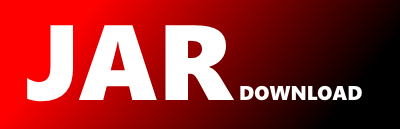
com.zuora.model.SignUpPaymentMethod Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.SignUpCreatePaymentMethodCardholderInfo;
import com.zuora.model.SignUpCreatePaymentMethodCreditCardMitProfileType;
import com.zuora.model.StoredCredentialProfileAction;
import com.zuora.model.StoredCredentialProfileConsentAgreementSrc;
import java.io.IOException;
import java.time.LocalDate;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* SignUpPaymentMethod
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class SignUpPaymentMethod {
public static final String SERIALIZED_NAME_SECOND_TOKEN_ID = "secondTokenId";
@SerializedName(SERIALIZED_NAME_SECOND_TOKEN_ID)
private String secondTokenId;
public static final String SERIALIZED_NAME_TOKEN_ID = "tokenId";
@SerializedName(SERIALIZED_NAME_TOKEN_ID)
private String tokenId;
public static final String SERIALIZED_NAME_B_A_I_D = "BAID";
@SerializedName(SERIALIZED_NAME_B_A_I_D)
private String BAID;
public static final String SERIALIZED_NAME_EMAIL = "email";
@SerializedName(SERIALIZED_NAME_EMAIL)
private String email;
public static final String SERIALIZED_NAME_PREAPPROVAL_KEY = "preapprovalKey";
@SerializedName(SERIALIZED_NAME_PREAPPROVAL_KEY)
private String preapprovalKey;
public static final String SERIALIZED_NAME_CARD_HOLDER_INFO = "cardHolderInfo";
@SerializedName(SERIALIZED_NAME_CARD_HOLDER_INFO)
private SignUpCreatePaymentMethodCardholderInfo cardHolderInfo;
public static final String SERIALIZED_NAME_CARD_NUMBER = "cardNumber";
@SerializedName(SERIALIZED_NAME_CARD_NUMBER)
private String cardNumber;
public static final String SERIALIZED_NAME_CARD_TYPE = "cardType";
@SerializedName(SERIALIZED_NAME_CARD_TYPE)
private String cardType;
public static final String SERIALIZED_NAME_CHECK_DUPLICATED = "checkDuplicated";
@SerializedName(SERIALIZED_NAME_CHECK_DUPLICATED)
private Boolean checkDuplicated;
public static final String SERIALIZED_NAME_EXPIRATION_MONTH = "expirationMonth";
@SerializedName(SERIALIZED_NAME_EXPIRATION_MONTH)
private String expirationMonth;
public static final String SERIALIZED_NAME_EXPIRATION_YEAR = "expirationYear";
@SerializedName(SERIALIZED_NAME_EXPIRATION_YEAR)
private String expirationYear;
public static final String SERIALIZED_NAME_MIT_CONSENT_AGREEMENT_REF = "mitConsentAgreementRef";
@SerializedName(SERIALIZED_NAME_MIT_CONSENT_AGREEMENT_REF)
private String mitConsentAgreementRef;
public static final String SERIALIZED_NAME_MIT_CONSENT_AGREEMENT_SRC = "mitConsentAgreementSrc";
@SerializedName(SERIALIZED_NAME_MIT_CONSENT_AGREEMENT_SRC)
private StoredCredentialProfileConsentAgreementSrc mitConsentAgreementSrc;
public static final String SERIALIZED_NAME_MIT_NETWORK_TRANSACTION_ID = "mitNetworkTransactionId";
@SerializedName(SERIALIZED_NAME_MIT_NETWORK_TRANSACTION_ID)
private String mitNetworkTransactionId;
public static final String SERIALIZED_NAME_MIT_PROFILE_ACTION = "mitProfileAction";
@SerializedName(SERIALIZED_NAME_MIT_PROFILE_ACTION)
private StoredCredentialProfileAction mitProfileAction;
public static final String SERIALIZED_NAME_MIT_PROFILE_AGREED_ON = "mitProfileAgreedOn";
@SerializedName(SERIALIZED_NAME_MIT_PROFILE_AGREED_ON)
private LocalDate mitProfileAgreedOn;
public static final String SERIALIZED_NAME_MIT_PROFILE_TYPE = "mitProfileType";
@SerializedName(SERIALIZED_NAME_MIT_PROFILE_TYPE)
private SignUpCreatePaymentMethodCreditCardMitProfileType mitProfileType;
public static final String SERIALIZED_NAME_SECURITY_CODE = "securityCode";
@SerializedName(SERIALIZED_NAME_SECURITY_CODE)
private String securityCode;
public static final String SERIALIZED_NAME_ACCOUNT_KEY = "accountKey";
@SerializedName(SERIALIZED_NAME_ACCOUNT_KEY)
private String accountKey;
public static final String SERIALIZED_NAME_AUTH_GATEWAY = "authGateway";
@SerializedName(SERIALIZED_NAME_AUTH_GATEWAY)
private String authGateway;
public static final String SERIALIZED_NAME_IP_ADDRESS = "ipAddress";
@SerializedName(SERIALIZED_NAME_IP_ADDRESS)
private String ipAddress;
public static final String SERIALIZED_NAME_MAKE_DEFAULT = "makeDefault";
@SerializedName(SERIALIZED_NAME_MAKE_DEFAULT)
private Boolean makeDefault = false;
/**
* Type of payment method. The following types of the payment method are supported:
*/
@JsonAdapter(TypeEnum.Adapter.class)
public enum TypeEnum {
PAYPALEC("PayPalEC"),
PAYPALNATIVEEC("PayPalNativeEC"),
PAYPALADAPTIVE("PayPalAdaptive"),
CREDITCARD("CreditCard"),
CREDITCARDREFERENCETRANSACTION("CreditCardReferenceTransaction");
private String value;
TypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TypeEnum.fromValue(value);
}
}
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
String value = jsonElement.getAsString();
TypeEnum.fromValue(value);
}
}
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private TypeEnum type;
public SignUpPaymentMethod() {
}
public SignUpPaymentMethod secondTokenId(String secondTokenId) {
this.secondTokenId = secondTokenId;
return this;
}
/**
* The second token id of CreditCardReferenceTransaction.
* @return secondTokenId
*/
@javax.annotation.Nullable
public String getSecondTokenId() {
return secondTokenId;
}
public void setSecondTokenId(String secondTokenId) {
this.secondTokenId = secondTokenId;
}
public SignUpPaymentMethod tokenId(String tokenId) {
this.tokenId = tokenId;
return this;
}
/**
* The token id of payment method, required field of CreditCardReferenceTransaction type.
* @return tokenId
*/
@javax.annotation.Nullable
public String getTokenId() {
return tokenId;
}
public void setTokenId(String tokenId) {
this.tokenId = tokenId;
}
public SignUpPaymentMethod BAID(String BAID) {
this.BAID = BAID;
return this;
}
/**
* ID of a PayPal billing agreement, for example, I-1TJ3GAGG82Y9.
* @return BAID
*/
@javax.annotation.Nullable
public String getBAID() {
return BAID;
}
public void setBAID(String BAID) {
this.BAID = BAID;
}
public SignUpPaymentMethod email(String email) {
this.email = email;
return this;
}
/**
* Email address associated with the payment method. This field is only supported for PayPal payment methods and is required if you want to create any of the following PayPal payment methods: - PayPal Express Checkout payment method - PayPal Adaptive payment method - PayPal Commerce Platform payment method
* @return email
*/
@javax.annotation.Nullable
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public SignUpPaymentMethod preapprovalKey(String preapprovalKey) {
this.preapprovalKey = preapprovalKey;
return this;
}
/**
* The PayPal preapproval key.
* @return preapprovalKey
*/
@javax.annotation.Nullable
public String getPreapprovalKey() {
return preapprovalKey;
}
public void setPreapprovalKey(String preapprovalKey) {
this.preapprovalKey = preapprovalKey;
}
public SignUpPaymentMethod cardHolderInfo(SignUpCreatePaymentMethodCardholderInfo cardHolderInfo) {
this.cardHolderInfo = cardHolderInfo;
return this;
}
/**
* Get cardHolderInfo
* @return cardHolderInfo
*/
@javax.annotation.Nullable
public SignUpCreatePaymentMethodCardholderInfo getCardHolderInfo() {
return cardHolderInfo;
}
public void setCardHolderInfo(SignUpCreatePaymentMethodCardholderInfo cardHolderInfo) {
this.cardHolderInfo = cardHolderInfo;
}
public SignUpPaymentMethod cardNumber(String cardNumber) {
this.cardNumber = cardNumber;
return this;
}
/**
* Credit card number.
* @return cardNumber
*/
@javax.annotation.Nullable
public String getCardNumber() {
return cardNumber;
}
public void setCardNumber(String cardNumber) {
this.cardNumber = cardNumber;
}
public SignUpPaymentMethod cardType(String cardType) {
this.cardType = cardType;
return this;
}
/**
* The type of the credit card. Possible values include `Visa`, `MasterCard`, `AmericanExpress`, `Discover`, `JCB`, and `Diners`. For more information about credit card types supported by different payment gateways, see [Supported Payment Gateways](https://knowledgecenter.zuora.com/CB_Billing/M_Payment_Gateways/Supported_Payment_Gateways).
* @return cardType
*/
@javax.annotation.Nullable
public String getCardType() {
return cardType;
}
public void setCardType(String cardType) {
this.cardType = cardType;
}
public SignUpPaymentMethod checkDuplicated(Boolean checkDuplicated) {
this.checkDuplicated = checkDuplicated;
return this;
}
/**
* Get checkDuplicated
* @return checkDuplicated
*/
@javax.annotation.Nullable
public Boolean getCheckDuplicated() {
return checkDuplicated;
}
public void setCheckDuplicated(Boolean checkDuplicated) {
this.checkDuplicated = checkDuplicated;
}
public SignUpPaymentMethod expirationMonth(String expirationMonth) {
this.expirationMonth = expirationMonth;
return this;
}
/**
* One or two digit expiration month (1-12) of the credit card.
* @return expirationMonth
*/
@javax.annotation.Nullable
public String getExpirationMonth() {
return expirationMonth;
}
public void setExpirationMonth(String expirationMonth) {
this.expirationMonth = expirationMonth;
}
public SignUpPaymentMethod expirationYear(String expirationYear) {
this.expirationYear = expirationYear;
return this;
}
/**
* Four-digit expiration year of the credit card.
* @return expirationYear
*/
@javax.annotation.Nullable
public String getExpirationYear() {
return expirationYear;
}
public void setExpirationYear(String expirationYear) {
this.expirationYear = expirationYear;
}
public SignUpPaymentMethod mitConsentAgreementRef(String mitConsentAgreementRef) {
this.mitConsentAgreementRef = mitConsentAgreementRef;
return this;
}
/**
* Specifies your reference for the stored credential consent agreement that you have established with the customer. Only applicable if you set the `mitProfileAction` field.
* @return mitConsentAgreementRef
*/
@javax.annotation.Nullable
public String getMitConsentAgreementRef() {
return mitConsentAgreementRef;
}
public void setMitConsentAgreementRef(String mitConsentAgreementRef) {
this.mitConsentAgreementRef = mitConsentAgreementRef;
}
public SignUpPaymentMethod mitConsentAgreementSrc(StoredCredentialProfileConsentAgreementSrc mitConsentAgreementSrc) {
this.mitConsentAgreementSrc = mitConsentAgreementSrc;
return this;
}
/**
* Get mitConsentAgreementSrc
* @return mitConsentAgreementSrc
*/
@javax.annotation.Nullable
public StoredCredentialProfileConsentAgreementSrc getMitConsentAgreementSrc() {
return mitConsentAgreementSrc;
}
public void setMitConsentAgreementSrc(StoredCredentialProfileConsentAgreementSrc mitConsentAgreementSrc) {
this.mitConsentAgreementSrc = mitConsentAgreementSrc;
}
public SignUpPaymentMethod mitNetworkTransactionId(String mitNetworkTransactionId) {
this.mitNetworkTransactionId = mitNetworkTransactionId;
return this;
}
/**
* Specifies the ID of a network transaction. Only applicable if you set the `mitProfileAction` field to `Persist`.
* @return mitNetworkTransactionId
*/
@javax.annotation.Nullable
public String getMitNetworkTransactionId() {
return mitNetworkTransactionId;
}
public void setMitNetworkTransactionId(String mitNetworkTransactionId) {
this.mitNetworkTransactionId = mitNetworkTransactionId;
}
public SignUpPaymentMethod mitProfileAction(StoredCredentialProfileAction mitProfileAction) {
this.mitProfileAction = mitProfileAction;
return this;
}
/**
* Get mitProfileAction
* @return mitProfileAction
*/
@javax.annotation.Nullable
public StoredCredentialProfileAction getMitProfileAction() {
return mitProfileAction;
}
public void setMitProfileAction(StoredCredentialProfileAction mitProfileAction) {
this.mitProfileAction = mitProfileAction;
}
public SignUpPaymentMethod mitProfileAgreedOn(LocalDate mitProfileAgreedOn) {
this.mitProfileAgreedOn = mitProfileAgreedOn;
return this;
}
/**
* The date on which the profile is agreed. The date format is `yyyy-mm-dd`.
* @return mitProfileAgreedOn
*/
@javax.annotation.Nullable
public LocalDate getMitProfileAgreedOn() {
return mitProfileAgreedOn;
}
public void setMitProfileAgreedOn(LocalDate mitProfileAgreedOn) {
this.mitProfileAgreedOn = mitProfileAgreedOn;
}
public SignUpPaymentMethod mitProfileType(SignUpCreatePaymentMethodCreditCardMitProfileType mitProfileType) {
this.mitProfileType = mitProfileType;
return this;
}
/**
* Get mitProfileType
* @return mitProfileType
*/
@javax.annotation.Nullable
public SignUpCreatePaymentMethodCreditCardMitProfileType getMitProfileType() {
return mitProfileType;
}
public void setMitProfileType(SignUpCreatePaymentMethodCreditCardMitProfileType mitProfileType) {
this.mitProfileType = mitProfileType;
}
public SignUpPaymentMethod securityCode(String securityCode) {
this.securityCode = securityCode;
return this;
}
/**
* CVV or CVV2 security code of the credit card. To ensure PCI compliance, this value is not stored and cannot be queried.
* @return securityCode
*/
@javax.annotation.Nullable
public String getSecurityCode() {
return securityCode;
}
public void setSecurityCode(String securityCode) {
this.securityCode = securityCode;
}
public SignUpPaymentMethod accountKey(String accountKey) {
this.accountKey = accountKey;
return this;
}
/**
* Internal ID of the customer account that will own the payment method.
* @return accountKey
*/
@javax.annotation.Nullable
public String getAccountKey() {
return accountKey;
}
public void setAccountKey(String accountKey) {
this.accountKey = accountKey;
}
public SignUpPaymentMethod authGateway(String authGateway) {
this.authGateway = authGateway;
return this;
}
/**
* Internal ID of the payment gateway that Zuora will use to authorize the payments that are made with the payment method. If you do not set this field, Zuora will use one of the following payment gateways instead: * The default payment gateway of the customer account that owns the payment method, if the `accountKey` field is set. * The default payment gateway of your Zuora tenant, if the `accountKey` field is not set.
* @return authGateway
*/
@javax.annotation.Nullable
public String getAuthGateway() {
return authGateway;
}
public void setAuthGateway(String authGateway) {
this.authGateway = authGateway;
}
public SignUpPaymentMethod ipAddress(String ipAddress) {
this.ipAddress = ipAddress;
return this;
}
/**
* The IPv4 or IPv6 information of the user when the payment method is created or updated. Some gateways use this field for fraud prevention. If this field is passed to Zuora, Zuora directly passes it to gateways. If the IP address length is beyond 45 characters, a validation error occurs.
* @return ipAddress
*/
@javax.annotation.Nullable
public String getIpAddress() {
return ipAddress;
}
public void setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
}
public SignUpPaymentMethod makeDefault(Boolean makeDefault) {
this.makeDefault = makeDefault;
return this;
}
/**
* Specifies whether the payment method will be the default payment method of the customer account that owns the payment method. Only applicable if the `accountKey` field is set.
* @return makeDefault
*/
@javax.annotation.Nullable
public Boolean getMakeDefault() {
return makeDefault;
}
public void setMakeDefault(Boolean makeDefault) {
this.makeDefault = makeDefault;
}
public SignUpPaymentMethod type(TypeEnum type) {
this.type = type;
return this;
}
/**
* Type of payment method. The following types of the payment method are supported:
* @return type
*/
@javax.annotation.Nonnull
public TypeEnum getType() {
return type;
}
public void setType(TypeEnum type) {
this.type = type;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the SignUpPaymentMethod instance itself
*/
public SignUpPaymentMethod putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SignUpPaymentMethod signUpPaymentMethod = (SignUpPaymentMethod) o;
return Objects.equals(this.secondTokenId, signUpPaymentMethod.secondTokenId) &&
Objects.equals(this.tokenId, signUpPaymentMethod.tokenId) &&
Objects.equals(this.BAID, signUpPaymentMethod.BAID) &&
Objects.equals(this.email, signUpPaymentMethod.email) &&
Objects.equals(this.preapprovalKey, signUpPaymentMethod.preapprovalKey) &&
Objects.equals(this.cardHolderInfo, signUpPaymentMethod.cardHolderInfo) &&
Objects.equals(this.cardNumber, signUpPaymentMethod.cardNumber) &&
Objects.equals(this.cardType, signUpPaymentMethod.cardType) &&
Objects.equals(this.checkDuplicated, signUpPaymentMethod.checkDuplicated) &&
Objects.equals(this.expirationMonth, signUpPaymentMethod.expirationMonth) &&
Objects.equals(this.expirationYear, signUpPaymentMethod.expirationYear) &&
Objects.equals(this.mitConsentAgreementRef, signUpPaymentMethod.mitConsentAgreementRef) &&
Objects.equals(this.mitConsentAgreementSrc, signUpPaymentMethod.mitConsentAgreementSrc) &&
Objects.equals(this.mitNetworkTransactionId, signUpPaymentMethod.mitNetworkTransactionId) &&
Objects.equals(this.mitProfileAction, signUpPaymentMethod.mitProfileAction) &&
Objects.equals(this.mitProfileAgreedOn, signUpPaymentMethod.mitProfileAgreedOn) &&
Objects.equals(this.mitProfileType, signUpPaymentMethod.mitProfileType) &&
Objects.equals(this.securityCode, signUpPaymentMethod.securityCode) &&
Objects.equals(this.accountKey, signUpPaymentMethod.accountKey) &&
Objects.equals(this.authGateway, signUpPaymentMethod.authGateway) &&
Objects.equals(this.ipAddress, signUpPaymentMethod.ipAddress) &&
Objects.equals(this.makeDefault, signUpPaymentMethod.makeDefault) &&
Objects.equals(this.type, signUpPaymentMethod.type)&&
Objects.equals(this.additionalProperties, signUpPaymentMethod.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(secondTokenId, tokenId, BAID, email, preapprovalKey, cardHolderInfo, cardNumber, cardType, checkDuplicated, expirationMonth, expirationYear, mitConsentAgreementRef, mitConsentAgreementSrc, mitNetworkTransactionId, mitProfileAction, mitProfileAgreedOn, mitProfileType, securityCode, accountKey, authGateway, ipAddress, makeDefault, type, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SignUpPaymentMethod {\n");
sb.append(" secondTokenId: ").append(toIndentedString(secondTokenId)).append("\n");
sb.append(" tokenId: ").append(toIndentedString(tokenId)).append("\n");
sb.append(" BAID: ").append(toIndentedString(BAID)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" preapprovalKey: ").append(toIndentedString(preapprovalKey)).append("\n");
sb.append(" cardHolderInfo: ").append(toIndentedString(cardHolderInfo)).append("\n");
sb.append(" cardNumber: ").append(toIndentedString(cardNumber)).append("\n");
sb.append(" cardType: ").append(toIndentedString(cardType)).append("\n");
sb.append(" checkDuplicated: ").append(toIndentedString(checkDuplicated)).append("\n");
sb.append(" expirationMonth: ").append(toIndentedString(expirationMonth)).append("\n");
sb.append(" expirationYear: ").append(toIndentedString(expirationYear)).append("\n");
sb.append(" mitConsentAgreementRef: ").append(toIndentedString(mitConsentAgreementRef)).append("\n");
sb.append(" mitConsentAgreementSrc: ").append(toIndentedString(mitConsentAgreementSrc)).append("\n");
sb.append(" mitNetworkTransactionId: ").append(toIndentedString(mitNetworkTransactionId)).append("\n");
sb.append(" mitProfileAction: ").append(toIndentedString(mitProfileAction)).append("\n");
sb.append(" mitProfileAgreedOn: ").append(toIndentedString(mitProfileAgreedOn)).append("\n");
sb.append(" mitProfileType: ").append(toIndentedString(mitProfileType)).append("\n");
sb.append(" securityCode: ").append(toIndentedString(securityCode)).append("\n");
sb.append(" accountKey: ").append(toIndentedString(accountKey)).append("\n");
sb.append(" authGateway: ").append(toIndentedString(authGateway)).append("\n");
sb.append(" ipAddress: ").append(toIndentedString(ipAddress)).append("\n");
sb.append(" makeDefault: ").append(toIndentedString(makeDefault)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("secondTokenId");
openapiFields.add("tokenId");
openapiFields.add("BAID");
openapiFields.add("email");
openapiFields.add("preapprovalKey");
openapiFields.add("cardHolderInfo");
openapiFields.add("cardNumber");
openapiFields.add("cardType");
openapiFields.add("checkDuplicated");
openapiFields.add("expirationMonth");
openapiFields.add("expirationYear");
openapiFields.add("mitConsentAgreementRef");
openapiFields.add("mitConsentAgreementSrc");
openapiFields.add("mitNetworkTransactionId");
openapiFields.add("mitProfileAction");
openapiFields.add("mitProfileAgreedOn");
openapiFields.add("mitProfileType");
openapiFields.add("securityCode");
openapiFields.add("accountKey");
openapiFields.add("authGateway");
openapiFields.add("ipAddress");
openapiFields.add("makeDefault");
openapiFields.add("type");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("type");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to SignUpPaymentMethod
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!SignUpPaymentMethod.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in SignUpPaymentMethod is not found in the empty JSON string", SignUpPaymentMethod.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : SignUpPaymentMethod.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("secondTokenId") != null && !jsonObj.get("secondTokenId").isJsonNull()) && !jsonObj.get("secondTokenId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `secondTokenId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("secondTokenId").toString()));
}
if ((jsonObj.get("tokenId") != null && !jsonObj.get("tokenId").isJsonNull()) && !jsonObj.get("tokenId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `tokenId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("tokenId").toString()));
}
if ((jsonObj.get("BAID") != null && !jsonObj.get("BAID").isJsonNull()) && !jsonObj.get("BAID").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `BAID` to be a primitive type in the JSON string but got `%s`", jsonObj.get("BAID").toString()));
}
if ((jsonObj.get("email") != null && !jsonObj.get("email").isJsonNull()) && !jsonObj.get("email").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `email` to be a primitive type in the JSON string but got `%s`", jsonObj.get("email").toString()));
}
if ((jsonObj.get("preapprovalKey") != null && !jsonObj.get("preapprovalKey").isJsonNull()) && !jsonObj.get("preapprovalKey").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `preapprovalKey` to be a primitive type in the JSON string but got `%s`", jsonObj.get("preapprovalKey").toString()));
}
// validate the optional field `cardHolderInfo`
if (jsonObj.get("cardHolderInfo") != null && !jsonObj.get("cardHolderInfo").isJsonNull()) {
SignUpCreatePaymentMethodCardholderInfo.validateJsonElement(jsonObj.get("cardHolderInfo"));
}
if ((jsonObj.get("cardNumber") != null && !jsonObj.get("cardNumber").isJsonNull()) && !jsonObj.get("cardNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cardNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cardNumber").toString()));
}
if ((jsonObj.get("cardType") != null && !jsonObj.get("cardType").isJsonNull()) && !jsonObj.get("cardType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cardType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cardType").toString()));
}
if ((jsonObj.get("expirationMonth") != null && !jsonObj.get("expirationMonth").isJsonNull()) && !jsonObj.get("expirationMonth").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `expirationMonth` to be a primitive type in the JSON string but got `%s`", jsonObj.get("expirationMonth").toString()));
}
if ((jsonObj.get("expirationYear") != null && !jsonObj.get("expirationYear").isJsonNull()) && !jsonObj.get("expirationYear").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `expirationYear` to be a primitive type in the JSON string but got `%s`", jsonObj.get("expirationYear").toString()));
}
if ((jsonObj.get("mitConsentAgreementRef") != null && !jsonObj.get("mitConsentAgreementRef").isJsonNull()) && !jsonObj.get("mitConsentAgreementRef").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mitConsentAgreementRef` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mitConsentAgreementRef").toString()));
}
if ((jsonObj.get("mitConsentAgreementSrc") != null && !jsonObj.get("mitConsentAgreementSrc").isJsonNull()) && !jsonObj.get("mitConsentAgreementSrc").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mitConsentAgreementSrc` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mitConsentAgreementSrc").toString()));
}
// validate the optional field `mitConsentAgreementSrc`
if (jsonObj.get("mitConsentAgreementSrc") != null && !jsonObj.get("mitConsentAgreementSrc").isJsonNull()) {
StoredCredentialProfileConsentAgreementSrc.validateJsonElement(jsonObj.get("mitConsentAgreementSrc"));
}
if ((jsonObj.get("mitNetworkTransactionId") != null && !jsonObj.get("mitNetworkTransactionId").isJsonNull()) && !jsonObj.get("mitNetworkTransactionId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mitNetworkTransactionId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mitNetworkTransactionId").toString()));
}
if ((jsonObj.get("mitProfileAction") != null && !jsonObj.get("mitProfileAction").isJsonNull()) && !jsonObj.get("mitProfileAction").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mitProfileAction` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mitProfileAction").toString()));
}
// validate the optional field `mitProfileAction`
if (jsonObj.get("mitProfileAction") != null && !jsonObj.get("mitProfileAction").isJsonNull()) {
StoredCredentialProfileAction.validateJsonElement(jsonObj.get("mitProfileAction"));
}
if ((jsonObj.get("mitProfileType") != null && !jsonObj.get("mitProfileType").isJsonNull()) && !jsonObj.get("mitProfileType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mitProfileType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mitProfileType").toString()));
}
// validate the optional field `mitProfileType`
if (jsonObj.get("mitProfileType") != null && !jsonObj.get("mitProfileType").isJsonNull()) {
SignUpCreatePaymentMethodCreditCardMitProfileType.validateJsonElement(jsonObj.get("mitProfileType"));
}
if ((jsonObj.get("securityCode") != null && !jsonObj.get("securityCode").isJsonNull()) && !jsonObj.get("securityCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `securityCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("securityCode").toString()));
}
if ((jsonObj.get("accountKey") != null && !jsonObj.get("accountKey").isJsonNull()) && !jsonObj.get("accountKey").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountKey` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountKey").toString()));
}
if ((jsonObj.get("authGateway") != null && !jsonObj.get("authGateway").isJsonNull()) && !jsonObj.get("authGateway").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `authGateway` to be a primitive type in the JSON string but got `%s`", jsonObj.get("authGateway").toString()));
}
if ((jsonObj.get("ipAddress") != null && !jsonObj.get("ipAddress").isJsonNull()) && !jsonObj.get("ipAddress").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ipAddress` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ipAddress").toString()));
}
if (!jsonObj.get("type").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `type` to be a primitive type in the JSON string but got `%s`", jsonObj.get("type").toString()));
}
// validate the required field `type`
TypeEnum.validateJsonElement(jsonObj.get("type"));
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!SignUpPaymentMethod.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'SignUpPaymentMethod' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(SignUpPaymentMethod.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, SignUpPaymentMethod value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public SignUpPaymentMethod read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
SignUpPaymentMethod instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of SignUpPaymentMethod given an JSON string
*
* @param jsonString JSON string
* @return An instance of SignUpPaymentMethod
* @throws IOException if the JSON string is invalid with respect to SignUpPaymentMethod
*/
public static SignUpPaymentMethod fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, SignUpPaymentMethod.class);
}
/**
* Convert an instance of SignUpPaymentMethod to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy