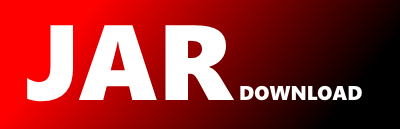
com.zuora.model.UpdateInvoiceItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.CreateTaxationItem;
import com.zuora.model.RevRecTrigger;
import com.zuora.model.TaxMode;
import com.zuora.model.UpdateDiscountInvoiceItem;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* UpdateInvoiceItem
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class UpdateInvoiceItem {
public static final String SERIALIZED_NAME_INTEGRATION_ID_N_S = "IntegrationId__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_ID_N_S)
private String integrationIdNS;
public static final String SERIALIZED_NAME_INTEGRATION_STATUS_N_S = "IntegrationStatus__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_STATUS_N_S)
private String integrationStatusNS;
public static final String SERIALIZED_NAME_SYNC_DATE_N_S = "SyncDate__NS";
@SerializedName(SERIALIZED_NAME_SYNC_DATE_N_S)
private String syncDateNS;
public static final String SERIALIZED_NAME_ACCOUNTING_CODE = "accountingCode";
@SerializedName(SERIALIZED_NAME_ACCOUNTING_CODE)
private String accountingCode;
public static final String SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNTING_CODE = "adjustmentLiabilityAccountingCode";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNTING_CODE)
private String adjustmentLiabilityAccountingCode;
public static final String SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNTING_CODE = "adjustmentRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNTING_CODE)
private String adjustmentRevenueAccountingCode;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_BOOKING_REFERENCE = "bookingReference";
@SerializedName(SERIALIZED_NAME_BOOKING_REFERENCE)
private String bookingReference;
public static final String SERIALIZED_NAME_CHARGE_DATE = "chargeDate";
@SerializedName(SERIALIZED_NAME_CHARGE_DATE)
private String chargeDate;
public static final String SERIALIZED_NAME_CHARGE_NAME = "chargeName";
@SerializedName(SERIALIZED_NAME_CHARGE_NAME)
private String chargeName;
public static final String SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNTING_CODE = "contractAssetAccountingCode";
@SerializedName(SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNTING_CODE)
private String contractAssetAccountingCode;
public static final String SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNTING_CODE = "contractLiabilityAccountingCode";
@SerializedName(SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNTING_CODE)
private String contractLiabilityAccountingCode;
public static final String SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNTING_CODE = "contractRecognizedRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNTING_CODE)
private String contractRecognizedRevenueAccountingCode;
public static final String SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE = "deferredRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE)
private String deferredRevenueAccountingCode;
public static final String SERIALIZED_NAME_DELETE = "delete";
@SerializedName(SERIALIZED_NAME_DELETE)
private Boolean delete;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_DISCOUNT_ITEMS = "discountItems";
@SerializedName(SERIALIZED_NAME_DISCOUNT_ITEMS)
private List discountItems;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING = "excludeItemBillingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBillingFromRevenueAccounting;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_ITEM_TYPE = "itemType";
@SerializedName(SERIALIZED_NAME_ITEM_TYPE)
private String itemType;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_ID = "productRatePlanChargeId";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_ID)
private String productRatePlanChargeId;
public static final String SERIALIZED_NAME_PURCHASE_ORDER_NUMBER = "purchaseOrderNumber";
@SerializedName(SERIALIZED_NAME_PURCHASE_ORDER_NUMBER)
private String purchaseOrderNumber;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE = "recognizedRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE)
private String recognizedRevenueAccountingCode;
public static final String SERIALIZED_NAME_REV_REC_CODE = "revRecCode";
@SerializedName(SERIALIZED_NAME_REV_REC_CODE)
private String revRecCode;
public static final String SERIALIZED_NAME_REV_REC_TRIGGER_CONDITION = "revRecTriggerCondition";
@SerializedName(SERIALIZED_NAME_REV_REC_TRIGGER_CONDITION)
private RevRecTrigger revRecTriggerCondition;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME = "revenueRecognitionRuleName";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME)
private String revenueRecognitionRuleName;
public static final String SERIALIZED_NAME_SERVICE_END_DATE = "serviceEndDate";
@SerializedName(SERIALIZED_NAME_SERVICE_END_DATE)
private LocalDate serviceEndDate;
public static final String SERIALIZED_NAME_SERVICE_START_DATE = "serviceStartDate";
@SerializedName(SERIALIZED_NAME_SERVICE_START_DATE)
private LocalDate serviceStartDate;
public static final String SERIALIZED_NAME_SKU = "sku";
@SerializedName(SERIALIZED_NAME_SKU)
private String sku;
public static final String SERIALIZED_NAME_TAX_CODE = "taxCode";
@SerializedName(SERIALIZED_NAME_TAX_CODE)
private String taxCode;
public static final String SERIALIZED_NAME_TAX_ITEMS = "taxItems";
@SerializedName(SERIALIZED_NAME_TAX_ITEMS)
private List taxItems;
public static final String SERIALIZED_NAME_TAX_MODE = "taxMode";
@SerializedName(SERIALIZED_NAME_TAX_MODE)
private TaxMode taxMode;
public static final String SERIALIZED_NAME_UNBILLED_RECEIVABLES_ACCOUNTING_CODE = "unbilledReceivablesAccountingCode";
@SerializedName(SERIALIZED_NAME_UNBILLED_RECEIVABLES_ACCOUNTING_CODE)
private String unbilledReceivablesAccountingCode;
public static final String SERIALIZED_NAME_UNIT_PRICE = "unitPrice";
@SerializedName(SERIALIZED_NAME_UNIT_PRICE)
private BigDecimal unitPrice;
public static final String SERIALIZED_NAME_UOM = "uom";
@SerializedName(SERIALIZED_NAME_UOM)
private String uom;
public UpdateInvoiceItem() {
}
public UpdateInvoiceItem integrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
return this;
}
/**
* ID of the corresponding object in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationIdNS
*/
@javax.annotation.Nullable
public String getIntegrationIdNS() {
return integrationIdNS;
}
public void setIntegrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
}
public UpdateInvoiceItem integrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
return this;
}
/**
* Status of the invoice item's synchronization with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationStatusNS
*/
@javax.annotation.Nullable
public String getIntegrationStatusNS() {
return integrationStatusNS;
}
public void setIntegrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
}
public UpdateInvoiceItem syncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
return this;
}
/**
* Date when the invoice item was synchronized with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return syncDateNS
*/
@javax.annotation.Nullable
public String getSyncDateNS() {
return syncDateNS;
}
public void setSyncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
}
public UpdateInvoiceItem accountingCode(String accountingCode) {
this.accountingCode = accountingCode;
return this;
}
/**
* The accounting code associated with the invoice item.
* @return accountingCode
*/
@javax.annotation.Nullable
public String getAccountingCode() {
return accountingCode;
}
public void setAccountingCode(String accountingCode) {
this.accountingCode = accountingCode;
}
public UpdateInvoiceItem adjustmentLiabilityAccountingCode(String adjustmentLiabilityAccountingCode) {
this.adjustmentLiabilityAccountingCode = adjustmentLiabilityAccountingCode;
return this;
}
/**
* The accounting code for adjustment liability. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return adjustmentLiabilityAccountingCode
*/
@javax.annotation.Nullable
public String getAdjustmentLiabilityAccountingCode() {
return adjustmentLiabilityAccountingCode;
}
public void setAdjustmentLiabilityAccountingCode(String adjustmentLiabilityAccountingCode) {
this.adjustmentLiabilityAccountingCode = adjustmentLiabilityAccountingCode;
}
public UpdateInvoiceItem adjustmentRevenueAccountingCode(String adjustmentRevenueAccountingCode) {
this.adjustmentRevenueAccountingCode = adjustmentRevenueAccountingCode;
return this;
}
/**
* The accounting code for adjustment revenue. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return adjustmentRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getAdjustmentRevenueAccountingCode() {
return adjustmentRevenueAccountingCode;
}
public void setAdjustmentRevenueAccountingCode(String adjustmentRevenueAccountingCode) {
this.adjustmentRevenueAccountingCode = adjustmentRevenueAccountingCode;
}
public UpdateInvoiceItem amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The amount of the invoice item. - For tax-inclusive invoice items, the amount indicates the invoice item amount including tax. - For tax-exclusive invoice items, the amount indicates the invoice item amount excluding tax.
* @return amount
*/
@javax.annotation.Nullable
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public UpdateInvoiceItem bookingReference(String bookingReference) {
this.bookingReference = bookingReference;
return this;
}
/**
* The booking reference of the invoice item. \\n**Note**: This field is only available if id is null. \\n
* @return bookingReference
*/
@javax.annotation.Nullable
public String getBookingReference() {
return bookingReference;
}
public void setBookingReference(String bookingReference) {
this.bookingReference = bookingReference;
}
public UpdateInvoiceItem chargeDate(String chargeDate) {
this.chargeDate = chargeDate;
return this;
}
/**
* The date when the invoice item is charged, in `yyyy-mm-dd hh:mm:ss` format.
* @return chargeDate
*/
@javax.annotation.Nullable
public String getChargeDate() {
return chargeDate;
}
public void setChargeDate(String chargeDate) {
this.chargeDate = chargeDate;
}
public UpdateInvoiceItem chargeName(String chargeName) {
this.chargeName = chargeName;
return this;
}
/**
* The name of the charge associated with the invoice item. This field is required if the `productRatePlanChargeId` field is not specified in the request.
* @return chargeName
*/
@javax.annotation.Nullable
public String getChargeName() {
return chargeName;
}
public void setChargeName(String chargeName) {
this.chargeName = chargeName;
}
public UpdateInvoiceItem contractAssetAccountingCode(String contractAssetAccountingCode) {
this.contractAssetAccountingCode = contractAssetAccountingCode;
return this;
}
/**
* The accounting code for contract asset. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return contractAssetAccountingCode
*/
@javax.annotation.Nullable
public String getContractAssetAccountingCode() {
return contractAssetAccountingCode;
}
public void setContractAssetAccountingCode(String contractAssetAccountingCode) {
this.contractAssetAccountingCode = contractAssetAccountingCode;
}
public UpdateInvoiceItem contractLiabilityAccountingCode(String contractLiabilityAccountingCode) {
this.contractLiabilityAccountingCode = contractLiabilityAccountingCode;
return this;
}
/**
* The accounting code for contract liability. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return contractLiabilityAccountingCode
*/
@javax.annotation.Nullable
public String getContractLiabilityAccountingCode() {
return contractLiabilityAccountingCode;
}
public void setContractLiabilityAccountingCode(String contractLiabilityAccountingCode) {
this.contractLiabilityAccountingCode = contractLiabilityAccountingCode;
}
public UpdateInvoiceItem contractRecognizedRevenueAccountingCode(String contractRecognizedRevenueAccountingCode) {
this.contractRecognizedRevenueAccountingCode = contractRecognizedRevenueAccountingCode;
return this;
}
/**
* The accounting code for contract recognized revenue. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return contractRecognizedRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getContractRecognizedRevenueAccountingCode() {
return contractRecognizedRevenueAccountingCode;
}
public void setContractRecognizedRevenueAccountingCode(String contractRecognizedRevenueAccountingCode) {
this.contractRecognizedRevenueAccountingCode = contractRecognizedRevenueAccountingCode;
}
public UpdateInvoiceItem deferredRevenueAccountingCode(String deferredRevenueAccountingCode) {
this.deferredRevenueAccountingCode = deferredRevenueAccountingCode;
return this;
}
/**
* The accounting code for the deferred revenue, such as Monthly Recurring Liability. **Note:** This field is only available if you have Zuora Finance enabled.
* @return deferredRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getDeferredRevenueAccountingCode() {
return deferredRevenueAccountingCode;
}
public void setDeferredRevenueAccountingCode(String deferredRevenueAccountingCode) {
this.deferredRevenueAccountingCode = deferredRevenueAccountingCode;
}
public UpdateInvoiceItem delete(Boolean delete) {
this.delete = delete;
return this;
}
/**
* Indicates whether to delete the existing invoice item. **Note**: This field is only available if id is not null.
* @return delete
*/
@javax.annotation.Nullable
public Boolean getDelete() {
return delete;
}
public void setDelete(Boolean delete) {
this.delete = delete;
}
public UpdateInvoiceItem description(String description) {
this.description = description;
return this;
}
/**
* The description of the invoice item.
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public UpdateInvoiceItem discountItems(List discountItems) {
this.discountItems = discountItems;
return this;
}
public UpdateInvoiceItem addDiscountItemsItem(UpdateDiscountInvoiceItem discountItemsItem) {
if (this.discountItems == null) {
this.discountItems = new ArrayList<>();
}
this.discountItems.add(discountItemsItem);
return this;
}
/**
* Container for discount items. The maximum number of discount items is 10.
* @return discountItems
*/
@javax.annotation.Nullable
public List getDiscountItems() {
return discountItems;
}
public void setDiscountItems(List discountItems) {
this.discountItems = discountItems;
}
public UpdateInvoiceItem excludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
return this;
}
/**
* The flag to exclude the invoice item from revenue accounting. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return excludeItemBillingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBillingFromRevenueAccounting() {
return excludeItemBillingFromRevenueAccounting;
}
public void setExcludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
}
public UpdateInvoiceItem id(String id) {
this.id = id;
return this;
}
/**
* The unique ID of the invoice item.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public UpdateInvoiceItem itemType(String itemType) {
this.itemType = itemType;
return this;
}
/**
* The type of the invoice item.
* @return itemType
*/
@javax.annotation.Nullable
public String getItemType() {
return itemType;
}
public void setItemType(String itemType) {
this.itemType = itemType;
}
public UpdateInvoiceItem productRatePlanChargeId(String productRatePlanChargeId) {
this.productRatePlanChargeId = productRatePlanChargeId;
return this;
}
/**
* The ID of the product rate plan charge that the invoice item is created from. If you specify a value for the `productRatePlanChargeId` field in the request, Zuora directly copies the values of the following fields from the corresponding product rate plan charge, regardless of the values specified in the request body: - `chargeName` - `sku` - `uom` - `taxCode` - `taxMode` - `accountingCode` - `deferredRevenueAccountingCode` - `recognizedRevenueAccountingCode` **Note**: This field is only available if id is null.
* @return productRatePlanChargeId
*/
@javax.annotation.Nullable
public String getProductRatePlanChargeId() {
return productRatePlanChargeId;
}
public void setProductRatePlanChargeId(String productRatePlanChargeId) {
this.productRatePlanChargeId = productRatePlanChargeId;
}
public UpdateInvoiceItem purchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
return this;
}
/**
* The purchase order number associated the invoice item.
* @return purchaseOrderNumber
*/
@javax.annotation.Nullable
public String getPurchaseOrderNumber() {
return purchaseOrderNumber;
}
public void setPurchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
}
public UpdateInvoiceItem quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* The number of units for the invoice item.
* @return quantity
*/
@javax.annotation.Nullable
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public UpdateInvoiceItem recognizedRevenueAccountingCode(String recognizedRevenueAccountingCode) {
this.recognizedRevenueAccountingCode = recognizedRevenueAccountingCode;
return this;
}
/**
* The accounting code for the recognized revenue, such as Monthly Recurring Charges or Overage Charges. **Note:** This field is only available if you have Zuora Finance enabled.
* @return recognizedRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getRecognizedRevenueAccountingCode() {
return recognizedRevenueAccountingCode;
}
public void setRecognizedRevenueAccountingCode(String recognizedRevenueAccountingCode) {
this.recognizedRevenueAccountingCode = recognizedRevenueAccountingCode;
}
public UpdateInvoiceItem revRecCode(String revRecCode) {
this.revRecCode = revRecCode;
return this;
}
/**
* The revenue recognition code.
* @return revRecCode
*/
@javax.annotation.Nullable
public String getRevRecCode() {
return revRecCode;
}
public void setRevRecCode(String revRecCode) {
this.revRecCode = revRecCode;
}
public UpdateInvoiceItem revRecTriggerCondition(RevRecTrigger revRecTriggerCondition) {
this.revRecTriggerCondition = revRecTriggerCondition;
return this;
}
/**
* Get revRecTriggerCondition
* @return revRecTriggerCondition
*/
@javax.annotation.Nullable
public RevRecTrigger getRevRecTriggerCondition() {
return revRecTriggerCondition;
}
public void setRevRecTriggerCondition(RevRecTrigger revRecTriggerCondition) {
this.revRecTriggerCondition = revRecTriggerCondition;
}
public UpdateInvoiceItem revenueRecognitionRuleName(String revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
return this;
}
/**
* The name of the revenue recognition rule governing the revenueschedule. **Note:** This field is only available if you have Zuora Finance enabled.
* @return revenueRecognitionRuleName
*/
@javax.annotation.Nullable
public String getRevenueRecognitionRuleName() {
return revenueRecognitionRuleName;
}
public void setRevenueRecognitionRuleName(String revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
}
public UpdateInvoiceItem serviceEndDate(LocalDate serviceEndDate) {
this.serviceEndDate = serviceEndDate;
return this;
}
/**
* The service end date of the invoice item.
* @return serviceEndDate
*/
@javax.annotation.Nullable
public LocalDate getServiceEndDate() {
return serviceEndDate;
}
public void setServiceEndDate(LocalDate serviceEndDate) {
this.serviceEndDate = serviceEndDate;
}
public UpdateInvoiceItem serviceStartDate(LocalDate serviceStartDate) {
this.serviceStartDate = serviceStartDate;
return this;
}
/**
* The service start date of the invoice item.
* @return serviceStartDate
*/
@javax.annotation.Nullable
public LocalDate getServiceStartDate() {
return serviceStartDate;
}
public void setServiceStartDate(LocalDate serviceStartDate) {
this.serviceStartDate = serviceStartDate;
}
public UpdateInvoiceItem sku(String sku) {
this.sku = sku;
return this;
}
/**
* The SKU of the invoice item. The SKU of the invoice item must be different from the SKU of any existing product.
* @return sku
*/
@javax.annotation.Nullable
public String getSku() {
return sku;
}
public void setSku(String sku) {
this.sku = sku;
}
public UpdateInvoiceItem taxCode(String taxCode) {
this.taxCode = taxCode;
return this;
}
/**
* The tax code identifies which tax rules and tax rates to apply to the invoice item. **Note:** - This field is only available if you have Taxation enabled. - If the values of both `taxCode` and `taxMode` fields are changed to `null` when updating a standalone invoice, the corresponding `invoiceItems` > `taxItems` field and its nested fields specified in the creation request will be removed.
* @return taxCode
*/
@javax.annotation.Nullable
public String getTaxCode() {
return taxCode;
}
public void setTaxCode(String taxCode) {
this.taxCode = taxCode;
}
public UpdateInvoiceItem taxItems(List taxItems) {
this.taxItems = taxItems;
return this;
}
public UpdateInvoiceItem addTaxItemsItem(CreateTaxationItem taxItemsItem) {
if (this.taxItems == null) {
this.taxItems = new ArrayList<>();
}
this.taxItems.add(taxItemsItem);
return this;
}
/**
* Container for taxation items. The maximum number of taxation items is 5. **Note**: This field is only available only if id is null and you have Taxation enabled.
* @return taxItems
*/
@javax.annotation.Nullable
public List getTaxItems() {
return taxItems;
}
public void setTaxItems(List taxItems) {
this.taxItems = taxItems;
}
public UpdateInvoiceItem taxMode(TaxMode taxMode) {
this.taxMode = taxMode;
return this;
}
/**
* Get taxMode
* @return taxMode
*/
@javax.annotation.Nullable
public TaxMode getTaxMode() {
return taxMode;
}
public void setTaxMode(TaxMode taxMode) {
this.taxMode = taxMode;
}
public UpdateInvoiceItem unbilledReceivablesAccountingCode(String unbilledReceivablesAccountingCode) {
this.unbilledReceivablesAccountingCode = unbilledReceivablesAccountingCode;
return this;
}
/**
* The accounting code for unbilled receivables. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return unbilledReceivablesAccountingCode
*/
@javax.annotation.Nullable
public String getUnbilledReceivablesAccountingCode() {
return unbilledReceivablesAccountingCode;
}
public void setUnbilledReceivablesAccountingCode(String unbilledReceivablesAccountingCode) {
this.unbilledReceivablesAccountingCode = unbilledReceivablesAccountingCode;
}
public UpdateInvoiceItem unitPrice(BigDecimal unitPrice) {
this.unitPrice = unitPrice;
return this;
}
/**
* The per-unit price of the invoice item.
* @return unitPrice
*/
@javax.annotation.Nullable
public BigDecimal getUnitPrice() {
return unitPrice;
}
public void setUnitPrice(BigDecimal unitPrice) {
this.unitPrice = unitPrice;
}
public UpdateInvoiceItem uom(String uom) {
this.uom = uom;
return this;
}
/**
* The unit of measure.
* @return uom
*/
@javax.annotation.Nullable
public String getUom() {
return uom;
}
public void setUom(String uom) {
this.uom = uom;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the UpdateInvoiceItem instance itself
*/
public UpdateInvoiceItem putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UpdateInvoiceItem updateInvoiceItem = (UpdateInvoiceItem) o;
return Objects.equals(this.integrationIdNS, updateInvoiceItem.integrationIdNS) &&
Objects.equals(this.integrationStatusNS, updateInvoiceItem.integrationStatusNS) &&
Objects.equals(this.syncDateNS, updateInvoiceItem.syncDateNS) &&
Objects.equals(this.accountingCode, updateInvoiceItem.accountingCode) &&
Objects.equals(this.adjustmentLiabilityAccountingCode, updateInvoiceItem.adjustmentLiabilityAccountingCode) &&
Objects.equals(this.adjustmentRevenueAccountingCode, updateInvoiceItem.adjustmentRevenueAccountingCode) &&
Objects.equals(this.amount, updateInvoiceItem.amount) &&
Objects.equals(this.bookingReference, updateInvoiceItem.bookingReference) &&
Objects.equals(this.chargeDate, updateInvoiceItem.chargeDate) &&
Objects.equals(this.chargeName, updateInvoiceItem.chargeName) &&
Objects.equals(this.contractAssetAccountingCode, updateInvoiceItem.contractAssetAccountingCode) &&
Objects.equals(this.contractLiabilityAccountingCode, updateInvoiceItem.contractLiabilityAccountingCode) &&
Objects.equals(this.contractRecognizedRevenueAccountingCode, updateInvoiceItem.contractRecognizedRevenueAccountingCode) &&
Objects.equals(this.deferredRevenueAccountingCode, updateInvoiceItem.deferredRevenueAccountingCode) &&
Objects.equals(this.delete, updateInvoiceItem.delete) &&
Objects.equals(this.description, updateInvoiceItem.description) &&
Objects.equals(this.discountItems, updateInvoiceItem.discountItems) &&
Objects.equals(this.excludeItemBillingFromRevenueAccounting, updateInvoiceItem.excludeItemBillingFromRevenueAccounting) &&
Objects.equals(this.id, updateInvoiceItem.id) &&
Objects.equals(this.itemType, updateInvoiceItem.itemType) &&
Objects.equals(this.productRatePlanChargeId, updateInvoiceItem.productRatePlanChargeId) &&
Objects.equals(this.purchaseOrderNumber, updateInvoiceItem.purchaseOrderNumber) &&
Objects.equals(this.quantity, updateInvoiceItem.quantity) &&
Objects.equals(this.recognizedRevenueAccountingCode, updateInvoiceItem.recognizedRevenueAccountingCode) &&
Objects.equals(this.revRecCode, updateInvoiceItem.revRecCode) &&
Objects.equals(this.revRecTriggerCondition, updateInvoiceItem.revRecTriggerCondition) &&
Objects.equals(this.revenueRecognitionRuleName, updateInvoiceItem.revenueRecognitionRuleName) &&
Objects.equals(this.serviceEndDate, updateInvoiceItem.serviceEndDate) &&
Objects.equals(this.serviceStartDate, updateInvoiceItem.serviceStartDate) &&
Objects.equals(this.sku, updateInvoiceItem.sku) &&
Objects.equals(this.taxCode, updateInvoiceItem.taxCode) &&
Objects.equals(this.taxItems, updateInvoiceItem.taxItems) &&
Objects.equals(this.taxMode, updateInvoiceItem.taxMode) &&
Objects.equals(this.unbilledReceivablesAccountingCode, updateInvoiceItem.unbilledReceivablesAccountingCode) &&
Objects.equals(this.unitPrice, updateInvoiceItem.unitPrice) &&
Objects.equals(this.uom, updateInvoiceItem.uom)&&
Objects.equals(this.additionalProperties, updateInvoiceItem.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(integrationIdNS, integrationStatusNS, syncDateNS, accountingCode, adjustmentLiabilityAccountingCode, adjustmentRevenueAccountingCode, amount, bookingReference, chargeDate, chargeName, contractAssetAccountingCode, contractLiabilityAccountingCode, contractRecognizedRevenueAccountingCode, deferredRevenueAccountingCode, delete, description, discountItems, excludeItemBillingFromRevenueAccounting, id, itemType, productRatePlanChargeId, purchaseOrderNumber, quantity, recognizedRevenueAccountingCode, revRecCode, revRecTriggerCondition, revenueRecognitionRuleName, serviceEndDate, serviceStartDate, sku, taxCode, taxItems, taxMode, unbilledReceivablesAccountingCode, unitPrice, uom, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UpdateInvoiceItem {\n");
sb.append(" integrationIdNS: ").append(toIndentedString(integrationIdNS)).append("\n");
sb.append(" integrationStatusNS: ").append(toIndentedString(integrationStatusNS)).append("\n");
sb.append(" syncDateNS: ").append(toIndentedString(syncDateNS)).append("\n");
sb.append(" accountingCode: ").append(toIndentedString(accountingCode)).append("\n");
sb.append(" adjustmentLiabilityAccountingCode: ").append(toIndentedString(adjustmentLiabilityAccountingCode)).append("\n");
sb.append(" adjustmentRevenueAccountingCode: ").append(toIndentedString(adjustmentRevenueAccountingCode)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" bookingReference: ").append(toIndentedString(bookingReference)).append("\n");
sb.append(" chargeDate: ").append(toIndentedString(chargeDate)).append("\n");
sb.append(" chargeName: ").append(toIndentedString(chargeName)).append("\n");
sb.append(" contractAssetAccountingCode: ").append(toIndentedString(contractAssetAccountingCode)).append("\n");
sb.append(" contractLiabilityAccountingCode: ").append(toIndentedString(contractLiabilityAccountingCode)).append("\n");
sb.append(" contractRecognizedRevenueAccountingCode: ").append(toIndentedString(contractRecognizedRevenueAccountingCode)).append("\n");
sb.append(" deferredRevenueAccountingCode: ").append(toIndentedString(deferredRevenueAccountingCode)).append("\n");
sb.append(" delete: ").append(toIndentedString(delete)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" discountItems: ").append(toIndentedString(discountItems)).append("\n");
sb.append(" excludeItemBillingFromRevenueAccounting: ").append(toIndentedString(excludeItemBillingFromRevenueAccounting)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" itemType: ").append(toIndentedString(itemType)).append("\n");
sb.append(" productRatePlanChargeId: ").append(toIndentedString(productRatePlanChargeId)).append("\n");
sb.append(" purchaseOrderNumber: ").append(toIndentedString(purchaseOrderNumber)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" recognizedRevenueAccountingCode: ").append(toIndentedString(recognizedRevenueAccountingCode)).append("\n");
sb.append(" revRecCode: ").append(toIndentedString(revRecCode)).append("\n");
sb.append(" revRecTriggerCondition: ").append(toIndentedString(revRecTriggerCondition)).append("\n");
sb.append(" revenueRecognitionRuleName: ").append(toIndentedString(revenueRecognitionRuleName)).append("\n");
sb.append(" serviceEndDate: ").append(toIndentedString(serviceEndDate)).append("\n");
sb.append(" serviceStartDate: ").append(toIndentedString(serviceStartDate)).append("\n");
sb.append(" sku: ").append(toIndentedString(sku)).append("\n");
sb.append(" taxCode: ").append(toIndentedString(taxCode)).append("\n");
sb.append(" taxItems: ").append(toIndentedString(taxItems)).append("\n");
sb.append(" taxMode: ").append(toIndentedString(taxMode)).append("\n");
sb.append(" unbilledReceivablesAccountingCode: ").append(toIndentedString(unbilledReceivablesAccountingCode)).append("\n");
sb.append(" unitPrice: ").append(toIndentedString(unitPrice)).append("\n");
sb.append(" uom: ").append(toIndentedString(uom)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("IntegrationId__NS");
openapiFields.add("IntegrationStatus__NS");
openapiFields.add("SyncDate__NS");
openapiFields.add("accountingCode");
openapiFields.add("adjustmentLiabilityAccountingCode");
openapiFields.add("adjustmentRevenueAccountingCode");
openapiFields.add("amount");
openapiFields.add("bookingReference");
openapiFields.add("chargeDate");
openapiFields.add("chargeName");
openapiFields.add("contractAssetAccountingCode");
openapiFields.add("contractLiabilityAccountingCode");
openapiFields.add("contractRecognizedRevenueAccountingCode");
openapiFields.add("deferredRevenueAccountingCode");
openapiFields.add("delete");
openapiFields.add("description");
openapiFields.add("discountItems");
openapiFields.add("excludeItemBillingFromRevenueAccounting");
openapiFields.add("id");
openapiFields.add("itemType");
openapiFields.add("productRatePlanChargeId");
openapiFields.add("purchaseOrderNumber");
openapiFields.add("quantity");
openapiFields.add("recognizedRevenueAccountingCode");
openapiFields.add("revRecCode");
openapiFields.add("revRecTriggerCondition");
openapiFields.add("revenueRecognitionRuleName");
openapiFields.add("serviceEndDate");
openapiFields.add("serviceStartDate");
openapiFields.add("sku");
openapiFields.add("taxCode");
openapiFields.add("taxItems");
openapiFields.add("taxMode");
openapiFields.add("unbilledReceivablesAccountingCode");
openapiFields.add("unitPrice");
openapiFields.add("uom");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to UpdateInvoiceItem
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!UpdateInvoiceItem.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in UpdateInvoiceItem is not found in the empty JSON string", UpdateInvoiceItem.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("IntegrationId__NS") != null && !jsonObj.get("IntegrationId__NS").isJsonNull()) && !jsonObj.get("IntegrationId__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationId__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationId__NS").toString()));
}
if ((jsonObj.get("IntegrationStatus__NS") != null && !jsonObj.get("IntegrationStatus__NS").isJsonNull()) && !jsonObj.get("IntegrationStatus__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationStatus__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationStatus__NS").toString()));
}
if ((jsonObj.get("SyncDate__NS") != null && !jsonObj.get("SyncDate__NS").isJsonNull()) && !jsonObj.get("SyncDate__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SyncDate__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SyncDate__NS").toString()));
}
if ((jsonObj.get("accountingCode") != null && !jsonObj.get("accountingCode").isJsonNull()) && !jsonObj.get("accountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountingCode").toString()));
}
if ((jsonObj.get("adjustmentLiabilityAccountingCode") != null && !jsonObj.get("adjustmentLiabilityAccountingCode").isJsonNull()) && !jsonObj.get("adjustmentLiabilityAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `adjustmentLiabilityAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("adjustmentLiabilityAccountingCode").toString()));
}
if ((jsonObj.get("adjustmentRevenueAccountingCode") != null && !jsonObj.get("adjustmentRevenueAccountingCode").isJsonNull()) && !jsonObj.get("adjustmentRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `adjustmentRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("adjustmentRevenueAccountingCode").toString()));
}
if ((jsonObj.get("bookingReference") != null && !jsonObj.get("bookingReference").isJsonNull()) && !jsonObj.get("bookingReference").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bookingReference` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bookingReference").toString()));
}
if ((jsonObj.get("chargeDate") != null && !jsonObj.get("chargeDate").isJsonNull()) && !jsonObj.get("chargeDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeDate").toString()));
}
if ((jsonObj.get("chargeName") != null && !jsonObj.get("chargeName").isJsonNull()) && !jsonObj.get("chargeName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeName").toString()));
}
if ((jsonObj.get("contractAssetAccountingCode") != null && !jsonObj.get("contractAssetAccountingCode").isJsonNull()) && !jsonObj.get("contractAssetAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractAssetAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractAssetAccountingCode").toString()));
}
if ((jsonObj.get("contractLiabilityAccountingCode") != null && !jsonObj.get("contractLiabilityAccountingCode").isJsonNull()) && !jsonObj.get("contractLiabilityAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractLiabilityAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractLiabilityAccountingCode").toString()));
}
if ((jsonObj.get("contractRecognizedRevenueAccountingCode") != null && !jsonObj.get("contractRecognizedRevenueAccountingCode").isJsonNull()) && !jsonObj.get("contractRecognizedRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractRecognizedRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractRecognizedRevenueAccountingCode").toString()));
}
if ((jsonObj.get("deferredRevenueAccountingCode") != null && !jsonObj.get("deferredRevenueAccountingCode").isJsonNull()) && !jsonObj.get("deferredRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `deferredRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("deferredRevenueAccountingCode").toString()));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if (jsonObj.get("discountItems") != null && !jsonObj.get("discountItems").isJsonNull()) {
JsonArray jsonArraydiscountItems = jsonObj.getAsJsonArray("discountItems");
if (jsonArraydiscountItems != null) {
// ensure the json data is an array
if (!jsonObj.get("discountItems").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `discountItems` to be an array in the JSON string but got `%s`", jsonObj.get("discountItems").toString()));
}
// validate the optional field `discountItems` (array)
for (int i = 0; i < jsonArraydiscountItems.size(); i++) {
UpdateDiscountInvoiceItem.validateJsonElement(jsonArraydiscountItems.get(i));
};
}
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("itemType") != null && !jsonObj.get("itemType").isJsonNull()) && !jsonObj.get("itemType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `itemType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("itemType").toString()));
}
if ((jsonObj.get("productRatePlanChargeId") != null && !jsonObj.get("productRatePlanChargeId").isJsonNull()) && !jsonObj.get("productRatePlanChargeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanChargeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productRatePlanChargeId").toString()));
}
if ((jsonObj.get("purchaseOrderNumber") != null && !jsonObj.get("purchaseOrderNumber").isJsonNull()) && !jsonObj.get("purchaseOrderNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `purchaseOrderNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("purchaseOrderNumber").toString()));
}
if ((jsonObj.get("recognizedRevenueAccountingCode") != null && !jsonObj.get("recognizedRevenueAccountingCode").isJsonNull()) && !jsonObj.get("recognizedRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `recognizedRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("recognizedRevenueAccountingCode").toString()));
}
if ((jsonObj.get("revRecCode") != null && !jsonObj.get("revRecCode").isJsonNull()) && !jsonObj.get("revRecCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revRecCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revRecCode").toString()));
}
if ((jsonObj.get("revRecTriggerCondition") != null && !jsonObj.get("revRecTriggerCondition").isJsonNull()) && !jsonObj.get("revRecTriggerCondition").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revRecTriggerCondition` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revRecTriggerCondition").toString()));
}
// validate the optional field `revRecTriggerCondition`
if (jsonObj.get("revRecTriggerCondition") != null && !jsonObj.get("revRecTriggerCondition").isJsonNull()) {
RevRecTrigger.validateJsonElement(jsonObj.get("revRecTriggerCondition"));
}
if ((jsonObj.get("revenueRecognitionRuleName") != null && !jsonObj.get("revenueRecognitionRuleName").isJsonNull()) && !jsonObj.get("revenueRecognitionRuleName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueRecognitionRuleName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueRecognitionRuleName").toString()));
}
if ((jsonObj.get("sku") != null && !jsonObj.get("sku").isJsonNull()) && !jsonObj.get("sku").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sku` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sku").toString()));
}
if ((jsonObj.get("taxCode") != null && !jsonObj.get("taxCode").isJsonNull()) && !jsonObj.get("taxCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxCode").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("taxItems") != null && !jsonObj.get("taxItems").isJsonNull() && !jsonObj.get("taxItems").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `taxItems` to be an array in the JSON string but got `%s`", jsonObj.get("taxItems").toString()));
}
if ((jsonObj.get("taxMode") != null && !jsonObj.get("taxMode").isJsonNull()) && !jsonObj.get("taxMode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxMode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxMode").toString()));
}
// validate the optional field `taxMode`
if (jsonObj.get("taxMode") != null && !jsonObj.get("taxMode").isJsonNull()) {
TaxMode.validateJsonElement(jsonObj.get("taxMode"));
}
if ((jsonObj.get("unbilledReceivablesAccountingCode") != null && !jsonObj.get("unbilledReceivablesAccountingCode").isJsonNull()) && !jsonObj.get("unbilledReceivablesAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `unbilledReceivablesAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("unbilledReceivablesAccountingCode").toString()));
}
if ((jsonObj.get("uom") != null && !jsonObj.get("uom").isJsonNull()) && !jsonObj.get("uom").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `uom` to be a primitive type in the JSON string but got `%s`", jsonObj.get("uom").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!UpdateInvoiceItem.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'UpdateInvoiceItem' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(UpdateInvoiceItem.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, UpdateInvoiceItem value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public UpdateInvoiceItem read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
UpdateInvoiceItem instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of UpdateInvoiceItem given an JSON string
*
* @param jsonString JSON string
* @return An instance of UpdateInvoiceItem
* @throws IOException if the JSON string is invalid with respect to UpdateInvoiceItem
*/
public static UpdateInvoiceItem fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, UpdateInvoiceItem.class);
}
/**
* Convert an instance of UpdateInvoiceItem to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy