the initial term. Supported values are: * `Month` * `Year` * `Day` * `Week` The default period type is `Month`.
* @return initialTermPeriodType
*/
@javax.annotation.Nullable
public String getInitialTermPeriodType() {
return initialTermPeriodType;
}
public void setInitialTermPeriodType(String initialTermPeriodType) {
this.initialTermPeriodType = initialTermPeriodType;
}
public PreviewSubscriptionRequest invoiceOwnerAccountKey(String invoiceOwnerAccountKey) {
this.invoiceOwnerAccountKey = invoiceOwnerAccountKey;
return this;
}
/**
* Invoice owner account number or ID. **Note:** This feature is in **Limited Availability**. If you wish to have access to the feature, submit a request at [Zuora Global Support](http://support.zuora.com/).
* @return invoiceOwnerAccountKey
*/
@javax.annotation.Nullable
public String getInvoiceOwnerAccountKey() {
return invoiceOwnerAccountKey;
}
public void setInvoiceOwnerAccountKey(String invoiceOwnerAccountKey) {
this.invoiceOwnerAccountKey = invoiceOwnerAccountKey;
}
@Deprecated
public PreviewSubscriptionRequest invoiceTargetDate(LocalDate invoiceTargetDate) {
this.invoiceTargetDate = invoiceTargetDate;
return this;
}
/**
* Date through which to calculate charges if an invoice is generated, as yyyy-mm-dd. Default is current date. **Note:** This field is in Zuora REST API version control. Supported minor versions are 186.0, 187.0, 188.0, 189.0, 196.0, and 206.0. See [Zuora REST API Versions](https://www.zuora.com/developer/api-references/api/overview/#section/API-Versions) for more information.
* @return invoiceTargetDate
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public LocalDate getInvoiceTargetDate() {
return invoiceTargetDate;
}
@Deprecated
public void setInvoiceTargetDate(LocalDate invoiceTargetDate) {
this.invoiceTargetDate = invoiceTargetDate;
}
public PreviewSubscriptionRequest notes(String notes) {
this.notes = notes;
return this;
}
/**
* String of up to 500 characters.
* @return notes
*/
@javax.annotation.Nullable
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
this.notes = notes;
}
public PreviewSubscriptionRequest previewAccountInfo(PreviewSubscriptionAccountInfo previewAccountInfo) {
this.previewAccountInfo = previewAccountInfo;
return this;
}
/**
* Get previewAccountInfo
* @return previewAccountInfo
*/
@javax.annotation.Nullable
public PreviewSubscriptionAccountInfo getPreviewAccountInfo() {
return previewAccountInfo;
}
public void setPreviewAccountInfo(PreviewSubscriptionAccountInfo previewAccountInfo) {
this.previewAccountInfo = previewAccountInfo;
}
public PreviewSubscriptionRequest previewType(String previewType) {
this.previewType = previewType;
return this;
}
/**
* The type of preview you will receive. This field is in Zuora REST API version control. The supported values of this field depend on the REST API minor version you specified in the request header. * If you do not specify the REST API minor version or specify the minor version number to one of following values in the request header: * 186.0 * 187.0 * 188.0 * 189.0 * 196.0 * 206.0 The following values are supported in the **previewType** field: * InvoiceItem * ChargeMetrics * InvoiceItemChargeMetrics The default value is InvoiceItem. * If you specify the REST API minor version to 207.0 or later in the request header, the following values are supported in the **previewType** field: - LegalDoc - ChargeMetrics - LegalDocChargeMetrics The default value is LegalDoc. See [Zuora REST API Versions](https://www.zuora.com/developer/api-references/api/overview/#section/API-Versions) for more information.
* @return previewType
*/
@javax.annotation.Nullable
public String getPreviewType() {
return previewType;
}
public void setPreviewType(String previewType) {
this.previewType = previewType;
}
public PreviewSubscriptionRequest serviceActivationDate(LocalDate serviceActivationDate) {
this.serviceActivationDate = serviceActivationDate;
return this;
}
/**
* The date on which the services or products within a subscription have been activated and access has been provided to the customer, as yyyy-mm-dd. Default value is dependent on the value of other fields. See **Notes** section for more details.
* @return serviceActivationDate
*/
@javax.annotation.Nullable
public LocalDate getServiceActivationDate() {
return serviceActivationDate;
}
public void setServiceActivationDate(LocalDate serviceActivationDate) {
this.serviceActivationDate = serviceActivationDate;
}
public PreviewSubscriptionRequest subscribeToRatePlans(List subscribeToRatePlans) {
this.subscribeToRatePlans = subscribeToRatePlans;
return this;
}
public PreviewSubscriptionRequest addSubscribeToRatePlansItem(CreateSubscriptionRatePlan subscribeToRatePlansItem) {
if (this.subscribeToRatePlans == null) {
this.subscribeToRatePlans = new ArrayList<>();
}
this.subscribeToRatePlans.add(subscribeToRatePlansItem);
return this;
}
/**
* Container for one or more rate plans for this subscription.
* @return subscribeToRatePlans
*/
@javax.annotation.Nonnull
public List getSubscribeToRatePlans() {
return subscribeToRatePlans;
}
public void setSubscribeToRatePlans(List subscribeToRatePlans) {
this.subscribeToRatePlans = subscribeToRatePlans;
}
public PreviewSubscriptionRequest targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* Date through which to calculate charges if an invoice is generated, as yyyy-mm-dd. Default is current date. **Note:** This field is in Zuora REST API version control. Supported minor versions are 207.0 or later. To use this field in the method, you must set the **zuora-version** parameter to the minor version number in the request header. See [Zuora REST API Versions](https://www.zuora.com/developer/api-references/api/overview/#section/API-Versions) for more information.
* @return targetDate
*/
@javax.annotation.Nullable
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public PreviewSubscriptionRequest termStartDate(LocalDate termStartDate) {
this.termStartDate = termStartDate;
return this;
}
/**
* The date on which the subscription term begins, as yyyy-mm-dd. If this is a renewal subscription, this date is different from the subscription start date.
* @return termStartDate
*/
@javax.annotation.Nullable
public LocalDate getTermStartDate() {
return termStartDate;
}
public void setTermStartDate(LocalDate termStartDate) {
this.termStartDate = termStartDate;
}
public PreviewSubscriptionRequest termType(String termType) {
this.termType = termType;
return this;
}
/**
* Possible values are: `TERMED`, `EVERGREEN`.
* @return termType
*/
@javax.annotation.Nonnull
public String getTermType() {
return termType;
}
public void setTermType(String termType) {
this.termType = termType;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the PreviewSubscriptionRequest instance itself
*/
public PreviewSubscriptionRequest putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PreviewSubscriptionRequest previewSubscriptionRequest = (PreviewSubscriptionRequest) o;
return Objects.equals(this.accountKey, previewSubscriptionRequest.accountKey) &&
Objects.equals(this.contractEffectiveDate, previewSubscriptionRequest.contractEffectiveDate) &&
Objects.equals(this.customerAcceptanceDate, previewSubscriptionRequest.customerAcceptanceDate) &&
Objects.equals(this.documentDate, previewSubscriptionRequest.documentDate) &&
Objects.equals(this.includeExistingDraftDocItems, previewSubscriptionRequest.includeExistingDraftDocItems) &&
Objects.equals(this.includeExistingDraftInvoiceItems, previewSubscriptionRequest.includeExistingDraftInvoiceItems) &&
Objects.equals(this.initialTerm, previewSubscriptionRequest.initialTerm) &&
Objects.equals(this.initialTermPeriodType, previewSubscriptionRequest.initialTermPeriodType) &&
Objects.equals(this.invoiceOwnerAccountKey, previewSubscriptionRequest.invoiceOwnerAccountKey) &&
Objects.equals(this.invoiceTargetDate, previewSubscriptionRequest.invoiceTargetDate) &&
Objects.equals(this.notes, previewSubscriptionRequest.notes) &&
Objects.equals(this.previewAccountInfo, previewSubscriptionRequest.previewAccountInfo) &&
Objects.equals(this.previewType, previewSubscriptionRequest.previewType) &&
Objects.equals(this.serviceActivationDate, previewSubscriptionRequest.serviceActivationDate) &&
Objects.equals(this.subscribeToRatePlans, previewSubscriptionRequest.subscribeToRatePlans) &&
Objects.equals(this.targetDate, previewSubscriptionRequest.targetDate) &&
Objects.equals(this.termStartDate, previewSubscriptionRequest.termStartDate) &&
Objects.equals(this.termType, previewSubscriptionRequest.termType)&&
Objects.equals(this.additionalProperties, previewSubscriptionRequest.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(accountKey, contractEffectiveDate, customerAcceptanceDate, documentDate, includeExistingDraftDocItems, includeExistingDraftInvoiceItems, initialTerm, initialTermPeriodType, invoiceOwnerAccountKey, invoiceTargetDate, notes, previewAccountInfo, previewType, serviceActivationDate, subscribeToRatePlans, targetDate, termStartDate, termType, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PreviewSubscriptionRequest {\n");
sb.append(" accountKey: ").append(toIndentedString(accountKey)).append("\n");
sb.append(" contractEffectiveDate: ").append(toIndentedString(contractEffectiveDate)).append("\n");
sb.append(" customerAcceptanceDate: ").append(toIndentedString(customerAcceptanceDate)).append("\n");
sb.append(" documentDate: ").append(toIndentedString(documentDate)).append("\n");
sb.append(" includeExistingDraftDocItems: ").append(toIndentedString(includeExistingDraftDocItems)).append("\n");
sb.append(" includeExistingDraftInvoiceItems: ").append(toIndentedString(includeExistingDraftInvoiceItems)).append("\n");
sb.append(" initialTerm: ").append(toIndentedString(initialTerm)).append("\n");
sb.append(" initialTermPeriodType: ").append(toIndentedString(initialTermPeriodType)).append("\n");
sb.append(" invoiceOwnerAccountKey: ").append(toIndentedString(invoiceOwnerAccountKey)).append("\n");
sb.append(" invoiceTargetDate: ").append(toIndentedString(invoiceTargetDate)).append("\n");
sb.append(" notes: ").append(toIndentedString(notes)).append("\n");
sb.append(" previewAccountInfo: ").append(toIndentedString(previewAccountInfo)).append("\n");
sb.append(" previewType: ").append(toIndentedString(previewType)).append("\n");
sb.append(" serviceActivationDate: ").append(toIndentedString(serviceActivationDate)).append("\n");
sb.append(" subscribeToRatePlans: ").append(toIndentedString(subscribeToRatePlans)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" termStartDate: ").append(toIndentedString(termStartDate)).append("\n");
sb.append(" termType: ").append(toIndentedString(termType)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("accountKey");
openapiFields.add("contractEffectiveDate");
openapiFields.add("customerAcceptanceDate");
openapiFields.add("documentDate");
openapiFields.add("includeExistingDraftDocItems");
openapiFields.add("includeExistingDraftInvoiceItems");
openapiFields.add("initialTerm");
openapiFields.add("initialTermPeriodType");
openapiFields.add("invoiceOwnerAccountKey");
openapiFields.add("invoiceTargetDate");
openapiFields.add("notes");
openapiFields.add("previewAccountInfo");
openapiFields.add("previewType");
openapiFields.add("serviceActivationDate");
openapiFields.add("subscribeToRatePlans");
openapiFields.add("targetDate");
openapiFields.add("termStartDate");
openapiFields.add("termType");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("contractEffectiveDate");
openapiRequiredFields.add("subscribeToRatePlans");
openapiRequiredFields.add("termType");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to PreviewSubscriptionRequest
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!PreviewSubscriptionRequest.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in PreviewSubscriptionRequest is not found in the empty JSON string", PreviewSubscriptionRequest.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : PreviewSubscriptionRequest.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("accountKey") != null && !jsonObj.get("accountKey").isJsonNull()) && !jsonObj.get("accountKey").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountKey` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountKey").toString()));
}
if ((jsonObj.get("initialTermPeriodType") != null && !jsonObj.get("initialTermPeriodType").isJsonNull()) && !jsonObj.get("initialTermPeriodType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `initialTermPeriodType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("initialTermPeriodType").toString()));
}
if ((jsonObj.get("invoiceOwnerAccountKey") != null && !jsonObj.get("invoiceOwnerAccountKey").isJsonNull()) && !jsonObj.get("invoiceOwnerAccountKey").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceOwnerAccountKey` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceOwnerAccountKey").toString()));
}
if ((jsonObj.get("notes") != null && !jsonObj.get("notes").isJsonNull()) && !jsonObj.get("notes").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `notes` to be a primitive type in the JSON string but got `%s`", jsonObj.get("notes").toString()));
}
// validate the optional field `previewAccountInfo`
if (jsonObj.get("previewAccountInfo") != null && !jsonObj.get("previewAccountInfo").isJsonNull()) {
PreviewSubscriptionAccountInfo.validateJsonElement(jsonObj.get("previewAccountInfo"));
}
if ((jsonObj.get("previewType") != null && !jsonObj.get("previewType").isJsonNull()) && !jsonObj.get("previewType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `previewType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("previewType").toString()));
}
// ensure the json data is an array
if (!jsonObj.get("subscribeToRatePlans").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `subscribeToRatePlans` to be an array in the JSON string but got `%s`", jsonObj.get("subscribeToRatePlans").toString()));
}
JsonArray jsonArraysubscribeToRatePlans = jsonObj.getAsJsonArray("subscribeToRatePlans");
// validate the required field `subscribeToRatePlans` (array)
for (int i = 0; i < jsonArraysubscribeToRatePlans.size(); i++) {
CreateSubscriptionRatePlan.validateJsonElement(jsonArraysubscribeToRatePlans.get(i));
};
if (!jsonObj.get("termType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `termType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("termType").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!PreviewSubscriptionRequest.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'PreviewSubscriptionRequest' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(PreviewSubscriptionRequest.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, PreviewSubscriptionRequest value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public PreviewSubscriptionRequest read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
PreviewSubscriptionRequest instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of PreviewSubscriptionRequest given an JSON string
*
* @param jsonString JSON string
* @return An instance of PreviewSubscriptionRequest
* @throws IOException if the JSON string is invalid with respect to PreviewSubscriptionRequest
*/
public static PreviewSubscriptionRequest fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, PreviewSubscriptionRequest.class);
}
/**
* Convert an instance of PreviewSubscriptionRequest to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy