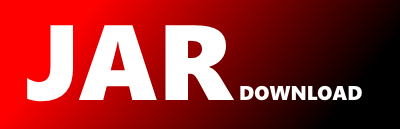
com.zuora.model.UpdateOrderLineItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.OrderLineItemBillingRule;
import com.zuora.model.OrderLineItemInlineDiscountType;
import com.zuora.model.OrderLineItemState;
import com.zuora.model.OrderLineItemType;
import com.zuora.model.TaxMode;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* UpdateOrderLineItem
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class UpdateOrderLineItem {
public static final String SERIALIZED_NAME_U_O_M = "UOM";
@SerializedName(SERIALIZED_NAME_U_O_M)
private String UOM;
public static final String SERIALIZED_NAME_ACCOUNTING_CODE = "accountingCode";
@SerializedName(SERIALIZED_NAME_ACCOUNTING_CODE)
private String accountingCode;
public static final String SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNTING_CODE = "adjustmentLiabilityAccountingCode";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNTING_CODE)
private String adjustmentLiabilityAccountingCode;
public static final String SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNTING_CODE = "adjustmentRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNTING_CODE)
private String adjustmentRevenueAccountingCode;
public static final String SERIALIZED_NAME_AMOUNT_PER_UNIT = "amountPerUnit";
@SerializedName(SERIALIZED_NAME_AMOUNT_PER_UNIT)
private BigDecimal amountPerUnit;
public static final String SERIALIZED_NAME_BILL_TARGET_DATE = "billTargetDate";
@SerializedName(SERIALIZED_NAME_BILL_TARGET_DATE)
private LocalDate billTargetDate;
public static final String SERIALIZED_NAME_BILL_TO = "billTo";
@SerializedName(SERIALIZED_NAME_BILL_TO)
private String billTo;
public static final String SERIALIZED_NAME_BILLING_RULE = "billingRule";
@SerializedName(SERIALIZED_NAME_BILLING_RULE)
private OrderLineItemBillingRule billingRule = OrderLineItemBillingRule.TRIGGERWITHOUTFULFILLMENT;
public static final String SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNTING_CODE = "contractAssetAccountingCode";
@SerializedName(SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNTING_CODE)
private String contractAssetAccountingCode;
public static final String SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNTING_CODE = "contractLiabilityAccountingCode";
@SerializedName(SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNTING_CODE)
private String contractLiabilityAccountingCode;
public static final String SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNTING_CODE = "contractRecognizedRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNTING_CODE)
private String contractRecognizedRevenueAccountingCode;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "customFields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
private Map customFields;
public static final String SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE = "deferredRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE)
private String deferredRevenueAccountingCode;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_INLINE_DISCOUNT_PER_UNIT = "inlineDiscountPerUnit";
@SerializedName(SERIALIZED_NAME_INLINE_DISCOUNT_PER_UNIT)
private BigDecimal inlineDiscountPerUnit;
public static final String SERIALIZED_NAME_INLINE_DISCOUNT_TYPE = "inlineDiscountType";
@SerializedName(SERIALIZED_NAME_INLINE_DISCOUNT_TYPE)
private OrderLineItemInlineDiscountType inlineDiscountType;
public static final String SERIALIZED_NAME_INVOICE_GROUP_NUMBER = "invoiceGroupNumber";
@SerializedName(SERIALIZED_NAME_INVOICE_GROUP_NUMBER)
private String invoiceGroupNumber;
public static final String SERIALIZED_NAME_IS_ALLOCATION_ELIGIBLE = "isAllocationEligible";
@SerializedName(SERIALIZED_NAME_IS_ALLOCATION_ELIGIBLE)
private Boolean isAllocationEligible;
public static final String SERIALIZED_NAME_IS_UNBILLED = "isUnbilled";
@SerializedName(SERIALIZED_NAME_IS_UNBILLED)
private Boolean isUnbilled;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING = "excludeItemBillingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBillingFromRevenueAccounting;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING = "excludeItemBookingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBookingFromRevenueAccounting;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_TIMING = "revenueRecognitionTiming";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_TIMING)
private String revenueRecognitionTiming;
public static final String SERIALIZED_NAME_REVENUE_AMORTIZATION_METHOD = "revenueAmortizationMethod";
@SerializedName(SERIALIZED_NAME_REVENUE_AMORTIZATION_METHOD)
private String revenueAmortizationMethod;
public static final String SERIALIZED_NAME_ITEM_NAME = "itemName";
@SerializedName(SERIALIZED_NAME_ITEM_NAME)
private String itemName;
public static final String SERIALIZED_NAME_ITEM_NUMBER = "itemNumber";
@SerializedName(SERIALIZED_NAME_ITEM_NUMBER)
private String itemNumber;
public static final String SERIALIZED_NAME_ITEM_STATE = "itemState";
@SerializedName(SERIALIZED_NAME_ITEM_STATE)
private OrderLineItemState itemState;
public static final String SERIALIZED_NAME_ITEM_TYPE = "itemType";
@SerializedName(SERIALIZED_NAME_ITEM_TYPE)
private OrderLineItemType itemType;
public static final String SERIALIZED_NAME_LIST_PRICE_PER_UNIT = "listPricePerUnit";
@SerializedName(SERIALIZED_NAME_LIST_PRICE_PER_UNIT)
private BigDecimal listPricePerUnit;
public static final String SERIALIZED_NAME_OWNER_ACCOUNT_NUMBER = "ownerAccountNumber";
@SerializedName(SERIALIZED_NAME_OWNER_ACCOUNT_NUMBER)
private String ownerAccountNumber;
public static final String SERIALIZED_NAME_PRODUCT_CODE = "productCode";
@SerializedName(SERIALIZED_NAME_PRODUCT_CODE)
private String productCode;
public static final String SERIALIZED_NAME_PURCHASE_ORDER_NUMBER = "purchaseOrderNumber";
@SerializedName(SERIALIZED_NAME_PURCHASE_ORDER_NUMBER)
private String purchaseOrderNumber;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE = "recognizedRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE)
private String recognizedRevenueAccountingCode;
public static final String SERIALIZED_NAME_RELATED_SUBSCRIPTION_NUMBER = "relatedSubscriptionNumber";
@SerializedName(SERIALIZED_NAME_RELATED_SUBSCRIPTION_NUMBER)
private String relatedSubscriptionNumber;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_RULE = "revenueRecognitionRule";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_RULE)
private String revenueRecognitionRule;
public static final String SERIALIZED_NAME_SEQUENCE_SET_ID = "sequenceSetId";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_ID)
private String sequenceSetId;
public static final String SERIALIZED_NAME_PAYMENT_TERM = "paymentTerm";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERM)
private String paymentTerm;
public static final String SERIALIZED_NAME_INVOICE_TEMPLATE_ID = "invoiceTemplateId";
@SerializedName(SERIALIZED_NAME_INVOICE_TEMPLATE_ID)
private String invoiceTemplateId;
public static final String SERIALIZED_NAME_SOLD_TO = "soldTo";
@SerializedName(SERIALIZED_NAME_SOLD_TO)
private String soldTo;
public static final String SERIALIZED_NAME_SHIP_TO = "shipTo";
@SerializedName(SERIALIZED_NAME_SHIP_TO)
private String shipTo;
public static final String SERIALIZED_NAME_TAX_CODE = "taxCode";
@SerializedName(SERIALIZED_NAME_TAX_CODE)
private String taxCode;
public static final String SERIALIZED_NAME_TAX_MODE = "taxMode";
@SerializedName(SERIALIZED_NAME_TAX_MODE)
private TaxMode taxMode;
public static final String SERIALIZED_NAME_TRANSACTION_END_DATE = "transactionEndDate";
@SerializedName(SERIALIZED_NAME_TRANSACTION_END_DATE)
private LocalDate transactionEndDate;
public static final String SERIALIZED_NAME_TRANSACTION_START_DATE = "transactionStartDate";
@SerializedName(SERIALIZED_NAME_TRANSACTION_START_DATE)
private LocalDate transactionStartDate;
public static final String SERIALIZED_NAME_UNBILLED_RECEIVABLES_ACCOUNTING_CODE = "unbilledReceivablesAccountingCode";
@SerializedName(SERIALIZED_NAME_UNBILLED_RECEIVABLES_ACCOUNTING_CODE)
private String unbilledReceivablesAccountingCode;
public UpdateOrderLineItem() {
}
public UpdateOrderLineItem UOM(String UOM) {
this.UOM = UOM;
return this;
}
/**
* Specifies the units to measure usage. You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return UOM
*/
@javax.annotation.Nullable
public String getUOM() {
return UOM;
}
public void setUOM(String UOM) {
this.UOM = UOM;
}
public UpdateOrderLineItem accountingCode(String accountingCode) {
this.accountingCode = accountingCode;
return this;
}
/**
* The accountingCode for the Order Line Item (OLI). You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return accountingCode
*/
@javax.annotation.Nullable
public String getAccountingCode() {
return accountingCode;
}
public void setAccountingCode(String accountingCode) {
this.accountingCode = accountingCode;
}
public UpdateOrderLineItem adjustmentLiabilityAccountingCode(String adjustmentLiabilityAccountingCode) {
this.adjustmentLiabilityAccountingCode = adjustmentLiabilityAccountingCode;
return this;
}
/**
* The accounting code on the Order Line Item object for customers using [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration). You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return adjustmentLiabilityAccountingCode
*/
@javax.annotation.Nullable
public String getAdjustmentLiabilityAccountingCode() {
return adjustmentLiabilityAccountingCode;
}
public void setAdjustmentLiabilityAccountingCode(String adjustmentLiabilityAccountingCode) {
this.adjustmentLiabilityAccountingCode = adjustmentLiabilityAccountingCode;
}
public UpdateOrderLineItem adjustmentRevenueAccountingCode(String adjustmentRevenueAccountingCode) {
this.adjustmentRevenueAccountingCode = adjustmentRevenueAccountingCode;
return this;
}
/**
* The accounting code on the Order Line Item object for customers using [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration). You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return adjustmentRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getAdjustmentRevenueAccountingCode() {
return adjustmentRevenueAccountingCode;
}
public void setAdjustmentRevenueAccountingCode(String adjustmentRevenueAccountingCode) {
this.adjustmentRevenueAccountingCode = adjustmentRevenueAccountingCode;
}
public UpdateOrderLineItem amountPerUnit(BigDecimal amountPerUnit) {
this.amountPerUnit = amountPerUnit;
return this;
}
/**
* The actual charged amount per unit for the Order Line Item (OLI). You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return amountPerUnit
*/
@javax.annotation.Nullable
public BigDecimal getAmountPerUnit() {
return amountPerUnit;
}
public void setAmountPerUnit(BigDecimal amountPerUnit) {
this.amountPerUnit = amountPerUnit;
}
public UpdateOrderLineItem billTargetDate(LocalDate billTargetDate) {
this.billTargetDate = billTargetDate;
return this;
}
/**
* The target date for the Order Line Item (OLI) to be picked up by bill run for generating billing documents. To generate billing documents for an OLI, you must set this field and set the `itemState` field to `SentToBilling`. You can update this field for a sales or return OLI only when the OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return billTargetDate
*/
@javax.annotation.Nullable
public LocalDate getBillTargetDate() {
return billTargetDate;
}
public void setBillTargetDate(LocalDate billTargetDate) {
this.billTargetDate = billTargetDate;
}
public UpdateOrderLineItem billTo(String billTo) {
this.billTo = billTo;
return this;
}
/**
* The ID of a contact that belongs to the billing account of the order line item. Use this field to assign an existing account as the bill-to contact of an order line item. You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return billTo
*/
@javax.annotation.Nullable
public String getBillTo() {
return billTo;
}
public void setBillTo(String billTo) {
this.billTo = billTo;
}
public UpdateOrderLineItem billingRule(OrderLineItemBillingRule billingRule) {
this.billingRule = billingRule;
return this;
}
/**
* Get billingRule
* @return billingRule
*/
@javax.annotation.Nullable
public OrderLineItemBillingRule getBillingRule() {
return billingRule;
}
public void setBillingRule(OrderLineItemBillingRule billingRule) {
this.billingRule = billingRule;
}
public UpdateOrderLineItem contractAssetAccountingCode(String contractAssetAccountingCode) {
this.contractAssetAccountingCode = contractAssetAccountingCode;
return this;
}
/**
* The accounting code on the Order Line Item object for customers using [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration). You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return contractAssetAccountingCode
*/
@javax.annotation.Nullable
public String getContractAssetAccountingCode() {
return contractAssetAccountingCode;
}
public void setContractAssetAccountingCode(String contractAssetAccountingCode) {
this.contractAssetAccountingCode = contractAssetAccountingCode;
}
public UpdateOrderLineItem contractLiabilityAccountingCode(String contractLiabilityAccountingCode) {
this.contractLiabilityAccountingCode = contractLiabilityAccountingCode;
return this;
}
/**
* The accounting code on the Order Line Item object for customers using [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration). You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return contractLiabilityAccountingCode
*/
@javax.annotation.Nullable
public String getContractLiabilityAccountingCode() {
return contractLiabilityAccountingCode;
}
public void setContractLiabilityAccountingCode(String contractLiabilityAccountingCode) {
this.contractLiabilityAccountingCode = contractLiabilityAccountingCode;
}
public UpdateOrderLineItem contractRecognizedRevenueAccountingCode(String contractRecognizedRevenueAccountingCode) {
this.contractRecognizedRevenueAccountingCode = contractRecognizedRevenueAccountingCode;
return this;
}
/**
* The accounting code on the Order Line Item object for customers using [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration). You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return contractRecognizedRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getContractRecognizedRevenueAccountingCode() {
return contractRecognizedRevenueAccountingCode;
}
public void setContractRecognizedRevenueAccountingCode(String contractRecognizedRevenueAccountingCode) {
this.contractRecognizedRevenueAccountingCode = contractRecognizedRevenueAccountingCode;
}
public UpdateOrderLineItem customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public UpdateOrderLineItem putCustomFieldsItem(String key, Object customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap<>();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Container for custom fields of an Order Line Item object.
* @return customFields
*/
@javax.annotation.Nullable
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public UpdateOrderLineItem deferredRevenueAccountingCode(String deferredRevenueAccountingCode) {
this.deferredRevenueAccountingCode = deferredRevenueAccountingCode;
return this;
}
/**
* The deferred revenue accounting code for the Order Line Item (OLI). You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return deferredRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getDeferredRevenueAccountingCode() {
return deferredRevenueAccountingCode;
}
public void setDeferredRevenueAccountingCode(String deferredRevenueAccountingCode) {
this.deferredRevenueAccountingCode = deferredRevenueAccountingCode;
}
public UpdateOrderLineItem description(String description) {
this.description = description;
return this;
}
/**
* The description of the Order Line Item (OLI). You can update this field for a sales or return OLI only when the OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public UpdateOrderLineItem inlineDiscountPerUnit(BigDecimal inlineDiscountPerUnit) {
this.inlineDiscountPerUnit = inlineDiscountPerUnit;
return this;
}
/**
* You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`). Use this field in accordance with the `inlineDiscountType` field, in the following manner: * If the `inlineDiscountType` field is set as `Percentage`, this field specifies the discount percentage for each unit of the order line item. For exmaple, if you specify `5` in this field, the discount percentage is 5%. * If the `inlineDiscountType` field is set as `FixedAmount`, this field specifies the discount amount on each unit of the order line item. For exmaple, if you specify `10` in this field, the discount amount on each unit of the order line item is 10. Once you set the `inlineDiscountType`, `inlineDiscountPerUnit`, and `listPricePerUnit` fields, the system will automatically generate the `amountPerUnit` field. You shall not set the `amountPerUnit` field by yourself.
* @return inlineDiscountPerUnit
*/
@javax.annotation.Nullable
public BigDecimal getInlineDiscountPerUnit() {
return inlineDiscountPerUnit;
}
public void setInlineDiscountPerUnit(BigDecimal inlineDiscountPerUnit) {
this.inlineDiscountPerUnit = inlineDiscountPerUnit;
}
public UpdateOrderLineItem inlineDiscountType(OrderLineItemInlineDiscountType inlineDiscountType) {
this.inlineDiscountType = inlineDiscountType;
return this;
}
/**
* Get inlineDiscountType
* @return inlineDiscountType
*/
@javax.annotation.Nullable
public OrderLineItemInlineDiscountType getInlineDiscountType() {
return inlineDiscountType;
}
public void setInlineDiscountType(OrderLineItemInlineDiscountType inlineDiscountType) {
this.inlineDiscountType = inlineDiscountType;
}
public UpdateOrderLineItem invoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
return this;
}
/**
* The invoice group number associated with the order line item. You can update this field only for a sales OLI and only when the sales OLI is in the `Executing`/`Booked`/`SentToBilling` state (when the `itemState` field is set as `Executing`/`Booked`/`SentToBilling`).
* @return invoiceGroupNumber
*/
@javax.annotation.Nullable
public String getInvoiceGroupNumber() {
return invoiceGroupNumber;
}
public void setInvoiceGroupNumber(String invoiceGroupNumber) {
this.invoiceGroupNumber = invoiceGroupNumber;
}
public UpdateOrderLineItem isAllocationEligible(Boolean isAllocationEligible) {
this.isAllocationEligible = isAllocationEligible;
return this;
}
/**
* This field is used to identify if the charge segment is allocation eligible in revenue recognition. **Note**: This feature is in the **Early Adopter** phase. If you want to use the feature, submit a request at <a href=\"https://support.zuora.com/\" target=\"_blank\">Zuora Global Support</a>, and we will evaluate whether the feature is suitable for your use cases.
* @return isAllocationEligible
*/
@javax.annotation.Nullable
public Boolean getIsAllocationEligible() {
return isAllocationEligible;
}
public void setIsAllocationEligible(Boolean isAllocationEligible) {
this.isAllocationEligible = isAllocationEligible;
}
public UpdateOrderLineItem isUnbilled(Boolean isUnbilled) {
this.isUnbilled = isUnbilled;
return this;
}
/**
* This field is used to dictate how to perform the accounting during revenue recognition. **Note**: This feature is in the **Early Adopter** phase. If you want to use the feature, submit a request at <a href=\"https://support.zuora.com/\" target=\"_blank\">Zuora Global Support</a>, and we will evaluate whether the feature is suitable for your use cases.
* @return isUnbilled
*/
@javax.annotation.Nullable
public Boolean getIsUnbilled() {
return isUnbilled;
}
public void setIsUnbilled(Boolean isUnbilled) {
this.isUnbilled = isUnbilled;
}
public UpdateOrderLineItem excludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
return this;
}
/**
* The flag to exclude Order Line Item related invoice items, invoice item adjustments, credit memo items, and debit memo items from revenue accounting. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return excludeItemBillingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBillingFromRevenueAccounting() {
return excludeItemBillingFromRevenueAccounting;
}
public void setExcludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
}
public UpdateOrderLineItem excludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
return this;
}
/**
* The flag to exclude Order Line Item from revenue accounting. **Note**: This field is only available if you have the Billing - Revenue Integration feature enabled.
* @return excludeItemBookingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBookingFromRevenueAccounting() {
return excludeItemBookingFromRevenueAccounting;
}
public void setExcludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
}
public UpdateOrderLineItem revenueRecognitionTiming(String revenueRecognitionTiming) {
this.revenueRecognitionTiming = revenueRecognitionTiming;
return this;
}
/**
* This field is used to dictate the type of revenue recognition timing.
* @return revenueRecognitionTiming
*/
@javax.annotation.Nullable
public String getRevenueRecognitionTiming() {
return revenueRecognitionTiming;
}
public void setRevenueRecognitionTiming(String revenueRecognitionTiming) {
this.revenueRecognitionTiming = revenueRecognitionTiming;
}
public UpdateOrderLineItem revenueAmortizationMethod(String revenueAmortizationMethod) {
this.revenueAmortizationMethod = revenueAmortizationMethod;
return this;
}
/**
* This field is used to dictate the type of revenue amortization method.
* @return revenueAmortizationMethod
*/
@javax.annotation.Nullable
public String getRevenueAmortizationMethod() {
return revenueAmortizationMethod;
}
public void setRevenueAmortizationMethod(String revenueAmortizationMethod) {
this.revenueAmortizationMethod = revenueAmortizationMethod;
}
public UpdateOrderLineItem itemName(String itemName) {
this.itemName = itemName;
return this;
}
/**
* The name of the Order Line Item (OLI). You can update this field for a sales or return OLI only when the OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return itemName
*/
@javax.annotation.Nullable
public String getItemName() {
return itemName;
}
public void setItemName(String itemName) {
this.itemName = itemName;
}
public UpdateOrderLineItem itemNumber(String itemNumber) {
this.itemNumber = itemNumber;
return this;
}
/**
* The number for the Order Line Item (OLI). You can update this field for a sales or return OLI only when the OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return itemNumber
*/
@javax.annotation.Nullable
public String getItemNumber() {
return itemNumber;
}
public void setItemNumber(String itemNumber) {
this.itemNumber = itemNumber;
}
public UpdateOrderLineItem itemState(OrderLineItemState itemState) {
this.itemState = itemState;
return this;
}
/**
* Get itemState
* @return itemState
*/
@javax.annotation.Nullable
public OrderLineItemState getItemState() {
return itemState;
}
public void setItemState(OrderLineItemState itemState) {
this.itemState = itemState;
}
public UpdateOrderLineItem itemType(OrderLineItemType itemType) {
this.itemType = itemType;
return this;
}
/**
* Get itemType
* @return itemType
*/
@javax.annotation.Nullable
public OrderLineItemType getItemType() {
return itemType;
}
public void setItemType(OrderLineItemType itemType) {
this.itemType = itemType;
}
public UpdateOrderLineItem listPricePerUnit(BigDecimal listPricePerUnit) {
this.listPricePerUnit = listPricePerUnit;
return this;
}
/**
* The list price per unit for the Order Line Item (OLI). You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return listPricePerUnit
*/
@javax.annotation.Nullable
public BigDecimal getListPricePerUnit() {
return listPricePerUnit;
}
public void setListPricePerUnit(BigDecimal listPricePerUnit) {
this.listPricePerUnit = listPricePerUnit;
}
public UpdateOrderLineItem ownerAccountNumber(String ownerAccountNumber) {
this.ownerAccountNumber = ownerAccountNumber;
return this;
}
/**
* Use this field to assign an existing account as the owner of an order line item. You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return ownerAccountNumber
*/
@javax.annotation.Nullable
public String getOwnerAccountNumber() {
return ownerAccountNumber;
}
public void setOwnerAccountNumber(String ownerAccountNumber) {
this.ownerAccountNumber = ownerAccountNumber;
}
public UpdateOrderLineItem productCode(String productCode) {
this.productCode = productCode;
return this;
}
/**
* The product code for the Order Line Item (OLI). You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return productCode
*/
@javax.annotation.Nullable
public String getProductCode() {
return productCode;
}
public void setProductCode(String productCode) {
this.productCode = productCode;
}
public UpdateOrderLineItem purchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
return this;
}
/**
* Used by customers to specify the Purchase Order Number provided by the buyer. You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return purchaseOrderNumber
*/
@javax.annotation.Nullable
public String getPurchaseOrderNumber() {
return purchaseOrderNumber;
}
public void setPurchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
}
public UpdateOrderLineItem quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* The quantity of units, such as the number of authors in a hosted wiki service. You can update this field for a sales or return OLI only when the OLI in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return quantity
*/
@javax.annotation.Nullable
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public UpdateOrderLineItem recognizedRevenueAccountingCode(String recognizedRevenueAccountingCode) {
this.recognizedRevenueAccountingCode = recognizedRevenueAccountingCode;
return this;
}
/**
* The recognized revenue accounting code for the Order Line Item (OLI). You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return recognizedRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getRecognizedRevenueAccountingCode() {
return recognizedRevenueAccountingCode;
}
public void setRecognizedRevenueAccountingCode(String recognizedRevenueAccountingCode) {
this.recognizedRevenueAccountingCode = recognizedRevenueAccountingCode;
}
public UpdateOrderLineItem relatedSubscriptionNumber(String relatedSubscriptionNumber) {
this.relatedSubscriptionNumber = relatedSubscriptionNumber;
return this;
}
/**
* Use this field to relate an order line item to an subscription. Specify this field to the subscription number of the subscription to relate. You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return relatedSubscriptionNumber
*/
@javax.annotation.Nullable
public String getRelatedSubscriptionNumber() {
return relatedSubscriptionNumber;
}
public void setRelatedSubscriptionNumber(String relatedSubscriptionNumber) {
this.relatedSubscriptionNumber = relatedSubscriptionNumber;
}
public UpdateOrderLineItem revenueRecognitionRule(String revenueRecognitionRule) {
this.revenueRecognitionRule = revenueRecognitionRule;
return this;
}
/**
* The Revenue Recognition rule for the Order Line Item (OLI). You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return revenueRecognitionRule
*/
@javax.annotation.Nullable
public String getRevenueRecognitionRule() {
return revenueRecognitionRule;
}
public void setRevenueRecognitionRule(String revenueRecognitionRule) {
this.revenueRecognitionRule = revenueRecognitionRule;
}
public UpdateOrderLineItem sequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
return this;
}
/**
* The ID of the sequence set associated with the orderLineItem. You can update this field only for a sales OLI and only when the sales OLI is in the `Executing`/`Booked`/`SentToBilling` state (when the `itemState` field is set as `Executing`/`Booked`/`SentToBilling`).
* @return sequenceSetId
*/
@javax.annotation.Nullable
public String getSequenceSetId() {
return sequenceSetId;
}
public void setSequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
}
public UpdateOrderLineItem paymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
return this;
}
/**
* The payment term name associated with the orderLineItem. You can update this field only for a sales OLI and only when the sales OLI is in the `Executing`/`Booked`/`SentToBilling` state (when the `itemState` field is set as `Executing`/`Booked`/`SentToBilling`).
* @return paymentTerm
*/
@javax.annotation.Nullable
public String getPaymentTerm() {
return paymentTerm;
}
public void setPaymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
}
public UpdateOrderLineItem invoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
return this;
}
/**
* The ID of the invoice template associated with the orderLineItem. You can update this field only for a sales OLI and only when the sales OLI is in the `Executing`/`Booked`/`SentToBilling` state (when the `itemState` field is set as `Executing`/`Booked`/`SentToBilling`).
* @return invoiceTemplateId
*/
@javax.annotation.Nullable
public String getInvoiceTemplateId() {
return invoiceTemplateId;
}
public void setInvoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
}
public UpdateOrderLineItem soldTo(String soldTo) {
this.soldTo = soldTo;
return this;
}
/**
* Use this field to assign an existing account as the sold-to contact of an order line item, by the following rules: * If the `ownerAccountNumber` field is set, then this field must be the ID of a contact that belongs to the owner account of the order line item. * If the `ownerAccountNumber` field is not set, then this field must be the ID of a contact that belongs to the billing account of the order line item. You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return soldTo
*/
@javax.annotation.Nullable
public String getSoldTo() {
return soldTo;
}
public void setSoldTo(String soldTo) {
this.soldTo = soldTo;
}
public UpdateOrderLineItem shipTo(String shipTo) {
this.shipTo = shipTo;
return this;
}
/**
* Use this field to assign an existing account as the ship-to contact of an order line item, by the following rules: * If the `ownerAccountNumber` field is set, then this field must be the ID of a contact that belongs to the owner account of the order line item. * If the `ownerAccountNumber` field is not set, then this field must be the ID of a contact that belongs to the billing account of the order line item. You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return shipTo
*/
@javax.annotation.Nullable
public String getShipTo() {
return shipTo;
}
public void setShipTo(String shipTo) {
this.shipTo = shipTo;
}
public UpdateOrderLineItem taxCode(String taxCode) {
this.taxCode = taxCode;
return this;
}
/**
* The tax code for the Order Line Item (OLI). You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return taxCode
*/
@javax.annotation.Nullable
public String getTaxCode() {
return taxCode;
}
public void setTaxCode(String taxCode) {
this.taxCode = taxCode;
}
public UpdateOrderLineItem taxMode(TaxMode taxMode) {
this.taxMode = taxMode;
return this;
}
/**
* Get taxMode
* @return taxMode
*/
@javax.annotation.Nullable
public TaxMode getTaxMode() {
return taxMode;
}
public void setTaxMode(TaxMode taxMode) {
this.taxMode = taxMode;
}
public UpdateOrderLineItem transactionEndDate(LocalDate transactionEndDate) {
this.transactionEndDate = transactionEndDate;
return this;
}
/**
* The date a transaction is completed. The default value of this field is the transaction start date. Also, the value of this field should always equal or be later than the value of the `transactionStartDate` field. You can update this field for a sales or return OLI only when the OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return transactionEndDate
*/
@javax.annotation.Nullable
public LocalDate getTransactionEndDate() {
return transactionEndDate;
}
public void setTransactionEndDate(LocalDate transactionEndDate) {
this.transactionEndDate = transactionEndDate;
}
public UpdateOrderLineItem transactionStartDate(LocalDate transactionStartDate) {
this.transactionStartDate = transactionStartDate;
return this;
}
/**
* The date a transaction starts. The default value of this field is the order date. You can update this field for a sales or return OLI only when the OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return transactionStartDate
*/
@javax.annotation.Nullable
public LocalDate getTransactionStartDate() {
return transactionStartDate;
}
public void setTransactionStartDate(LocalDate transactionStartDate) {
this.transactionStartDate = transactionStartDate;
}
public UpdateOrderLineItem unbilledReceivablesAccountingCode(String unbilledReceivablesAccountingCode) {
this.unbilledReceivablesAccountingCode = unbilledReceivablesAccountingCode;
return this;
}
/**
* The accounting code on the Order Line Item object for customers using [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration). You can update this field only for a sales OLI and only when the sales OLI is in the `Executing` state (when the `itemState` field is set as `Executing`).
* @return unbilledReceivablesAccountingCode
*/
@javax.annotation.Nullable
public String getUnbilledReceivablesAccountingCode() {
return unbilledReceivablesAccountingCode;
}
public void setUnbilledReceivablesAccountingCode(String unbilledReceivablesAccountingCode) {
this.unbilledReceivablesAccountingCode = unbilledReceivablesAccountingCode;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the UpdateOrderLineItem instance itself
*/
public UpdateOrderLineItem putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UpdateOrderLineItem updateOrderLineItem = (UpdateOrderLineItem) o;
return Objects.equals(this.UOM, updateOrderLineItem.UOM) &&
Objects.equals(this.accountingCode, updateOrderLineItem.accountingCode) &&
Objects.equals(this.adjustmentLiabilityAccountingCode, updateOrderLineItem.adjustmentLiabilityAccountingCode) &&
Objects.equals(this.adjustmentRevenueAccountingCode, updateOrderLineItem.adjustmentRevenueAccountingCode) &&
Objects.equals(this.amountPerUnit, updateOrderLineItem.amountPerUnit) &&
Objects.equals(this.billTargetDate, updateOrderLineItem.billTargetDate) &&
Objects.equals(this.billTo, updateOrderLineItem.billTo) &&
Objects.equals(this.billingRule, updateOrderLineItem.billingRule) &&
Objects.equals(this.contractAssetAccountingCode, updateOrderLineItem.contractAssetAccountingCode) &&
Objects.equals(this.contractLiabilityAccountingCode, updateOrderLineItem.contractLiabilityAccountingCode) &&
Objects.equals(this.contractRecognizedRevenueAccountingCode, updateOrderLineItem.contractRecognizedRevenueAccountingCode) &&
Objects.equals(this.customFields, updateOrderLineItem.customFields) &&
Objects.equals(this.deferredRevenueAccountingCode, updateOrderLineItem.deferredRevenueAccountingCode) &&
Objects.equals(this.description, updateOrderLineItem.description) &&
Objects.equals(this.inlineDiscountPerUnit, updateOrderLineItem.inlineDiscountPerUnit) &&
Objects.equals(this.inlineDiscountType, updateOrderLineItem.inlineDiscountType) &&
Objects.equals(this.invoiceGroupNumber, updateOrderLineItem.invoiceGroupNumber) &&
Objects.equals(this.isAllocationEligible, updateOrderLineItem.isAllocationEligible) &&
Objects.equals(this.isUnbilled, updateOrderLineItem.isUnbilled) &&
Objects.equals(this.excludeItemBillingFromRevenueAccounting, updateOrderLineItem.excludeItemBillingFromRevenueAccounting) &&
Objects.equals(this.excludeItemBookingFromRevenueAccounting, updateOrderLineItem.excludeItemBookingFromRevenueAccounting) &&
Objects.equals(this.revenueRecognitionTiming, updateOrderLineItem.revenueRecognitionTiming) &&
Objects.equals(this.revenueAmortizationMethod, updateOrderLineItem.revenueAmortizationMethod) &&
Objects.equals(this.itemName, updateOrderLineItem.itemName) &&
Objects.equals(this.itemNumber, updateOrderLineItem.itemNumber) &&
Objects.equals(this.itemState, updateOrderLineItem.itemState) &&
Objects.equals(this.itemType, updateOrderLineItem.itemType) &&
Objects.equals(this.listPricePerUnit, updateOrderLineItem.listPricePerUnit) &&
Objects.equals(this.ownerAccountNumber, updateOrderLineItem.ownerAccountNumber) &&
Objects.equals(this.productCode, updateOrderLineItem.productCode) &&
Objects.equals(this.purchaseOrderNumber, updateOrderLineItem.purchaseOrderNumber) &&
Objects.equals(this.quantity, updateOrderLineItem.quantity) &&
Objects.equals(this.recognizedRevenueAccountingCode, updateOrderLineItem.recognizedRevenueAccountingCode) &&
Objects.equals(this.relatedSubscriptionNumber, updateOrderLineItem.relatedSubscriptionNumber) &&
Objects.equals(this.revenueRecognitionRule, updateOrderLineItem.revenueRecognitionRule) &&
Objects.equals(this.sequenceSetId, updateOrderLineItem.sequenceSetId) &&
Objects.equals(this.paymentTerm, updateOrderLineItem.paymentTerm) &&
Objects.equals(this.invoiceTemplateId, updateOrderLineItem.invoiceTemplateId) &&
Objects.equals(this.soldTo, updateOrderLineItem.soldTo) &&
Objects.equals(this.shipTo, updateOrderLineItem.shipTo) &&
Objects.equals(this.taxCode, updateOrderLineItem.taxCode) &&
Objects.equals(this.taxMode, updateOrderLineItem.taxMode) &&
Objects.equals(this.transactionEndDate, updateOrderLineItem.transactionEndDate) &&
Objects.equals(this.transactionStartDate, updateOrderLineItem.transactionStartDate) &&
Objects.equals(this.unbilledReceivablesAccountingCode, updateOrderLineItem.unbilledReceivablesAccountingCode)&&
Objects.equals(this.additionalProperties, updateOrderLineItem.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(UOM, accountingCode, adjustmentLiabilityAccountingCode, adjustmentRevenueAccountingCode, amountPerUnit, billTargetDate, billTo, billingRule, contractAssetAccountingCode, contractLiabilityAccountingCode, contractRecognizedRevenueAccountingCode, customFields, deferredRevenueAccountingCode, description, inlineDiscountPerUnit, inlineDiscountType, invoiceGroupNumber, isAllocationEligible, isUnbilled, excludeItemBillingFromRevenueAccounting, excludeItemBookingFromRevenueAccounting, revenueRecognitionTiming, revenueAmortizationMethod, itemName, itemNumber, itemState, itemType, listPricePerUnit, ownerAccountNumber, productCode, purchaseOrderNumber, quantity, recognizedRevenueAccountingCode, relatedSubscriptionNumber, revenueRecognitionRule, sequenceSetId, paymentTerm, invoiceTemplateId, soldTo, shipTo, taxCode, taxMode, transactionEndDate, transactionStartDate, unbilledReceivablesAccountingCode, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UpdateOrderLineItem {\n");
sb.append(" UOM: ").append(toIndentedString(UOM)).append("\n");
sb.append(" accountingCode: ").append(toIndentedString(accountingCode)).append("\n");
sb.append(" adjustmentLiabilityAccountingCode: ").append(toIndentedString(adjustmentLiabilityAccountingCode)).append("\n");
sb.append(" adjustmentRevenueAccountingCode: ").append(toIndentedString(adjustmentRevenueAccountingCode)).append("\n");
sb.append(" amountPerUnit: ").append(toIndentedString(amountPerUnit)).append("\n");
sb.append(" billTargetDate: ").append(toIndentedString(billTargetDate)).append("\n");
sb.append(" billTo: ").append(toIndentedString(billTo)).append("\n");
sb.append(" billingRule: ").append(toIndentedString(billingRule)).append("\n");
sb.append(" contractAssetAccountingCode: ").append(toIndentedString(contractAssetAccountingCode)).append("\n");
sb.append(" contractLiabilityAccountingCode: ").append(toIndentedString(contractLiabilityAccountingCode)).append("\n");
sb.append(" contractRecognizedRevenueAccountingCode: ").append(toIndentedString(contractRecognizedRevenueAccountingCode)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" deferredRevenueAccountingCode: ").append(toIndentedString(deferredRevenueAccountingCode)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" inlineDiscountPerUnit: ").append(toIndentedString(inlineDiscountPerUnit)).append("\n");
sb.append(" inlineDiscountType: ").append(toIndentedString(inlineDiscountType)).append("\n");
sb.append(" invoiceGroupNumber: ").append(toIndentedString(invoiceGroupNumber)).append("\n");
sb.append(" isAllocationEligible: ").append(toIndentedString(isAllocationEligible)).append("\n");
sb.append(" isUnbilled: ").append(toIndentedString(isUnbilled)).append("\n");
sb.append(" excludeItemBillingFromRevenueAccounting: ").append(toIndentedString(excludeItemBillingFromRevenueAccounting)).append("\n");
sb.append(" excludeItemBookingFromRevenueAccounting: ").append(toIndentedString(excludeItemBookingFromRevenueAccounting)).append("\n");
sb.append(" revenueRecognitionTiming: ").append(toIndentedString(revenueRecognitionTiming)).append("\n");
sb.append(" revenueAmortizationMethod: ").append(toIndentedString(revenueAmortizationMethod)).append("\n");
sb.append(" itemName: ").append(toIndentedString(itemName)).append("\n");
sb.append(" itemNumber: ").append(toIndentedString(itemNumber)).append("\n");
sb.append(" itemState: ").append(toIndentedString(itemState)).append("\n");
sb.append(" itemType: ").append(toIndentedString(itemType)).append("\n");
sb.append(" listPricePerUnit: ").append(toIndentedString(listPricePerUnit)).append("\n");
sb.append(" ownerAccountNumber: ").append(toIndentedString(ownerAccountNumber)).append("\n");
sb.append(" productCode: ").append(toIndentedString(productCode)).append("\n");
sb.append(" purchaseOrderNumber: ").append(toIndentedString(purchaseOrderNumber)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" recognizedRevenueAccountingCode: ").append(toIndentedString(recognizedRevenueAccountingCode)).append("\n");
sb.append(" relatedSubscriptionNumber: ").append(toIndentedString(relatedSubscriptionNumber)).append("\n");
sb.append(" revenueRecognitionRule: ").append(toIndentedString(revenueRecognitionRule)).append("\n");
sb.append(" sequenceSetId: ").append(toIndentedString(sequenceSetId)).append("\n");
sb.append(" paymentTerm: ").append(toIndentedString(paymentTerm)).append("\n");
sb.append(" invoiceTemplateId: ").append(toIndentedString(invoiceTemplateId)).append("\n");
sb.append(" soldTo: ").append(toIndentedString(soldTo)).append("\n");
sb.append(" shipTo: ").append(toIndentedString(shipTo)).append("\n");
sb.append(" taxCode: ").append(toIndentedString(taxCode)).append("\n");
sb.append(" taxMode: ").append(toIndentedString(taxMode)).append("\n");
sb.append(" transactionEndDate: ").append(toIndentedString(transactionEndDate)).append("\n");
sb.append(" transactionStartDate: ").append(toIndentedString(transactionStartDate)).append("\n");
sb.append(" unbilledReceivablesAccountingCode: ").append(toIndentedString(unbilledReceivablesAccountingCode)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("UOM");
openapiFields.add("accountingCode");
openapiFields.add("adjustmentLiabilityAccountingCode");
openapiFields.add("adjustmentRevenueAccountingCode");
openapiFields.add("amountPerUnit");
openapiFields.add("billTargetDate");
openapiFields.add("billTo");
openapiFields.add("billingRule");
openapiFields.add("contractAssetAccountingCode");
openapiFields.add("contractLiabilityAccountingCode");
openapiFields.add("contractRecognizedRevenueAccountingCode");
openapiFields.add("customFields");
openapiFields.add("deferredRevenueAccountingCode");
openapiFields.add("description");
openapiFields.add("inlineDiscountPerUnit");
openapiFields.add("inlineDiscountType");
openapiFields.add("invoiceGroupNumber");
openapiFields.add("isAllocationEligible");
openapiFields.add("isUnbilled");
openapiFields.add("excludeItemBillingFromRevenueAccounting");
openapiFields.add("excludeItemBookingFromRevenueAccounting");
openapiFields.add("revenueRecognitionTiming");
openapiFields.add("revenueAmortizationMethod");
openapiFields.add("itemName");
openapiFields.add("itemNumber");
openapiFields.add("itemState");
openapiFields.add("itemType");
openapiFields.add("listPricePerUnit");
openapiFields.add("ownerAccountNumber");
openapiFields.add("productCode");
openapiFields.add("purchaseOrderNumber");
openapiFields.add("quantity");
openapiFields.add("recognizedRevenueAccountingCode");
openapiFields.add("relatedSubscriptionNumber");
openapiFields.add("revenueRecognitionRule");
openapiFields.add("sequenceSetId");
openapiFields.add("paymentTerm");
openapiFields.add("invoiceTemplateId");
openapiFields.add("soldTo");
openapiFields.add("shipTo");
openapiFields.add("taxCode");
openapiFields.add("taxMode");
openapiFields.add("transactionEndDate");
openapiFields.add("transactionStartDate");
openapiFields.add("unbilledReceivablesAccountingCode");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to UpdateOrderLineItem
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!UpdateOrderLineItem.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in UpdateOrderLineItem is not found in the empty JSON string", UpdateOrderLineItem.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("UOM") != null && !jsonObj.get("UOM").isJsonNull()) && !jsonObj.get("UOM").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `UOM` to be a primitive type in the JSON string but got `%s`", jsonObj.get("UOM").toString()));
}
if ((jsonObj.get("accountingCode") != null && !jsonObj.get("accountingCode").isJsonNull()) && !jsonObj.get("accountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountingCode").toString()));
}
if ((jsonObj.get("adjustmentLiabilityAccountingCode") != null && !jsonObj.get("adjustmentLiabilityAccountingCode").isJsonNull()) && !jsonObj.get("adjustmentLiabilityAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `adjustmentLiabilityAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("adjustmentLiabilityAccountingCode").toString()));
}
if ((jsonObj.get("adjustmentRevenueAccountingCode") != null && !jsonObj.get("adjustmentRevenueAccountingCode").isJsonNull()) && !jsonObj.get("adjustmentRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `adjustmentRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("adjustmentRevenueAccountingCode").toString()));
}
if ((jsonObj.get("billTo") != null && !jsonObj.get("billTo").isJsonNull()) && !jsonObj.get("billTo").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billTo` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billTo").toString()));
}
// validate the optional field `billingRule`
if (jsonObj.get("billingRule") != null && !jsonObj.get("billingRule").isJsonNull()) {
OrderLineItemBillingRule.validateJsonElement(jsonObj.get("billingRule"));
}
if ((jsonObj.get("contractAssetAccountingCode") != null && !jsonObj.get("contractAssetAccountingCode").isJsonNull()) && !jsonObj.get("contractAssetAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractAssetAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractAssetAccountingCode").toString()));
}
if ((jsonObj.get("contractLiabilityAccountingCode") != null && !jsonObj.get("contractLiabilityAccountingCode").isJsonNull()) && !jsonObj.get("contractLiabilityAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractLiabilityAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractLiabilityAccountingCode").toString()));
}
if ((jsonObj.get("contractRecognizedRevenueAccountingCode") != null && !jsonObj.get("contractRecognizedRevenueAccountingCode").isJsonNull()) && !jsonObj.get("contractRecognizedRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractRecognizedRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractRecognizedRevenueAccountingCode").toString()));
}
if ((jsonObj.get("deferredRevenueAccountingCode") != null && !jsonObj.get("deferredRevenueAccountingCode").isJsonNull()) && !jsonObj.get("deferredRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `deferredRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("deferredRevenueAccountingCode").toString()));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
// validate the optional field `inlineDiscountType`
if (jsonObj.get("inlineDiscountType") != null && !jsonObj.get("inlineDiscountType").isJsonNull()) {
OrderLineItemInlineDiscountType.validateJsonElement(jsonObj.get("inlineDiscountType"));
}
if ((jsonObj.get("invoiceGroupNumber") != null && !jsonObj.get("invoiceGroupNumber").isJsonNull()) && !jsonObj.get("invoiceGroupNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceGroupNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceGroupNumber").toString()));
}
if ((jsonObj.get("revenueRecognitionTiming") != null && !jsonObj.get("revenueRecognitionTiming").isJsonNull()) && !jsonObj.get("revenueRecognitionTiming").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueRecognitionTiming` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueRecognitionTiming").toString()));
}
if ((jsonObj.get("revenueAmortizationMethod") != null && !jsonObj.get("revenueAmortizationMethod").isJsonNull()) && !jsonObj.get("revenueAmortizationMethod").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueAmortizationMethod` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueAmortizationMethod").toString()));
}
if ((jsonObj.get("itemName") != null && !jsonObj.get("itemName").isJsonNull()) && !jsonObj.get("itemName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `itemName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("itemName").toString()));
}
if ((jsonObj.get("itemNumber") != null && !jsonObj.get("itemNumber").isJsonNull()) && !jsonObj.get("itemNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `itemNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("itemNumber").toString()));
}
// validate the optional field `itemState`
if (jsonObj.get("itemState") != null && !jsonObj.get("itemState").isJsonNull()) {
OrderLineItemState.validateJsonElement(jsonObj.get("itemState"));
}
// validate the optional field `itemType`
if (jsonObj.get("itemType") != null && !jsonObj.get("itemType").isJsonNull()) {
OrderLineItemType.validateJsonElement(jsonObj.get("itemType"));
}
if ((jsonObj.get("ownerAccountNumber") != null && !jsonObj.get("ownerAccountNumber").isJsonNull()) && !jsonObj.get("ownerAccountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ownerAccountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ownerAccountNumber").toString()));
}
if ((jsonObj.get("productCode") != null && !jsonObj.get("productCode").isJsonNull()) && !jsonObj.get("productCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productCode").toString()));
}
if ((jsonObj.get("purchaseOrderNumber") != null && !jsonObj.get("purchaseOrderNumber").isJsonNull()) && !jsonObj.get("purchaseOrderNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `purchaseOrderNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("purchaseOrderNumber").toString()));
}
if ((jsonObj.get("recognizedRevenueAccountingCode") != null && !jsonObj.get("recognizedRevenueAccountingCode").isJsonNull()) && !jsonObj.get("recognizedRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `recognizedRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("recognizedRevenueAccountingCode").toString()));
}
if ((jsonObj.get("relatedSubscriptionNumber") != null && !jsonObj.get("relatedSubscriptionNumber").isJsonNull()) && !jsonObj.get("relatedSubscriptionNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `relatedSubscriptionNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("relatedSubscriptionNumber").toString()));
}
if ((jsonObj.get("revenueRecognitionRule") != null && !jsonObj.get("revenueRecognitionRule").isJsonNull()) && !jsonObj.get("revenueRecognitionRule").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueRecognitionRule` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueRecognitionRule").toString()));
}
if ((jsonObj.get("sequenceSetId") != null && !jsonObj.get("sequenceSetId").isJsonNull()) && !jsonObj.get("sequenceSetId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sequenceSetId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sequenceSetId").toString()));
}
if ((jsonObj.get("paymentTerm") != null && !jsonObj.get("paymentTerm").isJsonNull()) && !jsonObj.get("paymentTerm").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentTerm` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentTerm").toString()));
}
if ((jsonObj.get("invoiceTemplateId") != null && !jsonObj.get("invoiceTemplateId").isJsonNull()) && !jsonObj.get("invoiceTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceTemplateId").toString()));
}
if ((jsonObj.get("soldTo") != null && !jsonObj.get("soldTo").isJsonNull()) && !jsonObj.get("soldTo").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `soldTo` to be a primitive type in the JSON string but got `%s`", jsonObj.get("soldTo").toString()));
}
if ((jsonObj.get("shipTo") != null && !jsonObj.get("shipTo").isJsonNull()) && !jsonObj.get("shipTo").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `shipTo` to be a primitive type in the JSON string but got `%s`", jsonObj.get("shipTo").toString()));
}
if ((jsonObj.get("taxCode") != null && !jsonObj.get("taxCode").isJsonNull()) && !jsonObj.get("taxCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxCode").toString()));
}
// validate the optional field `taxMode`
if (jsonObj.get("taxMode") != null && !jsonObj.get("taxMode").isJsonNull()) {
TaxMode.validateJsonElement(jsonObj.get("taxMode"));
}
if ((jsonObj.get("unbilledReceivablesAccountingCode") != null && !jsonObj.get("unbilledReceivablesAccountingCode").isJsonNull()) && !jsonObj.get("unbilledReceivablesAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `unbilledReceivablesAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("unbilledReceivablesAccountingCode").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!UpdateOrderLineItem.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'UpdateOrderLineItem' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(UpdateOrderLineItem.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, UpdateOrderLineItem value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public UpdateOrderLineItem read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
UpdateOrderLineItem instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of UpdateOrderLineItem given an JSON string
*
* @param jsonString JSON string
* @return An instance of UpdateOrderLineItem
* @throws IOException if the JSON string is invalid with respect to UpdateOrderLineItem
*/
public static UpdateOrderLineItem fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, UpdateOrderLineItem.class);
}
/**
* Convert an instance of UpdateOrderLineItem to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy