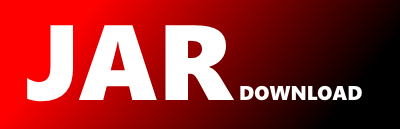
org.openapitools.client.api.BillRunPreviewsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.api;
import org.openapitools.client.ApiCallback;
import org.openapitools.client.ApiClient;
import org.openapitools.client.ApiException;
import org.openapitools.client.model.Headers;
import org.openapitools.client.model.ListQueryParams;
import org.openapitools.client.model.GetByIdQueryParams;
import org.openapitools.client.ApiResponse;
import org.openapitools.client.Configuration;
import org.openapitools.client.Pair;
import org.openapitools.client.ProgressRequestBody;
import org.openapitools.client.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import java.math.BigDecimal;
import org.openapitools.client.model.BillRunPreview;
import org.openapitools.client.model.BillRunPreviewCreateRequest;
import org.openapitools.client.model.ErrorResponse;
import org.openapitools.client.model.ErrorResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class BillRunPreviewsApi {
private ApiClient localVarApiClient;
private int localHostIndex;
private String localCustomBaseUrl;
public BillRunPreviewsApi() {
this(Configuration.getDefaultApiClient());
}
public BillRunPreviewsApi(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public ApiClient getApiClient() {
return localVarApiClient;
}
public void setApiClient(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public int getHostIndex() {
return localHostIndex;
}
public void setHostIndex(int hostIndex) {
this.localHostIndex = hostIndex;
}
public String getCustomBaseUrl() {
return localCustomBaseUrl;
}
public void setCustomBaseUrl(String customBaseUrl) {
this.localCustomBaseUrl = customBaseUrl;
}
private okhttp3.Call createBillRunPreviewCall(BillRunPreviewCreateRequest billRunPreviewCreateRequest, List fields, List billRunPreviewFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = billRunPreviewCreateRequest;
// create path and map variables
String localVarPath = "/bill_run_previews";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
if (fields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "fields[]", fields));
}
if (billRunPreviewFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "bill_run_preview.fields[]", billRunPreviewFields));
}
if (expand != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "expand[]", expand));
}
if (filter != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "filter[]", filter));
}
if (pageSize != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("page_size", pageSize));
}
if (zuoraTrackId != null) {
localVarHeaderParams.put("zuora-track-id", localVarApiClient.parameterToString(zuoraTrackId));
}
if (async != null) {
localVarHeaderParams.put("async", localVarApiClient.parameterToString(async));
}
if (zuoraCacheEnabled != null) {
localVarHeaderParams.put("zuora-cache-enabled", localVarApiClient.parameterToString(zuoraCacheEnabled));
}
if (zuoraEntityIds != null) {
localVarHeaderParams.put("zuora-entity-ids", localVarApiClient.parameterToString(zuoraEntityIds));
}
if (idempotencyKey != null) {
localVarHeaderParams.put("idempotency-key", localVarApiClient.parameterToString(idempotencyKey));
}
if (acceptEncoding != null) {
localVarHeaderParams.put("accept-encoding", localVarApiClient.parameterToString(acceptEncoding));
}
if (contentEncoding != null) {
localVarHeaderParams.put("content-encoding", localVarApiClient.parameterToString(contentEncoding));
}
if (zuoraOrgIds != null) {
localVarHeaderParams.put("zuora-org-ids", localVarApiClient.parameterToString(zuoraOrgIds));
}
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "bearerAuth" };
return localVarApiClient.buildCall(basePath, localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call createBillRunPreviewValidateBeforeCall(BillRunPreviewCreateRequest billRunPreviewCreateRequest, List fields, List billRunPreviewFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'billRunPreviewCreateRequest' is set
if (billRunPreviewCreateRequest == null) {
throw new ApiException("Missing the required parameter 'billRunPreviewCreateRequest' when calling createBillRunPreview(Async)");
}
return createBillRunPreviewCall(billRunPreviewCreateRequest, fields, billRunPreviewFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
}
private ApiResponse createBillRunPreviewWithHttpInfo(BillRunPreviewCreateRequest billRunPreviewCreateRequest, List fields, List billRunPreviewFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds) throws ApiException {
okhttp3.Call localVarCall = createBillRunPreviewValidateBeforeCall(billRunPreviewCreateRequest, fields, billRunPreviewFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, null);
try {
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
} catch (ApiException e) {
e.setErrorObject(localVarApiClient.getJSON().getGson().fromJson(e.getResponseBody(), new TypeToken(){}.getType()));
throw e;
}
}
private okhttp3.Call createBillRunPreviewAsync(BillRunPreviewCreateRequest billRunPreviewCreateRequest, List fields, List billRunPreviewFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = createBillRunPreviewValidateBeforeCall(billRunPreviewCreateRequest, fields, billRunPreviewFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
public static class CreateBillRunPreviewParams {
private final BillRunPreviewCreateRequest billRunPreviewCreateRequest;
private Headers headers;
/*
* Check for list params
*/
private GetByIdQueryParams getByIdQueryParams;
private List fields;
private List billRunPreviewFields;
private List expand;
private List filter;
private Integer pageSize;
private String zuoraTrackId;
private Boolean async;
private Boolean zuoraCacheEnabled;
private String zuoraEntityIds;
private String idempotencyKey;
private String acceptEncoding;
private String contentEncoding;
private String zuoraOrgIds;
public CreateBillRunPreviewParams(BillRunPreviewCreateRequest billRunPreviewCreateRequest) {
this.billRunPreviewCreateRequest = billRunPreviewCreateRequest;
}
/**
* Set getByIdQueryParams
* @param getByIdQueryParams GetByIdQueryParams
* @return CreateBillRunPreviewParams
*/
public CreateBillRunPreviewParams getByIdQueryParams(GetByIdQueryParams getByIdQueryParams) {
this.getByIdQueryParams = getByIdQueryParams;
if (expand != null) {
this.expand = getByIdQueryParams.getExpand();
}
if (filter != null) {
this.filter = getByIdQueryParams.getFilter();
}
if (pageSize != null) {
this.pageSize = getByIdQueryParams.getPageSize();
}
return this;
}
/**
* Set headers
* @param headers Headers
* @return CreateBillRunPreviewParams
*/
public CreateBillRunPreviewParams headers(Headers headers) {
this.headers = headers;
if (headers.getZuoraTrackId() != null) {
this.zuoraTrackId = headers.getZuoraTrackId();
}
if (headers.getAsync() != null) {
this.async = headers.getAsync();
}
if (headers.getZuoraCacheEnabled() != null) {
this.zuoraCacheEnabled = headers.getZuoraCacheEnabled();
}
if (headers.getZuoraEntityIds() != null) {
this.zuoraEntityIds = headers.getZuoraEntityIds();
}
if (headers.getIdempotencyKey() != null) {
this.idempotencyKey = headers.getIdempotencyKey();
}
if (headers.getAcceptEncoding() != null) {
this.acceptEncoding = headers.getAcceptEncoding();
}
if (headers.getContentEncoding() != null) {
this.contentEncoding = headers.getContentEncoding();
}
if (headers.getZuoraOrgIds() != null) {
this.zuoraOrgIds = headers.getZuoraOrgIds();
}
return this;
}
/**
* Set fields
* @param fields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `assume_renewal`, `charges_excluded`, `batches`, `target_date`, `state`, `number_of_accounts`, `number_of_accounts_succeeded`, `state_transitions`, `billing_preview_run_number`, `file`, `include_draft_items`, `include_evergreen_subscriptions` </details> (optional)
* @return CreateBillRunPreviewParams
*/
public CreateBillRunPreviewParams fields(List fields) {
this.fields = fields;
return this;
}
/**
* Set billRunPreviewFields
* @param billRunPreviewFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `assume_renewal`, `charges_excluded`, `batches`, `target_date`, `state`, `number_of_accounts`, `number_of_accounts_succeeded`, `state_transitions`, `billing_preview_run_number`, `file`, `include_draft_items`, `include_evergreen_subscriptions` </details> (optional)
* @return CreateBillRunPreviewParams
*/
public CreateBillRunPreviewParams billRunPreviewFields(List billRunPreviewFields) {
this.billRunPreviewFields = billRunPreviewFields;
return this;
}
/**
* Set expand
* @param expand Allows you to expand responses by including related object information in a single call. See the [Expand responses](https://developer.zuora.com/quickstart-api/tutorial/expand-responses/) section of the Quickstart API Tutorials for detailed instructions. (optional)
* @return CreateBillRunPreviewParams
*/
public CreateBillRunPreviewParams expand(List expand) {
this.expand = expand;
return this;
}
/**
* Set filter
* @param filter A case-sensitive filter on the list. See the [Filter lists](https://developer.zuora.com/quickstart-api/tutorial/filter-lists/) section of the Quickstart API Tutorial for detailed instructions. Note that the filters on this operation are only applicable to the related objects. For example, when you are calling the \"Retrieve a billing document\" operation, you can use the `filter[]` parameter on the related objects such as `filter[]=items[account_id].EQ:8ad09e208858b5cf0188595208151c63` (optional)
* @return CreateBillRunPreviewParams
*/
public CreateBillRunPreviewParams filter(List filter) {
this.filter = filter;
return this;
}
/**
* Set pageSize
* @param pageSize The maximum number of results to return in a single page. If the specified `page_size` is less than 1 or greater than 99, Zuora will return a 400 error. (optional)
* @return CreateBillRunPreviewParams
*/
public CreateBillRunPreviewParams pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
}
/**
* Create a bill run preview
* Creates a bill run preview for a batch of customer accounts.
* @param billRunPreviewCreateRequest (required)
* @return BillRunPreview
* @http.response.details
Status Code Description Response Headers
201 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public CreateBillRunPreviewParams createBillRunPreviewParams(BillRunPreviewCreateRequest billRunPreviewCreateRequest) {
return new CreateBillRunPreviewParams(billRunPreviewCreateRequest);
}
public BillRunPreview createBillRunPreview(BillRunPreviewCreateRequest billRunPreviewCreateRequest) throws ApiException {
CreateBillRunPreviewParams params = new CreateBillRunPreviewParams(billRunPreviewCreateRequest);
return executeCreateBillRunPreviewAPICall(params).getData();
}
/**
* Expose HTTP info
public ApiResponse createBillRunPreviewWithHttpInfo(BillRunPreviewCreateRequest billRunPreviewCreateRequest) throws ApiException {
CreateBillRunPreviewParams params = new CreateBillRunPreviewParams(billRunPreviewCreateRequest);
return executeCreateBillRunPreviewAPICall(params);
}
**/
/**
Legacy Query Params Requests
**/
public BillRunPreview createBillRunPreview( BillRunPreviewCreateRequest billRunPreviewCreateRequest , List expand) throws ApiException {
CreateBillRunPreviewParams params = new CreateBillRunPreviewParams(billRunPreviewCreateRequest);
params.expand(expand);
return executeCreateBillRunPreviewAPICall(params).getData();
}
/*
* add header methods
*/
public BillRunPreview createBillRunPreview(BillRunPreviewCreateRequest billRunPreviewCreateRequest ,List expand,Headers headers) throws ApiException {
CreateBillRunPreviewParams params = new CreateBillRunPreviewParams(billRunPreviewCreateRequest)
.expand(expand)
.headers(headers);
return executeCreateBillRunPreviewAPICall(params).getData();
}
/**
* Create a bill run preview
* Creates a bill run preview for a batch of customer accounts.
* @param params CreateBillRunPreviewParams
* @return BillRunPreview
* @http.response.details
Status Code Description Response Headers
201 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public BillRunPreview createBillRunPreview(CreateBillRunPreviewParams params) throws ApiException {
return executeCreateBillRunPreviewAPICall(params).getData();
}
public ApiResponse createBillRunPreviewWithHttpInfo(CreateBillRunPreviewParams params) throws ApiException {
return executeCreateBillRunPreviewAPICall(params);
}
ApiResponse executeCreateBillRunPreviewAPICall(CreateBillRunPreviewParams params) throws ApiException {
return createBillRunPreviewWithHttpInfo(params.billRunPreviewCreateRequest, params.fields, params.billRunPreviewFields, params.expand, params.filter, params.pageSize, params.zuoraTrackId, params.async, params.zuoraCacheEnabled, params.zuoraEntityIds, params.idempotencyKey, params.acceptEncoding, params.contentEncoding, params.zuoraOrgIds);
}
private okhttp3.Call getBillRunPreviewCall(String billRunPreviewId, List fields, List billRunPreviewFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/bill_run_previews/{bill_run_preview_id}"
.replace("{" + "bill_run_preview_id" + "}", localVarApiClient.escapeString(billRunPreviewId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
if (fields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "fields[]", fields));
}
if (billRunPreviewFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "bill_run_preview.fields[]", billRunPreviewFields));
}
if (expand != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "expand[]", expand));
}
if (filter != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "filter[]", filter));
}
if (pageSize != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("page_size", pageSize));
}
if (zuoraTrackId != null) {
localVarHeaderParams.put("zuora-track-id", localVarApiClient.parameterToString(zuoraTrackId));
}
if (async != null) {
localVarHeaderParams.put("async", localVarApiClient.parameterToString(async));
}
if (zuoraCacheEnabled != null) {
localVarHeaderParams.put("zuora-cache-enabled", localVarApiClient.parameterToString(zuoraCacheEnabled));
}
if (zuoraEntityIds != null) {
localVarHeaderParams.put("zuora-entity-ids", localVarApiClient.parameterToString(zuoraEntityIds));
}
if (idempotencyKey != null) {
localVarHeaderParams.put("idempotency-key", localVarApiClient.parameterToString(idempotencyKey));
}
if (acceptEncoding != null) {
localVarHeaderParams.put("accept-encoding", localVarApiClient.parameterToString(acceptEncoding));
}
if (contentEncoding != null) {
localVarHeaderParams.put("content-encoding", localVarApiClient.parameterToString(contentEncoding));
}
if (zuoraOrgIds != null) {
localVarHeaderParams.put("zuora-org-ids", localVarApiClient.parameterToString(zuoraOrgIds));
}
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "bearerAuth" };
return localVarApiClient.buildCall(basePath, localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getBillRunPreviewValidateBeforeCall(String billRunPreviewId, List fields, List billRunPreviewFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'billRunPreviewId' is set
if (billRunPreviewId == null) {
throw new ApiException("Missing the required parameter 'billRunPreviewId' when calling getBillRunPreview(Async)");
}
return getBillRunPreviewCall(billRunPreviewId, fields, billRunPreviewFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
}
private ApiResponse getBillRunPreviewWithHttpInfo(String billRunPreviewId, List fields, List billRunPreviewFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds) throws ApiException {
okhttp3.Call localVarCall = getBillRunPreviewValidateBeforeCall(billRunPreviewId, fields, billRunPreviewFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, null);
try {
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
} catch (ApiException e) {
e.setErrorObject(localVarApiClient.getJSON().getGson().fromJson(e.getResponseBody(), new TypeToken(){}.getType()));
throw e;
}
}
private okhttp3.Call getBillRunPreviewAsync(String billRunPreviewId, List fields, List billRunPreviewFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = getBillRunPreviewValidateBeforeCall(billRunPreviewId, fields, billRunPreviewFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
public static class GetBillRunPreviewParams {
private final String billRunPreviewId;
private Headers headers;
/*
* Check for list params
*/
private GetByIdQueryParams getByIdQueryParams;
private List fields;
private List billRunPreviewFields;
private List expand;
private List filter;
private Integer pageSize;
private String zuoraTrackId;
private Boolean async;
private Boolean zuoraCacheEnabled;
private String zuoraEntityIds;
private String idempotencyKey;
private String acceptEncoding;
private String contentEncoding;
private String zuoraOrgIds;
public GetBillRunPreviewParams(String billRunPreviewId) {
this.billRunPreviewId = billRunPreviewId;
}
/**
* Set getByIdQueryParams
* @param getByIdQueryParams GetByIdQueryParams
* @return GetBillRunPreviewParams
*/
public GetBillRunPreviewParams getByIdQueryParams(GetByIdQueryParams getByIdQueryParams) {
this.getByIdQueryParams = getByIdQueryParams;
if (expand != null) {
this.expand = getByIdQueryParams.getExpand();
}
if (filter != null) {
this.filter = getByIdQueryParams.getFilter();
}
if (pageSize != null) {
this.pageSize = getByIdQueryParams.getPageSize();
}
return this;
}
/**
* Set headers
* @param headers Headers
* @return GetBillRunPreviewParams
*/
public GetBillRunPreviewParams headers(Headers headers) {
this.headers = headers;
if (headers.getZuoraTrackId() != null) {
this.zuoraTrackId = headers.getZuoraTrackId();
}
if (headers.getAsync() != null) {
this.async = headers.getAsync();
}
if (headers.getZuoraCacheEnabled() != null) {
this.zuoraCacheEnabled = headers.getZuoraCacheEnabled();
}
if (headers.getZuoraEntityIds() != null) {
this.zuoraEntityIds = headers.getZuoraEntityIds();
}
if (headers.getIdempotencyKey() != null) {
this.idempotencyKey = headers.getIdempotencyKey();
}
if (headers.getAcceptEncoding() != null) {
this.acceptEncoding = headers.getAcceptEncoding();
}
if (headers.getContentEncoding() != null) {
this.contentEncoding = headers.getContentEncoding();
}
if (headers.getZuoraOrgIds() != null) {
this.zuoraOrgIds = headers.getZuoraOrgIds();
}
return this;
}
/**
* Set fields
* @param fields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `assume_renewal`, `charges_excluded`, `batches`, `target_date`, `state`, `number_of_accounts`, `number_of_accounts_succeeded`, `state_transitions`, `billing_preview_run_number`, `file`, `include_draft_items`, `include_evergreen_subscriptions` </details> (optional)
* @return GetBillRunPreviewParams
*/
public GetBillRunPreviewParams fields(List fields) {
this.fields = fields;
return this;
}
/**
* Set billRunPreviewFields
* @param billRunPreviewFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `assume_renewal`, `charges_excluded`, `batches`, `target_date`, `state`, `number_of_accounts`, `number_of_accounts_succeeded`, `state_transitions`, `billing_preview_run_number`, `file`, `include_draft_items`, `include_evergreen_subscriptions` </details> (optional)
* @return GetBillRunPreviewParams
*/
public GetBillRunPreviewParams billRunPreviewFields(List billRunPreviewFields) {
this.billRunPreviewFields = billRunPreviewFields;
return this;
}
/**
* Set expand
* @param expand Allows you to expand responses by including related object information in a single call. See the [Expand responses](https://developer.zuora.com/quickstart-api/tutorial/expand-responses/) section of the Quickstart API Tutorials for detailed instructions. (optional)
* @return GetBillRunPreviewParams
*/
public GetBillRunPreviewParams expand(List expand) {
this.expand = expand;
return this;
}
/**
* Set filter
* @param filter A case-sensitive filter on the list. See the [Filter lists](https://developer.zuora.com/quickstart-api/tutorial/filter-lists/) section of the Quickstart API Tutorial for detailed instructions. Note that the filters on this operation are only applicable to the related objects. For example, when you are calling the \"Retrieve a billing document\" operation, you can use the `filter[]` parameter on the related objects such as `filter[]=items[account_id].EQ:8ad09e208858b5cf0188595208151c63` (optional)
* @return GetBillRunPreviewParams
*/
public GetBillRunPreviewParams filter(List filter) {
this.filter = filter;
return this;
}
/**
* Set pageSize
* @param pageSize The maximum number of results to return in a single page. If the specified `page_size` is less than 1 or greater than 99, Zuora will return a 400 error. (optional)
* @return GetBillRunPreviewParams
*/
public GetBillRunPreviewParams pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
}
/**
* Retrieve a bill run preview
* Retrieves the bill run preview information with the given ID.
* @param billRunPreviewId Identifier for the bill run preview. (required)
* @return BillRunPreview
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public GetBillRunPreviewParams getBillRunPreviewParams(String billRunPreviewId) {
return new GetBillRunPreviewParams(billRunPreviewId);
}
public BillRunPreview getBillRunPreview(String billRunPreviewId) throws ApiException {
GetBillRunPreviewParams params = new GetBillRunPreviewParams(billRunPreviewId);
return executeGetBillRunPreviewAPICall(params).getData();
}
/**
* Expose HTTP info
public ApiResponse getBillRunPreviewWithHttpInfo(String billRunPreviewId) throws ApiException {
GetBillRunPreviewParams params = new GetBillRunPreviewParams(billRunPreviewId);
return executeGetBillRunPreviewAPICall(params);
}
**/
/**
Legacy Query Params Requests
**/
public BillRunPreview getBillRunPreview( String billRunPreviewId , List expand) throws ApiException {
GetBillRunPreviewParams params = new GetBillRunPreviewParams(billRunPreviewId);
params.expand(expand);
return executeGetBillRunPreviewAPICall(params).getData();
}
/*
* add header methods
*/
public BillRunPreview getBillRunPreview(String billRunPreviewId ,List expand,Headers headers) throws ApiException {
GetBillRunPreviewParams params = new GetBillRunPreviewParams(billRunPreviewId)
.expand(expand)
.headers(headers);
return executeGetBillRunPreviewAPICall(params).getData();
}
/**
* Retrieve a bill run preview
* Retrieves the bill run preview information with the given ID.
* @param params GetBillRunPreviewParams
* @return BillRunPreview
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public BillRunPreview getBillRunPreview(GetBillRunPreviewParams params) throws ApiException {
return executeGetBillRunPreviewAPICall(params).getData();
}
public ApiResponse getBillRunPreviewWithHttpInfo(GetBillRunPreviewParams params) throws ApiException {
return executeGetBillRunPreviewAPICall(params);
}
ApiResponse executeGetBillRunPreviewAPICall(GetBillRunPreviewParams params) throws ApiException {
return getBillRunPreviewWithHttpInfo(params.billRunPreviewId, params.fields, params.billRunPreviewFields, params.expand, params.filter, params.pageSize, params.zuoraTrackId, params.async, params.zuoraCacheEnabled, params.zuoraEntityIds, params.idempotencyKey, params.acceptEncoding, params.contentEncoding, params.zuoraOrgIds);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy