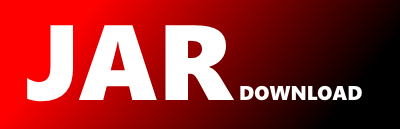
com.zuora.model.RatePlanChargeSegmentInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.ApplyDiscountTo;
import com.zuora.model.BillingPeriodAlignment;
import com.zuora.model.ChargeFunction;
import com.zuora.model.ChargeModelConfigurationForSubscription;
import com.zuora.model.CommitmentType;
import com.zuora.model.DeliverySchedule;
import com.zuora.model.DiscountApplyDetail;
import com.zuora.model.DiscountLevel;
import com.zuora.model.EndDateCondition;
import com.zuora.model.IntervalPricing;
import com.zuora.model.PriceChangeOption;
import com.zuora.model.RatePlanChargeTier;
import com.zuora.model.TriggerEvent;
import com.zuora.model.UpToPeriodsType;
import com.zuora.model.ValidityPeriodType;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* RatePlanChargeSegmentInfo
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class RatePlanChargeSegmentInfo {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_AMENDED_BY_ORDER_ON = "amendedByOrderOn";
@SerializedName(SERIALIZED_NAME_AMENDED_BY_ORDER_ON)
private LocalDate amendedByOrderOn;
public static final String SERIALIZED_NAME_APPLY_DISCOUNT_TO = "applyDiscountTo";
@SerializedName(SERIALIZED_NAME_APPLY_DISCOUNT_TO)
private ApplyDiscountTo applyDiscountTo;
public static final String SERIALIZED_NAME_CHARGE_FUNCTION = "chargeFunction";
@SerializedName(SERIALIZED_NAME_CHARGE_FUNCTION)
private ChargeFunction chargeFunction;
public static final String SERIALIZED_NAME_CHARGE_MODEL_CONFIGURATION = "chargeModelConfiguration";
@SerializedName(SERIALIZED_NAME_CHARGE_MODEL_CONFIGURATION)
private ChargeModelConfigurationForSubscription chargeModelConfiguration;
public static final String SERIALIZED_NAME_CHARGED_THROUGH_DATE = "chargedThroughDate";
@SerializedName(SERIALIZED_NAME_CHARGED_THROUGH_DATE)
private LocalDate chargedThroughDate;
public static final String SERIALIZED_NAME_COMMITMENT_TYPE = "commitmentType";
@SerializedName(SERIALIZED_NAME_COMMITMENT_TYPE)
private CommitmentType commitmentType;
public static final String SERIALIZED_NAME_PREPAID_COMMITTED_AMOUNT = "prepaidCommittedAmount";
@SerializedName(SERIALIZED_NAME_PREPAID_COMMITTED_AMOUNT)
private String prepaidCommittedAmount;
/**
* **Note**: This field is only available if you have the [Prepaid with Drawdown](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown) feature enabled. To use this field, you must set the `X-Zuora-WSDL-Version` request header to 114 or higher. Otherwise, an error occurs. The way to calculate credit. See [Credit Option](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown/Create_prepayment_charge#Credit_Option) for more information.
*/
@JsonAdapter(CreditOptionEnum.Adapter.class)
public enum CreditOptionEnum {
TIMEBASED("TimeBased"),
CONSUMPTIONBASED("ConsumptionBased"),
FULLCREDITBACK("FullCreditBack");
private String value;
CreditOptionEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static CreditOptionEnum fromValue(String value) {
for (CreditOptionEnum b : CreditOptionEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final CreditOptionEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public CreditOptionEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return CreditOptionEnum.fromValue(value);
}
}
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
String value = jsonElement.getAsString();
CreditOptionEnum.fromValue(value);
}
}
public static final String SERIALIZED_NAME_CREDIT_OPTION = "creditOption";
@SerializedName(SERIALIZED_NAME_CREDIT_OPTION)
private CreditOptionEnum creditOption;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_DELIVERY_SCHEDULE = "deliverySchedule";
@SerializedName(SERIALIZED_NAME_DELIVERY_SCHEDULE)
private DeliverySchedule deliverySchedule;
public static final String SERIALIZED_NAME_NUMBER_OF_DELIVERIES = "numberOfDeliveries";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_DELIVERIES)
private BigDecimal numberOfDeliveries;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_DISCOUNT_AMOUNT = "discountAmount";
@SerializedName(SERIALIZED_NAME_DISCOUNT_AMOUNT)
private BigDecimal discountAmount;
public static final String SERIALIZED_NAME_DISCOUNT_APPLY_DETAILS = "discountApplyDetails";
@SerializedName(SERIALIZED_NAME_DISCOUNT_APPLY_DETAILS)
private List discountApplyDetails;
public static final String SERIALIZED_NAME_DISCOUNT_CLASS = "discountClass";
@SerializedName(SERIALIZED_NAME_DISCOUNT_CLASS)
private String discountClass;
public static final String SERIALIZED_NAME_DISCOUNT_LEVEL = "discountLevel";
@SerializedName(SERIALIZED_NAME_DISCOUNT_LEVEL)
private DiscountLevel discountLevel;
public static final String SERIALIZED_NAME_DISCOUNT_PERCENTAGE = "discountPercentage";
@SerializedName(SERIALIZED_NAME_DISCOUNT_PERCENTAGE)
private BigDecimal discountPercentage;
public static final String SERIALIZED_NAME_APPLY_TO_BILLING_PERIOD_PARTIALLY = "applyToBillingPeriodPartially";
@SerializedName(SERIALIZED_NAME_APPLY_TO_BILLING_PERIOD_PARTIALLY)
private Boolean applyToBillingPeriodPartially;
public static final String SERIALIZED_NAME_DMRC = "dmrc";
@SerializedName(SERIALIZED_NAME_DMRC)
private BigDecimal dmrc;
public static final String SERIALIZED_NAME_DONE = "done";
@SerializedName(SERIALIZED_NAME_DONE)
private Boolean done;
public static final String SERIALIZED_NAME_DRAWDOWN_RATE = "drawdownRate";
@SerializedName(SERIALIZED_NAME_DRAWDOWN_RATE)
private BigDecimal drawdownRate;
public static final String SERIALIZED_NAME_DRAWDOWN_UOM = "drawdownUom";
@SerializedName(SERIALIZED_NAME_DRAWDOWN_UOM)
private String drawdownUom;
public static final String SERIALIZED_NAME_DTCV = "dtcv";
@SerializedName(SERIALIZED_NAME_DTCV)
private BigDecimal dtcv;
public static final String SERIALIZED_NAME_EFFECTIVE_END_DATE = "effectiveEndDate";
@SerializedName(SERIALIZED_NAME_EFFECTIVE_END_DATE)
private LocalDate effectiveEndDate;
public static final String SERIALIZED_NAME_EFFECTIVE_START_DATE = "effectiveStartDate";
@SerializedName(SERIALIZED_NAME_EFFECTIVE_START_DATE)
private LocalDate effectiveStartDate;
public static final String SERIALIZED_NAME_END_DATE_CONDITION = "endDateCondition";
@SerializedName(SERIALIZED_NAME_END_DATE_CONDITION)
private EndDateCondition endDateCondition;
public static final String SERIALIZED_NAME_INCLUDED_UNITS = "includedUnits";
@SerializedName(SERIALIZED_NAME_INCLUDED_UNITS)
private BigDecimal includedUnits;
public static final String SERIALIZED_NAME_INPUT_ARGUMENT_ID = "inputArgumentId";
@SerializedName(SERIALIZED_NAME_INPUT_ARGUMENT_ID)
private String inputArgumentId;
public static final String SERIALIZED_NAME_IS_COMMITTED = "isCommitted";
@SerializedName(SERIALIZED_NAME_IS_COMMITTED)
private Boolean isCommitted;
public static final String SERIALIZED_NAME_IS_PREPAID = "isPrepaid";
@SerializedName(SERIALIZED_NAME_IS_PREPAID)
private Boolean isPrepaid;
public static final String SERIALIZED_NAME_IS_ROLLOVER = "isRollover";
@SerializedName(SERIALIZED_NAME_IS_ROLLOVER)
private Boolean isRollover;
public static final String SERIALIZED_NAME_MRR = "mrr";
@SerializedName(SERIALIZED_NAME_MRR)
private BigDecimal mrr;
public static final String SERIALIZED_NAME_ORIGINAL_ORDER_DATE = "originalOrderDate";
@SerializedName(SERIALIZED_NAME_ORIGINAL_ORDER_DATE)
private LocalDate originalOrderDate;
public static final String SERIALIZED_NAME_OVERAGE_PRICE = "overagePrice";
@SerializedName(SERIALIZED_NAME_OVERAGE_PRICE)
private BigDecimal overagePrice;
/**
* Gets or Sets prepaidOperationType
*/
@JsonAdapter(PrepaidOperationTypeEnum.Adapter.class)
public enum PrepaidOperationTypeEnum {
TOPUP("topup"),
DRAWDOWN("drawdown");
private String value;
PrepaidOperationTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static PrepaidOperationTypeEnum fromValue(String value) {
for (PrepaidOperationTypeEnum b : PrepaidOperationTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final PrepaidOperationTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public PrepaidOperationTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return PrepaidOperationTypeEnum.fromValue(value);
}
}
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
String value = jsonElement.getAsString();
PrepaidOperationTypeEnum.fromValue(value);
}
}
public static final String SERIALIZED_NAME_PREPAID_OPERATION_TYPE = "prepaidOperationType";
@SerializedName(SERIALIZED_NAME_PREPAID_OPERATION_TYPE)
private PrepaidOperationTypeEnum prepaidOperationType;
public static final String SERIALIZED_NAME_PREPAID_QUANTITY = "prepaidQuantity";
@SerializedName(SERIALIZED_NAME_PREPAID_QUANTITY)
private BigDecimal prepaidQuantity;
public static final String SERIALIZED_NAME_PREPAID_TOTAL_QUANTITY = "prepaidTotalQuantity";
@SerializedName(SERIALIZED_NAME_PREPAID_TOTAL_QUANTITY)
private BigDecimal prepaidTotalQuantity;
public static final String SERIALIZED_NAME_PREPAID_U_O_M = "prepaidUOM";
@SerializedName(SERIALIZED_NAME_PREPAID_U_O_M)
private String prepaidUOM;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_PRICE = "price";
@SerializedName(SERIALIZED_NAME_PRICE)
private BigDecimal price;
public static final String SERIALIZED_NAME_PRICE_CHANGE_OPTION = "priceChangeOption";
@SerializedName(SERIALIZED_NAME_PRICE_CHANGE_OPTION)
private PriceChangeOption priceChangeOption = PriceChangeOption.NOCHANGE;
public static final String SERIALIZED_NAME_PRICE_INCREASE_PERCENTAGE = "priceIncreasePercentage";
@SerializedName(SERIALIZED_NAME_PRICE_INCREASE_PERCENTAGE)
private BigDecimal priceIncreasePercentage;
public static final String SERIALIZED_NAME_PRICING_SUMMARY = "pricingSummary";
@SerializedName(SERIALIZED_NAME_PRICING_SUMMARY)
private String pricingSummary;
public static final String SERIALIZED_NAME_ORIGINAL_LIST_PRICE = "originalListPrice";
@SerializedName(SERIALIZED_NAME_ORIGINAL_LIST_PRICE)
private BigDecimal originalListPrice;
public static final String SERIALIZED_NAME_PROCESSED_THROUGH_DATE = "processedThroughDate";
@SerializedName(SERIALIZED_NAME_PROCESSED_THROUGH_DATE)
private LocalDate processedThroughDate;
/**
* Gets or Sets rolloverApply
*/
@JsonAdapter(RolloverApplyEnum.Adapter.class)
public enum RolloverApplyEnum {
APPLYFIRST("ApplyFirst"),
APPLYLAST("ApplyLast");
private String value;
RolloverApplyEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static RolloverApplyEnum fromValue(String value) {
for (RolloverApplyEnum b : RolloverApplyEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final RolloverApplyEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public RolloverApplyEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return RolloverApplyEnum.fromValue(value);
}
}
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
String value = jsonElement.getAsString();
RolloverApplyEnum.fromValue(value);
}
}
public static final String SERIALIZED_NAME_ROLLOVER_APPLY = "rolloverApply";
@SerializedName(SERIALIZED_NAME_ROLLOVER_APPLY)
private RolloverApplyEnum rolloverApply;
public static final String SERIALIZED_NAME_ROLLOVER_PERIOD_LENGTH = "rolloverPeriodLength";
@SerializedName(SERIALIZED_NAME_ROLLOVER_PERIOD_LENGTH)
private Integer rolloverPeriodLength;
public static final String SERIALIZED_NAME_ROLLOVER_PERIODS = "rolloverPeriods";
@SerializedName(SERIALIZED_NAME_ROLLOVER_PERIODS)
private Long rolloverPeriods;
public static final String SERIALIZED_NAME_PRORATION_OPTION = "prorationOption";
@SerializedName(SERIALIZED_NAME_PRORATION_OPTION)
private String prorationOption;
public static final String SERIALIZED_NAME_SEGMENT = "segment";
@SerializedName(SERIALIZED_NAME_SEGMENT)
private Long segment;
public static final String SERIALIZED_NAME_SPECIFIC_END_DATE = "specificEndDate";
@SerializedName(SERIALIZED_NAME_SPECIFIC_END_DATE)
private LocalDate specificEndDate;
public static final String SERIALIZED_NAME_SUBSCRIPTION_CHARGE_INTERVAL_PRICING = "subscriptionChargeIntervalPricing";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_CHARGE_INTERVAL_PRICING)
private List subscriptionChargeIntervalPricing;
public static final String SERIALIZED_NAME_TCV = "tcv";
@SerializedName(SERIALIZED_NAME_TCV)
private BigDecimal tcv;
public static final String SERIALIZED_NAME_TIERS = "tiers";
@SerializedName(SERIALIZED_NAME_TIERS)
private List tiers;
public static final String SERIALIZED_NAME_TRIGGER_DATE = "triggerDate";
@SerializedName(SERIALIZED_NAME_TRIGGER_DATE)
private LocalDate triggerDate;
public static final String SERIALIZED_NAME_BILLING_PERIOD_ALIGNMENT = "billingPeriodAlignment";
@SerializedName(SERIALIZED_NAME_BILLING_PERIOD_ALIGNMENT)
private BillingPeriodAlignment billingPeriodAlignment;
public static final String SERIALIZED_NAME_TRIGGER_EVENT = "triggerEvent";
@SerializedName(SERIALIZED_NAME_TRIGGER_EVENT)
private TriggerEvent triggerEvent;
public static final String SERIALIZED_NAME_UP_TO_PERIODS = "upToPeriods";
@SerializedName(SERIALIZED_NAME_UP_TO_PERIODS)
private Long upToPeriods;
public static final String SERIALIZED_NAME_UP_TO_PERIODS_TYPE = "upToPeriodsType";
@SerializedName(SERIALIZED_NAME_UP_TO_PERIODS_TYPE)
private UpToPeriodsType upToPeriodsType;
public static final String SERIALIZED_NAME_VALIDITY_PERIOD_TYPE = "validityPeriodType";
@SerializedName(SERIALIZED_NAME_VALIDITY_PERIOD_TYPE)
private ValidityPeriodType validityPeriodType;
public static final String SERIALIZED_NAME_SALES_PRICE = "salesPrice";
@SerializedName(SERIALIZED_NAME_SALES_PRICE)
private BigDecimal salesPrice;
public static final String SERIALIZED_NAME_ACCOUNTING_CODE = "accountingCode";
@SerializedName(SERIALIZED_NAME_ACCOUNTING_CODE)
private String accountingCode;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_CODE = "revenueRecognitionCode";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_CODE)
private String revenueRecognitionCode;
public static final String SERIALIZED_NAME_REV_REC_TRIGGER_CONDITION = "revRecTriggerCondition";
@SerializedName(SERIALIZED_NAME_REV_REC_TRIGGER_CONDITION)
private String revRecTriggerCondition;
public RatePlanChargeSegmentInfo() {
}
public RatePlanChargeSegmentInfo id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public RatePlanChargeSegmentInfo amendedByOrderOn(LocalDate amendedByOrderOn) {
this.amendedByOrderOn = amendedByOrderOn;
return this;
}
/**
* The date when the rate plan charge is amended through an order or amendment. This field is to standardize the booking date information to increase audit ability and traceability of data between Zuora Billing and Zuora Revenue. It is mapped as the booking date for a sale order line in Zuora Revenue.
* @return amendedByOrderOn
*/
@javax.annotation.Nullable
public LocalDate getAmendedByOrderOn() {
return amendedByOrderOn;
}
public void setAmendedByOrderOn(LocalDate amendedByOrderOn) {
this.amendedByOrderOn = amendedByOrderOn;
}
public RatePlanChargeSegmentInfo applyDiscountTo(ApplyDiscountTo applyDiscountTo) {
this.applyDiscountTo = applyDiscountTo;
return this;
}
/**
* Get applyDiscountTo
* @return applyDiscountTo
*/
@javax.annotation.Nullable
public ApplyDiscountTo getApplyDiscountTo() {
return applyDiscountTo;
}
public void setApplyDiscountTo(ApplyDiscountTo applyDiscountTo) {
this.applyDiscountTo = applyDiscountTo;
}
public RatePlanChargeSegmentInfo chargeFunction(ChargeFunction chargeFunction) {
this.chargeFunction = chargeFunction;
return this;
}
/**
* Get chargeFunction
* @return chargeFunction
*/
@javax.annotation.Nullable
public ChargeFunction getChargeFunction() {
return chargeFunction;
}
public void setChargeFunction(ChargeFunction chargeFunction) {
this.chargeFunction = chargeFunction;
}
public RatePlanChargeSegmentInfo chargeModelConfiguration(ChargeModelConfigurationForSubscription chargeModelConfiguration) {
this.chargeModelConfiguration = chargeModelConfiguration;
return this;
}
/**
* Get chargeModelConfiguration
* @return chargeModelConfiguration
*/
@javax.annotation.Nullable
public ChargeModelConfigurationForSubscription getChargeModelConfiguration() {
return chargeModelConfiguration;
}
public void setChargeModelConfiguration(ChargeModelConfigurationForSubscription chargeModelConfiguration) {
this.chargeModelConfiguration = chargeModelConfiguration;
}
public RatePlanChargeSegmentInfo chargedThroughDate(LocalDate chargedThroughDate) {
this.chargedThroughDate = chargedThroughDate;
return this;
}
/**
* The date through which a customer has been billed for the charge.
* @return chargedThroughDate
*/
@javax.annotation.Nullable
public LocalDate getChargedThroughDate() {
return chargedThroughDate;
}
public void setChargedThroughDate(LocalDate chargedThroughDate) {
this.chargedThroughDate = chargedThroughDate;
}
public RatePlanChargeSegmentInfo commitmentType(CommitmentType commitmentType) {
this.commitmentType = commitmentType;
return this;
}
/**
* Get commitmentType
* @return commitmentType
*/
@javax.annotation.Nullable
public CommitmentType getCommitmentType() {
return commitmentType;
}
public void setCommitmentType(CommitmentType commitmentType) {
this.commitmentType = commitmentType;
}
public RatePlanChargeSegmentInfo prepaidCommittedAmount(String prepaidCommittedAmount) {
this.prepaidCommittedAmount = prepaidCommittedAmount;
return this;
}
/**
* Get prepaidCommittedAmount
* @return prepaidCommittedAmount
*/
@javax.annotation.Nullable
public String getPrepaidCommittedAmount() {
return prepaidCommittedAmount;
}
public void setPrepaidCommittedAmount(String prepaidCommittedAmount) {
this.prepaidCommittedAmount = prepaidCommittedAmount;
}
public RatePlanChargeSegmentInfo creditOption(CreditOptionEnum creditOption) {
this.creditOption = creditOption;
return this;
}
/**
* **Note**: This field is only available if you have the [Prepaid with Drawdown](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown) feature enabled. To use this field, you must set the `X-Zuora-WSDL-Version` request header to 114 or higher. Otherwise, an error occurs. The way to calculate credit. See [Credit Option](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown/Create_prepayment_charge#Credit_Option) for more information.
* @return creditOption
*/
@javax.annotation.Nullable
public CreditOptionEnum getCreditOption() {
return creditOption;
}
public void setCreditOption(CreditOptionEnum creditOption) {
this.creditOption = creditOption;
}
public RatePlanChargeSegmentInfo currency(String currency) {
this.currency = currency;
return this;
}
/**
* Currency used by the account. For example, `USD` or `EUR`.
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public RatePlanChargeSegmentInfo deliverySchedule(DeliverySchedule deliverySchedule) {
this.deliverySchedule = deliverySchedule;
return this;
}
/**
* Get deliverySchedule
* @return deliverySchedule
*/
@javax.annotation.Nullable
public DeliverySchedule getDeliverySchedule() {
return deliverySchedule;
}
public void setDeliverySchedule(DeliverySchedule deliverySchedule) {
this.deliverySchedule = deliverySchedule;
}
public RatePlanChargeSegmentInfo numberOfDeliveries(BigDecimal numberOfDeliveries) {
this.numberOfDeliveries = numberOfDeliveries;
return this;
}
/**
* Get numberOfDeliveries
* @return numberOfDeliveries
*/
@javax.annotation.Nullable
public BigDecimal getNumberOfDeliveries() {
return numberOfDeliveries;
}
public void setNumberOfDeliveries(BigDecimal numberOfDeliveries) {
this.numberOfDeliveries = numberOfDeliveries;
}
public RatePlanChargeSegmentInfo description(String description) {
this.description = description;
return this;
}
/**
* Description of the rate plan charge.
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public RatePlanChargeSegmentInfo discountAmount(BigDecimal discountAmount) {
this.discountAmount = discountAmount;
return this;
}
/**
* The amount of the discount.
* @return discountAmount
*/
@javax.annotation.Nullable
public BigDecimal getDiscountAmount() {
return discountAmount;
}
public void setDiscountAmount(BigDecimal discountAmount) {
this.discountAmount = discountAmount;
}
public RatePlanChargeSegmentInfo discountApplyDetails(List discountApplyDetails) {
this.discountApplyDetails = discountApplyDetails;
return this;
}
public RatePlanChargeSegmentInfo addDiscountApplyDetailsItem(DiscountApplyDetail discountApplyDetailsItem) {
if (this.discountApplyDetails == null) {
this.discountApplyDetails = new ArrayList<>();
}
this.discountApplyDetails.add(discountApplyDetailsItem);
return this;
}
/**
* Container for the application details about a discount rate plan charge. Only discount rate plan charges have values in this field.
* @return discountApplyDetails
*/
@javax.annotation.Nullable
public List getDiscountApplyDetails() {
return discountApplyDetails;
}
public void setDiscountApplyDetails(List discountApplyDetails) {
this.discountApplyDetails = discountApplyDetails;
}
public RatePlanChargeSegmentInfo discountClass(String discountClass) {
this.discountClass = discountClass;
return this;
}
/**
* The class that the discount belongs to. The discount class defines the order in which discount rate plan charges are applied. For more information, see [Manage Discount Classes](https://knowledgecenter.zuora.com/BC_Subscription_Management/Product_Catalog/B_Charge_Models/Manage_Discount_Classes).
* @return discountClass
*/
@javax.annotation.Nullable
public String getDiscountClass() {
return discountClass;
}
public void setDiscountClass(String discountClass) {
this.discountClass = discountClass;
}
public RatePlanChargeSegmentInfo discountLevel(DiscountLevel discountLevel) {
this.discountLevel = discountLevel;
return this;
}
/**
* Get discountLevel
* @return discountLevel
*/
@javax.annotation.Nullable
public DiscountLevel getDiscountLevel() {
return discountLevel;
}
public void setDiscountLevel(DiscountLevel discountLevel) {
this.discountLevel = discountLevel;
}
public RatePlanChargeSegmentInfo discountPercentage(BigDecimal discountPercentage) {
this.discountPercentage = discountPercentage;
return this;
}
/**
* The amount of the discount as a percentage.
* @return discountPercentage
*/
@javax.annotation.Nullable
public BigDecimal getDiscountPercentage() {
return discountPercentage;
}
public void setDiscountPercentage(BigDecimal discountPercentage) {
this.discountPercentage = discountPercentage;
}
public RatePlanChargeSegmentInfo applyToBillingPeriodPartially(Boolean applyToBillingPeriodPartially) {
this.applyToBillingPeriodPartially = applyToBillingPeriodPartially;
return this;
}
/**
* Get applyToBillingPeriodPartially
* @return applyToBillingPeriodPartially
*/
@javax.annotation.Nullable
public Boolean getApplyToBillingPeriodPartially() {
return applyToBillingPeriodPartially;
}
public void setApplyToBillingPeriodPartially(Boolean applyToBillingPeriodPartially) {
this.applyToBillingPeriodPartially = applyToBillingPeriodPartially;
}
public RatePlanChargeSegmentInfo dmrc(BigDecimal dmrc) {
this.dmrc = dmrc;
return this;
}
/**
* The change (delta) of monthly recurring charge exists when the change in monthly recurring revenue caused by an amendment or a new subscription.
* @return dmrc
*/
@javax.annotation.Nullable
public BigDecimal getDmrc() {
return dmrc;
}
public void setDmrc(BigDecimal dmrc) {
this.dmrc = dmrc;
}
public RatePlanChargeSegmentInfo done(Boolean done) {
this.done = done;
return this;
}
/**
* A value of `true` indicates that an invoice for a charge segment has been completed. A value of `false` indicates that an invoice has not been completed for the charge segment.
* @return done
*/
@javax.annotation.Nullable
public Boolean getDone() {
return done;
}
public void setDone(Boolean done) {
this.done = done;
}
public RatePlanChargeSegmentInfo drawdownRate(BigDecimal drawdownRate) {
this.drawdownRate = drawdownRate;
return this;
}
/**
* Get drawdownRate
* @return drawdownRate
*/
@javax.annotation.Nullable
public BigDecimal getDrawdownRate() {
return drawdownRate;
}
public void setDrawdownRate(BigDecimal drawdownRate) {
this.drawdownRate = drawdownRate;
}
public RatePlanChargeSegmentInfo drawdownUom(String drawdownUom) {
this.drawdownUom = drawdownUom;
return this;
}
/**
* Specifies the units to measure usage.
* @return drawdownUom
*/
@javax.annotation.Nullable
public String getDrawdownUom() {
return drawdownUom;
}
public void setDrawdownUom(String drawdownUom) {
this.drawdownUom = drawdownUom;
}
public RatePlanChargeSegmentInfo dtcv(BigDecimal dtcv) {
this.dtcv = dtcv;
return this;
}
/**
* After an amendment or an AutomatedPriceChange event, `dtcv` displays the change (delta) for the total contract value (TCV) amount for this charge, compared with its previous value with recurring charge types.
* @return dtcv
*/
@javax.annotation.Nullable
public BigDecimal getDtcv() {
return dtcv;
}
public void setDtcv(BigDecimal dtcv) {
this.dtcv = dtcv;
}
public RatePlanChargeSegmentInfo effectiveEndDate(LocalDate effectiveEndDate) {
this.effectiveEndDate = effectiveEndDate;
return this;
}
/**
* Get effectiveEndDate
* @return effectiveEndDate
*/
@javax.annotation.Nullable
public LocalDate getEffectiveEndDate() {
return effectiveEndDate;
}
public void setEffectiveEndDate(LocalDate effectiveEndDate) {
this.effectiveEndDate = effectiveEndDate;
}
public RatePlanChargeSegmentInfo effectiveStartDate(LocalDate effectiveStartDate) {
this.effectiveStartDate = effectiveStartDate;
return this;
}
/**
* Get effectiveStartDate
* @return effectiveStartDate
*/
@javax.annotation.Nullable
public LocalDate getEffectiveStartDate() {
return effectiveStartDate;
}
public void setEffectiveStartDate(LocalDate effectiveStartDate) {
this.effectiveStartDate = effectiveStartDate;
}
public RatePlanChargeSegmentInfo endDateCondition(EndDateCondition endDateCondition) {
this.endDateCondition = endDateCondition;
return this;
}
/**
* Get endDateCondition
* @return endDateCondition
*/
@javax.annotation.Nullable
public EndDateCondition getEndDateCondition() {
return endDateCondition;
}
public void setEndDateCondition(EndDateCondition endDateCondition) {
this.endDateCondition = endDateCondition;
}
public RatePlanChargeSegmentInfo includedUnits(BigDecimal includedUnits) {
this.includedUnits = includedUnits;
return this;
}
/**
* Get includedUnits
* @return includedUnits
*/
@javax.annotation.Nullable
public BigDecimal getIncludedUnits() {
return includedUnits;
}
public void setIncludedUnits(BigDecimal includedUnits) {
this.includedUnits = includedUnits;
}
public RatePlanChargeSegmentInfo inputArgumentId(String inputArgumentId) {
this.inputArgumentId = inputArgumentId;
return this;
}
/**
* Get inputArgumentId
* @return inputArgumentId
*/
@javax.annotation.Nullable
public String getInputArgumentId() {
return inputArgumentId;
}
public void setInputArgumentId(String inputArgumentId) {
this.inputArgumentId = inputArgumentId;
}
public RatePlanChargeSegmentInfo isCommitted(Boolean isCommitted) {
this.isCommitted = isCommitted;
return this;
}
/**
* Get isCommitted
* @return isCommitted
*/
@javax.annotation.Nullable
public Boolean getIsCommitted() {
return isCommitted;
}
public void setIsCommitted(Boolean isCommitted) {
this.isCommitted = isCommitted;
}
public RatePlanChargeSegmentInfo isPrepaid(Boolean isPrepaid) {
this.isPrepaid = isPrepaid;
return this;
}
/**
* Get isPrepaid
* @return isPrepaid
*/
@javax.annotation.Nullable
public Boolean getIsPrepaid() {
return isPrepaid;
}
public void setIsPrepaid(Boolean isPrepaid) {
this.isPrepaid = isPrepaid;
}
public RatePlanChargeSegmentInfo isRollover(Boolean isRollover) {
this.isRollover = isRollover;
return this;
}
/**
* Get isRollover
* @return isRollover
*/
@javax.annotation.Nullable
public Boolean getIsRollover() {
return isRollover;
}
public void setIsRollover(Boolean isRollover) {
this.isRollover = isRollover;
}
public RatePlanChargeSegmentInfo mrr(BigDecimal mrr) {
this.mrr = mrr;
return this;
}
/**
* Get mrr
* @return mrr
*/
@javax.annotation.Nullable
public BigDecimal getMrr() {
return mrr;
}
public void setMrr(BigDecimal mrr) {
this.mrr = mrr;
}
public RatePlanChargeSegmentInfo originalOrderDate(LocalDate originalOrderDate) {
this.originalOrderDate = originalOrderDate;
return this;
}
/**
* Get originalOrderDate
* @return originalOrderDate
*/
@javax.annotation.Nullable
public LocalDate getOriginalOrderDate() {
return originalOrderDate;
}
public void setOriginalOrderDate(LocalDate originalOrderDate) {
this.originalOrderDate = originalOrderDate;
}
public RatePlanChargeSegmentInfo overagePrice(BigDecimal overagePrice) {
this.overagePrice = overagePrice;
return this;
}
/**
* Get overagePrice
* @return overagePrice
*/
@javax.annotation.Nullable
public BigDecimal getOveragePrice() {
return overagePrice;
}
public void setOveragePrice(BigDecimal overagePrice) {
this.overagePrice = overagePrice;
}
public RatePlanChargeSegmentInfo prepaidOperationType(PrepaidOperationTypeEnum prepaidOperationType) {
this.prepaidOperationType = prepaidOperationType;
return this;
}
/**
* Get prepaidOperationType
* @return prepaidOperationType
*/
@javax.annotation.Nullable
public PrepaidOperationTypeEnum getPrepaidOperationType() {
return prepaidOperationType;
}
public void setPrepaidOperationType(PrepaidOperationTypeEnum prepaidOperationType) {
this.prepaidOperationType = prepaidOperationType;
}
public RatePlanChargeSegmentInfo prepaidQuantity(BigDecimal prepaidQuantity) {
this.prepaidQuantity = prepaidQuantity;
return this;
}
/**
* Get prepaidQuantity
* @return prepaidQuantity
*/
@javax.annotation.Nullable
public BigDecimal getPrepaidQuantity() {
return prepaidQuantity;
}
public void setPrepaidQuantity(BigDecimal prepaidQuantity) {
this.prepaidQuantity = prepaidQuantity;
}
public RatePlanChargeSegmentInfo prepaidTotalQuantity(BigDecimal prepaidTotalQuantity) {
this.prepaidTotalQuantity = prepaidTotalQuantity;
return this;
}
/**
* Get prepaidTotalQuantity
* @return prepaidTotalQuantity
*/
@javax.annotation.Nullable
public BigDecimal getPrepaidTotalQuantity() {
return prepaidTotalQuantity;
}
public void setPrepaidTotalQuantity(BigDecimal prepaidTotalQuantity) {
this.prepaidTotalQuantity = prepaidTotalQuantity;
}
public RatePlanChargeSegmentInfo prepaidUOM(String prepaidUOM) {
this.prepaidUOM = prepaidUOM;
return this;
}
/**
* Specifies the units to measure usage.
* @return prepaidUOM
*/
@javax.annotation.Nullable
public String getPrepaidUOM() {
return prepaidUOM;
}
public void setPrepaidUOM(String prepaidUOM) {
this.prepaidUOM = prepaidUOM;
}
public RatePlanChargeSegmentInfo quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* Get quantity
* @return quantity
*/
@javax.annotation.Nullable
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public RatePlanChargeSegmentInfo price(BigDecimal price) {
this.price = price;
return this;
}
/**
* Get price
* @return price
*/
@javax.annotation.Nullable
public BigDecimal getPrice() {
return price;
}
public void setPrice(BigDecimal price) {
this.price = price;
}
public RatePlanChargeSegmentInfo priceChangeOption(PriceChangeOption priceChangeOption) {
this.priceChangeOption = priceChangeOption;
return this;
}
/**
* Get priceChangeOption
* @return priceChangeOption
*/
@javax.annotation.Nullable
public PriceChangeOption getPriceChangeOption() {
return priceChangeOption;
}
public void setPriceChangeOption(PriceChangeOption priceChangeOption) {
this.priceChangeOption = priceChangeOption;
}
public RatePlanChargeSegmentInfo priceIncreasePercentage(BigDecimal priceIncreasePercentage) {
this.priceIncreasePercentage = priceIncreasePercentage;
return this;
}
/**
* Get priceIncreasePercentage
* @return priceIncreasePercentage
*/
@javax.annotation.Nullable
public BigDecimal getPriceIncreasePercentage() {
return priceIncreasePercentage;
}
public void setPriceIncreasePercentage(BigDecimal priceIncreasePercentage) {
this.priceIncreasePercentage = priceIncreasePercentage;
}
public RatePlanChargeSegmentInfo pricingSummary(String pricingSummary) {
this.pricingSummary = pricingSummary;
return this;
}
/**
* Get pricingSummary
* @return pricingSummary
*/
@javax.annotation.Nullable
public String getPricingSummary() {
return pricingSummary;
}
public void setPricingSummary(String pricingSummary) {
this.pricingSummary = pricingSummary;
}
public RatePlanChargeSegmentInfo originalListPrice(BigDecimal originalListPrice) {
this.originalListPrice = originalListPrice;
return this;
}
/**
* Get originalListPrice
* @return originalListPrice
*/
@javax.annotation.Nullable
public BigDecimal getOriginalListPrice() {
return originalListPrice;
}
public void setOriginalListPrice(BigDecimal originalListPrice) {
this.originalListPrice = originalListPrice;
}
public RatePlanChargeSegmentInfo processedThroughDate(LocalDate processedThroughDate) {
this.processedThroughDate = processedThroughDate;
return this;
}
/**
* Get processedThroughDate
* @return processedThroughDate
*/
@javax.annotation.Nullable
public LocalDate getProcessedThroughDate() {
return processedThroughDate;
}
public void setProcessedThroughDate(LocalDate processedThroughDate) {
this.processedThroughDate = processedThroughDate;
}
public RatePlanChargeSegmentInfo rolloverApply(RolloverApplyEnum rolloverApply) {
this.rolloverApply = rolloverApply;
return this;
}
/**
* Get rolloverApply
* @return rolloverApply
*/
@javax.annotation.Nullable
public RolloverApplyEnum getRolloverApply() {
return rolloverApply;
}
public void setRolloverApply(RolloverApplyEnum rolloverApply) {
this.rolloverApply = rolloverApply;
}
public RatePlanChargeSegmentInfo rolloverPeriodLength(Integer rolloverPeriodLength) {
this.rolloverPeriodLength = rolloverPeriodLength;
return this;
}
/**
* Get rolloverPeriodLength
* @return rolloverPeriodLength
*/
@javax.annotation.Nullable
public Integer getRolloverPeriodLength() {
return rolloverPeriodLength;
}
public void setRolloverPeriodLength(Integer rolloverPeriodLength) {
this.rolloverPeriodLength = rolloverPeriodLength;
}
public RatePlanChargeSegmentInfo rolloverPeriods(Long rolloverPeriods) {
this.rolloverPeriods = rolloverPeriods;
return this;
}
/**
* Get rolloverPeriods
* @return rolloverPeriods
*/
@javax.annotation.Nullable
public Long getRolloverPeriods() {
return rolloverPeriods;
}
public void setRolloverPeriods(Long rolloverPeriods) {
this.rolloverPeriods = rolloverPeriods;
}
public RatePlanChargeSegmentInfo prorationOption(String prorationOption) {
this.prorationOption = prorationOption;
return this;
}
/**
* Get prorationOption
* @return prorationOption
*/
@javax.annotation.Nullable
public String getProrationOption() {
return prorationOption;
}
public void setProrationOption(String prorationOption) {
this.prorationOption = prorationOption;
}
public RatePlanChargeSegmentInfo segment(Long segment) {
this.segment = segment;
return this;
}
/**
* Get segment
* @return segment
*/
@javax.annotation.Nullable
public Long getSegment() {
return segment;
}
public void setSegment(Long segment) {
this.segment = segment;
}
public RatePlanChargeSegmentInfo specificEndDate(LocalDate specificEndDate) {
this.specificEndDate = specificEndDate;
return this;
}
/**
* Get specificEndDate
* @return specificEndDate
*/
@javax.annotation.Nullable
public LocalDate getSpecificEndDate() {
return specificEndDate;
}
public void setSpecificEndDate(LocalDate specificEndDate) {
this.specificEndDate = specificEndDate;
}
public RatePlanChargeSegmentInfo subscriptionChargeIntervalPricing(List subscriptionChargeIntervalPricing) {
this.subscriptionChargeIntervalPricing = subscriptionChargeIntervalPricing;
return this;
}
public RatePlanChargeSegmentInfo addSubscriptionChargeIntervalPricingItem(IntervalPricing subscriptionChargeIntervalPricingItem) {
if (this.subscriptionChargeIntervalPricing == null) {
this.subscriptionChargeIntervalPricing = new ArrayList<>();
}
this.subscriptionChargeIntervalPricing.add(subscriptionChargeIntervalPricingItem);
return this;
}
/**
* Get subscriptionChargeIntervalPricing
* @return subscriptionChargeIntervalPricing
*/
@javax.annotation.Nullable
public List getSubscriptionChargeIntervalPricing() {
return subscriptionChargeIntervalPricing;
}
public void setSubscriptionChargeIntervalPricing(List subscriptionChargeIntervalPricing) {
this.subscriptionChargeIntervalPricing = subscriptionChargeIntervalPricing;
}
public RatePlanChargeSegmentInfo tcv(BigDecimal tcv) {
this.tcv = tcv;
return this;
}
/**
* Get tcv
* @return tcv
*/
@javax.annotation.Nullable
public BigDecimal getTcv() {
return tcv;
}
public void setTcv(BigDecimal tcv) {
this.tcv = tcv;
}
public RatePlanChargeSegmentInfo tiers(List tiers) {
this.tiers = tiers;
return this;
}
public RatePlanChargeSegmentInfo addTiersItem(RatePlanChargeTier tiersItem) {
if (this.tiers == null) {
this.tiers = new ArrayList<>();
}
this.tiers.add(tiersItem);
return this;
}
/**
* Get tiers
* @return tiers
*/
@javax.annotation.Nullable
public List getTiers() {
return tiers;
}
public void setTiers(List tiers) {
this.tiers = tiers;
}
public RatePlanChargeSegmentInfo triggerDate(LocalDate triggerDate) {
this.triggerDate = triggerDate;
return this;
}
/**
* Get triggerDate
* @return triggerDate
*/
@javax.annotation.Nullable
public LocalDate getTriggerDate() {
return triggerDate;
}
public void setTriggerDate(LocalDate triggerDate) {
this.triggerDate = triggerDate;
}
public RatePlanChargeSegmentInfo billingPeriodAlignment(BillingPeriodAlignment billingPeriodAlignment) {
this.billingPeriodAlignment = billingPeriodAlignment;
return this;
}
/**
* Get billingPeriodAlignment
* @return billingPeriodAlignment
*/
@javax.annotation.Nullable
public BillingPeriodAlignment getBillingPeriodAlignment() {
return billingPeriodAlignment;
}
public void setBillingPeriodAlignment(BillingPeriodAlignment billingPeriodAlignment) {
this.billingPeriodAlignment = billingPeriodAlignment;
}
public RatePlanChargeSegmentInfo triggerEvent(TriggerEvent triggerEvent) {
this.triggerEvent = triggerEvent;
return this;
}
/**
* Get triggerEvent
* @return triggerEvent
*/
@javax.annotation.Nullable
public TriggerEvent getTriggerEvent() {
return triggerEvent;
}
public void setTriggerEvent(TriggerEvent triggerEvent) {
this.triggerEvent = triggerEvent;
}
public RatePlanChargeSegmentInfo upToPeriods(Long upToPeriods) {
this.upToPeriods = upToPeriods;
return this;
}
/**
* Get upToPeriods
* @return upToPeriods
*/
@javax.annotation.Nullable
public Long getUpToPeriods() {
return upToPeriods;
}
public void setUpToPeriods(Long upToPeriods) {
this.upToPeriods = upToPeriods;
}
public RatePlanChargeSegmentInfo upToPeriodsType(UpToPeriodsType upToPeriodsType) {
this.upToPeriodsType = upToPeriodsType;
return this;
}
/**
* Get upToPeriodsType
* @return upToPeriodsType
*/
@javax.annotation.Nullable
public UpToPeriodsType getUpToPeriodsType() {
return upToPeriodsType;
}
public void setUpToPeriodsType(UpToPeriodsType upToPeriodsType) {
this.upToPeriodsType = upToPeriodsType;
}
public RatePlanChargeSegmentInfo validityPeriodType(ValidityPeriodType validityPeriodType) {
this.validityPeriodType = validityPeriodType;
return this;
}
/**
* Get validityPeriodType
* @return validityPeriodType
*/
@javax.annotation.Nullable
public ValidityPeriodType getValidityPeriodType() {
return validityPeriodType;
}
public void setValidityPeriodType(ValidityPeriodType validityPeriodType) {
this.validityPeriodType = validityPeriodType;
}
public RatePlanChargeSegmentInfo salesPrice(BigDecimal salesPrice) {
this.salesPrice = salesPrice;
return this;
}
/**
* Get salesPrice
* @return salesPrice
*/
@javax.annotation.Nullable
public BigDecimal getSalesPrice() {
return salesPrice;
}
public void setSalesPrice(BigDecimal salesPrice) {
this.salesPrice = salesPrice;
}
public RatePlanChargeSegmentInfo accountingCode(String accountingCode) {
this.accountingCode = accountingCode;
return this;
}
/**
* Get accountingCode
* @return accountingCode
*/
@javax.annotation.Nullable
public String getAccountingCode() {
return accountingCode;
}
public void setAccountingCode(String accountingCode) {
this.accountingCode = accountingCode;
}
public RatePlanChargeSegmentInfo revenueRecognitionCode(String revenueRecognitionCode) {
this.revenueRecognitionCode = revenueRecognitionCode;
return this;
}
/**
* Get revenueRecognitionCode
* @return revenueRecognitionCode
*/
@javax.annotation.Nullable
public String getRevenueRecognitionCode() {
return revenueRecognitionCode;
}
public void setRevenueRecognitionCode(String revenueRecognitionCode) {
this.revenueRecognitionCode = revenueRecognitionCode;
}
public RatePlanChargeSegmentInfo revRecTriggerCondition(String revRecTriggerCondition) {
this.revRecTriggerCondition = revRecTriggerCondition;
return this;
}
/**
* Get revRecTriggerCondition
* @return revRecTriggerCondition
*/
@javax.annotation.Nullable
public String getRevRecTriggerCondition() {
return revRecTriggerCondition;
}
public void setRevRecTriggerCondition(String revRecTriggerCondition) {
this.revRecTriggerCondition = revRecTriggerCondition;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the RatePlanChargeSegmentInfo instance itself
*/
public RatePlanChargeSegmentInfo putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RatePlanChargeSegmentInfo ratePlanChargeSegmentInfo = (RatePlanChargeSegmentInfo) o;
return Objects.equals(this.id, ratePlanChargeSegmentInfo.id) &&
Objects.equals(this.amendedByOrderOn, ratePlanChargeSegmentInfo.amendedByOrderOn) &&
Objects.equals(this.applyDiscountTo, ratePlanChargeSegmentInfo.applyDiscountTo) &&
Objects.equals(this.chargeFunction, ratePlanChargeSegmentInfo.chargeFunction) &&
Objects.equals(this.chargeModelConfiguration, ratePlanChargeSegmentInfo.chargeModelConfiguration) &&
Objects.equals(this.chargedThroughDate, ratePlanChargeSegmentInfo.chargedThroughDate) &&
Objects.equals(this.commitmentType, ratePlanChargeSegmentInfo.commitmentType) &&
Objects.equals(this.prepaidCommittedAmount, ratePlanChargeSegmentInfo.prepaidCommittedAmount) &&
Objects.equals(this.creditOption, ratePlanChargeSegmentInfo.creditOption) &&
Objects.equals(this.currency, ratePlanChargeSegmentInfo.currency) &&
Objects.equals(this.deliverySchedule, ratePlanChargeSegmentInfo.deliverySchedule) &&
Objects.equals(this.numberOfDeliveries, ratePlanChargeSegmentInfo.numberOfDeliveries) &&
Objects.equals(this.description, ratePlanChargeSegmentInfo.description) &&
Objects.equals(this.discountAmount, ratePlanChargeSegmentInfo.discountAmount) &&
Objects.equals(this.discountApplyDetails, ratePlanChargeSegmentInfo.discountApplyDetails) &&
Objects.equals(this.discountClass, ratePlanChargeSegmentInfo.discountClass) &&
Objects.equals(this.discountLevel, ratePlanChargeSegmentInfo.discountLevel) &&
Objects.equals(this.discountPercentage, ratePlanChargeSegmentInfo.discountPercentage) &&
Objects.equals(this.applyToBillingPeriodPartially, ratePlanChargeSegmentInfo.applyToBillingPeriodPartially) &&
Objects.equals(this.dmrc, ratePlanChargeSegmentInfo.dmrc) &&
Objects.equals(this.done, ratePlanChargeSegmentInfo.done) &&
Objects.equals(this.drawdownRate, ratePlanChargeSegmentInfo.drawdownRate) &&
Objects.equals(this.drawdownUom, ratePlanChargeSegmentInfo.drawdownUom) &&
Objects.equals(this.dtcv, ratePlanChargeSegmentInfo.dtcv) &&
Objects.equals(this.effectiveEndDate, ratePlanChargeSegmentInfo.effectiveEndDate) &&
Objects.equals(this.effectiveStartDate, ratePlanChargeSegmentInfo.effectiveStartDate) &&
Objects.equals(this.endDateCondition, ratePlanChargeSegmentInfo.endDateCondition) &&
Objects.equals(this.includedUnits, ratePlanChargeSegmentInfo.includedUnits) &&
Objects.equals(this.inputArgumentId, ratePlanChargeSegmentInfo.inputArgumentId) &&
Objects.equals(this.isCommitted, ratePlanChargeSegmentInfo.isCommitted) &&
Objects.equals(this.isPrepaid, ratePlanChargeSegmentInfo.isPrepaid) &&
Objects.equals(this.isRollover, ratePlanChargeSegmentInfo.isRollover) &&
Objects.equals(this.mrr, ratePlanChargeSegmentInfo.mrr) &&
Objects.equals(this.originalOrderDate, ratePlanChargeSegmentInfo.originalOrderDate) &&
Objects.equals(this.overagePrice, ratePlanChargeSegmentInfo.overagePrice) &&
Objects.equals(this.prepaidOperationType, ratePlanChargeSegmentInfo.prepaidOperationType) &&
Objects.equals(this.prepaidQuantity, ratePlanChargeSegmentInfo.prepaidQuantity) &&
Objects.equals(this.prepaidTotalQuantity, ratePlanChargeSegmentInfo.prepaidTotalQuantity) &&
Objects.equals(this.prepaidUOM, ratePlanChargeSegmentInfo.prepaidUOM) &&
Objects.equals(this.quantity, ratePlanChargeSegmentInfo.quantity) &&
Objects.equals(this.price, ratePlanChargeSegmentInfo.price) &&
Objects.equals(this.priceChangeOption, ratePlanChargeSegmentInfo.priceChangeOption) &&
Objects.equals(this.priceIncreasePercentage, ratePlanChargeSegmentInfo.priceIncreasePercentage) &&
Objects.equals(this.pricingSummary, ratePlanChargeSegmentInfo.pricingSummary) &&
Objects.equals(this.originalListPrice, ratePlanChargeSegmentInfo.originalListPrice) &&
Objects.equals(this.processedThroughDate, ratePlanChargeSegmentInfo.processedThroughDate) &&
Objects.equals(this.rolloverApply, ratePlanChargeSegmentInfo.rolloverApply) &&
Objects.equals(this.rolloverPeriodLength, ratePlanChargeSegmentInfo.rolloverPeriodLength) &&
Objects.equals(this.rolloverPeriods, ratePlanChargeSegmentInfo.rolloverPeriods) &&
Objects.equals(this.prorationOption, ratePlanChargeSegmentInfo.prorationOption) &&
Objects.equals(this.segment, ratePlanChargeSegmentInfo.segment) &&
Objects.equals(this.specificEndDate, ratePlanChargeSegmentInfo.specificEndDate) &&
Objects.equals(this.subscriptionChargeIntervalPricing, ratePlanChargeSegmentInfo.subscriptionChargeIntervalPricing) &&
Objects.equals(this.tcv, ratePlanChargeSegmentInfo.tcv) &&
Objects.equals(this.tiers, ratePlanChargeSegmentInfo.tiers) &&
Objects.equals(this.triggerDate, ratePlanChargeSegmentInfo.triggerDate) &&
Objects.equals(this.billingPeriodAlignment, ratePlanChargeSegmentInfo.billingPeriodAlignment) &&
Objects.equals(this.triggerEvent, ratePlanChargeSegmentInfo.triggerEvent) &&
Objects.equals(this.upToPeriods, ratePlanChargeSegmentInfo.upToPeriods) &&
Objects.equals(this.upToPeriodsType, ratePlanChargeSegmentInfo.upToPeriodsType) &&
Objects.equals(this.validityPeriodType, ratePlanChargeSegmentInfo.validityPeriodType) &&
Objects.equals(this.salesPrice, ratePlanChargeSegmentInfo.salesPrice) &&
Objects.equals(this.accountingCode, ratePlanChargeSegmentInfo.accountingCode) &&
Objects.equals(this.revenueRecognitionCode, ratePlanChargeSegmentInfo.revenueRecognitionCode) &&
Objects.equals(this.revRecTriggerCondition, ratePlanChargeSegmentInfo.revRecTriggerCondition)&&
Objects.equals(this.additionalProperties, ratePlanChargeSegmentInfo.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(id, amendedByOrderOn, applyDiscountTo, chargeFunction, chargeModelConfiguration, chargedThroughDate, commitmentType, prepaidCommittedAmount, creditOption, currency, deliverySchedule, numberOfDeliveries, description, discountAmount, discountApplyDetails, discountClass, discountLevel, discountPercentage, applyToBillingPeriodPartially, dmrc, done, drawdownRate, drawdownUom, dtcv, effectiveEndDate, effectiveStartDate, endDateCondition, includedUnits, inputArgumentId, isCommitted, isPrepaid, isRollover, mrr, originalOrderDate, overagePrice, prepaidOperationType, prepaidQuantity, prepaidTotalQuantity, prepaidUOM, quantity, price, priceChangeOption, priceIncreasePercentage, pricingSummary, originalListPrice, processedThroughDate, rolloverApply, rolloverPeriodLength, rolloverPeriods, prorationOption, segment, specificEndDate, subscriptionChargeIntervalPricing, tcv, tiers, triggerDate, billingPeriodAlignment, triggerEvent, upToPeriods, upToPeriodsType, validityPeriodType, salesPrice, accountingCode, revenueRecognitionCode, revRecTriggerCondition, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RatePlanChargeSegmentInfo {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" amendedByOrderOn: ").append(toIndentedString(amendedByOrderOn)).append("\n");
sb.append(" applyDiscountTo: ").append(toIndentedString(applyDiscountTo)).append("\n");
sb.append(" chargeFunction: ").append(toIndentedString(chargeFunction)).append("\n");
sb.append(" chargeModelConfiguration: ").append(toIndentedString(chargeModelConfiguration)).append("\n");
sb.append(" chargedThroughDate: ").append(toIndentedString(chargedThroughDate)).append("\n");
sb.append(" commitmentType: ").append(toIndentedString(commitmentType)).append("\n");
sb.append(" prepaidCommittedAmount: ").append(toIndentedString(prepaidCommittedAmount)).append("\n");
sb.append(" creditOption: ").append(toIndentedString(creditOption)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" deliverySchedule: ").append(toIndentedString(deliverySchedule)).append("\n");
sb.append(" numberOfDeliveries: ").append(toIndentedString(numberOfDeliveries)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" discountAmount: ").append(toIndentedString(discountAmount)).append("\n");
sb.append(" discountApplyDetails: ").append(toIndentedString(discountApplyDetails)).append("\n");
sb.append(" discountClass: ").append(toIndentedString(discountClass)).append("\n");
sb.append(" discountLevel: ").append(toIndentedString(discountLevel)).append("\n");
sb.append(" discountPercentage: ").append(toIndentedString(discountPercentage)).append("\n");
sb.append(" applyToBillingPeriodPartially: ").append(toIndentedString(applyToBillingPeriodPartially)).append("\n");
sb.append(" dmrc: ").append(toIndentedString(dmrc)).append("\n");
sb.append(" done: ").append(toIndentedString(done)).append("\n");
sb.append(" drawdownRate: ").append(toIndentedString(drawdownRate)).append("\n");
sb.append(" drawdownUom: ").append(toIndentedString(drawdownUom)).append("\n");
sb.append(" dtcv: ").append(toIndentedString(dtcv)).append("\n");
sb.append(" effectiveEndDate: ").append(toIndentedString(effectiveEndDate)).append("\n");
sb.append(" effectiveStartDate: ").append(toIndentedString(effectiveStartDate)).append("\n");
sb.append(" endDateCondition: ").append(toIndentedString(endDateCondition)).append("\n");
sb.append(" includedUnits: ").append(toIndentedString(includedUnits)).append("\n");
sb.append(" inputArgumentId: ").append(toIndentedString(inputArgumentId)).append("\n");
sb.append(" isCommitted: ").append(toIndentedString(isCommitted)).append("\n");
sb.append(" isPrepaid: ").append(toIndentedString(isPrepaid)).append("\n");
sb.append(" isRollover: ").append(toIndentedString(isRollover)).append("\n");
sb.append(" mrr: ").append(toIndentedString(mrr)).append("\n");
sb.append(" originalOrderDate: ").append(toIndentedString(originalOrderDate)).append("\n");
sb.append(" overagePrice: ").append(toIndentedString(overagePrice)).append("\n");
sb.append(" prepaidOperationType: ").append(toIndentedString(prepaidOperationType)).append("\n");
sb.append(" prepaidQuantity: ").append(toIndentedString(prepaidQuantity)).append("\n");
sb.append(" prepaidTotalQuantity: ").append(toIndentedString(prepaidTotalQuantity)).append("\n");
sb.append(" prepaidUOM: ").append(toIndentedString(prepaidUOM)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" price: ").append(toIndentedString(price)).append("\n");
sb.append(" priceChangeOption: ").append(toIndentedString(priceChangeOption)).append("\n");
sb.append(" priceIncreasePercentage: ").append(toIndentedString(priceIncreasePercentage)).append("\n");
sb.append(" pricingSummary: ").append(toIndentedString(pricingSummary)).append("\n");
sb.append(" originalListPrice: ").append(toIndentedString(originalListPrice)).append("\n");
sb.append(" processedThroughDate: ").append(toIndentedString(processedThroughDate)).append("\n");
sb.append(" rolloverApply: ").append(toIndentedString(rolloverApply)).append("\n");
sb.append(" rolloverPeriodLength: ").append(toIndentedString(rolloverPeriodLength)).append("\n");
sb.append(" rolloverPeriods: ").append(toIndentedString(rolloverPeriods)).append("\n");
sb.append(" prorationOption: ").append(toIndentedString(prorationOption)).append("\n");
sb.append(" segment: ").append(toIndentedString(segment)).append("\n");
sb.append(" specificEndDate: ").append(toIndentedString(specificEndDate)).append("\n");
sb.append(" subscriptionChargeIntervalPricing: ").append(toIndentedString(subscriptionChargeIntervalPricing)).append("\n");
sb.append(" tcv: ").append(toIndentedString(tcv)).append("\n");
sb.append(" tiers: ").append(toIndentedString(tiers)).append("\n");
sb.append(" triggerDate: ").append(toIndentedString(triggerDate)).append("\n");
sb.append(" billingPeriodAlignment: ").append(toIndentedString(billingPeriodAlignment)).append("\n");
sb.append(" triggerEvent: ").append(toIndentedString(triggerEvent)).append("\n");
sb.append(" upToPeriods: ").append(toIndentedString(upToPeriods)).append("\n");
sb.append(" upToPeriodsType: ").append(toIndentedString(upToPeriodsType)).append("\n");
sb.append(" validityPeriodType: ").append(toIndentedString(validityPeriodType)).append("\n");
sb.append(" salesPrice: ").append(toIndentedString(salesPrice)).append("\n");
sb.append(" accountingCode: ").append(toIndentedString(accountingCode)).append("\n");
sb.append(" revenueRecognitionCode: ").append(toIndentedString(revenueRecognitionCode)).append("\n");
sb.append(" revRecTriggerCondition: ").append(toIndentedString(revRecTriggerCondition)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("id");
openapiFields.add("amendedByOrderOn");
openapiFields.add("applyDiscountTo");
openapiFields.add("chargeFunction");
openapiFields.add("chargeModelConfiguration");
openapiFields.add("chargedThroughDate");
openapiFields.add("commitmentType");
openapiFields.add("prepaidCommittedAmount");
openapiFields.add("creditOption");
openapiFields.add("currency");
openapiFields.add("deliverySchedule");
openapiFields.add("numberOfDeliveries");
openapiFields.add("description");
openapiFields.add("discountAmount");
openapiFields.add("discountApplyDetails");
openapiFields.add("discountClass");
openapiFields.add("discountLevel");
openapiFields.add("discountPercentage");
openapiFields.add("applyToBillingPeriodPartially");
openapiFields.add("dmrc");
openapiFields.add("done");
openapiFields.add("drawdownRate");
openapiFields.add("drawdownUom");
openapiFields.add("dtcv");
openapiFields.add("effectiveEndDate");
openapiFields.add("effectiveStartDate");
openapiFields.add("endDateCondition");
openapiFields.add("includedUnits");
openapiFields.add("inputArgumentId");
openapiFields.add("isCommitted");
openapiFields.add("isPrepaid");
openapiFields.add("isRollover");
openapiFields.add("mrr");
openapiFields.add("originalOrderDate");
openapiFields.add("overagePrice");
openapiFields.add("prepaidOperationType");
openapiFields.add("prepaidQuantity");
openapiFields.add("prepaidTotalQuantity");
openapiFields.add("prepaidUOM");
openapiFields.add("quantity");
openapiFields.add("price");
openapiFields.add("priceChangeOption");
openapiFields.add("priceIncreasePercentage");
openapiFields.add("pricingSummary");
openapiFields.add("originalListPrice");
openapiFields.add("processedThroughDate");
openapiFields.add("rolloverApply");
openapiFields.add("rolloverPeriodLength");
openapiFields.add("rolloverPeriods");
openapiFields.add("prorationOption");
openapiFields.add("segment");
openapiFields.add("specificEndDate");
openapiFields.add("subscriptionChargeIntervalPricing");
openapiFields.add("tcv");
openapiFields.add("tiers");
openapiFields.add("triggerDate");
openapiFields.add("billingPeriodAlignment");
openapiFields.add("triggerEvent");
openapiFields.add("upToPeriods");
openapiFields.add("upToPeriodsType");
openapiFields.add("validityPeriodType");
openapiFields.add("salesPrice");
openapiFields.add("accountingCode");
openapiFields.add("revenueRecognitionCode");
openapiFields.add("revRecTriggerCondition");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to RatePlanChargeSegmentInfo
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!RatePlanChargeSegmentInfo.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in RatePlanChargeSegmentInfo is not found in the empty JSON string", RatePlanChargeSegmentInfo.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
// validate the optional field `applyDiscountTo`
if (jsonObj.get("applyDiscountTo") != null && !jsonObj.get("applyDiscountTo").isJsonNull()) {
ApplyDiscountTo.validateJsonElement(jsonObj.get("applyDiscountTo"));
}
// validate the optional field `chargeFunction`
if (jsonObj.get("chargeFunction") != null && !jsonObj.get("chargeFunction").isJsonNull()) {
ChargeFunction.validateJsonElement(jsonObj.get("chargeFunction"));
}
// validate the optional field `chargeModelConfiguration`
if (jsonObj.get("chargeModelConfiguration") != null && !jsonObj.get("chargeModelConfiguration").isJsonNull()) {
ChargeModelConfigurationForSubscription.validateJsonElement(jsonObj.get("chargeModelConfiguration"));
}
// validate the optional field `commitmentType`
if (jsonObj.get("commitmentType") != null && !jsonObj.get("commitmentType").isJsonNull()) {
CommitmentType.validateJsonElement(jsonObj.get("commitmentType"));
}
if ((jsonObj.get("prepaidCommittedAmount") != null && !jsonObj.get("prepaidCommittedAmount").isJsonNull()) && !jsonObj.get("prepaidCommittedAmount").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `prepaidCommittedAmount` to be a primitive type in the JSON string but got `%s`", jsonObj.get("prepaidCommittedAmount").toString()));
}
if ((jsonObj.get("creditOption") != null && !jsonObj.get("creditOption").isJsonNull()) && !jsonObj.get("creditOption").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creditOption` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creditOption").toString()));
}
// validate the optional field `creditOption`
if (jsonObj.get("creditOption") != null && !jsonObj.get("creditOption").isJsonNull()) {
CreditOptionEnum.validateJsonElement(jsonObj.get("creditOption"));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
// validate the optional field `deliverySchedule`
if (jsonObj.get("deliverySchedule") != null && !jsonObj.get("deliverySchedule").isJsonNull()) {
DeliverySchedule.validateJsonElement(jsonObj.get("deliverySchedule"));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if (jsonObj.get("discountApplyDetails") != null && !jsonObj.get("discountApplyDetails").isJsonNull()) {
JsonArray jsonArraydiscountApplyDetails = jsonObj.getAsJsonArray("discountApplyDetails");
if (jsonArraydiscountApplyDetails != null) {
// ensure the json data is an array
if (!jsonObj.get("discountApplyDetails").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `discountApplyDetails` to be an array in the JSON string but got `%s`", jsonObj.get("discountApplyDetails").toString()));
}
// validate the optional field `discountApplyDetails` (array)
for (int i = 0; i < jsonArraydiscountApplyDetails.size(); i++) {
DiscountApplyDetail.validateJsonElement(jsonArraydiscountApplyDetails.get(i));
};
}
}
if ((jsonObj.get("discountClass") != null && !jsonObj.get("discountClass").isJsonNull()) && !jsonObj.get("discountClass").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `discountClass` to be a primitive type in the JSON string but got `%s`", jsonObj.get("discountClass").toString()));
}
// validate the optional field `discountLevel`
if (jsonObj.get("discountLevel") != null && !jsonObj.get("discountLevel").isJsonNull()) {
DiscountLevel.validateJsonElement(jsonObj.get("discountLevel"));
}
if ((jsonObj.get("drawdownUom") != null && !jsonObj.get("drawdownUom").isJsonNull()) && !jsonObj.get("drawdownUom").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `drawdownUom` to be a primitive type in the JSON string but got `%s`", jsonObj.get("drawdownUom").toString()));
}
// validate the optional field `endDateCondition`
if (jsonObj.get("endDateCondition") != null && !jsonObj.get("endDateCondition").isJsonNull()) {
EndDateCondition.validateJsonElement(jsonObj.get("endDateCondition"));
}
if ((jsonObj.get("inputArgumentId") != null && !jsonObj.get("inputArgumentId").isJsonNull()) && !jsonObj.get("inputArgumentId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `inputArgumentId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("inputArgumentId").toString()));
}
if ((jsonObj.get("prepaidOperationType") != null && !jsonObj.get("prepaidOperationType").isJsonNull()) && !jsonObj.get("prepaidOperationType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `prepaidOperationType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("prepaidOperationType").toString()));
}
// validate the optional field `prepaidOperationType`
if (jsonObj.get("prepaidOperationType") != null && !jsonObj.get("prepaidOperationType").isJsonNull()) {
PrepaidOperationTypeEnum.validateJsonElement(jsonObj.get("prepaidOperationType"));
}
if ((jsonObj.get("prepaidUOM") != null && !jsonObj.get("prepaidUOM").isJsonNull()) && !jsonObj.get("prepaidUOM").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `prepaidUOM` to be a primitive type in the JSON string but got `%s`", jsonObj.get("prepaidUOM").toString()));
}
// validate the optional field `priceChangeOption`
if (jsonObj.get("priceChangeOption") != null && !jsonObj.get("priceChangeOption").isJsonNull()) {
PriceChangeOption.validateJsonElement(jsonObj.get("priceChangeOption"));
}
if ((jsonObj.get("pricingSummary") != null && !jsonObj.get("pricingSummary").isJsonNull()) && !jsonObj.get("pricingSummary").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `pricingSummary` to be a primitive type in the JSON string but got `%s`", jsonObj.get("pricingSummary").toString()));
}
if ((jsonObj.get("rolloverApply") != null && !jsonObj.get("rolloverApply").isJsonNull()) && !jsonObj.get("rolloverApply").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `rolloverApply` to be a primitive type in the JSON string but got `%s`", jsonObj.get("rolloverApply").toString()));
}
// validate the optional field `rolloverApply`
if (jsonObj.get("rolloverApply") != null && !jsonObj.get("rolloverApply").isJsonNull()) {
RolloverApplyEnum.validateJsonElement(jsonObj.get("rolloverApply"));
}
if ((jsonObj.get("prorationOption") != null && !jsonObj.get("prorationOption").isJsonNull()) && !jsonObj.get("prorationOption").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `prorationOption` to be a primitive type in the JSON string but got `%s`", jsonObj.get("prorationOption").toString()));
}
if (jsonObj.get("subscriptionChargeIntervalPricing") != null && !jsonObj.get("subscriptionChargeIntervalPricing").isJsonNull()) {
JsonArray jsonArraysubscriptionChargeIntervalPricing = jsonObj.getAsJsonArray("subscriptionChargeIntervalPricing");
if (jsonArraysubscriptionChargeIntervalPricing != null) {
// ensure the json data is an array
if (!jsonObj.get("subscriptionChargeIntervalPricing").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionChargeIntervalPricing` to be an array in the JSON string but got `%s`", jsonObj.get("subscriptionChargeIntervalPricing").toString()));
}
// validate the optional field `subscriptionChargeIntervalPricing` (array)
for (int i = 0; i < jsonArraysubscriptionChargeIntervalPricing.size(); i++) {
IntervalPricing.validateJsonElement(jsonArraysubscriptionChargeIntervalPricing.get(i));
};
}
}
if (jsonObj.get("tiers") != null && !jsonObj.get("tiers").isJsonNull()) {
JsonArray jsonArraytiers = jsonObj.getAsJsonArray("tiers");
if (jsonArraytiers != null) {
// ensure the json data is an array
if (!jsonObj.get("tiers").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `tiers` to be an array in the JSON string but got `%s`", jsonObj.get("tiers").toString()));
}
// validate the optional field `tiers` (array)
for (int i = 0; i < jsonArraytiers.size(); i++) {
RatePlanChargeTier.validateJsonElement(jsonArraytiers.get(i));
};
}
}
// validate the optional field `billingPeriodAlignment`
if (jsonObj.get("billingPeriodAlignment") != null && !jsonObj.get("billingPeriodAlignment").isJsonNull()) {
BillingPeriodAlignment.validateJsonElement(jsonObj.get("billingPeriodAlignment"));
}
// validate the optional field `triggerEvent`
if (jsonObj.get("triggerEvent") != null && !jsonObj.get("triggerEvent").isJsonNull()) {
TriggerEvent.validateJsonElement(jsonObj.get("triggerEvent"));
}
// validate the optional field `upToPeriodsType`
if (jsonObj.get("upToPeriodsType") != null && !jsonObj.get("upToPeriodsType").isJsonNull()) {
UpToPeriodsType.validateJsonElement(jsonObj.get("upToPeriodsType"));
}
// validate the optional field `validityPeriodType`
if (jsonObj.get("validityPeriodType") != null && !jsonObj.get("validityPeriodType").isJsonNull()) {
ValidityPeriodType.validateJsonElement(jsonObj.get("validityPeriodType"));
}
if ((jsonObj.get("accountingCode") != null && !jsonObj.get("accountingCode").isJsonNull()) && !jsonObj.get("accountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountingCode").toString()));
}
if ((jsonObj.get("revenueRecognitionCode") != null && !jsonObj.get("revenueRecognitionCode").isJsonNull()) && !jsonObj.get("revenueRecognitionCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueRecognitionCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueRecognitionCode").toString()));
}
if ((jsonObj.get("revRecTriggerCondition") != null && !jsonObj.get("revRecTriggerCondition").isJsonNull()) && !jsonObj.get("revRecTriggerCondition").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revRecTriggerCondition` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revRecTriggerCondition").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!RatePlanChargeSegmentInfo.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'RatePlanChargeSegmentInfo' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(RatePlanChargeSegmentInfo.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, RatePlanChargeSegmentInfo value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public RatePlanChargeSegmentInfo read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
RatePlanChargeSegmentInfo instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of RatePlanChargeSegmentInfo given an JSON string
*
* @param jsonString JSON string
* @return An instance of RatePlanChargeSegmentInfo
* @throws IOException if the JSON string is invalid with respect to RatePlanChargeSegmentInfo
*/
public static RatePlanChargeSegmentInfo fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, RatePlanChargeSegmentInfo.class);
}
/**
* Convert an instance of RatePlanChargeSegmentInfo to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy