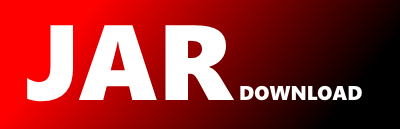
com.zuora.model.RefundResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.FailedReason;
import com.zuora.model.GatewayState;
import com.zuora.model.GetRefundCreditMemoFinanceInformation;
import com.zuora.model.PaymentMethodType;
import com.zuora.model.RefundStatus;
import com.zuora.model.RefundTransactionType;
import com.zuora.model.RefundType;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* RefundResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class RefundResponse {
public static final String SERIALIZED_NAME_ACCOUNT_ID = "accountId";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private Double amount;
public static final String SERIALIZED_NAME_CANCELLED_ON = "cancelledOn";
@SerializedName(SERIALIZED_NAME_CANCELLED_ON)
private String cancelledOn;
public static final String SERIALIZED_NAME_COMMENT = "comment";
@SerializedName(SERIALIZED_NAME_COMMENT)
private String comment;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_CREDIT_MEMO_ID = "creditMemoId";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_ID)
private String creditMemoId;
public static final String SERIALIZED_NAME_FINANCE_INFORMATION = "financeInformation";
@SerializedName(SERIALIZED_NAME_FINANCE_INFORMATION)
private GetRefundCreditMemoFinanceInformation financeInformation;
public static final String SERIALIZED_NAME_GATEWAY_ID = "gatewayId";
@SerializedName(SERIALIZED_NAME_GATEWAY_ID)
private String gatewayId;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY_NUMBER = "paymentGatewayNumber";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY_NUMBER)
private String paymentGatewayNumber;
public static final String SERIALIZED_NAME_GATEWAY_RECONCILIATION_REASON = "gatewayReconciliationReason";
@SerializedName(SERIALIZED_NAME_GATEWAY_RECONCILIATION_REASON)
private String gatewayReconciliationReason;
public static final String SERIALIZED_NAME_GATEWAY_RECONCILIATION_STATUS = "gatewayReconciliationStatus";
@SerializedName(SERIALIZED_NAME_GATEWAY_RECONCILIATION_STATUS)
private String gatewayReconciliationStatus;
public static final String SERIALIZED_NAME_GATEWAY_RESPONSE = "gatewayResponse";
@SerializedName(SERIALIZED_NAME_GATEWAY_RESPONSE)
private String gatewayResponse;
public static final String SERIALIZED_NAME_GATEWAY_RESPONSE_CODE = "gatewayResponseCode";
@SerializedName(SERIALIZED_NAME_GATEWAY_RESPONSE_CODE)
private String gatewayResponseCode;
public static final String SERIALIZED_NAME_GATEWAY_STATE = "gatewayState";
@SerializedName(SERIALIZED_NAME_GATEWAY_STATE)
private GatewayState gatewayState;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_MARKED_FOR_SUBMISSION_ON = "markedForSubmissionOn";
@SerializedName(SERIALIZED_NAME_MARKED_FOR_SUBMISSION_ON)
private String markedForSubmissionOn;
public static final String SERIALIZED_NAME_METHOD_TYPE = "methodType";
@SerializedName(SERIALIZED_NAME_METHOD_TYPE)
private PaymentMethodType methodType;
public static final String SERIALIZED_NAME_NUMBER = "number";
@SerializedName(SERIALIZED_NAME_NUMBER)
private String number;
public static final String SERIALIZED_NAME_PAYMENT_ID = "paymentId";
@SerializedName(SERIALIZED_NAME_PAYMENT_ID)
private String paymentId;
public static final String SERIALIZED_NAME_PAYMENT_NUMBER = "paymentNumber";
@SerializedName(SERIALIZED_NAME_PAYMENT_NUMBER)
private String paymentNumber;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "paymentMethodId";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_SNAPSHOT_ID = "paymentMethodSnapshotId";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_SNAPSHOT_ID)
private String paymentMethodSnapshotId;
public static final String SERIALIZED_NAME_PAYOUT_ID = "payoutId";
@SerializedName(SERIALIZED_NAME_PAYOUT_ID)
private String payoutId;
public static final String SERIALIZED_NAME_REASON_CODE = "reasonCode";
@SerializedName(SERIALIZED_NAME_REASON_CODE)
private String reasonCode;
public static final String SERIALIZED_NAME_REFERENCE_ID = "referenceId";
@SerializedName(SERIALIZED_NAME_REFERENCE_ID)
private String referenceId;
public static final String SERIALIZED_NAME_REFUND_DATE = "refundDate";
@SerializedName(SERIALIZED_NAME_REFUND_DATE)
private String refundDate;
public static final String SERIALIZED_NAME_REFUND_TRANSACTION_TIME = "refundTransactionTime";
@SerializedName(SERIALIZED_NAME_REFUND_TRANSACTION_TIME)
private String refundTransactionTime;
public static final String SERIALIZED_NAME_SECOND_REFUND_REFERENCE_ID = "secondRefundReferenceId";
@SerializedName(SERIALIZED_NAME_SECOND_REFUND_REFERENCE_ID)
private String secondRefundReferenceId;
public static final String SERIALIZED_NAME_SETTLED_ON = "settledOn";
@SerializedName(SERIALIZED_NAME_SETTLED_ON)
private String settledOn;
public static final String SERIALIZED_NAME_SOFT_DESCRIPTOR = "softDescriptor";
@SerializedName(SERIALIZED_NAME_SOFT_DESCRIPTOR)
private String softDescriptor;
public static final String SERIALIZED_NAME_SOFT_DESCRIPTOR_PHONE = "softDescriptorPhone";
@SerializedName(SERIALIZED_NAME_SOFT_DESCRIPTOR_PHONE)
private String softDescriptorPhone;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private RefundStatus status;
public static final String SERIALIZED_NAME_SUBMITTED_ON = "submittedOn";
@SerializedName(SERIALIZED_NAME_SUBMITTED_ON)
private String submittedOn;
public static final String SERIALIZED_NAME_ORGANIZATION_LABEL = "organizationLabel";
@SerializedName(SERIALIZED_NAME_ORGANIZATION_LABEL)
private String organizationLabel;
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private RefundType type;
public static final String SERIALIZED_NAME_REFUND_TRANSACTION_TYPE = "refundTransactionType";
@SerializedName(SERIALIZED_NAME_REFUND_TRANSACTION_TYPE)
private RefundTransactionType refundTransactionType;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public static final String SERIALIZED_NAME_PROCESS_ID = "processId";
@SerializedName(SERIALIZED_NAME_PROCESS_ID)
private String processId;
public static final String SERIALIZED_NAME_REQUEST_ID = "requestId";
@SerializedName(SERIALIZED_NAME_REQUEST_ID)
private String requestId;
public static final String SERIALIZED_NAME_REASONS = "reasons";
@SerializedName(SERIALIZED_NAME_REASONS)
private List reasons;
public static final String SERIALIZED_NAME_SUCCESS = "success";
@SerializedName(SERIALIZED_NAME_SUCCESS)
private Boolean success;
public RefundResponse() {
}
public RefundResponse accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* The ID of the account associated with this refund. Zuora associates the refund automatically with the account from the associated payment or credit memo.
* @return accountId
*/
@javax.annotation.Nullable
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public RefundResponse amount(Double amount) {
this.amount = amount;
return this;
}
/**
* The total amount of the refund.
* @return amount
*/
@javax.annotation.Nullable
public Double getAmount() {
return amount;
}
public void setAmount(Double amount) {
this.amount = amount;
}
public RefundResponse cancelledOn(String cancelledOn) {
this.cancelledOn = cancelledOn;
return this;
}
/**
* The date and time when the refund was cancelled, in `yyyy-mm-dd hh:mm:ss` format.
* @return cancelledOn
*/
@javax.annotation.Nullable
public String getCancelledOn() {
return cancelledOn;
}
public void setCancelledOn(String cancelledOn) {
this.cancelledOn = cancelledOn;
}
public RefundResponse comment(String comment) {
this.comment = comment;
return this;
}
/**
* Comments about the refund.
* @return comment
*/
@javax.annotation.Nullable
public String getComment() {
return comment;
}
public void setComment(String comment) {
this.comment = comment;
}
public RefundResponse createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* The ID of the Zuora user who created the refund.
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public RefundResponse createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* The date and time when the refund was created, in `yyyy-mm-dd hh:mm:ss` format. For example, 2017-03-01 15:31:10.
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public RefundResponse creditMemoId(String creditMemoId) {
this.creditMemoId = creditMemoId;
return this;
}
/**
* The ID of the credit memo that is refunded.
* @return creditMemoId
*/
@javax.annotation.Nullable
public String getCreditMemoId() {
return creditMemoId;
}
public void setCreditMemoId(String creditMemoId) {
this.creditMemoId = creditMemoId;
}
public RefundResponse financeInformation(GetRefundCreditMemoFinanceInformation financeInformation) {
this.financeInformation = financeInformation;
return this;
}
/**
* Get financeInformation
* @return financeInformation
*/
@javax.annotation.Nullable
public GetRefundCreditMemoFinanceInformation getFinanceInformation() {
return financeInformation;
}
public void setFinanceInformation(GetRefundCreditMemoFinanceInformation financeInformation) {
this.financeInformation = financeInformation;
}
public RefundResponse gatewayId(String gatewayId) {
this.gatewayId = gatewayId;
return this;
}
/**
* The ID of the gateway instance that processes the refund.
* @return gatewayId
*/
@javax.annotation.Nullable
public String getGatewayId() {
return gatewayId;
}
public void setGatewayId(String gatewayId) {
this.gatewayId = gatewayId;
}
public RefundResponse paymentGatewayNumber(String paymentGatewayNumber) {
this.paymentGatewayNumber = paymentGatewayNumber;
return this;
}
/**
* Get paymentGatewayNumber
* @return paymentGatewayNumber
*/
@javax.annotation.Nullable
public String getPaymentGatewayNumber() {
return paymentGatewayNumber;
}
public void setPaymentGatewayNumber(String paymentGatewayNumber) {
this.paymentGatewayNumber = paymentGatewayNumber;
}
public RefundResponse gatewayReconciliationReason(String gatewayReconciliationReason) {
this.gatewayReconciliationReason = gatewayReconciliationReason;
return this;
}
/**
* The reason of gateway reconciliation.
* @return gatewayReconciliationReason
*/
@javax.annotation.Nullable
public String getGatewayReconciliationReason() {
return gatewayReconciliationReason;
}
public void setGatewayReconciliationReason(String gatewayReconciliationReason) {
this.gatewayReconciliationReason = gatewayReconciliationReason;
}
public RefundResponse gatewayReconciliationStatus(String gatewayReconciliationStatus) {
this.gatewayReconciliationStatus = gatewayReconciliationStatus;
return this;
}
/**
* The status of gateway reconciliation.
* @return gatewayReconciliationStatus
*/
@javax.annotation.Nullable
public String getGatewayReconciliationStatus() {
return gatewayReconciliationStatus;
}
public void setGatewayReconciliationStatus(String gatewayReconciliationStatus) {
this.gatewayReconciliationStatus = gatewayReconciliationStatus;
}
public RefundResponse gatewayResponse(String gatewayResponse) {
this.gatewayResponse = gatewayResponse;
return this;
}
/**
* The message returned from the payment gateway for the refund. This message is gateway-dependent.
* @return gatewayResponse
*/
@javax.annotation.Nullable
public String getGatewayResponse() {
return gatewayResponse;
}
public void setGatewayResponse(String gatewayResponse) {
this.gatewayResponse = gatewayResponse;
}
public RefundResponse gatewayResponseCode(String gatewayResponseCode) {
this.gatewayResponseCode = gatewayResponseCode;
return this;
}
/**
* The code returned from the payment gateway for the refund. This code is gateway-dependent.
* @return gatewayResponseCode
*/
@javax.annotation.Nullable
public String getGatewayResponseCode() {
return gatewayResponseCode;
}
public void setGatewayResponseCode(String gatewayResponseCode) {
this.gatewayResponseCode = gatewayResponseCode;
}
public RefundResponse gatewayState(GatewayState gatewayState) {
this.gatewayState = gatewayState;
return this;
}
/**
* Get gatewayState
* @return gatewayState
*/
@javax.annotation.Nullable
public GatewayState getGatewayState() {
return gatewayState;
}
public void setGatewayState(GatewayState gatewayState) {
this.gatewayState = gatewayState;
}
public RefundResponse id(String id) {
this.id = id;
return this;
}
/**
* The ID of the refund.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public RefundResponse markedForSubmissionOn(String markedForSubmissionOn) {
this.markedForSubmissionOn = markedForSubmissionOn;
return this;
}
/**
* The date and time when a refund was marked and waiting for batch submission to the payment process, in `yyyy-mm-dd hh:mm:ss` format.
* @return markedForSubmissionOn
*/
@javax.annotation.Nullable
public String getMarkedForSubmissionOn() {
return markedForSubmissionOn;
}
public void setMarkedForSubmissionOn(String markedForSubmissionOn) {
this.markedForSubmissionOn = markedForSubmissionOn;
}
public RefundResponse methodType(PaymentMethodType methodType) {
this.methodType = methodType;
return this;
}
/**
* Get methodType
* @return methodType
*/
@javax.annotation.Nullable
public PaymentMethodType getMethodType() {
return methodType;
}
public void setMethodType(PaymentMethodType methodType) {
this.methodType = methodType;
}
public RefundResponse number(String number) {
this.number = number;
return this;
}
/**
* The unique identification number of the refund.
* @return number
*/
@javax.annotation.Nullable
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public RefundResponse paymentId(String paymentId) {
this.paymentId = paymentId;
return this;
}
/**
* The ID of the payment that is refunded.
* @return paymentId
*/
@javax.annotation.Nullable
public String getPaymentId() {
return paymentId;
}
public void setPaymentId(String paymentId) {
this.paymentId = paymentId;
}
public RefundResponse paymentNumber(String paymentNumber) {
this.paymentNumber = paymentNumber;
return this;
}
/**
* Get paymentNumber
* @return paymentNumber
*/
@javax.annotation.Nullable
public String getPaymentNumber() {
return paymentNumber;
}
public void setPaymentNumber(String paymentNumber) {
this.paymentNumber = paymentNumber;
}
public RefundResponse paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
return this;
}
/**
* The unique ID of the payment method that the customer used to make the refund.
* @return paymentMethodId
*/
@javax.annotation.Nullable
public String getPaymentMethodId() {
return paymentMethodId;
}
public void setPaymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
}
public RefundResponse paymentMethodSnapshotId(String paymentMethodSnapshotId) {
this.paymentMethodSnapshotId = paymentMethodSnapshotId;
return this;
}
/**
* The unique ID of the payment method snapshot, which is a copy of the particular payment method used in a transaction.
* @return paymentMethodSnapshotId
*/
@javax.annotation.Nullable
public String getPaymentMethodSnapshotId() {
return paymentMethodSnapshotId;
}
public void setPaymentMethodSnapshotId(String paymentMethodSnapshotId) {
this.paymentMethodSnapshotId = paymentMethodSnapshotId;
}
public RefundResponse payoutId(String payoutId) {
this.payoutId = payoutId;
return this;
}
/**
* The payout ID of the refund from the gateway side.
* @return payoutId
*/
@javax.annotation.Nullable
public String getPayoutId() {
return payoutId;
}
public void setPayoutId(String payoutId) {
this.payoutId = payoutId;
}
public RefundResponse reasonCode(String reasonCode) {
this.reasonCode = reasonCode;
return this;
}
/**
* A code identifying the reason for the transaction.
* @return reasonCode
*/
@javax.annotation.Nullable
public String getReasonCode() {
return reasonCode;
}
public void setReasonCode(String reasonCode) {
this.reasonCode = reasonCode;
}
public RefundResponse referenceId(String referenceId) {
this.referenceId = referenceId;
return this;
}
/**
* The transaction ID returned by the payment gateway for an electronic refund. Use this field to reconcile refunds between your gateway and Zuora Payments.
* @return referenceId
*/
@javax.annotation.Nullable
public String getReferenceId() {
return referenceId;
}
public void setReferenceId(String referenceId) {
this.referenceId = referenceId;
}
public RefundResponse refundDate(String refundDate) {
this.refundDate = refundDate;
return this;
}
/**
* The date when the refund takes effect, in `yyyy-mm-dd` format. For example, 2017-03-01.
* @return refundDate
*/
@javax.annotation.Nullable
public String getRefundDate() {
return refundDate;
}
public void setRefundDate(String refundDate) {
this.refundDate = refundDate;
}
public RefundResponse refundTransactionTime(String refundTransactionTime) {
this.refundTransactionTime = refundTransactionTime;
return this;
}
/**
* The date and time when the refund was issued, in `yyyy-mm-dd hh:mm:ss` format.
* @return refundTransactionTime
*/
@javax.annotation.Nullable
public String getRefundTransactionTime() {
return refundTransactionTime;
}
public void setRefundTransactionTime(String refundTransactionTime) {
this.refundTransactionTime = refundTransactionTime;
}
public RefundResponse secondRefundReferenceId(String secondRefundReferenceId) {
this.secondRefundReferenceId = secondRefundReferenceId;
return this;
}
/**
* The transaction ID returned by the payment gateway if there is an additional transaction for the refund. Use this field to reconcile payments between your gateway and Zuora Payments.
* @return secondRefundReferenceId
*/
@javax.annotation.Nullable
public String getSecondRefundReferenceId() {
return secondRefundReferenceId;
}
public void setSecondRefundReferenceId(String secondRefundReferenceId) {
this.secondRefundReferenceId = secondRefundReferenceId;
}
public RefundResponse settledOn(String settledOn) {
this.settledOn = settledOn;
return this;
}
/**
* The date and time when the refund was settled in the payment processor, in `yyyy-mm-dd hh:mm:ss` format. This field is used by the Spectrum gateway only and not applicable to other gateways.
* @return settledOn
*/
@javax.annotation.Nullable
public String getSettledOn() {
return settledOn;
}
public void setSettledOn(String settledOn) {
this.settledOn = settledOn;
}
public RefundResponse softDescriptor(String softDescriptor) {
this.softDescriptor = softDescriptor;
return this;
}
/**
* A payment gateway-specific field that maps Zuora to other gateways.
* @return softDescriptor
*/
@javax.annotation.Nullable
public String getSoftDescriptor() {
return softDescriptor;
}
public void setSoftDescriptor(String softDescriptor) {
this.softDescriptor = softDescriptor;
}
public RefundResponse softDescriptorPhone(String softDescriptorPhone) {
this.softDescriptorPhone = softDescriptorPhone;
return this;
}
/**
* A payment gateway-specific field that maps Zuora to other gateways.
* @return softDescriptorPhone
*/
@javax.annotation.Nullable
public String getSoftDescriptorPhone() {
return softDescriptorPhone;
}
public void setSoftDescriptorPhone(String softDescriptorPhone) {
this.softDescriptorPhone = softDescriptorPhone;
}
public RefundResponse status(RefundStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public RefundStatus getStatus() {
return status;
}
public void setStatus(RefundStatus status) {
this.status = status;
}
public RefundResponse submittedOn(String submittedOn) {
this.submittedOn = submittedOn;
return this;
}
/**
* The date and time when the refund was submitted, in `yyyy-mm-dd hh:mm:ss` format.
* @return submittedOn
*/
@javax.annotation.Nullable
public String getSubmittedOn() {
return submittedOn;
}
public void setSubmittedOn(String submittedOn) {
this.submittedOn = submittedOn;
}
public RefundResponse organizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
return this;
}
/**
* Get organizationLabel
* @return organizationLabel
*/
@javax.annotation.Nullable
public String getOrganizationLabel() {
return organizationLabel;
}
public void setOrganizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
}
public RefundResponse type(RefundType type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
*/
@javax.annotation.Nullable
public RefundType getType() {
return type;
}
public void setType(RefundType type) {
this.type = type;
}
public RefundResponse refundTransactionType(RefundTransactionType refundTransactionType) {
this.refundTransactionType = refundTransactionType;
return this;
}
/**
* Get refundTransactionType
* @return refundTransactionType
*/
@javax.annotation.Nullable
public RefundTransactionType getRefundTransactionType() {
return refundTransactionType;
}
public void setRefundTransactionType(RefundTransactionType refundTransactionType) {
this.refundTransactionType = refundTransactionType;
}
public RefundResponse updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* The ID of the Zuora user who last updated the refund.
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public RefundResponse updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* The date and time when the refund was last updated, in `yyyy-mm-dd hh:mm:ss` format. For example, 2017-03-02 15:36:10.
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
public RefundResponse processId(String processId) {
this.processId = processId;
return this;
}
/**
* The Id of the process that handle the operation.
* @return processId
*/
@javax.annotation.Nullable
public String getProcessId() {
return processId;
}
public void setProcessId(String processId) {
this.processId = processId;
}
public RefundResponse requestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* Unique request identifier. If you need to contact us about a specific request, providing the request identifier will ensure the fastest possible resolution.
* @return requestId
*/
@javax.annotation.Nullable
public String getRequestId() {
return requestId;
}
public void setRequestId(String requestId) {
this.requestId = requestId;
}
public RefundResponse reasons(List reasons) {
this.reasons = reasons;
return this;
}
public RefundResponse addReasonsItem(FailedReason reasonsItem) {
if (this.reasons == null) {
this.reasons = new ArrayList<>();
}
this.reasons.add(reasonsItem);
return this;
}
/**
* Get reasons
* @return reasons
*/
@javax.annotation.Nullable
public List getReasons() {
return reasons;
}
public void setReasons(List reasons) {
this.reasons = reasons;
}
public RefundResponse success(Boolean success) {
this.success = success;
return this;
}
/**
* Indicates whether the call succeeded.
* @return success
*/
@javax.annotation.Nullable
public Boolean getSuccess() {
return success;
}
public void setSuccess(Boolean success) {
this.success = success;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the RefundResponse instance itself
*/
public RefundResponse putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RefundResponse refundResponse = (RefundResponse) o;
return Objects.equals(this.accountId, refundResponse.accountId) &&
Objects.equals(this.amount, refundResponse.amount) &&
Objects.equals(this.cancelledOn, refundResponse.cancelledOn) &&
Objects.equals(this.comment, refundResponse.comment) &&
Objects.equals(this.createdById, refundResponse.createdById) &&
Objects.equals(this.createdDate, refundResponse.createdDate) &&
Objects.equals(this.creditMemoId, refundResponse.creditMemoId) &&
Objects.equals(this.financeInformation, refundResponse.financeInformation) &&
Objects.equals(this.gatewayId, refundResponse.gatewayId) &&
Objects.equals(this.paymentGatewayNumber, refundResponse.paymentGatewayNumber) &&
Objects.equals(this.gatewayReconciliationReason, refundResponse.gatewayReconciliationReason) &&
Objects.equals(this.gatewayReconciliationStatus, refundResponse.gatewayReconciliationStatus) &&
Objects.equals(this.gatewayResponse, refundResponse.gatewayResponse) &&
Objects.equals(this.gatewayResponseCode, refundResponse.gatewayResponseCode) &&
Objects.equals(this.gatewayState, refundResponse.gatewayState) &&
Objects.equals(this.id, refundResponse.id) &&
Objects.equals(this.markedForSubmissionOn, refundResponse.markedForSubmissionOn) &&
Objects.equals(this.methodType, refundResponse.methodType) &&
Objects.equals(this.number, refundResponse.number) &&
Objects.equals(this.paymentId, refundResponse.paymentId) &&
Objects.equals(this.paymentNumber, refundResponse.paymentNumber) &&
Objects.equals(this.paymentMethodId, refundResponse.paymentMethodId) &&
Objects.equals(this.paymentMethodSnapshotId, refundResponse.paymentMethodSnapshotId) &&
Objects.equals(this.payoutId, refundResponse.payoutId) &&
Objects.equals(this.reasonCode, refundResponse.reasonCode) &&
Objects.equals(this.referenceId, refundResponse.referenceId) &&
Objects.equals(this.refundDate, refundResponse.refundDate) &&
Objects.equals(this.refundTransactionTime, refundResponse.refundTransactionTime) &&
Objects.equals(this.secondRefundReferenceId, refundResponse.secondRefundReferenceId) &&
Objects.equals(this.settledOn, refundResponse.settledOn) &&
Objects.equals(this.softDescriptor, refundResponse.softDescriptor) &&
Objects.equals(this.softDescriptorPhone, refundResponse.softDescriptorPhone) &&
Objects.equals(this.status, refundResponse.status) &&
Objects.equals(this.submittedOn, refundResponse.submittedOn) &&
Objects.equals(this.organizationLabel, refundResponse.organizationLabel) &&
Objects.equals(this.type, refundResponse.type) &&
Objects.equals(this.refundTransactionType, refundResponse.refundTransactionType) &&
Objects.equals(this.updatedById, refundResponse.updatedById) &&
Objects.equals(this.updatedDate, refundResponse.updatedDate) &&
Objects.equals(this.processId, refundResponse.processId) &&
Objects.equals(this.requestId, refundResponse.requestId) &&
Objects.equals(this.reasons, refundResponse.reasons) &&
Objects.equals(this.success, refundResponse.success)&&
Objects.equals(this.additionalProperties, refundResponse.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(accountId, amount, cancelledOn, comment, createdById, createdDate, creditMemoId, financeInformation, gatewayId, paymentGatewayNumber, gatewayReconciliationReason, gatewayReconciliationStatus, gatewayResponse, gatewayResponseCode, gatewayState, id, markedForSubmissionOn, methodType, number, paymentId, paymentNumber, paymentMethodId, paymentMethodSnapshotId, payoutId, reasonCode, referenceId, refundDate, refundTransactionTime, secondRefundReferenceId, settledOn, softDescriptor, softDescriptorPhone, status, submittedOn, organizationLabel, type, refundTransactionType, updatedById, updatedDate, processId, requestId, reasons, success, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RefundResponse {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" cancelledOn: ").append(toIndentedString(cancelledOn)).append("\n");
sb.append(" comment: ").append(toIndentedString(comment)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" creditMemoId: ").append(toIndentedString(creditMemoId)).append("\n");
sb.append(" financeInformation: ").append(toIndentedString(financeInformation)).append("\n");
sb.append(" gatewayId: ").append(toIndentedString(gatewayId)).append("\n");
sb.append(" paymentGatewayNumber: ").append(toIndentedString(paymentGatewayNumber)).append("\n");
sb.append(" gatewayReconciliationReason: ").append(toIndentedString(gatewayReconciliationReason)).append("\n");
sb.append(" gatewayReconciliationStatus: ").append(toIndentedString(gatewayReconciliationStatus)).append("\n");
sb.append(" gatewayResponse: ").append(toIndentedString(gatewayResponse)).append("\n");
sb.append(" gatewayResponseCode: ").append(toIndentedString(gatewayResponseCode)).append("\n");
sb.append(" gatewayState: ").append(toIndentedString(gatewayState)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" markedForSubmissionOn: ").append(toIndentedString(markedForSubmissionOn)).append("\n");
sb.append(" methodType: ").append(toIndentedString(methodType)).append("\n");
sb.append(" number: ").append(toIndentedString(number)).append("\n");
sb.append(" paymentId: ").append(toIndentedString(paymentId)).append("\n");
sb.append(" paymentNumber: ").append(toIndentedString(paymentNumber)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" paymentMethodSnapshotId: ").append(toIndentedString(paymentMethodSnapshotId)).append("\n");
sb.append(" payoutId: ").append(toIndentedString(payoutId)).append("\n");
sb.append(" reasonCode: ").append(toIndentedString(reasonCode)).append("\n");
sb.append(" referenceId: ").append(toIndentedString(referenceId)).append("\n");
sb.append(" refundDate: ").append(toIndentedString(refundDate)).append("\n");
sb.append(" refundTransactionTime: ").append(toIndentedString(refundTransactionTime)).append("\n");
sb.append(" secondRefundReferenceId: ").append(toIndentedString(secondRefundReferenceId)).append("\n");
sb.append(" settledOn: ").append(toIndentedString(settledOn)).append("\n");
sb.append(" softDescriptor: ").append(toIndentedString(softDescriptor)).append("\n");
sb.append(" softDescriptorPhone: ").append(toIndentedString(softDescriptorPhone)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" submittedOn: ").append(toIndentedString(submittedOn)).append("\n");
sb.append(" organizationLabel: ").append(toIndentedString(organizationLabel)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" refundTransactionType: ").append(toIndentedString(refundTransactionType)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" processId: ").append(toIndentedString(processId)).append("\n");
sb.append(" requestId: ").append(toIndentedString(requestId)).append("\n");
sb.append(" reasons: ").append(toIndentedString(reasons)).append("\n");
sb.append(" success: ").append(toIndentedString(success)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("accountId");
openapiFields.add("amount");
openapiFields.add("cancelledOn");
openapiFields.add("comment");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("creditMemoId");
openapiFields.add("financeInformation");
openapiFields.add("gatewayId");
openapiFields.add("paymentGatewayNumber");
openapiFields.add("gatewayReconciliationReason");
openapiFields.add("gatewayReconciliationStatus");
openapiFields.add("gatewayResponse");
openapiFields.add("gatewayResponseCode");
openapiFields.add("gatewayState");
openapiFields.add("id");
openapiFields.add("markedForSubmissionOn");
openapiFields.add("methodType");
openapiFields.add("number");
openapiFields.add("paymentId");
openapiFields.add("paymentNumber");
openapiFields.add("paymentMethodId");
openapiFields.add("paymentMethodSnapshotId");
openapiFields.add("payoutId");
openapiFields.add("reasonCode");
openapiFields.add("referenceId");
openapiFields.add("refundDate");
openapiFields.add("refundTransactionTime");
openapiFields.add("secondRefundReferenceId");
openapiFields.add("settledOn");
openapiFields.add("softDescriptor");
openapiFields.add("softDescriptorPhone");
openapiFields.add("status");
openapiFields.add("submittedOn");
openapiFields.add("organizationLabel");
openapiFields.add("type");
openapiFields.add("refundTransactionType");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
openapiFields.add("processId");
openapiFields.add("requestId");
openapiFields.add("reasons");
openapiFields.add("success");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to RefundResponse
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!RefundResponse.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in RefundResponse is not found in the empty JSON string", RefundResponse.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("accountId") != null && !jsonObj.get("accountId").isJsonNull()) && !jsonObj.get("accountId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountId").toString()));
}
if ((jsonObj.get("cancelledOn") != null && !jsonObj.get("cancelledOn").isJsonNull()) && !jsonObj.get("cancelledOn").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cancelledOn` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cancelledOn").toString()));
}
if ((jsonObj.get("comment") != null && !jsonObj.get("comment").isJsonNull()) && !jsonObj.get("comment").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `comment` to be a primitive type in the JSON string but got `%s`", jsonObj.get("comment").toString()));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("creditMemoId") != null && !jsonObj.get("creditMemoId").isJsonNull()) && !jsonObj.get("creditMemoId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creditMemoId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creditMemoId").toString()));
}
// validate the optional field `financeInformation`
if (jsonObj.get("financeInformation") != null && !jsonObj.get("financeInformation").isJsonNull()) {
GetRefundCreditMemoFinanceInformation.validateJsonElement(jsonObj.get("financeInformation"));
}
if ((jsonObj.get("gatewayId") != null && !jsonObj.get("gatewayId").isJsonNull()) && !jsonObj.get("gatewayId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayId").toString()));
}
if ((jsonObj.get("paymentGatewayNumber") != null && !jsonObj.get("paymentGatewayNumber").isJsonNull()) && !jsonObj.get("paymentGatewayNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentGatewayNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentGatewayNumber").toString()));
}
if ((jsonObj.get("gatewayReconciliationReason") != null && !jsonObj.get("gatewayReconciliationReason").isJsonNull()) && !jsonObj.get("gatewayReconciliationReason").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayReconciliationReason` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayReconciliationReason").toString()));
}
if ((jsonObj.get("gatewayReconciliationStatus") != null && !jsonObj.get("gatewayReconciliationStatus").isJsonNull()) && !jsonObj.get("gatewayReconciliationStatus").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayReconciliationStatus` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayReconciliationStatus").toString()));
}
if ((jsonObj.get("gatewayResponse") != null && !jsonObj.get("gatewayResponse").isJsonNull()) && !jsonObj.get("gatewayResponse").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayResponse` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayResponse").toString()));
}
if ((jsonObj.get("gatewayResponseCode") != null && !jsonObj.get("gatewayResponseCode").isJsonNull()) && !jsonObj.get("gatewayResponseCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayResponseCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayResponseCode").toString()));
}
if ((jsonObj.get("gatewayState") != null && !jsonObj.get("gatewayState").isJsonNull()) && !jsonObj.get("gatewayState").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayState` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayState").toString()));
}
// validate the optional field `gatewayState`
if (jsonObj.get("gatewayState") != null && !jsonObj.get("gatewayState").isJsonNull()) {
GatewayState.validateJsonElement(jsonObj.get("gatewayState"));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("markedForSubmissionOn") != null && !jsonObj.get("markedForSubmissionOn").isJsonNull()) && !jsonObj.get("markedForSubmissionOn").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `markedForSubmissionOn` to be a primitive type in the JSON string but got `%s`", jsonObj.get("markedForSubmissionOn").toString()));
}
if ((jsonObj.get("methodType") != null && !jsonObj.get("methodType").isJsonNull()) && !jsonObj.get("methodType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `methodType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("methodType").toString()));
}
// validate the optional field `methodType`
if (jsonObj.get("methodType") != null && !jsonObj.get("methodType").isJsonNull()) {
PaymentMethodType.validateJsonElement(jsonObj.get("methodType"));
}
if ((jsonObj.get("number") != null && !jsonObj.get("number").isJsonNull()) && !jsonObj.get("number").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `number` to be a primitive type in the JSON string but got `%s`", jsonObj.get("number").toString()));
}
if ((jsonObj.get("paymentId") != null && !jsonObj.get("paymentId").isJsonNull()) && !jsonObj.get("paymentId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentId").toString()));
}
if ((jsonObj.get("paymentNumber") != null && !jsonObj.get("paymentNumber").isJsonNull()) && !jsonObj.get("paymentNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentNumber").toString()));
}
if ((jsonObj.get("paymentMethodId") != null && !jsonObj.get("paymentMethodId").isJsonNull()) && !jsonObj.get("paymentMethodId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentMethodId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentMethodId").toString()));
}
if ((jsonObj.get("paymentMethodSnapshotId") != null && !jsonObj.get("paymentMethodSnapshotId").isJsonNull()) && !jsonObj.get("paymentMethodSnapshotId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentMethodSnapshotId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentMethodSnapshotId").toString()));
}
if ((jsonObj.get("payoutId") != null && !jsonObj.get("payoutId").isJsonNull()) && !jsonObj.get("payoutId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `payoutId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("payoutId").toString()));
}
if ((jsonObj.get("reasonCode") != null && !jsonObj.get("reasonCode").isJsonNull()) && !jsonObj.get("reasonCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `reasonCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("reasonCode").toString()));
}
if ((jsonObj.get("referenceId") != null && !jsonObj.get("referenceId").isJsonNull()) && !jsonObj.get("referenceId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `referenceId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("referenceId").toString()));
}
if ((jsonObj.get("refundDate") != null && !jsonObj.get("refundDate").isJsonNull()) && !jsonObj.get("refundDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `refundDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("refundDate").toString()));
}
if ((jsonObj.get("refundTransactionTime") != null && !jsonObj.get("refundTransactionTime").isJsonNull()) && !jsonObj.get("refundTransactionTime").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `refundTransactionTime` to be a primitive type in the JSON string but got `%s`", jsonObj.get("refundTransactionTime").toString()));
}
if ((jsonObj.get("secondRefundReferenceId") != null && !jsonObj.get("secondRefundReferenceId").isJsonNull()) && !jsonObj.get("secondRefundReferenceId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `secondRefundReferenceId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("secondRefundReferenceId").toString()));
}
if ((jsonObj.get("settledOn") != null && !jsonObj.get("settledOn").isJsonNull()) && !jsonObj.get("settledOn").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `settledOn` to be a primitive type in the JSON string but got `%s`", jsonObj.get("settledOn").toString()));
}
if ((jsonObj.get("softDescriptor") != null && !jsonObj.get("softDescriptor").isJsonNull()) && !jsonObj.get("softDescriptor").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `softDescriptor` to be a primitive type in the JSON string but got `%s`", jsonObj.get("softDescriptor").toString()));
}
if ((jsonObj.get("softDescriptorPhone") != null && !jsonObj.get("softDescriptorPhone").isJsonNull()) && !jsonObj.get("softDescriptorPhone").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `softDescriptorPhone` to be a primitive type in the JSON string but got `%s`", jsonObj.get("softDescriptorPhone").toString()));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
// validate the optional field `status`
if (jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) {
RefundStatus.validateJsonElement(jsonObj.get("status"));
}
if ((jsonObj.get("submittedOn") != null && !jsonObj.get("submittedOn").isJsonNull()) && !jsonObj.get("submittedOn").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `submittedOn` to be a primitive type in the JSON string but got `%s`", jsonObj.get("submittedOn").toString()));
}
if ((jsonObj.get("organizationLabel") != null && !jsonObj.get("organizationLabel").isJsonNull()) && !jsonObj.get("organizationLabel").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `organizationLabel` to be a primitive type in the JSON string but got `%s`", jsonObj.get("organizationLabel").toString()));
}
if ((jsonObj.get("type") != null && !jsonObj.get("type").isJsonNull()) && !jsonObj.get("type").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `type` to be a primitive type in the JSON string but got `%s`", jsonObj.get("type").toString()));
}
// validate the optional field `type`
if (jsonObj.get("type") != null && !jsonObj.get("type").isJsonNull()) {
RefundType.validateJsonElement(jsonObj.get("type"));
}
if ((jsonObj.get("refundTransactionType") != null && !jsonObj.get("refundTransactionType").isJsonNull()) && !jsonObj.get("refundTransactionType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `refundTransactionType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("refundTransactionType").toString()));
}
// validate the optional field `refundTransactionType`
if (jsonObj.get("refundTransactionType") != null && !jsonObj.get("refundTransactionType").isJsonNull()) {
RefundTransactionType.validateJsonElement(jsonObj.get("refundTransactionType"));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
if ((jsonObj.get("processId") != null && !jsonObj.get("processId").isJsonNull()) && !jsonObj.get("processId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `processId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("processId").toString()));
}
if ((jsonObj.get("requestId") != null && !jsonObj.get("requestId").isJsonNull()) && !jsonObj.get("requestId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `requestId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("requestId").toString()));
}
if (jsonObj.get("reasons") != null && !jsonObj.get("reasons").isJsonNull()) {
JsonArray jsonArrayreasons = jsonObj.getAsJsonArray("reasons");
if (jsonArrayreasons != null) {
// ensure the json data is an array
if (!jsonObj.get("reasons").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `reasons` to be an array in the JSON string but got `%s`", jsonObj.get("reasons").toString()));
}
// validate the optional field `reasons` (array)
for (int i = 0; i < jsonArrayreasons.size(); i++) {
FailedReason.validateJsonElement(jsonArrayreasons.get(i));
};
}
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!RefundResponse.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'RefundResponse' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(RefundResponse.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, RefundResponse value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public RefundResponse read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
RefundResponse instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of RefundResponse given an JSON string
*
* @param jsonString JSON string
* @return An instance of RefundResponse
* @throws IOException if the JSON string is invalid with respect to RefundResponse
*/
public static RefundResponse fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, RefundResponse.class);
}
/**
* Convert an instance of RefundResponse to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy