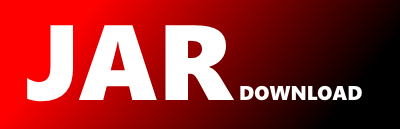
com.zuora.model.ResumeSubscriptionRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* ResumeSubscriptionRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class ResumeSubscriptionRequest {
public static final String SERIALIZED_NAME_APPLICATION_ORDER = "applicationOrder";
@SerializedName(SERIALIZED_NAME_APPLICATION_ORDER)
private List applicationOrder;
public static final String SERIALIZED_NAME_APPLY_CREDIT = "applyCredit";
@SerializedName(SERIALIZED_NAME_APPLY_CREDIT)
private Boolean applyCredit;
public static final String SERIALIZED_NAME_APPLY_CREDIT_BALANCE = "applyCreditBalance";
@SerializedName(SERIALIZED_NAME_APPLY_CREDIT_BALANCE)
private Boolean applyCreditBalance;
public static final String SERIALIZED_NAME_BOOKING_DATE = "bookingDate";
@SerializedName(SERIALIZED_NAME_BOOKING_DATE)
private LocalDate bookingDate;
public static final String SERIALIZED_NAME_COLLECT = "collect";
@SerializedName(SERIALIZED_NAME_COLLECT)
private Boolean collect;
public static final String SERIALIZED_NAME_CONTRACT_EFFECTIVE_DATE = "contractEffectiveDate";
@SerializedName(SERIALIZED_NAME_CONTRACT_EFFECTIVE_DATE)
private LocalDate contractEffectiveDate;
public static final String SERIALIZED_NAME_CREDIT_MEMO_REASON_CODE = "creditMemoReasonCode";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_REASON_CODE)
private String creditMemoReasonCode;
public static final String SERIALIZED_NAME_DOCUMENT_DATE = "documentDate";
@SerializedName(SERIALIZED_NAME_DOCUMENT_DATE)
private LocalDate documentDate;
public static final String SERIALIZED_NAME_EXTENDS_TERM = "extendsTerm";
@SerializedName(SERIALIZED_NAME_EXTENDS_TERM)
private Boolean extendsTerm;
public static final String SERIALIZED_NAME_INVOICE = "invoice";
@Deprecated
@SerializedName(SERIALIZED_NAME_INVOICE)
private Boolean invoice;
public static final String SERIALIZED_NAME_INVOICE_COLLECT = "invoiceCollect";
@Deprecated
@SerializedName(SERIALIZED_NAME_INVOICE_COLLECT)
private Boolean invoiceCollect;
public static final String SERIALIZED_NAME_INVOICE_TARGET_DATE = "invoiceTargetDate";
@Deprecated
@SerializedName(SERIALIZED_NAME_INVOICE_TARGET_DATE)
private LocalDate invoiceTargetDate;
public static final String SERIALIZED_NAME_ORDER_DATE = "orderDate";
@SerializedName(SERIALIZED_NAME_ORDER_DATE)
private LocalDate orderDate;
public static final String SERIALIZED_NAME_RESUME_PERIODS = "resumePeriods";
@SerializedName(SERIALIZED_NAME_RESUME_PERIODS)
private String resumePeriods;
public static final String SERIALIZED_NAME_RESUME_PERIODS_TYPE = "resumePeriodsType";
@SerializedName(SERIALIZED_NAME_RESUME_PERIODS_TYPE)
private String resumePeriodsType;
public static final String SERIALIZED_NAME_RESUME_POLICY = "resumePolicy";
@SerializedName(SERIALIZED_NAME_RESUME_POLICY)
private String resumePolicy;
public static final String SERIALIZED_NAME_RESUME_SPECIFIC_DATE = "resumeSpecificDate";
@SerializedName(SERIALIZED_NAME_RESUME_SPECIFIC_DATE)
private LocalDate resumeSpecificDate;
public static final String SERIALIZED_NAME_RUN_BILLING = "runBilling";
@SerializedName(SERIALIZED_NAME_RUN_BILLING)
private Boolean runBilling;
public static final String SERIALIZED_NAME_TARGET_DATE = "targetDate";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public ResumeSubscriptionRequest() {
}
public ResumeSubscriptionRequest applicationOrder(List applicationOrder) {
this.applicationOrder = applicationOrder;
return this;
}
public ResumeSubscriptionRequest addApplicationOrderItem(String applicationOrderItem) {
if (this.applicationOrder == null) {
this.applicationOrder = new ArrayList<>();
}
this.applicationOrder.add(applicationOrderItem);
return this;
}
/**
* The priority order to apply credit memos and/or unapplied payments to an invoice. Possible item values are: `CreditMemo`, `UnappliedPayment`. **Note:** - This field is valid only if the `applyCredit` field is set to `true`. - If no value is specified for this field, the default priority order is used, [\"CreditMemo\", \"UnappliedPayment\"], to apply credit memos first and then apply unapplied payments. - If only one item is specified, only the items of the spedified type are applied to invoices. For example, if the value is `[\"CreditMemo\"]`, only credit memos are used to apply to invoices.
* @return applicationOrder
*/
@javax.annotation.Nullable
public List getApplicationOrder() {
return applicationOrder;
}
public void setApplicationOrder(List applicationOrder) {
this.applicationOrder = applicationOrder;
}
public ResumeSubscriptionRequest applyCredit(Boolean applyCredit) {
this.applyCredit = applyCredit;
return this;
}
/**
* If the value is true, the credit memo or unapplied payment on the order account will be automatically applied to the invoices generated by this order. The credit memo generated by this order will not be automatically applied to any invoices. **Note:** This field is only available if you have [Invoice Settlement](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement) enabled. The Invoice Settlement feature is generally available as of Zuora Billing Release 296 (March 2021). This feature includes Unapplied Payments, Credit and Debit Memo, and Invoice Item Settlement. If you want to enable Invoice Settlement, see [Invoice Settlement Enablement and Checklist Guide](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement/Invoice_Settlement_Migration_Checklist_and_Guide) for more information.
* @return applyCredit
*/
@javax.annotation.Nullable
public Boolean getApplyCredit() {
return applyCredit;
}
public void setApplyCredit(Boolean applyCredit) {
this.applyCredit = applyCredit;
}
public ResumeSubscriptionRequest applyCreditBalance(Boolean applyCreditBalance) {
this.applyCreditBalance = applyCreditBalance;
return this;
}
/**
* Whether to automatically apply a credit balance to an invoice. If the value is `true`, the credit balance is applied to the invoice. If the value is `false`, no action is taken. To view the credit balance adjustment, retrieve the details of the invoice using the Get Invoices method. Prerequisite: `invoice` must be `true`. **Note:** - If you are using the field `invoiceCollect` rather than the field `invoice`, the `invoiceCollect` value must be `true`. - This field is deprecated if you have the Invoice Settlement feature enabled.
* @return applyCreditBalance
*/
@javax.annotation.Nullable
public Boolean getApplyCreditBalance() {
return applyCreditBalance;
}
public void setApplyCreditBalance(Boolean applyCreditBalance) {
this.applyCreditBalance = applyCreditBalance;
}
public ResumeSubscriptionRequest bookingDate(LocalDate bookingDate) {
this.bookingDate = bookingDate;
return this;
}
/**
* The booking date that you want to set for the amendment contract when you resume the subscription. If `extendsTerm` is `true`, which means you also extend the term, then this field is also the booking date for the Terms and Conditions amendment contract. This field must be in the `yyyy-mm-dd` format. The default value of this field is the current date when you make the API call.
* @return bookingDate
*/
@javax.annotation.Nullable
public LocalDate getBookingDate() {
return bookingDate;
}
public void setBookingDate(LocalDate bookingDate) {
this.bookingDate = bookingDate;
}
public ResumeSubscriptionRequest collect(Boolean collect) {
this.collect = collect;
return this;
}
/**
* Collects an automatic payment for a subscription. The collection generated in this operation is only for this subscription, not for the entire customer account. If the value is `true`, the automatic payment is collected. If the value is `false`, no action is taken. Prerequisite: The `invoice` or `runBilling` field must be `true`. **Note**: This field is only available if you set the `zuora-version` request header to `196.0` or later.
* @return collect
*/
@javax.annotation.Nullable
public Boolean getCollect() {
return collect;
}
public void setCollect(Boolean collect) {
this.collect = collect;
}
public ResumeSubscriptionRequest contractEffectiveDate(LocalDate contractEffectiveDate) {
this.contractEffectiveDate = contractEffectiveDate;
return this;
}
/**
* The date when the customer notifies you that they want to resume their subscription.
* @return contractEffectiveDate
*/
@javax.annotation.Nullable
public LocalDate getContractEffectiveDate() {
return contractEffectiveDate;
}
public void setContractEffectiveDate(LocalDate contractEffectiveDate) {
this.contractEffectiveDate = contractEffectiveDate;
}
public ResumeSubscriptionRequest creditMemoReasonCode(String creditMemoReasonCode) {
this.creditMemoReasonCode = creditMemoReasonCode;
return this;
}
/**
* A code identifying the reason for the credit memo transaction that is generated by the request. The value must be an existing reason code. If you do not pass the field or pass the field with empty value, Zuora uses the default reason code.
* @return creditMemoReasonCode
*/
@javax.annotation.Nullable
public String getCreditMemoReasonCode() {
return creditMemoReasonCode;
}
public void setCreditMemoReasonCode(String creditMemoReasonCode) {
this.creditMemoReasonCode = creditMemoReasonCode;
}
public ResumeSubscriptionRequest documentDate(LocalDate documentDate) {
this.documentDate = documentDate;
return this;
}
/**
* The date of the billing document, in `yyyy-mm-dd` format. It represents the invoice date for invoices, credit memo date for credit memos, and debit memo date for debit memos. - If this field is specified, the specified date is used as the billing document date. - If this field is not specified, the date specified in the `targetDate` is used as the billing document date.
* @return documentDate
*/
@javax.annotation.Nullable
public LocalDate getDocumentDate() {
return documentDate;
}
public void setDocumentDate(LocalDate documentDate) {
this.documentDate = documentDate;
}
public ResumeSubscriptionRequest extendsTerm(Boolean extendsTerm) {
this.extendsTerm = extendsTerm;
return this;
}
/**
* Whether to extend the subscription term by the length of time the suspension is in effect. Values: `true`, `false`.
* @return extendsTerm
*/
@javax.annotation.Nullable
public Boolean getExtendsTerm() {
return extendsTerm;
}
public void setExtendsTerm(Boolean extendsTerm) {
this.extendsTerm = extendsTerm;
}
@Deprecated
public ResumeSubscriptionRequest invoice(Boolean invoice) {
this.invoice = invoice;
return this;
}
/**
* **Note:** This field has been replaced by the `runBilling` field. The `invoice` field is only available for backward compatibility. Creates an invoice for a subscription. The invoice generated in this operation is only for this subscription, not for the entire customer account. If the value is `true`, an invoice is created. If the value is `false`, no action is taken. The default value is `false`. This field is in Zuora REST API version control. Supported minor versions are `196.0` and `207.0`. To use this field in the method, you must set the `zuora-version` parameter to the minor version number in the request header.
* @return invoice
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public Boolean getInvoice() {
return invoice;
}
@Deprecated
public void setInvoice(Boolean invoice) {
this.invoice = invoice;
}
@Deprecated
public ResumeSubscriptionRequest invoiceCollect(Boolean invoiceCollect) {
this.invoiceCollect = invoiceCollect;
return this;
}
/**
* **Note:** This field has been replaced by the `invoice` field and the `collect` field. `invoiceCollect` is available only for backward compatibility. If `true`, an invoice is generated and payment collected automatically during the subscription process. If `false`, no invoicing or payment takes place. The invoice generated in this operation is only for this subscription, not for the entire customer account. **Note**: This field is only available if you set the `zuora-version` request header to `186.0`, `187.0`, `188.0`, or `189.0`.
* @return invoiceCollect
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public Boolean getInvoiceCollect() {
return invoiceCollect;
}
@Deprecated
public void setInvoiceCollect(Boolean invoiceCollect) {
this.invoiceCollect = invoiceCollect;
}
@Deprecated
public ResumeSubscriptionRequest invoiceTargetDate(LocalDate invoiceTargetDate) {
this.invoiceTargetDate = invoiceTargetDate;
return this;
}
/**
* **Note:** This field has been replaced by the `targetDate` field. The `invoiceTargetDate` field is only available for backward compatibility. Date through which to calculate charges if an invoice is generated, as yyyy-mm-dd. Default is current date. This field is in Zuora REST API version control. Supported minor versions are `207.0` and earlier.
* @return invoiceTargetDate
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public LocalDate getInvoiceTargetDate() {
return invoiceTargetDate;
}
@Deprecated
public void setInvoiceTargetDate(LocalDate invoiceTargetDate) {
this.invoiceTargetDate = invoiceTargetDate;
}
public ResumeSubscriptionRequest orderDate(LocalDate orderDate) {
this.orderDate = orderDate;
return this;
}
/**
* The date when the order is signed. If no additinal contractEffectiveDate is provided, this order will use this order date as the contract effective date. This field must be in the `yyyy-mm-dd` format. This field is required for Orders customers only, not applicable to Orders Harmonization customers.
* @return orderDate
*/
@javax.annotation.Nullable
public LocalDate getOrderDate() {
return orderDate;
}
public void setOrderDate(LocalDate orderDate) {
this.orderDate = orderDate;
}
public ResumeSubscriptionRequest resumePeriods(String resumePeriods) {
this.resumePeriods = resumePeriods;
return this;
}
/**
* The length of the period used to specify when the subscription is resumed. The subscription resumption takes effect after a specified period based on the suspend date or today's date. You must use this field together with the `resumePeriodsType` field to specify the period. **Note:** This field is only applicable when the `suspendPolicy` field is set to `FixedPeriodsFromToday` or `FixedPeriodsFromSuspendDate`.
* @return resumePeriods
*/
@javax.annotation.Nullable
public String getResumePeriods() {
return resumePeriods;
}
public void setResumePeriods(String resumePeriods) {
this.resumePeriods = resumePeriods;
}
public ResumeSubscriptionRequest resumePeriodsType(String resumePeriodsType) {
this.resumePeriodsType = resumePeriodsType;
return this;
}
/**
* The period type used to define when the subscription resumption takes effect. The subscription resumption takes effect after a specified period based on the suspend date or today's date. You must use this field together with the `resumePeriods` field to specify the period. Values: `Day`, `Week`, `Month`, `Year` **Note:** This field is only applicable when the `suspendPolicy` field is set to `FixedPeriodsFromToday` or `FixedPeriodsFromSuspendDate`.
* @return resumePeriodsType
*/
@javax.annotation.Nullable
public String getResumePeriodsType() {
return resumePeriodsType;
}
public void setResumePeriodsType(String resumePeriodsType) {
this.resumePeriodsType = resumePeriodsType;
}
public ResumeSubscriptionRequest resumePolicy(String resumePolicy) {
this.resumePolicy = resumePolicy;
return this;
}
/**
* Resume methods. Specify a way to resume a subscription. Values: * `Today`: The subscription resumption takes effect on today's date. * `FixedPeriodsFromSuspendDate`: The subscription resumption takes effect after a specified period based on the suspend date. You must specify the `resumePeriods` and `resumePeriodsType` fields to define the period. * `SpecificDate`: The subscription resumption takes effect on a specific date. You must define the specific date in the `resumeSpecificDate` field. * `FixedPeriodsFromToday`: The subscription resumption takes effect after a specified period based on the today's date. You must specify the `resumePeriods` and `resumePeriodsType` fields to define the period. * `suspendDate`: The subscription resumption takes effect on the date of suspension of the subscription.
* @return resumePolicy
*/
@javax.annotation.Nonnull
public String getResumePolicy() {
return resumePolicy;
}
public void setResumePolicy(String resumePolicy) {
this.resumePolicy = resumePolicy;
}
public ResumeSubscriptionRequest resumeSpecificDate(LocalDate resumeSpecificDate) {
this.resumeSpecificDate = resumeSpecificDate;
return this;
}
/**
* A specific date when the subscription resumption takes effect, in the format yyyy-mm-dd. **Note:** This field is only applicable only when the `resumePolicy` field is set to `SpecificDate`. The value should not be earlier than the subscription suspension date.
* @return resumeSpecificDate
*/
@javax.annotation.Nullable
public LocalDate getResumeSpecificDate() {
return resumeSpecificDate;
}
public void setResumeSpecificDate(LocalDate resumeSpecificDate) {
this.resumeSpecificDate = resumeSpecificDate;
}
public ResumeSubscriptionRequest runBilling(Boolean runBilling) {
this.runBilling = runBilling;
return this;
}
/**
* Creates an invoice for a subscription. If you have the Invoice Settlement feature enabled, a credit memo might also be created based on the [invoice and credit memo generation rule](https://knowledgecenter.zuora.com/CB_Billing/Invoice_Settlement/Credit_and_Debit_Memos/Rules_for_Generating_Invoices_and_Credit_Memos). The billing documents generated in this operation is only for this subscription, not for the entire customer account. Possible values: - `true`: An invoice is created. If you have the Invoice Settlement feature enabled, a credit memo might also be created. - `false`: No invoice is created. **Note:** This field is in Zuora REST API version control. Supported minor versions are `211.0` or later. To use this field in the method, you must set the `zuora-version` parameter to the minor version number in the request header.
* @return runBilling
*/
@javax.annotation.Nullable
public Boolean getRunBilling() {
return runBilling;
}
public void setRunBilling(Boolean runBilling) {
this.runBilling = runBilling;
}
public ResumeSubscriptionRequest targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* Date through which to calculate charges if an invoice or a credit memo is generated, as yyyy-mm-dd. Default is current date. **Note:** The credit memo is only available if you have the Invoice Settlement feature enabled. This field is in Zuora REST API version control. Supported minor versions are `211.0` and later. To use this field in the method, you must set the `zuora-version` parameter to the minor version number in the request header.
* @return targetDate
*/
@javax.annotation.Nullable
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the ResumeSubscriptionRequest instance itself
*/
public ResumeSubscriptionRequest putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ResumeSubscriptionRequest resumeSubscriptionRequest = (ResumeSubscriptionRequest) o;
return Objects.equals(this.applicationOrder, resumeSubscriptionRequest.applicationOrder) &&
Objects.equals(this.applyCredit, resumeSubscriptionRequest.applyCredit) &&
Objects.equals(this.applyCreditBalance, resumeSubscriptionRequest.applyCreditBalance) &&
Objects.equals(this.bookingDate, resumeSubscriptionRequest.bookingDate) &&
Objects.equals(this.collect, resumeSubscriptionRequest.collect) &&
Objects.equals(this.contractEffectiveDate, resumeSubscriptionRequest.contractEffectiveDate) &&
Objects.equals(this.creditMemoReasonCode, resumeSubscriptionRequest.creditMemoReasonCode) &&
Objects.equals(this.documentDate, resumeSubscriptionRequest.documentDate) &&
Objects.equals(this.extendsTerm, resumeSubscriptionRequest.extendsTerm) &&
Objects.equals(this.invoice, resumeSubscriptionRequest.invoice) &&
Objects.equals(this.invoiceCollect, resumeSubscriptionRequest.invoiceCollect) &&
Objects.equals(this.invoiceTargetDate, resumeSubscriptionRequest.invoiceTargetDate) &&
Objects.equals(this.orderDate, resumeSubscriptionRequest.orderDate) &&
Objects.equals(this.resumePeriods, resumeSubscriptionRequest.resumePeriods) &&
Objects.equals(this.resumePeriodsType, resumeSubscriptionRequest.resumePeriodsType) &&
Objects.equals(this.resumePolicy, resumeSubscriptionRequest.resumePolicy) &&
Objects.equals(this.resumeSpecificDate, resumeSubscriptionRequest.resumeSpecificDate) &&
Objects.equals(this.runBilling, resumeSubscriptionRequest.runBilling) &&
Objects.equals(this.targetDate, resumeSubscriptionRequest.targetDate)&&
Objects.equals(this.additionalProperties, resumeSubscriptionRequest.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(applicationOrder, applyCredit, applyCreditBalance, bookingDate, collect, contractEffectiveDate, creditMemoReasonCode, documentDate, extendsTerm, invoice, invoiceCollect, invoiceTargetDate, orderDate, resumePeriods, resumePeriodsType, resumePolicy, resumeSpecificDate, runBilling, targetDate, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ResumeSubscriptionRequest {\n");
sb.append(" applicationOrder: ").append(toIndentedString(applicationOrder)).append("\n");
sb.append(" applyCredit: ").append(toIndentedString(applyCredit)).append("\n");
sb.append(" applyCreditBalance: ").append(toIndentedString(applyCreditBalance)).append("\n");
sb.append(" bookingDate: ").append(toIndentedString(bookingDate)).append("\n");
sb.append(" collect: ").append(toIndentedString(collect)).append("\n");
sb.append(" contractEffectiveDate: ").append(toIndentedString(contractEffectiveDate)).append("\n");
sb.append(" creditMemoReasonCode: ").append(toIndentedString(creditMemoReasonCode)).append("\n");
sb.append(" documentDate: ").append(toIndentedString(documentDate)).append("\n");
sb.append(" extendsTerm: ").append(toIndentedString(extendsTerm)).append("\n");
sb.append(" invoice: ").append(toIndentedString(invoice)).append("\n");
sb.append(" invoiceCollect: ").append(toIndentedString(invoiceCollect)).append("\n");
sb.append(" invoiceTargetDate: ").append(toIndentedString(invoiceTargetDate)).append("\n");
sb.append(" orderDate: ").append(toIndentedString(orderDate)).append("\n");
sb.append(" resumePeriods: ").append(toIndentedString(resumePeriods)).append("\n");
sb.append(" resumePeriodsType: ").append(toIndentedString(resumePeriodsType)).append("\n");
sb.append(" resumePolicy: ").append(toIndentedString(resumePolicy)).append("\n");
sb.append(" resumeSpecificDate: ").append(toIndentedString(resumeSpecificDate)).append("\n");
sb.append(" runBilling: ").append(toIndentedString(runBilling)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("applicationOrder");
openapiFields.add("applyCredit");
openapiFields.add("applyCreditBalance");
openapiFields.add("bookingDate");
openapiFields.add("collect");
openapiFields.add("contractEffectiveDate");
openapiFields.add("creditMemoReasonCode");
openapiFields.add("documentDate");
openapiFields.add("extendsTerm");
openapiFields.add("invoice");
openapiFields.add("invoiceCollect");
openapiFields.add("invoiceTargetDate");
openapiFields.add("orderDate");
openapiFields.add("resumePeriods");
openapiFields.add("resumePeriodsType");
openapiFields.add("resumePolicy");
openapiFields.add("resumeSpecificDate");
openapiFields.add("runBilling");
openapiFields.add("targetDate");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("resumePolicy");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to ResumeSubscriptionRequest
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!ResumeSubscriptionRequest.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in ResumeSubscriptionRequest is not found in the empty JSON string", ResumeSubscriptionRequest.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : ResumeSubscriptionRequest.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
// ensure the optional json data is an array if present
if (jsonObj.get("applicationOrder") != null && !jsonObj.get("applicationOrder").isJsonNull() && !jsonObj.get("applicationOrder").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `applicationOrder` to be an array in the JSON string but got `%s`", jsonObj.get("applicationOrder").toString()));
}
if ((jsonObj.get("creditMemoReasonCode") != null && !jsonObj.get("creditMemoReasonCode").isJsonNull()) && !jsonObj.get("creditMemoReasonCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creditMemoReasonCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creditMemoReasonCode").toString()));
}
if ((jsonObj.get("resumePeriods") != null && !jsonObj.get("resumePeriods").isJsonNull()) && !jsonObj.get("resumePeriods").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `resumePeriods` to be a primitive type in the JSON string but got `%s`", jsonObj.get("resumePeriods").toString()));
}
if ((jsonObj.get("resumePeriodsType") != null && !jsonObj.get("resumePeriodsType").isJsonNull()) && !jsonObj.get("resumePeriodsType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `resumePeriodsType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("resumePeriodsType").toString()));
}
if (!jsonObj.get("resumePolicy").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `resumePolicy` to be a primitive type in the JSON string but got `%s`", jsonObj.get("resumePolicy").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!ResumeSubscriptionRequest.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'ResumeSubscriptionRequest' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(ResumeSubscriptionRequest.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, ResumeSubscriptionRequest value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public ResumeSubscriptionRequest read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
ResumeSubscriptionRequest instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of ResumeSubscriptionRequest given an JSON string
*
* @param jsonString JSON string
* @return An instance of ResumeSubscriptionRequest
* @throws IOException if the JSON string is invalid with respect to ResumeSubscriptionRequest
*/
public static ResumeSubscriptionRequest fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, ResumeSubscriptionRequest.class);
}
/**
* Convert an instance of ResumeSubscriptionRequest to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy