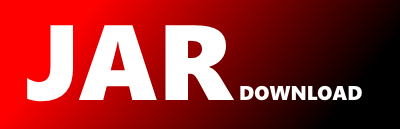
com.zuora.model.UpdateAccountRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.AccountEInvoiceProfile;
import com.zuora.model.AccountObjectNSFieldsCustomerTypeNS;
import com.zuora.model.AccountObjectNSFieldsSynctoNetSuiteNS;
import com.zuora.model.TaxInfo;
import com.zuora.model.UpdateAccountContact;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* UpdateAccountRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class UpdateAccountRequest {
public static final String SERIALIZED_NAME_CLASS_N_S = "Class__NS";
@SerializedName(SERIALIZED_NAME_CLASS_N_S)
private String classNS;
public static final String SERIALIZED_NAME_CUSTOMER_TYPE_N_S = "CustomerType__NS";
@SerializedName(SERIALIZED_NAME_CUSTOMER_TYPE_N_S)
private AccountObjectNSFieldsCustomerTypeNS customerTypeNS;
public static final String SERIALIZED_NAME_DEPARTMENT_N_S = "Department__NS";
@SerializedName(SERIALIZED_NAME_DEPARTMENT_N_S)
private String departmentNS;
public static final String SERIALIZED_NAME_INTEGRATION_ID_N_S = "IntegrationId__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_ID_N_S)
private String integrationIdNS;
public static final String SERIALIZED_NAME_INTEGRATION_STATUS_N_S = "IntegrationStatus__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_STATUS_N_S)
private String integrationStatusNS;
public static final String SERIALIZED_NAME_LOCATION_N_S = "Location__NS";
@SerializedName(SERIALIZED_NAME_LOCATION_N_S)
private String locationNS;
public static final String SERIALIZED_NAME_SUBSIDIARY_N_S = "Subsidiary__NS";
@SerializedName(SERIALIZED_NAME_SUBSIDIARY_N_S)
private String subsidiaryNS;
public static final String SERIALIZED_NAME_SYNC_DATE_N_S = "SyncDate__NS";
@SerializedName(SERIALIZED_NAME_SYNC_DATE_N_S)
private String syncDateNS;
public static final String SERIALIZED_NAME_SYNCTO_NET_SUITE_N_S = "SynctoNetSuite__NS";
@SerializedName(SERIALIZED_NAME_SYNCTO_NET_SUITE_N_S)
private AccountObjectNSFieldsSynctoNetSuiteNS synctoNetSuiteNS;
public static final String SERIALIZED_NAME_ADDITIONAL_EMAIL_ADDRESSES = "additionalEmailAddresses";
@SerializedName(SERIALIZED_NAME_ADDITIONAL_EMAIL_ADDRESSES)
private List additionalEmailAddresses;
public static final String SERIALIZED_NAME_AUTO_PAY = "autoPay";
@SerializedName(SERIALIZED_NAME_AUTO_PAY)
private Boolean autoPay;
public static final String SERIALIZED_NAME_BATCH = "batch";
@SerializedName(SERIALIZED_NAME_BATCH)
private String batch;
public static final String SERIALIZED_NAME_BILL_CYCLE_DAY = "billCycleDay";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_DAY)
private Integer billCycleDay;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT_ID = "billToContactId";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT_ID)
private String billToContactId;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT = "billToContact";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT)
private UpdateAccountContact billToContact;
public static final String SERIALIZED_NAME_SHIP_TO_CONTACT_ID = "shipToContactId";
@SerializedName(SERIALIZED_NAME_SHIP_TO_CONTACT_ID)
private String shipToContactId;
public static final String SERIALIZED_NAME_SHIP_TO_CONTACT = "shipToContact";
@SerializedName(SERIALIZED_NAME_SHIP_TO_CONTACT)
private UpdateAccountContact shipToContact;
public static final String SERIALIZED_NAME_COMMUNICATION_PROFILE_ID = "communicationProfileId";
@SerializedName(SERIALIZED_NAME_COMMUNICATION_PROFILE_ID)
private String communicationProfileId;
public static final String SERIALIZED_NAME_CREDIT_MEMO_TEMPLATE_ID = "creditMemoTemplateId";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_TEMPLATE_ID)
private String creditMemoTemplateId;
public static final String SERIALIZED_NAME_CRM_ID = "crmId";
@SerializedName(SERIALIZED_NAME_CRM_ID)
private String crmId;
public static final String SERIALIZED_NAME_CUSTOMER_SERVICE_REP_NAME = "customerServiceRepName";
@SerializedName(SERIALIZED_NAME_CUSTOMER_SERVICE_REP_NAME)
private String customerServiceRepName;
public static final String SERIALIZED_NAME_DEBIT_MEMO_TEMPLATE_ID = "debitMemoTemplateId";
@SerializedName(SERIALIZED_NAME_DEBIT_MEMO_TEMPLATE_ID)
private String debitMemoTemplateId;
public static final String SERIALIZED_NAME_DEFAULT_PAYMENT_METHOD_ID = "defaultPaymentMethodId";
@SerializedName(SERIALIZED_NAME_DEFAULT_PAYMENT_METHOD_ID)
private String defaultPaymentMethodId;
public static final String SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_EMAIL = "invoiceDeliveryPrefsEmail";
@SerializedName(SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_EMAIL)
private Boolean invoiceDeliveryPrefsEmail;
public static final String SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_PRINT = "invoiceDeliveryPrefsPrint";
@SerializedName(SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_PRINT)
private Boolean invoiceDeliveryPrefsPrint;
public static final String SERIALIZED_NAME_INVOICE_TEMPLATE_ID = "invoiceTemplateId";
@SerializedName(SERIALIZED_NAME_INVOICE_TEMPLATE_ID)
private String invoiceTemplateId;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_NOTES = "notes";
@SerializedName(SERIALIZED_NAME_NOTES)
private String notes;
public static final String SERIALIZED_NAME_PARENT_ID = "parentId";
@SerializedName(SERIALIZED_NAME_PARENT_ID)
private String parentId;
public static final String SERIALIZED_NAME_PARTNER_ACCOUNT = "partnerAccount";
@SerializedName(SERIALIZED_NAME_PARTNER_ACCOUNT)
private Boolean partnerAccount;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY = "paymentGateway";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY)
private String paymentGateway;
public static final String SERIALIZED_NAME_PAYMENT_TERM = "paymentTerm";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERM)
private String paymentTerm;
public static final String SERIALIZED_NAME_PROFILE_NUMBER = "profileNumber";
@SerializedName(SERIALIZED_NAME_PROFILE_NUMBER)
private String profileNumber;
public static final String SERIALIZED_NAME_PURCHASE_ORDER_NUMBER = "purchaseOrderNumber";
@SerializedName(SERIALIZED_NAME_PURCHASE_ORDER_NUMBER)
private String purchaseOrderNumber;
public static final String SERIALIZED_NAME_ROLL_UP_USAGE = "rollUpUsage";
@SerializedName(SERIALIZED_NAME_ROLL_UP_USAGE)
private Boolean rollUpUsage;
public static final String SERIALIZED_NAME_SALES_REP = "salesRep";
@SerializedName(SERIALIZED_NAME_SALES_REP)
private String salesRep;
public static final String SERIALIZED_NAME_SEQUENCE_SET_ID = "sequenceSetId";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_ID)
private String sequenceSetId;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT_ID = "soldToContactId";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT_ID)
private String soldToContactId;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT = "soldToContact";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT)
private UpdateAccountContact soldToContact;
public static final String SERIALIZED_NAME_TAGGING = "tagging";
@SerializedName(SERIALIZED_NAME_TAGGING)
private String tagging;
public static final String SERIALIZED_NAME_TAX_INFO = "taxInfo";
@SerializedName(SERIALIZED_NAME_TAX_INFO)
private TaxInfo taxInfo;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY_NUMBER = "paymentGatewayNumber";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY_NUMBER)
private String paymentGatewayNumber;
public static final String SERIALIZED_NAME_SUMMARY_STATEMENT_TEMPLATE_ID = "summaryStatementTemplateId";
@SerializedName(SERIALIZED_NAME_SUMMARY_STATEMENT_TEMPLATE_ID)
private String summaryStatementTemplateId;
public static final String SERIALIZED_NAME_EINVOICE_PROFILE = "einvoiceProfile";
@SerializedName(SERIALIZED_NAME_EINVOICE_PROFILE)
private AccountEInvoiceProfile einvoiceProfile;
public static final String SERIALIZED_NAME_ORGANIZATION_LABEL = "organizationLabel";
@SerializedName(SERIALIZED_NAME_ORGANIZATION_LABEL)
private String organizationLabel;
public UpdateAccountRequest() {
}
public UpdateAccountRequest classNS(String classNS) {
this.classNS = classNS;
return this;
}
/**
* Value of the Class field for the corresponding customer account in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return classNS
*/
@javax.annotation.Nullable
public String getClassNS() {
return classNS;
}
public void setClassNS(String classNS) {
this.classNS = classNS;
}
public UpdateAccountRequest customerTypeNS(AccountObjectNSFieldsCustomerTypeNS customerTypeNS) {
this.customerTypeNS = customerTypeNS;
return this;
}
/**
* Get customerTypeNS
* @return customerTypeNS
*/
@javax.annotation.Nullable
public AccountObjectNSFieldsCustomerTypeNS getCustomerTypeNS() {
return customerTypeNS;
}
public void setCustomerTypeNS(AccountObjectNSFieldsCustomerTypeNS customerTypeNS) {
this.customerTypeNS = customerTypeNS;
}
public UpdateAccountRequest departmentNS(String departmentNS) {
this.departmentNS = departmentNS;
return this;
}
/**
* Value of the Department field for the corresponding customer account in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return departmentNS
*/
@javax.annotation.Nullable
public String getDepartmentNS() {
return departmentNS;
}
public void setDepartmentNS(String departmentNS) {
this.departmentNS = departmentNS;
}
public UpdateAccountRequest integrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
return this;
}
/**
* ID of the corresponding object in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationIdNS
*/
@javax.annotation.Nullable
public String getIntegrationIdNS() {
return integrationIdNS;
}
public void setIntegrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
}
public UpdateAccountRequest integrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
return this;
}
/**
* Status of the account's synchronization with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationStatusNS
*/
@javax.annotation.Nullable
public String getIntegrationStatusNS() {
return integrationStatusNS;
}
public void setIntegrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
}
public UpdateAccountRequest locationNS(String locationNS) {
this.locationNS = locationNS;
return this;
}
/**
* Value of the Location field for the corresponding customer account in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return locationNS
*/
@javax.annotation.Nullable
public String getLocationNS() {
return locationNS;
}
public void setLocationNS(String locationNS) {
this.locationNS = locationNS;
}
public UpdateAccountRequest subsidiaryNS(String subsidiaryNS) {
this.subsidiaryNS = subsidiaryNS;
return this;
}
/**
* Value of the Subsidiary field for the corresponding customer account in NetSuite. The Subsidiary field is required if you use NetSuite OneWorld. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return subsidiaryNS
*/
@javax.annotation.Nullable
public String getSubsidiaryNS() {
return subsidiaryNS;
}
public void setSubsidiaryNS(String subsidiaryNS) {
this.subsidiaryNS = subsidiaryNS;
}
public UpdateAccountRequest syncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
return this;
}
/**
* Date when the account was sychronized with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return syncDateNS
*/
@javax.annotation.Nullable
public String getSyncDateNS() {
return syncDateNS;
}
public void setSyncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
}
public UpdateAccountRequest synctoNetSuiteNS(AccountObjectNSFieldsSynctoNetSuiteNS synctoNetSuiteNS) {
this.synctoNetSuiteNS = synctoNetSuiteNS;
return this;
}
/**
* Get synctoNetSuiteNS
* @return synctoNetSuiteNS
*/
@javax.annotation.Nullable
public AccountObjectNSFieldsSynctoNetSuiteNS getSynctoNetSuiteNS() {
return synctoNetSuiteNS;
}
public void setSynctoNetSuiteNS(AccountObjectNSFieldsSynctoNetSuiteNS synctoNetSuiteNS) {
this.synctoNetSuiteNS = synctoNetSuiteNS;
}
public UpdateAccountRequest additionalEmailAddresses(List additionalEmailAddresses) {
this.additionalEmailAddresses = additionalEmailAddresses;
return this;
}
public UpdateAccountRequest addAdditionalEmailAddressesItem(String additionalEmailAddressesItem) {
if (this.additionalEmailAddresses == null) {
this.additionalEmailAddresses = new ArrayList<>();
}
this.additionalEmailAddresses.add(additionalEmailAddressesItem);
return this;
}
/**
* A list of additional email addresses to receive email notifications. Use commas to separate email addresses.
* @return additionalEmailAddresses
*/
@javax.annotation.Nullable
public List getAdditionalEmailAddresses() {
return additionalEmailAddresses;
}
public void setAdditionalEmailAddresses(List additionalEmailAddresses) {
this.additionalEmailAddresses = additionalEmailAddresses;
}
public UpdateAccountRequest autoPay(Boolean autoPay) {
this.autoPay = autoPay;
return this;
}
/**
* Whether future payments are to be automatically billed when they are due.
* @return autoPay
*/
@javax.annotation.Nullable
public Boolean getAutoPay() {
return autoPay;
}
public void setAutoPay(Boolean autoPay) {
this.autoPay = autoPay;
}
public UpdateAccountRequest batch(String batch) {
this.batch = batch;
return this;
}
/**
* The alias name given to a batch. A string of 50 characters or less.
* @return batch
*/
@javax.annotation.Nullable
public String getBatch() {
return batch;
}
public void setBatch(String batch) {
this.batch = batch;
}
public UpdateAccountRequest billCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
return this;
}
/**
* Sets the bill cycle day (BCD) for the charge. The BCD determines which day of the month the customer is billed. Values: Any activated system-defined bill cycle day (`1`-`31`)
* @return billCycleDay
*/
@javax.annotation.Nullable
public Integer getBillCycleDay() {
return billCycleDay;
}
public void setBillCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
}
public UpdateAccountRequest billToContactId(String billToContactId) {
this.billToContactId = billToContactId;
return this;
}
/**
* The ID of the contact that receives the bill. The contact must be associated with the account.
* @return billToContactId
*/
@javax.annotation.Nullable
public String getBillToContactId() {
return billToContactId;
}
public void setBillToContactId(String billToContactId) {
this.billToContactId = billToContactId;
}
public UpdateAccountRequest billToContact(UpdateAccountContact billToContact) {
this.billToContact = billToContact;
return this;
}
/**
* Get billToContact
* @return billToContact
*/
@javax.annotation.Nullable
public UpdateAccountContact getBillToContact() {
return billToContact;
}
public void setBillToContact(UpdateAccountContact billToContact) {
this.billToContact = billToContact;
}
public UpdateAccountRequest shipToContactId(String shipToContactId) {
this.shipToContactId = shipToContactId;
return this;
}
/**
* The ID of the contact that receives the goods or services. The contact must be associated with the account.
* @return shipToContactId
*/
@javax.annotation.Nullable
public String getShipToContactId() {
return shipToContactId;
}
public void setShipToContactId(String shipToContactId) {
this.shipToContactId = shipToContactId;
}
public UpdateAccountRequest shipToContact(UpdateAccountContact shipToContact) {
this.shipToContact = shipToContact;
return this;
}
/**
* Get shipToContact
* @return shipToContact
*/
@javax.annotation.Nullable
public UpdateAccountContact getShipToContact() {
return shipToContact;
}
public void setShipToContact(UpdateAccountContact shipToContact) {
this.shipToContact = shipToContact;
}
public UpdateAccountRequest communicationProfileId(String communicationProfileId) {
this.communicationProfileId = communicationProfileId;
return this;
}
/**
* The ID of the communication profile that this account is linked to. You can provide either or both of the `communicationProfileId` and `profileNumber` fields. If both are provided, the request will fail if they do not refer to the same communication profile.
* @return communicationProfileId
*/
@javax.annotation.Nullable
public String getCommunicationProfileId() {
return communicationProfileId;
}
public void setCommunicationProfileId(String communicationProfileId) {
this.communicationProfileId = communicationProfileId;
}
public UpdateAccountRequest creditMemoTemplateId(String creditMemoTemplateId) {
this.creditMemoTemplateId = creditMemoTemplateId;
return this;
}
/**
* **Note:** This field is only available if you have [Invoice Settlement](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement) enabled. The Invoice Settlement feature is generally available as of Zuora Billing Release 296 (March 2021). This feature includes Unapplied Payments, Credit and Debit Memo, and Invoice Item Settlement. If you want to enable Invoice Settlement, see [Invoice Settlement Enablement and Checklist Guide](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement/Invoice_Settlement_Migration_Checklist_and_Guide) for more information. The unique ID of the credit memo template, configured in **Billing Settings** > **Manage Billing Document Configuration** through the Zuora UI. For example, 2c92c08a6246fdf101626b1b3fe0144b.
* @return creditMemoTemplateId
*/
@javax.annotation.Nullable
public String getCreditMemoTemplateId() {
return creditMemoTemplateId;
}
public void setCreditMemoTemplateId(String creditMemoTemplateId) {
this.creditMemoTemplateId = creditMemoTemplateId;
}
public UpdateAccountRequest crmId(String crmId) {
this.crmId = crmId;
return this;
}
/**
* CRM account ID for the account, up to 100 characters.
* @return crmId
*/
@javax.annotation.Nullable
public String getCrmId() {
return crmId;
}
public void setCrmId(String crmId) {
this.crmId = crmId;
}
public UpdateAccountRequest customerServiceRepName(String customerServiceRepName) {
this.customerServiceRepName = customerServiceRepName;
return this;
}
/**
* Name of the account’s customer service representative, if applicable.
* @return customerServiceRepName
*/
@javax.annotation.Nullable
public String getCustomerServiceRepName() {
return customerServiceRepName;
}
public void setCustomerServiceRepName(String customerServiceRepName) {
this.customerServiceRepName = customerServiceRepName;
}
public UpdateAccountRequest debitMemoTemplateId(String debitMemoTemplateId) {
this.debitMemoTemplateId = debitMemoTemplateId;
return this;
}
/**
* **Note:** This field is only available if you have [Invoice Settlement](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement) enabled. The Invoice Settlement feature is generally available as of Zuora Billing Release 296 (March 2021). This feature includes Unapplied Payments, Credit and Debit Memo, and Invoice Item Settlement. If you want to enable Invoice Settlement, see [Invoice Settlement Enablement and Checklist Guide](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement/Invoice_Settlement_Migration_Checklist_and_Guide) for more information. The unique ID of the debit memo template, configured in **Billing Settings** > **Manage Billing Document Configuration** through the Zuora UI. For example, 2c92c08d62470a8501626b19d24f19e2.
* @return debitMemoTemplateId
*/
@javax.annotation.Nullable
public String getDebitMemoTemplateId() {
return debitMemoTemplateId;
}
public void setDebitMemoTemplateId(String debitMemoTemplateId) {
this.debitMemoTemplateId = debitMemoTemplateId;
}
public UpdateAccountRequest defaultPaymentMethodId(String defaultPaymentMethodId) {
this.defaultPaymentMethodId = defaultPaymentMethodId;
return this;
}
/**
* ID of the default payment method for the account. Values: a valid ID for an existing payment method.
* @return defaultPaymentMethodId
*/
@javax.annotation.Nullable
public String getDefaultPaymentMethodId() {
return defaultPaymentMethodId;
}
public void setDefaultPaymentMethodId(String defaultPaymentMethodId) {
this.defaultPaymentMethodId = defaultPaymentMethodId;
}
public UpdateAccountRequest invoiceDeliveryPrefsEmail(Boolean invoiceDeliveryPrefsEmail) {
this.invoiceDeliveryPrefsEmail = invoiceDeliveryPrefsEmail;
return this;
}
/**
* Whether the customer wants to receive invoices through email. The default value is `false`.
* @return invoiceDeliveryPrefsEmail
*/
@javax.annotation.Nullable
public Boolean getInvoiceDeliveryPrefsEmail() {
return invoiceDeliveryPrefsEmail;
}
public void setInvoiceDeliveryPrefsEmail(Boolean invoiceDeliveryPrefsEmail) {
this.invoiceDeliveryPrefsEmail = invoiceDeliveryPrefsEmail;
}
public UpdateAccountRequest invoiceDeliveryPrefsPrint(Boolean invoiceDeliveryPrefsPrint) {
this.invoiceDeliveryPrefsPrint = invoiceDeliveryPrefsPrint;
return this;
}
/**
* Whether the customer wants to receive printed invoices, such as through postal mail. The default value is `false`.
* @return invoiceDeliveryPrefsPrint
*/
@javax.annotation.Nullable
public Boolean getInvoiceDeliveryPrefsPrint() {
return invoiceDeliveryPrefsPrint;
}
public void setInvoiceDeliveryPrefsPrint(Boolean invoiceDeliveryPrefsPrint) {
this.invoiceDeliveryPrefsPrint = invoiceDeliveryPrefsPrint;
}
public UpdateAccountRequest invoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
return this;
}
/**
* Invoice template ID, configured in Billing Settings in the Zuora UI.
* @return invoiceTemplateId
*/
@javax.annotation.Nullable
public String getInvoiceTemplateId() {
return invoiceTemplateId;
}
public void setInvoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
}
public UpdateAccountRequest name(String name) {
this.name = name;
return this;
}
/**
* Account name, up to 255 characters.
* @return name
*/
@javax.annotation.Nullable
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public UpdateAccountRequest notes(String notes) {
this.notes = notes;
return this;
}
/**
* A string of up to 65,535 characters.
* @return notes
*/
@javax.annotation.Nullable
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
this.notes = notes;
}
public UpdateAccountRequest parentId(String parentId) {
this.parentId = parentId;
return this;
}
/**
* Identifier of the parent customer account for this Account object. The length is 32 characters. Use this field if you have <a href=\"https://knowledgecenter.zuora.com/Billing/Subscriptions/Customer_Accounts/A_Customer_Account_Introduction#Customer_Hierarchy\" target=\"_blank\">Customer Hierarchy</a> enabled.
* @return parentId
*/
@javax.annotation.Nullable
public String getParentId() {
return parentId;
}
public void setParentId(String parentId) {
this.parentId = parentId;
}
public UpdateAccountRequest partnerAccount(Boolean partnerAccount) {
this.partnerAccount = partnerAccount;
return this;
}
/**
* Whether the customer account is a partner, distributor, or reseller. You can set this field to `true` if you have business with distributors or resellers, or operating in B2B model to manage numerous subscriptions through concurrent API requests. After this field is set to `true`, the calculation of account metrics is performed asynchronously during operations such as subscription creation, order changes, invoice generation, and payments. **Note**: This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_customer_accounts/AAA_Overview_of_customer_accounts/Reseller_Account\" target=\"_blank\">Reseller Account</a> feature enabled.
* @return partnerAccount
*/
@javax.annotation.Nullable
public Boolean getPartnerAccount() {
return partnerAccount;
}
public void setPartnerAccount(Boolean partnerAccount) {
this.partnerAccount = partnerAccount;
}
public UpdateAccountRequest paymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
return this;
}
/**
* The name of the payment gateway instance. If null or left unassigned, the Account will use the Default Gateway.
* @return paymentGateway
*/
@javax.annotation.Nullable
public String getPaymentGateway() {
return paymentGateway;
}
public void setPaymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
}
public UpdateAccountRequest paymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
return this;
}
/**
* Payment terms for this account. Possible values are `Due Upon Receipt`, `Net 30`, `Net 60`, `Net 90`.
* @return paymentTerm
*/
@javax.annotation.Nullable
public String getPaymentTerm() {
return paymentTerm;
}
public void setPaymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
}
public UpdateAccountRequest profileNumber(String profileNumber) {
this.profileNumber = profileNumber;
return this;
}
/**
* The number of the communication profile that this account is linked to. You can provide either or both of the `communicationProfileId` and `profileNumber` fields. If both are provided, the request will fail if they do not refer to the same communication profile.
* @return profileNumber
*/
@javax.annotation.Nullable
public String getProfileNumber() {
return profileNumber;
}
public void setProfileNumber(String profileNumber) {
this.profileNumber = profileNumber;
}
public UpdateAccountRequest purchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
return this;
}
/**
* The purchase order number provided by your customer for services, products, or both purchased.
* @return purchaseOrderNumber
*/
@javax.annotation.Nullable
public String getPurchaseOrderNumber() {
return purchaseOrderNumber;
}
public void setPurchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
}
public UpdateAccountRequest rollUpUsage(Boolean rollUpUsage) {
this.rollUpUsage = rollUpUsage;
return this;
}
/**
* Whether the usage of the account roll up to its parent account
* @return rollUpUsage
*/
@javax.annotation.Nullable
public Boolean getRollUpUsage() {
return rollUpUsage;
}
public void setRollUpUsage(Boolean rollUpUsage) {
this.rollUpUsage = rollUpUsage;
}
public UpdateAccountRequest salesRep(String salesRep) {
this.salesRep = salesRep;
return this;
}
/**
* The name of the sales representative associated with this account, if applicable. Maximum of 50 characters.
* @return salesRep
*/
@javax.annotation.Nullable
public String getSalesRep() {
return salesRep;
}
public void setSalesRep(String salesRep) {
this.salesRep = salesRep;
}
public UpdateAccountRequest sequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
return this;
}
/**
* The ID of the billing document sequence set to assign to the customer account. The billing documents to generate for this account will adopt the prefix and starting document number configured in the sequence set. If a customer account has no assigned billing document sequence set, billing documents generated for this account adopt the prefix and starting document number from the default sequence set.
* @return sequenceSetId
*/
@javax.annotation.Nullable
public String getSequenceSetId() {
return sequenceSetId;
}
public void setSequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
}
public UpdateAccountRequest soldToContactId(String soldToContactId) {
this.soldToContactId = soldToContactId;
return this;
}
/**
* The ID of the contact that receives the goods or services. The contact must be associated with the account.
* @return soldToContactId
*/
@javax.annotation.Nullable
public String getSoldToContactId() {
return soldToContactId;
}
public void setSoldToContactId(String soldToContactId) {
this.soldToContactId = soldToContactId;
}
public UpdateAccountRequest soldToContact(UpdateAccountContact soldToContact) {
this.soldToContact = soldToContact;
return this;
}
/**
* Get soldToContact
* @return soldToContact
*/
@javax.annotation.Nullable
public UpdateAccountContact getSoldToContact() {
return soldToContact;
}
public void setSoldToContact(UpdateAccountContact soldToContact) {
this.soldToContact = soldToContact;
}
public UpdateAccountRequest tagging(String tagging) {
this.tagging = tagging;
return this;
}
/**
*
* @return tagging
*/
@javax.annotation.Nullable
public String getTagging() {
return tagging;
}
public void setTagging(String tagging) {
this.tagging = tagging;
}
public UpdateAccountRequest taxInfo(TaxInfo taxInfo) {
this.taxInfo = taxInfo;
return this;
}
/**
* Get taxInfo
* @return taxInfo
*/
@javax.annotation.Nullable
public TaxInfo getTaxInfo() {
return taxInfo;
}
public void setTaxInfo(TaxInfo taxInfo) {
this.taxInfo = taxInfo;
}
public UpdateAccountRequest paymentGatewayNumber(String paymentGatewayNumber) {
this.paymentGatewayNumber = paymentGatewayNumber;
return this;
}
/**
* paymentGatewayNumber
* @return paymentGatewayNumber
*/
@javax.annotation.Nullable
public String getPaymentGatewayNumber() {
return paymentGatewayNumber;
}
public void setPaymentGatewayNumber(String paymentGatewayNumber) {
this.paymentGatewayNumber = paymentGatewayNumber;
}
public UpdateAccountRequest summaryStatementTemplateId(String summaryStatementTemplateId) {
this.summaryStatementTemplateId = summaryStatementTemplateId;
return this;
}
/**
* summaryStatementTemplateId
* @return summaryStatementTemplateId
*/
@javax.annotation.Nullable
public String getSummaryStatementTemplateId() {
return summaryStatementTemplateId;
}
public void setSummaryStatementTemplateId(String summaryStatementTemplateId) {
this.summaryStatementTemplateId = summaryStatementTemplateId;
}
public UpdateAccountRequest einvoiceProfile(AccountEInvoiceProfile einvoiceProfile) {
this.einvoiceProfile = einvoiceProfile;
return this;
}
/**
* Get einvoiceProfile
* @return einvoiceProfile
*/
@javax.annotation.Nullable
public AccountEInvoiceProfile getEinvoiceProfile() {
return einvoiceProfile;
}
public void setEinvoiceProfile(AccountEInvoiceProfile einvoiceProfile) {
this.einvoiceProfile = einvoiceProfile;
}
public UpdateAccountRequest organizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
return this;
}
/**
* Get organizationLabel
* @return organizationLabel
*/
@javax.annotation.Nullable
public String getOrganizationLabel() {
return organizationLabel;
}
public void setOrganizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the UpdateAccountRequest instance itself
*/
public UpdateAccountRequest putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UpdateAccountRequest updateAccountRequest = (UpdateAccountRequest) o;
return Objects.equals(this.classNS, updateAccountRequest.classNS) &&
Objects.equals(this.customerTypeNS, updateAccountRequest.customerTypeNS) &&
Objects.equals(this.departmentNS, updateAccountRequest.departmentNS) &&
Objects.equals(this.integrationIdNS, updateAccountRequest.integrationIdNS) &&
Objects.equals(this.integrationStatusNS, updateAccountRequest.integrationStatusNS) &&
Objects.equals(this.locationNS, updateAccountRequest.locationNS) &&
Objects.equals(this.subsidiaryNS, updateAccountRequest.subsidiaryNS) &&
Objects.equals(this.syncDateNS, updateAccountRequest.syncDateNS) &&
Objects.equals(this.synctoNetSuiteNS, updateAccountRequest.synctoNetSuiteNS) &&
Objects.equals(this.additionalEmailAddresses, updateAccountRequest.additionalEmailAddresses) &&
Objects.equals(this.autoPay, updateAccountRequest.autoPay) &&
Objects.equals(this.batch, updateAccountRequest.batch) &&
Objects.equals(this.billCycleDay, updateAccountRequest.billCycleDay) &&
Objects.equals(this.billToContactId, updateAccountRequest.billToContactId) &&
Objects.equals(this.billToContact, updateAccountRequest.billToContact) &&
Objects.equals(this.shipToContactId, updateAccountRequest.shipToContactId) &&
Objects.equals(this.shipToContact, updateAccountRequest.shipToContact) &&
Objects.equals(this.communicationProfileId, updateAccountRequest.communicationProfileId) &&
Objects.equals(this.creditMemoTemplateId, updateAccountRequest.creditMemoTemplateId) &&
Objects.equals(this.crmId, updateAccountRequest.crmId) &&
Objects.equals(this.customerServiceRepName, updateAccountRequest.customerServiceRepName) &&
Objects.equals(this.debitMemoTemplateId, updateAccountRequest.debitMemoTemplateId) &&
Objects.equals(this.defaultPaymentMethodId, updateAccountRequest.defaultPaymentMethodId) &&
Objects.equals(this.invoiceDeliveryPrefsEmail, updateAccountRequest.invoiceDeliveryPrefsEmail) &&
Objects.equals(this.invoiceDeliveryPrefsPrint, updateAccountRequest.invoiceDeliveryPrefsPrint) &&
Objects.equals(this.invoiceTemplateId, updateAccountRequest.invoiceTemplateId) &&
Objects.equals(this.name, updateAccountRequest.name) &&
Objects.equals(this.notes, updateAccountRequest.notes) &&
Objects.equals(this.parentId, updateAccountRequest.parentId) &&
Objects.equals(this.partnerAccount, updateAccountRequest.partnerAccount) &&
Objects.equals(this.paymentGateway, updateAccountRequest.paymentGateway) &&
Objects.equals(this.paymentTerm, updateAccountRequest.paymentTerm) &&
Objects.equals(this.profileNumber, updateAccountRequest.profileNumber) &&
Objects.equals(this.purchaseOrderNumber, updateAccountRequest.purchaseOrderNumber) &&
Objects.equals(this.rollUpUsage, updateAccountRequest.rollUpUsage) &&
Objects.equals(this.salesRep, updateAccountRequest.salesRep) &&
Objects.equals(this.sequenceSetId, updateAccountRequest.sequenceSetId) &&
Objects.equals(this.soldToContactId, updateAccountRequest.soldToContactId) &&
Objects.equals(this.soldToContact, updateAccountRequest.soldToContact) &&
Objects.equals(this.tagging, updateAccountRequest.tagging) &&
Objects.equals(this.taxInfo, updateAccountRequest.taxInfo) &&
Objects.equals(this.paymentGatewayNumber, updateAccountRequest.paymentGatewayNumber) &&
Objects.equals(this.summaryStatementTemplateId, updateAccountRequest.summaryStatementTemplateId) &&
Objects.equals(this.einvoiceProfile, updateAccountRequest.einvoiceProfile) &&
Objects.equals(this.organizationLabel, updateAccountRequest.organizationLabel)&&
Objects.equals(this.additionalProperties, updateAccountRequest.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(classNS, customerTypeNS, departmentNS, integrationIdNS, integrationStatusNS, locationNS, subsidiaryNS, syncDateNS, synctoNetSuiteNS, additionalEmailAddresses, autoPay, batch, billCycleDay, billToContactId, billToContact, shipToContactId, shipToContact, communicationProfileId, creditMemoTemplateId, crmId, customerServiceRepName, debitMemoTemplateId, defaultPaymentMethodId, invoiceDeliveryPrefsEmail, invoiceDeliveryPrefsPrint, invoiceTemplateId, name, notes, parentId, partnerAccount, paymentGateway, paymentTerm, profileNumber, purchaseOrderNumber, rollUpUsage, salesRep, sequenceSetId, soldToContactId, soldToContact, tagging, taxInfo, paymentGatewayNumber, summaryStatementTemplateId, einvoiceProfile, organizationLabel, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UpdateAccountRequest {\n");
sb.append(" classNS: ").append(toIndentedString(classNS)).append("\n");
sb.append(" customerTypeNS: ").append(toIndentedString(customerTypeNS)).append("\n");
sb.append(" departmentNS: ").append(toIndentedString(departmentNS)).append("\n");
sb.append(" integrationIdNS: ").append(toIndentedString(integrationIdNS)).append("\n");
sb.append(" integrationStatusNS: ").append(toIndentedString(integrationStatusNS)).append("\n");
sb.append(" locationNS: ").append(toIndentedString(locationNS)).append("\n");
sb.append(" subsidiaryNS: ").append(toIndentedString(subsidiaryNS)).append("\n");
sb.append(" syncDateNS: ").append(toIndentedString(syncDateNS)).append("\n");
sb.append(" synctoNetSuiteNS: ").append(toIndentedString(synctoNetSuiteNS)).append("\n");
sb.append(" additionalEmailAddresses: ").append(toIndentedString(additionalEmailAddresses)).append("\n");
sb.append(" autoPay: ").append(toIndentedString(autoPay)).append("\n");
sb.append(" batch: ").append(toIndentedString(batch)).append("\n");
sb.append(" billCycleDay: ").append(toIndentedString(billCycleDay)).append("\n");
sb.append(" billToContactId: ").append(toIndentedString(billToContactId)).append("\n");
sb.append(" billToContact: ").append(toIndentedString(billToContact)).append("\n");
sb.append(" shipToContactId: ").append(toIndentedString(shipToContactId)).append("\n");
sb.append(" shipToContact: ").append(toIndentedString(shipToContact)).append("\n");
sb.append(" communicationProfileId: ").append(toIndentedString(communicationProfileId)).append("\n");
sb.append(" creditMemoTemplateId: ").append(toIndentedString(creditMemoTemplateId)).append("\n");
sb.append(" crmId: ").append(toIndentedString(crmId)).append("\n");
sb.append(" customerServiceRepName: ").append(toIndentedString(customerServiceRepName)).append("\n");
sb.append(" debitMemoTemplateId: ").append(toIndentedString(debitMemoTemplateId)).append("\n");
sb.append(" defaultPaymentMethodId: ").append(toIndentedString(defaultPaymentMethodId)).append("\n");
sb.append(" invoiceDeliveryPrefsEmail: ").append(toIndentedString(invoiceDeliveryPrefsEmail)).append("\n");
sb.append(" invoiceDeliveryPrefsPrint: ").append(toIndentedString(invoiceDeliveryPrefsPrint)).append("\n");
sb.append(" invoiceTemplateId: ").append(toIndentedString(invoiceTemplateId)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" notes: ").append(toIndentedString(notes)).append("\n");
sb.append(" parentId: ").append(toIndentedString(parentId)).append("\n");
sb.append(" partnerAccount: ").append(toIndentedString(partnerAccount)).append("\n");
sb.append(" paymentGateway: ").append(toIndentedString(paymentGateway)).append("\n");
sb.append(" paymentTerm: ").append(toIndentedString(paymentTerm)).append("\n");
sb.append(" profileNumber: ").append(toIndentedString(profileNumber)).append("\n");
sb.append(" purchaseOrderNumber: ").append(toIndentedString(purchaseOrderNumber)).append("\n");
sb.append(" rollUpUsage: ").append(toIndentedString(rollUpUsage)).append("\n");
sb.append(" salesRep: ").append(toIndentedString(salesRep)).append("\n");
sb.append(" sequenceSetId: ").append(toIndentedString(sequenceSetId)).append("\n");
sb.append(" soldToContactId: ").append(toIndentedString(soldToContactId)).append("\n");
sb.append(" soldToContact: ").append(toIndentedString(soldToContact)).append("\n");
sb.append(" tagging: ").append(toIndentedString(tagging)).append("\n");
sb.append(" taxInfo: ").append(toIndentedString(taxInfo)).append("\n");
sb.append(" paymentGatewayNumber: ").append(toIndentedString(paymentGatewayNumber)).append("\n");
sb.append(" summaryStatementTemplateId: ").append(toIndentedString(summaryStatementTemplateId)).append("\n");
sb.append(" einvoiceProfile: ").append(toIndentedString(einvoiceProfile)).append("\n");
sb.append(" organizationLabel: ").append(toIndentedString(organizationLabel)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("Class__NS");
openapiFields.add("CustomerType__NS");
openapiFields.add("Department__NS");
openapiFields.add("IntegrationId__NS");
openapiFields.add("IntegrationStatus__NS");
openapiFields.add("Location__NS");
openapiFields.add("Subsidiary__NS");
openapiFields.add("SyncDate__NS");
openapiFields.add("SynctoNetSuite__NS");
openapiFields.add("additionalEmailAddresses");
openapiFields.add("autoPay");
openapiFields.add("batch");
openapiFields.add("billCycleDay");
openapiFields.add("billToContactId");
openapiFields.add("billToContact");
openapiFields.add("shipToContactId");
openapiFields.add("shipToContact");
openapiFields.add("communicationProfileId");
openapiFields.add("creditMemoTemplateId");
openapiFields.add("crmId");
openapiFields.add("customerServiceRepName");
openapiFields.add("debitMemoTemplateId");
openapiFields.add("defaultPaymentMethodId");
openapiFields.add("invoiceDeliveryPrefsEmail");
openapiFields.add("invoiceDeliveryPrefsPrint");
openapiFields.add("invoiceTemplateId");
openapiFields.add("name");
openapiFields.add("notes");
openapiFields.add("parentId");
openapiFields.add("partnerAccount");
openapiFields.add("paymentGateway");
openapiFields.add("paymentTerm");
openapiFields.add("profileNumber");
openapiFields.add("purchaseOrderNumber");
openapiFields.add("rollUpUsage");
openapiFields.add("salesRep");
openapiFields.add("sequenceSetId");
openapiFields.add("soldToContactId");
openapiFields.add("soldToContact");
openapiFields.add("tagging");
openapiFields.add("taxInfo");
openapiFields.add("paymentGatewayNumber");
openapiFields.add("summaryStatementTemplateId");
openapiFields.add("einvoiceProfile");
openapiFields.add("organizationLabel");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to UpdateAccountRequest
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!UpdateAccountRequest.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in UpdateAccountRequest is not found in the empty JSON string", UpdateAccountRequest.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("Class__NS") != null && !jsonObj.get("Class__NS").isJsonNull()) && !jsonObj.get("Class__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Class__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Class__NS").toString()));
}
if ((jsonObj.get("CustomerType__NS") != null && !jsonObj.get("CustomerType__NS").isJsonNull()) && !jsonObj.get("CustomerType__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `CustomerType__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("CustomerType__NS").toString()));
}
// validate the optional field `CustomerType__NS`
if (jsonObj.get("CustomerType__NS") != null && !jsonObj.get("CustomerType__NS").isJsonNull()) {
AccountObjectNSFieldsCustomerTypeNS.validateJsonElement(jsonObj.get("CustomerType__NS"));
}
if ((jsonObj.get("Department__NS") != null && !jsonObj.get("Department__NS").isJsonNull()) && !jsonObj.get("Department__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Department__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Department__NS").toString()));
}
if ((jsonObj.get("IntegrationId__NS") != null && !jsonObj.get("IntegrationId__NS").isJsonNull()) && !jsonObj.get("IntegrationId__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationId__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationId__NS").toString()));
}
if ((jsonObj.get("IntegrationStatus__NS") != null && !jsonObj.get("IntegrationStatus__NS").isJsonNull()) && !jsonObj.get("IntegrationStatus__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationStatus__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationStatus__NS").toString()));
}
if ((jsonObj.get("Location__NS") != null && !jsonObj.get("Location__NS").isJsonNull()) && !jsonObj.get("Location__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Location__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Location__NS").toString()));
}
if ((jsonObj.get("Subsidiary__NS") != null && !jsonObj.get("Subsidiary__NS").isJsonNull()) && !jsonObj.get("Subsidiary__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Subsidiary__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Subsidiary__NS").toString()));
}
if ((jsonObj.get("SyncDate__NS") != null && !jsonObj.get("SyncDate__NS").isJsonNull()) && !jsonObj.get("SyncDate__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SyncDate__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SyncDate__NS").toString()));
}
if ((jsonObj.get("SynctoNetSuite__NS") != null && !jsonObj.get("SynctoNetSuite__NS").isJsonNull()) && !jsonObj.get("SynctoNetSuite__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SynctoNetSuite__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SynctoNetSuite__NS").toString()));
}
// validate the optional field `SynctoNetSuite__NS`
if (jsonObj.get("SynctoNetSuite__NS") != null && !jsonObj.get("SynctoNetSuite__NS").isJsonNull()) {
AccountObjectNSFieldsSynctoNetSuiteNS.validateJsonElement(jsonObj.get("SynctoNetSuite__NS"));
}
// ensure the optional json data is an array if present
if (jsonObj.get("additionalEmailAddresses") != null && !jsonObj.get("additionalEmailAddresses").isJsonNull() && !jsonObj.get("additionalEmailAddresses").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `additionalEmailAddresses` to be an array in the JSON string but got `%s`", jsonObj.get("additionalEmailAddresses").toString()));
}
if ((jsonObj.get("batch") != null && !jsonObj.get("batch").isJsonNull()) && !jsonObj.get("batch").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `batch` to be a primitive type in the JSON string but got `%s`", jsonObj.get("batch").toString()));
}
if ((jsonObj.get("billToContactId") != null && !jsonObj.get("billToContactId").isJsonNull()) && !jsonObj.get("billToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billToContactId").toString()));
}
if ((jsonObj.get("shipToContactId") != null && !jsonObj.get("shipToContactId").isJsonNull()) && !jsonObj.get("shipToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `shipToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("shipToContactId").toString()));
}
if ((jsonObj.get("communicationProfileId") != null && !jsonObj.get("communicationProfileId").isJsonNull()) && !jsonObj.get("communicationProfileId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `communicationProfileId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("communicationProfileId").toString()));
}
if ((jsonObj.get("creditMemoTemplateId") != null && !jsonObj.get("creditMemoTemplateId").isJsonNull()) && !jsonObj.get("creditMemoTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creditMemoTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creditMemoTemplateId").toString()));
}
if ((jsonObj.get("crmId") != null && !jsonObj.get("crmId").isJsonNull()) && !jsonObj.get("crmId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `crmId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("crmId").toString()));
}
if ((jsonObj.get("customerServiceRepName") != null && !jsonObj.get("customerServiceRepName").isJsonNull()) && !jsonObj.get("customerServiceRepName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `customerServiceRepName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("customerServiceRepName").toString()));
}
if ((jsonObj.get("debitMemoTemplateId") != null && !jsonObj.get("debitMemoTemplateId").isJsonNull()) && !jsonObj.get("debitMemoTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `debitMemoTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("debitMemoTemplateId").toString()));
}
if ((jsonObj.get("defaultPaymentMethodId") != null && !jsonObj.get("defaultPaymentMethodId").isJsonNull()) && !jsonObj.get("defaultPaymentMethodId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `defaultPaymentMethodId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("defaultPaymentMethodId").toString()));
}
if ((jsonObj.get("invoiceTemplateId") != null && !jsonObj.get("invoiceTemplateId").isJsonNull()) && !jsonObj.get("invoiceTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceTemplateId").toString()));
}
if ((jsonObj.get("name") != null && !jsonObj.get("name").isJsonNull()) && !jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if ((jsonObj.get("notes") != null && !jsonObj.get("notes").isJsonNull()) && !jsonObj.get("notes").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `notes` to be a primitive type in the JSON string but got `%s`", jsonObj.get("notes").toString()));
}
if ((jsonObj.get("parentId") != null && !jsonObj.get("parentId").isJsonNull()) && !jsonObj.get("parentId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `parentId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("parentId").toString()));
}
if ((jsonObj.get("paymentGateway") != null && !jsonObj.get("paymentGateway").isJsonNull()) && !jsonObj.get("paymentGateway").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentGateway` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentGateway").toString()));
}
if ((jsonObj.get("paymentTerm") != null && !jsonObj.get("paymentTerm").isJsonNull()) && !jsonObj.get("paymentTerm").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentTerm` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentTerm").toString()));
}
if ((jsonObj.get("profileNumber") != null && !jsonObj.get("profileNumber").isJsonNull()) && !jsonObj.get("profileNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `profileNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("profileNumber").toString()));
}
if ((jsonObj.get("purchaseOrderNumber") != null && !jsonObj.get("purchaseOrderNumber").isJsonNull()) && !jsonObj.get("purchaseOrderNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `purchaseOrderNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("purchaseOrderNumber").toString()));
}
if ((jsonObj.get("salesRep") != null && !jsonObj.get("salesRep").isJsonNull()) && !jsonObj.get("salesRep").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `salesRep` to be a primitive type in the JSON string but got `%s`", jsonObj.get("salesRep").toString()));
}
if ((jsonObj.get("sequenceSetId") != null && !jsonObj.get("sequenceSetId").isJsonNull()) && !jsonObj.get("sequenceSetId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sequenceSetId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sequenceSetId").toString()));
}
if ((jsonObj.get("soldToContactId") != null && !jsonObj.get("soldToContactId").isJsonNull()) && !jsonObj.get("soldToContactId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldToContactId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldToContactId").toString()));
}
if ((jsonObj.get("tagging") != null && !jsonObj.get("tagging").isJsonNull()) && !jsonObj.get("tagging").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `tagging` to be a primitive type in the JSON string but got `%s`", jsonObj.get("tagging").toString()));
}
// validate the optional field `taxInfo`
if (jsonObj.get("taxInfo") != null && !jsonObj.get("taxInfo").isJsonNull()) {
TaxInfo.validateJsonElement(jsonObj.get("taxInfo"));
}
if ((jsonObj.get("paymentGatewayNumber") != null && !jsonObj.get("paymentGatewayNumber").isJsonNull()) && !jsonObj.get("paymentGatewayNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentGatewayNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentGatewayNumber").toString()));
}
if ((jsonObj.get("summaryStatementTemplateId") != null && !jsonObj.get("summaryStatementTemplateId").isJsonNull()) && !jsonObj.get("summaryStatementTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `summaryStatementTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("summaryStatementTemplateId").toString()));
}
// validate the optional field `einvoiceProfile`
if (jsonObj.get("einvoiceProfile") != null && !jsonObj.get("einvoiceProfile").isJsonNull()) {
AccountEInvoiceProfile.validateJsonElement(jsonObj.get("einvoiceProfile"));
}
if ((jsonObj.get("organizationLabel") != null && !jsonObj.get("organizationLabel").isJsonNull()) && !jsonObj.get("organizationLabel").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `organizationLabel` to be a primitive type in the JSON string but got `%s`", jsonObj.get("organizationLabel").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!UpdateAccountRequest.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'UpdateAccountRequest' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(UpdateAccountRequest.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, UpdateAccountRequest value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public UpdateAccountRequest read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
UpdateAccountRequest instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of UpdateAccountRequest given an JSON string
*
* @param jsonString JSON string
* @return An instance of UpdateAccountRequest
* @throws IOException if the JSON string is invalid with respect to UpdateAccountRequest
*/
public static UpdateAccountRequest fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, UpdateAccountRequest.class);
}
/**
* Convert an instance of UpdateAccountRequest to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy