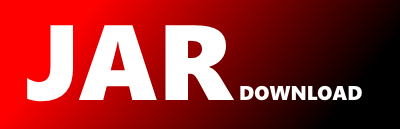
org.openapitools.client.api.BillingDocumentsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.api;
import org.openapitools.client.ApiCallback;
import org.openapitools.client.ApiClient;
import org.openapitools.client.ApiException;
import org.openapitools.client.model.Headers;
import org.openapitools.client.model.ListQueryParams;
import org.openapitools.client.model.GetByIdQueryParams;
import org.openapitools.client.ApiResponse;
import org.openapitools.client.Configuration;
import org.openapitools.client.Pair;
import org.openapitools.client.ProgressRequestBody;
import org.openapitools.client.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import java.math.BigDecimal;
import org.openapitools.client.model.BillingDocument;
import org.openapitools.client.model.BillingDocumentCreateRequest;
import org.openapitools.client.model.BillingDocumentListResponse;
import org.openapitools.client.model.ErrorResponse;
import org.openapitools.client.model.ErrorResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class BillingDocumentsApi {
private ApiClient localVarApiClient;
private int localHostIndex;
private String localCustomBaseUrl;
public BillingDocumentsApi() {
this(Configuration.getDefaultApiClient());
}
public BillingDocumentsApi(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public ApiClient getApiClient() {
return localVarApiClient;
}
public void setApiClient(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public int getHostIndex() {
return localHostIndex;
}
public void setHostIndex(int hostIndex) {
this.localHostIndex = hostIndex;
}
public String getCustomBaseUrl() {
return localCustomBaseUrl;
}
public void setCustomBaseUrl(String customBaseUrl) {
this.localCustomBaseUrl = customBaseUrl;
}
private okhttp3.Call getBillingDocumentCall(String billingDocumentId, List fields, List billingDocumentFields, List billingDocumentItemsFields, List taxationItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/billing_documents/{billing_document_id}"
.replace("{" + "billing_document_id" + "}", localVarApiClient.escapeString(billingDocumentId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
if (fields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "fields[]", fields));
}
if (billingDocumentFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "billing_document.fields[]", billingDocumentFields));
}
if (billingDocumentItemsFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "billing_document_items.fields[]", billingDocumentItemsFields));
}
if (taxationItemsFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "taxation_items.fields[]", taxationItemsFields));
}
if (expand != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "expand[]", expand));
}
if (filter != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "filter[]", filter));
}
if (pageSize != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("page_size", pageSize));
}
if (zuoraTrackId != null) {
localVarHeaderParams.put("zuora-track-id", localVarApiClient.parameterToString(zuoraTrackId));
}
if (async != null) {
localVarHeaderParams.put("async", localVarApiClient.parameterToString(async));
}
if (zuoraCacheEnabled != null) {
localVarHeaderParams.put("zuora-cache-enabled", localVarApiClient.parameterToString(zuoraCacheEnabled));
}
if (zuoraEntityIds != null) {
localVarHeaderParams.put("zuora-entity-ids", localVarApiClient.parameterToString(zuoraEntityIds));
}
if (idempotencyKey != null) {
localVarHeaderParams.put("idempotency-key", localVarApiClient.parameterToString(idempotencyKey));
}
if (acceptEncoding != null) {
localVarHeaderParams.put("accept-encoding", localVarApiClient.parameterToString(acceptEncoding));
}
if (contentEncoding != null) {
localVarHeaderParams.put("content-encoding", localVarApiClient.parameterToString(contentEncoding));
}
if (zuoraOrgIds != null) {
localVarHeaderParams.put("zuora-org-ids", localVarApiClient.parameterToString(zuoraOrgIds));
}
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "bearerAuth" };
return localVarApiClient.buildCall(basePath, localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getBillingDocumentValidateBeforeCall(String billingDocumentId, List fields, List billingDocumentFields, List billingDocumentItemsFields, List taxationItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'billingDocumentId' is set
if (billingDocumentId == null) {
throw new ApiException("Missing the required parameter 'billingDocumentId' when calling getBillingDocument(Async)");
}
return getBillingDocumentCall(billingDocumentId, fields, billingDocumentFields, billingDocumentItemsFields, taxationItemsFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
}
private ApiResponse getBillingDocumentWithHttpInfo(String billingDocumentId, List fields, List billingDocumentFields, List billingDocumentItemsFields, List taxationItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds) throws ApiException {
okhttp3.Call localVarCall = getBillingDocumentValidateBeforeCall(billingDocumentId, fields, billingDocumentFields, billingDocumentItemsFields, taxationItemsFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, null);
try {
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
} catch (ApiException e) {
e.setErrorObject(localVarApiClient.getJSON().getGson().fromJson(e.getResponseBody(), new TypeToken(){}.getType()));
throw e;
}
}
private okhttp3.Call getBillingDocumentAsync(String billingDocumentId, List fields, List billingDocumentFields, List billingDocumentItemsFields, List taxationItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = getBillingDocumentValidateBeforeCall(billingDocumentId, fields, billingDocumentFields, billingDocumentItemsFields, taxationItemsFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
public static class GetBillingDocumentParams {
private final String billingDocumentId;
private Headers headers;
/*
* Check for list params
*/
private GetByIdQueryParams getByIdQueryParams;
private List fields;
private List billingDocumentFields;
private List billingDocumentItemsFields;
private List taxationItemsFields;
private List expand;
private List filter;
private Integer pageSize;
private String zuoraTrackId;
private Boolean async;
private Boolean zuoraCacheEnabled;
private String zuoraEntityIds;
private String idempotencyKey;
private String acceptEncoding;
private String contentEncoding;
private String zuoraOrgIds;
public GetBillingDocumentParams(String billingDocumentId) {
this.billingDocumentId = billingDocumentId;
}
/**
* Set getByIdQueryParams
* @param getByIdQueryParams GetByIdQueryParams
* @return GetBillingDocumentParams
*/
public GetBillingDocumentParams getByIdQueryParams(GetByIdQueryParams getByIdQueryParams) {
this.getByIdQueryParams = getByIdQueryParams;
if (expand != null) {
this.expand = getByIdQueryParams.getExpand();
}
if (filter != null) {
this.filter = getByIdQueryParams.getFilter();
}
if (pageSize != null) {
this.pageSize = getByIdQueryParams.getPageSize();
}
return this;
}
/**
* Set headers
* @param headers Headers
* @return GetBillingDocumentParams
*/
public GetBillingDocumentParams headers(Headers headers) {
this.headers = headers;
if (headers.getZuoraTrackId() != null) {
this.zuoraTrackId = headers.getZuoraTrackId();
}
if (headers.getAsync() != null) {
this.async = headers.getAsync();
}
if (headers.getZuoraCacheEnabled() != null) {
this.zuoraCacheEnabled = headers.getZuoraCacheEnabled();
}
if (headers.getZuoraEntityIds() != null) {
this.zuoraEntityIds = headers.getZuoraEntityIds();
}
if (headers.getIdempotencyKey() != null) {
this.idempotencyKey = headers.getIdempotencyKey();
}
if (headers.getAcceptEncoding() != null) {
this.acceptEncoding = headers.getAcceptEncoding();
}
if (headers.getContentEncoding() != null) {
this.contentEncoding = headers.getContentEncoding();
}
if (headers.getZuoraOrgIds() != null) {
this.zuoraOrgIds = headers.getZuoraOrgIds();
}
return this;
}
/**
* Set fields
* @param fields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `account_id`, `balance`, `description`, `state`, `tax` </details> (optional)
* @return GetBillingDocumentParams
*/
public GetBillingDocumentParams fields(List fields) {
this.fields = fields;
return this;
}
/**
* Set billingDocumentFields
* @param billingDocumentFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `account_id`, `balance`, `description`, `state`, `tax` </details> (optional)
* @return GetBillingDocumentParams
*/
public GetBillingDocumentParams billingDocumentFields(List billingDocumentFields) {
this.billingDocumentFields = billingDocumentFields;
return this;
}
/**
* Set billingDocumentItemsFields
* @param billingDocumentItemsFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `price_id`, `discount_item`, `deferred_revenue_account`, `description`, `name`, `quantity`, `recognized_revenue_account`, `service_end`, `service_start`, `accounts_receivable_account`, `subscription_id`, `subscription_item_id`, `tax`, `tax_inclusive`, `unit_amount` </details> (optional)
* @return GetBillingDocumentParams
*/
public GetBillingDocumentParams billingDocumentItemsFields(List billingDocumentItemsFields) {
this.billingDocumentItemsFields = billingDocumentItemsFields;
return this;
}
/**
* Set taxationItemsFields
* @param taxationItemsFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `amount`, `amount_exempt`, `tax_date`, `jurisdiction`, `location_code`, `name`, `sales_tax_payable_account`, `tax_code`, `tax_code_name`, `tax_rate`, `tax_rate_name`, `tax_inclusive`, `tax_rate_type` </details> (optional)
* @return GetBillingDocumentParams
*/
public GetBillingDocumentParams taxationItemsFields(List taxationItemsFields) {
this.taxationItemsFields = taxationItemsFields;
return this;
}
/**
* Set expand
* @param expand Allows you to expand responses by including related object information in a single call. See the [Expand responses](https://developer.zuora.com/quickstart-api/tutorial/expand-responses/) section of the Quickstart API Tutorials for detailed instructions. (optional)
* @return GetBillingDocumentParams
*/
public GetBillingDocumentParams expand(List expand) {
this.expand = expand;
return this;
}
/**
* Set filter
* @param filter A case-sensitive filter on the list. See the [Filter lists](https://developer.zuora.com/quickstart-api/tutorial/filter-lists/) section of the Quickstart API Tutorial for detailed instructions. Note that the filters on this operation are only applicable to the related objects. For example, when you are calling the \"Retrieve a billing document\" operation, you can use the `filter[]` parameter on the related objects such as `filter[]=items[account_id].EQ:8ad09e208858b5cf0188595208151c63` (optional)
* @return GetBillingDocumentParams
*/
public GetBillingDocumentParams filter(List filter) {
this.filter = filter;
return this;
}
/**
* Set pageSize
* @param pageSize The maximum number of results to return in a single page. If the specified `page_size` is less than 1 or greater than 99, Zuora will return a 400 error. (optional)
* @return GetBillingDocumentParams
*/
public GetBillingDocumentParams pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
}
/**
* Retrieve a billing document
* Retrieves the billing document with the given ID.
* @param billingDocumentId Identifier for the billingDocument, either `billing_document_number` or `billing_document_id` (required)
* @return BillingDocument
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public GetBillingDocumentParams getBillingDocumentParams(String billingDocumentId) {
return new GetBillingDocumentParams(billingDocumentId);
}
public BillingDocument getBillingDocument(String billingDocumentId) throws ApiException {
GetBillingDocumentParams params = new GetBillingDocumentParams(billingDocumentId);
return executeGetBillingDocumentAPICall(params).getData();
}
/**
* Expose HTTP info
public ApiResponse getBillingDocumentWithHttpInfo(String billingDocumentId) throws ApiException {
GetBillingDocumentParams params = new GetBillingDocumentParams(billingDocumentId);
return executeGetBillingDocumentAPICall(params);
}
**/
/**
Legacy Query Params Requests
**/
public BillingDocument getBillingDocument( String billingDocumentId , List expand) throws ApiException {
GetBillingDocumentParams params = new GetBillingDocumentParams(billingDocumentId);
params.expand(expand);
return executeGetBillingDocumentAPICall(params).getData();
}
/*
* add header methods
*/
public BillingDocument getBillingDocument(String billingDocumentId ,List expand,Headers headers) throws ApiException {
GetBillingDocumentParams params = new GetBillingDocumentParams(billingDocumentId)
.expand(expand)
.headers(headers);
return executeGetBillingDocumentAPICall(params).getData();
}
/**
* Retrieve a billing document
* Retrieves the billing document with the given ID.
* @param params GetBillingDocumentParams
* @return BillingDocument
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public BillingDocument get(GetBillingDocumentParams params) throws ApiException {
return executeGetBillingDocumentAPICall(params).getData();
}
public ApiResponse getWithHttpInfo(GetBillingDocumentParams params) throws ApiException {
return executeGetBillingDocumentAPICall(params);
}
ApiResponse executeGetBillingDocumentAPICall(GetBillingDocumentParams params) throws ApiException {
return getBillingDocumentWithHttpInfo(params.billingDocumentId, params.fields, params.billingDocumentFields, params.billingDocumentItemsFields, params.taxationItemsFields, params.expand, params.filter, params.pageSize, params.zuoraTrackId, params.async, params.zuoraCacheEnabled, params.zuoraEntityIds, params.idempotencyKey, params.acceptEncoding, params.contentEncoding, params.zuoraOrgIds);
}
private okhttp3.Call getBillingDocumentsCall(String cursor, List expand, List filter, List sort, Integer pageSize, List fields, List billingDocumentFields, List billingDocumentItemsFields, List taxationItemsFields, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/billing_documents";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
if (cursor != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("cursor", cursor));
}
if (expand != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "expand[]", expand));
}
if (filter != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "filter[]", filter));
}
if (sort != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "sort[]", sort));
}
if (pageSize != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("page_size", pageSize));
}
if (fields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "fields[]", fields));
}
if (billingDocumentFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "billing_document.fields[]", billingDocumentFields));
}
if (billingDocumentItemsFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "billing_document_items.fields[]", billingDocumentItemsFields));
}
if (taxationItemsFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "taxation_items.fields[]", taxationItemsFields));
}
if (zuoraTrackId != null) {
localVarHeaderParams.put("zuora-track-id", localVarApiClient.parameterToString(zuoraTrackId));
}
if (async != null) {
localVarHeaderParams.put("async", localVarApiClient.parameterToString(async));
}
if (zuoraCacheEnabled != null) {
localVarHeaderParams.put("zuora-cache-enabled", localVarApiClient.parameterToString(zuoraCacheEnabled));
}
if (zuoraEntityIds != null) {
localVarHeaderParams.put("zuora-entity-ids", localVarApiClient.parameterToString(zuoraEntityIds));
}
if (idempotencyKey != null) {
localVarHeaderParams.put("idempotency-key", localVarApiClient.parameterToString(idempotencyKey));
}
if (acceptEncoding != null) {
localVarHeaderParams.put("accept-encoding", localVarApiClient.parameterToString(acceptEncoding));
}
if (contentEncoding != null) {
localVarHeaderParams.put("content-encoding", localVarApiClient.parameterToString(contentEncoding));
}
if (zuoraOrgIds != null) {
localVarHeaderParams.put("zuora-org-ids", localVarApiClient.parameterToString(zuoraOrgIds));
}
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "bearerAuth" };
return localVarApiClient.buildCall(basePath, localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getBillingDocumentsValidateBeforeCall(String cursor, List expand, List filter, List sort, Integer pageSize, List fields, List billingDocumentFields, List billingDocumentItemsFields, List taxationItemsFields, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
return getBillingDocumentsCall(cursor, expand, filter, sort, pageSize, fields, billingDocumentFields, billingDocumentItemsFields, taxationItemsFields, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
}
private ApiResponse getBillingDocumentsWithHttpInfo(String cursor, List expand, List filter, List sort, Integer pageSize, List fields, List billingDocumentFields, List billingDocumentItemsFields, List taxationItemsFields, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds) throws ApiException {
okhttp3.Call localVarCall = getBillingDocumentsValidateBeforeCall(cursor, expand, filter, sort, pageSize, fields, billingDocumentFields, billingDocumentItemsFields, taxationItemsFields, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, null);
try {
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
} catch (ApiException e) {
e.setErrorObject(localVarApiClient.getJSON().getGson().fromJson(e.getResponseBody(), new TypeToken(){}.getType()));
throw e;
}
}
private okhttp3.Call getBillingDocumentsAsync(String cursor, List expand, List filter, List sort, Integer pageSize, List fields, List billingDocumentFields, List billingDocumentItemsFields, List taxationItemsFields, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = getBillingDocumentsValidateBeforeCall(cursor, expand, filter, sort, pageSize, fields, billingDocumentFields, billingDocumentItemsFields, taxationItemsFields, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
public static class GetBillingDocumentsParams {
private Headers headers;
/*
* Check for list params
*/
private ListQueryParams listQueryParams;
private String cursor;
private List expand;
private List filter;
private List sort;
private Integer pageSize;
private List fields;
private List billingDocumentFields;
private List billingDocumentItemsFields;
private List taxationItemsFields;
private String zuoraTrackId;
private Boolean async;
private Boolean zuoraCacheEnabled;
private String zuoraEntityIds;
private String idempotencyKey;
private String acceptEncoding;
private String contentEncoding;
private String zuoraOrgIds;
public GetBillingDocumentsParams() {
}
/**
* Set listQueryParams
* @param listQueryParams ListQueryParams
* @return GetBillingDocumentsParams
*/
public GetBillingDocumentsParams listQueryParams(ListQueryParams listQueryParams) {
this.listQueryParams = listQueryParams;
if (cursor != null) {
this.cursor = listQueryParams.getCursor();
}
if (expand != null) {
this.expand = listQueryParams.getExpand();
}
if (filter != null) {
this.filter = listQueryParams.getFilter();
}
if (sort != null) {
this.sort = listQueryParams.getSort();
}
if (pageSize != null) {
this.pageSize = listQueryParams.getPageSize();
}
return this;
}
/**
* Set headers
* @param headers Headers
* @return GetBillingDocumentsParams
*/
public GetBillingDocumentsParams headers(Headers headers) {
this.headers = headers;
if (headers.getZuoraTrackId() != null) {
this.zuoraTrackId = headers.getZuoraTrackId();
}
if (headers.getAsync() != null) {
this.async = headers.getAsync();
}
if (headers.getZuoraCacheEnabled() != null) {
this.zuoraCacheEnabled = headers.getZuoraCacheEnabled();
}
if (headers.getZuoraEntityIds() != null) {
this.zuoraEntityIds = headers.getZuoraEntityIds();
}
if (headers.getIdempotencyKey() != null) {
this.idempotencyKey = headers.getIdempotencyKey();
}
if (headers.getAcceptEncoding() != null) {
this.acceptEncoding = headers.getAcceptEncoding();
}
if (headers.getContentEncoding() != null) {
this.contentEncoding = headers.getContentEncoding();
}
if (headers.getZuoraOrgIds() != null) {
this.zuoraOrgIds = headers.getZuoraOrgIds();
}
return this;
}
/**
* Set cursor
* @param cursor A cursor for use in pagination. A cursor defines the starting place in a list. For instance, if you make a list request and receive 100 objects, ending with `next_page=W3sib3JkZXJ=`, your subsequent call can include `cursor=W3sib3JkZXJ=` in order to fetch the next page of the list. (optional)
* @return GetBillingDocumentsParams
*/
public GetBillingDocumentsParams cursor(String cursor) {
this.cursor = cursor;
return this;
}
/**
* Set expand
* @param expand Allows you to expand responses by including related object information in a single call. See the [Expand responses](https://developer.zuora.com/quickstart-api/tutorial/expand-responses/) section of the Quickstart API Tutorials for detailed instructions. (optional)
* @return GetBillingDocumentsParams
*/
public GetBillingDocumentsParams expand(List expand) {
this.expand = expand;
return this;
}
/**
* Set filter
* @param filter A case-sensitive filter on the list. See the [Filter lists](https://developer.zuora.com/quickstart-api/tutorial/filter-lists/) section of the Quickstart API Tutorials for detailed instructions. (optional)
* @return GetBillingDocumentsParams
*/
public GetBillingDocumentsParams filter(List filter) {
this.filter = filter;
return this;
}
/**
* Set sort
* @param sort A case-sensitive query parameter that specifies the sort order of the list, which can be either ascending (e.g. `account_number.asc`) or descending (e.g. `account_number.desc`). You cannot sort on properties that are arrays. If the array-type properties are specified for the `sort[]` parameter, they are ignored. (optional)
* @return GetBillingDocumentsParams
*/
public GetBillingDocumentsParams sort(List sort) {
this.sort = sort;
return this;
}
/**
* Set pageSize
* @param pageSize The maximum number of results to return in a single page. If the specified `page_size` is less than 1 or greater than 99, Zuora will return a 400 error. (optional)
* @return GetBillingDocumentsParams
*/
public GetBillingDocumentsParams pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Set fields
* @param fields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `account_id`, `balance`, `description`, `state`, `tax` </details> (optional)
* @return GetBillingDocumentsParams
*/
public GetBillingDocumentsParams fields(List fields) {
this.fields = fields;
return this;
}
/**
* Set billingDocumentFields
* @param billingDocumentFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `account_id`, `balance`, `description`, `state`, `tax` </details> (optional)
* @return GetBillingDocumentsParams
*/
public GetBillingDocumentsParams billingDocumentFields(List billingDocumentFields) {
this.billingDocumentFields = billingDocumentFields;
return this;
}
/**
* Set billingDocumentItemsFields
* @param billingDocumentItemsFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `price_id`, `discount_item`, `deferred_revenue_account`, `description`, `name`, `quantity`, `recognized_revenue_account`, `service_end`, `service_start`, `accounts_receivable_account`, `subscription_id`, `subscription_item_id`, `tax`, `tax_inclusive`, `unit_amount` </details> (optional)
* @return GetBillingDocumentsParams
*/
public GetBillingDocumentsParams billingDocumentItemsFields(List billingDocumentItemsFields) {
this.billingDocumentItemsFields = billingDocumentItemsFields;
return this;
}
/**
* Set taxationItemsFields
* @param taxationItemsFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `amount`, `amount_exempt`, `tax_date`, `jurisdiction`, `location_code`, `name`, `sales_tax_payable_account`, `tax_code`, `tax_code_name`, `tax_rate`, `tax_rate_name`, `tax_inclusive`, `tax_rate_type` </details> (optional)
* @return GetBillingDocumentsParams
*/
public GetBillingDocumentsParams taxationItemsFields(List taxationItemsFields) {
this.taxationItemsFields = taxationItemsFields;
return this;
}
}
/**
* List billing documents
* Returns a dictionary with a data property that contains an array of billing documents, starting after cursor. Each entry in the array is a separate billing document object. If no more billing documents are available, the resulting array will be empty. This request should never return an error.
* @return BillingDocumentListResponse
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public GetBillingDocumentsParams getBillingDocumentsParams() {
return new GetBillingDocumentsParams();
}
public BillingDocumentListResponse getBillingDocuments() throws ApiException {
GetBillingDocumentsParams params = new GetBillingDocumentsParams();
return executeGetBillingDocumentsAPICall(params).getData();
}
/**
* Expose HTTP info
public ApiResponse getBillingDocumentsWithHttpInfo() throws ApiException {
GetBillingDocumentsParams params = new GetBillingDocumentsParams();
return executeGetBillingDocumentsAPICall(params);
}
**/
/**
Legacy Query Params Requests
**/
public BillingDocumentListResponse getBillingDocuments(String cursor, List expand, List filter) throws ApiException {
GetBillingDocumentsParams params = new GetBillingDocumentsParams();
params.cursor(cursor);
params.expand(expand);
params.filter(filter);
return executeGetBillingDocumentsAPICall(params).getData();
}
/*
* add header methods
*/
public BillingDocumentListResponse getBillingDocuments(String cursor,List expand,List filter,Headers headers) throws ApiException {
GetBillingDocumentsParams params = new GetBillingDocumentsParams()
.cursor(cursor)
.expand(expand)
.filter(filter)
.headers(headers);
return executeGetBillingDocumentsAPICall(params).getData();
}
/**
* List billing documents
* Returns a dictionary with a data property that contains an array of billing documents, starting after cursor. Each entry in the array is a separate billing document object. If no more billing documents are available, the resulting array will be empty. This request should never return an error.
* @param params GetBillingDocumentsParams
* @return BillingDocumentListResponse
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public BillingDocumentListResponse list(GetBillingDocumentsParams params) throws ApiException {
return executeGetBillingDocumentsAPICall(params).getData();
}
public ApiResponse listWithHttpInfo(GetBillingDocumentsParams params) throws ApiException {
return executeGetBillingDocumentsAPICall(params);
}
ApiResponse executeGetBillingDocumentsAPICall(GetBillingDocumentsParams params) throws ApiException {
return getBillingDocumentsWithHttpInfo(params.cursor, params.expand, params.filter, params.sort, params.pageSize, params.fields, params.billingDocumentFields, params.billingDocumentItemsFields, params.taxationItemsFields, params.zuoraTrackId, params.async, params.zuoraCacheEnabled, params.zuoraEntityIds, params.idempotencyKey, params.acceptEncoding, params.contentEncoding, params.zuoraOrgIds);
}
private okhttp3.Call postBillingDocumentCall(BillingDocumentCreateRequest billingDocumentCreateRequest, List fields, List billingDocumentFields, List billingDocumentItemsFields, List taxationItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = billingDocumentCreateRequest;
// create path and map variables
String localVarPath = "/billing_documents";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
if (fields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "fields[]", fields));
}
if (billingDocumentFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "billing_document.fields[]", billingDocumentFields));
}
if (billingDocumentItemsFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "billing_document_items.fields[]", billingDocumentItemsFields));
}
if (taxationItemsFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "taxation_items.fields[]", taxationItemsFields));
}
if (expand != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "expand[]", expand));
}
if (filter != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "filter[]", filter));
}
if (pageSize != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("page_size", pageSize));
}
if (zuoraTrackId != null) {
localVarHeaderParams.put("zuora-track-id", localVarApiClient.parameterToString(zuoraTrackId));
}
if (async != null) {
localVarHeaderParams.put("async", localVarApiClient.parameterToString(async));
}
if (zuoraCacheEnabled != null) {
localVarHeaderParams.put("zuora-cache-enabled", localVarApiClient.parameterToString(zuoraCacheEnabled));
}
if (zuoraEntityIds != null) {
localVarHeaderParams.put("zuora-entity-ids", localVarApiClient.parameterToString(zuoraEntityIds));
}
if (idempotencyKey != null) {
localVarHeaderParams.put("idempotency-key", localVarApiClient.parameterToString(idempotencyKey));
}
if (acceptEncoding != null) {
localVarHeaderParams.put("accept-encoding", localVarApiClient.parameterToString(acceptEncoding));
}
if (contentEncoding != null) {
localVarHeaderParams.put("content-encoding", localVarApiClient.parameterToString(contentEncoding));
}
if (zuoraOrgIds != null) {
localVarHeaderParams.put("zuora-org-ids", localVarApiClient.parameterToString(zuoraOrgIds));
}
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "bearerAuth" };
return localVarApiClient.buildCall(basePath, localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call postBillingDocumentValidateBeforeCall(BillingDocumentCreateRequest billingDocumentCreateRequest, List fields, List billingDocumentFields, List billingDocumentItemsFields, List taxationItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'billingDocumentCreateRequest' is set
if (billingDocumentCreateRequest == null) {
throw new ApiException("Missing the required parameter 'billingDocumentCreateRequest' when calling postBillingDocument(Async)");
}
return postBillingDocumentCall(billingDocumentCreateRequest, fields, billingDocumentFields, billingDocumentItemsFields, taxationItemsFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
}
private ApiResponse postBillingDocumentWithHttpInfo(BillingDocumentCreateRequest billingDocumentCreateRequest, List fields, List billingDocumentFields, List billingDocumentItemsFields, List taxationItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds) throws ApiException {
okhttp3.Call localVarCall = postBillingDocumentValidateBeforeCall(billingDocumentCreateRequest, fields, billingDocumentFields, billingDocumentItemsFields, taxationItemsFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, null);
try {
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
} catch (ApiException e) {
e.setErrorObject(localVarApiClient.getJSON().getGson().fromJson(e.getResponseBody(), new TypeToken(){}.getType()));
throw e;
}
}
private okhttp3.Call postBillingDocumentAsync(BillingDocumentCreateRequest billingDocumentCreateRequest, List fields, List billingDocumentFields, List billingDocumentItemsFields, List taxationItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = postBillingDocumentValidateBeforeCall(billingDocumentCreateRequest, fields, billingDocumentFields, billingDocumentItemsFields, taxationItemsFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
public static class PostBillingDocumentParams {
private final BillingDocumentCreateRequest billingDocumentCreateRequest;
private Headers headers;
/*
* Check for list params
*/
private GetByIdQueryParams getByIdQueryParams;
private List fields;
private List billingDocumentFields;
private List billingDocumentItemsFields;
private List taxationItemsFields;
private List expand;
private List filter;
private Integer pageSize;
private String zuoraTrackId;
private Boolean async;
private Boolean zuoraCacheEnabled;
private String zuoraEntityIds;
private String idempotencyKey;
private String acceptEncoding;
private String contentEncoding;
private String zuoraOrgIds;
public PostBillingDocumentParams(BillingDocumentCreateRequest billingDocumentCreateRequest) {
this.billingDocumentCreateRequest = billingDocumentCreateRequest;
}
/**
* Set getByIdQueryParams
* @param getByIdQueryParams GetByIdQueryParams
* @return PostBillingDocumentParams
*/
public PostBillingDocumentParams getByIdQueryParams(GetByIdQueryParams getByIdQueryParams) {
this.getByIdQueryParams = getByIdQueryParams;
if (expand != null) {
this.expand = getByIdQueryParams.getExpand();
}
if (filter != null) {
this.filter = getByIdQueryParams.getFilter();
}
if (pageSize != null) {
this.pageSize = getByIdQueryParams.getPageSize();
}
return this;
}
/**
* Set headers
* @param headers Headers
* @return PostBillingDocumentParams
*/
public PostBillingDocumentParams headers(Headers headers) {
this.headers = headers;
if (headers.getZuoraTrackId() != null) {
this.zuoraTrackId = headers.getZuoraTrackId();
}
if (headers.getAsync() != null) {
this.async = headers.getAsync();
}
if (headers.getZuoraCacheEnabled() != null) {
this.zuoraCacheEnabled = headers.getZuoraCacheEnabled();
}
if (headers.getZuoraEntityIds() != null) {
this.zuoraEntityIds = headers.getZuoraEntityIds();
}
if (headers.getIdempotencyKey() != null) {
this.idempotencyKey = headers.getIdempotencyKey();
}
if (headers.getAcceptEncoding() != null) {
this.acceptEncoding = headers.getAcceptEncoding();
}
if (headers.getContentEncoding() != null) {
this.contentEncoding = headers.getContentEncoding();
}
if (headers.getZuoraOrgIds() != null) {
this.zuoraOrgIds = headers.getZuoraOrgIds();
}
return this;
}
/**
* Set fields
* @param fields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `account_id`, `balance`, `description`, `state`, `tax` </details> (optional)
* @return PostBillingDocumentParams
*/
public PostBillingDocumentParams fields(List fields) {
this.fields = fields;
return this;
}
/**
* Set billingDocumentFields
* @param billingDocumentFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `account_id`, `balance`, `description`, `state`, `tax` </details> (optional)
* @return PostBillingDocumentParams
*/
public PostBillingDocumentParams billingDocumentFields(List billingDocumentFields) {
this.billingDocumentFields = billingDocumentFields;
return this;
}
/**
* Set billingDocumentItemsFields
* @param billingDocumentItemsFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `price_id`, `discount_item`, `deferred_revenue_account`, `description`, `name`, `quantity`, `recognized_revenue_account`, `service_end`, `service_start`, `accounts_receivable_account`, `subscription_id`, `subscription_item_id`, `tax`, `tax_inclusive`, `unit_amount` </details> (optional)
* @return PostBillingDocumentParams
*/
public PostBillingDocumentParams billingDocumentItemsFields(List billingDocumentItemsFields) {
this.billingDocumentItemsFields = billingDocumentItemsFields;
return this;
}
/**
* Set taxationItemsFields
* @param taxationItemsFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `amount`, `amount_exempt`, `tax_date`, `jurisdiction`, `location_code`, `name`, `sales_tax_payable_account`, `tax_code`, `tax_code_name`, `tax_rate`, `tax_rate_name`, `tax_inclusive`, `tax_rate_type` </details> (optional)
* @return PostBillingDocumentParams
*/
public PostBillingDocumentParams taxationItemsFields(List taxationItemsFields) {
this.taxationItemsFields = taxationItemsFields;
return this;
}
/**
* Set expand
* @param expand Allows you to expand responses by including related object information in a single call. See the [Expand responses](https://developer.zuora.com/quickstart-api/tutorial/expand-responses/) section of the Quickstart API Tutorials for detailed instructions. (optional)
* @return PostBillingDocumentParams
*/
public PostBillingDocumentParams expand(List expand) {
this.expand = expand;
return this;
}
/**
* Set filter
* @param filter A case-sensitive filter on the list. See the [Filter lists](https://developer.zuora.com/quickstart-api/tutorial/filter-lists/) section of the Quickstart API Tutorial for detailed instructions. Note that the filters on this operation are only applicable to the related objects. For example, when you are calling the \"Retrieve a billing document\" operation, you can use the `filter[]` parameter on the related objects such as `filter[]=items[account_id].EQ:8ad09e208858b5cf0188595208151c63` (optional)
* @return PostBillingDocumentParams
*/
public PostBillingDocumentParams filter(List filter) {
this.filter = filter;
return this;
}
/**
* Set pageSize
* @param pageSize The maximum number of results to return in a single page. If the specified `page_size` is less than 1 or greater than 99, Zuora will return a 400 error. (optional)
* @return PostBillingDocumentParams
*/
public PostBillingDocumentParams pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
}
/**
* Create a billing document
* Creates different types of billing document objects, including invoices, credit memos, and debit memos.
* @param billingDocumentCreateRequest (required)
* @return BillingDocument
* @http.response.details
Status Code Description Response Headers
201 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public PostBillingDocumentParams postBillingDocumentParams(BillingDocumentCreateRequest billingDocumentCreateRequest) {
return new PostBillingDocumentParams(billingDocumentCreateRequest);
}
public BillingDocument postBillingDocument(BillingDocumentCreateRequest billingDocumentCreateRequest) throws ApiException {
PostBillingDocumentParams params = new PostBillingDocumentParams(billingDocumentCreateRequest);
return executePostBillingDocumentAPICall(params).getData();
}
/**
* Expose HTTP info
public ApiResponse postBillingDocumentWithHttpInfo(BillingDocumentCreateRequest billingDocumentCreateRequest) throws ApiException {
PostBillingDocumentParams params = new PostBillingDocumentParams(billingDocumentCreateRequest);
return executePostBillingDocumentAPICall(params);
}
**/
/**
Legacy Query Params Requests
**/
public BillingDocument postBillingDocument( BillingDocumentCreateRequest billingDocumentCreateRequest , List expand) throws ApiException {
PostBillingDocumentParams params = new PostBillingDocumentParams(billingDocumentCreateRequest);
params.expand(expand);
return executePostBillingDocumentAPICall(params).getData();
}
/*
* add header methods
*/
public BillingDocument postBillingDocument(BillingDocumentCreateRequest billingDocumentCreateRequest ,List expand,Headers headers) throws ApiException {
PostBillingDocumentParams params = new PostBillingDocumentParams(billingDocumentCreateRequest)
.expand(expand)
.headers(headers);
return executePostBillingDocumentAPICall(params).getData();
}
/**
* Create a billing document
* Creates different types of billing document objects, including invoices, credit memos, and debit memos.
* @param params PostBillingDocumentParams
* @return BillingDocument
* @http.response.details
Status Code Description Response Headers
201 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public BillingDocument create(PostBillingDocumentParams params) throws ApiException {
return executePostBillingDocumentAPICall(params).getData();
}
public ApiResponse createWithHttpInfo(PostBillingDocumentParams params) throws ApiException {
return executePostBillingDocumentAPICall(params);
}
ApiResponse executePostBillingDocumentAPICall(PostBillingDocumentParams params) throws ApiException {
return postBillingDocumentWithHttpInfo(params.billingDocumentCreateRequest, params.fields, params.billingDocumentFields, params.billingDocumentItemsFields, params.taxationItemsFields, params.expand, params.filter, params.pageSize, params.zuoraTrackId, params.async, params.zuoraCacheEnabled, params.zuoraEntityIds, params.idempotencyKey, params.acceptEncoding, params.contentEncoding, params.zuoraOrgIds);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy