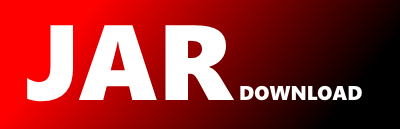
com.zuora.model.UpdateProductRatePlanRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.ProductRatePlanObjectNSFieldsBillingPeriodNS;
import com.zuora.model.ProductRatePlanObjectNSFieldsIncludeChildrenNS;
import com.zuora.model.ProductRatePlanObjectNSFieldsItemTypeNS;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* UpdateProductRatePlanRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class UpdateProductRatePlanRequest {
public static final String SERIALIZED_NAME_BILLING_PERIOD_N_S = "BillingPeriod__NS";
@SerializedName(SERIALIZED_NAME_BILLING_PERIOD_N_S)
private ProductRatePlanObjectNSFieldsBillingPeriodNS billingPeriodNS;
public static final String SERIALIZED_NAME_CLASS_N_S = "Class__NS";
@SerializedName(SERIALIZED_NAME_CLASS_N_S)
private String classNS;
public static final String SERIALIZED_NAME_DEPARTMENT_N_S = "Department__NS";
@SerializedName(SERIALIZED_NAME_DEPARTMENT_N_S)
private String departmentNS;
public static final String SERIALIZED_NAME_INCLUDE_CHILDREN_N_S = "IncludeChildren__NS";
@SerializedName(SERIALIZED_NAME_INCLUDE_CHILDREN_N_S)
private ProductRatePlanObjectNSFieldsIncludeChildrenNS includeChildrenNS;
public static final String SERIALIZED_NAME_INTEGRATION_ID_N_S = "IntegrationId__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_ID_N_S)
private String integrationIdNS;
public static final String SERIALIZED_NAME_INTEGRATION_STATUS_N_S = "IntegrationStatus__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_STATUS_N_S)
private String integrationStatusNS;
public static final String SERIALIZED_NAME_ITEM_TYPE_N_S = "ItemType__NS";
@SerializedName(SERIALIZED_NAME_ITEM_TYPE_N_S)
private ProductRatePlanObjectNSFieldsItemTypeNS itemTypeNS;
public static final String SERIALIZED_NAME_LOCATION_N_S = "Location__NS";
@SerializedName(SERIALIZED_NAME_LOCATION_N_S)
private String locationNS;
public static final String SERIALIZED_NAME_MULTI_CURRENCY_PRICE_N_S = "MultiCurrencyPrice__NS";
@SerializedName(SERIALIZED_NAME_MULTI_CURRENCY_PRICE_N_S)
private String multiCurrencyPriceNS;
public static final String SERIALIZED_NAME_PRICE_N_S = "Price__NS";
@SerializedName(SERIALIZED_NAME_PRICE_N_S)
private String priceNS;
public static final String SERIALIZED_NAME_SUBSIDIARY_N_S = "Subsidiary__NS";
@SerializedName(SERIALIZED_NAME_SUBSIDIARY_N_S)
private String subsidiaryNS;
public static final String SERIALIZED_NAME_SYNC_DATE_N_S = "SyncDate__NS";
@SerializedName(SERIALIZED_NAME_SYNC_DATE_N_S)
private String syncDateNS;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_NUMBER = "ProductRatePlanNumber";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_NUMBER)
private String productRatePlanNumber;
public static final String SERIALIZED_NAME_NAME = "Name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_PRODUCT_ID = "ProductId";
@SerializedName(SERIALIZED_NAME_PRODUCT_ID)
private String productId;
public static final String SERIALIZED_NAME_ACTIVE_CURRENCIES = "ActiveCurrencies";
@SerializedName(SERIALIZED_NAME_ACTIVE_CURRENCIES)
private List activeCurrencies;
public static final String SERIALIZED_NAME_DESCRIPTION = "Description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_EFFECTIVE_START_DATE = "EffectiveStartDate";
@SerializedName(SERIALIZED_NAME_EFFECTIVE_START_DATE)
private LocalDate effectiveStartDate;
public static final String SERIALIZED_NAME_EFFECTIVE_END_DATE = "EffectiveEndDate";
@SerializedName(SERIALIZED_NAME_EFFECTIVE_END_DATE)
private LocalDate effectiveEndDate;
public static final String SERIALIZED_NAME_GRADE = "Grade";
@SerializedName(SERIALIZED_NAME_GRADE)
private BigDecimal grade;
public UpdateProductRatePlanRequest() {
}
public UpdateProductRatePlanRequest billingPeriodNS(ProductRatePlanObjectNSFieldsBillingPeriodNS billingPeriodNS) {
this.billingPeriodNS = billingPeriodNS;
return this;
}
/**
* Get billingPeriodNS
* @return billingPeriodNS
*/
@javax.annotation.Nullable
public ProductRatePlanObjectNSFieldsBillingPeriodNS getBillingPeriodNS() {
return billingPeriodNS;
}
public void setBillingPeriodNS(ProductRatePlanObjectNSFieldsBillingPeriodNS billingPeriodNS) {
this.billingPeriodNS = billingPeriodNS;
}
public UpdateProductRatePlanRequest classNS(String classNS) {
this.classNS = classNS;
return this;
}
/**
* Class associated with the corresponding item in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return classNS
*/
@javax.annotation.Nullable
public String getClassNS() {
return classNS;
}
public void setClassNS(String classNS) {
this.classNS = classNS;
}
public UpdateProductRatePlanRequest departmentNS(String departmentNS) {
this.departmentNS = departmentNS;
return this;
}
/**
* Department associated with the corresponding item in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return departmentNS
*/
@javax.annotation.Nullable
public String getDepartmentNS() {
return departmentNS;
}
public void setDepartmentNS(String departmentNS) {
this.departmentNS = departmentNS;
}
public UpdateProductRatePlanRequest includeChildrenNS(ProductRatePlanObjectNSFieldsIncludeChildrenNS includeChildrenNS) {
this.includeChildrenNS = includeChildrenNS;
return this;
}
/**
* Get includeChildrenNS
* @return includeChildrenNS
*/
@javax.annotation.Nullable
public ProductRatePlanObjectNSFieldsIncludeChildrenNS getIncludeChildrenNS() {
return includeChildrenNS;
}
public void setIncludeChildrenNS(ProductRatePlanObjectNSFieldsIncludeChildrenNS includeChildrenNS) {
this.includeChildrenNS = includeChildrenNS;
}
public UpdateProductRatePlanRequest integrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
return this;
}
/**
* ID of the corresponding object in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationIdNS
*/
@javax.annotation.Nullable
public String getIntegrationIdNS() {
return integrationIdNS;
}
public void setIntegrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
}
public UpdateProductRatePlanRequest integrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
return this;
}
/**
* Status of the product rate plan's synchronization with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationStatusNS
*/
@javax.annotation.Nullable
public String getIntegrationStatusNS() {
return integrationStatusNS;
}
public void setIntegrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
}
public UpdateProductRatePlanRequest itemTypeNS(ProductRatePlanObjectNSFieldsItemTypeNS itemTypeNS) {
this.itemTypeNS = itemTypeNS;
return this;
}
/**
* Get itemTypeNS
* @return itemTypeNS
*/
@javax.annotation.Nullable
public ProductRatePlanObjectNSFieldsItemTypeNS getItemTypeNS() {
return itemTypeNS;
}
public void setItemTypeNS(ProductRatePlanObjectNSFieldsItemTypeNS itemTypeNS) {
this.itemTypeNS = itemTypeNS;
}
public UpdateProductRatePlanRequest locationNS(String locationNS) {
this.locationNS = locationNS;
return this;
}
/**
* Location associated with the corresponding item in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return locationNS
*/
@javax.annotation.Nullable
public String getLocationNS() {
return locationNS;
}
public void setLocationNS(String locationNS) {
this.locationNS = locationNS;
}
public UpdateProductRatePlanRequest multiCurrencyPriceNS(String multiCurrencyPriceNS) {
this.multiCurrencyPriceNS = multiCurrencyPriceNS;
return this;
}
/**
* Multi-currency price associated with the corresponding item in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return multiCurrencyPriceNS
*/
@javax.annotation.Nullable
public String getMultiCurrencyPriceNS() {
return multiCurrencyPriceNS;
}
public void setMultiCurrencyPriceNS(String multiCurrencyPriceNS) {
this.multiCurrencyPriceNS = multiCurrencyPriceNS;
}
public UpdateProductRatePlanRequest priceNS(String priceNS) {
this.priceNS = priceNS;
return this;
}
/**
* Price associated with the corresponding item in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return priceNS
*/
@javax.annotation.Nullable
public String getPriceNS() {
return priceNS;
}
public void setPriceNS(String priceNS) {
this.priceNS = priceNS;
}
public UpdateProductRatePlanRequest subsidiaryNS(String subsidiaryNS) {
this.subsidiaryNS = subsidiaryNS;
return this;
}
/**
* Subsidiary associated with the corresponding item in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return subsidiaryNS
*/
@javax.annotation.Nullable
public String getSubsidiaryNS() {
return subsidiaryNS;
}
public void setSubsidiaryNS(String subsidiaryNS) {
this.subsidiaryNS = subsidiaryNS;
}
public UpdateProductRatePlanRequest syncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
return this;
}
/**
* Date when the product rate plan was synchronized with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return syncDateNS
*/
@javax.annotation.Nullable
public String getSyncDateNS() {
return syncDateNS;
}
public void setSyncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
}
public UpdateProductRatePlanRequest productRatePlanNumber(String productRatePlanNumber) {
this.productRatePlanNumber = productRatePlanNumber;
return this;
}
/**
* The natural key of the product rate plan. For existing Product Rate Plan objects that are created before this field is introduced, this field will be null. Use this field to specify a value for only these objects. Zuora also provides a tool to help you automatically backfill this field with tenant ID for your existing product catalog. If you want to use this backfill tool, contact [Zuora Global Support](https://support.zuora.com/). **Note**: This field is only available if you set the `X-Zuora-WSDL-Version` request header to `133` or later.
* @return productRatePlanNumber
*/
@javax.annotation.Nullable
public String getProductRatePlanNumber() {
return productRatePlanNumber;
}
public void setProductRatePlanNumber(String productRatePlanNumber) {
this.productRatePlanNumber = productRatePlanNumber;
}
public UpdateProductRatePlanRequest name(String name) {
this.name = name;
return this;
}
/**
* The name of the product rate plan. The name doesn't have to be unique in a Product Catalog, but the name has to be unique within a product.
* @return name
*/
@javax.annotation.Nullable
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public UpdateProductRatePlanRequest productId(String productId) {
this.productId = productId;
return this;
}
/**
* The ID of the product that contains the product rate plan.
* @return productId
*/
@javax.annotation.Nullable
public String getProductId() {
return productId;
}
public void setProductId(String productId) {
this.productId = productId;
}
public UpdateProductRatePlanRequest activeCurrencies(List activeCurrencies) {
this.activeCurrencies = activeCurrencies;
return this;
}
public UpdateProductRatePlanRequest addActiveCurrenciesItem(String activeCurrenciesItem) {
if (this.activeCurrencies == null) {
this.activeCurrencies = new ArrayList<>();
}
this.activeCurrencies.add(activeCurrenciesItem);
return this;
}
/**
* A list of 3-letter currency codes representing active currencies for the product rate plan. Use a comma to separate each currency code. If the request body contains this field, the value of this field must contain the desired list of active currencies. The new list can never have more than four differences from the existing list. This field cannot be used to modify the status of more than four currencies in a single request. For example, in a single request, you can only activate four currencies, or deactivate four currencies, or activate two and deactivate two. Making more than four changes to currencies always requires more than one call. When specifying this field in the update request, you must provide the full list of active currencies you want, not just incremental changes. For each active currency update, provide the following currencies in the list: Current active currencies + at most four changes (additions or deletions)
* @return activeCurrencies
*/
@javax.annotation.Nullable
public List getActiveCurrencies() {
return activeCurrencies;
}
public void setActiveCurrencies(List activeCurrencies) {
this.activeCurrencies = activeCurrencies;
}
public UpdateProductRatePlanRequest description(String description) {
this.description = description;
return this;
}
/**
* A description of the product rate plan.
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public UpdateProductRatePlanRequest effectiveStartDate(LocalDate effectiveStartDate) {
this.effectiveStartDate = effectiveStartDate;
return this;
}
/**
* The date when the product rate plan becomes available and can be subscribed to, in `yyyy-mm-dd` format.
* @return effectiveStartDate
*/
@javax.annotation.Nullable
public LocalDate getEffectiveStartDate() {
return effectiveStartDate;
}
public void setEffectiveStartDate(LocalDate effectiveStartDate) {
this.effectiveStartDate = effectiveStartDate;
}
public UpdateProductRatePlanRequest effectiveEndDate(LocalDate effectiveEndDate) {
this.effectiveEndDate = effectiveEndDate;
return this;
}
/**
* The date when the product rate plan expires and can't be subscribed to, in `yyyy-mm-dd` format.
* @return effectiveEndDate
*/
@javax.annotation.Nullable
public LocalDate getEffectiveEndDate() {
return effectiveEndDate;
}
public void setEffectiveEndDate(LocalDate effectiveEndDate) {
this.effectiveEndDate = effectiveEndDate;
}
public UpdateProductRatePlanRequest grade(BigDecimal grade) {
this.grade = grade;
return this;
}
/**
* The grade that is assigned for the product rate plan. The value of this field must be a positive integer. The greater the value, the higher the grade. A product rate plan to be added to a Grading catalog group must have one grade. You can specify a grade for a product rate plan in this request or update the product rate plan individually. **Notes**: - To use this field, you must set the `X-Zuora-WSDL-Version` request header to `116` or later. Otherwise, an error occurs. - This field is in the **Early Adopter** phase. We are actively soliciting feedback from a small set of early adopters before releasing it as generally available. If you want to join this early adopter program, submit a request at [Zuora Global Support](http://support.zuora.com/).
* @return grade
*/
@javax.annotation.Nullable
public BigDecimal getGrade() {
return grade;
}
public void setGrade(BigDecimal grade) {
this.grade = grade;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the UpdateProductRatePlanRequest instance itself
*/
public UpdateProductRatePlanRequest putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UpdateProductRatePlanRequest updateProductRatePlanRequest = (UpdateProductRatePlanRequest) o;
return Objects.equals(this.billingPeriodNS, updateProductRatePlanRequest.billingPeriodNS) &&
Objects.equals(this.classNS, updateProductRatePlanRequest.classNS) &&
Objects.equals(this.departmentNS, updateProductRatePlanRequest.departmentNS) &&
Objects.equals(this.includeChildrenNS, updateProductRatePlanRequest.includeChildrenNS) &&
Objects.equals(this.integrationIdNS, updateProductRatePlanRequest.integrationIdNS) &&
Objects.equals(this.integrationStatusNS, updateProductRatePlanRequest.integrationStatusNS) &&
Objects.equals(this.itemTypeNS, updateProductRatePlanRequest.itemTypeNS) &&
Objects.equals(this.locationNS, updateProductRatePlanRequest.locationNS) &&
Objects.equals(this.multiCurrencyPriceNS, updateProductRatePlanRequest.multiCurrencyPriceNS) &&
Objects.equals(this.priceNS, updateProductRatePlanRequest.priceNS) &&
Objects.equals(this.subsidiaryNS, updateProductRatePlanRequest.subsidiaryNS) &&
Objects.equals(this.syncDateNS, updateProductRatePlanRequest.syncDateNS) &&
Objects.equals(this.productRatePlanNumber, updateProductRatePlanRequest.productRatePlanNumber) &&
Objects.equals(this.name, updateProductRatePlanRequest.name) &&
Objects.equals(this.productId, updateProductRatePlanRequest.productId) &&
Objects.equals(this.activeCurrencies, updateProductRatePlanRequest.activeCurrencies) &&
Objects.equals(this.description, updateProductRatePlanRequest.description) &&
Objects.equals(this.effectiveStartDate, updateProductRatePlanRequest.effectiveStartDate) &&
Objects.equals(this.effectiveEndDate, updateProductRatePlanRequest.effectiveEndDate) &&
Objects.equals(this.grade, updateProductRatePlanRequest.grade)&&
Objects.equals(this.additionalProperties, updateProductRatePlanRequest.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(billingPeriodNS, classNS, departmentNS, includeChildrenNS, integrationIdNS, integrationStatusNS, itemTypeNS, locationNS, multiCurrencyPriceNS, priceNS, subsidiaryNS, syncDateNS, productRatePlanNumber, name, productId, activeCurrencies, description, effectiveStartDate, effectiveEndDate, grade, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UpdateProductRatePlanRequest {\n");
sb.append(" billingPeriodNS: ").append(toIndentedString(billingPeriodNS)).append("\n");
sb.append(" classNS: ").append(toIndentedString(classNS)).append("\n");
sb.append(" departmentNS: ").append(toIndentedString(departmentNS)).append("\n");
sb.append(" includeChildrenNS: ").append(toIndentedString(includeChildrenNS)).append("\n");
sb.append(" integrationIdNS: ").append(toIndentedString(integrationIdNS)).append("\n");
sb.append(" integrationStatusNS: ").append(toIndentedString(integrationStatusNS)).append("\n");
sb.append(" itemTypeNS: ").append(toIndentedString(itemTypeNS)).append("\n");
sb.append(" locationNS: ").append(toIndentedString(locationNS)).append("\n");
sb.append(" multiCurrencyPriceNS: ").append(toIndentedString(multiCurrencyPriceNS)).append("\n");
sb.append(" priceNS: ").append(toIndentedString(priceNS)).append("\n");
sb.append(" subsidiaryNS: ").append(toIndentedString(subsidiaryNS)).append("\n");
sb.append(" syncDateNS: ").append(toIndentedString(syncDateNS)).append("\n");
sb.append(" productRatePlanNumber: ").append(toIndentedString(productRatePlanNumber)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" productId: ").append(toIndentedString(productId)).append("\n");
sb.append(" activeCurrencies: ").append(toIndentedString(activeCurrencies)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" effectiveStartDate: ").append(toIndentedString(effectiveStartDate)).append("\n");
sb.append(" effectiveEndDate: ").append(toIndentedString(effectiveEndDate)).append("\n");
sb.append(" grade: ").append(toIndentedString(grade)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("BillingPeriod__NS");
openapiFields.add("Class__NS");
openapiFields.add("Department__NS");
openapiFields.add("IncludeChildren__NS");
openapiFields.add("IntegrationId__NS");
openapiFields.add("IntegrationStatus__NS");
openapiFields.add("ItemType__NS");
openapiFields.add("Location__NS");
openapiFields.add("MultiCurrencyPrice__NS");
openapiFields.add("Price__NS");
openapiFields.add("Subsidiary__NS");
openapiFields.add("SyncDate__NS");
openapiFields.add("ProductRatePlanNumber");
openapiFields.add("Name");
openapiFields.add("ProductId");
openapiFields.add("ActiveCurrencies");
openapiFields.add("Description");
openapiFields.add("EffectiveStartDate");
openapiFields.add("EffectiveEndDate");
openapiFields.add("Grade");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to UpdateProductRatePlanRequest
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!UpdateProductRatePlanRequest.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in UpdateProductRatePlanRequest is not found in the empty JSON string", UpdateProductRatePlanRequest.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("BillingPeriod__NS") != null && !jsonObj.get("BillingPeriod__NS").isJsonNull()) && !jsonObj.get("BillingPeriod__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `BillingPeriod__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("BillingPeriod__NS").toString()));
}
// validate the optional field `BillingPeriod__NS`
if (jsonObj.get("BillingPeriod__NS") != null && !jsonObj.get("BillingPeriod__NS").isJsonNull()) {
ProductRatePlanObjectNSFieldsBillingPeriodNS.validateJsonElement(jsonObj.get("BillingPeriod__NS"));
}
if ((jsonObj.get("Class__NS") != null && !jsonObj.get("Class__NS").isJsonNull()) && !jsonObj.get("Class__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Class__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Class__NS").toString()));
}
if ((jsonObj.get("Department__NS") != null && !jsonObj.get("Department__NS").isJsonNull()) && !jsonObj.get("Department__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Department__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Department__NS").toString()));
}
if ((jsonObj.get("IncludeChildren__NS") != null && !jsonObj.get("IncludeChildren__NS").isJsonNull()) && !jsonObj.get("IncludeChildren__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IncludeChildren__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IncludeChildren__NS").toString()));
}
// validate the optional field `IncludeChildren__NS`
if (jsonObj.get("IncludeChildren__NS") != null && !jsonObj.get("IncludeChildren__NS").isJsonNull()) {
ProductRatePlanObjectNSFieldsIncludeChildrenNS.validateJsonElement(jsonObj.get("IncludeChildren__NS"));
}
if ((jsonObj.get("IntegrationId__NS") != null && !jsonObj.get("IntegrationId__NS").isJsonNull()) && !jsonObj.get("IntegrationId__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationId__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationId__NS").toString()));
}
if ((jsonObj.get("IntegrationStatus__NS") != null && !jsonObj.get("IntegrationStatus__NS").isJsonNull()) && !jsonObj.get("IntegrationStatus__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationStatus__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationStatus__NS").toString()));
}
if ((jsonObj.get("ItemType__NS") != null && !jsonObj.get("ItemType__NS").isJsonNull()) && !jsonObj.get("ItemType__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ItemType__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ItemType__NS").toString()));
}
// validate the optional field `ItemType__NS`
if (jsonObj.get("ItemType__NS") != null && !jsonObj.get("ItemType__NS").isJsonNull()) {
ProductRatePlanObjectNSFieldsItemTypeNS.validateJsonElement(jsonObj.get("ItemType__NS"));
}
if ((jsonObj.get("Location__NS") != null && !jsonObj.get("Location__NS").isJsonNull()) && !jsonObj.get("Location__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Location__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Location__NS").toString()));
}
if ((jsonObj.get("MultiCurrencyPrice__NS") != null && !jsonObj.get("MultiCurrencyPrice__NS").isJsonNull()) && !jsonObj.get("MultiCurrencyPrice__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `MultiCurrencyPrice__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("MultiCurrencyPrice__NS").toString()));
}
if ((jsonObj.get("Price__NS") != null && !jsonObj.get("Price__NS").isJsonNull()) && !jsonObj.get("Price__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Price__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Price__NS").toString()));
}
if ((jsonObj.get("Subsidiary__NS") != null && !jsonObj.get("Subsidiary__NS").isJsonNull()) && !jsonObj.get("Subsidiary__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Subsidiary__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Subsidiary__NS").toString()));
}
if ((jsonObj.get("SyncDate__NS") != null && !jsonObj.get("SyncDate__NS").isJsonNull()) && !jsonObj.get("SyncDate__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SyncDate__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SyncDate__NS").toString()));
}
if ((jsonObj.get("ProductRatePlanNumber") != null && !jsonObj.get("ProductRatePlanNumber").isJsonNull()) && !jsonObj.get("ProductRatePlanNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ProductRatePlanNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ProductRatePlanNumber").toString()));
}
if ((jsonObj.get("Name") != null && !jsonObj.get("Name").isJsonNull()) && !jsonObj.get("Name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Name").toString()));
}
if ((jsonObj.get("ProductId") != null && !jsonObj.get("ProductId").isJsonNull()) && !jsonObj.get("ProductId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ProductId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ProductId").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("ActiveCurrencies") != null && !jsonObj.get("ActiveCurrencies").isJsonNull() && !jsonObj.get("ActiveCurrencies").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `ActiveCurrencies` to be an array in the JSON string but got `%s`", jsonObj.get("ActiveCurrencies").toString()));
}
if ((jsonObj.get("Description") != null && !jsonObj.get("Description").isJsonNull()) && !jsonObj.get("Description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Description").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!UpdateProductRatePlanRequest.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'UpdateProductRatePlanRequest' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(UpdateProductRatePlanRequest.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, UpdateProductRatePlanRequest value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public UpdateProductRatePlanRequest read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
UpdateProductRatePlanRequest instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of UpdateProductRatePlanRequest given an JSON string
*
* @param jsonString JSON string
* @return An instance of UpdateProductRatePlanRequest
* @throws IOException if the JSON string is invalid with respect to UpdateProductRatePlanRequest
*/
public static UpdateProductRatePlanRequest fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, UpdateProductRatePlanRequest.class);
}
/**
* Convert an instance of UpdateProductRatePlanRequest to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy